package com.controller;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.ShukuxinxiEntity;
import com.entity.view.ShukuxinxiView;
import com.service.ShukuxinxiService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.MapUtils;
import com.utils.CommonUtil;
import java.io.IOException;
import com.service.YonghuService;
import com.entity.YonghuEntity;
/**
* 书库信息
* 后端接口
* @author
* @email
* @date 2023-12-13 14:20:14
*/
@RestController
@RequestMapping("/shukuxinxi")
public class ShukuxinxiController {
@Autowired
private ShukuxinxiService shukuxinxiService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,ShukuxinxiEntity shukuxinxi,
HttpServletRequest request){
EntityWrapper<ShukuxinxiEntity> ew = new EntityWrapper<ShukuxinxiEntity>();
PageUtils page = shukuxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, shukuxinxi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,ShukuxinxiEntity shukuxinxi,
HttpServletRequest request){
EntityWrapper<ShukuxinxiEntity> ew = new EntityWrapper<ShukuxinxiEntity>();
PageUtils page = shukuxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, shukuxinxi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( ShukuxinxiEntity shukuxinxi){
EntityWrapper<ShukuxinxiEntity> ew = new EntityWrapper<ShukuxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( shukuxinxi, "shukuxinxi"));
return R.ok().put("data", shukuxinxiService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(ShukuxinxiEntity shukuxinxi){
EntityWrapper< ShukuxinxiEntity> ew = new EntityWrapper< ShukuxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( shukuxinxi, "shukuxinxi"));
ShukuxinxiView shukuxinxiView = shukuxinxiService.selectView(ew);
return R.ok("查询书库信息成功").put("data", shukuxinxiView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
ShukuxinxiEntity shukuxinxi = shukuxinxiService.selectById(id);
shukuxinxi.setClicknum(shukuxinxi.getClicknum()+1);
shukuxinxi.setClicktime(new Date());
shukuxinxiService.updateById(shukuxinxi);
return R.ok().put("data", shukuxinxi);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
ShukuxinxiEntity shukuxinxi = shukuxinxiService.selectById(id);
shukuxinxi.setClicknum(shukuxinxi.getClicknum()+1);
shukuxinxi.setClicktime(new Date());
shukuxinxiService.updateById(shukuxinxi);
return R.ok().put("data", shukuxinxi);
}
/**
* 赞或踩
*/
@RequestMapping("/thumbsup/{id}")
public R vote(@PathVariable("id") String id,String type){
ShukuxinxiEntity shukuxinxi = shukuxinxiService.selectById(id);
if(type.equals("1")) {
shukuxinxi.setThumbsupnum(shukuxinxi.getThumbsupnum()+1);
} else {
shukuxinxi.setCrazilynum(shukuxinxi.getCrazilynum()+1);
}
shukuxinxiService.updateById(shukuxinxi);
return R.ok("投票成功");
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody ShukuxinxiEntity shukuxinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(shukuxinxi);
shukuxinxiService.insert(shukuxinxi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody ShukuxinxiEntity shukuxinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(shukuxinxi);
shukuxinxiService.insert(shukuxinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
public R update(@RequestBody ShukuxinxiEntity shukuxinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(shukuxinxi);
shukuxinxiService.updateById(shukuxinxi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
shukuxinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 前端智能排序
*/
@IgnoreAuth
@RequestMapping("/autoSort")
public R autoSort(@RequestParam Map<String, Object> params,ShukuxinxiEntity shukuxinxi, HttpServletRequest request,String pre){
EntityWrapper<ShukuxinxiEntity> ew = new EntityWrapper<ShukuxinxiEntity>();
Map<String, Object> newMap = new HashMap<String, Object>();
Map<String, Object> param = new HashMap<String, Object>();
Iterator<Map.Entry<String, Object>> it = param.entrySet().iterator();
while (it.hasNext()) {
Map.Entry<String, Object> entry = it.next();
String key = entry.getKey();
String newKey = entry.getKey();
if (pre.endsWith(".")) {
newMap.put(pre + newKey, entry.getValue());
} else if (StringUtils.isEmpty(pre)) {
newMap.put(newKey, entry.getValue());
} else {
newMap.put(pre + "." + newKey, entry.getValue());
}
}
params.put("sort", "clicknum");
params.put("order", "desc");
PageUtils page = shukuxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, shukuxinxi), params), params));
return R.ok().put("data", page);
}
@Autowired
private YonghuService yonghuService;
/**
* 协同算法(按注册选型推荐)
*/
@RequestMapping("/autoSort2")
public R autoSort2(@RequestParam Map<String, Object> params,ShukuxinxiEntity shukuxinxi, HttpServletRequest request){
String userId = request.getSession().getAttribute("userId").toString();
String inteltypeColumn = "xiaoshuofenlei";
String tableName = request.getSession().getAttribute("tableName").toString();
List<String> inteltypes = new ArrayList<String>();
if(tableName.equals("yonghu")) {
YonghuEntity yonghu = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("id", userId));
inteltypes = Arrays.asList(yonghu.getXiaoshuofenlei().split(","));
}
Integer limit = params.get("limit")==null?10:Integer.parseInt(p
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
项目经过测试均可完美运行! 环境说明: 开发语言:java 框架:springboot jdk版本:jdk1.8 数据库:mysql 5.7+ 数据库工具:Navicat11+ 管理工具:maven 开发工具:idea/eclipse 部署容器:tomcat7+
资源推荐
资源详情
资源评论
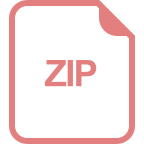
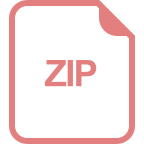
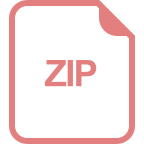
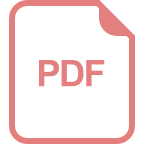
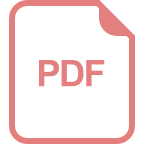
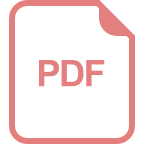
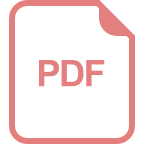
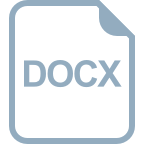
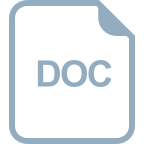
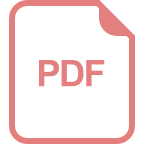
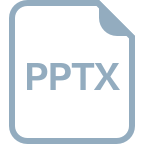
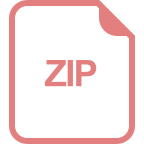
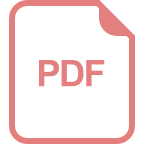
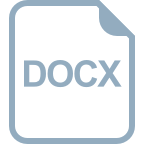
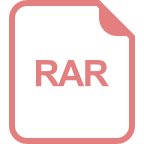
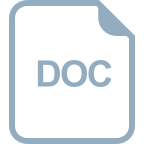
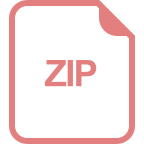
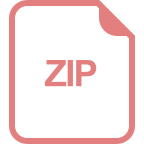
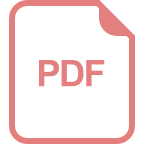
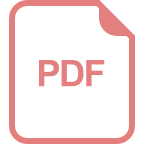
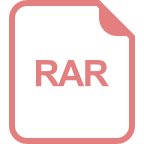
收起资源包目录

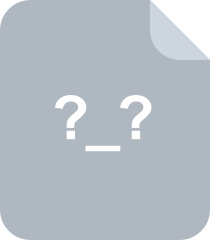
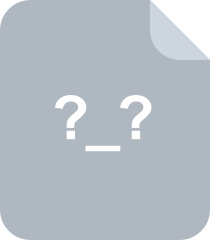
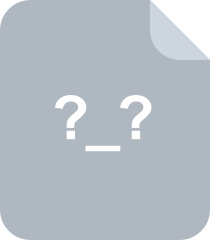
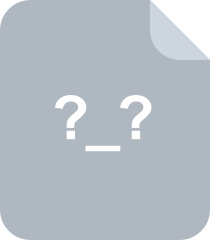
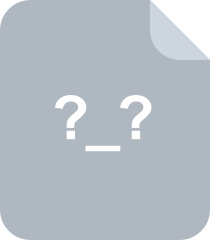
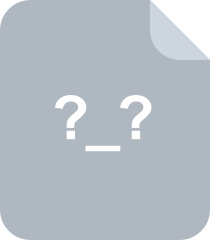
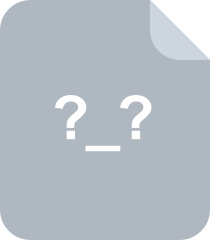
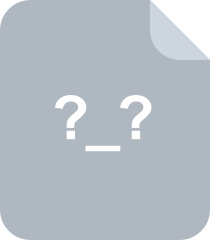
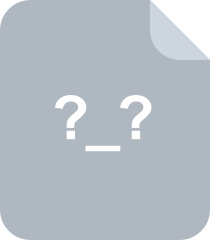
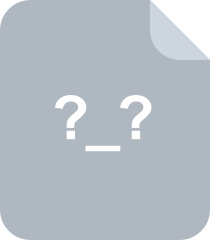
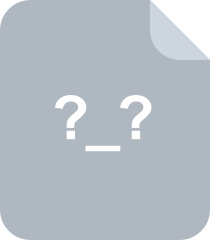
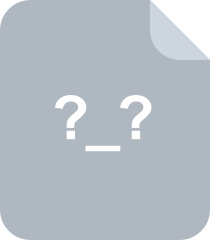
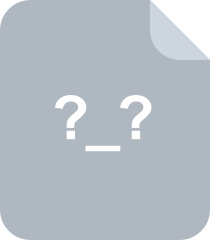
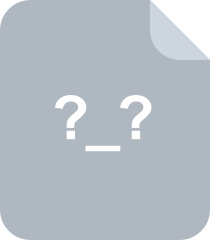
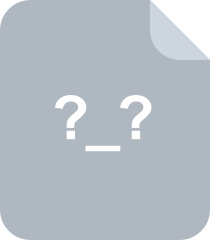
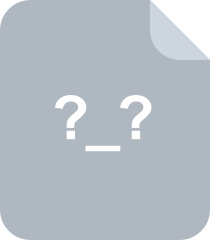
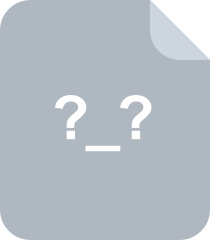
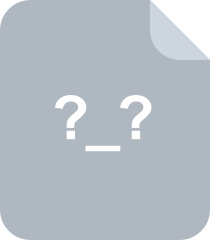
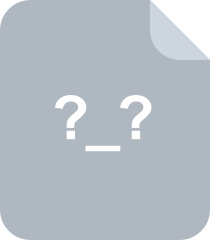
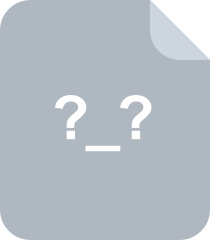
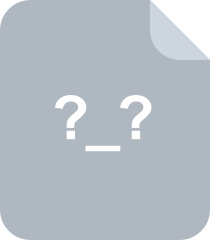
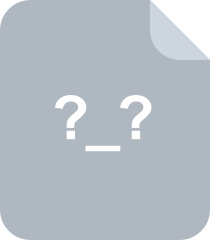
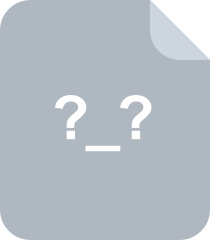
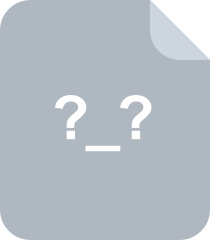
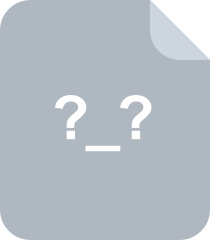
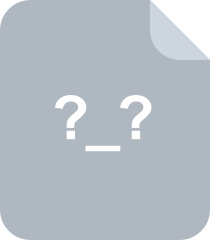
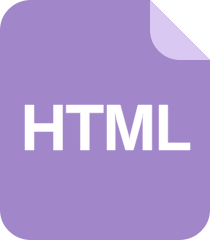
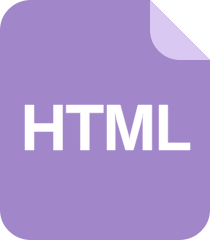
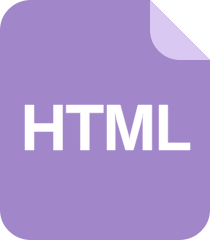
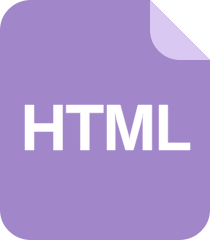
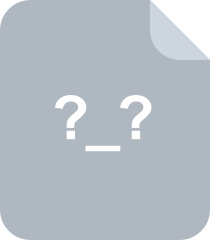
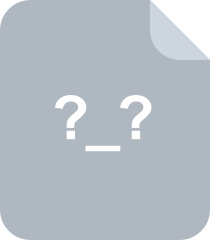
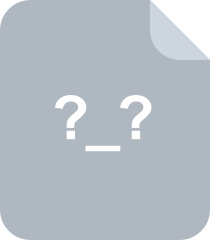
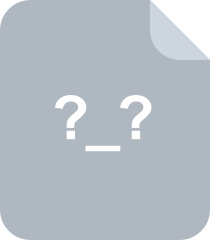
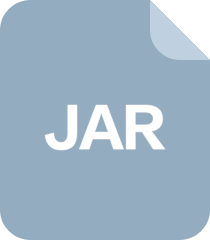
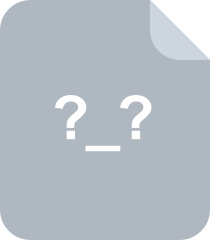
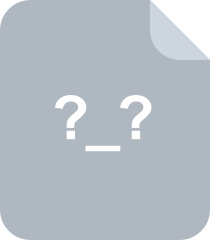
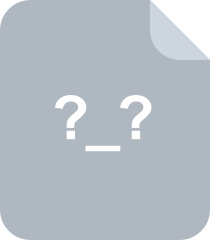
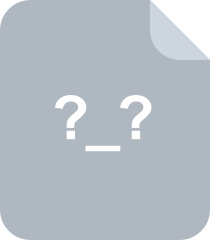
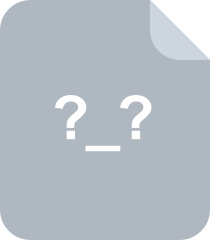
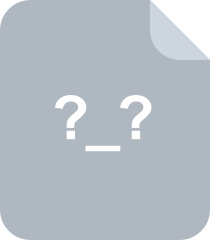
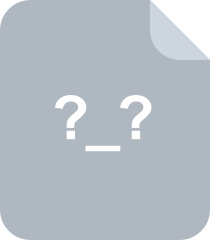
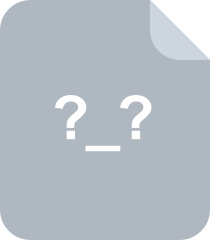
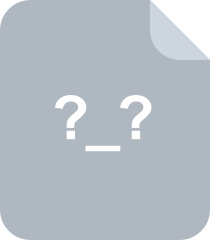
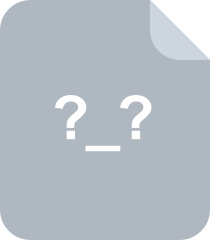
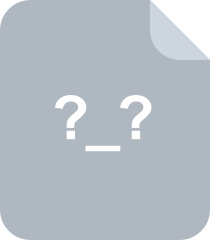
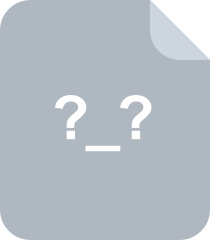
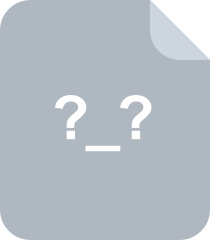
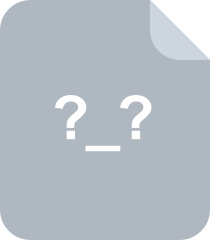
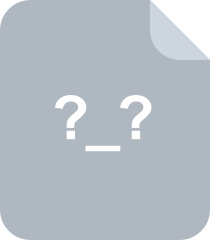
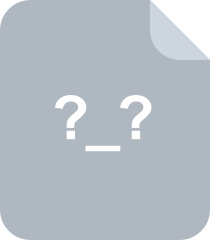
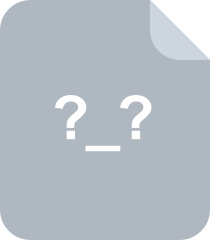
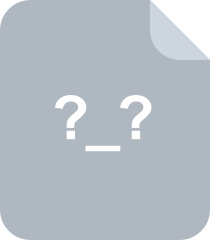
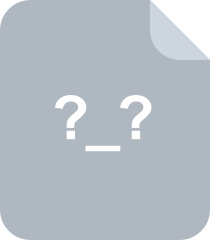
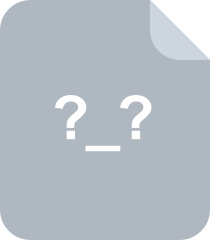
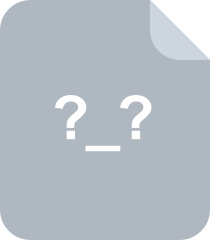
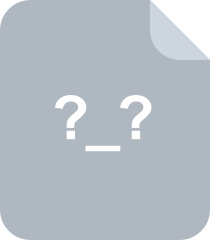
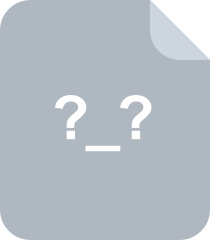
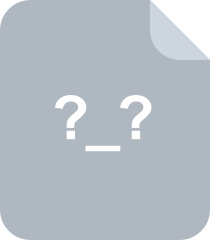
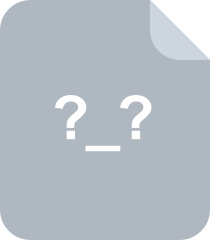
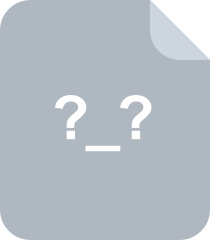
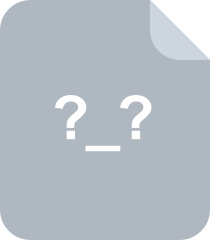
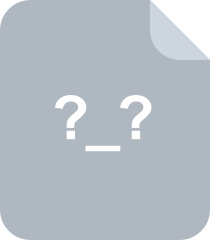
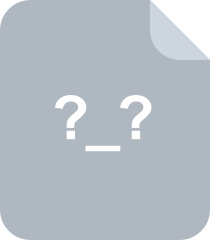
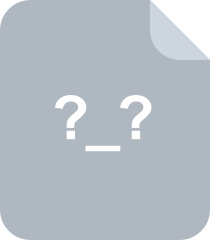
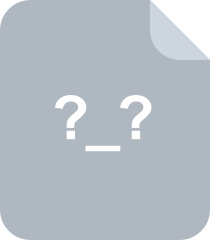
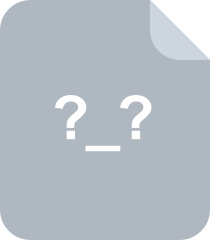
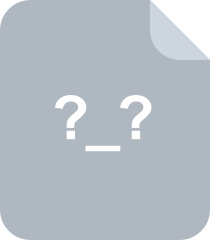
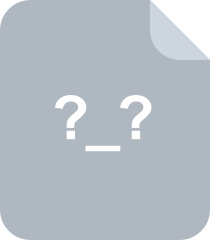
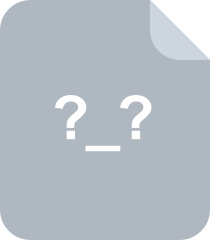
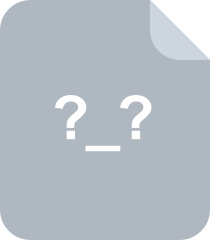
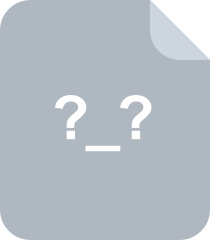
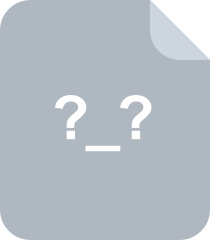
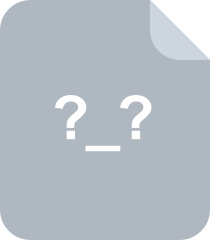
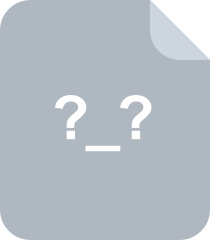
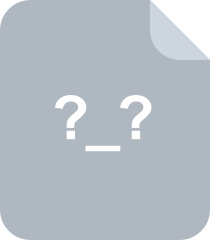
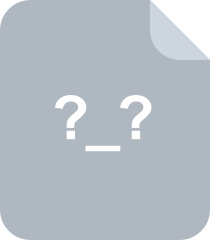
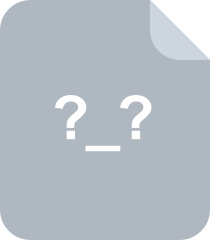
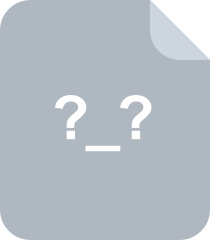
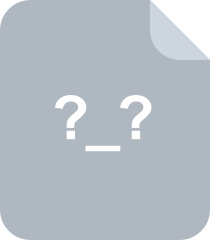
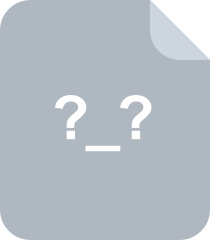
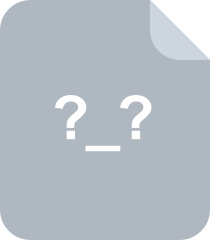
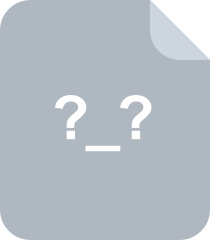
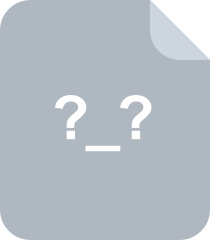
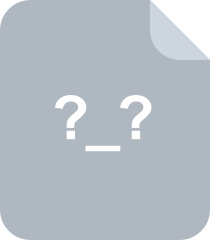
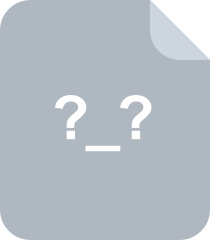
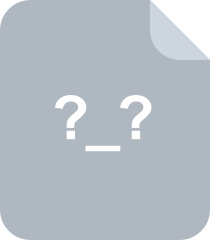
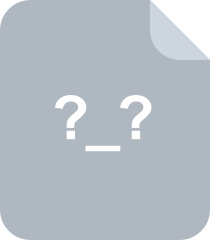
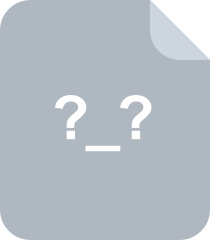
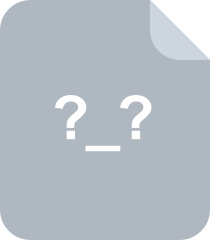
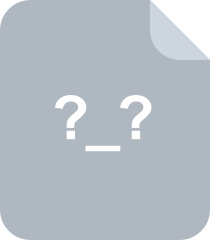
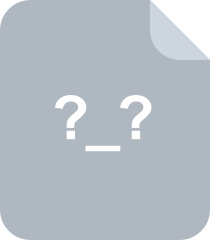
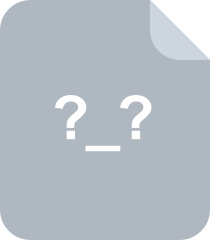
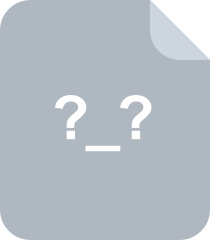
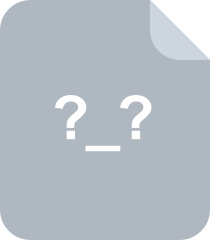
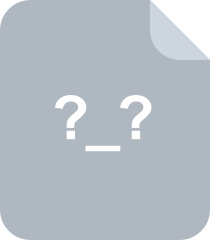
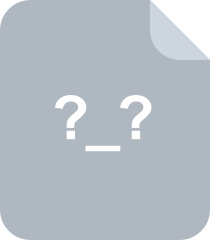
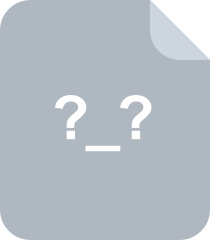
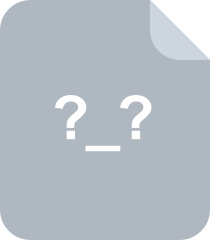
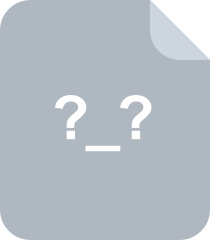
共 656 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
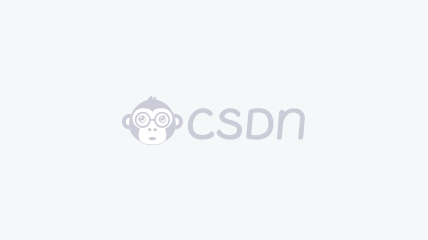

计算机学长阿伟
- 粉丝: 3192
- 资源: 698
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

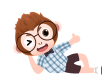
最新资源
- Example162.java
- Vert.x,应用监控 - 全链路跟踪,基于Zipkin
- 用于信捷忘记密码后升级固件
- 中国光伏电站安装时间的多边形地理空间数据集(2010-2022年)-最新出炉.zip
- 几种常见简单滤波器用于二维图像降噪,包括均值、中值、高斯、低通、双边滤波器,语言是python
- 二手车管理系统,pc端,小程序端,java后端
- 2011-2022年中国光伏电站遥感识别面矢量数据-最新出炉.zip
- 基于深度学习的边缘计算网络的卸载优化及资源优化python源码+文档说明(高分项目)
- 基于yolov5+超声图像的钢轨缺陷检测python源码+数据集(高分毕设)
- 基于大语言模型的智能审计问答系统python源码+文档说明(高分项目)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


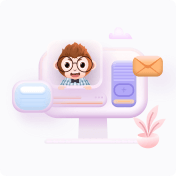
安全验证
文档复制为VIP权益,开通VIP直接复制
