魔方模拟器源码
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5
6 namespace ConsoleApplication
7 {
8 //记录操作历史
9 struct Option
10 {
11 public int turnOrSpin;
12 public int rowOrColumn, direction;
13 public int clockwiseOrCounterclockwise;
14 public int number;
15 }
16
17 class MagicCube
18 {
19 const int maxn = 20009; //句柄最大量
20
21 readonly int Red = 1; //颜色系统
22 readonly int Orange = 2; //实际颜色为粉色……系统颜色中无橙色
23 readonly int Blue = 3;
24 readonly int Green = 4;
25 readonly int Yellow = 5;
26 readonly int White = 6;
27
28 readonly int Front = 1; //朝向系统
29 readonly int Back = 2;
30 readonly int Left = 3;
31 readonly int Right = 4;
32 readonly int Up = 5;
33 readonly int Down = 6;
34 readonly int[] Opposite = { 0, 2, 1, 4, 3, 6, 5 }; //对立面
35
36 public int[,] F = new int[4, 4]; //存储对象
37 public int[,] B = new int[4, 4];
38 public int[,] L = new int[4, 4];
39 public int[,] R = new int[4, 4];
40 public int[,] U = new int[4, 4];
41 public int[,] D = new int[4, 4];
42
43 public Option[] theLastOptions = new Option[maxn]; //撤销操作
44 public int CountOptions;
45
46 public int nowFace; //定位系统
47 public int nowUnder;
48 public int[,] GetLeft = {{0,0,0,0,0,0,0},
49 {0,0,0,5,6,4,3},
50 {0,0,0,6,5,3,4},
51 {0,6,5,0,0,1,2},
52 {0,5,6,0,0,2,1},
53 {0,3,4,2,1,0,0},
54 {0,4,3,1,2,0,0}};
55
56 readonly int Turn = 1; //操作参数
57 readonly int Spin = 2;
58 readonly int Row = 1;
59 readonly int Column = 2;
60 readonly int LEFT = 1;
61 readonly int RIGHT = 2;
62 readonly int UP = 1;
63 readonly int DOWN = 2;
64 readonly int Clockwise = 3;
65 readonly int Counterclockwise = 4;
66
67 public MagicCube()
68 {
69 //颜色初始化
70 for (int i = 1; i <= 3; i++)
71 for (int j = 1; j <= 3; j++)
72 {
73 F[i, j] = Red; B[i, j] = Orange;
74 L[i, j] = Blue; R[i, j] = Green;
75 U[i, j] = Yellow; D[i, j] = White;
76 }
77
78 nowFace = Front; //位置初始化
79 nowUnder = Down;
80
81 CountOptions = 0; //操作数初始化
82 }
83
84 public void Reposition() //复位
85 {
86 for (int i = 1; i <= 3; i++)
87 for (int j = 1; j <= 3; j++)
88 {
89 F[i, j] = Red; B[i, j] = Orange;
90 L[i, j] = Blue; R[i, j] = Green;
91 U[i, j] = Yellow; D[i, j] = White;
92 }
93
94 nowFace = Front;
95 nowUnder = Down;
96
97 CountOptions = 0;
98 }
99
100 private void EnsureFront(ref int[,] Play)
101 {
102 if (nowFace == Front) Play = F;
103 if (nowFace == Back) Play = B;
104 if (nowFace == Left) Play = L;
105 if (nowFace == Right) Play = R;
106 if (nowFace == Up) Play = U;
107 if (nowFace == Down) Play = D;
108 }
109
110 private void EnsureBack(ref int[,] Play)
111 {
112 if (Opposite[nowFace] == Front) Play = F;
113 if (Opposite[nowFace] == Back) Play = B;
114 if (Opposite[nowFace] == Left) Play = L;
115 if (Opposite[nowFace] == Right) Play = R;
116 if (Opposite[nowFace] == Up) Play = U;
117 if (Opposite[nowFace] == Down) Play = D;
118 }
119
120 private void EnsureLeft(ref int[,] Play)
121 {
122 if (GetLeft[nowFace, nowUnder] == Front) Play = F;
123 if (GetLeft[nowFace, nowUnder] == Back) Play = B;
124 if (GetLeft[nowFace, nowUnder] == Left) Play = L;
125 if (GetLeft[nowFace, nowUnder] == Right) Play = R;
126 if (GetLeft[nowFace, nowUnder] == Up) Play = U;
127 if (GetLeft[nowFace, nowUnder] == Down) Play = D;
128 }
129
130 private void EnsureRight(ref int[,] Play)
131 {
132 if (Opposite[GetLeft[nowFace, nowUnder]] == Front) Play = F;
133 if (Opposite[GetLeft[nowFace, nowUnder]] == Back) Play = B;
134 if (Opposite[GetLeft[nowFace, nowUnder]] == Left) Play = L;
135 if (Opposite[GetLeft[nowFace, nowUnder]] == Right) Play = R;
136 if (Opposite[GetLeft[nowFace, nowUnder]] == Up) Play = U;
137 if (Opposite[GetLeft[nowFace, nowUnder]] == Down) Play = D;
138 }
139
140 private void EnsureUp(ref int[,] Play)
141 {
142 if (Opposite[nowUnder] == Front) Play = F;
143 if (Opposite[nowUnder] == Back) Play = B;
144 if (Opposite[nowUnder] == Left) Play = L;
145 if (Opposite[nowUnder] == Right) Play = R;
146 if (Opposite[nowUnder] == Up) Play = U;
147 if (Opposite[nowUnder] == Down) Play = D;
148 }
149
150 private void EnsureDown(ref int[,] Play)
151 {
152 if (nowUnder == Front) Play = F;
153 if (nowUnder == Back) Play = B;
154 if (nowUnder == Left) Play = L;
155 if (nowUnder == Right) Play = R;
156 if (nowUnder == Up) Play = U;
157 if (nowUnder == Down) Play = D;
158 }
159
160 public void SpinRight_UpFace()
161 {
162 int[,] refer = new int[4, 4];
163 EnsureUp(ref refer);
164 int[,] temporary = new int[4, 4];
165 for (int i = 1; i <= 3; i++)
166 for (int j = 1; j <= 3; j++)
167 temporary[i, j] = refer[i, j];
168 for (int i = 1; i <= 3; i++)
169 for (int j = 1; j <= 3; j++)
170 refer[3 - j + 1, i] = temporary[i, j];
171 }
172
173 public void SpinRight_DownFace()
174 {
175 int[,] refer = new int[4, 4];
176 EnsureDown(ref refer);
177 int[,] temporary = new int[4, 4];
178 for (int i = 1; i <= 3; i++)
179 for (int j = 1; j <= 3; j++)
180 temporary[i, j] = refer[i, j];
181 for (int i = 1; i <= 3; i++)
182 for (int j = 1; j <= 3; j++)
183 refer[j, 3 - i + 1] = temporary[i, j];
184 }
185
186 public void TurnToLeft()
187 {
188 SpinRight_UpFace();
189 SpinRight_DownFace();
190 nowFace = GetLeft[nowFace, nowUnder];
191 }
192
193 public void SpinLeft_UpFace()
194 {
195 int[,] refer = new int[4, 4];
196 EnsureUp(ref refer);
197 int[,] temporary = new int[4, 4];
198 for (int i = 1; i <= 3; i++)
199 for (int j = 1; j <= 3; j++)
200 temporary[i, j] = refer[i, j];
201 for (int i = 1; i <= 3; i++)

呼啸庄主
- 粉丝: 87
- 资源: 4695
最新资源
- 基于ANSYS的矿用圆环链焊接缺陷的有限元分析.pdf
- 基于ANSYS钢结构的焊接温度场仿真分析.pdf
- 基于BP神经网络的铲斗焊接残余应力预测.pdf
- 基于CATIA的动车司机室骨架焊接工装的参数化设计.pdf
- 基于ssm的大学生勤工助学管理系统源码(java毕业设计完整源码+LW).zip
- java项目文本编辑器源代码.zip
- ECharts地图-自定义25.zip
- java学生住宿管理系统源代码.zip
- 基于ssm的大学生社团管理系统源码(java毕业设计完整源码).zip
- C#与三菱,西门子,台达,基恩士,等各品牌plc通讯源码
- java阳光酒店管理系统源代码.zip
- 不同颜色球体和球架检测37-YOLO(v5至v11)、COCO数据集合集.rar
- 基于ssm的大学运动场地管理系统源码(java毕业设计完整源码+LW).zip
- 共5套立体仓库,堆垛机三维模型 sw打开,有零部件模型,有装 配体模型,有堆垛机的详细机械结构 可用于设计参考,结构了解,方案制作
- Liquid Volume.unitypackage
- 基于ssm的大众书评网源码(java毕业设计完整源码).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


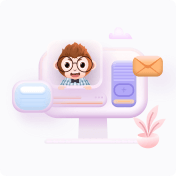