1 //--------------------------------------------------------------------------------------
2 // File: ParallaxOcclusionMapping.fx
3 //
4 // Parallax occlusion mapping implementation
5 //
6 // Implementation of the algorithm as described in "Dynamic Parallax Occlusion
7 // Mapping with Approximate Soft Shadows" paper, by N. Tatarchuk, ATI Research,
8 // to appear in the proceedings of ACM Symposium on Interactive 3D Graphics and Games, 2006.
9 //
10 // For examples of use in a real-time scene, see ATI X1K demo "ToyShop":
11 // http://www.ati.com/developer/demos/rx1800.html
12 //
13 // Copyright (c) ATI Research, Inc. All rights reserved.
14 //--------------------------------------------------------------------------------------
15
16 //--------------------------------------------------------------------------------------
17 // Global variables
18 //--------------------------------------------------------------------------------------
19
20 texture g_baseTexture; // Base color texture
21 texture g_nmhTexture; // Normal map and height map texture pair
22
23 float4 g_materialAmbientColor; // Material's ambient color
24 float4 g_materialDiffuseColor; // Material's diffuse color
25 float4 g_materialSpecularColor; // Material's specular color
26
27 float g_fSpecularExponent; // Material's specular exponent
28 bool g_bAddSpecular; // Toggles rendering with specular or without
29
30 // Light parameters:
31 float3 g_LightDir; // Light's direction in world space
32 float4 g_LightDiffuse; // Light's diffuse color
33 float4 g_LightAmbient; // Light's ambient color
34
35 float4 g_vEye; // Camera's location
36 float g_fBaseTextureRepeat; // The tiling factor for base and normal map textures
37 float g_fHeightMapScale; // Describes the useful range of values for the height field
38
39 // Matrices:
40 float4x4 g_mWorld; // World matrix for object
41 float4x4 g_mWorldViewProjection; // World * View * Projection matrix
42 float4x4 g_mView; // View matrix
43
44 bool g_bVisualizeLOD; // Toggles visualization of level of detail colors
45 bool g_bVisualizeMipLevel; // Toggles visualization of mip level
46 bool g_bDisplayShadows; // Toggles display of self-occlusion based shadows
47
48 float2 g_vTextureDims; // Specifies texture dimensions for computation of mip level at
49 // render time (width, height)
50
51 int g_nLODThreshold; // The mip level id for transitioning between the full computation
52 // for parallax occlusion mapping and the bump mapping computation
53
54 float g_fShadowSoftening; // Blurring factor for the soft shadows computation
55
56 int g_nMinSamples; // The minimum number of samples for sampling the height field profile
57 int g_nMaxSamples; // The maximum number of samples for sampling the height field profile
58
59
60
61 //--------------------------------------------------------------------------------------
62 // Texture samplers
63 //--------------------------------------------------------------------------------------
64 sampler tBase =
65 sampler_state
66 {
67 Texture = < g_baseTexture >;
68 MipFilter = LINEAR;
69 MinFilter = LINEAR;
70 MagFilter = LINEAR;
71 };
72 sampler tNormalHeightMap =
73 sampler_state
74 {
75 Texture = < g_nmhTexture >;
76 MipFilter = LINEAR;
77 MinFilter = LINEAR;
78 MagFilter = LINEAR;
79 };
80
81
82 //--------------------------------------------------------------------------------------
83 // Vertex shader output structure
84 //--------------------------------------------------------------------------------------
85 struct VS_OUTPUT
86 {
87 float4 position : POSITION;
88 float2 texCoord : TEXCOORD0;
89 float3 vLightTS : TEXCOORD1; // light vector in tangent space, denormalized
90 float3 vViewTS : TEXCOORD2; // view vector in tangent space, denormalized
91 float2 vParallaxOffsetTS : TEXCOORD3; // Parallax offset vector in tangent space
92 float3 vNormalWS : TEXCOORD4; // Normal vector in world space
93 float3 vViewWS : TEXCOORD5; // View vector in world space
94
95 };
96
97
98 //--------------------------------------------------------------------------------------
99 // This shader computes standard transform and lighting
100 //--------------------------------------------------------------------------------------
101 VS_OUTPUT RenderSceneVS( float4 inPositionOS : POSITION,
102 float2 inTexCoord : TEXCOORD0,
103 float3 vInNormalOS : NORMAL,
104 float3 vInBinormalOS : BINORMAL,
105 float3 vInTangentOS : TANGENT )
106 {
107 VS_OUTPUT Out;
108
109 // Transform and output input position
110 Out.position = mul( inPositionOS, g_mWorldViewProjection );
111
112 // Propagate texture coordinate through:
113 Out.texCoord = inTexCoord * g_fBaseTextureRepeat;
114
115 // Transform the normal, tangent and binormal vectors from object space to homogeneous projection space:
116 float3 vNormalWS = mul( vInNormalOS, (float3x3) g_mWorld );
117 float3 vTangentWS = mul( vInTangentOS, (float3x3) g_mWorld );
118 float3 vBinormalWS = mul( vInBinormalOS, (float3x3) g_mWorld );
119
120 // Propagate the world space vertex normal through:
121 Out.vNormalWS = vNormalWS;
122 vNormalWS = normalize( vNormalWS );
123 vTangentWS = normalize( vTangentWS );
124 vBinormalWS = normalize( vBinormalWS );
125
126 // Compute position in world space:
127 float4 vPositionWS = mul( inPositionOS, g_mWorld );
128
129 // Compute and output the world view vector (unnormalized):
130 float3 vViewWS = g_vEye - vPositionWS;
131 Out.vViewWS = vViewWS;
132
133 // Compute denormalized light vector in world space:
134 float3 vLightWS = g_LightDir;
135
136 // Normalize the light and view vectors and transform it to the tangent space:
137 float3x3 mWorldToTangent = float3x3( vTangentWS, vBinormalWS, vNormalWS );
138
139 // Propagate the view and the light vectors (in tangent space):
140 Out.vLightTS = mul( vLightWS, mWorldToTangent );
141 Out.vViewTS = mul( mWorldToTangent, vViewWS );
142
143 // Compute the ray direction for intersecting the height field profile with
144 // current view ray. See the above paper for derivation of this computation.
145
146 // Compute initial parallax displacement direction:
147 float2 vParallaxDirection = normalize( Out.vViewTS.xy );
148
149 // The length of this vector determines the furthest amount of displacement:
150 float fLength = length( Out.vViewTS );
151 float fParallaxLength = sqrt( fLength * fLength - Out.vViewTS.z * Out.vViewTS.z ) / Out.vViewTS.z;
152
153 // Compute the actual reverse parallax displacement vector:
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
parallax occlusion map是应用在计算机图形中的一项重要技术。该技术通过建立和利用描述物体表面的高度的height texture来模拟物体表面复杂的模型,以减少场景中多边形的数目,从而降低了性能的损耗。
资源推荐
资源详情
资源评论
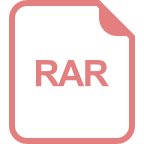
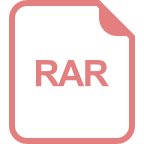
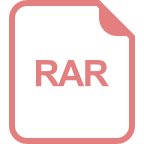
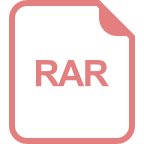
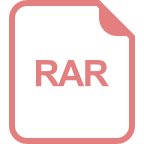
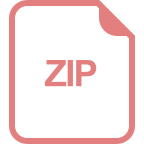
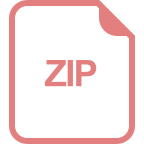
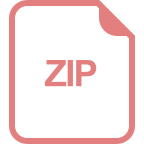
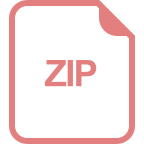
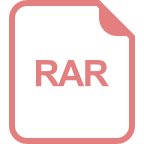
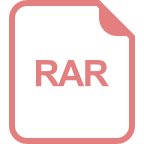
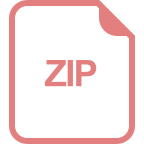
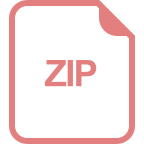
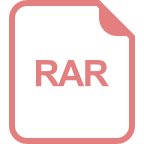
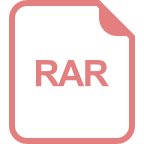
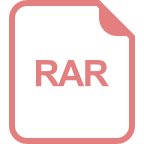
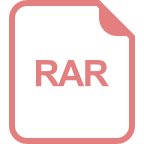
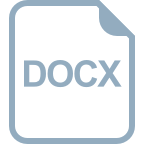
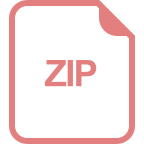
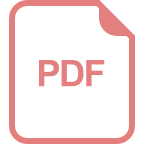
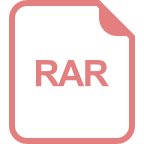
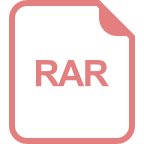
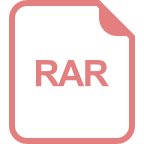
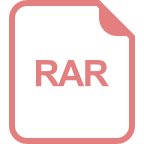
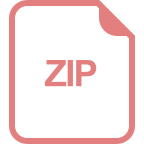
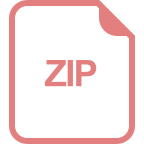
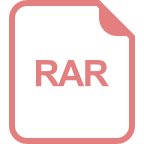
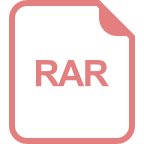
收起资源包目录

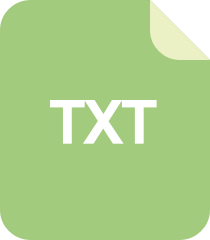
共 1 条
- 1
资源评论
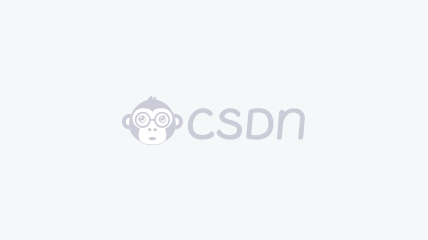

小贝德罗
- 粉丝: 86
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

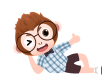
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


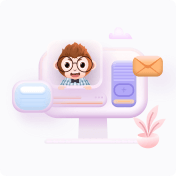
安全验证
文档复制为VIP权益,开通VIP直接复制
