package experiment4;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner;
public class VGroup {
private VNode[] VList = new VNode[12];//创建顶点结点
public void createAdjacencyList(){
for (int i = 0;i<12;i++){
VList[i] = new VNode();
}
VList[0].course = "CO1";
VList[0].credit = 2;
//co1是co2\3\4\12的先行课,创建四个边结点
VList[0].next = new EdgeNode(1,new EdgeNode(2,new EdgeNode(3,new EdgeNode(11,null))));
VList[1].course = "CO2";
VList[1].credit = 3;
VList[1].next = new EdgeNode(2,null);
VList[2].course = "CO3";
VList[2].credit = 4;
VList[2].next = new EdgeNode(4,new EdgeNode(6,new EdgeNode(7,null)));
VList[3].course = "CO4";
VList[3].credit = 3;
VList[3].next = new EdgeNode(4,null);
VList[4].course = "CO5";
VList[4].credit = 2;
VList[4].next = new EdgeNode(6,null);
VList[5].course = "CO6";
VList[5].credit = 3;
VList[5].next = new EdgeNode(7,null);
VList[6].course = "CO7";
VList[6].credit = 4;
VList[6].next = new EdgeNode();
VList[7].course = "CO8";
VList[7].credit = 4;
VList[7].next = new EdgeNode();
VList[8].course = "CO9";
VList[8].credit = 7;
VList[8].next = new EdgeNode(9,new EdgeNode(10,new EdgeNode(11,null)));
VList[9].course = "CO10";
VList[9].credit = 5;
VList[9].next = new EdgeNode(11,null);
VList[10].course = "CO11";
VList[10].credit = 2;
VList[10].next = new EdgeNode(5,new EdgeNode());
VList[11].course = "CO12";
VList[11].credit = 3;
VList[11].next = new EdgeNode();
for (int i = 0;i<VList.length;i++){
VList[i].inDegree = findInDegree()[i];
}
}
public int[] findInDegree(){//寻找入度
int[] inDegree = new int[VList.length];
for (int i = 0;i<inDegree.length;i++){
for (EdgeNode edge = VList[i].next;edge!=null;edge = edge.nextEdge){
if (edge.next != -1 ){
inDegree[edge.next]++;
}
}
}
return inDegree;
}
public void refreshList(EdgeNode edge){//更新邻接表
while (edge!=null){
if (edge.next != -1){
VList[edge.next].inDegree--;
}
edge = edge.nextEdge;
}
}
public void sortAdvanced() throws IOException {//排课尽早
Scanner sc = new Scanner(System.in);
for (int i = 0;i<VList.length;i++){
VList[i].inDegree = findInDegree()[i];
}
int creditLimit;
int count = 0;
System.out.println("请输入学期数:");
int termNum = sc.nextInt();
System.out.println("请输入一学期的学分上限:");
creditLimit = sc.nextInt();
LinkedList<VNode> queue = new LinkedList<>();
for (int j = 0;j<termNum;j++){
int credit = 0;
for (VNode a:VList){
if (a.flag&&a.inDegree==0){//改课程还未访问过同时入度为0即先行课为或已经上完
credit = credit + a.credit;
if (credit <= creditLimit ){
queue.offer(a);
a.flag = false;
count++;
String s = a.course + "学分为:" + a.credit + " 在第"+(j+1)+"学期";
BufferedWriter bw=null;
bw=new BufferedWriter(new FileWriter("course.txt",true));
bw.append(s+"\r\n");
bw.flush();
bw.close();
System.out.println(s);
}
}
}
while (!queue.isEmpty()){
refreshList(queue.poll().next);
}
}
if (count<VList.length){//是否有遗漏的课程
for (VNode a:VList){
if (a.flag){
System.out.println("课程"+a.course+"不存在");
}
}
}
System.out.println();
}
public void sortAverage() throws IOException {//排课平均
Scanner sc = new Scanner(System.in);
for (int i = 0;i<VList.length;i++){
VList[i].inDegree = findInDegree()[i];
}
int creditLimit;
int count = 0;
System.out.println("请输入学期数:");
int termNum = sc.nextInt();
System.out.println("请输入一学期的学分上限:");
creditLimit = sc.nextInt();
Queue<VNode> queue = new LinkedList<>();
int courseAverage;
if (VList.length%termNum == 0){
courseAverage = VList.length/termNum;//平均一学期排课数
}else {
courseAverage = VList.length / termNum + 1;
}
for (int j = 0;j<termNum;j++){
int credit = 0;
for (VNode a:VList){
if (a.flag&&a.inDegree==0){
credit = credit + a.credit;
if (credit <= creditLimit && queue.size()<courseAverage){
queue.offer(a);
a.flag = false;
count++;
String s = a.course + "学分为:" + a.credit + " 在第"+(j+1)+"学期";
BufferedWriter bw=null;
bw=new BufferedWriter(new FileWriter("course.txt",true));
bw.append(s+"\r\n");
bw.flush();
bw.close();
System.out.println(s);
}
}
}
while (!queue.isEmpty()){
refreshList(queue.poll().next);
}
}
if (count < VList.length){
for (VNode a: VList){
if (a.flag){
System.out.println("课程"+a.course+"不存在");
}
}
}
System.out.println();
}
}
Java数据结构课设选修课程安排
需积分: 11 153 浏览量
2021-03-08
13:32:06
上传
评论 3
收藏 5KB RAR 举报

ZemelWhitesell
- 粉丝: 4
- 资源: 11
最新资源
- 钢琴块2 v3.1.0.1054 Mod.apk
- 蓝桥杯第十一届省赛(第一场)
- 隐马尔可夫实践(生物序列)
- CLShanYanSDKDataList.sqlite
- 2024年度乐材教育春季高中数学教师专业测试答案.docx
- 选择题-数组&类I.docx
- cn-msdn-library-for-visual-studio-2008-service-pack-1-x86-dvd-x1
- cn-msdn-library-for-visual-studio-2008-service-pack-1-x86-dvd-x1
- cn-msdn-library-for-visual-studio-2008-service-pack-1-x86-dvd-x1
- Screenshot_20240517_181056.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


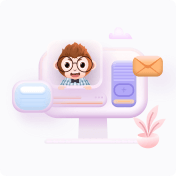
评论0