#include "rinex.h"
#include "sv.h"
#define ASHTECH 1
#define TRIMBLE 2
#define NAVSYMM 3
#define MOTOROLA 4
#define NOVATEL 5
#define EG_ASCII 0
#define EG_BINARY 1
/* globe varition */
RinexObsHeaderType rinex_obs_header;
char err_str[64]; /* string containing error message */
int WeekNo;
int MeasType[9];
/* each 10 words (3 bytes per word) corresponding to words 1-10
of subframes 1-3 of the satellite nav message. */
u1 sub1[10][3];
u1 sub2[10][3];
u1 sub3[10][3];
int EphPrn;
u1 hex_ephemeris[24][3] ;
/* [word][byte] : 24 words (3 bytes per word) corresponding to words 3-10
of subframes 1-3 of the satellite nav message. */
double tphase[3][8];
double F1[3][8],F2[3][8];
int Count1 = 0, Count2 =0;
/* programm describe */
int Ashtech2Rinex(char *SourceFile,char *ObsFile);
int Ashtech21Rinex(char *SourceFile,char *NavFile);
int ReadAshEpoch(FILE *f,int numobs,RinexEpochType *RinexEpoch);
int ReadAshEph(FILE *f,SVText *eph);
void Navsymm21Rinex(char *EPPFile,char *RinexNavFile);
int ReadNavsymmEph( FILE *f1,SVText *snv );
int Navsymm2Rinex(char *SourceFile,char *RinexFile);
int ReadNavstarEpoch(FILE *fname, RinexEpochType *RinexEpoch);
int Trimble2Rinex(char *SourceFile,char *RinexObsFile,char *RinexNavFile);
int ReadType11(FILE *fs);
int ReadType17(FILE *fs, RinexEpochType *Epoch);
int ReadType3(int len,FILE *fs,SVText far *eph,FILE *fn);
int ReadType21(int len,FILE *fs, SVText *eph,FILE *fn);
int Novatel2Rinex(char *SourceFile,char *RinexFile,char *RinexNavFile);
void ReadNovatelPos(char *s, float *X,float *Y,float *Z);
void ReadNovatelEpoch(char *s,RinexEpochType *RinexEpoch);
int ReadNovatelEph(char *s,SVText *snv);
void Convert_Ephemeris(SVText *eph);
i2 Hex(i1 ASCIIvalue);
int Motorola2Rinex(char *SourceFile,char *ObsFile,char *NavFile);
void ReadMotoEpoch(unsigned char *buf,RinexEpochType *RinexEpoch);
void ReadMotoEph(u1 eg_type, char *buf,SVText *eph);
void main()
{
int Type;
char *ObsInFile,*EphInFile, str[200];
char *RinexObsFile,*RinexNavFile;
FILE *f1,*f2;
clrscr();
printf(" ****** Transfer to Rinex File ******\n");
printf(" Source File Type: 1-Ashtech 2-Trimble 4000 3-Navsymm \n");
printf(" 4-Motorola 5-Novatel \n");
do
{
printf("Please Input GPS Type:");
scanf("%i",&Type);
if((Type>5)||(Type<1))
{ printf(" Invalid Type Input,Input Again!\n");return;}
else break;
}while(1);
InitRinexObsHeader();
RinexObsFile="rinex.98o";
RinexNavFile="rinex.98n";
WeekNo=0;
/*
Type=MOTOROLA;
ObsInFile="c:\\data\\moto.dif";
*/
switch(Type)
{
case ASHTECH:
case NAVSYMM:
printf("Please Input Soure Obs File Name:");
scanf("%20s",ObsInFile);
printf("Please Input Rinex Nav File Name:");
scanf("%20s",EphInFile);
if(Type==ASHTECH) {
Ashtech21Rinex(EphInFile,RinexNavFile);
Ashtech2Rinex(ObsInFile,"temp.obs"); }
else if(Type==NAVSYMM) {
Navsymm21Rinex(EphInFile,RinexNavFile);
Navsymm2Rinex(ObsInFile,"temp.obs");}
break;
case TRIMBLE:
case MOTOROLA:
case NOVATEL:
printf("Please Input Soure Data File Name:");
scanf("%20s",ObsInFile);
if(Type==TRIMBLE) {
Trimble2Rinex(ObsInFile,"temp.obs",RinexNavFile);}
else if(Type==NOVATEL) {
Novatel2Rinex(ObsInFile,"temp.obs",RinexNavFile);}
else if(Type==MOTOROLA) {
Motorola2Rinex(ObsInFile,"temp.obs",RinexNavFile);}
break;
default:break;
}
/* format obs file */
if ((f1 = fopen(RinexObsFile, "wt+"))== NULL) {
fprintf(stderr, "Cannot open output file.\n"); return;}
if ((f2 = fopen("temp.obs", "rt"))== NULL) {
fprintf(stderr, "Cannot open temp file.\n"); return ;}
MeasType[0]=1;MeasType[1]=2;
WriteRinexObsHeaderFile(f1, &rinex_obs_header);
while(!feof(f2)) { fgets(str,2000,f2);fputs(str,f1); }
fclose(f1); fclose(f2);
}
int Ashtech2Rinex(char *SourceFile,char *ObsFile)
{
FILE *f1,*f2;
int i,flag,FirstEpoch,numobs;
RinexEpochType RinexEpoch;
struct FileHeaderStruct
{
char version[10];
unsigned char iver;
char rtype[10];
char chnver[10];
char navver[10];
int cap; /* 1=L1 only 2=L1 L2 4=range only */
long reserved;
unsigned char numobs;
char spare[42];
} Header;
if ((f1=fopen(ObsFile,"wa+"))==NULL)
{ sprintf(err_str,"can not open file \n");return 1; }
if((f2=fopen(SourceFile,"rb"))==NULL)
{ sprintf(err_str,"cannot open file \n"); return 1; }
if(fread(&Header,sizeof(struct FileHeaderStruct),1,f2)!=1)
{ sprintf(err_str,"file read error"); return 1; }
numobs=Header.numobs;
flag=0; FirstEpoch=1;
do
{
ResetRinexEpoch(&RinexEpoch);
flag = ReadAshEpoch(f2,numobs,&RinexEpoch);
if(FirstEpoch==1)
{
rinex_obs_header.StartYear=rinex_obs_header.EndYear;
rinex_obs_header.StartMonth=rinex_obs_header.EndMonth;
rinex_obs_header.StartDay=rinex_obs_header.EndDay;
rinex_obs_header.StartHour=rinex_obs_header.EndHour;
rinex_obs_header.StartMinute=rinex_obs_header.EndMinute;
rinex_obs_header.StartSecond=rinex_obs_header.EndSecond;
FirstEpoch=0;
}
WriteRinexObsEpochFile(f1,&RinexEpoch);
}while(flag==0);
fclose(f1); fclose(f2); // free(RinexEpoch);
return 0;
}
int Ashtech21Rinex(char *SourceFile,char *NavFile)
{
FILE *f, *f2;
SVText *RinexEpp;
int First;
if((f=fopen(SourceFile,"rb"))==NULL)
{ sprintf(err_str,"cannot open file \n"); return 1; }
if ((f2=fopen(NavFile,"wa"))==NULL)
{ sprintf(err_str,"can not open file \n"); return 1; }
if((RinexEpp=(SVText *)malloc(sizeof(SVText)))==NULL)
{ sprintf(err_str,"not enough mem \n"); return 1; }
WriteRinexNavHeaderFile(f2);
while(!feof(f)) {
ResetRinexEpp(RinexEpp);
ReadAshEph(f,RinexEpp);
WriteRinexNavFile( f2, RinexEpp);}
fclose(f); fclose(f2); free(RinexEpp);
return 0;
}
int ReadAshEpoch(FILE *f,int numobs,RinexEpochType *RinexEpoch)
{
long t;
int i,j;
double C = 299792458.0;
double GPStime;
struct PosStruct
{
char sitename[4];
double rcv_time,navx,navy,navz,navt,navdot;
float navxdot,navydot,navzdot;
int PDOP;
unsigned char num_sats;
}PosData;
struct SatStruct /* measurement (data) record */
{
unsigned char svprn;
unsigned char elev; /* unit degree */
unsigned char azim; /* unit 10 degree */
unsigned char chnind;
}SatData;
struct RawDataStruct
{
double raw_range;
float smth_r;
int smth_n;
unsigned char polarity;
unsigned char warning;
unsigned char goodbad;
unsigned char ireg;
unsigned char qa_phase;
long doppler;
double carphase;
}RawData[3] ;
if(fread(&PosData,sizeof(struct PosStruct),1,f)!=1){
sprintf(err_str,"file read error"); return 1; }
GPStime=PosData.rcv_time-PosData.navt/C;
rinex_obs_header.AppX=PosData.navx;
rinex_obs_header.AppY=PosData.navy;
rinex_obs_header.AppZ=PosData.navz;
for(i=0;i<PosData.num_sats;i++) {
if(fread(&SatData,sizeof(struct SatStruct),1,f)!=1) {
sprintf(err_str,"file read error"); return 1; }
for(j=0;j<numobs;j++) {
if(fread(&RawData[j],sizeof(struct RawDataStruct),1,f)!=1) {
sprintf(err_str,"file read error"); return 1; } }
RinexEpoch->satnum++;
RinexEpoch->PRN[i]=SatData.svprn;
RinexEpoch->ca_range[i]=RawData[0].raw_range *C - PosData.navt;
RinexEpoch->phase_l1[i]=RawData[0].carphase ;
RinexEpoch->P1_range[i]=RawData[1].raw_range *C - PosData.navt;
RinexEpoch->phase_l2[i]=RawData[1].carphase ;
RinexEpoch->doppler_l1[i]=RawData[0].doppler/1000
没有合适的资源?快使用搜索试试~ 我知道了~
GPS.rar_RTCM _手持
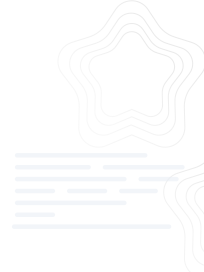
共96个文件
c:38个
exe:19个
h:13个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 197 浏览量
2022-09-24
16:09:20
上传
评论
收藏 10.38MB RAR 举报
温馨提示
本程序主要是应用嵌入式开发GPS手持模块
资源推荐
资源详情
资源评论
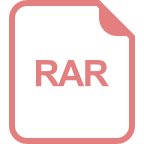
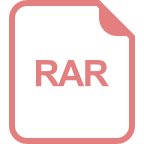
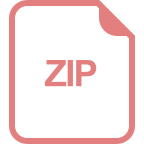
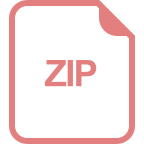
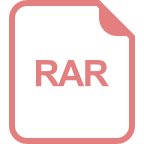
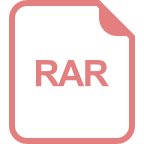
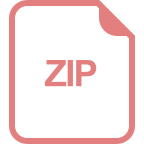
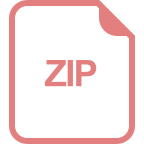
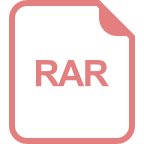
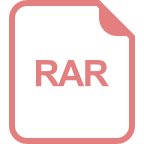
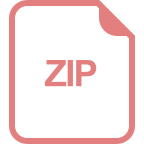
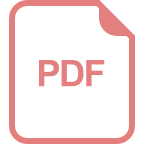
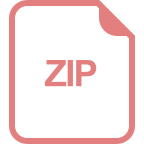
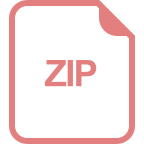
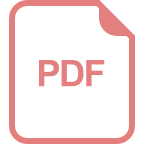
收起资源包目录



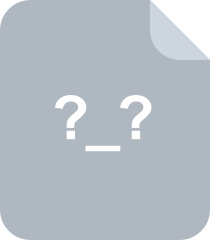
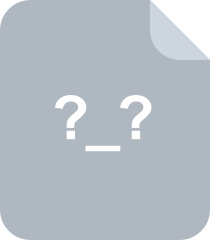
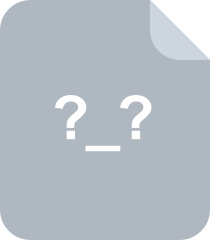
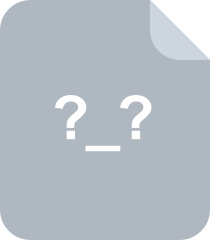
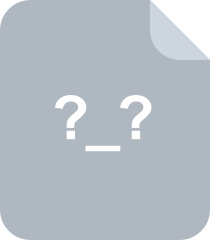
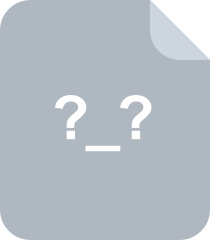
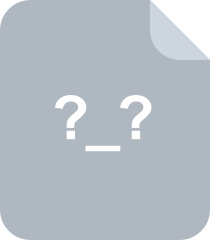
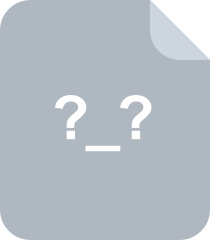
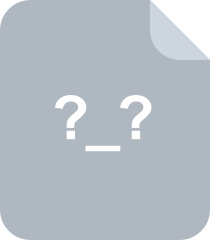
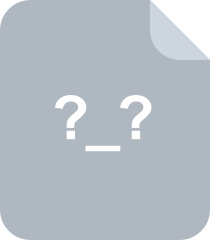
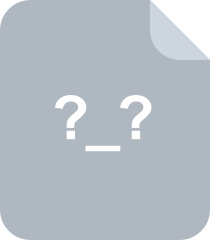
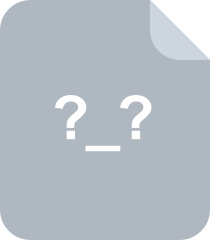
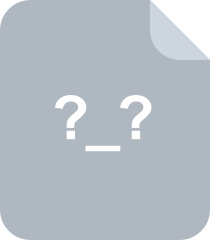
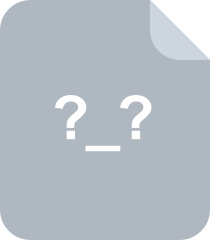
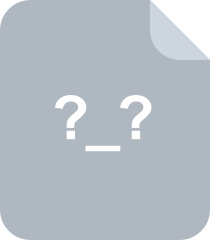
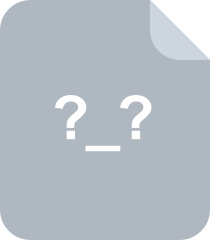
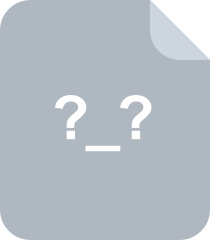
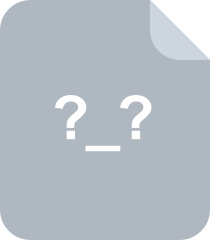
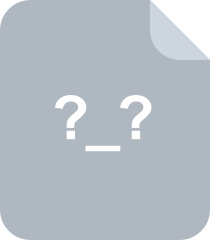
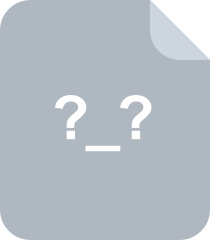
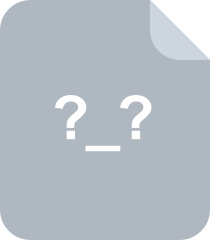
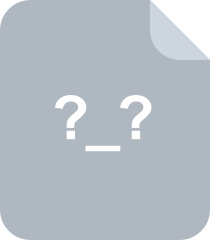
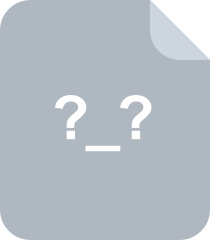
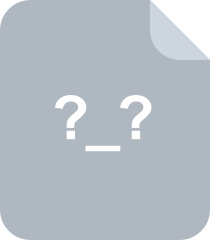
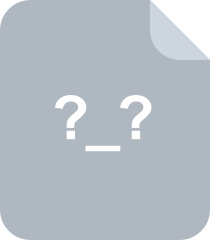
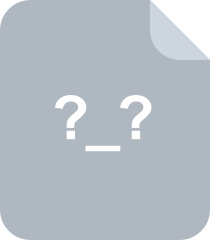
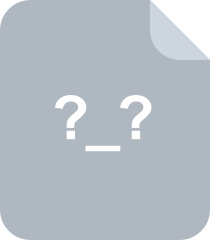
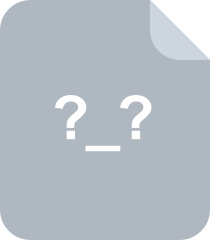
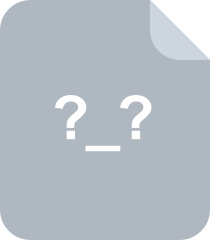
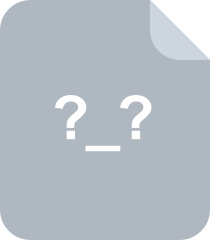
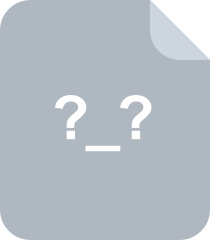
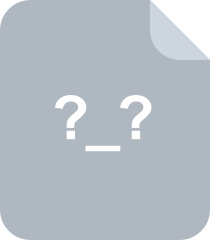
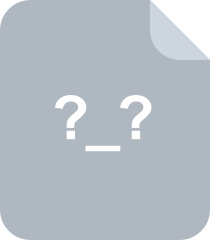
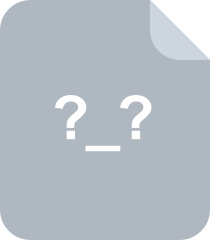
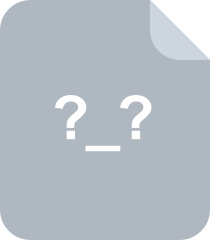
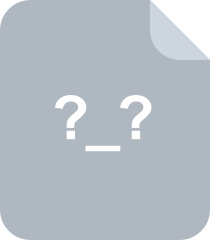
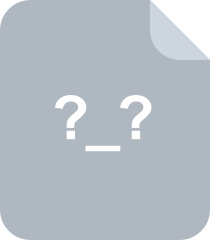
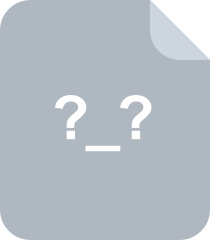

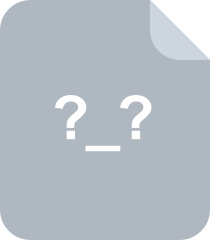
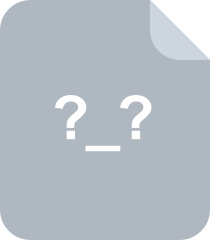
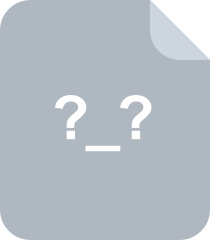
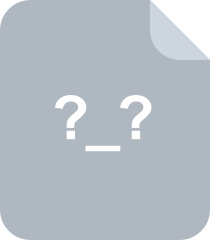
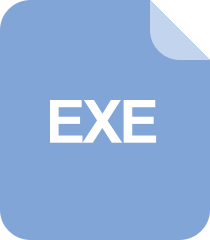
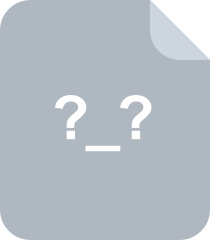
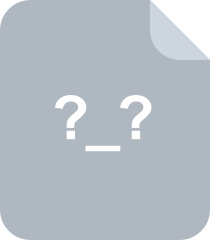
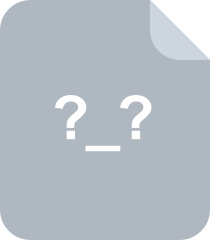
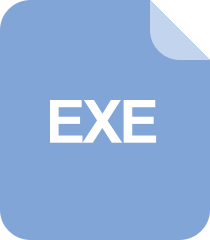
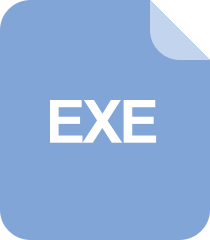
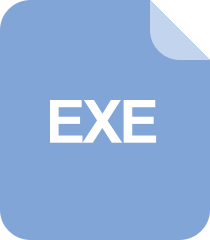
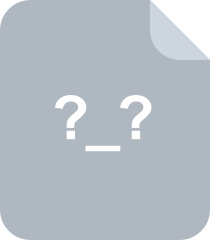
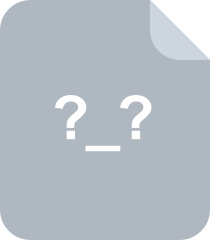
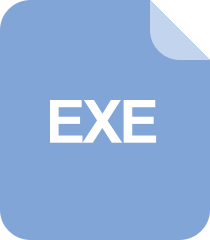
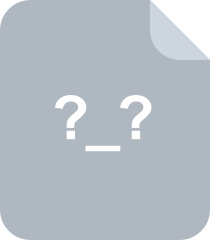
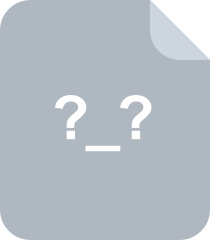
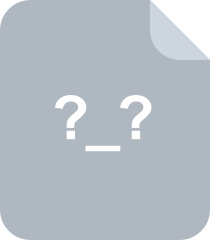
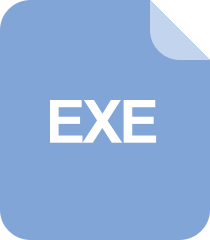
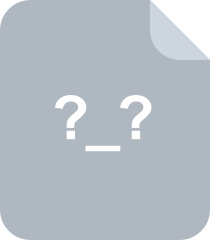
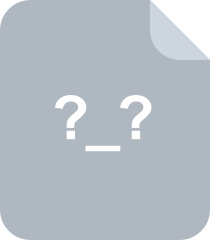
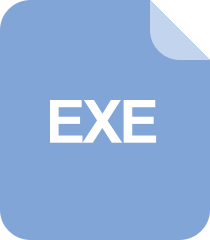
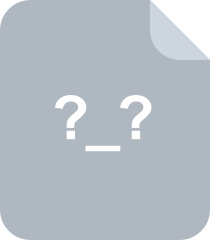
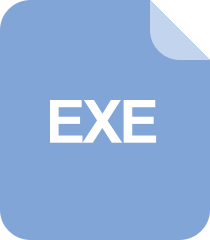
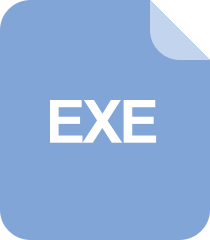
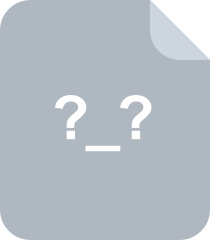
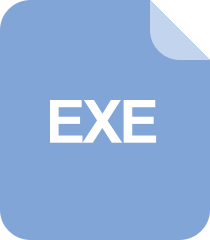
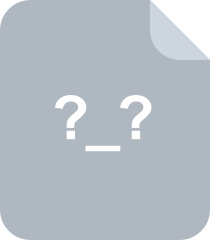
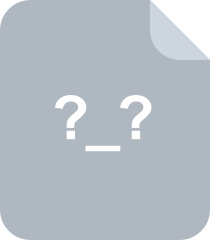
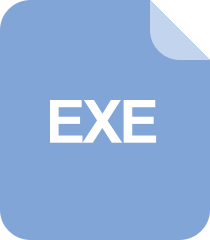
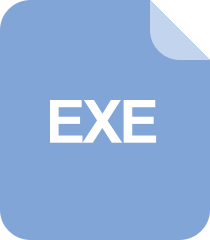
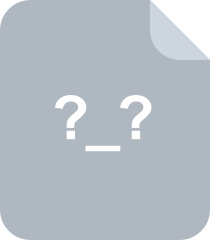
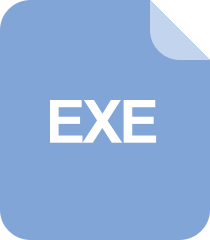
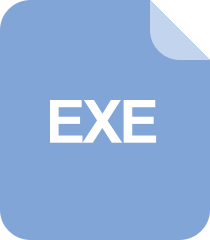
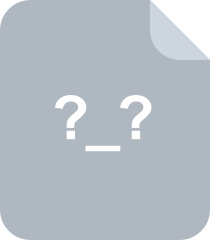
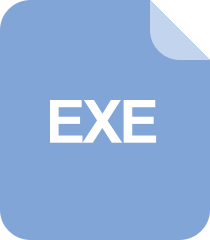
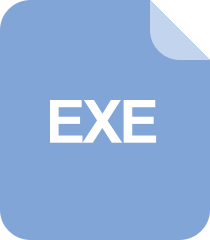
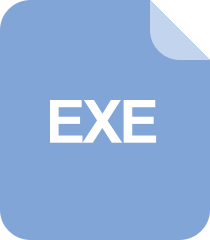
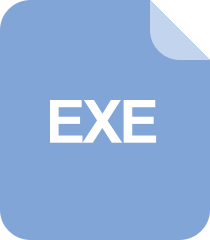
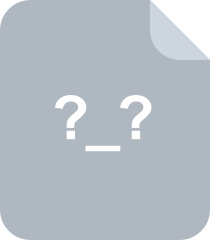
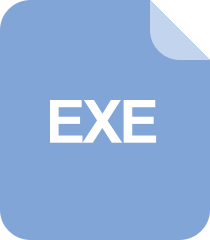

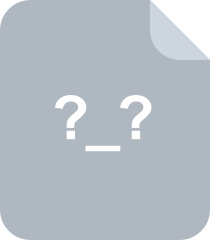
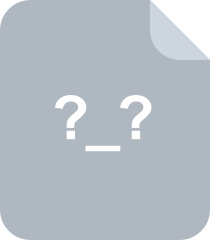
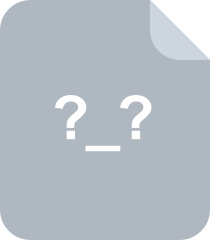
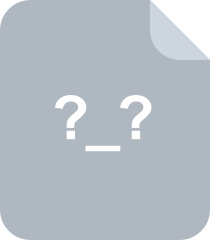
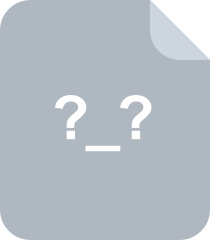
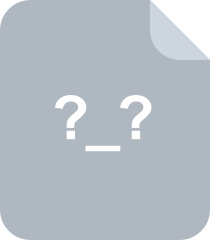
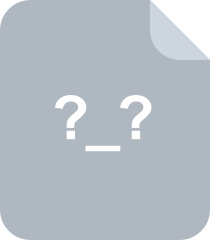
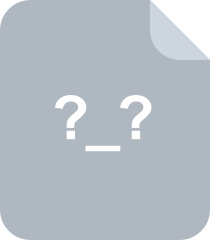
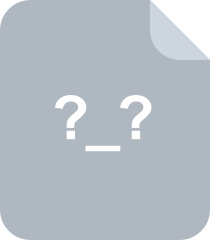
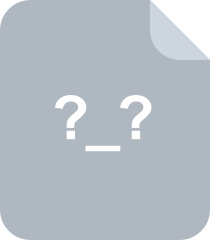
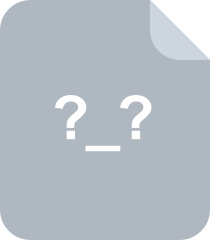
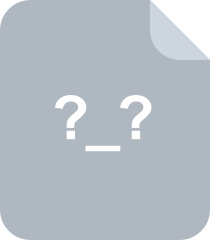
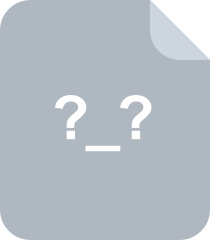
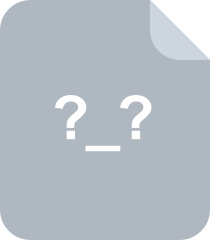

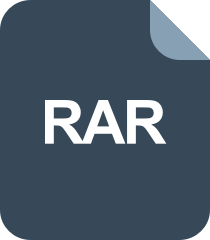
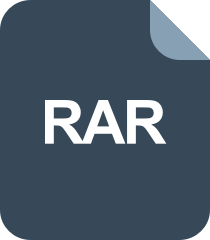
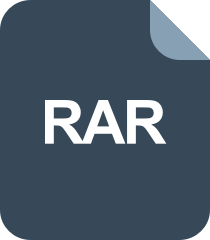
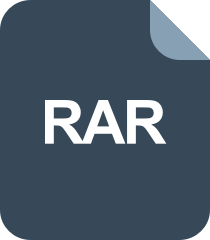
共 96 条
- 1
资源评论
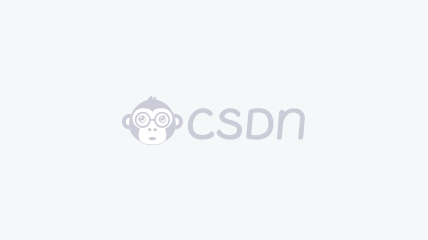

林当时
- 粉丝: 100
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

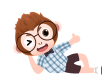
最新资源
- vue自定义指令( 复制、拖动、权限)
- json格式文件备份redis数据库 工具
- Multi-Agent-Flocking.zip
- 指标公式未来函数检测工具V1.2
- projectData
- SQL Server 性能监视器,它旨在提供开箱即用的全面监控,并作为您自己的项目或应用程序的监控框架 它在本地数据库中收集性能
- Python 程序语言设计模式思路-并发模式:线程池模式:管理线程池,优化线程创建和销毁
- 股事汇投资工具-实时新闻、财经日历、市场快讯、持仓查询、外汇兑换、换算工具、大盘云图、江恩工具、指标检测等
- webrtc-streamer
- html+css+'青春献礼二十大 红色旅游助乡村'为主题的网页设计 2022年参与学校网页设计比赛时完成的
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


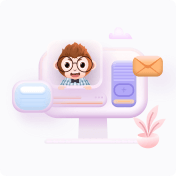
安全验证
文档复制为VIP权益,开通VIP直接复制
