Tricks of the Windows video Game Programming---part1
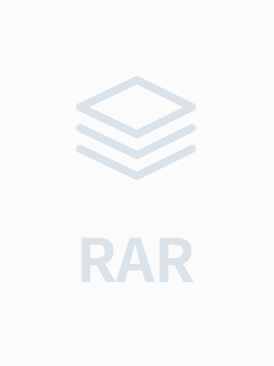


Tricks of the Windows video Game Programming<br><br>PART I Windows Programming Foundations 7<br>1 Journey into the Abyss 9<br>A Little History........................................................................................9<br>Designing Games ..................................................................................13<br>Types of Games ....................................................................................13<br>Brainstorming........................................................................................14<br>The Design Document and Storyboards................................................15<br>Making the Game Fun ..........................................................................16<br>The Components of a Game..................................................................16<br>Section 1: Initialization....................................................................17<br>Section 2: Enter Game Loop............................................................17<br>Section 3: Retrieve Player Input ......................................................17<br>Section 4: Perform AI and Game Logic ..........................................17<br>Section 5: Render Next Frame ........................................................18<br>Section 6: Synchronize Display ......................................................18<br>Section 7: Loop................................................................................18<br>Section 8: Shutdown ........................................................................18<br>General Game Programming Guidelines ..............................................21<br>Using Tools............................................................................................26<br>C/C++ Compilers ............................................................................26<br>2D Art Software................................................................................26<br>Sound Processing Software..............................................................26<br>3D Modelers ....................................................................................26<br>Music and MIDI Sequencing Programs ..........................................27<br>Setting Up to Get Down—Using the Compiler ....................................27<br>An Example: FreakOut..........................................................................29<br>Summary................................................................................................46<br>2 The Windows Programming Model 47<br>The Genesis of Windows ......................................................................48<br>Early Windows Versions ..................................................................48<br>Windows 3.x ....................................................................................48<br>Windows 95......................................................................................49<br>Windows 98......................................................................................50<br>Windows NT ....................................................................................50<br>Basic Windows Architecture:Win9X/NT........................................50<br>Multitasking and Multithreading ..........................................................51<br>Getting Info on the Threads ............................................................52<br>The Event Model..............................................................................53<br><br>0072313618 FM 10/26/99 9:32 AM Page vi<br>vi<br>TRICKS OF THE WINDOWS GAME PROGRAMMING GURUS <br>Programming the Microsoft Way: Hungarian Notation........................55<br>Variable Naming ..............................................................................56<br>Function Naming..............................................................................56<br>Type and Constant Naming..............................................................57<br>Class Naming....................................................................................57<br>Parameter Naming............................................................................58<br>The World’s Simplest Windows Program..............................................58<br>It All Begins with <br>WinMain() ..........................................................59<br>Dissecting the Program....................................................................60<br>Choosing a Message Box ................................................................63<br>Real-World Windows Applications (Without Puck)..............................66<br>The Windows Class ..............................................................................66<br>Registering the Windows Class ............................................................74<br>Creating the Window ............................................................................75<br>The Event Handler ................................................................................77<br>The Main Event Loop............................................................................84<br>Making a Real-Time Event Loop..........................................................89<br>Opening More Windows........................................................................90<br>Summary................................................................................................93<br>3 Advanced Windows Programming 95<br>Using Resources....................................................................................96<br>Putting Your Resources Together ....................................................98<br>Using Icon Resources ......................................................................99<br>Using Cursor Resources ................................................................102<br>Creating String Table Resources....................................................106<br>Using Sound <br>.WAV Resources ........................................................108<br>Last, But Not Least—Using the Compiler to Create <br>.RC Files ....114<br>Working with Menus ..........................................................................116<br>Creating a Menu ............................................................................116<br>Loading a Menu..............................................................................119<br>Responding to Menu Event Messages ..........................................122<br>Introduction to GDI ............................................................................128<br>The <br>WM_PAINT Message Once Again ..............................................128<br>Video Display Basics and Color....................................................133<br>RGB and Palletized Modes............................................................135<br>Basic Text Printing ........................................................................137<br>Handling Important Events..................................................................143<br>Window Manipulation....................................................................143<br>Banging on the Keyboard ..............................................................150<br>Squeezing the Mouse......................................................................158<br>Sending Messages Yourself ................................................................161<br>Summary..............................................................................................163<br><br>0072313618 FM 10/26/99 9:32 AM Page vii<br>vii<br>CONTENTS<br>4 Windows GDI, Controls, and Last-Minute Gift Ideas 165<br>Advanced GDI Graphics......................................................................166<br>Under the Hood with the Graphics Device Context......................166<br>Color, Pens, and Brushes................................................................167<br>Working with Pens ........................................................................168<br>Painting with Brushes ....................................................................172<br>Points, Lines, Polygons, and Circles ..................................................173<br>Straight to the Point........................................................................173<br>Getting a Line on Things................................................................175<br>Getting Rectangular........................................................................177<br>Round and Round She Goes—Circles ..........................................180<br>Polygon, Polygon,Wherefore Art Thou, Polygon? ......................181<br>More on Text and Fonts ......................................................................182<br>Timing Is Everything ..........................................................................184<br>The <br>WM_TIMER Message ..................................................................184<br>Low-Level Timing..........................................................................187<br>Playing with Controls..........................................................................190<br>Buttons............................................................................................191<br>Sending Messages to Child Controls ............................................195<br>Getting Information ............................................................................197<br>The T3D Game Console......................................................................205<br>Summary..............................................................................................210<br>PART II DirectX and 2D Fundamentals 211<br>5 DirectX Fundamentals and the Dreaded COM 213<br>DirectX Primer ....................................................................................214<br>The HEL and HAL ........................................................................216<br>The DirectX Foundation Classes in Depth ....................................216<br>COM: Is It the Work of Microsoft… or Demons?..............................218<br>What Exactly Is a COM Object?....................................................219<br>More on Interface IDs and GUIDs ................................................223<br>Building a Quasi-COM Object ......................................................224<br>A Quick Recap of COM ................................................................226<br>A Working COM Program ............................................................226<br>Working with DirectX COM Objects..................................................231<br>COM and Function Pointers ..........................................................232<br>Creating and Using DirectX Interfaces..........................................236<br>Querying for Interfaces..................................................................237<br>The Future of COM ............................................................................238<br>Summary..............................................................................................239<br><br>0072313618 FM 10/26/99 9:32 AM Page viii<br>viii<br>TRICKS OF THE WINDOWS GAME PROGRAMMING GURUS <br>6 First Contact: DirectDraw 241<br>The Interfaces of DirectDraw..............................................................242<br>Interface Characteristics ................................................................242<br>Using the Interfaces Together ........................................................244<br>Creating a DirectDraw Object ............................................................245<br>Error Handling with DirectDraw....................................................246<br>Getting an Interface Lift ................................................................247<br>Cooperating with Windows ................................................................250<br>Getting into the Mode of Things ........................................................255<br>The Subtleties of Color........................................................................259<br>Building a Display Surface..................................................................263<br>Creating a Primary Surface............................................................264<br>Attaching the Palette......................................................................272<br>Plotting Pixels ................................................................................272<br>Cleaning Up....................................................................................284<br>Summary..............................................................................................285<br>7 Advanced DirectDraw and Bitmapped Graphics 287<br>Working with High-Color Modes........................................................288<br>16-Bit High-Color Mode................................................................289<br>Getting the Pixel Format................................................................290<br>24/32-Bit High-Color Mode ..........................................................299<br>Double Buffering ................................................................................301<br>Surface Dynamics................................................................................307<br>Page Flipping ......................................................................................311<br>Using the Blitter ..................................................................................317<br>Using the Blitter for Memory Filling ............................................320<br>Copying Bitmaps from Surface to Surface....................................328<br>Clipper Fundamentals..........................................................................332<br>Clipping Pixels to a Viewport ........................................................332<br>Clipping Bitmaps the Hard Way....................................................334<br>Making a DirectDraw Clip with <br>IDirectDrawClipper..................339<br>Working with Bitmaps ........................................................................345<br>Loading <br>.BMP files..........................................................................345<br>Working with Bitmaps....................................................................352<br>Loading an 8-Bit Bitmap................................................................353<br>Loading a 16-Bit Bitmap................................................................354<br>Loading a 24-Bit Bitmap................................................................355<br>Last Word on Bitmaps....................................................................356<br>Offscreen Surfaces ..............................................................................356<br>Creating Offscreen Surfaces ..........................................................356<br>Blitting Offscreen Surfaces............................................................358<br>Setting Up the Blitter......................................................................359<br>Color Keys......................................................................................360<br>Source Color Keying......................................................................361<br><br>0072313618 FM 10/26/99 9:32 AM Page ix<br>ix<br>CONTENTS<br>Destination Color Keying ..............................................................364<br>Using the Blitter (Finally!) ............................................................365<br>Bitmap Rotation and Scaling ..............................................................366<br>Discrete Sampling Theory ..................................................................368<br>Color Effects........................................................................................373<br>Color Animation in 256-Color Modes ..........................................373<br>Color Rotation in 256-Color Modes..............................................379<br>Tricks with RGB Modes ................................................................381<br>Manual Color Transforms and Lookup Tables....................................381<br>The New DirectX Color and Gamma Controls Interface....................382<br>Mixing GDI and DirectX ....................................................................383<br>Getting the Lowdown on DirectDraw ................................................386<br>The Main DirectDraw Object ........................................................386<br>Surfing on Surfaces........................................................................388<br>Playing with Palettes......................................................................389<br>Using DirectDraw in Windowed Modes..............................................390<br>Drawing Pixels in a Window..........................................................392<br>Finding the Real Client Area (51)..................................................395<br>Clipping a DirectX Window ..........................................................397<br>Working with 8-Bit Windowed Modes..........................................398<br>Summary..............................................................................................400<br>8 Vector Rasterization and 2D Transformations 401<br>Drawing Lines......................................................................................402<br>Bresenham’s Algorithm..................................................................403<br>Speeding Up the Algorithm............................................................409<br>Basic 2D Clipping................................................................................411<br>Computing the Intersection of Two Lines Using the Point <br>Slope Form ..................................................................................413<br>Computing the Intersection of Two Lines Using <br>the General Form ........................................................................416<br>Computing the Intersection of Two Lines Using <br>the Matrix Form ..........................................................................416<br>Clipping the Line............................................................................419<br>The Cohen-Sutherland Algorithm..................................................420<br>Wireframe Polygons............................................................................427<br>Polygon Data Structures ................................................................428<br>Drawing and Clipping Polygons....................................................430<br>Transformations in the 2D Plane ........................................................432<br>Translation......................................................................................433<br>Rotation..........................................................................................435<br>Scaling............................................................................................445<br>Introduction to Matrices......................................................................446<br>The Identity Matrix........................................................................448<br>Matrix Addition..............................................................................449<br><br>0072313618 FM 10/26/99 9:32 AM Page x<br>x<br>TRICKS OF THE WINDOWS GAME PROGRAMMING GURUS <br>Matrix Multiplication ....................................................................449<br>Transformations Using Matrices....................................................452<br>Translation ..........................................................................................454<br>Scaling..................................................................................................455<br>Rotation................................................................................................455<br>Solid Filled Polygons ..........................................................................458<br>Types of Triangles and Quadrilaterals............................................459<br>Drawing Triangles and Quadrilaterals............................................461<br>Triangular Deconstruction Details ................................................464<br>The General Case of Rasterizing a Quadrilateral..........................472<br>Triangulating Quads ......................................................................473<br>Collision Detection with Polygons......................................................478<br>Proximity AKA Bounding Sphere/Circle ......................................478<br>Bounding Box ................................................................................481<br>Point Containment..........................................................................484<br>More on Timing and Synchronization ................................................486<br>Scrolling and Panning..........................................................................488<br>Page Scrolling Engines ..................................................................488<br>Homogeneous Tile Engines............................................................489<br>Sparse Bitmap Tile Engines ..........................................................494<br>Fake 3D Isometric Engines..................................................................496<br>Method 1: Cell-Based, Totally 2D ................................................496<br>Method 2: Full-Screen-Based, with 2D or 3D <br>Collision Networks......................................................................498<br>Method 3: Using Full 3D Math, with a Fixed Camera View ........500<br>The T3DLIB1 Library ........................................................................500<br>The Engine Architecture ................................................................500<br>Basic Definitions............................................................................501<br>Working Macros ............................................................................502<br>Data Types and Structures..............................................................503<br>Global Domination ........................................................................506<br>The DirectDraw Interface ..............................................................507<br>2D Polygon Functions....................................................................511<br>2D Graphic Primitives....................................................................513<br>Math and Error Functions..............................................................517<br>Bitmap Functions............................................................................519<br>Palette Functions............................................................................522<br>Utility Functions ............................................................................525<br>The BOB (Blitter Object) Engine........................................................527<br>Summary..............................................................................................535<br>9 Uplinking with DirectInput and Force Feedback 537<br>The Input Loop Revisited....................................................................538<br>DirectInput Overture............................................................................539<br>The Components of DirectInput ....................................................541<br>The General Steps for Setting Up DirectInput ..............................542<br><br>0072313618 FM 10/26/99 9:32 AM Page xi<br>xi<br>CONTENTS<br>Data Acquisition Modes ................................................................544<br>Creating the Main DirectInput Object............................................544<br>The 101-Key Control Pad ..............................................................546<br>Problem During Reading: Reacquisition........................................554<br>Trapping the Mouse........................................................................556<br>Working the Joystick......................................................................561<br>Massaging Your Input ....................................................................576<br>Going Deeper with Force Feedback....................................................579<br>The Physics of Force Feedback......................................................580<br>Setting Up Force Feedback............................................................580<br>A Force Feedback Demo................................................................581<br>Writing a Generalized Input System:<br>T3DLIB2.CPP ............................582<br>The T3D Library at a Glance ........................................................588<br>Summary..............................................................................................588<br>10 Sounding Off with DirectSound and DirectMusic 589<br>Sound Programming on the PC ..........................................................589<br>And Then There Was Sound…............................................................590<br>Digital versus MIDI—Sounds Great, Less Filling..............................594<br>Digital Sound—Let the Bits Begin................................................594<br>Synthesized Sound and MIDI........................................................596<br>It’s MIDI Time!..............................................................................597<br>Sound Hardware..................................................................................598<br>Wave Table Synthesis ....................................................................598<br>Wave Guide Synthesis....................................................................598<br>Digital Recording: Tools and Techniques............................................599<br>Recording Sounds ..........................................................................600<br>Processing Your Sounds ................................................................600<br>DirectSound on the Mic ......................................................................601<br>Starting Up DirectSound......................................................................602<br>Understanding the Cooperation Level............................................604<br>Setting the Cooperation Level........................................................605<br>Primary and Secondary Sound Buffers ..............................................606<br>Working with Secondary Buffers ..................................................606<br>Creating Secondary Sound Buffers................................................607<br>Writing Data to Secondary Buffers................................................610<br>Rendering Sounds................................................................................612<br>Playing a Sound..............................................................................612<br>Stopping a Sound............................................................................612<br>Controlling the Volume..................................................................612<br>Freaking with the Frequency..........................................................613<br>Panning in 3D ................................................................................614<br>Making DirectSound Talk Back..........................................................614<br>Reading Sounds from Disk..................................................................616<br>The <br>.WAV Format ............................................................................616<br>Reading <br>.WAV Files ........................................................................617<br><br>0072313618 FM 10/26/99 9:32 AM Page xii<br>xii<br>TRICKS OF THE WINDOWS GAME PROGRAMMING GURUS <br>DirectMusic: The Great Experiment ..................................................622<br>DirectMusic Architecture ....................................................................622<br>Starting Up DirectMusic......................................................................624<br>Initializing COM............................................................................624<br>Creating the Performance ..............................................................625<br>Adding a Port to the Performance..................................................626<br>Loading a MIDI Segment....................................................................626<br>Creating the Loader........................................................................627<br>Loading the MIDI File ..................................................................627<br>Manipulating MIDI Segments ............................................................630<br>Playing a MIDI Segment................................................................630<br>Stopping a MIDI Segment..............................................................631<br>Checking the Status of a MIDI Segment ......................................631<br>Releasing a MIDI Segment............................................................631<br>Shutting Down DirectMusic ..........................................................631<br>A Little DirectMusic Example ......................................................632<br>The <br>T3DLIB3 Sound and Music Library ..............................................632<br>The Header ....................................................................................633<br>The Types ......................................................................................633<br>Global Domination ........................................................................634<br>The DirectSound API Wrapper......................................................635<br>The DirectMusic API Rapper—Get It?..........................................640<br>Summary..............................................................................................643<br>PART III Hard Core Game Programming 645<br>11 Algorithms, Data Structures, Memory Management, <br>and Multithreading 647<br>Data Structures ....................................................................................648<br>Static Structures and Arrays ..........................................................648<br>Linked Lists....................................................................................649<br>Algorithmic Analysis ..........................................................................657<br>Recursion ............................................................................................659<br>Trees ....................................................................................................662<br>Building BSTs................................................................................666<br>Searching BSTs..............................................................................668<br>Optimization Theory............................................................................671<br>Using Your Head ............................................................................671<br>Mathematical Tricks ......................................................................672<br>Fixed-Point Math............................................................................673<br>Unrolling the Loop ........................................................................677<br>Look-Up Tables..............................................................................678<br>Assembly Language ......................................................................679<br>Making Demos ....................................................................................680<br>Prerecorded Demos........................................................................680<br>AI-Controlled Demos ....................................................................682<br><br>0072313618 FM 10/26/99 9:32 AM Page xiii<br>xiii<br>CONTENTS<br>Strategies for Saving the Game ..........................................................682<br>Implementing Multiple Players ..........................................................683<br>Taking Turns ..................................................................................683<br>Split-Screen Setups ........................................................................684<br>Multithreaded Programming Techniques ............................................685<br>Multithreaded Programming Terminology ....................................686<br>Why Use Threads in a Game?........................................................687<br>Conjuring a Thread from the Plasma Pool ....................................689<br>Sending Messages from Thread to Thread ....................................697<br>Waiting for the Right Moment ......................................................702<br>Multithreading and DirectX ..........................................................709<br>Advanced Multithreading ..............................................................711<br>Summary..............................................................................................711<br>12 Making Silicon Think with Artificial Intelligence 713<br>Artificial Intelligence Primer ..............................................................714<br>Deterministic AI Algorithms................................................................715<br>Random Motion..............................................................................716<br>Tracking Algorithms ......................................................................717<br>Anti-Tracking: Evasion Algorithms ..............................................722<br>Patterns and Basic Control Scripting ..................................................722<br>Basic Patterns ................................................................................723<br>Patterns with Conditional Logic Processing..................................727<br>Modeling Behavioral State Systems....................................................729<br>Elementary State Machines............................................................730<br>Adding More Robust Behaviors with Personality..........................734<br>Modeling Memory and Learning with Software ................................736<br>Planning and Decision Trees ..............................................................740<br>Coding Plans ..................................................................................742<br>Implementing a Real Planner ........................................................745<br>Pathfinding ..........................................................................................747<br>Trial and Error................................................................................748<br>Contour Tracing..............................................................................749<br>Collision Avoidance Tracks............................................................749<br>Waypoint Pathfinding ....................................................................750<br>A Racing Example..........................................................................753<br>Robust Pathfinding ........................................................................754<br>Advanced AI Scripting ........................................................................759<br>Designing the Scripting Language ................................................759<br>Using the C/C++ Compiler............................................................762<br>Artificial Neural Networks..................................................................767<br>Genetic Algorithms..............................................................................770<br>Fuzzy Logic ........................................................................................772<br>Normal Set Theory ........................................................................773<br>Fuzzy Set Theory............................................................................774<br><br>0072313618 FM 10/26/99 9:32 AM Page xiv<br>xiv<br>TRICKS OF THE WINDOWS GAME PROGRAMMING GURUS <br>Fuzzy Linguistic Variables and Rules............................................776<br>Fuzzy Manifolds and Membership ................................................779<br>Fuzzy Associative Matrices............................................................783<br>Processing the FAM with the Fuzzified Inputs..............................787<br>Warm and Fuzzy ............................................................................794<br>Building Real AI for Games................................................................794<br>Summary..............................................................................................795<br>13 Playing God: Basic Physics Modeling 797<br>Fundamental Laws of Physics ............................................................798<br>Mass (m) ........................................................................................799<br>Time (t) ..........................................................................................799<br>Position (s)......................................................................................800<br>Velocity (v) ....................................................................................802<br>Acceleration (a)..............................................................................804<br>Force (F) ........................................................................................807<br>Forces in Higher Dimensions ........................................................808<br>Momentum (P)................................................................................809<br>The Physics of Linear Momentum: Conservation and Transfer ........810<br>Modeling Gravity Effects....................................................................813<br>Modeling a Gravity Well................................................................815<br>Modeling Projectile Trajectories....................................................818<br>The Evil Head of Friction....................................................................821<br>Basic Friction Concepts..................................................................821<br>Friction on an Inclined Plane (Advanced) ....................................823<br>Basic Ad Hoc Collision Response ......................................................828<br>Simple x,y Bounce Physics............................................................828<br>Computing the Collision Response with Planes of <br>Any Orientation ..........................................................................830<br>An Example of Vector Reflection..................................................834<br>Intersection of Line Segments........................................................835<br>Real 2D Object-to-Object Collision Response (Advanced)................841<br>Resolving the n-t Coordinate System..................................................846<br>Simple Kinematics ..............................................................................853<br>Solving the Forward Kinematic Problem ......................................854<br>Solving the Inverse Kinematic Problem ........................................858<br>Particle Systems ..................................................................................859<br>What Every Particle Needs ............................................................859<br>Designing a Particle Engine ..........................................................860<br>The Particle Engine Software ........................................................861<br>Generating the Initial Conditions ..................................................866<br>Putting the Particle System Together ............................................869<br>Playing God: Constructing Physics Models for Games......................870<br>Data Structures for Physics Modeling............................................870<br>Frame-Based Versus Time-Based Modeling..................................871<br>Summary..............................................................................................873<br><br>0072313618 FM 10/26/99 9:32 AM Page xv<br>xv<br>CONTENTS<br>14 Putting It All Together: You Got Game! 875<br>The Initial Design of Outpost..............................................................876<br>The Story........................................................................................876<br>Designing the Gameplay................................................................877<br>The Tools Used to Write the Game ....................................................877<br>The Game Universe: Scrolling in Space..............................................878<br>The Player’s Ship: “The Wraith” ........................................................880<br>The Asteroid Field ..............................................................................882<br>The Enemies........................................................................................884<br>The Outposts ..................................................................................885<br>The Predator Mines........................................................................886<br>The Gunships..................................................................................888<br>The Power-Ups....................................................................................891<br>The HUDS ..........................................................................................892<br>The Particle System ............................................................................896<br>Playing the Game ................................................................................896<br>Compiling Outpost ..............................................................................897<br>Compilation Files ..........................................................................897<br>Runtime Files..................................................................................898<br>Epilogue ..............................................................................................898<br>P<br>ART IV Appendixes 901<br>A What’s on the CD 903<br>B Installing DirectX and Using the C/C++ Compiler 907<br>Using the C/C++ Compiler..................................................................908<br>C Math and Trigonometry Review 911<br>Trigonometry ......................................................................................911<br>Vectors..................................................................................................915<br>Vector Length ................................................................................916<br>Normalization ................................................................................917<br>Scalar Multiplication......................................................................917<br>Vector Addition ..............................................................................918<br>Vector Subtraction..........................................................................919<br>The Inner Product, or the “Dot” Product ......................................919<br>The Cross Product..........................................................................921<br>The Zero Vector..............................................................................923<br>Position Vectors..............................................................................923<br>Vectors as Linear Combinations ....................................................924<br><br>0072313618 FM 10/26/99 9:32 AM Page xvi<br>xvi<br>TRICKS OF THE WINDOWS GAME PROGRAMMING GURUS <br>D C++ Primer 925<br>What Is C++? ......................................................................................925<br>The Minimum You Need to Know About C++ ..................................928<br>New Types, Keywords, and Conventions............................................929<br>Comments ......................................................................................929<br>Constants........................................................................................929<br>Referential Variables ......................................................................929<br>Creating Variables On-the-Fly........................................................930<br>Memory Management..........................................................................931<br>Stream I/O............................................................................................932<br>Classes..................................................................................................934<br>The New Struct in Town ................................................................934<br>Just a Simple Class ........................................................................935<br>Public Versus Private......................................................................936<br>Class Member Functions (A.K.A. Methods) ................................937<br>Constructors and Destructors ........................................................938<br>Writing a Constructor ....................................................................939<br>Writing a Destructor ......................................................................941<br>The Scope Resolution Operator ..........................................................943<br>Function and Operator Overloading....................................................945<br>Summary..............................................................................................947<br>E Game Programming Resources 949<br>Game Programming Sites....................................................................949<br>Download Points..................................................................................950<br>2D/3D Engines ....................................................................................950<br>Game Programming Books..................................................................951<br>Microsoft DirectX Multimedia Exposition..........................................951<br>Usenet Newsgroups ............................................................................951<br>Keeping Up with the Industry: Blues News........................................952<br>Game Development Magazines ..........................................................952<br>Game Web Site Developers ................................................................953<br>Xtreme Games LLC ............................................................................953<br>F ASCII Tables 955<br>Index 961
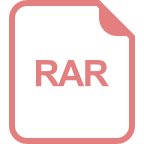
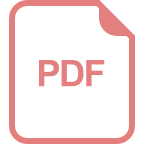
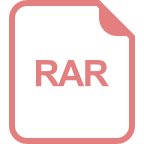
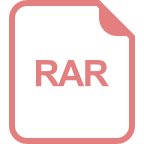
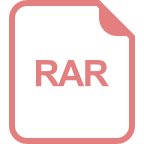
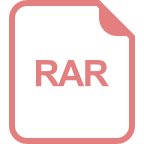
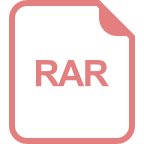
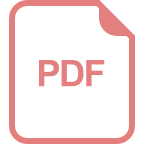
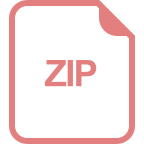
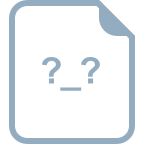
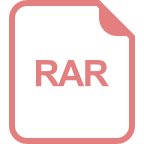
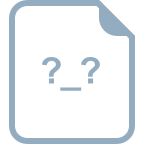
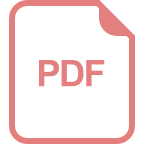
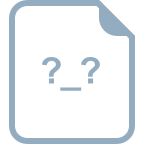
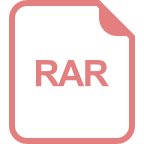
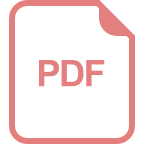
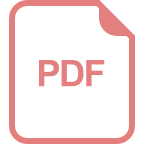
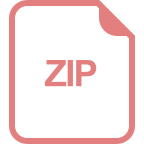
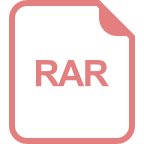
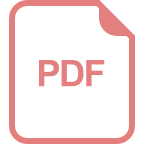
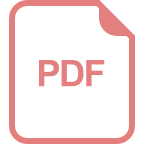
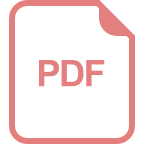
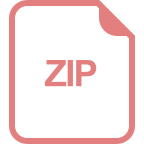
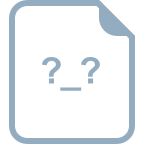
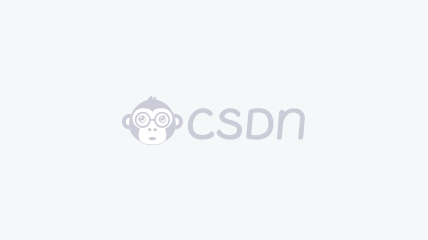
- tempins2012-03-03这本书内容很一般,英文读起来也不方便,没啥必要看

- 粉丝: 180
- 资源: 683
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

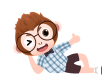
