package com.zx.dao.impl;
import com.zx.bean.Express;
import com.zx.dao.ExpressDao;
import com.zx.exception.DuplicateCodeException;
import com.zx.util.DruidUtil;
import java.sql.*;
import java.util.*;
/**
* @Author CaesarChang张旭
* @Date 2020/10/21 8:32 上午
* @Version 1.0
*/
public class ExpressDaoImpl implements ExpressDao {
/**
* 先写SQL语句
* @return
*/
private static final String SQL_CONSOLE="select count(id) data1_size,count(to_days(intime)=to_days(now()) and status=1 or null) data1_day," +
"count(status=0 or null) data2_size,count(to_days(intime)=to_days(now()) and status=0 or null) data2_day " +
"from express;";
private static final String SQL_FIND_ALL="select * from express"; //查询全部
private static final String SQL_FIND_LIMIT="select * from express limit ?,?"; //分页查询全部
private static final String SQL_FIND_BY_CODE="select * from express where code=?";
private static final String SQL_FIND_BY_NUMBER="select * from express where number=?";
private static final String SQL_FIND_BY_SYSPHONE="select * from express where sysphone=?";
private static final String SQL_USERPHONE="select * from express where userphone=?";
private static final String SQL_INSERT="insert into express (number,username,userphone,company,code,intime,status,sysphone) values(?,?,?,?,?,now(),0,?)";
private static final String SQL_UPDATE="update express set number=?,username=?,company=?,status=? where id=?";
private static final String SQL_DELETE="delete from express where id=?";
private static final String SQL_UPDATE_STATUS="update express set status=1,outtime=now(),code=-1 where code=?";
@Override
public List<Map<String, Integer>> console() {
//1 获取数据库连接
Connection connection = DruidUtil.getConnection();
PreparedStatement preparedStatement =null;
ResultSet resultSet =null;
ArrayList<Map<String ,Integer>> data=new ArrayList<>();
try {
//2预编译SQL
preparedStatement=connection.prepareStatement(SQL_CONSOLE);
//3 填充参数
//4 执行SQL
resultSet=preparedStatement.executeQuery();
//5 获取执行结果
if(resultSet.next()){
int data1_size = resultSet.getInt("data1_size");
int data1_day = resultSet.getInt("data1_day");
int data2_size = resultSet.getInt("data2_size");
int data2_day = resultSet.getInt("data2_day");
Map data1=new HashMap();
data1.put("data1_size",data1_size);
data1.put("data1_day",data1_day);
Map data2=new HashMap();
data2.put("data2_size",data2_size);
data2.put("data2_day",data2_day);
data.add(data1);
data.add(data2);
}
//6 释放资源
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
DruidUtil.close(connection,preparedStatement, resultSet);
}
return data;
}
@Override
public List<Express> findAll(boolean limit, int offset, int pageNumber) {
Connection connection = DruidUtil.getConnection();
PreparedStatement preparedStatement =null;
ResultSet resultSet =null;
List<Express> data=new ArrayList<>();
try {
//2预编译SQL
if(limit){
preparedStatement = connection.prepareStatement(SQL_FIND_LIMIT);
//3 填充参数
preparedStatement.setInt(1,offset);
preparedStatement.setInt(2,pageNumber);
}else{
preparedStatement=connection.prepareStatement(SQL_FIND_ALL);
}
//4 执行SQL
resultSet=preparedStatement.executeQuery();
//5 获取执行结果
while(resultSet.next()){
int id=resultSet.getInt("id");
String number=resultSet.getString("number");
String username=resultSet.getString("username");
String userphone=resultSet.getString("userphone");
String company=resultSet.getString("company");
String code=resultSet.getString("code");
Timestamp intime=resultSet.getTimestamp("intime");
Timestamp outtime=resultSet.getTimestamp("outtime");
int status=resultSet.getInt("status");
String sysPhone=resultSet.getString("sysPhone");
Express e=new Express(id,number,username,userphone,company,code,intime,outtime,status,sysPhone);
data.add(e);
}
//6 释放资源
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
DruidUtil.close(connection,preparedStatement, resultSet);
}
return data;
}
@Override
public Express findByNumber(String number) {
Connection connection = DruidUtil.getConnection();
PreparedStatement preparedStatement =null;
ResultSet resultSet =null;
try {
//2预编译SQL
preparedStatement=connection.prepareStatement(SQL_FIND_BY_NUMBER);
//3 填充参数
preparedStatement.setString(1,number);
//4 执行SQL
resultSet=preparedStatement.executeQuery();
//5 获取执行结果
if(resultSet.next()){
int id=resultSet.getInt("id");
String username=resultSet.getString("username");
String userphone=resultSet.getString("userphone");
String company=resultSet.getString("company");
String code=resultSet.getString("code");
Timestamp intime=resultSet.getTimestamp("intime");
Timestamp outtime=resultSet.getTimestamp("outtime");
int status=resultSet.getInt("status");
String sysPhone=resultSet.getString("sysPhone");
Express e=new Express(id,number,username,userphone,company,code,intime,outtime,status,sysPhone);
return e;
}
//6 释放资源
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
DruidUtil.close(connection,preparedStatement, resultSet);
}
return null;
}
@Override
public Express findByCode(String code) {
Connection connection = DruidUtil.getConnection();
PreparedStatement preparedStatement =null;
ResultSet resultSet =null;
try {
//2预编译SQL
preparedStatement=connection.prepareStatement(SQL_FIND_BY_CODE);
//3 填充参数
preparedStatement.setString(1,code);
//4 执行SQL
resultSet=preparedStatement.executeQuery();
//5 获取执行结果
if(resultSet.next()){
int id=resultSet.getInt("id");
String userphone=resultSet.getString("userphone");
String number=resultSet.getString("number");
String username=resultSet.getString("username");
String company=resultSet.getString("company");
Timestamp intime=resultSet.getTimestamp("intime");
Timestamp outtime=resultSet.getTimestamp("outtime");
int status=resultSet.getInt("status");
String sysPhone=resultSet.getString("sysPhone");
Express e=new Express(id,number,username,userphone,company,code,intime,outtime,status,sysPhone);
return e;
}
//6 释放资源
} catch (SQLException throwables) {
throwabl
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目为基于数据挖掘与深度学习的中小微低碳企业绿色信贷云评级系统设计源码,包含544个文件,包括158个GIF图片、67个CSS样式表、67个JavaScript脚本、55个Java类文件、46个JAR包文件、34个CSS样式文件、28个HTML文件、22个PNG图片文件、11个XML配置文件以及少量其他文件。该系统旨在为中小微低碳企业提供绿色信贷评级服务,支持系统开发与部署。
资源推荐
资源详情
资源评论
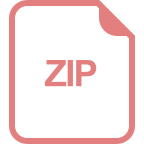
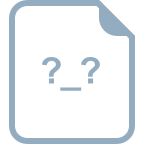
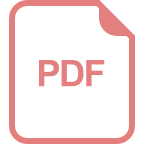
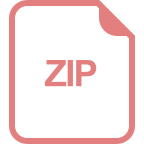
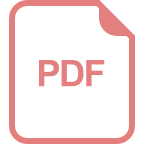
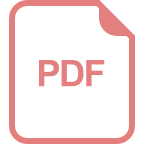
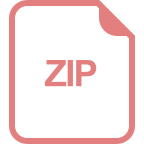
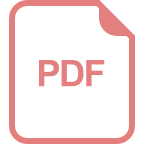
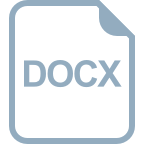
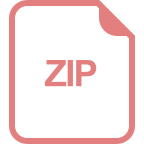
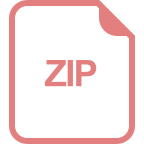
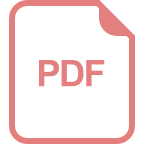
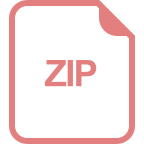
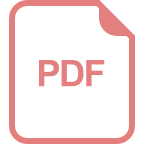
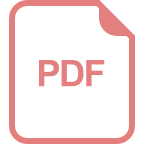
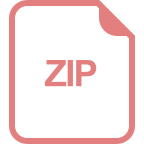
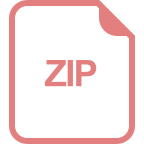
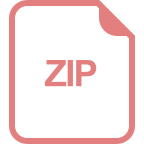
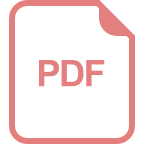
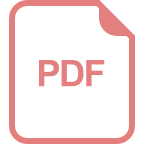
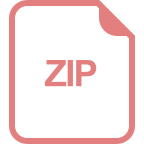
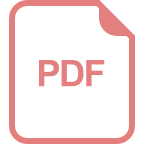
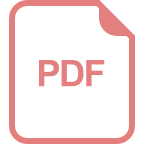
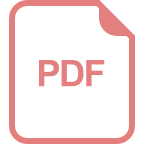
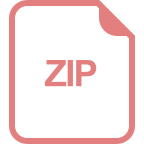
收起资源包目录

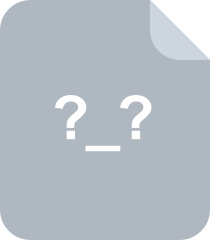
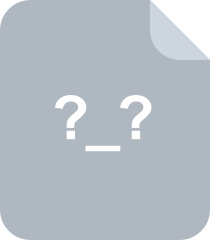
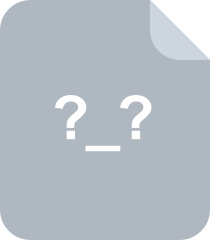
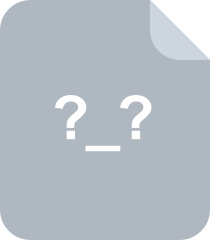
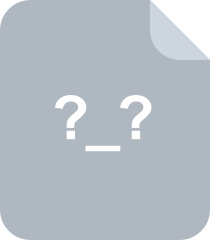
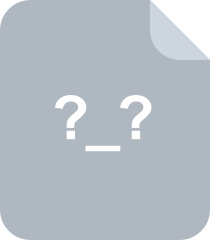
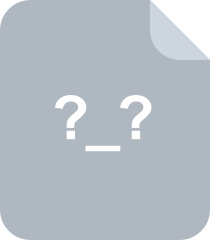
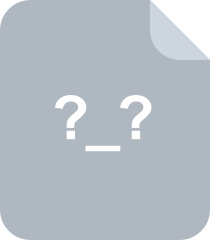
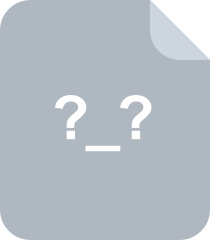
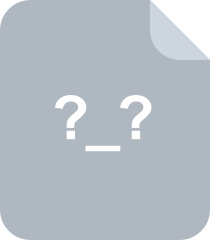
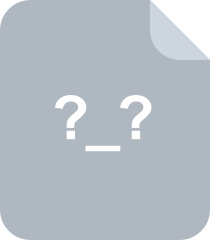
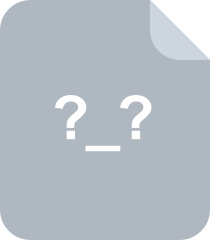
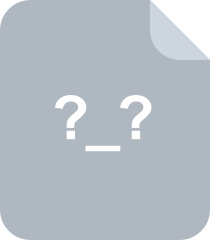
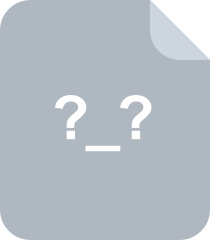
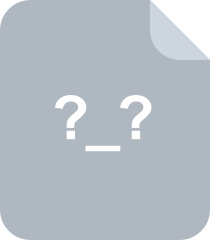
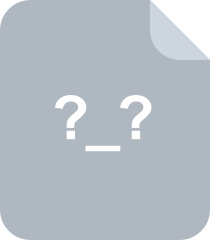
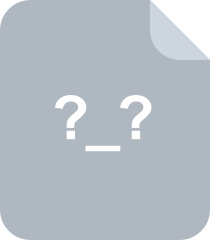
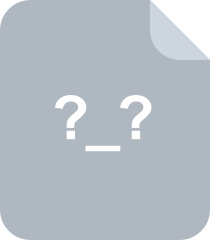
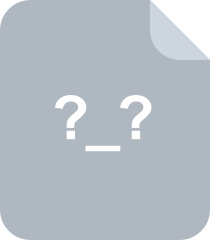
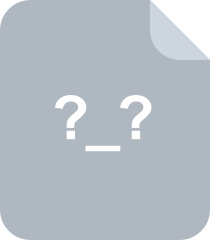
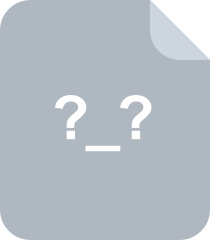
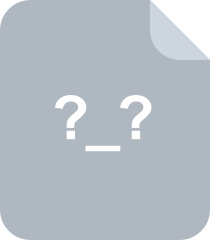
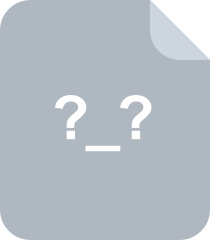
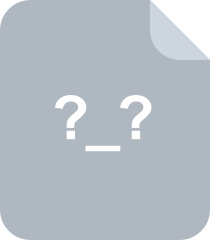
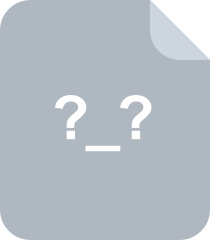
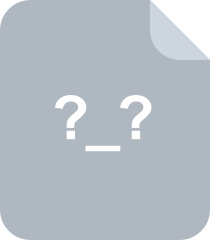
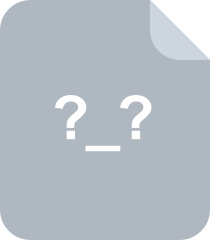
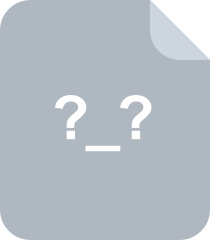
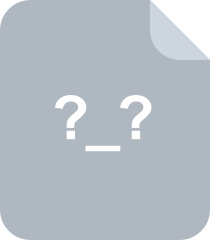
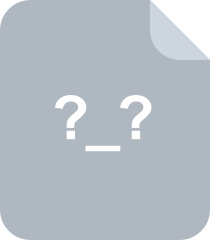
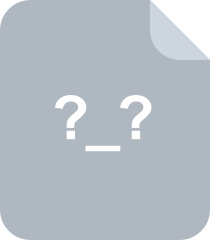
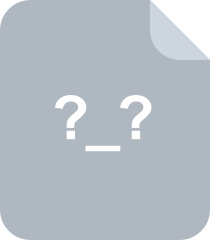
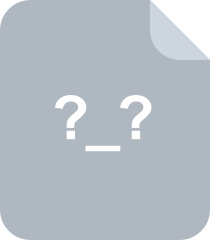
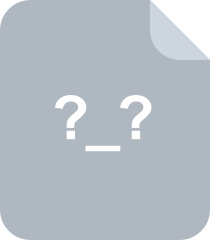
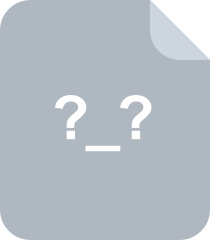
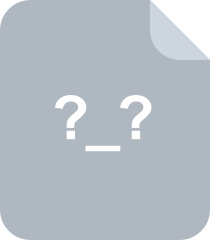
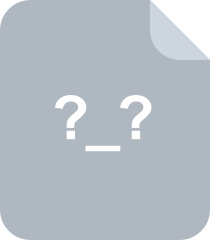
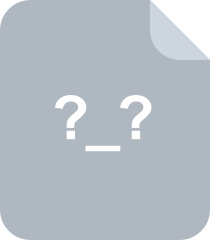
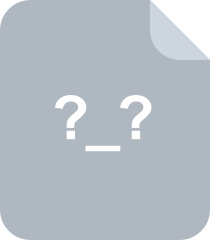
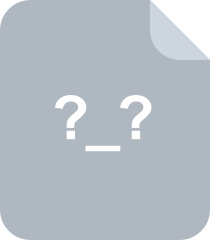
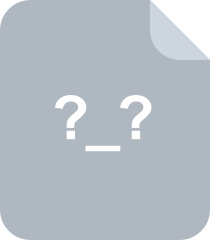
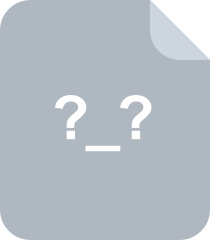
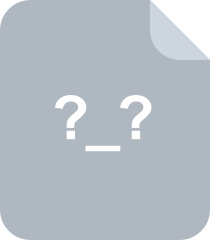
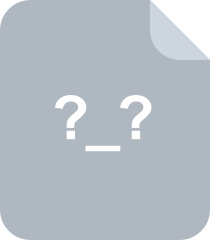
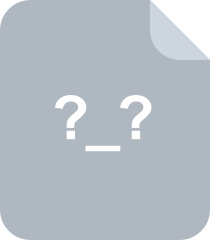
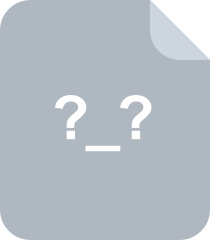
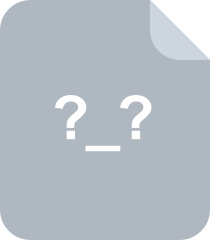
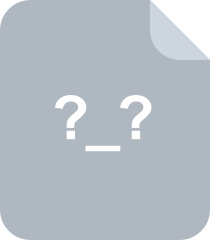
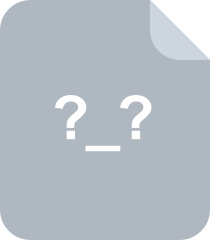
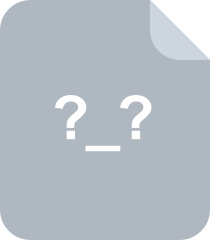
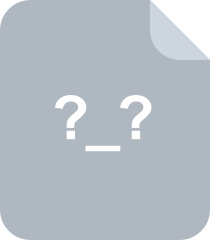
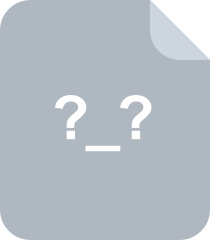
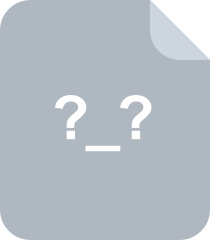
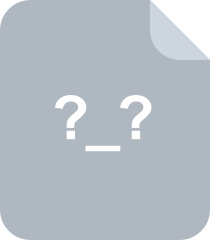
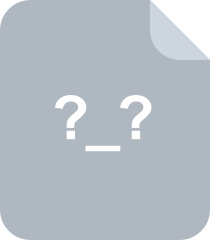
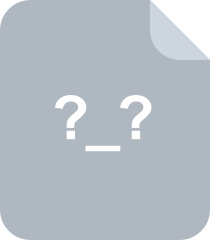
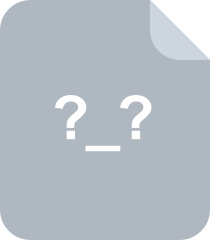
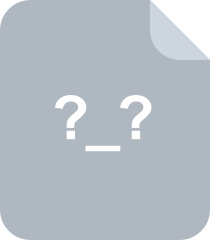
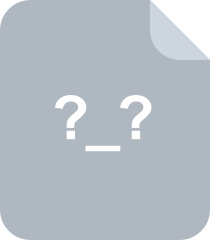
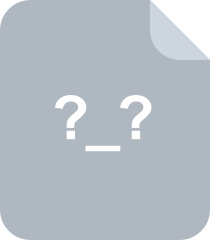
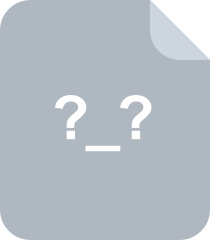
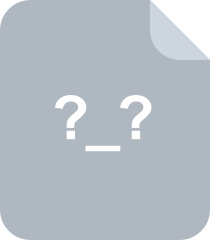
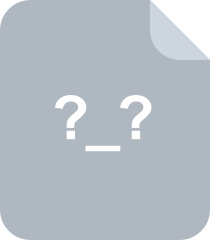
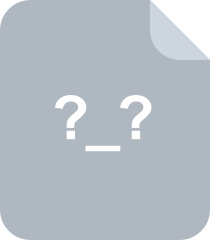
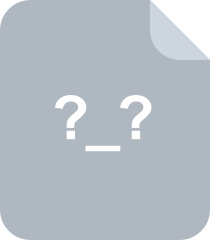
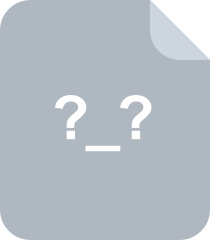
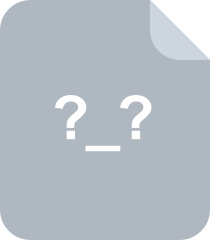
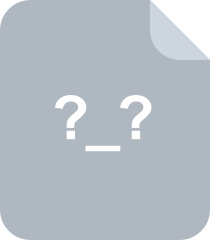
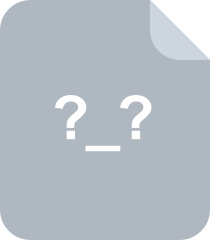
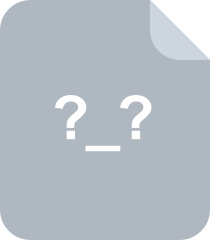
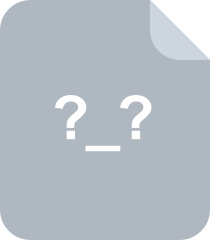
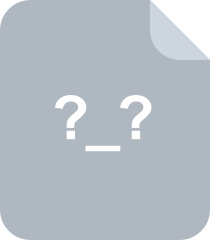
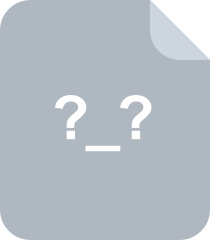
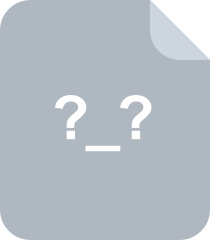
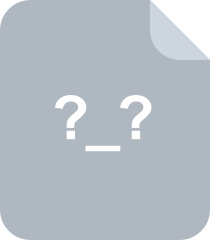
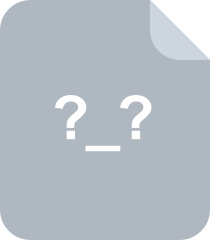
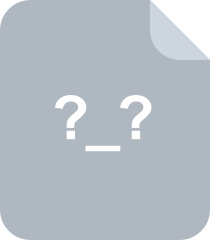
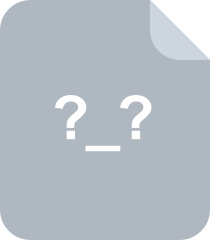
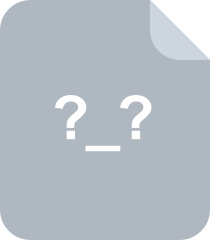
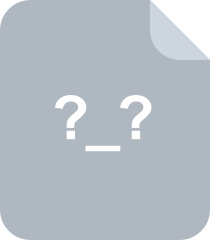
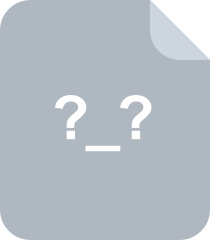
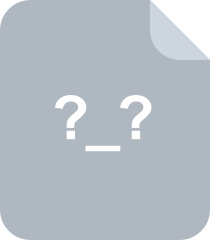
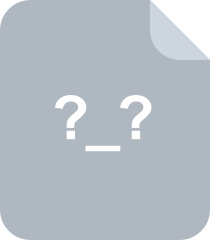
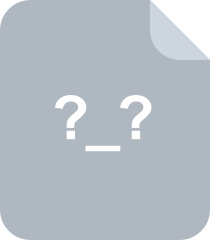
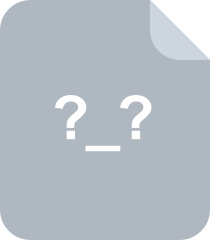
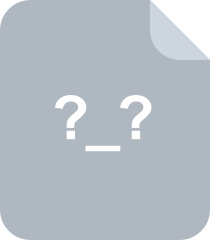
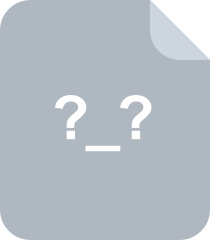
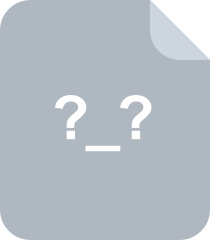
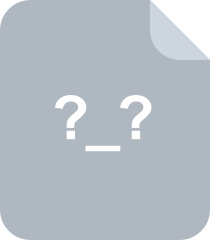
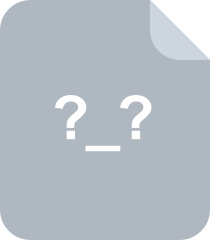
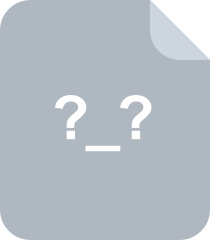
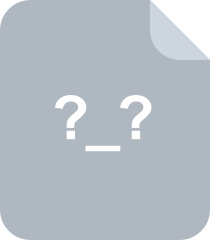
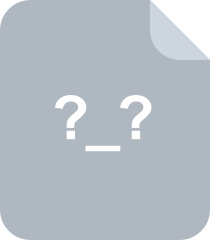
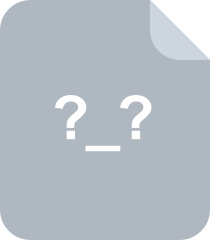
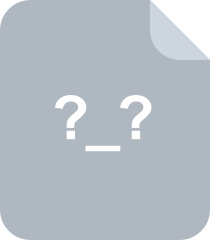
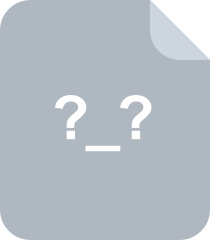
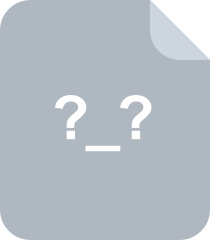
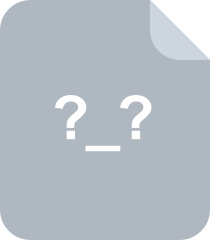
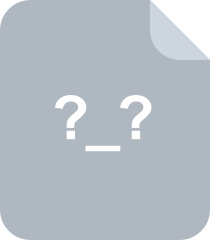
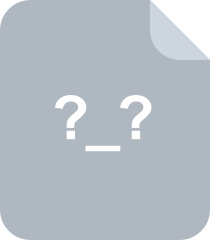
共 546 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
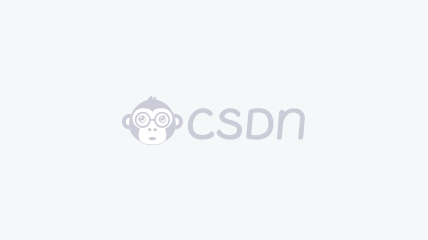

wjs2024
- 粉丝: 2322
- 资源: 5457
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

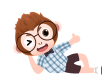
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


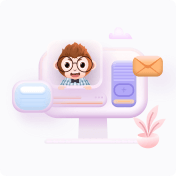
安全验证
文档复制为VIP权益,开通VIP直接复制
