## TensorRT for DETR
#### 0. 环境配置
+ TensorRT Docker镜像环境:`nvcr.io/nvidia/tensorrt:21.03-py3`(TensorRT-7.2.2.3),需要Host中安装好Docker和Nvidia-Docker2和版本为`Driver Version: 460.32.03`的显卡驱动.
+ 在Docker镜像内需要安装相应的Python库,可以在项目下执行`pip3 install -r requirements.txt -i http://mirrors.aliyun.com/pypi/simple/ --trusted-host mirrors.aliyun.com`进行安装.
+ 显卡类型:Tesla T4 (16G显存).
+ CUDA 11.2, cuDNN-8.1.
+ 系统信息为:`Linux version 4.15.0-139-generic (buildd@lgw01-amd64-035) (gcc version 7.5.0 (Ubuntu 7.5.0-3ubuntu1~18.04)) #143-Ubuntu SMP Tue Mar 16 01:30:17 UTC 2021`.
**项目结构及说明**
```shell
.
├── model # DETR模型相关的python代码(主要来源https://github.com/facebookresearch/detr)
│ ├── README.md
│ ├── __init__.py
│ ├── backbone.py # backbone resnet50
│ ├── box_ops.py
│ ├── detr.py # DETR model build
│ ├── hubconf.py
│ ├── matcher.py
│ ├── misc.py
│ ├── position_encoding.py # position_encoding,支持sine和自学习,默认是sine
│ ├── segmentation.py # 分割的模型的build
│ └── transformer.py # transformer的encoder和decoder包括多头的自注意力,Skip, FFN
|
├── trt_util # TensorRT相关的辅助方法
│ ├── __init__.py
│ ├── calibrator.py # INT8量化的calibrator
│ ├── common.py #host与device数据交互,TensorRT序列化engine及调用(支持FP32,FP16,INT8),Dynamic shape序列化engine及调用(支持FP32,FP16,INT8)
│ ├── plot_box.py # 画出detr推断预测的box
│ ├── process_img.py # detr图像预处理,支持numpy,torchvision, cupy
│ └── trt_lite.py # tensorrt性能测试辅助方法,基于https://github.com/NVIDIA/trt-samples-for-hackathon-cn/blob/master/python/修改
|
├── calib_train_image # INT8量化的数据约30660张, 开源代码该部分内容被删除
│ ├── A_57b26b46_2e1e_11eb_9d64_00d861c69d42.jpg
│ ├── ... ...
│ └── N9_50667548_2e21_11eb_ac9b_00d861c69d42.jpg
|
├── test # 性能测试需要的测试图像约1000张,开源代码该部分内容被删除
│ ├── test_c6d6ecec_2fd1_11eb_b773_00d861c69d42.jpg
│ ├── ... ...
│ └── test_d4c4ea34_2fd1_11eb_9f0e_00d861c69d42.jpg
|
├── checkpoint # DETR Pytorch 模型,开源代码该部分仅提供模型下载链接
│ ├── detr_resnet50.pth
│ └── log.txt
├── pic # README 静态资源文件
|
├── detr_pth2onnx.py # pytorch 转onnx支持static,dynamic shape, btached, onnx check, onnx-simplifier, onnx-graphsurgeon
├── generate_batch_plan.py # 生成batched static tensorrt 序列化engine文件,支持FP32,FP16,任意batch size
├── inference_detr_onnx.py # onnx runtime模型推断,支持static,dynamic shape,用于验证onnx的正确性
├── inference_detr_trt.py # tensorrt模型推断,支持,static,dynamic shape,FP32,FP16,INT8并检验engine是否存在,不存在调用序列化程序
├── performance_accuracy_detr.py # TensorRT识别精度的计算和可视化
├── performance_time_detr.py # TensorRT benchmark的计算和可视化
├── trt_int8_quant.py # INT8量化,并生成量化模型的engine和cache文件
|
├── requirements.txt # Python package list
├── LICENSE
└── README.md
# 说明:
# 1. README提供过程中用到的Linux 相关命令,比如 trtexec, polygraphy, Nsight Systems的使用
# 2. 用到的模型文件包括.pth,.onnx,.plan文件在README中提供百度云盘的下载地址
# 3. 项目过程中产生的log文件比如,测试benchmark生成的数据,序列化engine过程中的日志,polygraphy日志,Nsight Systems生成UI文件均在README中提供百度云盘下载地址
```
### 1.Pytorch checkpoint to ONNX
```shell
# pytorch to onnx
$ python3 detr_pth2onnx.py -h
# batch_size=1, static
# 在项目下生成detr.onnx和detr_sim.onnx(simplify后的onnx)
$ python3 detr_pth2onnx.py --model_dir ./checkpoint/detr_resnet50.pth --check --onnx_dir ./detr.onnx
# dynamic shape
# 在项目下生成detr_dynamic.onnx和detr_dynamic_sim.onnx
$ python3 detr_pth2onnx.py --model_dir ./checkpoint/detr_resnet50.pth --check --onnx_dir ./detr_dynamic.onnx --dynamic_axes
# batch_size=n, static
# 生成./output/detr_batch_{n}.onnx和output/detr_batch_{n}_sim.onnx
$ python3 detr_pth2onnx.py --model_dir ./checkpoint/detr_resnet50.pth --check --onnx_dir ./output/detr_batch_2.onnx --batch_size=2
```
**simplify的其他方式**
```shell
# onnx-simplifier
# static
$ python3 -m onnxsim detr.onnx detr_sim.onnx
# dynamic
$ python3 -m onnxsim detr_dynamic.onnx detr_dynamic_sim.onnx --input-shape "inputs:1,3,800,800" --dynamic-input-shape
```
onnxruntime测试onnx模型
```shell
$ python3 inference_detr_onnx.py
```
**注意**:上述过程生成的detr_sim.onnx文件序列化engine后,TensorRT推断结果全部为0!
```python
# 需要onnx-graphsurgeon做如下修改 (该代码导师提供)
import onnx
import onnx_graphsurgeon as gs
graph = gs.import_onnx(onnx.load("./detr_sim.onnx"))
for node in graph.nodes:
if node.name == "Gather_2682":
print(node.inputs[1])
node.inputs[1].values = np.int64(5)
print(node.inputs[1])
elif node.name == "Gather_2684":
print(node.inputs[1])
node.inputs[1].values = np.int64(5)
print(node.inputs[1])
onnx.save(gs.export_onnx(graph),'changed.onnx')
```
### 2.TensorRT Inference in FP32 or FP16 Mode
生成TensorRT序列化engine文件并调用,有两种方式:
+ 1.使用python实现
```shell
# 提供build engine和反序列化engine进行推断
# inference_detr_trt.py支持FP32,FP16的build engine和engine的推断,同时支持static shape和Dynamic shape的推断,前处理,后处理和结果可视化,支持INT8量化后engine的推断,包括static shape, dynamic shape及前处理后处理和结果可视化
# static shape
# FP32
$ python3 inference_detr_trt.py -h
$ python3 inference_detr_trt.py --model_dir ./detr_sim.onnx --engine_dir ./detr.plan --image_dir ./test
# FP16
$ python3 inference_detr_trt.py --model_dir ./detr_sim.onnx --engine_dir ./detr_fp16.plan --image_dir ./test --fp16
# INT8
$ python3 inference_detr_trt.py --model_dir ./detr_sim.onnx --engine_dir ./detr_int8.plan --image_dir ./test --int8
# dynamic shape
$ python3 inference_detr_trt.py --model_dir ./detr_sim.onnx --engine_dir ./detr.plan --image_dir ./test --dynamic --batch_size=8
# 生成batch的engine
$ python3 generate_batch_plan.py --model_dir ./output/detr_batch_{n}_sim.onnx --engine_dir ./output/detr_batch_{n}_fp16.plan --batch_size={n} --fp16
# eg
$ python3 generate_batch_plan.py --model_dir ./output/detr_batch_2_sim.onnx --engine_dir ./output/detr_batch_2.plan --batch_size=2
$ python3 generate_batch_plan.py --model_dir ./output/detr_batch_2_sim.onnx --engine_dir ./output/detr_batch_2_fp16.plan --batch_size=2 --fp16
```
TensorRT Inference的结果Demo(上trt fp32,下trt fp16):
<center class="half"> <img src="./pic/test_fp32.jpg"/><img src="./pic/test_fp16.jpg" /> </center>
+ 2.使用trtexec
```shell
# static shape
trtexec --verbose --onnx=detr.onnx --saveEngine=detr.plan # error
trtexec --verbose --onnx=detr_sim.onnx --saveEngine=detr.plan
trtexec --verbose --onnx=detr_sim.onnx --saveEngine=detr.plan --fp16
# dynamic shape (error)
# FP32
trtexec --verbose --onnx=detr_dynamic_sim.onnx --saveEngine=detr_dynamic.plan --optShapes=input:1x3x800x800 --minShapes=input:1x3x800x800 --maxShapes=input:16x3x800x800 --workspace=10240
# FP16
trtexec --verbose --onnx=detr_dynamic_sim.onnx --saveEngine=detr_dynamic_fp16.plan --optShapes=input:1x3x800x800 --minShapes=input:1x3x800x800 --maxSha
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
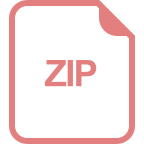
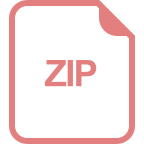
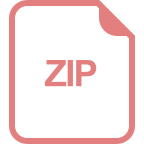
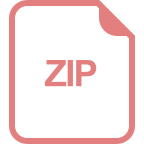
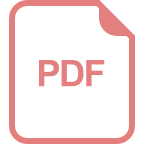
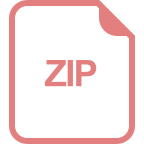
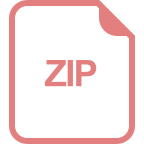
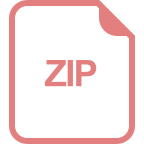
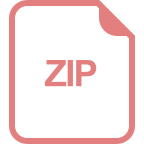
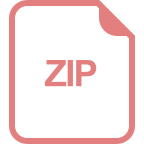
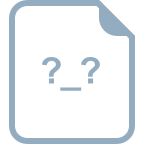
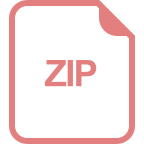
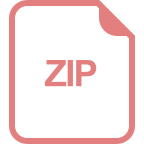
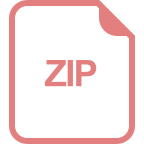
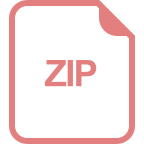
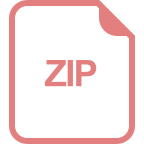
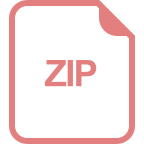
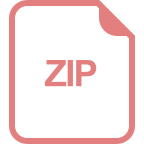
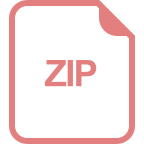
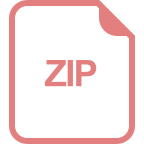
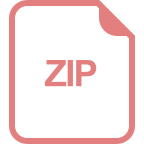
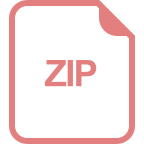
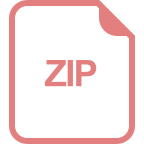
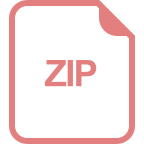
收起资源包目录



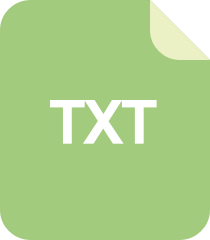
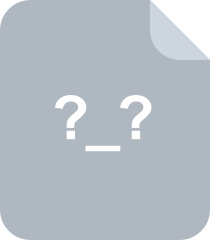

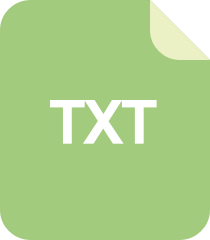

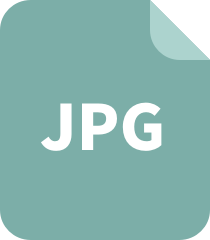
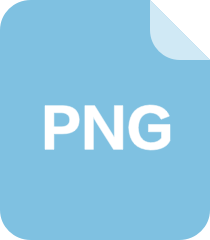
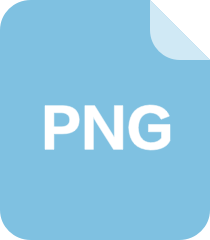
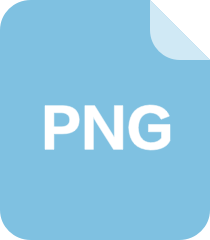
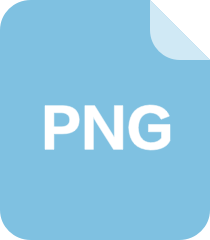
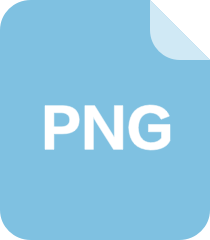
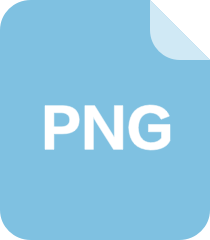
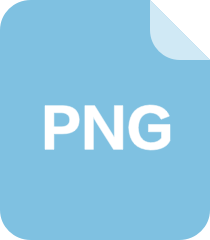
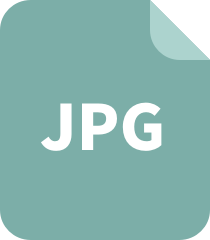
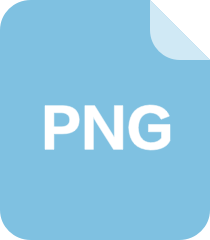
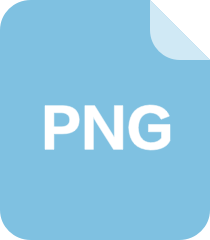
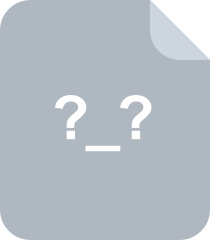

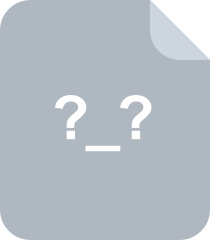
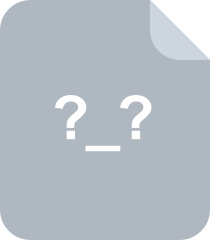
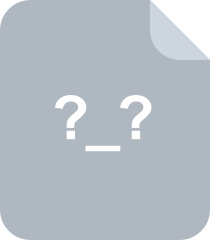
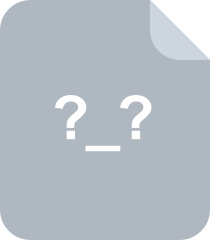
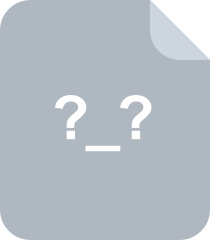
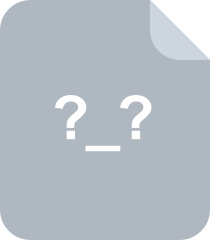
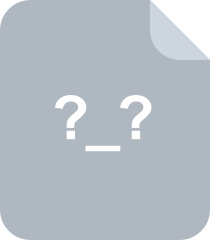

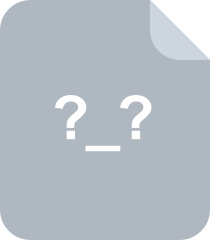
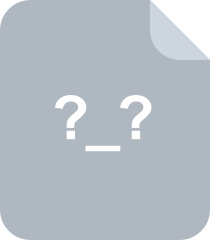
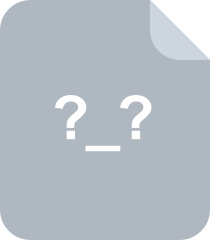
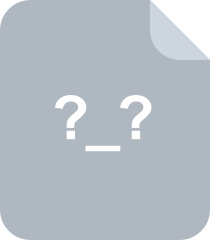
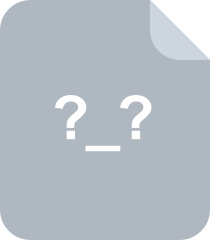
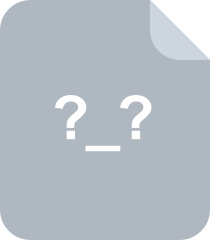
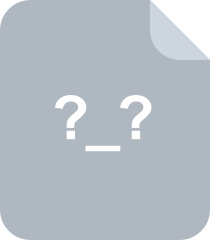
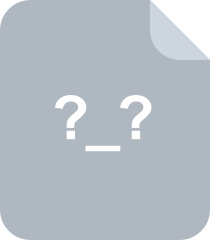
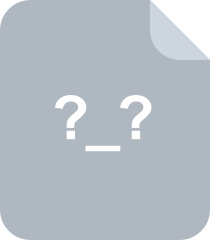
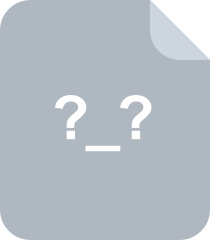
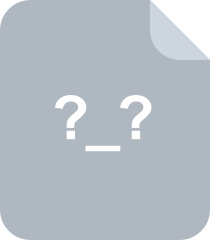
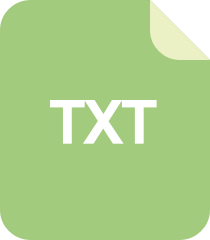
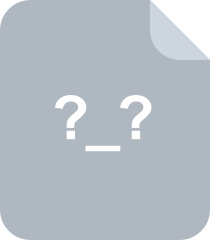

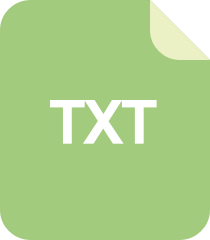

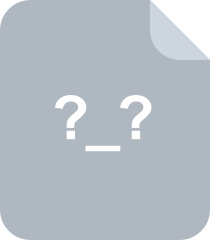
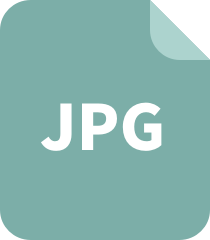

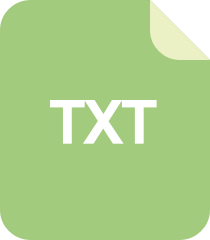
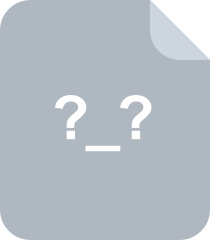
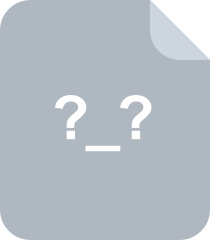
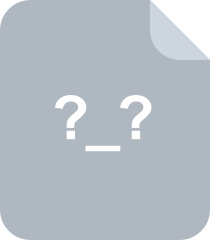
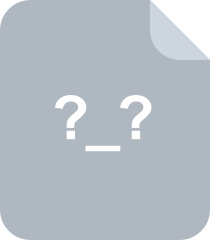
共 43 条
- 1
资源评论
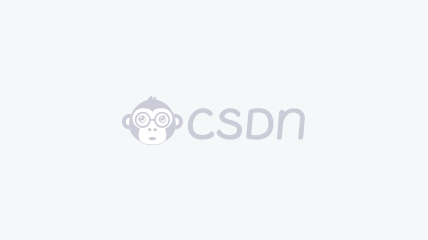

__AtYou__
- 粉丝: 3383
- 资源: 2110
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

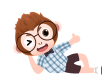
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


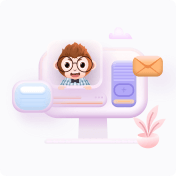
安全验证
文档复制为VIP权益,开通VIP直接复制
