package net.jjjshop.common.util.excel;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.apache.poi.hssf.usermodel.HSSFDataValidation;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.usermodel.ClientAnchor.AnchorType;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.poi.ss.util.CellRangeAddressList;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import org.apache.poi.xssf.usermodel.XSSFClientAnchor;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.lang.reflect.Field;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.net.URL;
import java.text.NumberFormat;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.Map.Entry;
import java.util.regex.Pattern;
/**
* Excel导入导出工具类
*
*/
@SuppressWarnings("unused")
public class ExcelUtils {
private static final String XLSX = ".xlsx";
private static final String XLS = ".xls";
public static final String ROW_MERGE = "row_merge";
public static final String COLUMN_MERGE = "column_merge";
private static final String DATE_FORMAT = "yyyy-MM-dd HH:mm:ss";
private static final String ROW_NUM = "rowNum";
private static final String ROW_DATA = "rowData";
private static final String ROW_TIPS = "rowTips";
private static final int CELL_OTHER = 0;
private static final int CELL_ROW_MERGE = 1;
private static final int CELL_COLUMN_MERGE = 2;
private static final int IMG_HEIGHT = 30;
private static final int IMG_WIDTH = 30;
private static final char LEAN_LINE = '/';
private static final int BYTES_DEFAULT_LENGTH = 10240;
private static final NumberFormat NUMBER_FORMAT = NumberFormat.getNumberInstance();
public static <T> List<T> readFile(File file, Class<T> clazz) throws Exception {
JSONArray array = readFile(file);
return getBeanList(array, clazz);
}
public static <T> List<T> readMultipartFile(MultipartFile mFile, Class<T> clazz) throws Exception {
JSONArray array = readMultipartFile(mFile);
return getBeanList(array, clazz);
}
public static JSONArray readFile(File file) throws Exception {
return readExcel(null, file);
}
public static JSONArray readMultipartFile(MultipartFile mFile) throws Exception {
return readExcel(mFile, null);
}
public static Map<String, JSONArray> readFileManySheet(File file) throws Exception {
return readExcelManySheet(null, file);
}
public static Map<String, JSONArray> readFileManySheet(MultipartFile file) throws Exception {
return readExcelManySheet(file, null);
}
private static <T> List<T> getBeanList(JSONArray array, Class<T> clazz) throws Exception {
List<T> list = new ArrayList<>();
Map<Integer, String> uniqueMap = new HashMap<>(16);
for (int i = 0; i < array.size(); i++) {
list.add(getBean(clazz, array.getJSONObject(i), uniqueMap));
}
return list;
}
/**
* 获取每个对象的数据
*/
private static <T> T getBean(Class<T> c, JSONObject obj, Map<Integer, String> uniqueMap) throws Exception {
T t = c.newInstance();
Field[] fields = c.getDeclaredFields();
List<String> errMsgList = new ArrayList<>();
boolean hasRowTipsField = false;
StringBuilder uniqueBuilder = new StringBuilder();
int rowNum = 0;
for (Field field : fields) {
// 行号
if (field.getName().equals(ROW_NUM)) {
rowNum = obj.getInteger(ROW_NUM);
field.setAccessible(true);
field.set(t, rowNum);
continue;
}
// 是否需要设置异常信息
if (field.getName().equals(ROW_TIPS)) {
hasRowTipsField = true;
continue;
}
// 原始数据
if (field.getName().equals(ROW_DATA)) {
field.setAccessible(true);
field.set(t, obj.toString());
continue;
}
// 设置对应属性值
setFieldValue(t, field, obj, uniqueBuilder, errMsgList);
}
// 数据唯一性校验
if (uniqueBuilder.length() > 0) {
if (uniqueMap.containsValue(uniqueBuilder.toString())) {
Set<Integer> rowNumKeys = uniqueMap.keySet();
for (Integer num : rowNumKeys) {
if (uniqueMap.get(num).equals(uniqueBuilder.toString())) {
errMsgList.add(String.format("数据唯一性校验失败,(%s)与第%s行重复)", uniqueBuilder, num));
}
}
} else {
uniqueMap.put(rowNum, uniqueBuilder.toString());
}
}
// 失败处理
if (errMsgList.isEmpty() && !hasRowTipsField) {
return t;
}
StringBuilder sb = new StringBuilder();
int size = errMsgList.size();
for (int i = 0; i < size; i++) {
if (i == size - 1) {
sb.append(errMsgList.get(i));
} else {
sb.append(errMsgList.get(i)).append(";");
}
}
// 设置错误信息
for (Field field : fields) {
if (field.getName().equals(ROW_TIPS)) {
field.setAccessible(true);
field.set(t, sb.toString());
}
}
return t;
}
private static <T> void setFieldValue(T t, Field field, JSONObject obj, StringBuilder uniqueBuilder, List<String> errMsgList) {
// 获取 ExcelImport 注解属性
ExcelImport annotation = field.getAnnotation(ExcelImport.class);
if (annotation == null) {
return;
}
String cname = annotation.value();
if (cname.trim().length() == 0) {
return;
}
// 获取具体值
String val = null;
if (obj.containsKey(cname)) {
val = getString(obj.getString(cname));
}
if (val == null) {
return;
}
field.setAccessible(true);
// 判断是否必填
boolean require = annotation.required();
if (require && val.isEmpty()) {
errMsgList.add(String.format("[%s]不能为空", cname));
return;
}
//判断是否有空格或特殊符号
// String regEx = ".*[\\s`~!#$%^&*()+=|{}':;',\\[\\].<>/?~!@#¥%……&*()——+|{}【】‘;:”“’。,、?\\\\]+.*";
// Pattern p = Pattern.compile(regEx);
// if(p.matcher(val).matches()){
// errMsgList.add(String.format("[%s]有空格或特殊符号", cname));
// return;
// }
// 数据唯一性获取
boolean unique = annotation.unique();
if (unique) {
if (uniqueBuilder.length() > 0) {
uniqueBuilder.append("--").append(val);
} else {
uniqueBuilder.append(val);
}
}
// 判断是否超过最大长度
int maxLength = annotation.maxLength();
if (maxLength > 0 && val.length() > maxLength) {
errMsgList.add(String.format("[%s]长度不能超过%s个字符(当前%s个字符)", cname, maxLength, val.length()));
}
// 判断当前属性是否有映射关系
LinkedHashMap<String, String> kvMap = getKvMap(annotation.kv());
if (!kvMap.isEmpty()) {
boolean isMatch = false;
for (String key : kvMap.keySet()) {
if (kvMap.get(key).equals(val)) {
val = key;
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Spring Boot和Vue 3的校园及门店餐饮点餐系统设计源码,该项目包含3639个文件,主要文件类型有1158个javascript文件,893个java源文件,以及475个png图像文件。此外,还包括282个vue前端文件,162个typescript文件,以及139个html页面文件。该项目是一个基于Spring Boot和Vue 3的校园及门店餐饮点餐系统设计源码,可能涉及用户界面设计、应用逻辑实现、数据存储等多个方面。
资源推荐
资源详情
资源评论
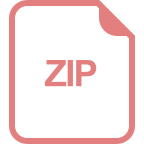
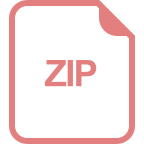
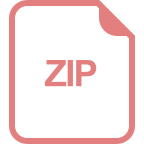
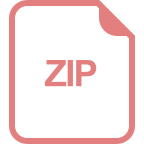
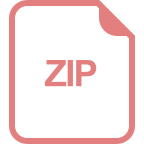
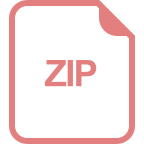
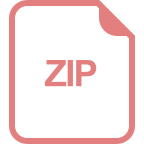
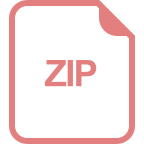
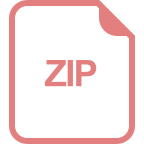
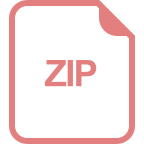
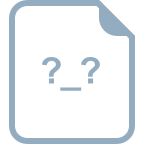
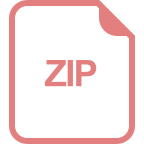
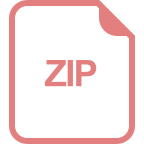
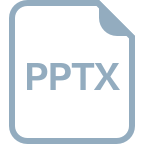
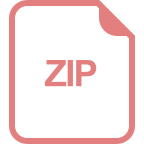
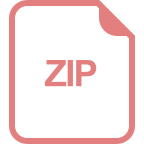
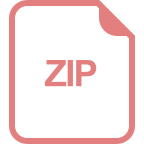
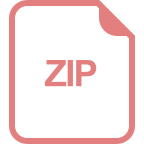
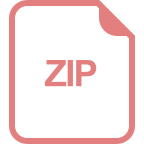
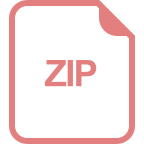
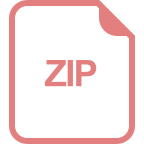
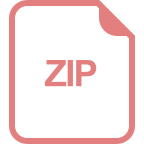
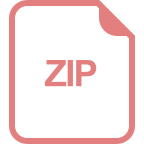
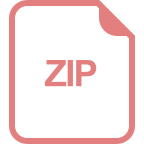
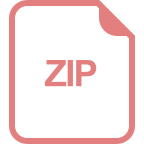
收起资源包目录

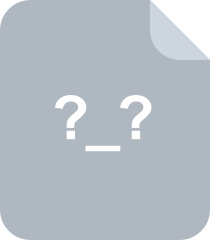
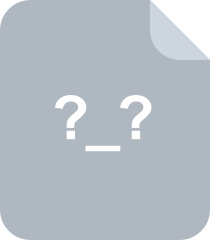
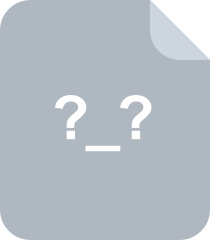
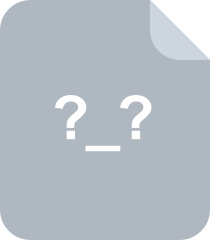
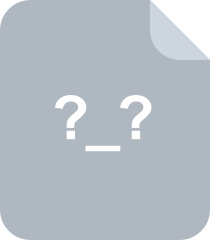
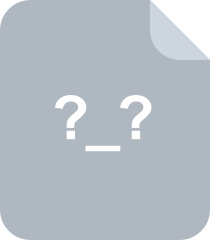
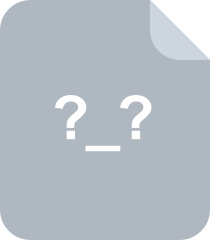
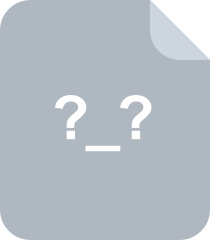
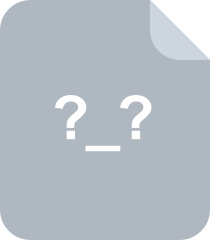
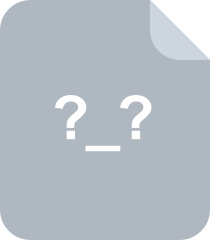
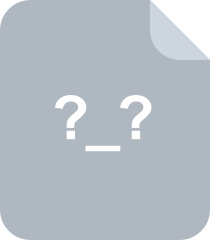
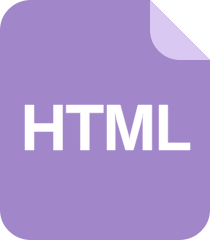
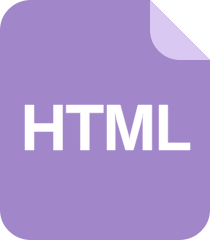
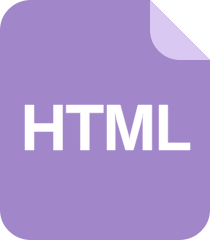
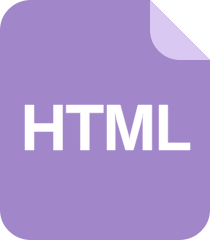
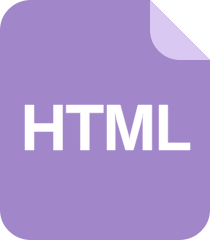
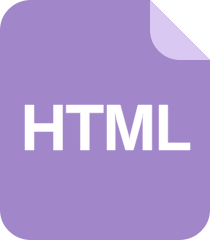
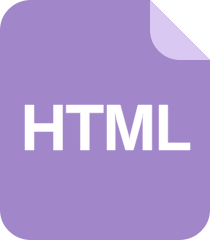
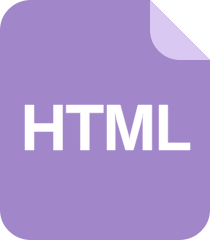
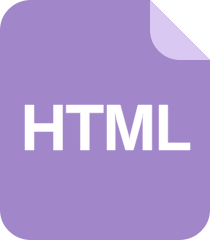
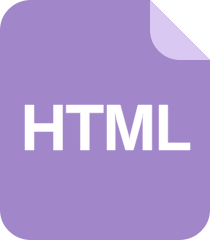
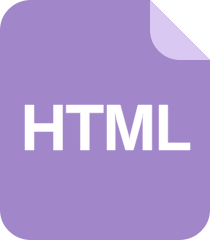
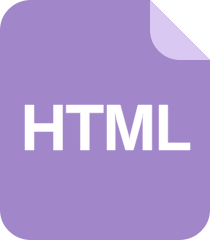
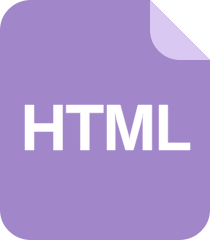
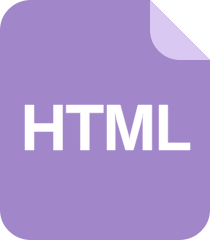
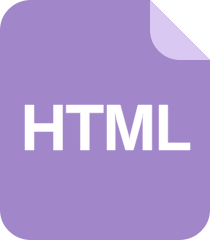
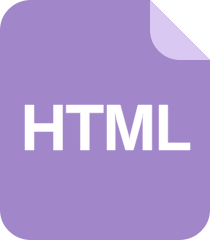
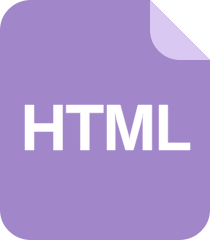
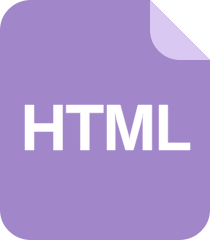
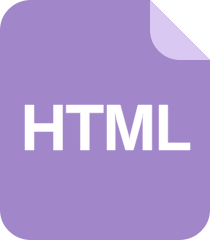
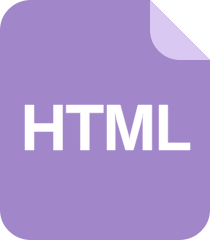
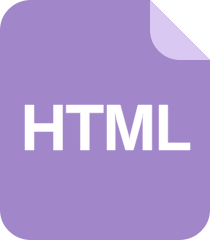
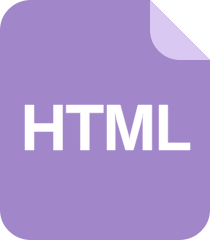
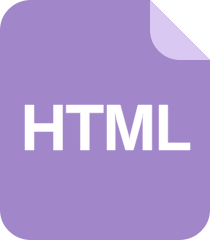
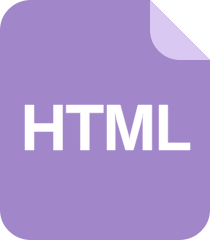
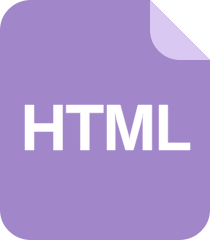
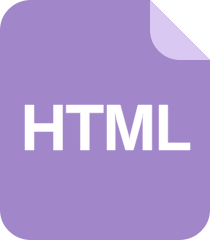
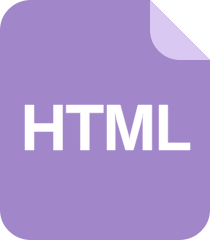
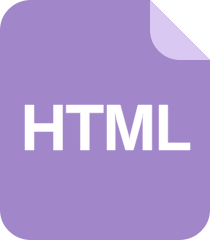
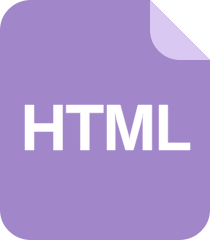
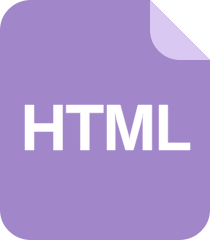
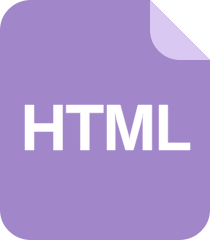
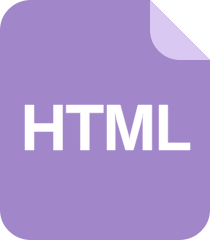
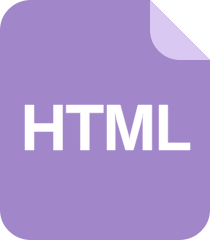
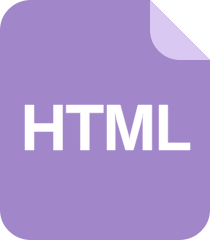
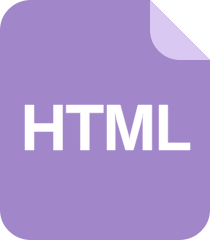
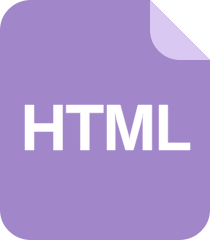
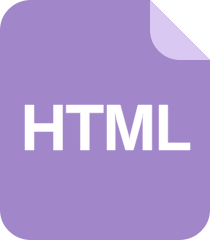
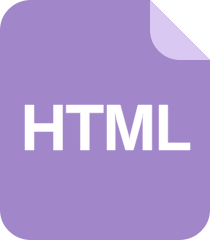
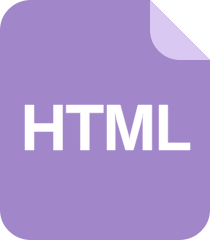
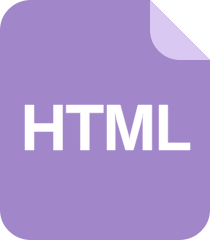
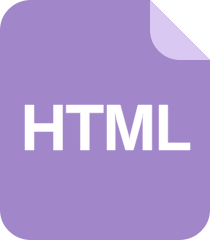
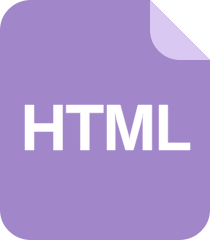
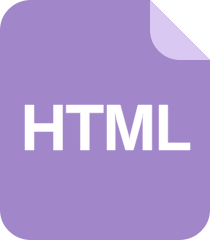
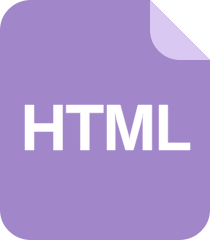
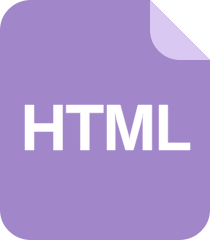
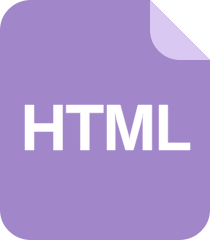
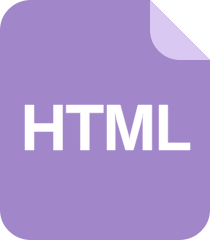
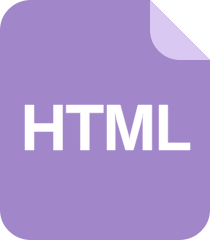
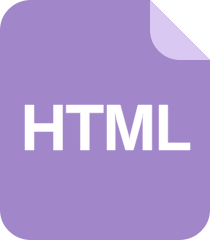
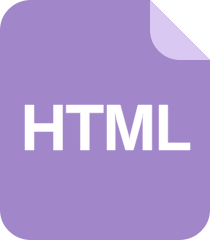
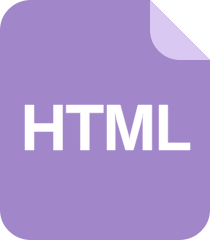
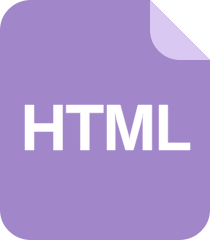
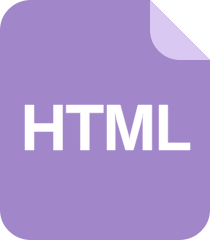
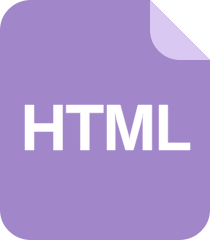
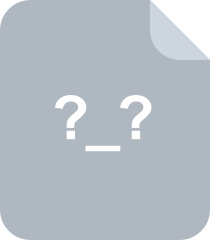
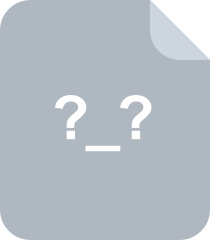
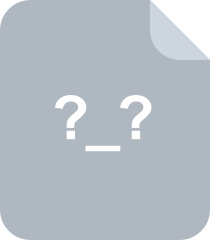
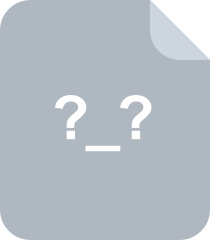
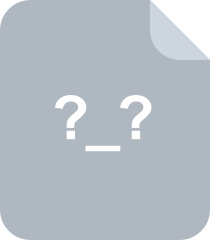
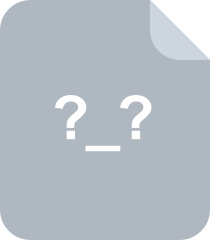
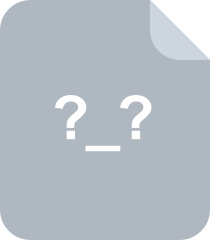
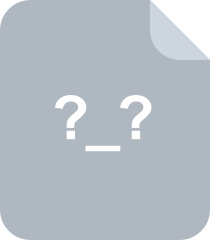
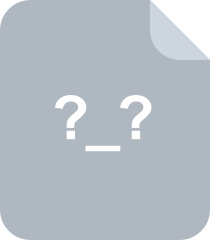
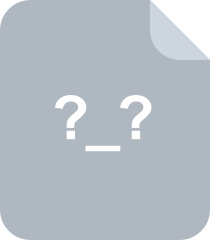
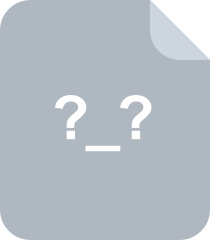
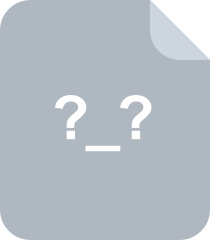
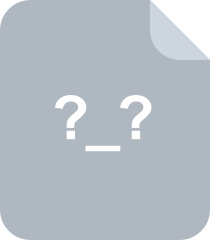
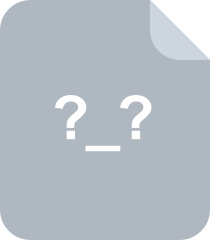
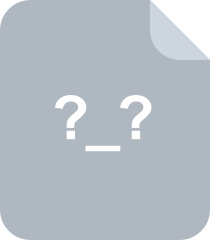
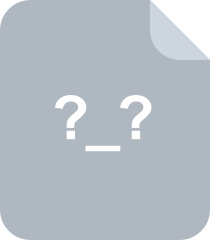
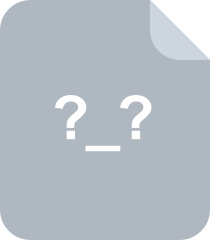
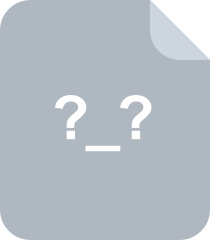
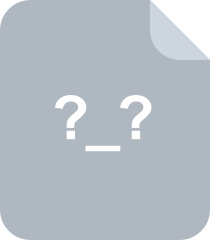
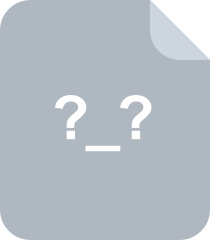
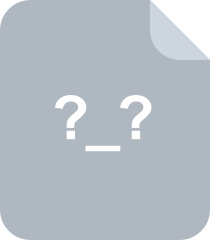
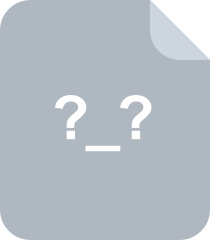
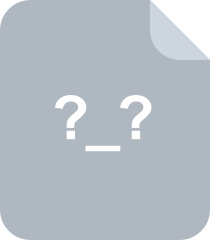
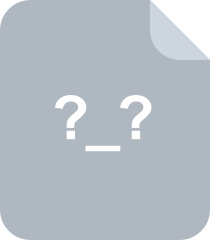
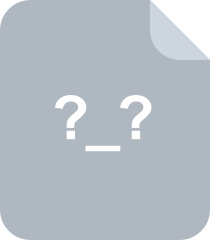
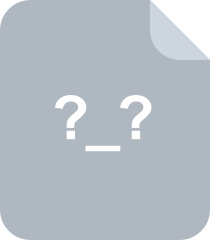
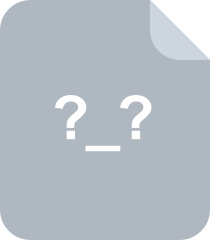
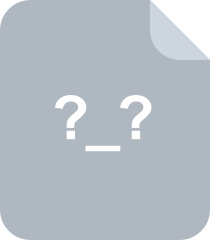
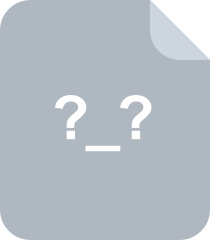
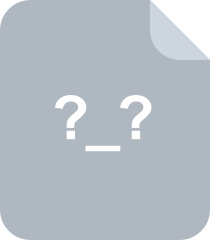
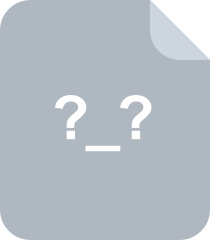
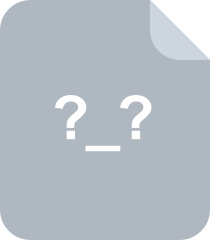
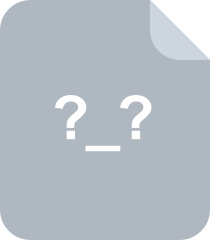
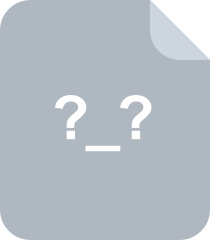
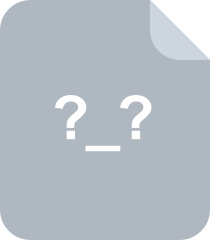
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
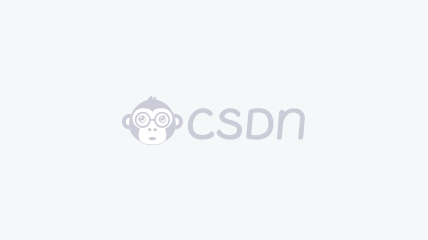

沐知全栈开发
- 粉丝: 5811
- 资源: 5227
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

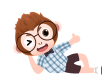
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


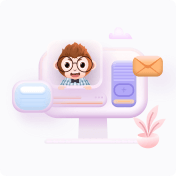
安全验证
文档复制为VIP权益,开通VIP直接复制
