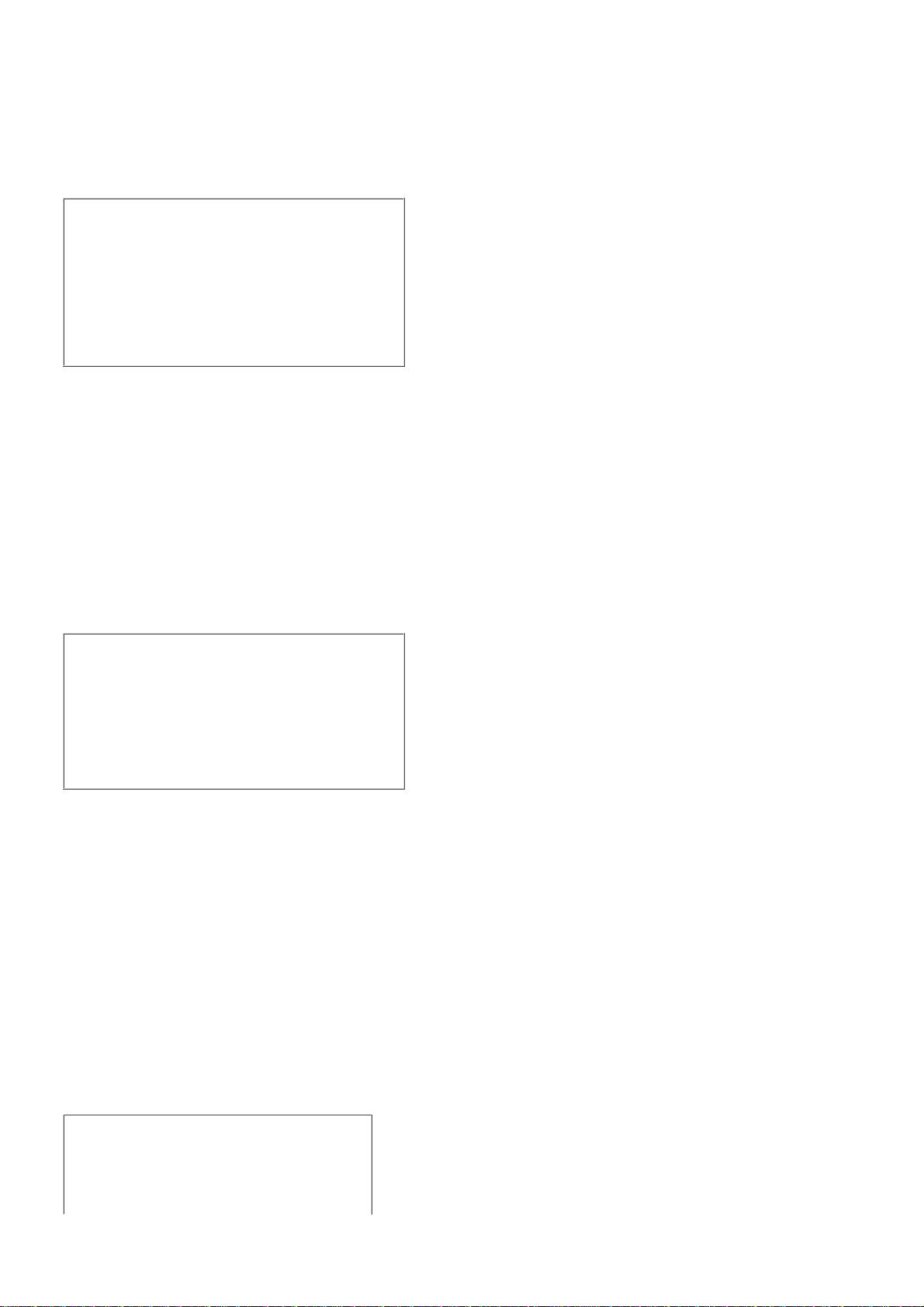
The code in Listing 3 causes the Stop button to be disabled and causes the Play button to be enabled
when the program first starts running. This is the situation shown in Figure 1.
Action listener on the Play button
The code in Listing 4 instantiates and register an action listener on the Play button.
playBtn.addActionListener(
new ActionListener(){
public void actionPerformed(
ActionEvent e){
stopBtn.setEnabled(true);
playBtn.setEnabled(false);
playAudio();//Play the file
}//end actionPerformed
}//end ActionListener
);//end addActionListener()
Listing 4
I explained this anonymous inner class syntax in the previous lesson. The most significant thing about
the code in Listing 4 is the statement highlighted in boldface. This statement invokes the playAudio
method to play the contents of the audio file when the user clicks the Play button.
The other two statements in Listing 4 simply reverse the enabled/disabled state of the Play and Stop
buttons.
Action listener on the Stop button
We are still discussing the constructor for the controlling class. The code in Listing 5 instantiates and
registers an action listener on the Stop button.
stopBtn.addActionListener(
new ActionListener(){
public void actionPerformed(
ActionEvent e){
//Terminate playback before EOF
stopPlayback = true;
}//end actionPerformed
}//end ActionListener
);//end addActionListener()
Listing 5
When the user clicks the Stop button, the code in Listing 5 sets a flag named stopPlayback to true. As
you will see later, the thread that handles the actual playback operation periodically tests this flag. If the
flag switches from false to true, the code in the run method of the playback thread terminates playback at
that point in time.
There may be a time delay before termination of playback
However, there are some rather large buffers used in the playback loop. These buffers must be emptied
before playback actually terminates. Therefore, there may be a time delay between the point in time that
this flag is set to true and the point in time that playback actually terminates.
Finish constructing the GUI
The code in Listing 6 finishes the construction of the GUI.
getContentPane().add(playBtn,"West");
getContentPane().add(stopBtn,"East");
getContentPane().add(textField,"North");
setTitle("Copyright 2003, R.G.Baldwin");
setDefaultCloseOperation(EXIT_ON_CLOSE);
Java Sound, Playing Back Audio Files using Java — Devel... http://www.developer.com/print.php/2173111
第4页 共13页 2009-9-28 16:22