!function (e, t) { "object" == typeof exports && "object" == typeof module ? module.exports = t() : "function" == typeof define && define.amd ? define([], t) : "object" == typeof exports ? exports.flvjs = t() : e.flvjs = t() }(self, (function () {
return function () {
var e = {
264: function (e, t, i) {
/*!
* @overview es6-promise - a tiny implementation of Promises/A+.
* @copyright Copyright (c) 2014 Yehuda Katz, Tom Dale, Stefan Penner and contributors (Conversion to ES6 API by Jake Archibald)
* @license Licensed under MIT license
* See https://raw.githubusercontent.com/stefanpenner/es6-promise/master/LICENSE
* @version v4.2.8+1e68dce6
*/
e.exports = function () { "use strict"; function e(e) { var t = typeof e; return null !== e && ("object" === t || "function" === t) } function t(e) { return "function" == typeof e } var n = Array.isArray ? Array.isArray : function (e) { return "[object Array]" === Object.prototype.toString.call(e) }, r = 0, s = void 0, o = void 0, a = function (e, t) { b[r] = e, b[r + 1] = t, 2 === (r += 2) && (o ? o(E) : A()) }; function h(e) { o = e } function u(e) { a = e } var l = "undefined" != typeof window ? window : void 0, d = l || {}, c = d.MutationObserver || d.WebKitMutationObserver, f = "undefined" == typeof self && "undefined" != typeof process && "[object process]" === {}.toString.call(process), _ = "undefined" != typeof Uint8ClampedArray && "undefined" != typeof importScripts && "undefined" != typeof MessageChannel; function p() { return function () { return process.nextTick(E) } } function m() { return void 0 !== s ? function () { s(E) } : y() } function g() { var e = 0, t = new c(E), i = document.createTextNode(""); return t.observe(i, { characterData: !0 }), function () { i.data = e = ++e % 2 } } function v() { var e = new MessageChannel; return e.port1.onmessage = E, function () { return e.port2.postMessage(0) } } function y() { var e = setTimeout; return function () { return e(E, 1) } } var b = new Array(1e3); function E() { for (var e = 0; e < r; e += 2)(0, b[e])(b[e + 1]), b[e] = void 0, b[e + 1] = void 0; r = 0 } function S() { try { var e = Function("return this")().require("vertx"); return s = e.runOnLoop || e.runOnContext, m() } catch (e) { return y() } } var A = void 0; function L(e, t) { var i = this, n = new this.constructor(O); void 0 === n[w] && W(n); var r = i._state; if (r) { var s = arguments[r - 1]; a((function () { return V(r, n, s, i._result) })) } else G(i, n, e, t); return n } function R(e) { var t = this; if (e && "object" == typeof e && e.constructor === t) return e; var i = new t(O); return U(i, e), i } A = f ? p() : c ? g() : _ ? v() : void 0 === l ? S() : y(); var w = Math.random().toString(36).substring(2); function O() { } var T = void 0, C = 1, k = 2; function D() { return new TypeError("You cannot resolve a promise with itself") } function I() { return new TypeError("A promises callback cannot return that same promise.") } function M(e, t, i, n) { try { e.call(t, i, n) } catch (e) { return e } } function B(e, t, i) { a((function (e) { var n = !1, r = M(i, t, (function (i) { n || (n = !0, t !== i ? U(e, i) : Z(e, i)) }), (function (t) { n || (n = !0, F(e, t)) }), "Settle: " + (e._label || " unknown promise")); !n && r && (n = !0, F(e, r)) }), e) } function x(e, t) { t._state === C ? Z(e, t._result) : t._state === k ? F(e, t._result) : G(t, void 0, (function (t) { return U(e, t) }), (function (t) { return F(e, t) })) } function P(e, i, n) { i.constructor === e.constructor && n === L && i.constructor.resolve === R ? x(e, i) : void 0 === n ? Z(e, i) : t(n) ? B(e, i, n) : Z(e, i) } function U(t, i) { if (t === i) F(t, D()); else if (e(i)) { var n = void 0; try { n = i.then } catch (e) { return void F(t, e) } P(t, i, n) } else Z(t, i) } function N(e) { e._onerror && e._onerror(e._result), j(e) } function Z(e, t) { e._state === T && (e._result = t, e._state = C, 0 !== e._subscribers.length && a(j, e)) } function F(e, t) { e._state === T && (e._state = k, e._result = t, a(N, e)) } function G(e, t, i, n) { var r = e._subscribers, s = r.length; e._onerror = null, r[s] = t, r[s + C] = i, r[s + k] = n, 0 === s && e._state && a(j, e) } function j(e) { var t = e._subscribers, i = e._state; if (0 !== t.length) { for (var n = void 0, r = void 0, s = e._result, o = 0; o < t.length; o += 3)n = t[o], r = t[o + i], n ? V(i, n, r, s) : r(s); e._subscribers.length = 0 } } function V(e, i, n, r) { var s = t(n), o = void 0, a = void 0, h = !0; if (s) { try { o = n(r) } catch (e) { h = !1, a = e } if (i === o) return void F(i, I()) } else o = r; i._state !== T || (s && h ? U(i, o) : !1 === h ? F(i, a) : e === C ? Z(i, o) : e === k && F(i, o)) } function z(e, t) { try { t((function (t) { U(e, t) }), (function (t) { F(e, t) })) } catch (t) { F(e, t) } } var K = 0; function H() { return K++ } function W(e) { e[w] = K++, e._state = void 0, e._result = void 0, e._subscribers = [] } function q() { return new Error("Array Methods must be provided an Array") } var Y = function () { function e(e, t) { this._instanceConstructor = e, this.promise = new e(O), this.promise[w] || W(this.promise), n(t) ? (this.length = t.length, this._remaining = t.length, this._result = new Array(this.length), 0 === this.length ? Z(this.promise, this._result) : (this.length = this.length || 0, this._enumerate(t), 0 === this._remaining && Z(this.promise, this._result))) : F(this.promise, q()) } return e.prototype._enumerate = function (e) { for (var t = 0; this._state === T && t < e.length; t++)this._eachEntry(e[t], t) }, e.prototype._eachEntry = function (e, t) { var i = this._instanceConstructor, n = i.resolve; if (n === R) { var r = void 0, s = void 0, o = !1; try { r = e.then } catch (e) { o = !0, s = e } if (r === L && e._state !== T) this._settledAt(e._state, t, e._result); else if ("function" != typeof r) this._remaining--, this._result[t] = e; else if (i === te) { var a = new i(O); o ? F(a, s) : P(a, e, r), this._willSettleAt(a, t) } else this._willSettleAt(new i((function (t) { return t(e) })), t) } else this._willSettleAt(n(e), t) }, e.prototype._settledAt = function (e, t, i) { var n = this.promise; n._state === T && (this._remaining--, e === k ? F(n, i) : this._result[t] = i), 0 === this._remaining && Z(n, this._result) }, e.prototype._willSettleAt = function (e, t) { var i = this; G(e, void 0, (function (e) { return i._settledAt(C, t, e) }), (function (e) { return i._settledAt(k, t, e) })) }, e }(); function X(e) { return new Y(this, e).promise } function J(e) { var t = this; return n(e) ? new t((function (i, n) { for (var r = e.length, s = 0; s < r; s++)t.resolve(e[s]).then(i, n) })) : new t((function (e, t) { return t(new TypeError("You must pass an array to race.")) })) } function Q(e) { var t = new this(O); return F(t, e), t } function $() { throw new TypeError("You must pass a resolver function as the first argument to the promise constructor") } function ee() { throw new TypeError("Failed to construct 'Promise': Please use the 'new' operator, this object constructor cannot be called as a function.") } var te = function () { function e(t) { this[w] = H(), this._result = this._state = void 0, this._subscribers = [], O !== t && ("function" != typeof t && $(), this instanceof e ? z(this, t) : ee()) } return e.prototype.catch = function (e) { return this.then(null, e) }, e.prototype.finally = function (e) { var i = this, n = i.constructor; return t(e) ? i.then((function (t) { return n.resolve(e()).then((function () { return t })) }), (function (t) { return n.resolve(e()).then((function () { throw t })) })) : i.then(e, e) }, e }(); function ie() { var e = void 0; if (void 0 !== i.g) e = i.g; else if ("undefined" != typeof self) e = self; else try { e = Function("return this")() } catch (e) { throw new Error("polyfill failed because global object is unavai
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本设计源码提供了一个基于Vue的原生认养后台管理系统。项目包含640个文件,主要使用Vue、CSS、JavaScript和HTML编程语言。文件类型包括247个Vue组件文件、217个PNG图片文件、82个CSS样式文件、68个JavaScript脚本文件、8个JPG图片文件、4个JSON配置文件、3个JPEG图片文件、2个TTF字体文件、2个WOFF字体文件和1个Babelrc配置文件。该系统适合用于学习和实践Vue、CSS、JavaScript和HTML技术,以及开发原生认养相关的后台管理系统。
资源推荐
资源详情
资源评论
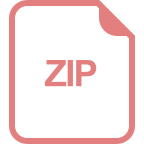
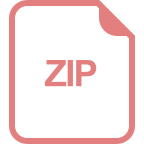
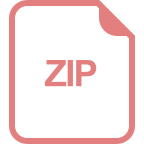
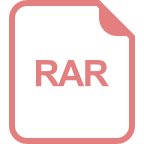
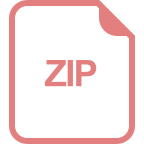
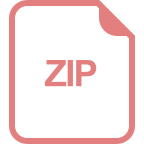
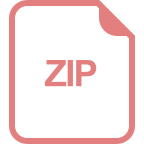
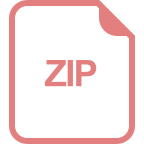
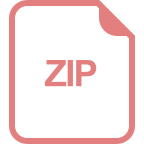
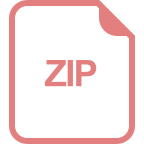
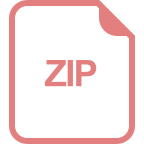
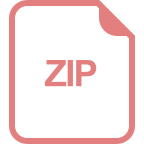
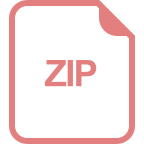
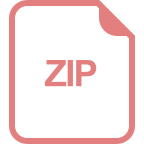
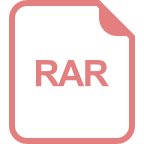
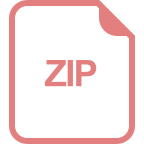
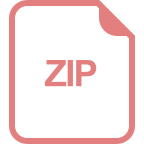
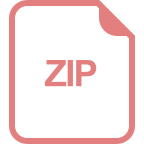
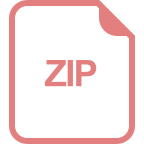
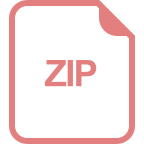
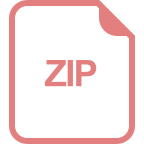
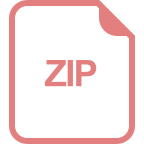
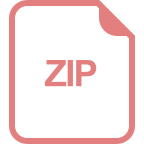
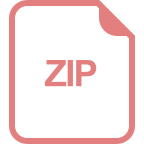
收起资源包目录

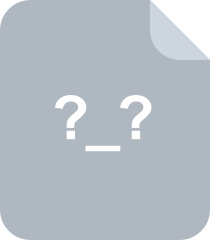
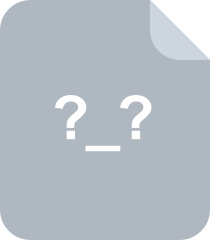
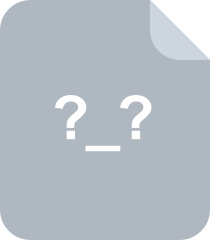
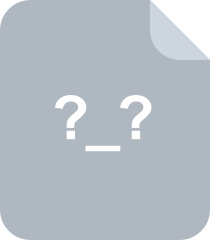
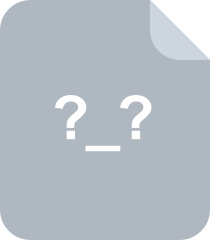
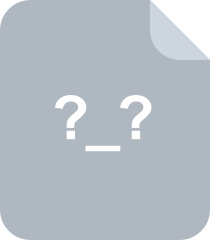
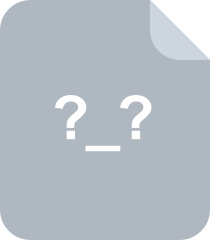
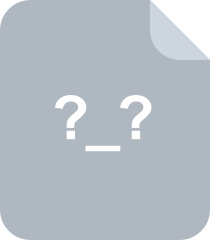
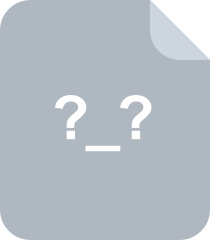
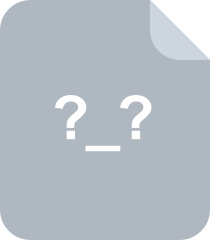
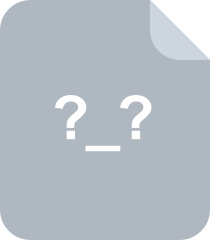
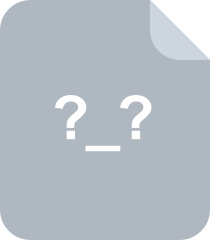
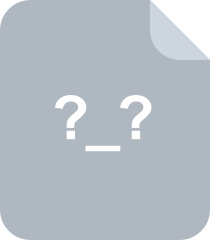
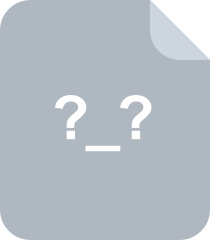
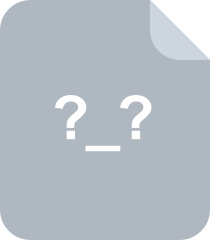
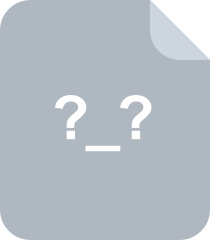
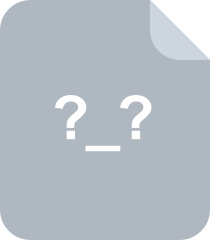
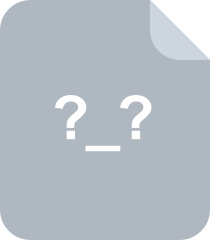
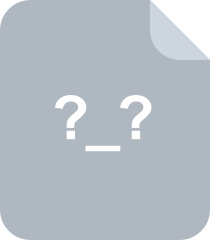
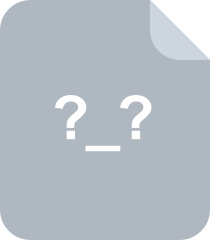
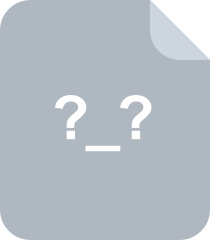
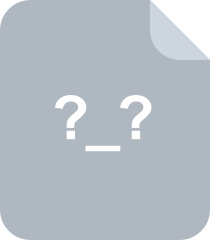
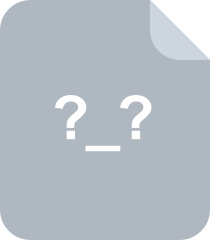
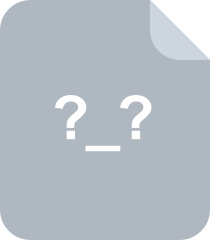
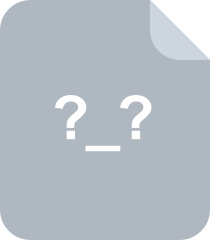
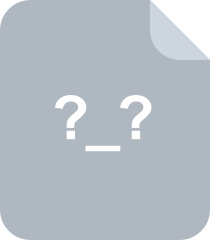
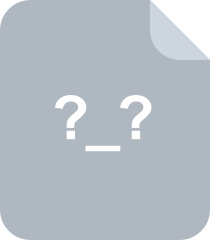
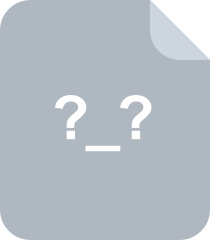
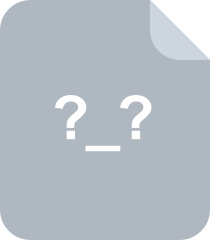
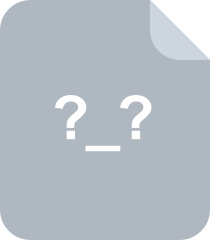
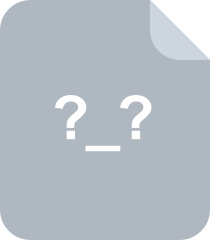
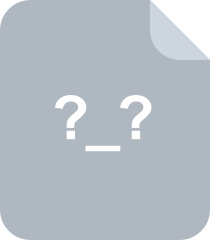
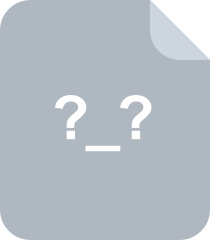
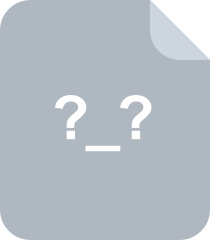
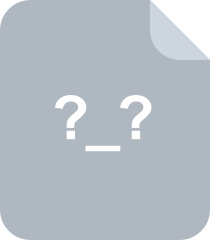
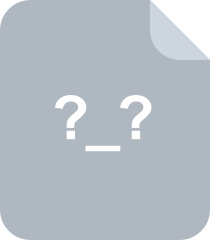
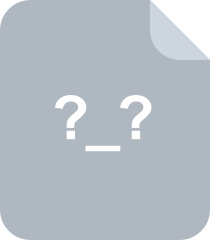
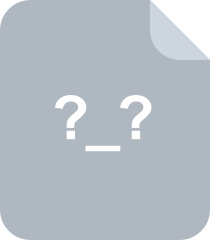
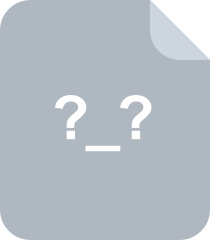
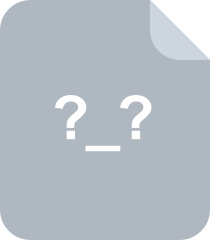
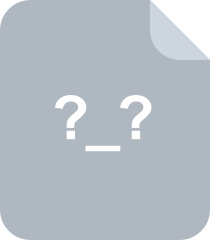
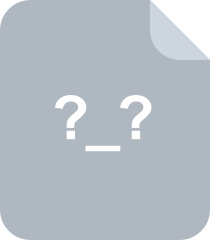
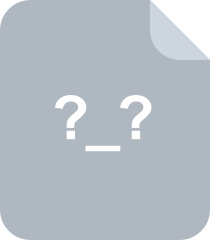
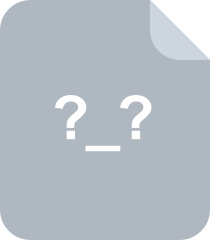
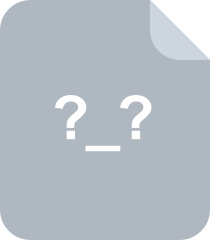
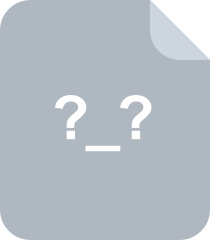
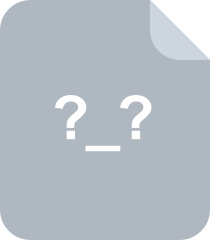
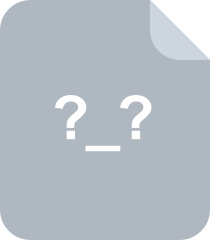
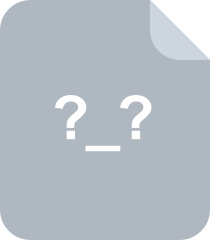
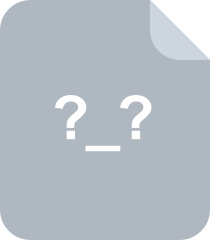
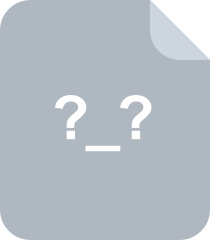
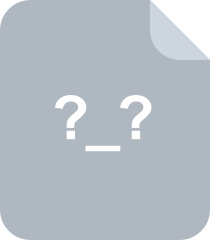
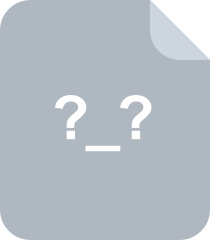
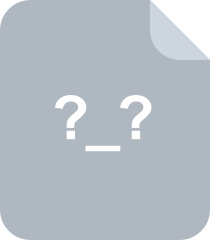
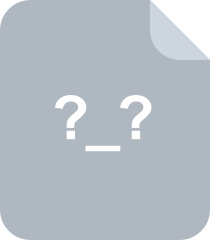
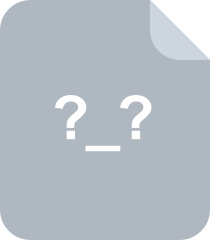
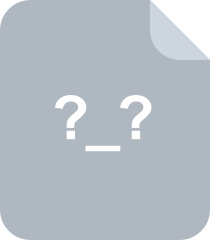
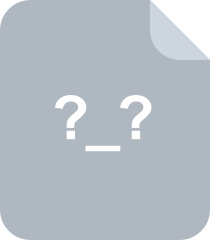
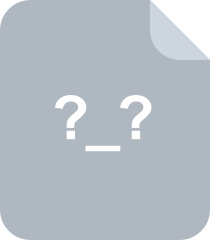
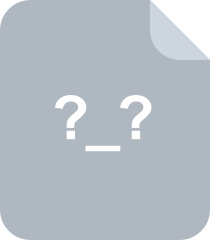
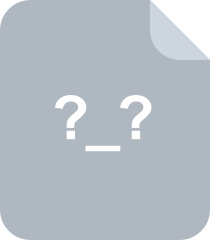
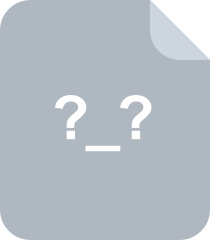
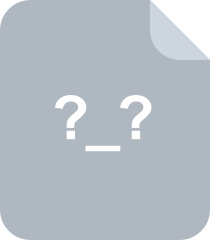
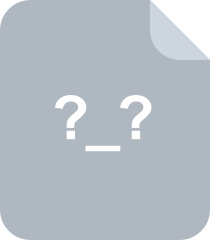
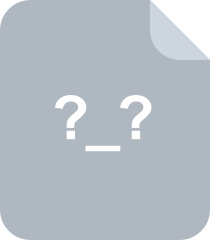
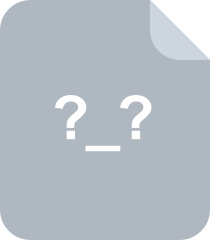
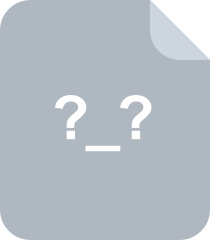
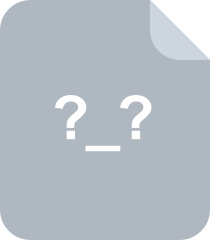
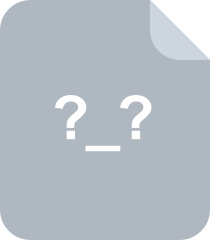
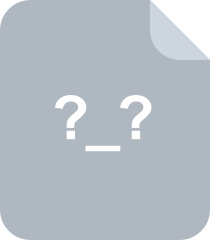
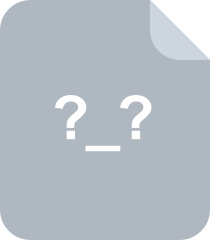
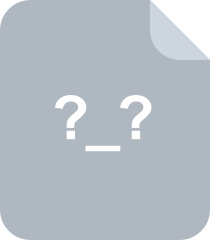
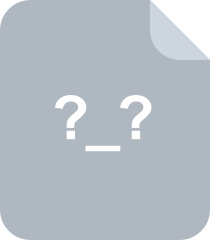
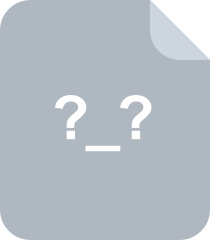
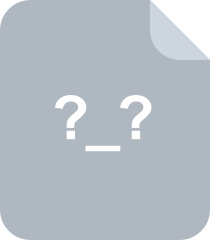
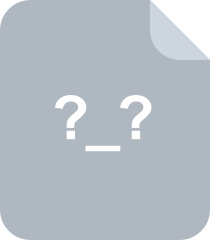
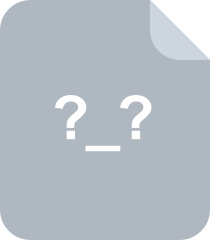
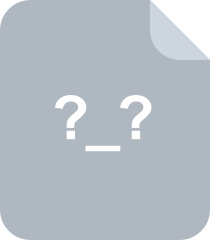
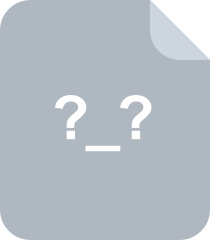
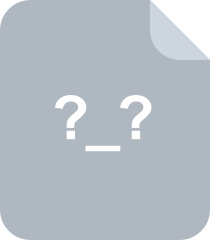
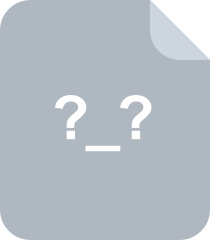
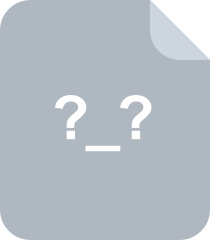
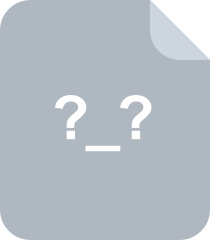
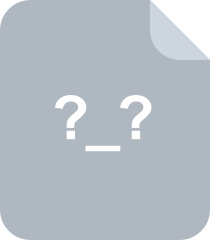
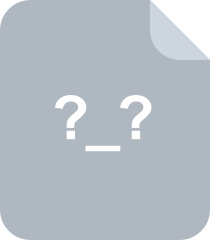
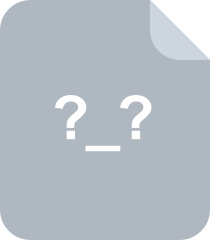
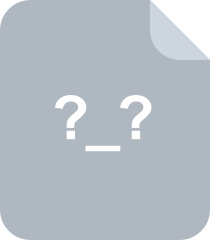
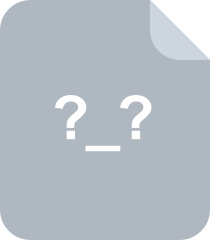
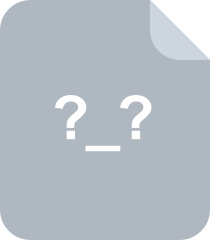
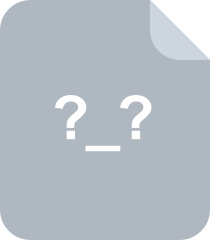
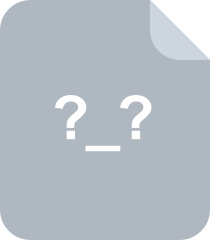
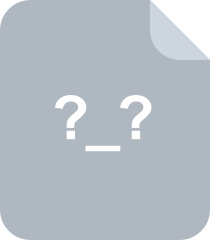
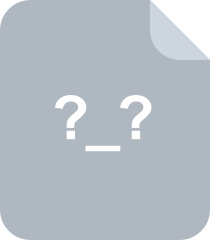
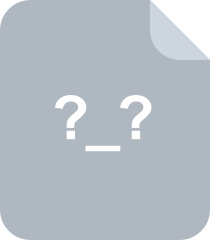
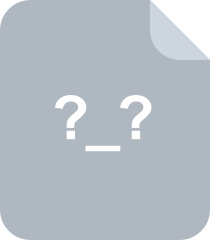
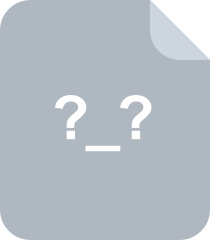
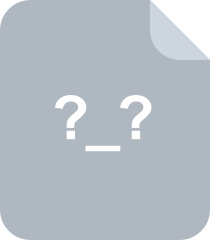
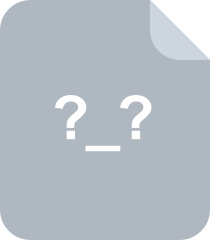
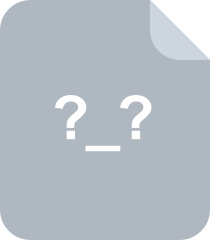
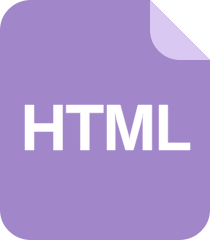
共 655 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
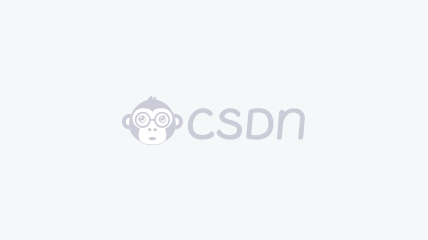

沐知全栈开发
- 粉丝: 5697
- 资源: 5224
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

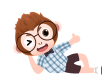
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


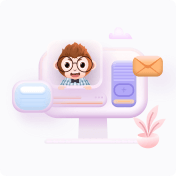
安全验证
文档复制为VIP权益,开通VIP直接复制
