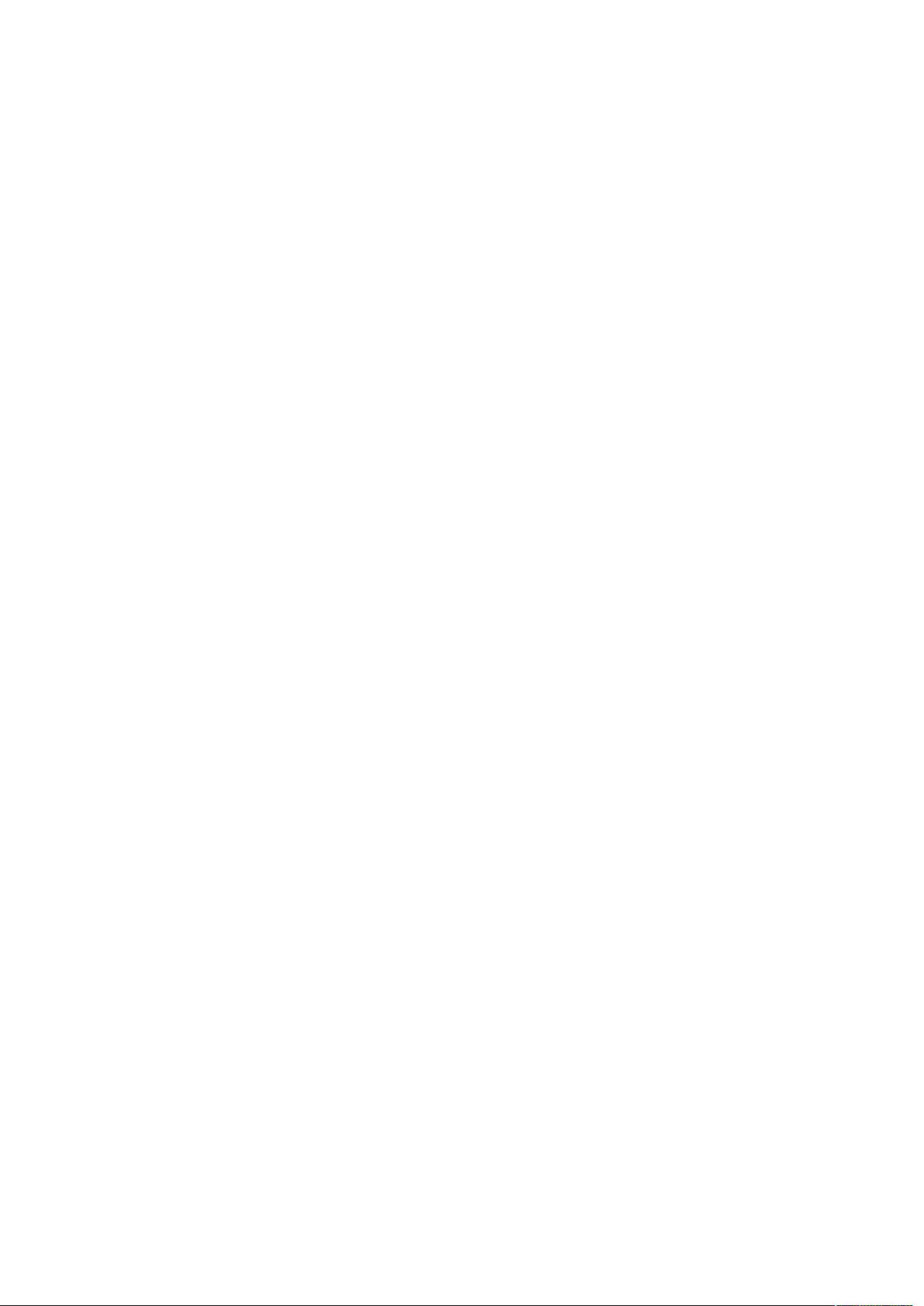
#include"stdio.h"
#include"malloc.h"
#include"time.h"
#include"dos.h"
#include"stdlib.h"
#include"string.h"
#include"fcntl.h"
#include"io.h"
#define LEN sizeof(struct PLANE)
struct time
{
int year;
int month;
int day;
int hour;
int minuter;
};
struct time1
{
int year;
int month;
};
struct PLANE
{
int planenumber;
int people;
char flyplace[15];
struct time fly;
char downplace[15];
struct time down;
int prize;
struct PLANE *next;
};
struct PASSAGE
{
int planenumber;
char ID[19];
char name[20];
char sex[6];
struct time1 csny;
int seatnumber;
struct PASSAGE *next;
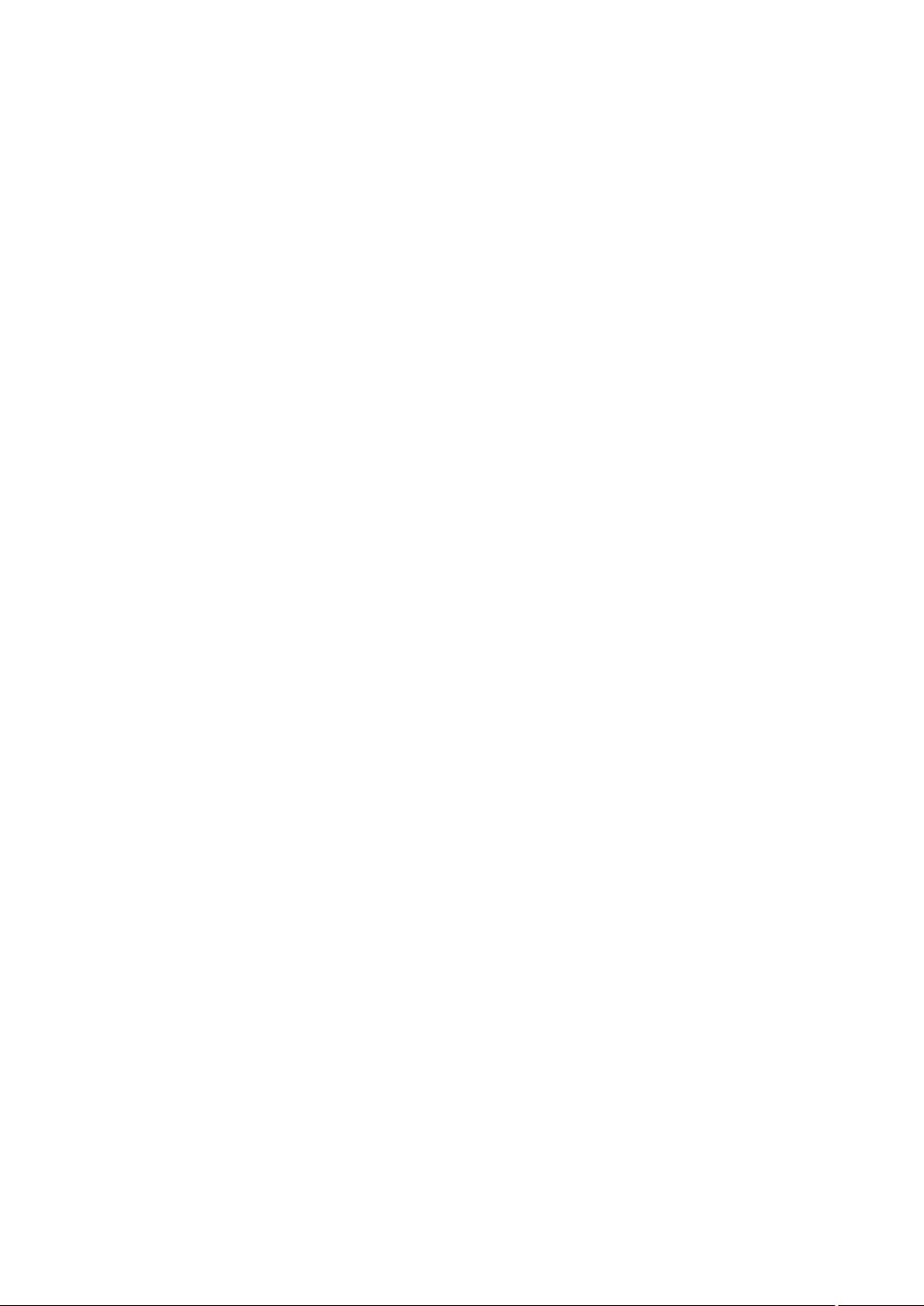
};
struct P
{
char flyplace[15];
struct PLANE *head;
struct P *next;
};
struct P *wjcl(void)//处理文件:读文件,如果文件存在,按航班的起飞地点建立不同的链表,
并返回指针 P,如果文件不存在返回 NULL
{
int fh,len=1,len1;
struct PLANE *head;
struct P *head1;
fh=open("hangbanxinxi.bin",O_RDWR|O_BINARY);
if(fh==-1)
return NULL;
else
{
struct PLANE *pt,*p2,*p3,*p4;
p3=(struct PLANE *)malloc(sizeof(struct PLANE));
len=read(fh,p3,sizeof(struct PLANE));//开始读文件,读出来的数据先做成链表
head=p4=p3; //head 指向等下用 p3 建立链表的头用于之后对读出来的数据按飞行
地点建立不同链表
if(len!=0)
{
do
{
p3=(struct PLANE *)malloc(sizeof(struct PLANE));
len=read(fh,p3,sizeof(struct PLANE));
p4->next=p3;
if(len!=0)
p4=p4->next;
}while(len!=0);
free(p3);
p4->next=NULL;
len1=close(fh);
pt=head;//对读出来的数据进行处理,按起飞地点建立不同的链表
struct P *p,*p1;
int i=1;
p=(struct P *)malloc(sizeof(struct P));
p->next=NULL;
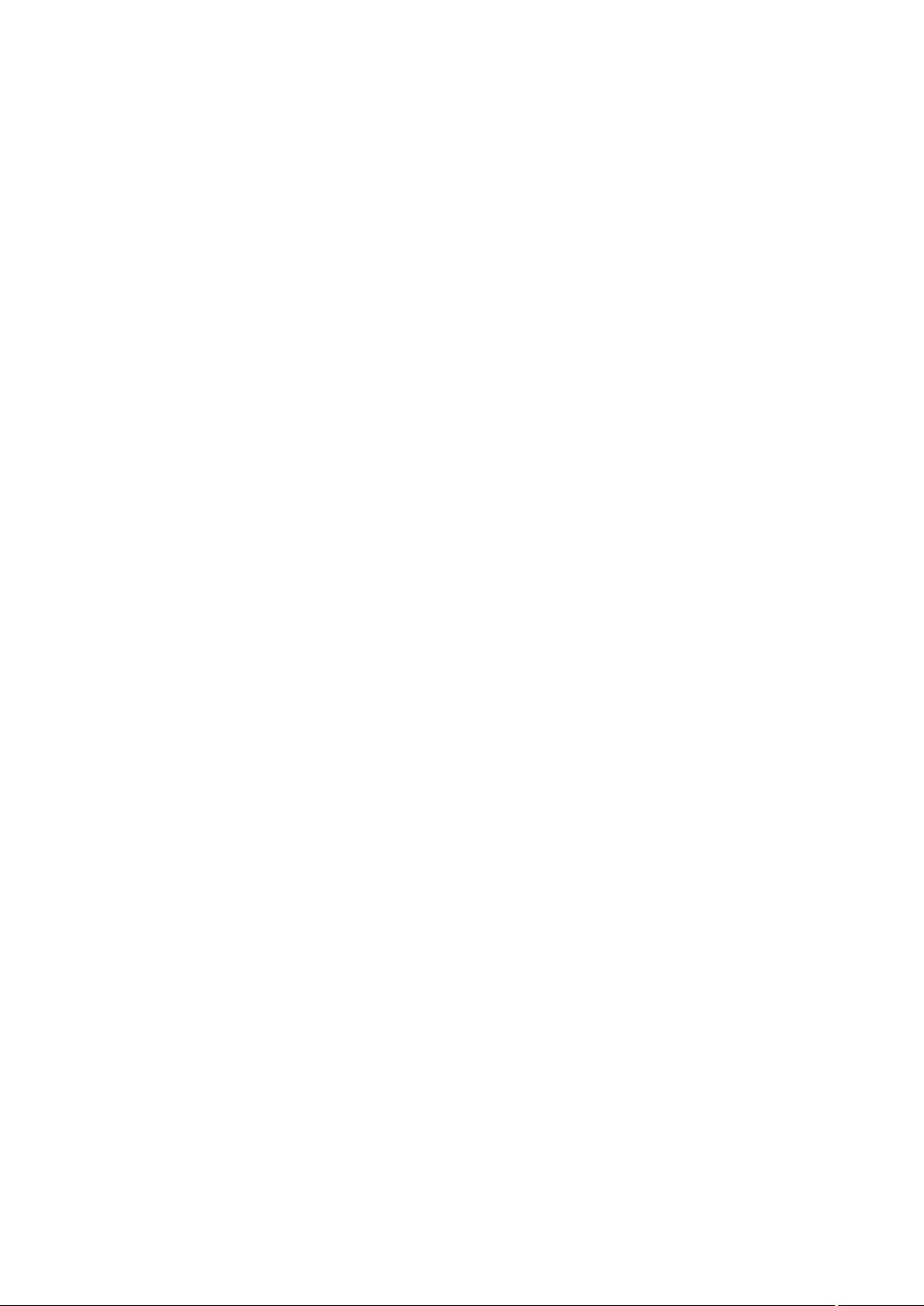
head1=p;
strcpy(head1->flyplace,head->flyplace);
head1->head=head;
for(;pt/*PLANE 指针,指向 head*/!=NULL;i=1)
{
for(p1=head1;p1!=NULL;p1=p1->next)
{
if(p1->flyplace==pt->flyplace) //如果起飞地点相同,则建立相应链
表存储文件相应信息
{
i=0;
for(p2=p1->head;p2->next!=NULL;p2=p2->next)
;
p2->next=pt;
pt=pt->next;
p2=p2->next;
p2->next=NULL;
}
}
if(i==1) //如果起飞地点没有发现匹配的,则新建链表
{
p=(struct P *)malloc(sizeof(struct P));
strcpy(p->flyplace,pt->flyplace);
p->next=NULL;
for(p1=head1;p1->next!=NULL;p1=p1->next)
;
p1->next=p;
p->head=pt;
pt=pt->next;
}
}
return head1;
}
len=close(fh);
return NULL;
}
}
void hbxx(struct PLANE *p)
{
printf("航班号:%d\n",p->planenumber);
printf("最大载客数:%d\n",p->people);
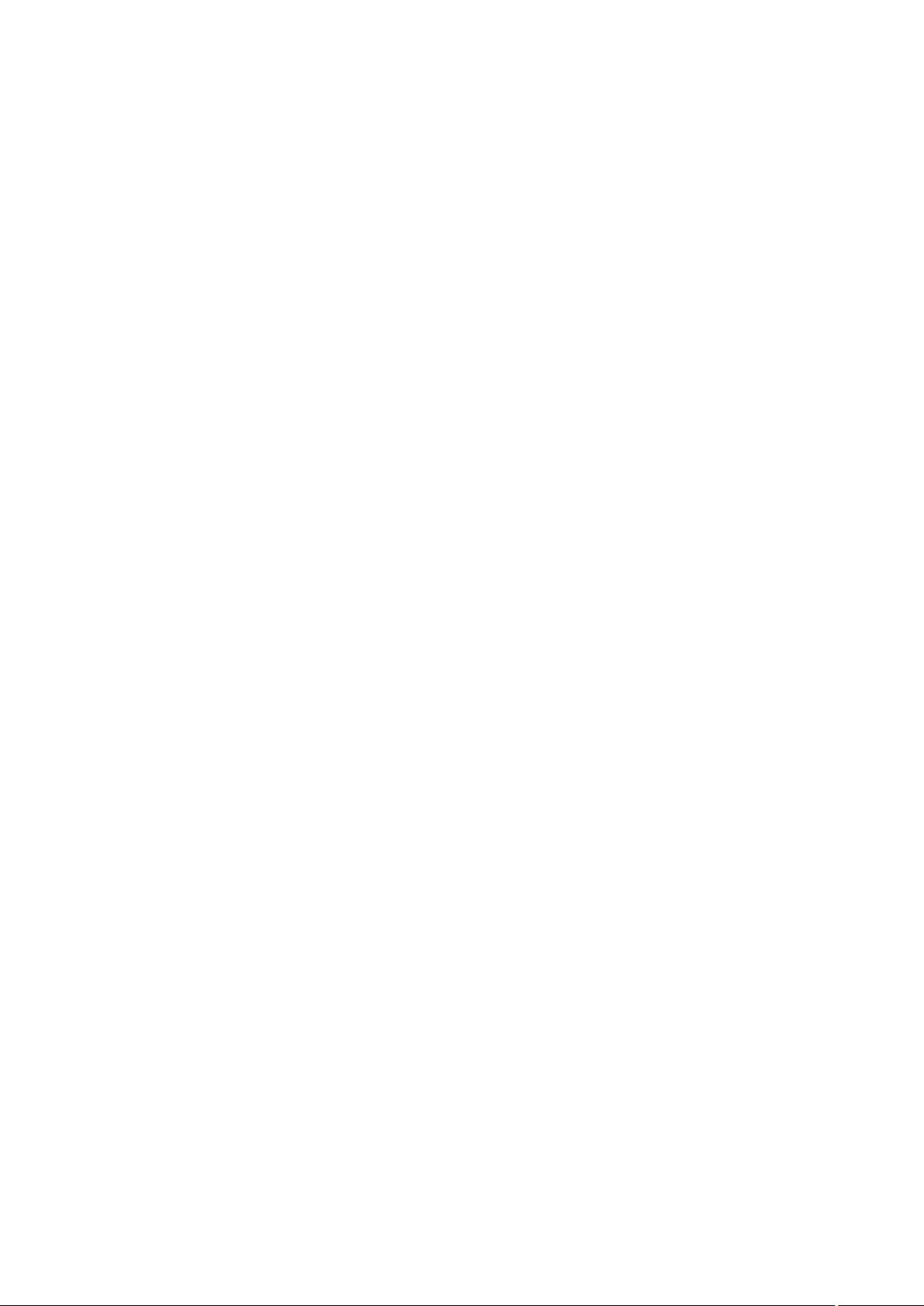
printf("起飞地点:%s\n",p->flyplace);
printf(" 起 飞 时 间 :
%d,%d,%d,%d,%d\n",p->fly.year,p->fly.month,p->fly.day,p->fly.hour,p->fly.minuter);
printf("降落地点:%s\n",p->downplace);
printf(" 降 落 时
间 :%d,%d,%d,%d,%d\n",p->down.year,p->down.month,p->down.day,p->down.hour,p->down.mi
nuter);
printf("单价:%d\n",p->prize);
}
void zjhb() //增加航班
{
int n=1,len1,fh;
struct P *head1,*p2;
struct PLANE *p,*p1;
head1=wjcl();
if(head1==NULL)
{
fh=open("hangbanxinxi.bin",O_RDWR|O_CREAT|O_BINARY,0666);
len1=close(fh);
head1=wjcl();
}
p=(struct PLANE *)malloc(sizeof(struct PLANE));
printf("请输入新增航班的航班号:");
scanf("%d",&p->planenumber);
for(p2=head1;p2!=NULL;p2=p2->next)
{
for(p1=p2->head;p1!=NULL;p1=p1->next)
{
if(p->planenumber==p1->planenumber)
{
printf("此航班号已存在\n");
n=0;
}
}
}
if(n==1)
{
printf("请输入新增航班的最大载客量:");
scanf("%d",&p->people);
printf("请输入新增航班的起飞地点:");
scanf("%s",&p->flyplace);
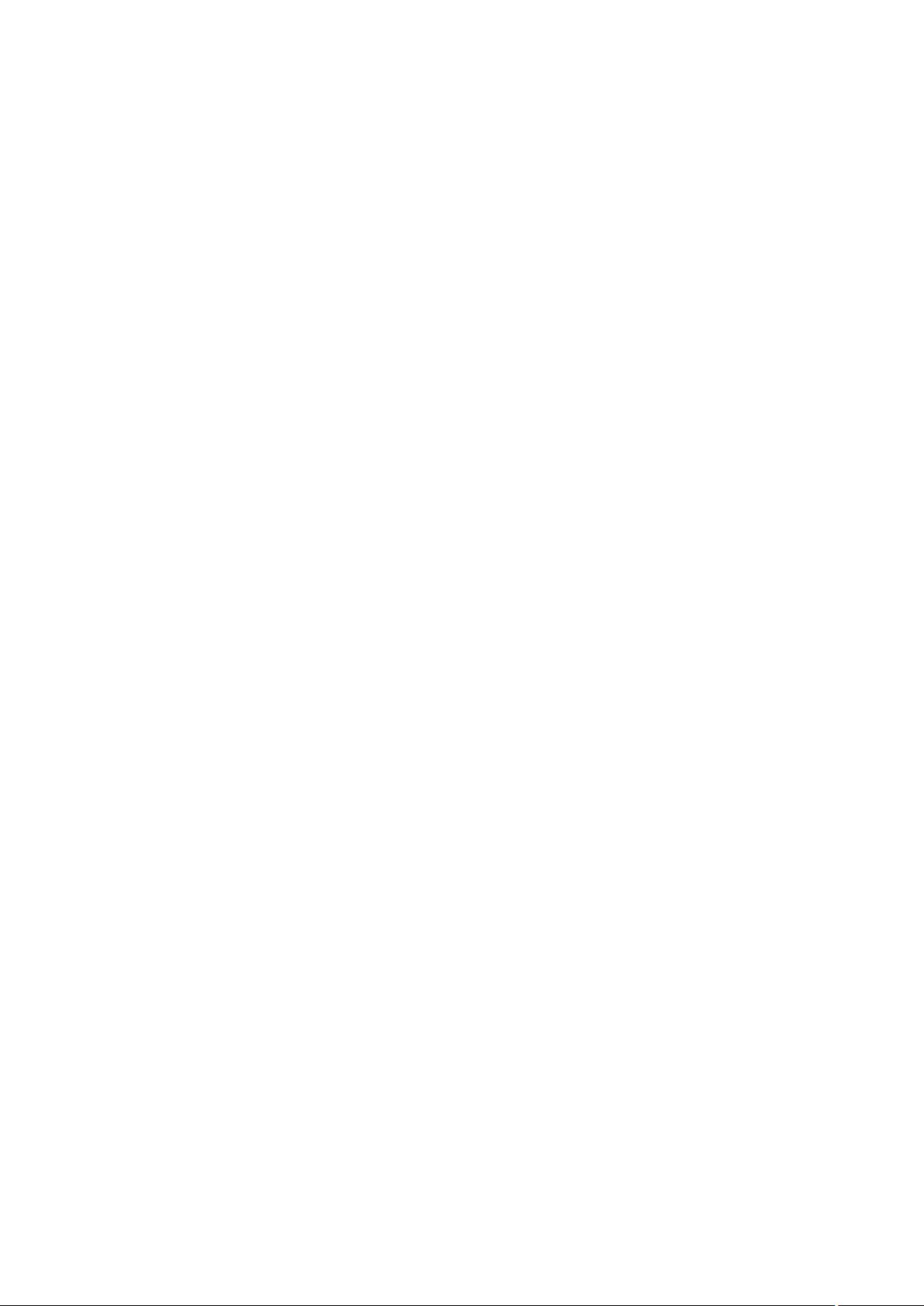
printf(" 请 输 入新 增 航 班 的 起 飞 时 间 ( 输 入 方 式 是 年 、 月 、 日 、 时 、 分 , 按
xxxx,xx,xx,xx,xx 的方式输入):");
scanf("%d,%d,%d,%d,%d",&p->fly.year,&p->fly.month,&p->fly.day,&p->fly.hour,&p->fly.
minuter);
printf("请输入新增航班的降落地点:");
scanf("%s",&p->downplace);
printf(" 请 输 入 新 增 航 班 的 降 落 时 间 ( 输 入 方 式 是 年 、 月 、 日 、 时 、 分 , 按
xxxx,xx,xx,xx,xx 的方式输入):");
scanf("%d,%d,%d,%d,%d",&p->down.year,&p->down.month,&p->down.day,&p->down.ho
ur,&p->down.minuter);
printf("请输入新增航班的票价:");
scanf("%d",&p->prize);
p->next=NULL;
for(p2=head1;p2!=NULL;p2=p2->next)
{
for(p1=p2->head;p1!=NULL;p1=p1->next)
{
if(p->people==p1->people&&strcmp(p->flyplace,p1->flyplace)==0&&(p->fly.year==p1->fl
y.year)&&(p->fly.month==p1->fly.month)&&(p->fly.day==p1->fly.day)&&(p->fly.hour==p1->f
ly.hour)&&(p->fly.minuter==p1->fly.minuter)&&strcmp(p->downplace,p1->downplace)==0&&(
p->down.year==p1->down.year)&&(p->down.month==p1->down.month)&&(p->down.day==p1-
>down.day)&&(p->down.hour==p1->down.hour)&&(p->down.minuter==p1->down.minuter))
{
n=0;
printf("此航班号已存在\n");
}
}
}
if(n==1)
{
fh=open("hangbanxinxi.bin",O_RDWR|O_APPEND|O_BINARY);
len1=write(fh,p,sizeof(struct PLANE));
printf("航班增加成功!");
len1=close(fh);
}
}
}
struct PASSAGE *qxhb(struct PASSAGE *head1)//取消航班
{