from tkinter import *
from tkinter.messagebox import *
import pymysql
from tkinter import ttk
host = '127.0.0.1'
username = 'root'
password = 'root'
db = 'test'
import time
class InputFrame(Frame): # 继承Frame类
def __init__(self, master=None):
self.conn = pymysql.connect(host, username, password, db)
Frame.__init__(self, master)
self.root = master # 定义内部变量root
self.kind = StringVar()
self.age = StringVar()
self.sex = StringVar()
self.health = StringVar()
self.sinkness = StringVar()
self.color = StringVar()
self.createPage()
def search(self):
root1 = Tk()
root1.title('查询流浪狗信息')
cursor = self.conn.cursor()
sql = "select id,kind,age,sex,health,sinkness,color from dog_info"
cursor.execute(sql)
result = cursor.fetchall()
tree = ttk.Treeview(root1, columns=["编号", "种类", "年龄", "性别", "健康状况", "疾病史", "颜色"], show='headings') # 表格
tree.column("编号", width=60)
tree.column("种类", width=60)
tree.column("年龄", width=60)
tree.column("性别", width=60)
tree.column("健康状况", width=80)
tree.column("疾病史", width=60)
tree.column("颜色", width=60)
tree.heading("编号", text="编号")
tree.heading("种类", text="种类")
tree.heading("年龄", text="年龄")
tree.heading("性别", text="性别")
tree.heading("健康状况", text="健康状况")
tree.heading("疾病史", text="疾病史")
tree.heading("颜色", text="颜色")
for i in result:
tree.insert('', 'end', values=i)
tree.pack()
def insert(self):
kind = self.kind.get()
age = self.age.get()
sex = self.sex.get()
health = self.health.get()
sinkness = self.sinkness.get()
color = self.color.get()
cursor = self.conn.cursor()
sql = "insert into dog_info (kind,age,sex,health,sinkness,color) values ('%s','%s','%s','%s','%s','%s')" % (kind, age, sex, health, sinkness, color)
cursor.execute(sql)
self.conn.commit()
showinfo(title='hi', message='录入成功')
self.kind.set('')
self.age.set('')
self.sex.set('')
self.health.set('')
self.sinkness.set('')
self.color.set('')
def update(self):
def togengxin():
kind = b4.get()
did = b2.get()
age = b6.get()
health = b10.get()
sex = b8.get()
sinkness = b12.get()
color = b14.get()
cursor = self.conn.cursor()
sql = "update dog_info set kind='%s',age='%s',sex= '%s' ,health='%s', sinkness='%s', color='%s' where id = '%s'" % (kind, age, sex, health, sinkness, color, did)
cursor.execute(sql)
self.conn.commit()
showinfo(title='hi', message='修改成功')
root1.destroy()
root1 = Tk()
root1.title('修改流浪狗信息')
root1.geometry('300x400')
did = StringVar()
kind = StringVar()
age = StringVar()
health = StringVar()
sex = StringVar()
sinkness = StringVar()
color = StringVar()
b1 = Label(root1, text='请输入要修改狗狗的编号: ')
b1.pack()
b2 = Entry(root1, textvariable=did)
b2.pack()
b3 = Label(root1, text='请输入要修改狗狗的品种: ')
b3.pack()
b4 = Entry(root1, textvariable=kind)
b4.pack()
b5 = Label(root1, text='请输入要修改狗狗的年龄: ')
b5.pack()
b6 = Entry(root1, textvariable=age)
b6.pack()
b7 = Label(root1, text='请输入要修改狗狗的性别: ')
b7.pack()
b8 = Entry(root1, textvariable=sex)
b8.pack()
b9 = Label(root1, text='请输入要修改狗狗的身体状况: ')
b9.pack()
b10 = Entry(root1, textvariable=health)
b10.pack()
b11 = Label(root1, text='请输入要修改狗狗的疾病史: ')
b11.pack()
b12 = Entry(root1, textvariable=sinkness)
b12.pack()
b13 = Label(root1, text='请输入要修改狗狗的颜色: ')
b13.pack()
b14 = Entry(root1, textvariable=color)
b14.pack()
btn = Button(root1, text='修改', command=togengxin)
btn.pack()
root1.mainloop()
def delete(self):
def todelete():
kind = b2.get()
cursor = self.conn.cursor()
sql = "delete from dog_info where id = '%s'" % (kind)
cursor.execute(sql)
self.conn.commit()
showinfo(title='hi', message='删除成功')
root1.destroy()
root1 = Tk()
root1.title('删除流浪狗信息')
root1.geometry('300x200')
kind = StringVar()
b1 = Label(root1, text='请输入要删除狗狗的编号: ')
b1.pack()
b2 = Entry(root1, textvariable=kind)
b2.pack()
btn = Button(root1, text='删除', command=todelete)
btn.pack()
root1.mainloop()
def lingyang(self):
def tolingyang():
kind = b2.get()
name = b4.get()
phone = b6.get()
sex = b8.get()
addr = b10.get()
cursor = self.conn.cursor()
now = time.strftime("%Y-%m-%d", time.localtime())
sql = "insert into dog_lose values ('%s','%s','%s')" % (kind, now, addr)
cursor.execute(sql)
sql = "insert into dog_adopt values ('%s','%s','%s','%s','%s')" % (name, phone, sex, addr, kind)
cursor.execute(sql)
sql = "delete from dog_info where id = '%s'" % (kind)
cursor.execute(sql)
self.conn.commit()
showinfo(title='hi', message='领养成功')
root1.destroy()
root1 = Tk()
root1.title('领养流浪狗')
root1.geometry('300x400')
kind = StringVar()
name = StringVar()
phone = StringVar()
sex = StringVar()
addr = StringVar()
b1 = Label(root1, text='请输入要领养狗狗的编号: ')
b1.pack()
b2 = Entry(root1, textvariable=kind)
b2.pack()
b3 = Label(root1, text='请输入领养人姓名: ')
b3.pack()
b4 = Entry(root1, textvariable=name)
b4.pack()
b5 = Label(root1, text='请输入领养人电话: ')
b5.pack()
b6 = Entry(root1, textvariable=phone)
b6.pack()
b7 = Label(root1, text='请输入领养人性别: ')
b7.pack()
b8 = Entry(root1, textvariable=sex)
b8.pack()
b9 = Label(root1, text='请输入领养人地址: ')
b9.pack()
b10 = Entry(root1, textvariable=addr)
b10.pack()
btn = Button(root1, text='领养', command=tolingyang)
btn.pack()
root1.mainloop()
def createPage(self):
Button(self, text='查询流浪狗信息', command=self.search).grid(row=1, column=1, stick=E, pady=10)
Button(self, text='修改流浪狗信息', command=self.update).grid(row=1, column=2, stick=E, pady=10)
Button(self, text='领养流浪狗', command=self.lingyang).grid(row=1, column=3, stick=E, pady=10)
Button(self, text='删除流浪狗信息', command=self.delete).grid(row=1, column=4, stick=E, pady=10)
Label(self, text='狗狗品种: ').grid(row=3, column=2, stick=W, pady=10)
Entry(self, textvariable=self.kind).grid(row=3, column=3, stick=E)
Label(self, text='狗狗年龄: ').grid(row=4, column=2, stick=W, pady=10)
Entry(self, textvariable=self.age).grid(row=4, column=3, stick=E)
Label(self, text='狗狗性别: ').grid(row=5, column=2, stick=W, pady=10)
Entry(self, textvariable=self.sex).grid(row=
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
管理系统是一种通过计算机技术实现的用于组织、监控和控制各种活动的软件系统。这些系统通常被设计用来提高效率、减少错误、加强安全性,同时提供数据和信息支持。以下是一些常见类型的管理系统: 学校管理系统: 用于学校或教育机构的学生信息、教职员工信息、课程管理、成绩记录、考勤管理等。学校管理系统帮助提高学校的组织效率和信息管理水平。 人力资源管理系统(HRM): 用于处理组织内的人事信息,包括员工招聘、培训记录、薪资管理、绩效评估等。HRM系统有助于企业更有效地管理人力资源,提高员工的工作效率和满意度。 库存管理系统: 用于追踪和管理商品或原材料的库存。这种系统可以帮助企业避免库存过剩或不足的问题,提高供应链的效率。 客户关系管理系统(CRM): 用于管理与客户之间的关系,包括客户信息、沟通记录、销售机会跟踪等。CRM系统有助于企业更好地理解客户需求,提高客户满意度和保留率。 医院管理系统: 用于管理医院或医疗机构的患者信息、医生排班、药品库存等。这种系统可以提高医疗服务的质量和效率。 财务管理系统: 用于记录和管理组织的财务信息,包括会计凭证、财务报表、预算管理等。财务管理系统
资源推荐
资源详情
资源评论
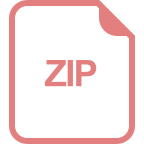
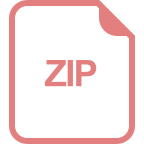
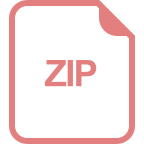
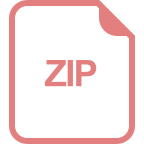
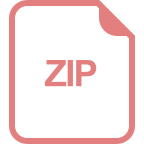
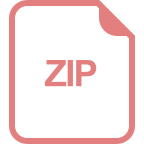
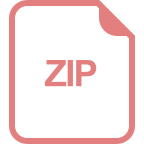
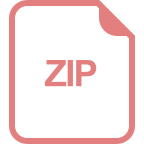
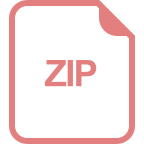
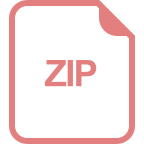
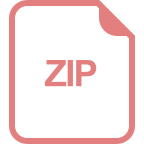
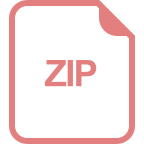
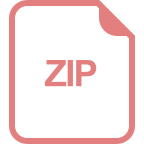
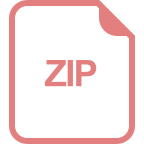
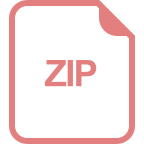
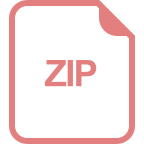
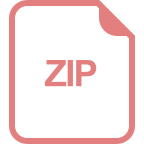
收起资源包目录




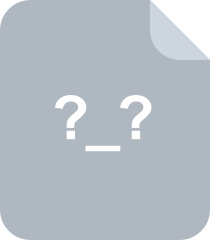
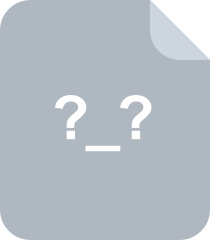
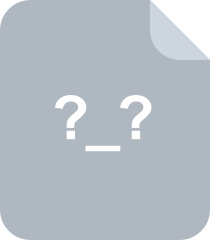
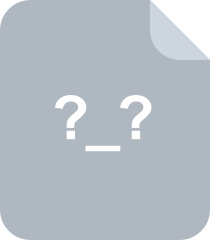
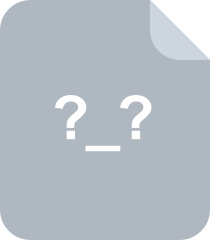
共 5 条
- 1
资源评论
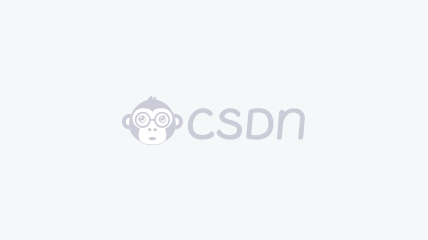

JJJ69
- 粉丝: 6341
- 资源: 5918
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

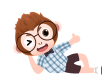
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


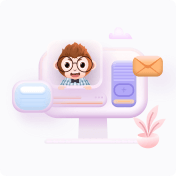
安全验证
文档复制为VIP权益,开通VIP直接复制
