没有合适的资源?快使用搜索试试~ 我知道了~
Initialization - (Swift 5.2).pdf
需积分: 9 0 下载量 121 浏览量
2020-05-12
02:57:13
上传
评论
收藏 442KB PDF 举报
温馨提示
Initialization is the process of preparing an instance of a class, structure, or enumeration for use. This process involves setting an initial value for each stored property on that instance and performing any other setup or initialization that is required before the new instance is ready for use.
资源推荐
资源详情
资源评论
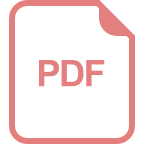
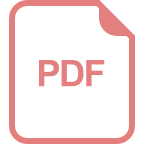
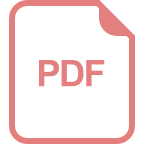
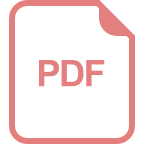
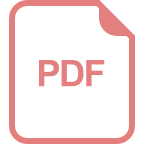
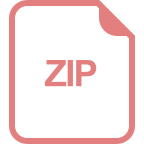
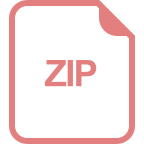
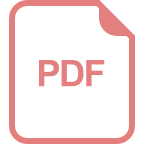
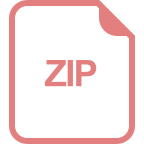
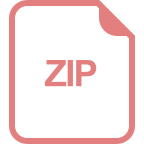
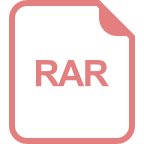
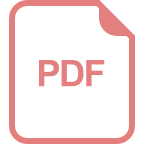
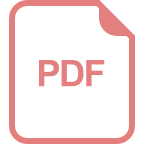
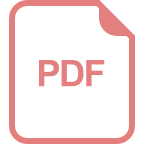
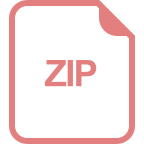
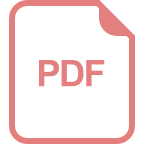
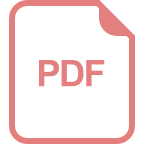
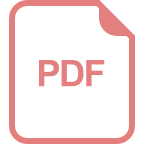
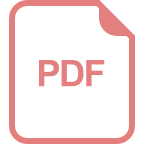
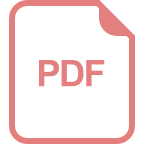
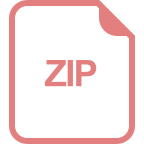
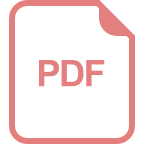
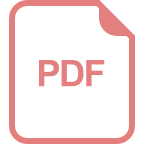
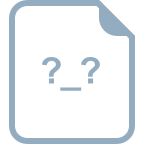
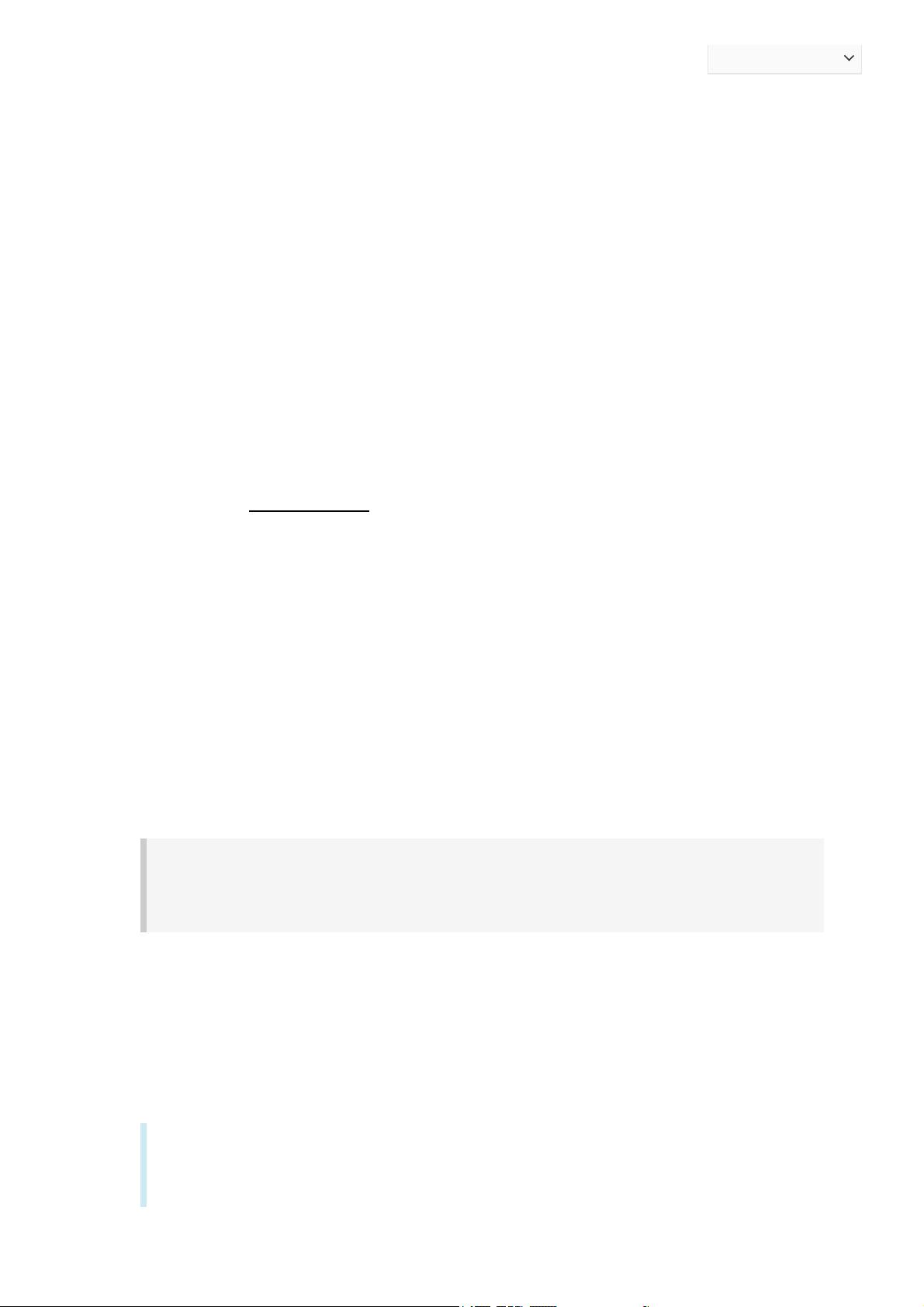
5/12/2020 Initialization — The Swift Programming Language (Swift 5.2)
https://docs.swift.org/swift-book/LanguageGuide/Initialization.html 2/35
Initialization
Initialization is the process of preparing an instance of a class, structure, or
enumeration for use. This process involves setting an initial value for each stored
property on that instance and performing any other setup or initialization that is required
before the new instance is ready for use.
You implement this initialization process by defining initializers, which are like special
methods that can be called to create a new instance of a particular type. Unlike
Objective-C initializers, Swift initializers do not return a value. Their primary role is to
ensure that new instances of a type are correctly initialized before they are used for the
first time.
Instances of class types can also implement a deinitializer, which performs any custom
cleanup just before an instance of that class is deallocated. For more information about
deinitializers, see Deinitialization.
Setting Initial Values for Stored Properties
Classes and structures must set all of their stored properties to an appropriate initial
value by the time an instance of that class or structure is created. Stored properties
cannot be left in an indeterminate state.
You can set an initial value for a stored property within an initializer, or by assigning a
default property value as part of the property’s definition. These actions are described
in the following sections.
N O T E
When you assign a default value to a stored property, or set its initial value within an
initializer, the value of that property is set directly, without calling any property observers.
Initializers
Initializers are called to create a new instance of a particular type. In its simplest form,
an initializer is like an instance method with no parameters, written using the init
keyword:
1 init() {
2 // perform some initialization here
3 }
ON THIS PAGE
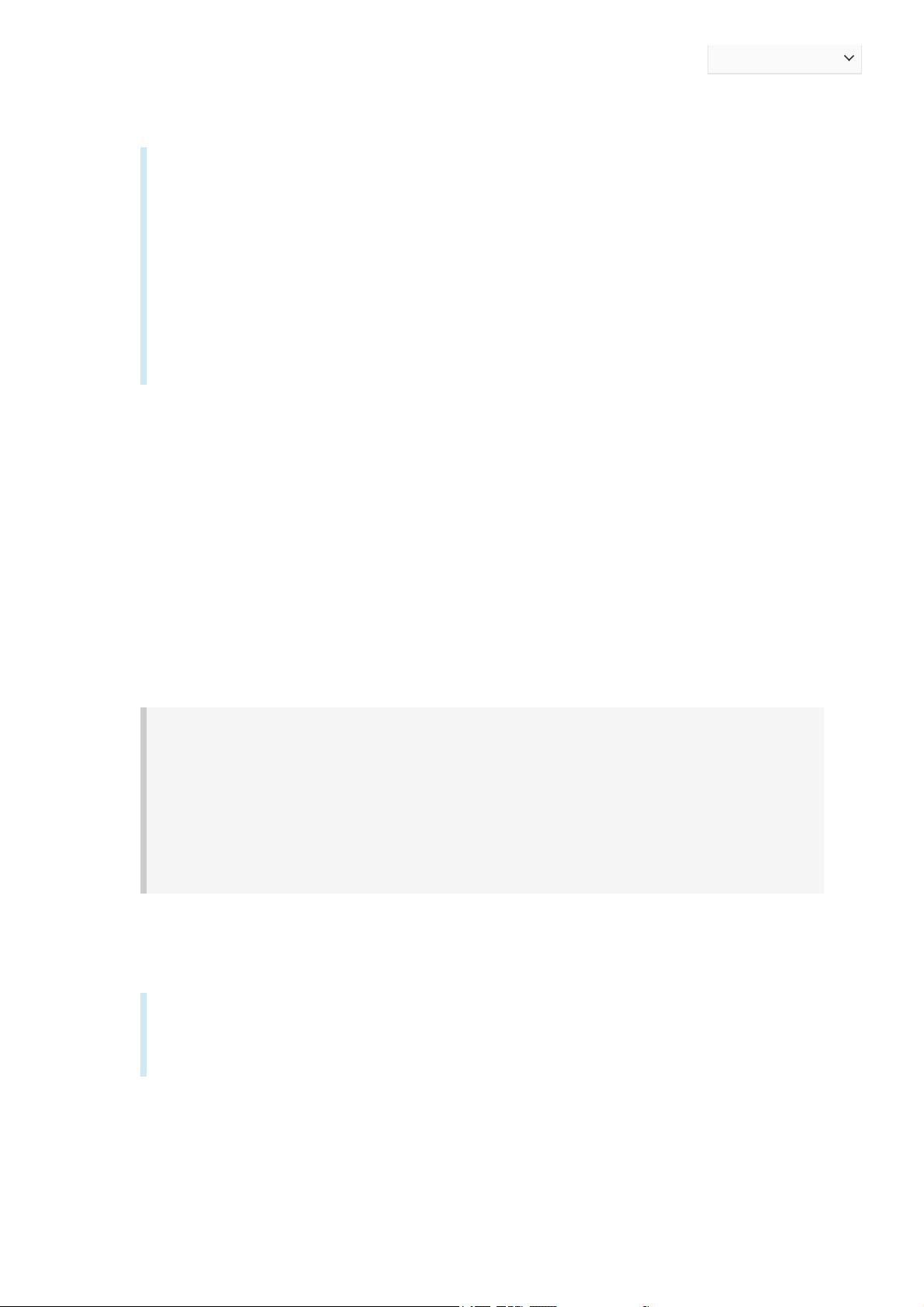
5/12/2020 Initialization — The Swift Programming Language (Swift 5.2)
https://docs.swift.org/swift-book/LanguageGuide/Initialization.html 3/35
The example below defines a new structure called Fahrenheit to store temperatures
expressed in the Fahrenheit scale. The Fahrenheit structure has one stored property,
temperature, which is of type Double:
1 struct Fahrenheit {
2 var temperature: Double
3 init() {
4 temperature = 32.0
5 }
6 }
7 var f = Fahrenheit()
8 print("The default temperature is \(f.temperature)° Fahrenheit")
9 // Prints "The default temperature is 32.0° Fahrenheit"
The structure defines a single initializer, init, with no parameters, which initializes the
stored temperature with a value of 32.0 (the freezing point of water in degrees
Fahrenheit).
Default Property Values
You can set the initial value of a stored property from within an initializer, as shown
above. Alternatively, specify a default property value as part of the property’s
declaration. You specify a default property value by assigning an initial value to the
property when it is defined.
N O T E
If a property always takes the same initial value, provide a default value rather than setting a
value within an initializer. The end result is the same, but the default value ties the property’s
initialization more closely to its declaration. It makes for shorter, clearer initializers and
enables you to infer the type of the property from its default value. The default value also
makes it easier for you to take advantage of default initializers and initializer inheritance, as
described later in this chapter.
You can write the Fahrenheit structure from above in a simpler form by providing a
default value for its temperature property at the point that the property is declared:
1 struct Fahrenheit {
2 var temperature = 32.0
3 }
Customizing Initialization
ON THIS PAGE
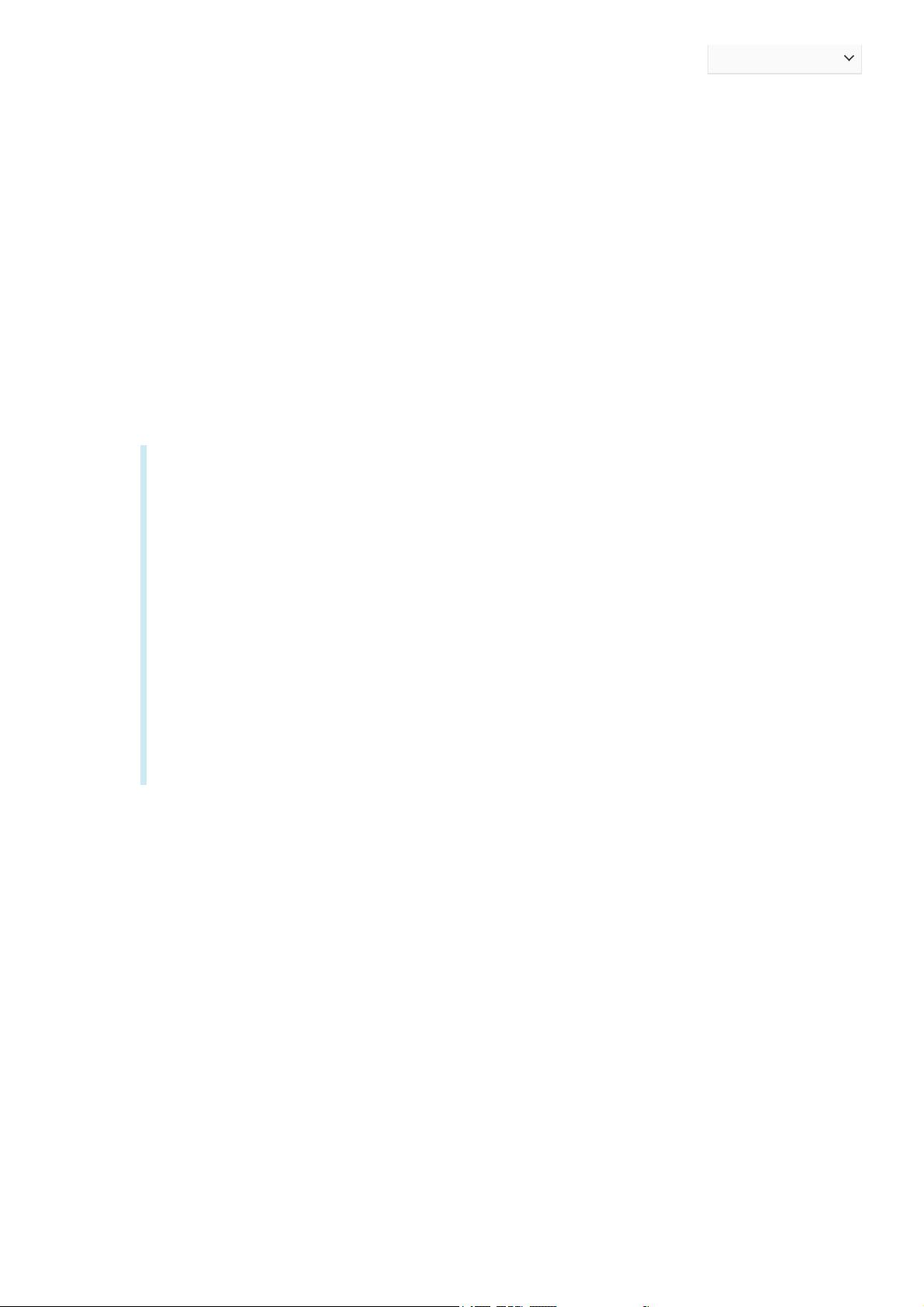
5/12/2020 Initialization — The Swift Programming Language (Swift 5.2)
https://docs.swift.org/swift-book/LanguageGuide/Initialization.html 4/35
You can customize the initialization process with input parameters and optional property
types, or by assigning constant properties during initialization, as described in the
following sections.
Initialization Parameters
You can provide initialization parameters as part of an initializer’s definition, to define
the types and names of values that customize the initialization process. Initialization
parameters have the same capabilities and syntax as function and method parameters.
The following example defines a structure called Celsius, which stores temperatures
expressed in degrees Celsius. The Celsius structure implements two custom initializers
called init(fromFahrenheit:) and init(fromKelvin:), which initialize a new instance
of the structure with a value from a different temperature scale:
1 struct Celsius {
2 var temperatureInCelsius: Double
3 init(fromFahrenheit fahrenheit: Double) {
4 temperatureInCelsius = (fahrenheit - 32.0) / 1.8
5 }
6 init(fromKelvin kelvin: Double) {
7 temperatureInCelsius = kelvin - 273.15
8 }
9 }
10 let boilingPointOfWater = Celsius(fromFahrenheit: 212.0)
11 // boilingPointOfWater.temperatureInCelsius is 100.0
12 let freezingPointOfWater = Celsius(fromKelvin: 273.15)
13 // freezingPointOfWater.temperatureInCelsius is 0.0
The first initializer has a single initialization parameter with an argument label of
fromFahrenheit and a parameter name of fahrenheit. The second initializer has a
single initialization parameter with an argument label of fromKelvin and a parameter
name of kelvin. Both initializers convert their single argument into the corresponding
Celsius value and store this value in a property called temperatureInCelsius.
Parameter Names and Argument Labels
As with function and method parameters, initialization parameters can have both a
parameter name for use within the initializer’s body and an argument label for use when
calling the initializer.
ON THIS PAGE
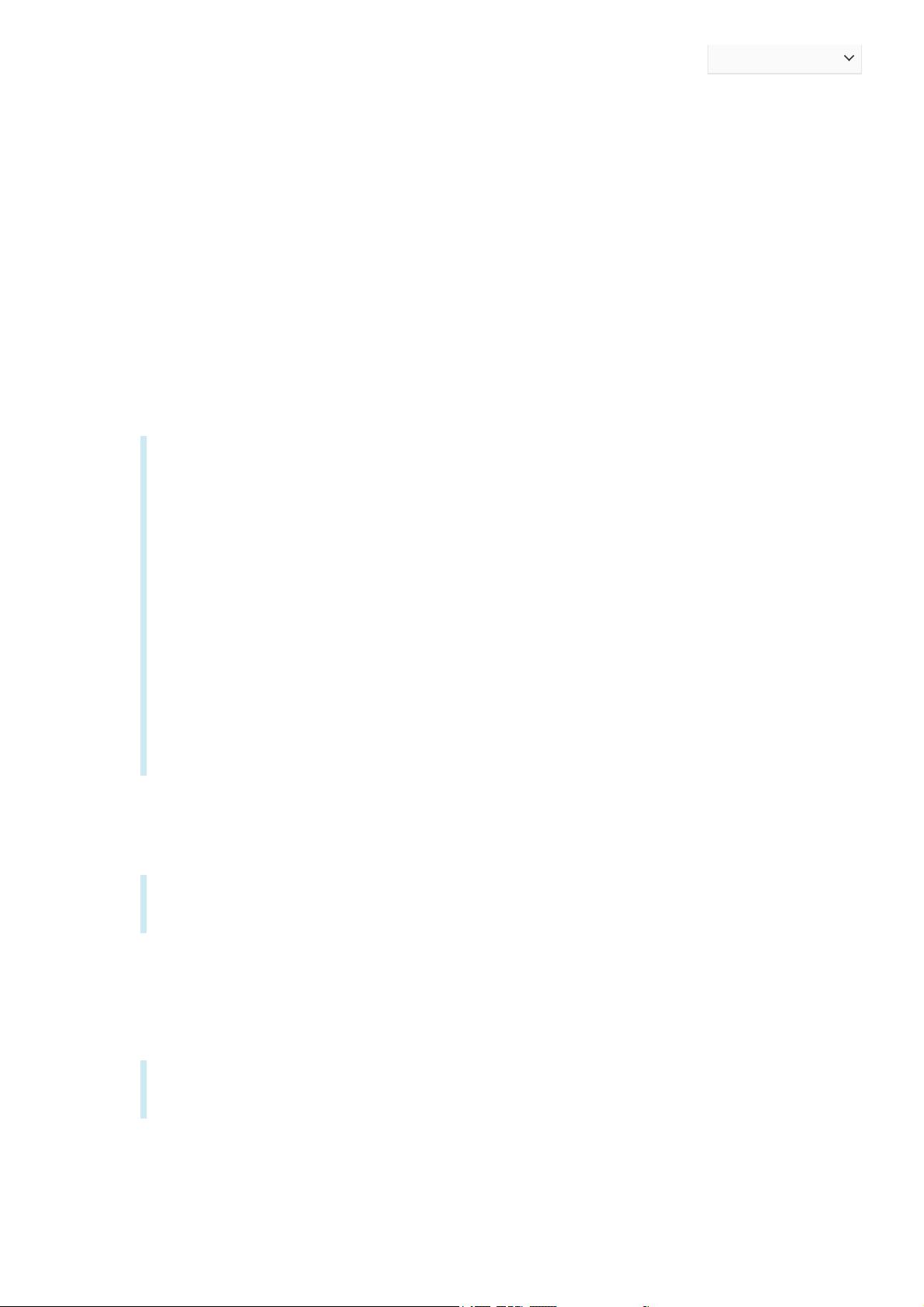
5/12/2020 Initialization — The Swift Programming Language (Swift 5.2)
https://docs.swift.org/swift-book/LanguageGuide/Initialization.html 5/35
However, initializers do not have an identifying function name before their parentheses
in the way that functions and methods do. Therefore, the names and types of an
initializer’s parameters play a particularly important role in identifying which initializer
should be called. Because of this, Swift provides an automatic argument label for every
parameter in an initializer if you don’t provide one.
The following example defines a structure called Color, with three constant properties
called red, green, and blue. These properties store a value between 0.0 and 1.0 to
indicate the amount of red, green, and blue in the color.
Color provides an initializer with three appropriately named parameters of type Double
for its red, green, and blue components. Color also provides a second initializer with a
single white parameter, which is used to provide the same value for all three color
components.
1 struct Color {
2 let red, green, blue: Double
3 init(red: Double, green: Double, blue: Double) {
4 self.red = red
5 self.green = green
6 self.blue = blue
7 }
8 init(white: Double) {
9 red = white
10 green = white
11 blue = white
12 }
13 }
Both initializers can be used to create a new Color instance, by providing named values
for each initializer parameter:
1 let magenta = Color(red: 1.0, green: 0.0, blue: 1.0)
2 let halfGray = Color(white: 0.5)
Note that it is not possible to call these initializers without using argument labels.
Argument labels must always be used in an initializer if they are defined, and omitting
them is a compile-time error:
1 let veryGreen = Color(0.0, 1.0, 0.0)
2 // this reports a compile-time error - argument labels are required
Initializer Parameters Without Argument Labels
ON THIS PAGE
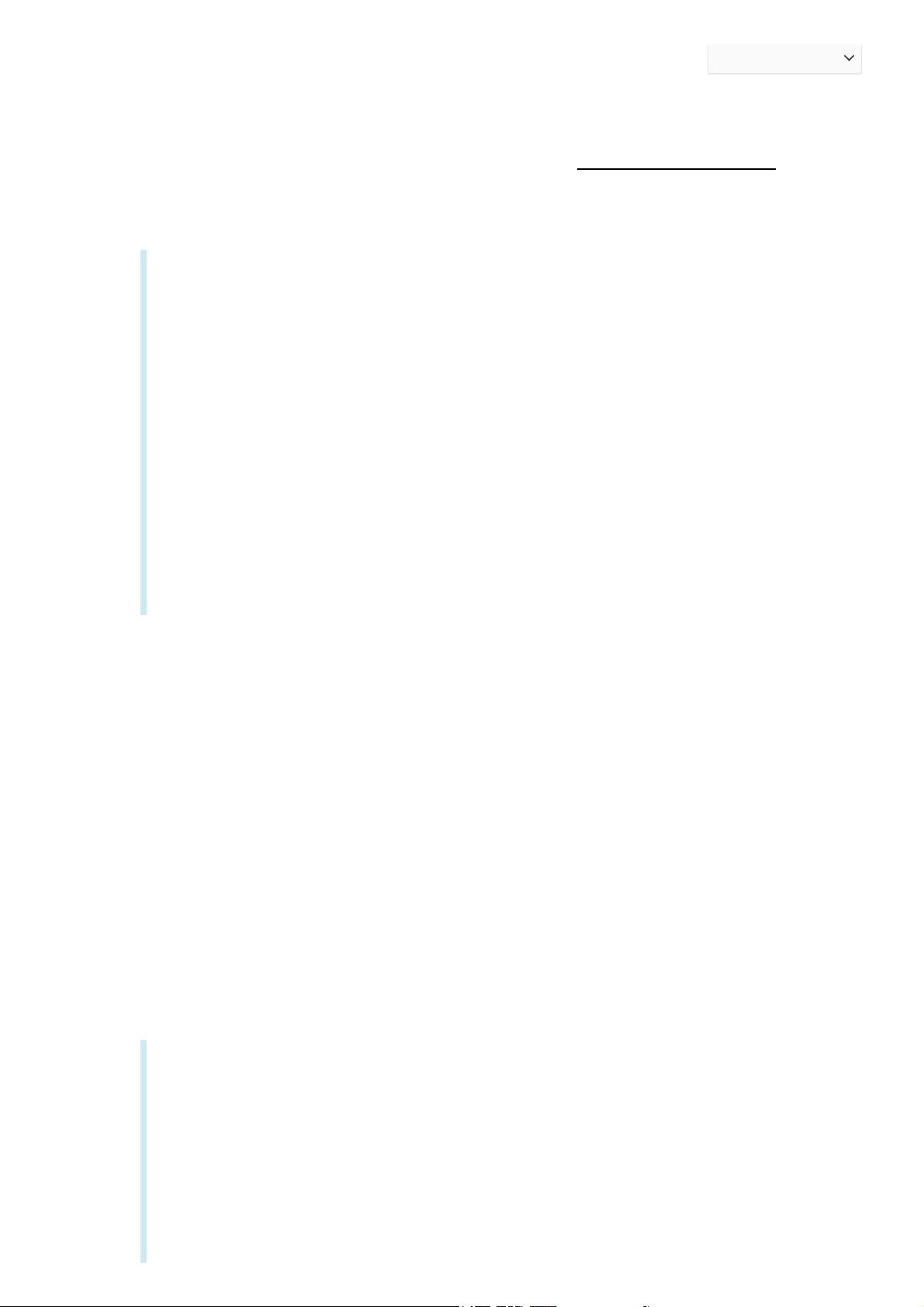
5/12/2020 Initialization — The Swift Programming Language (Swift 5.2)
https://docs.swift.org/swift-book/LanguageGuide/Initialization.html 6/35
If you do not want to use an argument label for an initializer parameter, write an
underscore (_) instead of an explicit argument label for that parameter to override the
default behavior.
Here’s an expanded version of the Celsius example from Initialization Parameters
above, with an additional initializer to create a new Celsius instance from a Double
value that is already in the Celsius scale:
1 struct Celsius {
2 var temperatureInCelsius: Double
3 init(fromFahrenheit fahrenheit: Double) {
4 temperatureInCelsius = (fahrenheit - 32.0) / 1.8
5 }
6 init(fromKelvin kelvin: Double) {
7 temperatureInCelsius = kelvin - 273.15
8 }
9 init(_ celsius: Double) {
10 temperatureInCelsius = celsius
11 }
12 }
13 let bodyTemperature = Celsius(37.0)
14 // bodyTemperature.temperatureInCelsius is 37.0
The initializer call Celsius(37.0) is clear in its intent without the need for an argument
label. It is therefore appropriate to write this initializer as init(_ celsius: Double) so
that it can be called by providing an unnamed Double value.
Optional Property Types
If your custom type has a stored property that is logically allowed to have “no value”—
perhaps because its value cannot be set during initialization, or because it is allowed to
have “no value” at some later point—declare the property with an optional type.
Properties of optional type are automatically initialized with a value of nil, indicating
that the property is deliberately intended to have “no value yet” during initialization.
The following example defines a class called SurveyQuestion, with an optional String
property called response:
1 class SurveyQuestion {
2 var text: String
3 var response: String?
4 init(text: String) {
5 self.text = text
6 }
7 func ask() {
8 print(text)
ON THIS PAGE
剩余33页未读,继续阅读
资源评论
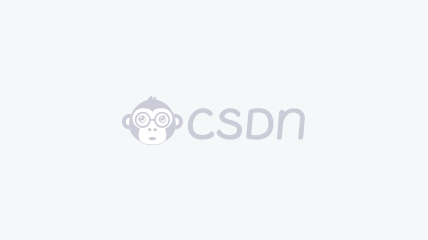

码海前行的猪
- 粉丝: 28
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

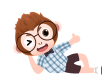
最新资源
- 【IT教程网】18.第5章网络_SSH.wmv
- 【IT教程网】D11_04_创建GUI_使用Django_1.wmv
- 【IT教程网】D08_04_操作系统_PyInotify.wmv
- 文件写入失败异常解决办法.md
- 【IT教程网】11.第3章文本_ElementTree.wmv
- 【IT教程网】D11_06_创建GUI_使用Django_3.wmv
- 【IT教程网】D12_02_数据持久性_简单序列化_shelve.wmv
- 【IT教程网】D12_03_数据持久性_简单序列化_YAML.wmv
- 【IT教程网】29.第8章操作系统什锦_Python中跨平台的UNIX编辑(三).wmv
- 【IT教程网】23.第6章数据(四)_合并数据.wmv
- 【IT教程网】D12_01_数据持久性_简单序列化_Pickle.wmv
- 【IT教程网】D12_06_数据持久性_关系序列化_SQLAlchemy.wmv
- 【IT教程网】6.第3章文本_Python字符串及内建功能函数.wmv
- wireshark4.2版本,s7 comm plus插件
- 【IT教程网】D12_05_数据持久性_关系序列化_StormORM.wmv
- 【IT教程网】D12_04_数据持久性_简单序列化_ZODB.wmv
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


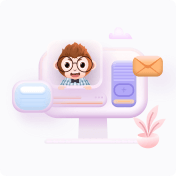
安全验证
文档复制为VIP权益,开通VIP直接复制
