// ==============================================================
// Oracle, ODBC and DB2/CLI Template Library, Version 4.0.121,
// Copyright (C) Sergei Kuchin, 1996,2005
// Author: Sergei Kuchin
// This library is free software. Permission to use, copy,
// modify and redistribute it for any purpose is hereby granted
// without fee, provided that the above copyright notice appear
// in all copies.
// ==============================================================
#ifndef __OTL_H__
#define __OTL_H__
#if defined(OTL_INCLUDE_0)
#include "otl_include_0.h"
#endif
#define OTL_VERSION_NUMBER (0x040079L)
#include <string.h>
#include <ctype.h>
#include <stdlib.h>
#include <stdio.h>
//======================= CONFIGURATION #DEFINEs ===========================
// Uncomment the following line in order to include the OTL for ODBC:
//#define OTL_ODBC
// Uncomment the following line in order to include the OTL for
// MySQL/MyODBC:
//#define OTL_ODBC_MYSQL
// Uncomment the following line in order to include the OTL for DB2 CLI:
//#define OTL_DB2_CLI
// Uncomment the following line in order to include the OTL for
// Oracle 7:
//#define OTL_ORA7
// Uncomment the following line in order to include the OTL for
// Oracle 8:
//#define OTL_ORA8
// Uncomment the following line in order to include the OTL for
// Oracle 8i:
//#define OTL_ORA8I
// Uncomment the following line in order to include the OTL for
// Oracle 9i:
//#define OTL_ORA9I
// The macro definitions may be also turned on via C++ compiler command line
// option, e.g.: -DOTL_ODBC, -DOTL_ORA7, -DOTL_ORA8, -DOTL_ORA8I, -DOTL_ODBC_UNIX
// -DOTL_ODBC_MYSQL, -DOTL_DB2_CLI
#if defined(OTL_STREAM_LEGACY_BUFFER_SIZE_TYPE)
typedef short int otl_stream_buffer_size_type;
#else
typedef int otl_stream_buffer_size_type;
#endif
#if defined(OTL_IODBC_BSD)
#define OTL_ODBC
#define OTL_ODBC_UNIX
#endif
#if defined(OTL_ODBC_POSTGRESQL)
#define OTL_ODBC
#endif
// Comment out this #define when using pre-ANSI C++ compiler
#if !defined(OTL_ODBC_zOS) && !defined (OTL_ANSI_CPP)
#define OTL_ANSI_CPP
#endif
#if defined(OTL_ODBC_zOS)
#define OTL_ODBC_UNIX
#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#endif
#ifdef OTL_ORA8I
#define OTL_ORA8
#define OTL_ORA8_8I_REFCUR
#define OTL_ORA8_8I_DESC_COLUMN_SCALE
#endif
#if defined(OTL_ORA10G)||defined(OTL_ORA10G_R2)
#define OTL_ORA9I
#endif
#ifdef OTL_ORA9I
#define OTL_ORA8
#define OTL_ORA8_8I_REFCUR
#define OTL_ORA8_8I_DESC_COLUMN_SCALE
#endif
#ifdef OTL_ODBC_MYSQL
#define OTL_ODBC
#endif
#ifdef OTL_ODBC_XTG_IBASE6
#define OTL_ODBC
#endif
#define OTL_VALUE_TEMPLATE
//#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#if defined(OTL_ODBC_UNIX) && !defined(OTL_ODBC)
#define OTL_ODBC
#endif
#if defined(OTL_BIND_VAR_STRICT_TYPE_CHECKING_ON)
#define OTL_CHECK_BIND_VARS \
if(strcmp(type_arr,"INT")==0|| \
strcmp(type_arr,"UNSIGNED")==0|| \
strcmp(type_arr,"SHORT")==0|| \
strcmp(type_arr,"LONG")==0|| \
strcmp(type_arr,"FLOAT")==0|| \
strcmp(type_arr,"DOUBLE")==0|| \
strcmp(type_arr,"TIMESTAMP")==0|| \
strcmp(type_arr,"TZ_TIMESTAMP")==0|| \
strcmp(type_arr,"LTZ_TIMESTAMP")==0|| \
strcmp(type_arr,"BIGINT")==0|| \
strcmp(type_arr,"CHAR")==0|| \
strcmp(type_arr,"CHARZ")==0|| \
strcmp(type_arr,"DB2DATE")==0|| \
strcmp(type_arr,"DB2TIME")==0|| \
strcmp(type_arr,"VARCHAR_LONG")==0|| \
strcmp(type_arr,"RAW_LONG")==0|| \
strcmp(type_arr,"CLOB")==0|| \
strcmp(type_arr,"BLOB")==0|| \
strcmp(type_arr,"NCHAR")==0|| \
strcmp(type_arr,"NCLOB")==0|| \
strcmp(type_arr,"REFCUR")==0) \
; \
else \
return 0;
#else
#define OTL_CHECK_BIND_VARS
#endif
// ------------------- Namespace generation ------------------------
#ifdef OTL_EXPLICIT_NAMESPACES
#ifdef OTL_DB2_CLI
#define OTL_ODBC_NAMESPACE_BEGIN namespace db2 {
#define OTL_ODBC_NAMESPACE_PREFIX db2::
#define OTL_ODBC_NAMESPACE_END }
#else
#define OTL_ODBC_NAMESPACE_BEGIN namespace odbc {
#define OTL_ODBC_NAMESPACE_PREFIX odbc::
#define OTL_ODBC_NAMESPACE_END }
#endif
#define OTL_ORA7_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA7_NAMESPACE_PREFIX oracle::
#define OTL_ORA7_NAMESPACE_END }
#define OTL_ORA8_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA8_NAMESPACE_PREFIX oracle::
#define OTL_ORA8_NAMESPACE_END }
#else
// Only one OTL is being intantiated
#if defined(OTL_ODBC)&&!defined(OTL_ORA8)&& \
!defined(OTL_ORA7)&&!defined(OTL_DB2_CLI) \
|| !defined(OTL_ODBC)&&defined(OTL_ORA8)&& \
!defined(OTL_ORA7)&&!defined(OTL_DB2_CLI) \
|| !defined(OTL_ODBC)&&!defined(OTL_ORA8)&& \
defined(OTL_ORA7)&&!defined(OTL_DB2_CLI) \
|| !defined(OTL_ODBC)&&!defined(OTL_ORA8)&& \
!defined(OTL_ORA7)&&defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEGIN
#define OTL_ODBC_NAMESPACE_PREFIX
#define OTL_ODBC_NAMESPACE_END
#define OTL_ORA7_NAMESPACE_BEGIN
#define OTL_ORA7_NAMESPACE_PREFIX
#define OTL_ORA7_NAMESPACE_END
#define OTL_ORA8_NAMESPACE_BEGIN
#define OTL_ORA8_NAMESPACE_PREFIX
#define OTL_ORA8_NAMESPACE_END
#endif
// ================ Combinations of two OTLs =========================
#if defined(OTL_ODBC) && defined(OTL_ORA7) && \
!defined(OTL_ORA8) && !defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEGIN namespace odbc{
#define OTL_ODBC_NAMESPACE_PREFIX odbc::
#define OTL_ODBC_NAMESPACE_END }
#define OTL_ORA7_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA7_NAMESPACE_PREFIX oracle::
#define OTL_ORA7_NAMESPACE_END }
#define OTL_ORA8_NAMESPACE_BEGIN
#define OTL_ORA8_NAMESPACE_PREFIX
#define OTL_ORA8_NAMESPACE_END
#endif
#if defined(OTL_ODBC) && !defined(OTL_ORA7) && \
defined(OTL_ORA8) && !defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEGIN namespace odbc{
#define OTL_ODBC_NAMESPACE_PREFIX odbc::
#define OTL_ODBC_NAMESPACE_END }
#define OTL_ORA8_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA8_NAMESPACE_PREFIX oracle::
#define OTL_ORA8_NAMESPACE_END }
#define OTL_ORA7_NAMESPACE_BEGIN
#define OTL_ORA7_NAMESPACE_PREFIX
#define OTL_ORA7_NAMESPACE_END
#endif
#if !defined(OTL_ODBC) && defined(OTL_ORA7) && \
!defined(OTL_ORA8) && defined(OTL_DB2_CLI)
#define OTL_ORA7_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA7_NAMESPACE_PREFIX oracle::
#define OTL_ORA7_NAMESPACE_END }
#define OTL_ORA8_NAMESPACE_BEGIN
#define OTL_ORA8_NAMESPACE_PREFIX
#define OTL_ORA8_NAMESPACE_END
#define OTL_ODBC_NAMESPACE_BEGIN namespace db2 {
#define OTL_ODBC_NAMESPACE_PREFIX db2::
#define OTL_ODBC_NAMESPACE_END }
#endif
#if !defined(OTL_ODBC) && !defined(OTL_ORA7) && \
defined(OTL_ORA8) && defined(OTL_DB2_CLI)
#define OTL_ORA8_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA8_NAMESPACE_PREFIX oracle::
#define OTL_ORA8_NAMESPACE_END }
#define OTL_ORA7_NAMESPACE_BEGIN
#define OTL_ORA7_NAMESPACE_PREFIX
#define OTL_ORA7_NAMESPACE_END
#define OTL_ODBC_NAMESPACE_BEGIN namespace db2 {
#define OTL_ODBC_NAMESPACE_PREFIX db2::
#define OTL_ODBC_NAMESPACE_END }
#endif
#endif
// -------------------- End of namespace generation -------------------
// --------------------- Invalid combinations --------------------------
#if defined(OTL_ODBC) && defined(OTL_DB2_CLI)
#error Invalid combination: OTL_ODBC && OTL_DB2_CLI together
#endif
#if defined(OTL_ORA7) && defined(OTL_ORA8)
#error Invalid combination: OTL_ORA7 && OTL_ORA8(I) together
#endif
#if (defined(OTL_ORA7) || defined(OTL_ORA8) || \
defined(OTL_ORA8I) || defined(OTL_ORA9I) ) && \
defined(OTL_BIGINT) && \
(defined(OTL_ODBC) || defined(OTL_DB2_CLI))
#error OTL_BIGINT is not supported when OTL_ORAXX and OTL_ODBC \
(or OTL_DB2_CLI) are defined together
#endif
#if defined (OTL_
otl.zip_OTL_The Oracle_c++ db2 CLI_db2 cli_otl recordset
版权申诉
110 浏览量
2022-09-19
12:53:44
上传
评论
收藏 85KB ZIP 举报

林当时
- 粉丝: 97
- 资源: 1万+
最新资源
- 蓝桥杯2024年第十五届省赛真题-前缀总分
- com.qihoo.appstore_300101305-1.apk
- tensorflow-gpu-2.7.1-cp37-cp37m-manylinux2010-x86-64.whl
- tensorflow-2.7.2-cp37-cp37m-manylinux2010-x86-64.whl
- tensorflow-2.7.1-cp39-cp39-manylinux2010-x86-64.whl
- 蓝桥杯2024年第十五届省赛真题-传送阵
- com.qihoo.appstore_300101305.apk
- linux之线程同步一.doc
- keil5配色方案10种
- python烟花代码.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


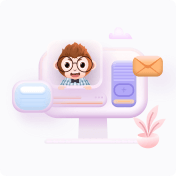
评论0