// ==============================================================
// ORACLE, ODBC and DB2/CLI Template Library, Version 4.0.187,
// Copyright (C) Sergei Kuchin, 1996,2009
// Author: Sergei Kuchin
// This library is free software. Permission to use, copy,
// modify and redistribute it for any purpose is hereby granted
// without fee, provided that the above copyright notice appear
// in all copies.
// ==============================================================
#ifndef __OTL_H__
#define __OTL_H__
#if defined(OTL_INCLUDE_0)
#include "otl_include_0.h"
#endif
#define OTL_VERSION_NUMBER (0x0400C1L)
#if defined(_MSC_VER) && (_MSC_VER >= 1400)
#pragma warning (disable:4351)
#pragma warning (disable:4290)
#define OTL_STRCAT_S(dest,dest_sz,src) strcat_s(dest,dest_sz,src)
#define OTL_STRCPY_S(dest,dest_sz,src) strcpy_s(dest,dest_sz,src)
#define OTL_STRNCPY_S(dest,dest_sz,src,count) strncpy_s(dest,dest_sz,src,count)
#else
#define OTL_STRCAT_S(dest,dest_sz,src) strcat(dest,src)
#define OTL_STRCPY_S(dest,dest_sz,src) strcpy(dest,src)
#define OTL_STRNCPY_S(dest,dest_sz,src,count) strncpy(dest,src,count)
#endif
#include <string.h>
#include <ctype.h>
#include <stdlib.h>
#include <stdio.h>
//======================= CONFIGURATION #DEFINEs ===========================
// Uncomment the following line in order to include the OTL for ODBC:
//#define OTL_ODBC
// Uncomment the following line in order to include the OTL for
// MySQL/MyODBC for MyODBC 2.5 (pretty old). Otherwise, use OTL_ODBC
//#define OTL_ODBC_MYSQL
// Uncomment the following line in order to include the OTL for DB2 CLI:
//#define OTL_DB2_CLI
// Uncomment the following line in order to include the OTL for
// Oracle 7:
//#define OTL_ORA7
// Uncomment the following line in order to include the OTL for
// Oracle 8:
//#define OTL_ORA8
// Uncomment the following line in order to include the OTL for
// Oracle 8i:
//#define OTL_ORA8I
// Uncomment the following line in order to include the OTL for
// Oracle 9i:
//#define OTL_ORA9I
// Uncomment the following line in order to include the OTL for
// Oracle 10g Release 1:
//#define OTL_ORA10G
// Uncomment the following line in order to include the OTL for
// Oracle 10g Release 2:
//#define OTL_ORA10G_R2
// Uncomment the following line in order to include the OTL for
// Oracle 11g Release 1
//#define OTL_ORA11G
// The macro definitions may be also turned on via C++ compiler command line
// option, e.g.: -DOTL_ODBC, -DOTL_ORA7, -DOTL_ORA8, -DOTL_ORA8I, -DOTL_ODBC_UNIX
// -DOTL_ODBC_MYSQL, -DOTL_DB2_CLI
// this becomes the default from version 4.0.162 and on.
// the #define is not enabled for vc++ 6.0 in version 4.0.167 and higher.
#if !defined(OTL_UNCAUGHT_EXCEPTION_ON) && !(defined(_MSC_VER)&&(_MSC_VER==1200))
#define OTL_UNCAUGHT_EXCEPTION_ON
#endif
#if defined(OTL_ORA11G)
#define OTL_ORA10G_R2
#endif
#if defined(OTL_STREAM_LEGACY_BUFFER_SIZE_TYPE)
typedef short int otl_stream_buffer_size_type;
#else
typedef int otl_stream_buffer_size_type;
#endif
#if defined(OTL_ODBC_MULTI_MODE)
#define OTL_ODBC
#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#endif
#if defined(OTL_ODBC_MSSQL_2005)
#define OTL_ODBC
#endif
#if defined(OTL_ODBC_MSSQL_2008)
#define OTL_ODBC
#define OTL_ODBC_MSSQL_2005
#endif
#if defined(OTL_IODBC_BSD)
#define OTL_ODBC
#define OTL_ODBC_UNIX
#endif
#if defined(OTL_ODBC_TIMESTEN_WIN)
#define OTL_ODBC_TIMESTEN
#define OTL_ODBC
#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#define ODBCVER 0x0250
#include <timesten.h>
#endif
#if defined(OTL_ODBC_TIMESTEN_UNIX)
#define OTL_ODBC_TIMESTEN
#define OTL_ODBC
#define OTL_ODBC_UNIX
#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#include <timesten.h>
#endif
#if defined(OTL_ODBC_ENTERPRISEDB)
#define OTL_ODBC_POSTGRESQL
#endif
#if defined(OTL_ODBC_POSTGRESQL)
#define OTL_ODBC
#endif
// Comment out this #define when using pre-ANSI C++ compiler
#if !defined(OTL_ODBC_zOS) && !defined (OTL_ANSI_CPP)
#define OTL_ANSI_CPP
#endif
#if defined(OTL_ODBC_zOS)
#define OTL_ODBC_UNIX
#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#endif
#if defined(OTL_ORA8I)
#define OTL_ORA8
#define OTL_ORA8_8I_REFCUR
#define OTL_ORA8_8I_DESC_COLUMN_SCALE
#endif
#if defined(OTL_ORA10G)||defined(OTL_ORA10G_R2)
#define OTL_ORA9I
#define OTL_ORA_NATIVE_TYPES
#endif
#if defined(OTL_ORA9I)
#define OTL_ORA8
#define OTL_ORA8_8I_REFCUR
#define OTL_ORA8_8I_DESC_COLUMN_SCALE
#endif
#if defined(OTL_ODBC_MYSQL)
#define OTL_ODBC
#endif
#if defined(OTL_ODBC_XTG_IBASE6)
#define OTL_ODBC
#endif
#define OTL_VALUE_TEMPLATE
//#define OTL_ODBC_SQL_EXTENDED_FETCH_ON
#if defined(OTL_ODBC_UNIX) && !defined(OTL_ODBC)
#define OTL_ODBC
#endif
#if defined(OTL_BIND_VAR_STRICT_TYPE_CHECKING_ON)
#define OTL_CHECK_BIND_VARS \
if(strcmp(type_arr,"INT")==0|| \
strcmp(type_arr,"UNSIGNED")==0|| \
strcmp(type_arr,"SHORT")==0|| \
strcmp(type_arr,"LONG")==0|| \
strcmp(type_arr,"FLOAT")==0|| \
strcmp(type_arr,"DOUBLE")==0|| \
strcmp(type_arr,"TIMESTAMP")==0|| \
strcmp(type_arr,"TZ_TIMESTAMP")==0|| \
strcmp(type_arr,"LTZ_TIMESTAMP")==0|| \
strcmp(type_arr,"BIGINT")==0|| \
strcmp(type_arr,"CHAR")==0|| \
strcmp(type_arr,"CHARZ")==0|| \
strcmp(type_arr,"DB2DATE")==0|| \
strcmp(type_arr,"DB2TIME")==0|| \
strcmp(type_arr,"VARCHAR_LONG")==0|| \
strcmp(type_arr,"RAW_LONG")==0|| \
strcmp(type_arr,"RAW")==0|| \
strcmp(type_arr,"CLOB")==0|| \
strcmp(type_arr,"BLOB")==0|| \
strcmp(type_arr,"NCHAR")==0|| \
strcmp(type_arr,"NCLOB")==0|| \
strcmp(type_arr,"REFCUR")==0) \
; \
else \
return 0;
#else
#define OTL_CHECK_BIND_VARS
#endif
// ------------------- Namespace generation ------------------------
#if defined(OTL_EXPLICIT_NAMESPACES)
#if defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEGIN namespace db2 {
#define OTL_ODBC_NAMESPACE_PREFIX db2::
#define OTL_ODBC_NAMESPACE_END }
#else
#define OTL_ODBC_NAMESPACE_BEGIN namespace odbc {
#define OTL_ODBC_NAMESPACE_PREFIX odbc::
#define OTL_ODBC_NAMESPACE_END }
#endif
#define OTL_ORA7_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA7_NAMESPACE_PREFIX oracle::
#define OTL_ORA7_NAMESPACE_END }
#define OTL_ORA8_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA8_NAMESPACE_PREFIX oracle::
#define OTL_ORA8_NAMESPACE_END }
#else
// Only one OTL is being intantiated
#if defined(OTL_ODBC)&&!defined(OTL_ORA8)&& \
!defined(OTL_ORA7)&&!defined(OTL_DB2_CLI) \
|| !defined(OTL_ODBC)&&defined(OTL_ORA8)&& \
!defined(OTL_ORA7)&&!defined(OTL_DB2_CLI) \
|| !defined(OTL_ODBC)&&!defined(OTL_ORA8)&& \
defined(OTL_ORA7)&&!defined(OTL_DB2_CLI) \
|| !defined(OTL_ODBC)&&!defined(OTL_ORA8)&& \
!defined(OTL_ORA7)&&defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEGIN
#define OTL_ODBC_NAMESPACE_PREFIX
#define OTL_ODBC_NAMESPACE_END
#define OTL_ORA7_NAMESPACE_BEGIN
#define OTL_ORA7_NAMESPACE_PREFIX
#define OTL_ORA7_NAMESPACE_END
#define OTL_ORA8_NAMESPACE_BEGIN
#define OTL_ORA8_NAMESPACE_PREFIX
#define OTL_ORA8_NAMESPACE_END
#endif
// ================ Combinations of two OTLs =========================
#if defined(OTL_ODBC) && defined(OTL_ORA7) && \
!defined(OTL_ORA8) && !defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEGIN namespace odbc{
#define OTL_ODBC_NAMESPACE_PREFIX odbc::
#define OTL_ODBC_NAMESPACE_END }
#define OTL_ORA7_NAMESPACE_BEGIN namespace oracle {
#define OTL_ORA7_NAMESPACE_PREFIX oracle::
#define OTL_ORA7_NAMESPACE_END }
#define OTL_ORA8_NAMESPACE_BEGIN
#define OTL_ORA8_NAMESPACE_PREFIX
#define OTL_ORA8_NAMESPACE_END
#endif
#if defined(OTL_ODBC) && !defined(OTL_ORA7) && \
defined(OTL_ORA8) && !defined(OTL_DB2_CLI)
#define OTL_ODBC_NAMESPACE_BEG


superlazy
- 粉丝: 28
- 资源: 18
最新资源
- 大二下学期,使用C++的MFC编写的游戏《连连看》-2025
- .NET 反编译工具 可修改代码
- TongWeb-V8.0命令行工具手册
- C++、在MFC中利用socket api制作多线程消息收-发送程序.zip
- 基于暗通道先验复原-ACE增强方法的图像去雾研究源代码
- AdvancedInstaller-16652 安装包打包工具
- C++、用于MFC添加GIF动图,并将背景设置为透明-2025
- TongWeb-V8.0REST API手册
- TongWeb-V8.0常见问题手册
- 基于MFC ActiveX的曲线、折线、柱状图绘制控件,工控行业必备 .zip
- MATLAB、有限元、悬臂梁、欧拉梁、位移求解
- TongWeb-V8.0嵌入式版-JavaEE标准容器用户指南
- uniapp 常用公共方法记录
- ROS2使用serial串口库源码读取串口数据
- TongWeb-V8.0嵌入式版-JakartaEE标准容器用户指南
- sdfsdfsdfsddddd
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


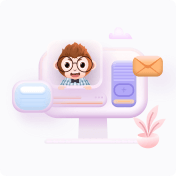
- 1
- 2
- 3
- 4
- 5
前往页