package com.lcx.servlet;
import com.lcx.domain.User;
import com.lcx.utils.AESUtils;
import com.lcx.utils.DMLUtils;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.List;
/**
* @author lcx
* @version 1.0
*/
@WebServlet("/dispatcher.do")
public class Dispatcher extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//查看当前是增删改查哪一个操作
String operate = req.getParameter("operate");
if (operate == null) {
resp.setContentType("text/html;charset=utf-8");
resp.getWriter().write("<script>alert('非法访问!');window.location.href='login.html'</script>");
} else {
//如果是增加操作
switch (operate) {
case "updateRole":
updateRole(req, resp);
break;
case "deleteRole":
deleteRole(req, resp);
break;
case "addRole":
addRole(req, resp);
break;
case "checkRole":
checkRoleInfo(req, resp);
break;
case "addUser":
addUser(req, resp);
break;
case "checkUserInfo":
checkUserInfo(req, resp);
break;
case "checkUserList":
checkUserList(req, resp);
break;
case "updateUserInfo":
updateUserInfo(req, resp);
break;
case "deleteUser":
deleteUser(req, resp);
break;
case "logout":
logout(req, resp);
break;
case "checkUserRole":
checkUserRole(req, resp);
break;
case "checkKey":
checkKey(req, resp);
break;
case "checkRoleList":
checkRoleList(req, resp);
break;
default:
break;
}
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws IOException, ServletException {
String action = req.getParameter("action");
switch (action) {
case "modifyRole":
modifyRole(req, resp);
break;
case "addRole":
insertRole(req, resp);
break;
case "modifyUser":
modifyUser(req, resp);
break;
case "addUser":
insertUser(req, resp);
break;
case "login":
login(req, resp);
break;
case "updateUserRole":
updateUserRole(req, resp);
break;
case "updateKey":
updateKey(req, resp);
break;
default:
break;
}
}
private void modifyRole(HttpServletRequest req, HttpServletResponse resp) throws IOException {
int roId = Integer.parseInt(req.getParameter("roId"));
String roName = req.getParameter("roName");
int roLevel = Integer.parseInt(req.getParameter("roLevel"));
List<String> opIdList = getRoleOperate(req);
if (DMLUtils.updateRole(roId, roName, roLevel, opIdList) == 1) {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('成功修改角色" + roName + "!');window.location.href='dispatcher.do?operate=checkRoleList'</script>");
} else {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('修改失败,请重试!');window.location.href='dispatcher.do?operate=updateRole&roId=" + roId + "'</script>");
}
}
private List<String> getRoleOperate(HttpServletRequest req) {
int opNum = Integer.parseInt(req.getParameter("opNum"));
List<String> opIdList = new ArrayList<>();
opIdList.add("1");
opIdList.add("2");
opIdList.add("3");
for (int i = 1; i <= opNum; i++) {
if (req.getParameter("op" + i) != null) {
opIdList.add(req.getParameter("op" + i));
}
}
return opIdList;
}
private void updateRole(HttpServletRequest req, HttpServletResponse resp) throws IOException, ServletException {
int roId = Integer.parseInt(req.getParameter("roId"));
String account = (String) req.getSession().getAttribute("account");
if (!DMLUtils.checkUserOp(account, 13)) {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('您没有权限执行该操作!');window.location.href='index.jsp'</script>");
} else {
req.getRequestDispatcher("/WEB-INF/pages/updateRole.jsp?roId=" + roId).forward(req, resp);
}
}
private void deleteRole(HttpServletRequest req, HttpServletResponse resp) throws IOException {
int roId = Integer.parseInt(req.getParameter("roId"));
String roName = DMLUtils.getRoleName(roId);
String account = (String) req.getSession().getAttribute("account");
if (!DMLUtils.checkUserOp(account, 12)) {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('您没有权限执行该操作!');window.location.href='index.jsp'</script>");
} else {
if (DMLUtils.deleteRole(roId) == 1) {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('删除失败,请重试!');window.location.href='dispatcher.do?operate=checkRoleList'</script>");
} else {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('成功删除角色" + roName + "!');window.location.href='dispatcher.do?operate=checkRoleList'</script>");
}
}
}
private void insertRole(HttpServletRequest req, HttpServletResponse resp) throws IOException {
String roName = req.getParameter("roName");
if (DMLUtils.isRoleNameExists(roName)) {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('该角色名已存在,请重新添加!');window.location.href='dispatcher.do?operate=addRole'</script>");
return;
}
int roLevel = Integer.parseInt(req.getParameter("roLevel"));
List<String> opIdList = getRoleOperate(req);
if (DMLUtils.addRole(roName, roLevel, opIdList) == 1) {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('成功添加角色" + roName + "!');window.location.href='dispatcher.do?operate=checkRoleList'</script>");
} else {
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().write("<script>alert('添加失败,请重试!');window.location.href='dispatcher.do?operate=addRole'</script>");
}
}
private void addRole(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String account = (String) re
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目实现了一个系统权限演示系统),功能如下: 1、可以管理用户; 2、可以管理权限,权限有:查询、修改、删除、增加等;3、可以分配权限 3、实现了一个系统登录界面(网页),用于输入用户名(大小均可)和密码(明文),点击登录铵键后,应实现对此用户的验证。如果验证成功,则进入系统(显示另外一个页面) ,并显示出该用户所拥有的权限(可直接以字符串形式显示在页面、界面上即可)。2、 用户的密码应实现相应的加密后保存在数据库中。 4、实现了用户的管理界面、权限管理界面或用户权限分配界面
资源推荐
资源详情
资源评论
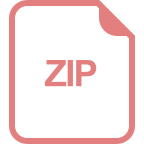
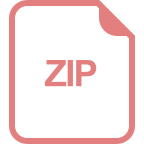
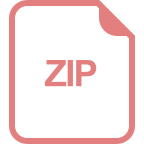
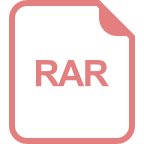
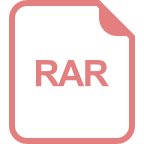
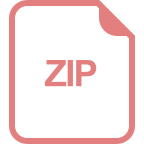
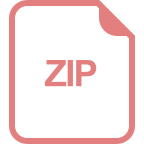
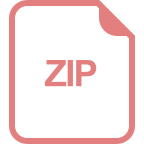
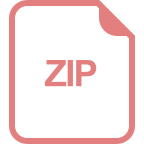
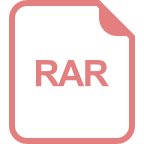
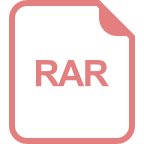
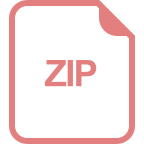
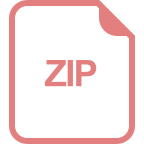
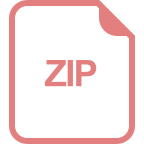
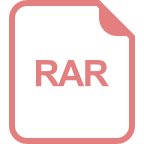
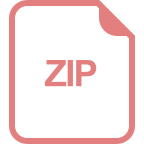
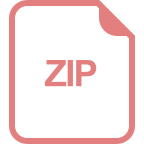
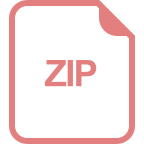
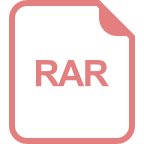
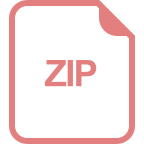
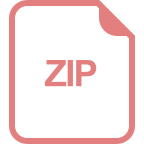
收起资源包目录

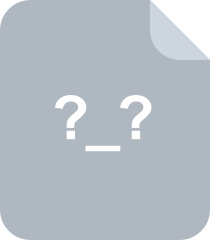
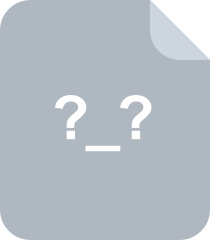
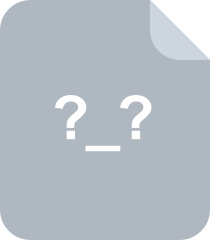
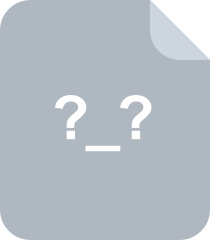
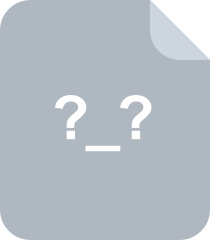
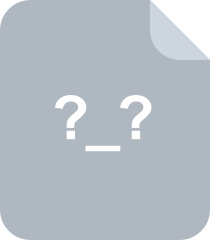
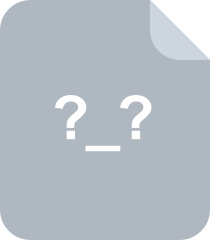
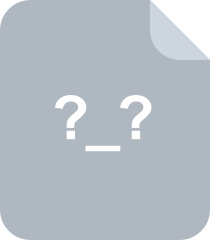
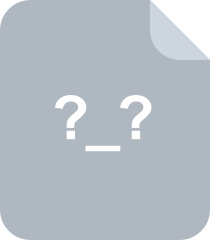
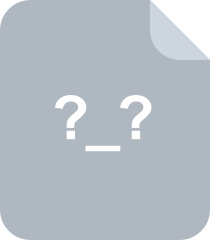
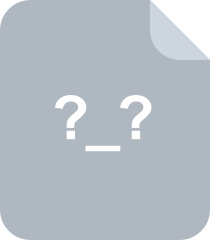
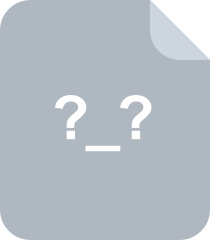
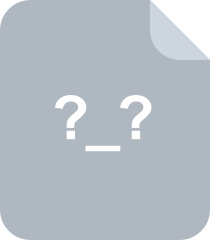
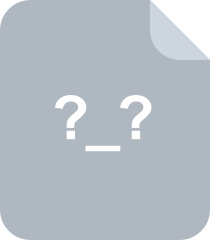
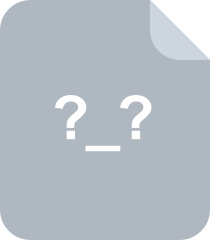
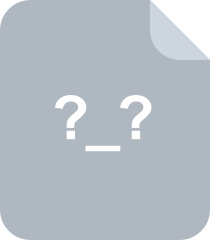
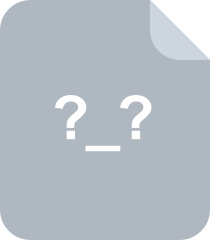
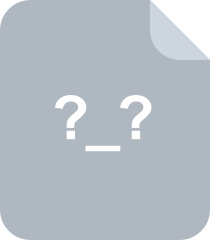
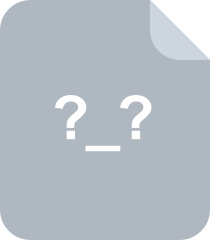
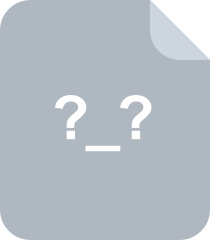
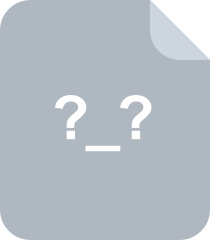
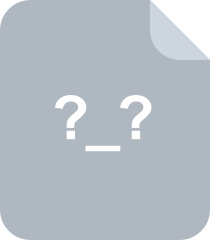
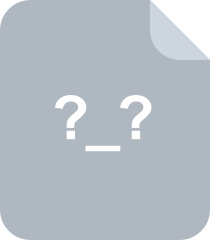
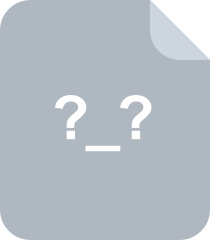
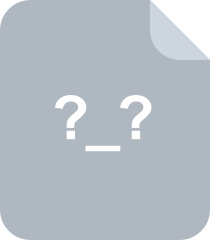
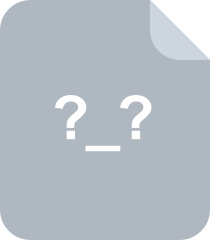
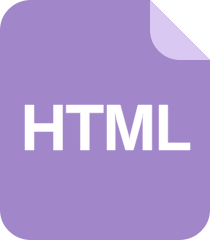
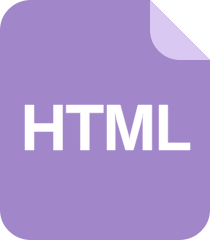
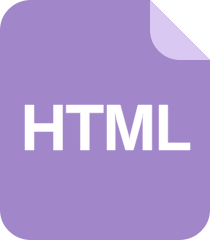
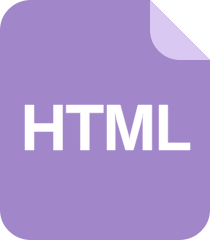
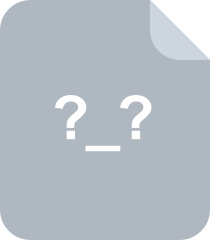
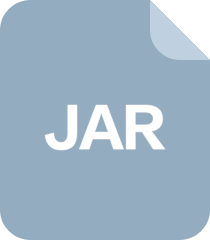
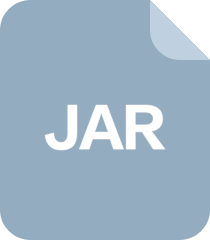
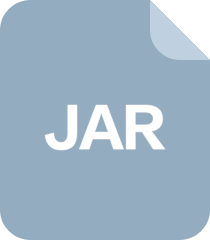
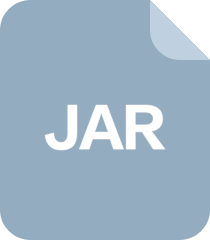
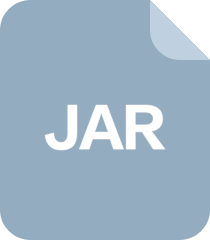
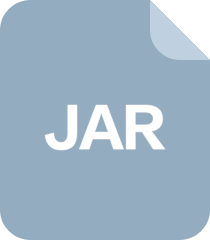
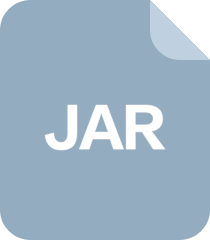
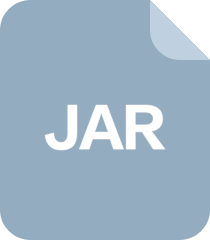
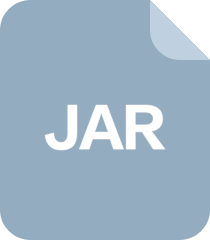
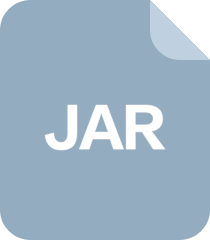
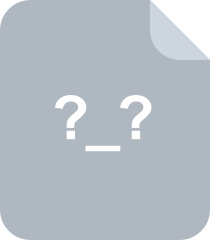
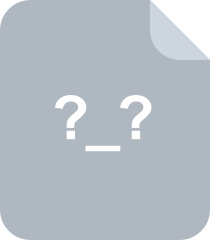
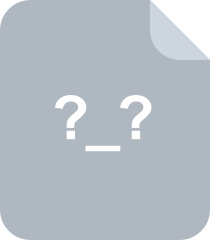
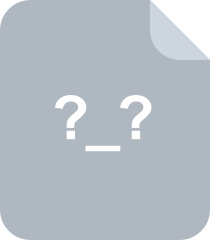
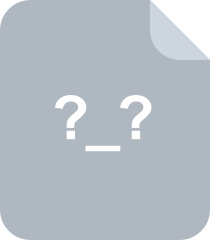
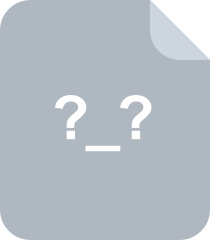
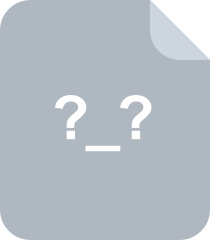
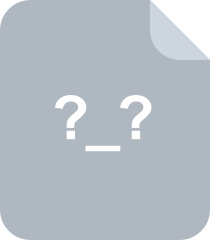
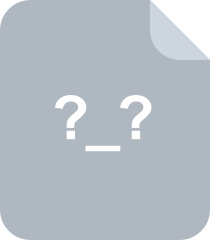
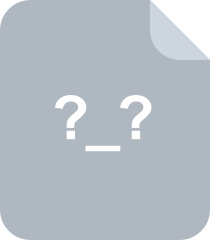
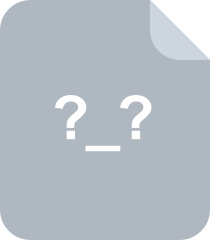
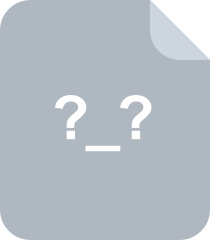
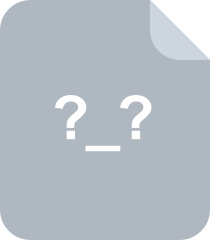
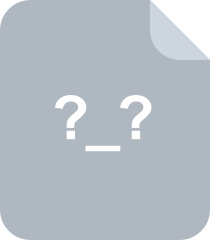
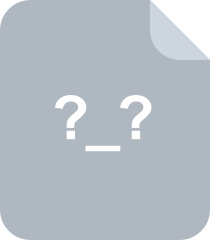
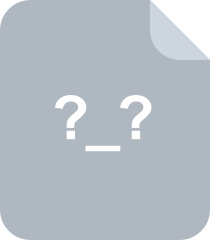
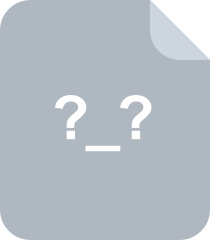
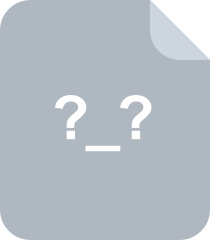
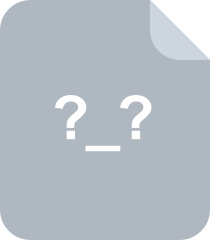
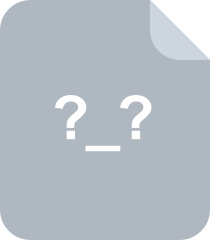
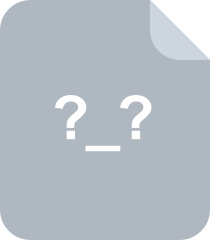
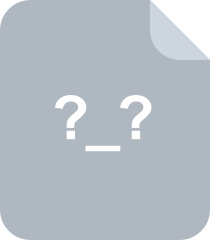
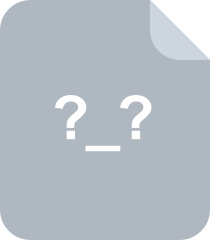
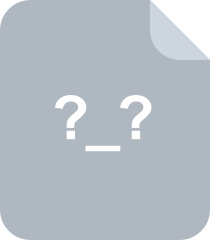
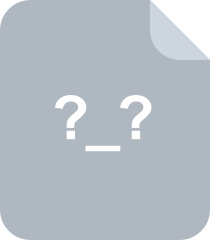
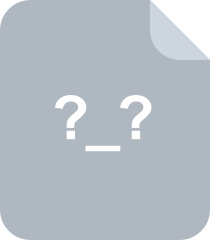
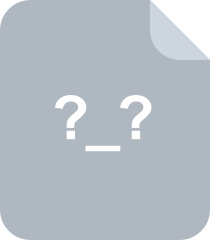
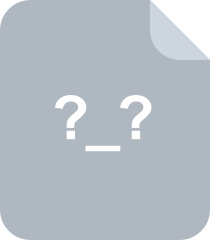
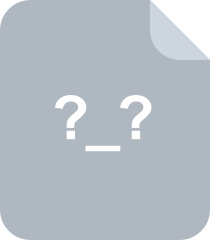
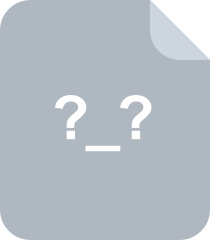
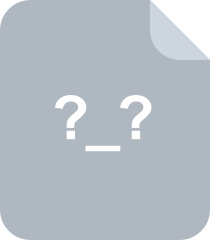
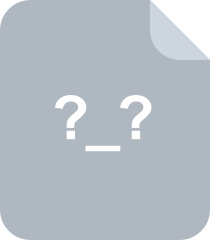
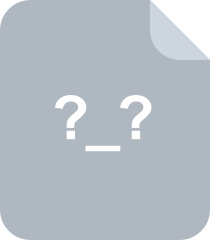
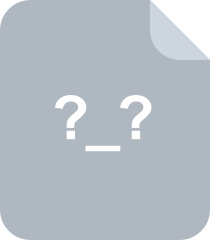
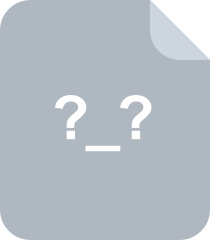
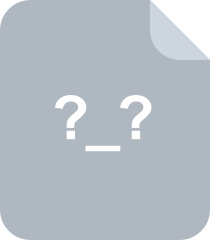
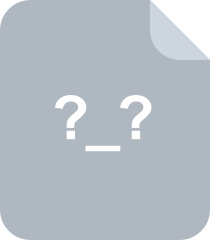
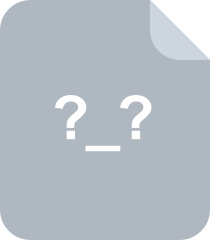
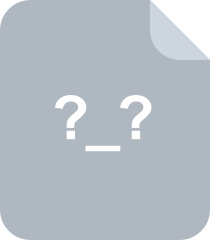
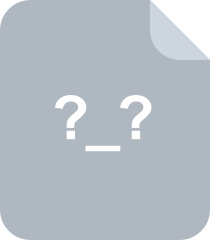
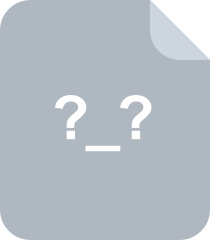
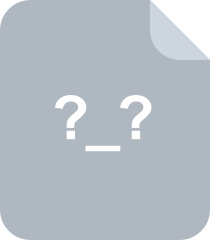
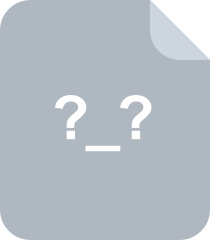
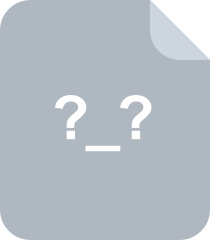
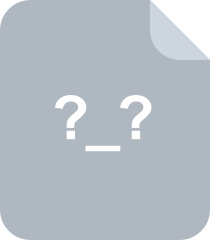
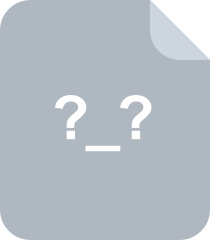
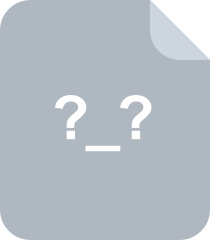
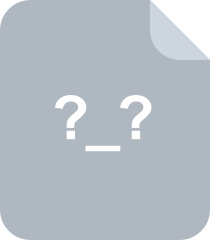
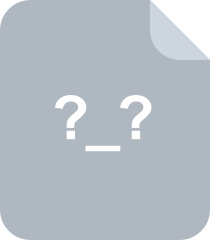
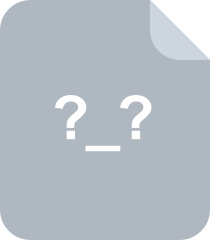
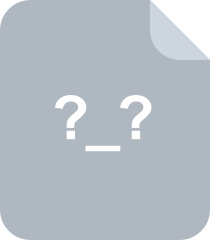
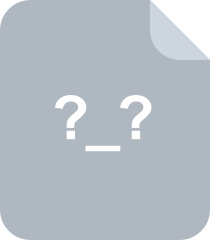
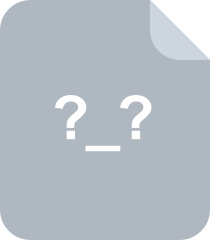
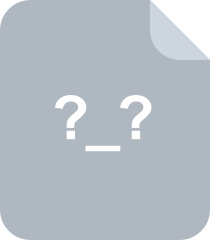
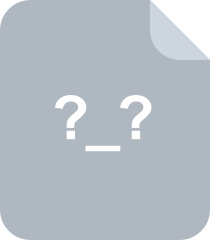
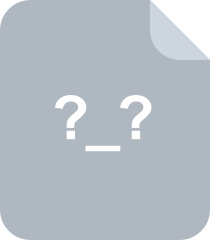
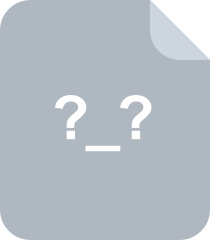
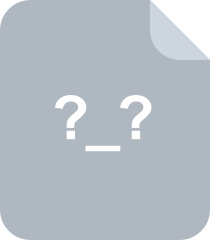
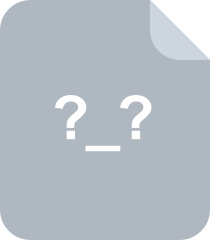
共 104 条
- 1
- 2
资源评论
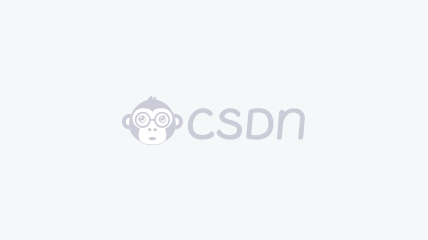

夜的旋粒_
- 粉丝: 43
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

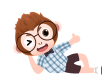
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


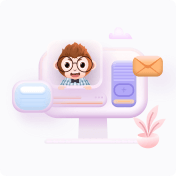
安全验证
文档复制为VIP权益,开通VIP直接复制
