package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.ShangpinxinxiEntity;
import com.entity.view.ShangpinxinxiView;
import com.service.ShangpinxinxiService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.CommonUtil;
import java.io.IOException;
/**
* 商品信息
* 后端接口
* @author
* @email
* @date 2022-03-26 17:54:50
*/
@RestController
@RequestMapping("/shangpinxinxi")
public class ShangpinxinxiController {
@Autowired
private ShangpinxinxiService shangpinxinxiService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,ShangpinxinxiEntity shangpinxinxi,
HttpServletRequest request){
EntityWrapper<ShangpinxinxiEntity> ew = new EntityWrapper<ShangpinxinxiEntity>();
PageUtils page = shangpinxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, shangpinxinxi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,ShangpinxinxiEntity shangpinxinxi,
HttpServletRequest request){
EntityWrapper<ShangpinxinxiEntity> ew = new EntityWrapper<ShangpinxinxiEntity>();
PageUtils page = shangpinxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, shangpinxinxi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( ShangpinxinxiEntity shangpinxinxi){
EntityWrapper<ShangpinxinxiEntity> ew = new EntityWrapper<ShangpinxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( shangpinxinxi, "shangpinxinxi"));
return R.ok().put("data", shangpinxinxiService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(ShangpinxinxiEntity shangpinxinxi){
EntityWrapper< ShangpinxinxiEntity> ew = new EntityWrapper< ShangpinxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( shangpinxinxi, "shangpinxinxi"));
ShangpinxinxiView shangpinxinxiView = shangpinxinxiService.selectView(ew);
return R.ok("查询商品信息成功").put("data", shangpinxinxiView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
ShangpinxinxiEntity shangpinxinxi = shangpinxinxiService.selectById(id);
return R.ok().put("data", shangpinxinxi);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
ShangpinxinxiEntity shangpinxinxi = shangpinxinxiService.selectById(id);
return R.ok().put("data", shangpinxinxi);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody ShangpinxinxiEntity shangpinxinxi, HttpServletRequest request){
shangpinxinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(shangpinxinxi);
shangpinxinxiService.insert(shangpinxinxi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody ShangpinxinxiEntity shangpinxinxi, HttpServletRequest request){
shangpinxinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(shangpinxinxi);
shangpinxinxiService.insert(shangpinxinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody ShangpinxinxiEntity shangpinxinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(shangpinxinxi);
shangpinxinxiService.updateById(shangpinxinxi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
shangpinxinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
Wrapper<ShangpinxinxiEntity> wrapper = new EntityWrapper<ShangpinxinxiEntity>();
if(map.get("remindstart")!=null) {
wrapper.ge(columnName, map.get("remindstart"));
}
if(map.get("remindend")!=null) {
wrapper.le(columnName, map.get("remindend"));
}
int count = shangpinxinxiService.selectCount(wrapper);
return R.ok().put("count", count);
}
/**
* (按值统计)
*/
@RequestMapping("/value/{xColumnName}/{yColumnName}")
public R value(@PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName,HttpServletRequest request) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("xColumn", xColumnName);
params.put("yColumn", yColumnName);
EntityWrapper<ShangpinxinxiEntity> ew = new EntityWrapper<ShangpinxinxiEntity>();
List<Map<String, Object>> result = shangpinxinxiService.selectValue(params, ew);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
for(Map<String, Object> m : result) {
for(String k : m.keySet()) {
if(m.get(k) instanceof Date) {
m.put(k, sdf.format((Date)m.get(k)));
}
}
}
return R.ok().put("data", result);
}
/**
* (按值统计)时间统计类型
*/
@RequestMapping("/value/{xColumnName}/{yColumnName}/{timeStatType}")
public R valueDay(@PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnNa
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
网吧管理系统是一个集成了多个功能模块的综合性系统,旨在提高网吧的运营效率和服务质量。以下是对该系统主要功能的详细介绍: 一、管理员功能 管理员是整个系统的核心,负责系统的整体管理和监控。管理员可以访问并管理所有功能模块,包括用户信息管理、会员管理、网管管理等,确保网吧的日常运营顺畅。 二、个人中心 个人中心是用户的个人信息管理区域。用户可以在此查看并编辑自己的个人信息,如姓名、联系方式、密码等。同时,个人中心还提供订单管理功能,用户可以查看自己的上机记录、消费记录等。 三、会员管理 会员管理模块用于管理网吧的会员信息。系统支持会员注册、登录、积分查询等功能,方便网吧对会员进行管理和营销。此外,会员还可以享受网吧提供的各种优惠和特权。 四、网管管理 网管管理模块用于管理网吧的网管人员。系统支持网管的添加、删除、修改等操作,方便网吧对网管人员进行考核和管理。同时,网管可以通过系统接收用户的呼叫和服务请求,提高服务质量。 五、商品与服务管理 品类型管理与品信息管理:系统支持对网吧提供的商品进行分类管理,如饮料、零食等。同时,可以详细记录每种商品的信息,如价格、库存等。 买
资源推荐
资源详情
资源评论
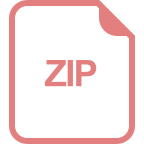
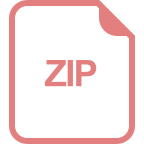
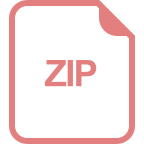
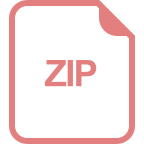
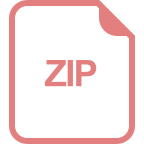
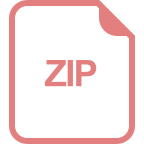
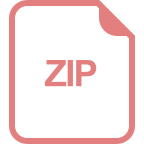
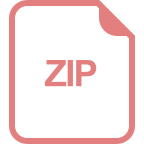
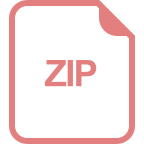
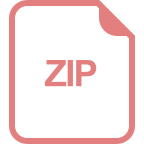
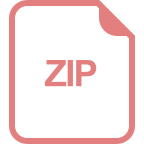
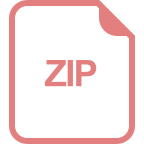
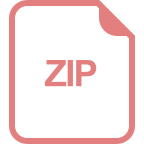
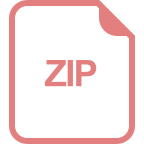
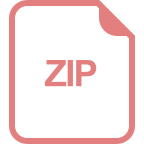
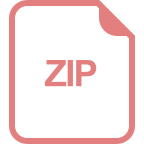
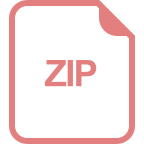
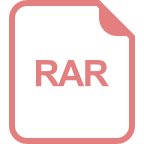
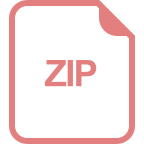
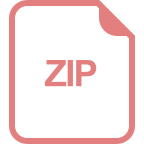
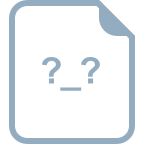
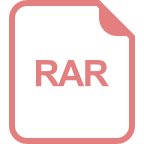
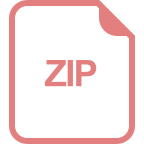
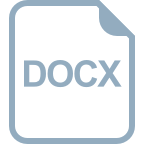
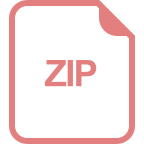
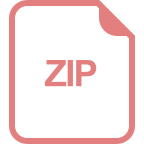
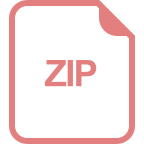
收起资源包目录

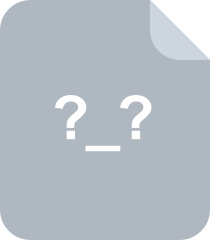
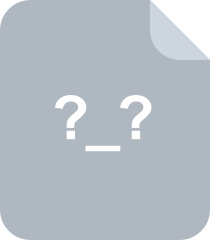
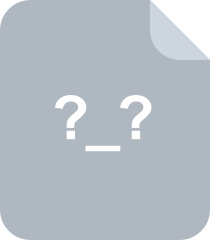
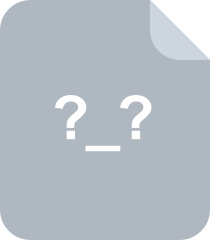
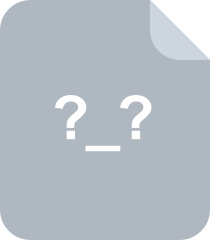
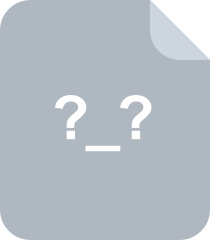
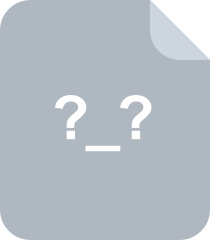
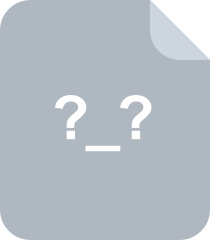
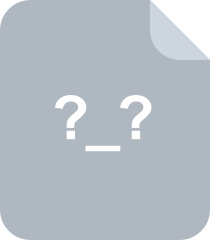
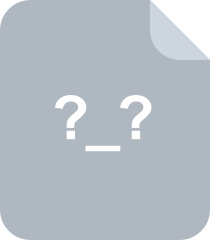
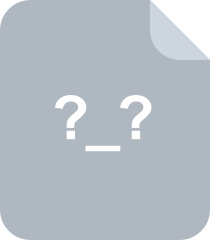
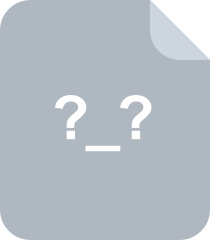
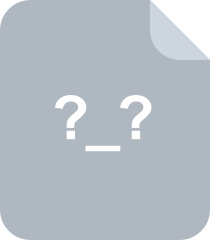
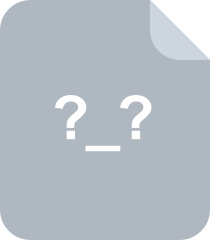
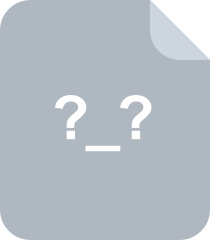
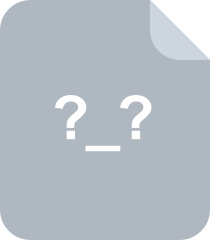
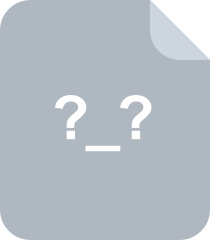
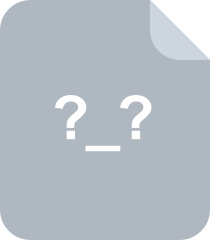
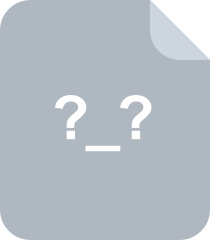
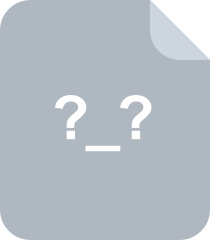
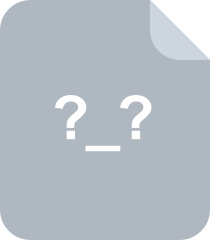
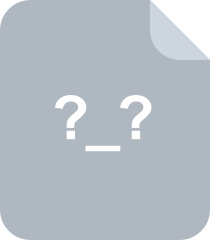
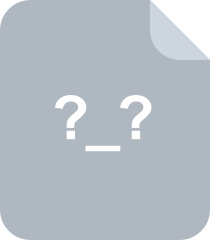
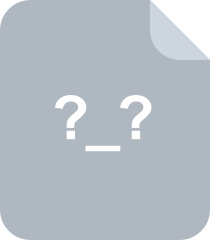
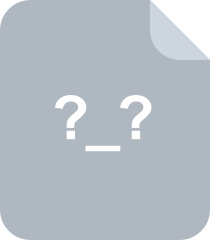
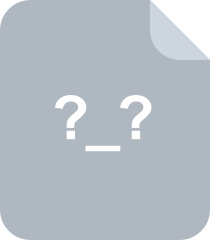
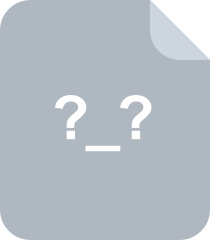
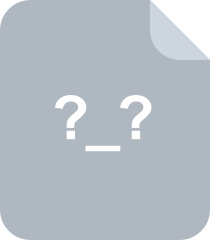
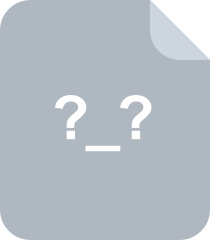
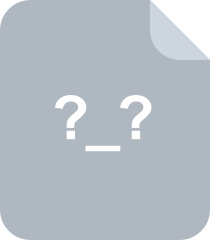
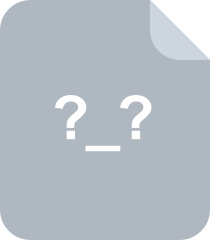
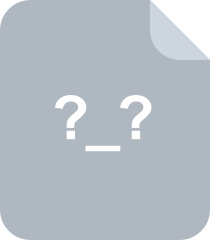
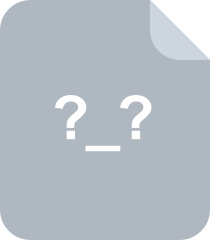
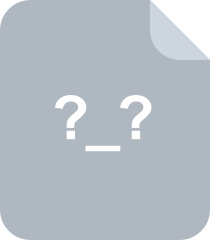
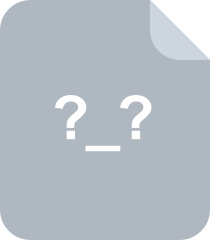
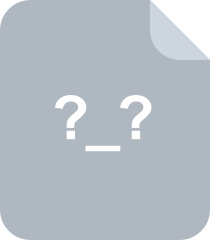
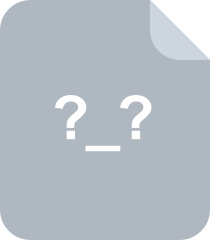
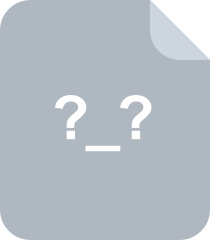
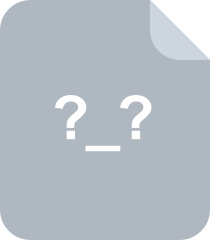
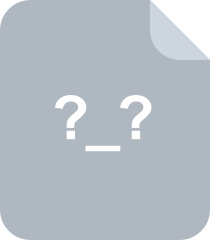
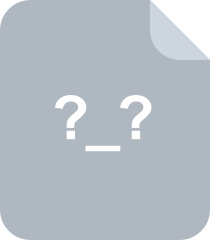
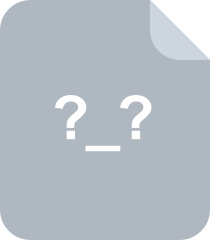
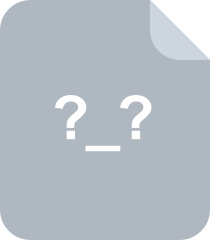
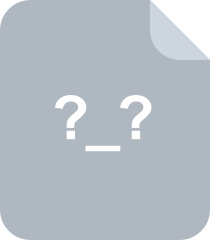
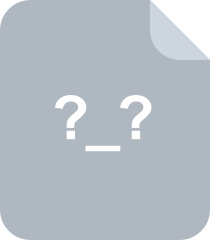
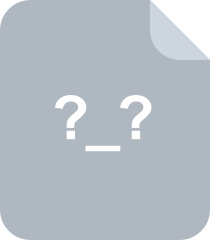
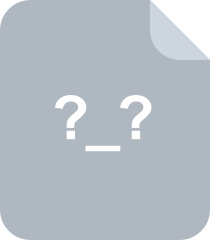
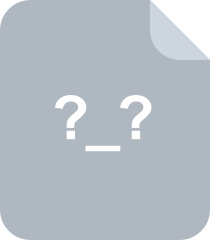
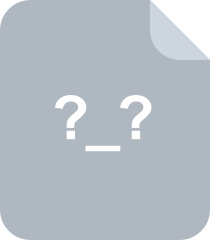
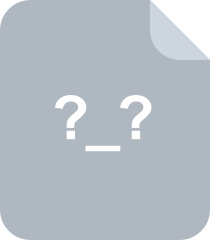
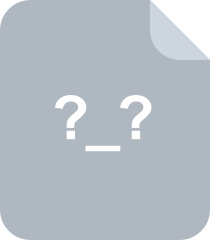
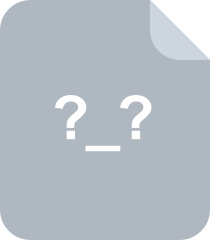
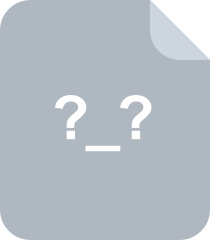
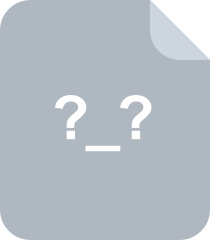
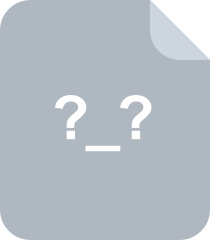
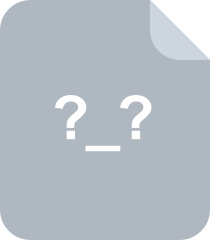
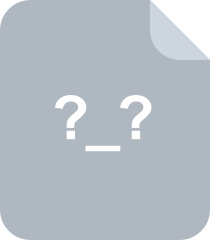
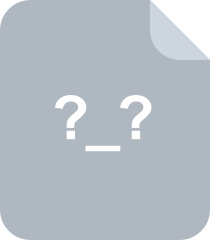
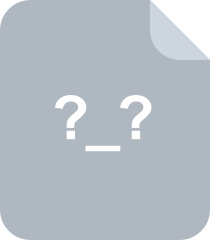
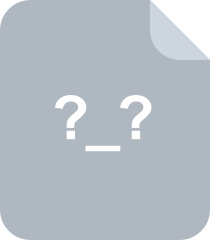
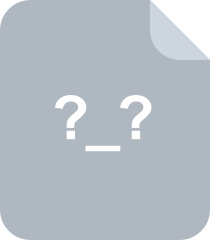
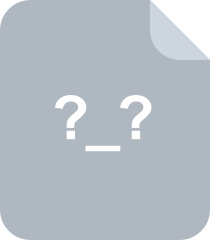
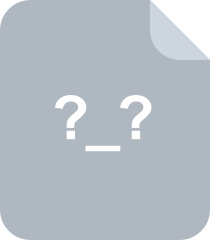
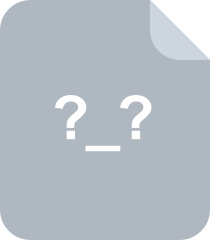
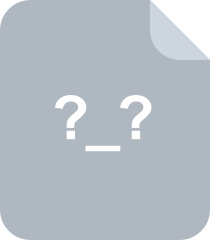
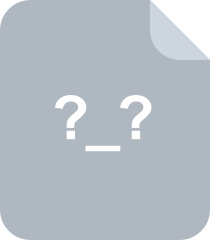
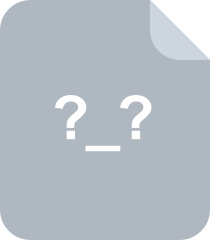
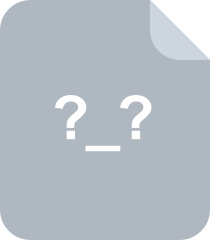
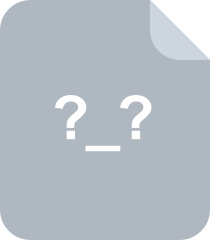
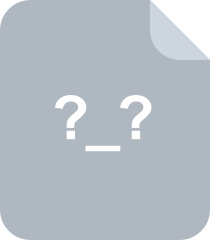
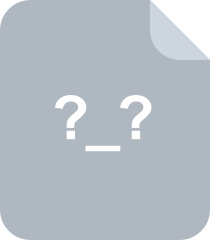
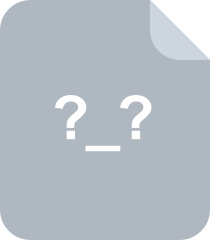
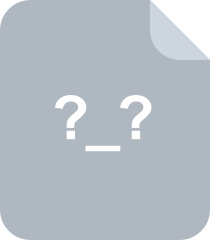
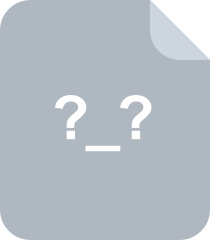
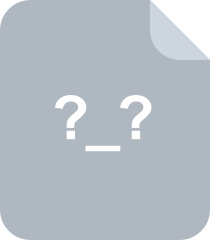
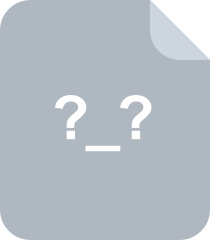
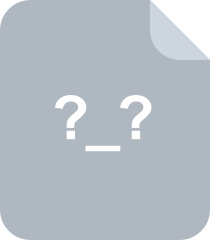
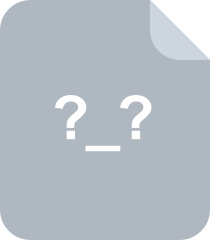
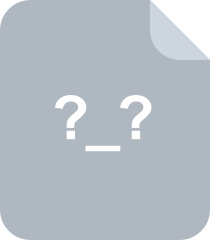
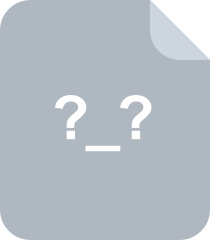
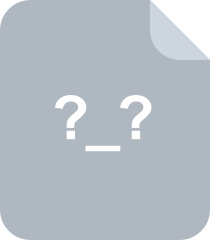
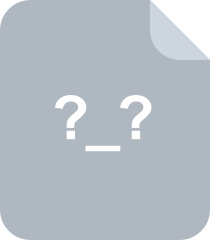
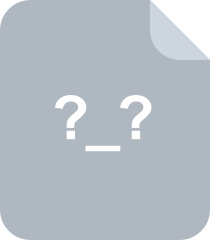
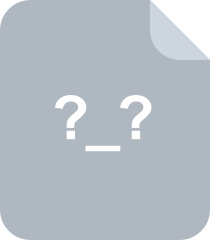
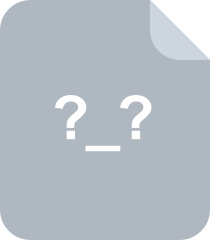
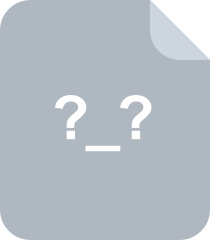
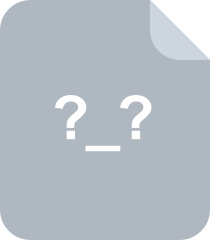
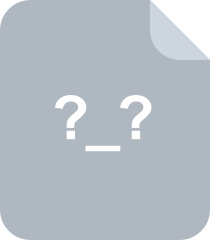
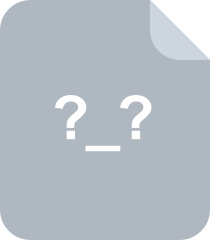
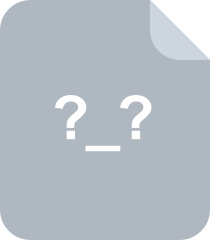
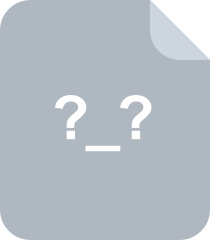
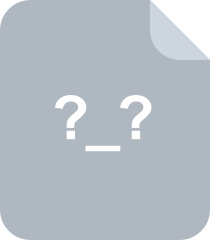
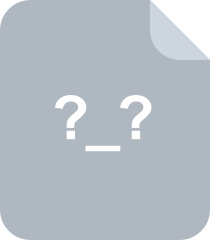
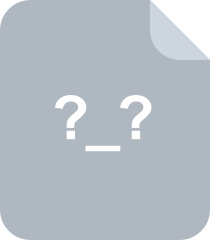
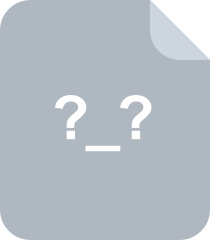
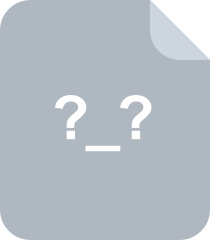
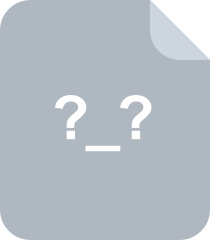
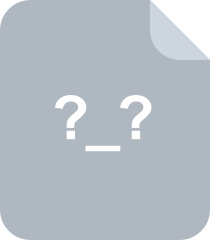
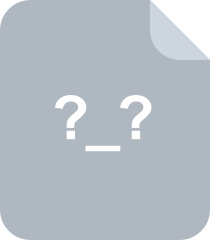
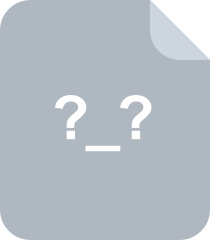
共 841 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
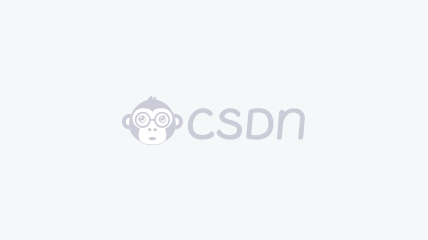

计算机学长阿伟
- 粉丝: 3198
- 资源: 848
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

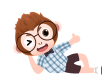
最新资源
- 荒地、农田、森林、湖、山姆、住宅检测11-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 基于epoll的reactor模型
- 人力资源领域人员简历模板docx文档
- 使用python基于CNN的10种水果识别-含1w张以上的数据集图片
- 基于Delaunay三角化的点云数据三维曲面重建matlab仿真,包括程序,中文注释,仿真操作步骤视频
- 船舶检测20-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 船舶检测19-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 华为ENSP基本配置!!!
- Java高级软件工程师简历模板-技能特长与项目经历
- 山东理工大学 SDUT 中外OS 操作系统 学习笔记 2024
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


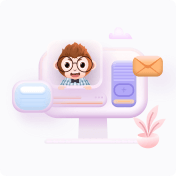
安全验证
文档复制为VIP权益,开通VIP直接复制
