# noqa
# type: ignore
# flake8: noqa
# pylint: skip-file
# mypy: ignore-errors
# yapf: disable
# pylama:skip=1
# *** PLEASE DO NOT MODIFY DIRECTLY: Automatically generated code ***
VERSION = "2.15.3"
import re
from .fastjsonschema_exceptions import JsonSchemaValueException
REGEX_PATTERNS = {
'^.*$': re.compile('^.*$'),
'.+': re.compile('.+'),
'^.+$': re.compile('^.+$'),
'idn-email_re_pattern': re.compile('^[^@]+@[^@]+\\.[^@]+\\Z')
}
NoneType = type(None)
def validate(data, custom_formats={}, name_prefix=None):
validate_https___packaging_python_org_en_latest_specifications_declaring_build_dependencies(data, custom_formats, (name_prefix or "data") + "")
return data
def validate_https___packaging_python_org_en_latest_specifications_declaring_build_dependencies(data, custom_formats={}, name_prefix=None):
if not isinstance(data, (dict)):
raise JsonSchemaValueException("" + (name_prefix or "data") + " must be object", value=data, name="" + (name_prefix or "data") + "", definition={'$schema': 'http://json-schema.org/draft-07/schema', '$id': 'https://packaging.python.org/en/latest/specifications/declaring-build-dependencies/', 'title': 'Data structure for ``pyproject.toml`` files', '$$description': ['File format containing build-time configurations for the Python ecosystem. ', ':pep:`517` initially defined a build-system independent format for source trees', 'which was complemented by :pep:`518` to provide a way of specifying dependencies ', 'for building Python projects.', 'Please notice the ``project`` table (as initially defined in :pep:`621`) is not included', 'in this schema and should be considered separately.'], 'type': 'object', 'additionalProperties': False, 'properties': {'build-system': {'type': 'object', 'description': 'Table used to store build-related data', 'additionalProperties': False, 'properties': {'requires': {'type': 'array', '$$description': ['List of dependencies in the :pep:`508` format required to execute the build', 'system. Please notice that the resulting dependency graph', '**MUST NOT contain cycles**'], 'items': {'type': 'string'}}, 'build-backend': {'type': 'string', 'description': 'Python object that will be used to perform the build according to :pep:`517`', 'format': 'pep517-backend-reference'}, 'backend-path': {'type': 'array', '$$description': ['List of directories to be prepended to ``sys.path`` when loading the', 'back-end, and running its hooks'], 'items': {'type': 'string', '$comment': 'Should be a path (TODO: enforce it with format?)'}}}, 'required': ['requires']}, 'project': {'$schema': 'http://json-schema.org/draft-07/schema', '$id': 'https://packaging.python.org/en/latest/specifications/declaring-project-metadata/', 'title': 'Package metadata stored in the ``project`` table', '$$description': ['Data structure for the **project** table inside ``pyproject.toml``', '(as initially defined in :pep:`621`)'], 'type': 'object', 'properties': {'name': {'type': 'string', 'description': 'The name (primary identifier) of the project. MUST be statically defined.', 'format': 'pep508-identifier'}, 'version': {'type': 'string', 'description': 'The version of the project as supported by :pep:`440`.', 'format': 'pep440'}, 'description': {'type': 'string', '$$description': ['The `summary description of the project', '<https://packaging.python.org/specifications/core-metadata/#summary>`_']}, 'readme': {'$$description': ['`Full/detailed description of the project in the form of a README', '<https://www.python.org/dev/peps/pep-0621/#readme>`_', "with meaning similar to the one defined in `core metadata's Description", '<https://packaging.python.org/specifications/core-metadata/#description>`_'], 'oneOf': [{'type': 'string', '$$description': ['Relative path to a text file (UTF-8) containing the full description', 'of the project. If the file path ends in case-insensitive ``.md`` or', '``.rst`` suffixes, then the content-type is respectively', '``text/markdown`` or ``text/x-rst``']}, {'type': 'object', 'allOf': [{'anyOf': [{'properties': {'file': {'type': 'string', '$$description': ['Relative path to a text file containing the full description', 'of the project.']}}, 'required': ['file']}, {'properties': {'text': {'type': 'string', 'description': 'Full text describing the project.'}}, 'required': ['text']}]}, {'properties': {'content-type': {'type': 'string', '$$description': ['Content-type (:rfc:`1341`) of the full description', '(e.g. ``text/markdown``). The ``charset`` parameter is assumed', 'UTF-8 when not present.'], '$comment': 'TODO: add regex pattern or format?'}}, 'required': ['content-type']}]}]}, 'requires-python': {'type': 'string', 'format': 'pep508-versionspec', '$$description': ['`The Python version requirements of the project', '<https://packaging.python.org/specifications/core-metadata/#requires-python>`_.']}, 'license': {'description': '`Project license <https://www.python.org/dev/peps/pep-0621/#license>`_.', 'oneOf': [{'properties': {'file': {'type': 'string', '$$description': ['Relative path to the file (UTF-8) which contains the license for the', 'project.']}}, 'required': ['file']}, {'properties': {'text': {'type': 'string', '$$description': ['The license of the project whose meaning is that of the', '`License field from the core metadata', '<https://packaging.python.org/specifications/core-metadata/#license>`_.']}}, 'required': ['text']}]}, 'authors': {'type': 'array', 'items': {'$ref': '#/definitions/author'}, '$$description': ["The people or organizations considered to be the 'authors' of the project.", 'The exact meaning is open to interpretation (e.g. original or primary authors,', 'current maintainers, or owners of the package).']}, 'maintainers': {'type': 'array', 'items': {'$ref': '#/definitions/author'}, '$$description': ["The people or organizations considered to be the 'maintainers' of the project.", 'Similarly to ``authors``, the exact meaning is open to interpretation.']}, 'keywords': {'type': 'array', 'items': {'type': 'string'}, 'description': 'List of keywords to assist searching for the distribution in a larger catalog.'}, 'classifiers': {'type': 'array', 'items': {'type': 'string', 'format': 'trove-classifier', 'description': '`PyPI classifier <https://pypi.org/classifiers/>`_.'}, '$$description': ['`Trove classifiers <https://pypi.org/classifiers/>`_', 'which apply to the project.']}, 'urls': {'type': 'object', 'description': 'URLs associated with the project in the form ``label => value``.', 'additionalProperties': False, 'patternProperties': {'^.+$': {'type': 'string', 'format': 'url'}}}, 'scripts': {'$ref': '#/definitions/entry-point-group', '$$description': ['Instruct the installer to create command-line wrappers for the given', '`entry points <https://packaging.python.org/specifications/entry-points/>`_.']}, 'gui-scripts': {'$ref': '#/definitions/entry-point-group', '$$description': ['Instruct the installer to create GUI wrappers for the given', '`entry points <https://packaging.python.org/specifications/entry-points/>`_.', 'The difference between ``scripts`` and ``gui-scripts`` is only relevant in', 'Windows.']}, 'entry-points': {'$$description': ['Instruct the installer to expose the given modules/functions via', '``entry-point`` discovery mechanism (useful for plugins).', 'More information available in the `Python packaging guide', '<https://packaging.python.org/specifications/entry-points/>`_.'], 'propertyNames': {'format': 'python-entrypoint-group'}, 'additionalProperties': False, 'patternProperties': {'^.+$': {'$ref': '#/definitions/entry-point-group'}}}, 'dependencies': {'type': 'array', 'description': 'Project (mandatory) dependencies.', 'items': {'$ref': '#/definitions/dependency'}}, 'optional-dependencies': {'type': 'object', 'description': 'Optional dependency for the project', 'propertyNames': {'format': 'pep508-identifier'}, 'additionalProperties': False, 'patternProperties': {'^.+$': {'type': 'array', 'items': {'$ref': '#/definition

程序员张小妍
- 粉丝: 1w+
- 资源: 3595
最新资源
- 含风电-光伏-光热电站电力系统N-k安全优化调度模型 关键词:N-K安全约束 光热电站 优化调度 参考文档:《光热电站促进风电消纳的电力系统优化调度》参考光热电站模型; 仿真平台: MATLAB +
- C++程序,基于元胞自动机法模拟枝晶生长,能实现任意角度(偏心正方算法),同时采用LBM考虑了对流作用对枝晶生长的影响
- MATLAB 用蚁群算法解决旅行商TSP问题
- 转速电流双闭环,无传感器无刷直流电机Simulink模型: 第一张图为转速,转矩 第二张图为反向电动势 第三张图为三相电流
- 风储调频 使用双馈发电机(DFIG)相关的电池储能系统(BESS)来支持一次频率,包含相关的控制策略 该模型包含2.0MW690V双馈发电机DFIG与电池储能系统BESS的Simulink模型,此
- 永磁同步电机PMSM模糊PI控制,内有详细的搭建过程以及对应详细的参考资料 另外有与传统PI对比的资料以及相关模型,全套一起打包 仿真效果非常好.
- comsol导模共振双BIC
- 滑模PMSG风力涡轮发电机Simulink模型
- comsol电磁超声压电接收EMAT 在1mm厚铝板中激励250kHz的电磁超声在200mm位置处设置一个深0.8mm的裂纹缺陷,左端面设为低反射边界 在85mm位置处放置一个压电片接收信号,信号如
- 多源联合系统的优化调度 包括风光火-抽水蓄能-蓄电池5种电源的优化调度 在MATLAB中采用yalmip进行编码 采用cplex求解 程序运行良好 注释详尽 适合电力系统优化初学者学习
- AGV全覆盖移动避障路径规划 扫地机器人路径规划 第一类算法 全覆盖智能算法 %% 基于深度优先搜索算法的路径规划-扫地机器人移动仿真 % 返回深度优先搜索实现全覆盖的运行次数 % 将栅格模型的每一个
- 多智能体系统,一致性,事件触发,一阶事件触发仿真文件,效果好,有对应参考文献
- VMD信号分解算法 1、VMD功率分解,VMD滚动轴承故障,将原始信号分解成多个模态 2、然后进行降噪、滤波等作用 光伏功率分解 3、滚动轴承故障检测 混合储能容量分配等 4、也可用于应用于时
- 蓄电池与超级电容器混合储能并网Matlab Simulink仿真模型,仅供学习交流 已测试,模型正确,曲线输出正常 可送相关参考文献 (1)混合储能,模型原创,储能并网,混合储能能量管理,蓄
- 小土堆学习之最大池化层与卷积层
- 基于ANN神经网络的无刷直流电机(BLDC)转速控制 适合课题研究与参考学习 主要包括: 1) BLDC的开环控制Simulink模型; 2) 基于PI的无刷直流电机BLDC的转速控制; 3) 基于A
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


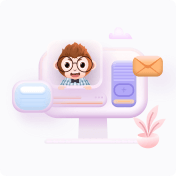