#include <C8051F120.h> // SFR declarations
#include "hmi_user_uart.h"
sfr16 TMR3 = 0xcc; // Timer3
#define TC_20MS 36864 // 定时器在 11.0592MHz/12时对应于
// 20ms的嘀答数
#define BAUDRATE 115200 // Baud rate of UART in bps
// SYSTEMCLOCK = System clock frequency in Hz
#define SYSTEMCLOCK 22118400L
sfr16 RCAP4 = 0xca; // Timer4 capture/reload
sfr16 TMR4 = 0xcc; // Timer4
/************************************************************************
函数定义
************************************************************************/
void OSCILLATOR_Init (void);
void PORT_Init (void);
void UART0_Init (void);
void ADC_init (void);
void Timer3_init (void);
/************************************************************************
全局变量
************************************************************************/
int i,j;
long temp_temp; // 临时温度值
long temperature; // 以百分之一度表示的温度值
//unsigned idata temp[16]; // 温度采样值的循环缓冲区
//int temp_ptr; // 指向 temp[]的指针
int temp_int; // 温度值的整数部分
int temp_frac; // 温度值的小数部分(以百分之一度为单位)
unsigned char number[7];
int main()
{
WDTCN = 0XDE;
WDTCN = 0XAD; //关开门狗必须注意
OSCILLATOR_Init (); //初始化晶振
PORT_Init (); //初始化I/O口
UART0_Init(); //初始化串口
Timer3_init (); //初始化定时器3
ADC_init (); //初始化AD
number[5]='d';
number[6]='u';
number[2]='.';
EA=1; //开总中断
while (1)
{
for (i=0; i < 256; i++);
EA=0; //在进行数据转换时并不进行中断响应
temp_int = temperature / 100;
temp_frac = temperature - (temp_int * 100);
number[0] = (temp_int-temp_int%10)/10+48; //十位
number[1] = temp_int%10+48; //个位
number[3] = (temp_frac-temp_frac%10)/10+48; //小数点后第一位
number[4] = temp_frac%10+48; //小数点后第二位
DisText(80,50,1,2,number); //向屏幕发送温度数据
EA=1;
}
}
/*****************************************************************
振荡器初始化
*****************************************************************/
void OSCILLATOR_Init (void)
{
int i; // Software timer
char SFRPAGE_SAVE = SFRPAGE; // Save Current SFR page
SFRPAGE = CONFIG_PAGE; // Set SFR page
OSCICN = 0x80; // Set internal oscillator to run
// at its slowest frequency
CLKSEL = 0x00; // Select the internal osc. as
// the SYSTEMCLOCK source
// Initialize external crystal oscillator to use 22.1184 MHz crystal,初始化外部晶体振荡器,使用22.1184 MHz晶体
OSCXCN = 0x67; // Enable external crystal osc.
CLKSEL = 0x01; //输出为SYSCLK时不分频,SYSCLK来自外部晶体振荡器
for (i=0; i < 256; i++); // Wait at least 1ms
//OSCXCN = OSCXCN|0x80 ;
while (!(OSCXCN & 0x80)); // Wait for crystal osc to settle
SFRPAGE = LEGACY_PAGE;
FLSCL |= 0x30; // Initially set FLASH read timing for
// 100MHz SYSTEMCLOCK (most conservative
// setting)
if (SYSTEMCLOCK <= 25000000) {
// Set FLASH read timing for <=25MHz
FLSCL &= ~0x30;
} else if (SYSTEMCLOCK <= 50000000) {
// Set FLASH read timing for <=50MHz
FLSCL &= ~0x20;
} else if (SYSTEMCLOCK <= 75000000) {
// Set FLASH read timing for <=75MHz
FLSCL &= ~0x10;
} else { // set FLASH read timing for <=100MHz
FLSCL &= ~0x00;
}
// Start PLL for 50MHz operation
/* SFRPAGE = PLL0_PAGE;
PLL0CN = 0x04; // Select EXTOSC as clk source
PLL0CN |= 0x01; // Enable PLL power
PLL0DIV = 0x01; // Divide by 4
PLL0FLT &= ~0x0f;
PLL0FLT |= 0x01; // Set Loop Filt for (22/4)MHz input clock
PLL0FLT &= ~0x30; // Set ICO for 30-60MHz
PLL0FLT |= 0x00;
PLL0MUL = 0x04; // Multiply by 9
// wait at least 5us
for (i = 0; i < 256; i++) ;
PLL0CN |= 0x02; // Enable PLL
while (PLL0CN & 0x10 == 0x00); // Wait for PLL to lock
*/
SFRPAGE = CONFIG_PAGE;
CLKSEL = 0x01; // Select PLL as SYSTEMCLOCK source
SFRPAGE = SFRPAGE_SAVE; // Restore SFRPAGE
}
/*****************************************************************
I/O口交叉开关设置(即I/O口复用设置)
*****************************************************************/
void PORT_Init (void)
{
char SFRPAGE_SAVE = SFRPAGE; // Save Current SFR page
SFRPAGE = CONFIG_PAGE; // Set SFR page
XBR0 = 0x04;
XBR1 = 0x04;
XBR2 = 0x40;
P0MDOUT = 0xf3; // Set TX pin to push-pull
P0=0xff;
P1MDIN=
P1MDOUT |= 0x40; // Set P1.6(LED) to push-pull
P2MDOUT |= 0x00; // Set P2 to output
P2=0xff;
P3MDOUT |= 0xff;
P4MDOUT |= 0xff; //sram
P5MDOUT |= 0xff;
P6MDOUT |= 0x00;
P6=0xff;
// P6MDOUT |= 0xff;
P7MDOUT |= 0xff;
SFRPAGE = SFRPAGE_SAVE; // Restore SFR page
}
/*****************************************************************
串口初始化设置
*****************************************************************/
void UART0_Init (void)
{
char SFRPAGE_SAVE;
SFRPAGE_SAVE = SFRPAGE; // Preserve SFRPAGE
SFRPAGE = TMR4_PAGE;
TMR4CN = 0x00; // Timer in 16-bit auto-reload up timer
// mode
TMR4CF = 0x08; // SYSCLK is time base; no output;
// up count only
RCAP4 = - ((long) SYSTEMCLOCK/BAUDRATE/16);
TMR4 = RCAP4;
TR4= 1; // Start Timer2
SFRPAGE = UART0_PAGE;
SCON0 = 0x50; // 8-bit variable baud rate; MODE 1
// 9th bit ignored; RX enabled
// clear all flags
SSTA0 = 0x1f; // Clear all flags; enable baud rate
// doubler (not relevant for these
// timers);
// Use Timer2 as RX and TX baud rate
// source;
ES0 = 0; // enable uart0 interrupt
//IP |= 0x10; // set uart0 as high priority
SFRPAGE = SFRPAGE_SAVE; // Restore SFRPAGE
}
/*****************************************************************
允许 ADC;定时器3溢出启动转换;允许ADC中断
*****************************************************************/
void ADC_init (void)
{
char SFRPAGE_SAVE = SFRPAGE; // Save Current SFR page
SFRPAGE = ADC0_PAGE; // Set SFR page
AD0EN = 0; // 禁止 ADC
REF0CN = 0x07; // 允许温度传感器,片内偏置发生器
// 和偏置输出缓冲器
AMX0SL = 0x0f; // 选择温度传感器为ADC多路开�
temp.rar_c8051f120_c8051f120 温度_c8051f120温度_大彩_大彩串口屏
版权申诉
3 浏览量
2022-07-14
21:55:28
上传
评论
收藏 31KB RAR 举报

钱亚锋
- 粉丝: 86
- 资源: 1万+
最新资源
- rainy-day.jpg
- IMG_20240501_171218.jpg
- Swift-内购封装swift版本
- 经典CNN网络之ResNet 图像分类网络实战项目:7种小麦叶片病害分类(迁移学习)
- Java毕设之ssm010基于ssm的新能源汽车在线租赁管理系统+vue.rar
- Java毕设之ssm009毕业生就业信息统计系统+vue.rar
- Java毕设之ssm008医院门诊挂号系统+jsp.rar
- Java毕设之ssm007亚盛汽车配件销售业绩管理统+jsp.rar
- Java毕设之ssm006基于java的少儿编程网上报名系统+vue.rar
- Java毕设之ssm005基于SSM框架的购物商城系统+jsp.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


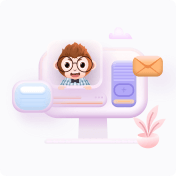