/* exif-tag.c
*
* Copyright (c) 2001 Lutz Mueller <lutz@users.sourceforge.net>
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the
* Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor,
* Boston, MA 02110-1301 USA.
*/
#include <config.h>
#include <libexif/exif-tag.h>
#include <libexif/i18n.h>
#include <stdlib.h>
#include <string.h>
#define ESL_NNNN { EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED }
#define ESL_OOOO { EXIF_SUPPORT_LEVEL_OPTIONAL, EXIF_SUPPORT_LEVEL_OPTIONAL, EXIF_SUPPORT_LEVEL_OPTIONAL, EXIF_SUPPORT_LEVEL_OPTIONAL }
#define ESL_MMMN { EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_NOT_RECORDED }
#define ESL_MMMM { EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_MANDATORY }
#define ESL_OMON { EXIF_SUPPORT_LEVEL_OPTIONAL, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_OPTIONAL, EXIF_SUPPORT_LEVEL_NOT_RECORDED }
#define ESL_NNOO { EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_OPTIONAL, EXIF_SUPPORT_LEVEL_OPTIONAL }
#define ESL_NNMN { EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_NOT_RECORDED }
#define ESL_NNMM { EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_MANDATORY, EXIF_SUPPORT_LEVEL_MANDATORY }
#define ESL_NNNM { EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_MANDATORY }
#define ESL_NNNO { EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_NOT_RECORDED, EXIF_SUPPORT_LEVEL_OPTIONAL }
#define ESL_GPS { ESL_NNNN, ESL_NNNN, ESL_NNNN, ESL_OOOO, ESL_NNNN }
/*!
* Table giving information about each EXIF tag.
* There may be more than one entry with the same tag value because some tags
* have different meanings depending on the IFD in which they appear.
* When there are such duplicate entries, there must be no overlap in their
* support levels.
* The entries MUST be sorted in tag order.
* The name and title are mandatory, but the description may be an empty
* string. None of the entries may be NULL except the final array terminator.
*/
static const struct TagEntry {
/*! Tag ID. There may be duplicate tags when the same number is used for
* different meanings in different IFDs. */
ExifTag tag;
const char *name;
const char *title;
const char *description;
/*! indexed by the types [ExifIfd][ExifDataType] */
ExifSupportLevel esl[EXIF_IFD_COUNT][EXIF_DATA_TYPE_COUNT];
} ExifTagTable[] = {
#ifndef NO_VERBOSE_TAG_STRINGS
{EXIF_TAG_GPS_VERSION_ID, "GPSVersionID", N_("GPS Tag Version"),
N_("Indicates the version of <GPSInfoIFD>. The version is given "
"as 2.0.0.0. This tag is mandatory when <GPSInfo> tag is "
"present. (Note: The <GPSVersionID> tag is given in bytes, "
"unlike the <ExifVersion> tag. When the version is "
"2.0.0.0, the tag value is 02000000.H)."), ESL_GPS},
{EXIF_TAG_INTEROPERABILITY_INDEX, "InteroperabilityIndex",
N_("Interoperability Index"),
N_("Indicates the identification of the Interoperability rule. "
"Use \"R98\" for stating ExifR98 Rules. Four bytes used "
"including the termination code (NULL). see the separate "
"volume of Recommended Exif Interoperability Rules (ExifR98) "
"for other tags used for ExifR98."),
{ ESL_NNNN, ESL_NNNN, ESL_NNNN, ESL_NNNN, ESL_OOOO } },
{EXIF_TAG_GPS_LATITUDE_REF, "GPSLatitudeRef", N_("North or South Latitude"),
N_("Indicates whether the latitude is north or south latitude. The "
"ASCII value 'N' indicates north latitude, and 'S' is south "
"latitude."), ESL_GPS},
{EXIF_TAG_INTEROPERABILITY_VERSION, "InteroperabilityVersion",
N_("Interoperability Version"), "",
{ ESL_NNNN, ESL_NNNN, ESL_NNNN, ESL_NNNN, ESL_OOOO } },
{EXIF_TAG_GPS_LATITUDE, "GPSLatitude", N_("Latitude"),
N_("Indicates the latitude. The latitude is expressed as three "
"RATIONAL values giving the degrees, minutes, and seconds, "
"respectively. When degrees, minutes and seconds are expressed, "
"the format is dd/1,mm/1,ss/1. When degrees and minutes are used "
"and, for example, fractions of minutes are given up to two "
"decimal places, the format is dd/1,mmmm/100,0/1."),
ESL_GPS},
{EXIF_TAG_GPS_LONGITUDE_REF, "GPSLongitudeRef", N_("East or West Longitude"),
N_("Indicates whether the longitude is east or west longitude. "
"ASCII 'E' indicates east longitude, and 'W' is west "
"longitude."), ESL_GPS},
{EXIF_TAG_GPS_LONGITUDE, "GPSLongitude", N_("Longitude"),
N_("Indicates the longitude. The longitude is expressed as three "
"RATIONAL values giving the degrees, minutes, and seconds, "
"respectively. When degrees, minutes and seconds are expressed, "
"the format is ddd/1,mm/1,ss/1. When degrees and minutes are "
"used and, for example, fractions of minutes are given up to "
"two decimal places, the format is ddd/1,mmmm/100,0/1."),
ESL_GPS},
{EXIF_TAG_GPS_ALTITUDE_REF, "GPSAltitudeRef", N_("Altitude Reference"),
N_("Indicates the altitude used as the reference altitude. If the "
"reference is sea level and the altitude is above sea level, 0 "
"is given. If the altitude is below sea level, a value of 1 is given "
"and the altitude is indicated as an absolute value in the "
"GSPAltitude tag. The reference unit is meters. Note that this tag "
"is BYTE type, unlike other reference tags."), ESL_GPS},
{EXIF_TAG_GPS_ALTITUDE, "GPSAltitude", N_("Altitude"),
N_("Indicates the altitude based on the reference in GPSAltitudeRef. "
"Altitude is expressed as one RATIONAL value. The reference unit "
"is meters."), ESL_GPS},
{EXIF_TAG_GPS_TIME_STAMP, "GPSTimeStamp", N_("GPS Time (Atomic Clock)"),
N_("Indicates the time as UTC (Coordinated Universal Time). "
"TimeStamp is expressed as three RATIONAL values giving "
"the hour, minute, and second."), ESL_GPS},
{EXIF_TAG_GPS_SATELLITES, "GPSSatellites", N_("GPS Satellites"),
N_("Indicates the GPS satellites used for measurements. This "
"tag can be used to describe the number of satellites, their ID "
"number, angle of elevation, azimuth, SNR and other information "
"in ASCII notation. The format is not specified. If the GPS "
"receiver is incapable of taking measurements, value of the tag "
"shall be set to NULL."), ESL_GPS},
{EXIF_TAG_GPS_STATUS, "GPSStatus", N_("GPS Receiver Status"),
N_("Indicates the status of the GPS receiver when the image is "
"recorded. 'A' means measurement is in progress, and 'V' means "
"the measurement is Interoperability."), ESL_GPS},
{EXIF_TAG_GPS_MEASURE_MODE, "GPSMeasureMode", N_("GPS Measurement Mode"),
N_("Indicates the GPS measurement mode. '2' means "
"two-dimensional measurement and '3' means three-dimensional "
"measurement is in progress."), ESL_GPS},
{EXIF_TAG_GPS_DOP, "GPSDOP", N_("Measurement Precision"),
N_("Indicates the GPS DOP (data degree of precision). An HDOP "
"value is written during two-dimensional measurement,
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
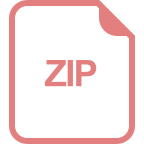
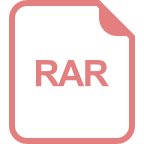
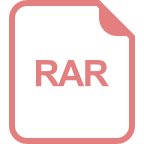
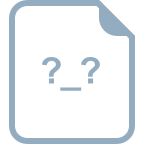
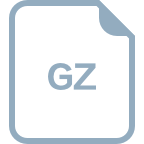
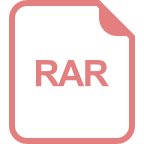
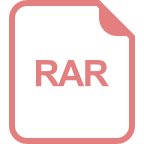
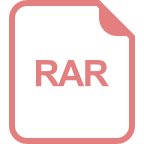
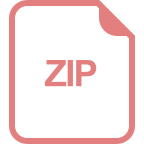
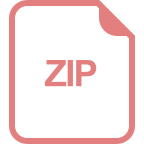
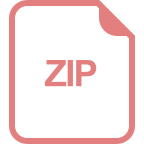
收起资源包目录


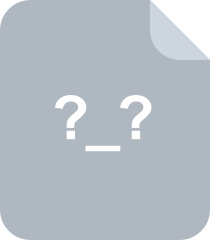
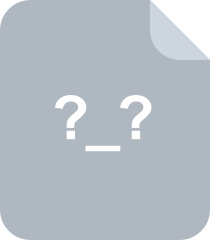
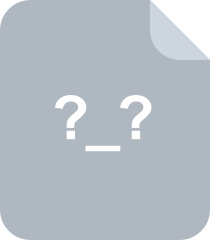
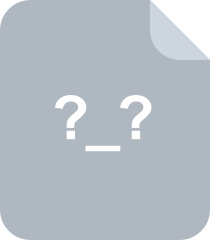
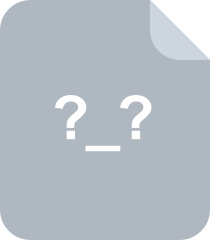
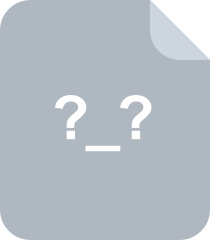
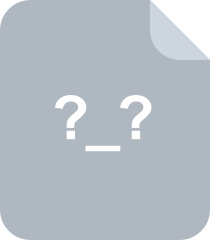
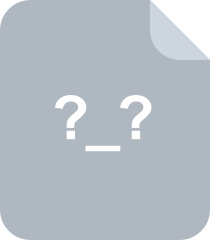
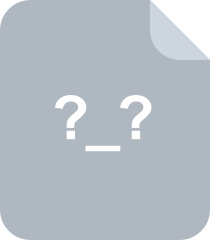
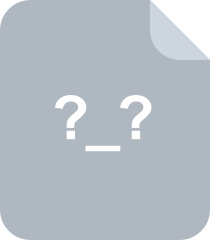

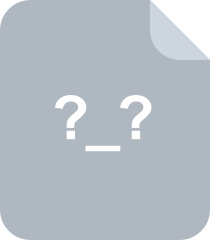
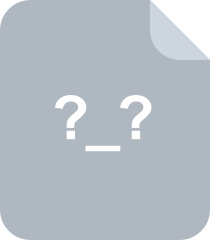
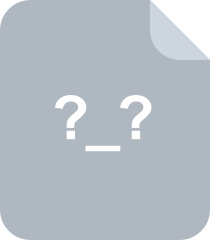
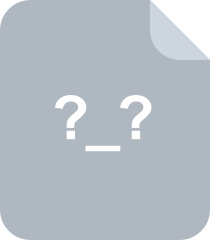
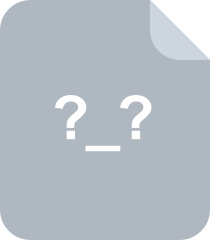
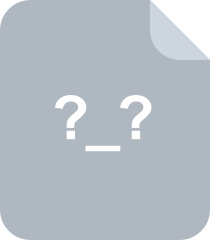

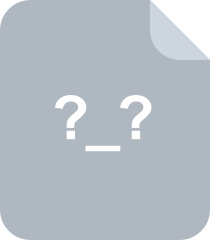
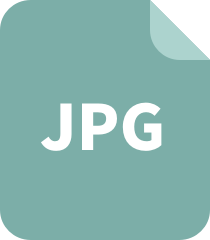
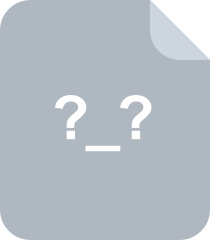
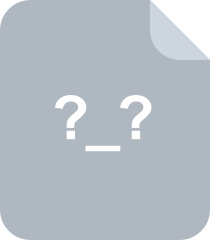
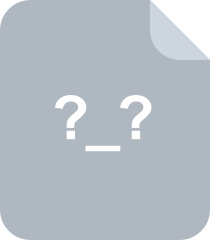
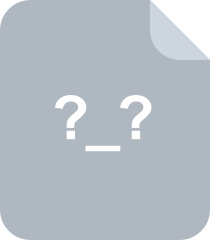
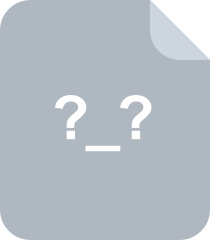
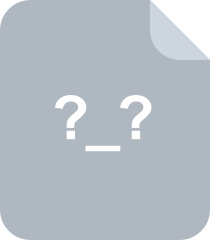
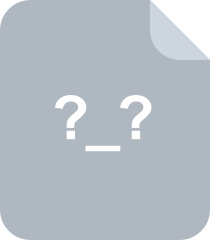
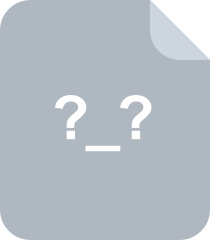
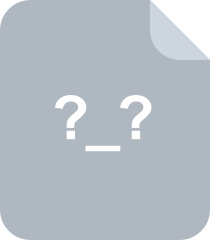
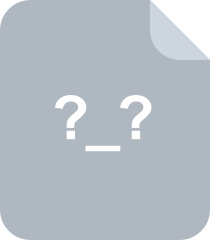
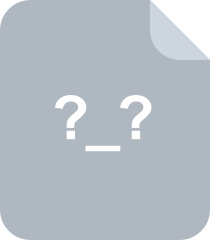


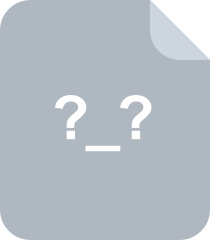
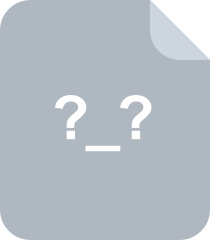

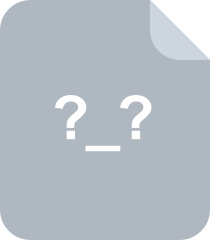
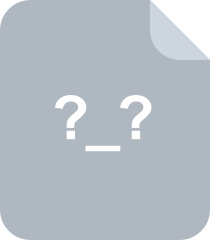
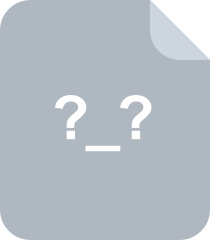
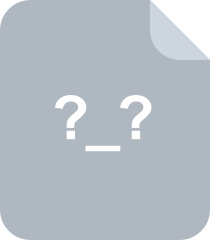
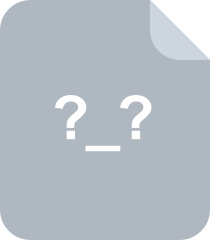
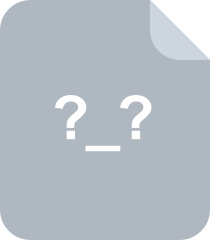
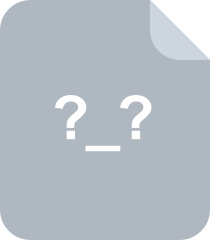
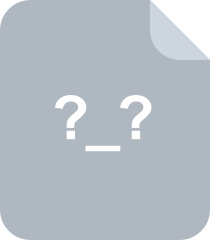

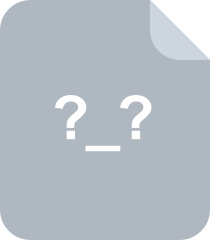
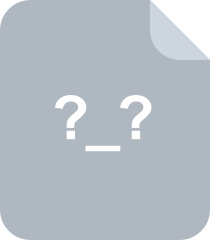
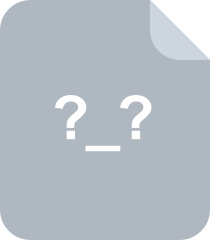

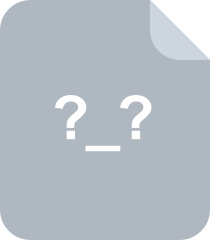
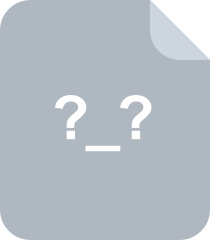
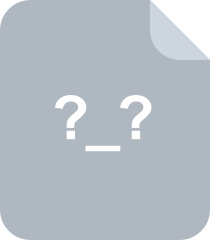
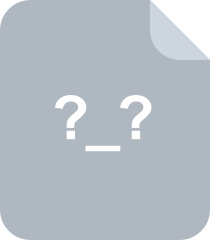
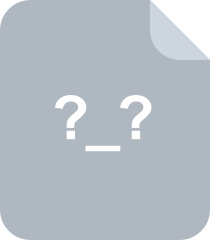
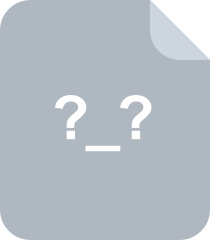

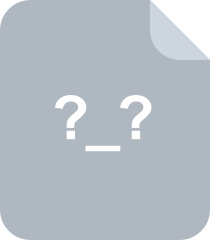
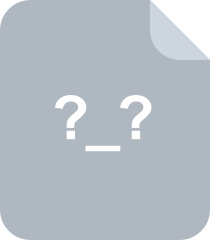
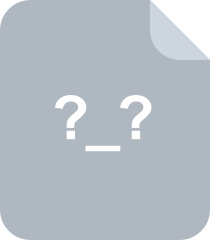
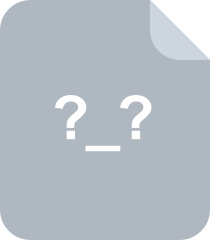
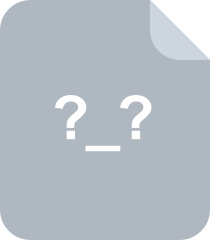
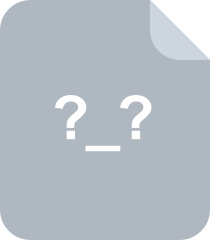
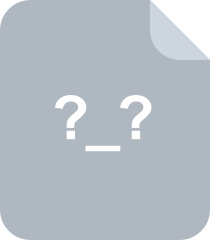
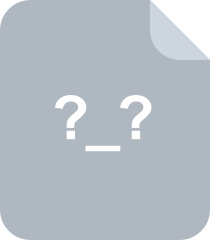
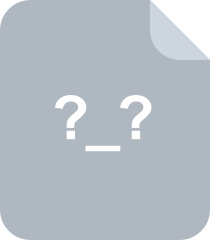
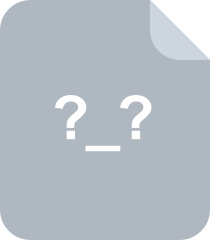
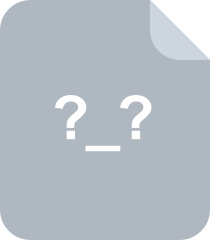
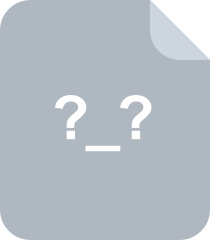


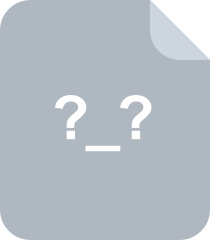


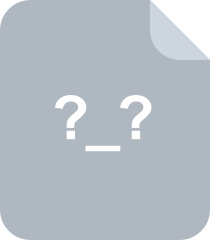
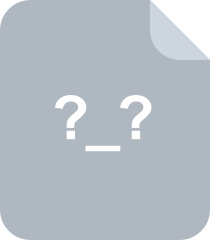
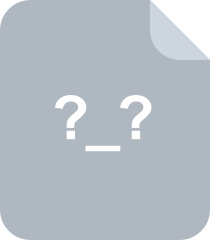
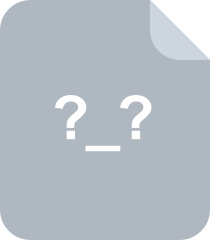
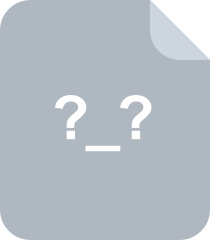
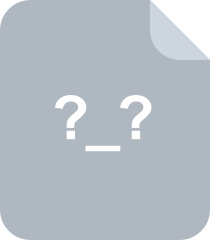
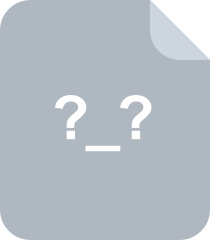
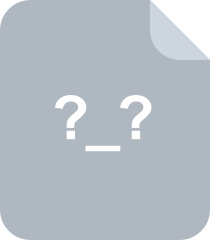
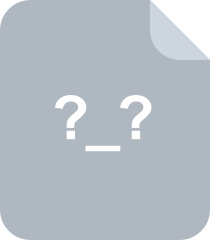
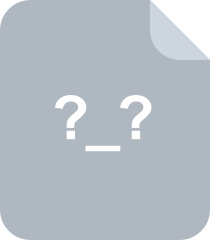
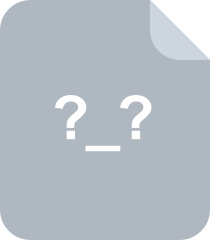
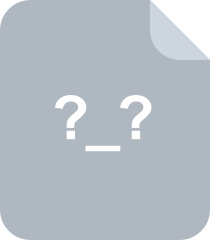
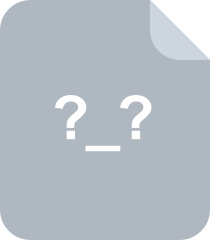
共 74 条
- 1
资源评论
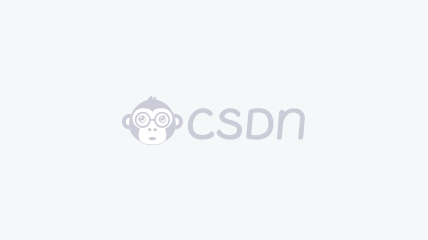
- 与夏同炙2019-12-09没有太多示例
- AdvanceBiliBili2021-12-01不错 好活当赏
- jinvbnet2019-01-10太棒了,学习了,同样用在hisi平台,谢谢
- adengou2019-02-20下载来学习,还没研究

LiaoJunXiong
- 粉丝: 86
- 资源: 11
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

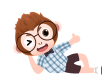
安全验证
文档复制为VIP权益,开通VIP直接复制
