#include<gl/glut.h>
#include<windows.h>
#include<math.h>
#include <stdio.h>
#include <stdlib.h>
GLfloat light_position1[]={0,28,-20,1.0};
GLfloat model_ambient[]={0.05f,0.05f,0.05f,1.0f};
GLfloat mat_specular[]={0.8,1.0,1.0,1.0};
GLfloat mat_shininess[]={5.0};
GLfloat mat_ambient[]={0.1,0.1,0.1,1};
GLfloat white_light[]={1.0,1.0,1.0,1.0};
GLfloat light[]={1.0,1.0,1.0,1};
GLfloat light_position0[]={0,28,20,1.0};
GLint WinWidth;
GLint WinHeight;
//define the eyepoint
typedef struct EyePoint
{
GLfloat x;
GLfloat y;
GLfloat z;
}EyePoint;
EyePoint myEye;
EyePoint vPoint;
GLfloat vAngle=0;
//the function about the texture
#define BMP_Header_Length 54
void grab(void) {
FILE* pDummyFile; FILE* pWritingFile;
GLubyte* pPixelData;
GLubyte BMP_Header[BMP_Header_Length];
GLint i, j;
GLint PixelDataLength;
i = WinWidth * 3;
while( i%4 != 0 )
++i;
PixelDataLength = i * WinHeight;
pPixelData = (GLubyte*)malloc(PixelDataLength);
if( pPixelData == 0 )
exit(0);
pDummyFile = fopen("dummy.bmp", "rb");
if( pDummyFile == 0 )
exit(0);
pWritingFile = fopen("grab.bmp", "wb");
if( pWritingFile == 0 )
exit(0);
glPixelStorei(GL_UNPACK_ALIGNMENT, 4);
glReadPixels(0, 0, WinWidth, WinHeight,GL_BGR_EXT, GL_UNSIGNED_BYTE, pPixelData);
fread(BMP_Header, sizeof(BMP_Header), 1, pDummyFile);
fwrite(BMP_Header, sizeof(BMP_Header), 1, pWritingFile);
fseek(pWritingFile, 0x0012, SEEK_SET);
i = WinWidth;
j = WinHeight;
fwrite(&i, sizeof(i), 1, pWritingFile);
fwrite(&j, sizeof(j), 1, pWritingFile);
fseek(pWritingFile, 0, SEEK_END);
fwrite(pPixelData, PixelDataLength, 1, pWritingFile);
fclose(pDummyFile); fclose(pWritingFile); free(pPixelData);
}
//power of two
int power_of_two(int n)
{
if( n <= 0 )
return 0;
return (n & (n-1)) == 0;
}
/* 函数load_texture
* 读取一个BMP文件作为纹理
* 如果失败,返回0,如果成功,返回纹理编号
*/
//load texture function
GLuint load_texture(const char* file_name)
{
GLint width, height, total_bytes;
GLubyte* pixels = 0;
GLint last_texture_ID=0;
GLuint texture_ID = 0;
//打开文件,如果失败,返回
FILE* pFile = fopen(file_name, "rb");
if( pFile == 0 )
return 0;
//读取文件中图像的宽度和高度
fseek(pFile, 0x0012, SEEK_SET);
fread(&width, 4, 1, pFile);
fread(&height, 4, 1, pFile);
fseek(pFile, BMP_Header_Length, SEEK_SET);
//计算每行像素所占字节数,并根据此数据计算总像素字节数
{
GLint line_bytes = width * 3;
while( line_bytes % 4 != 0 )
++line_bytes;
total_bytes = line_bytes * height;
} //{
//根据总像素字节数分配内存
pixels = (GLubyte*)malloc(total_bytes);
if( pixels == 0 ){
fclose(pFile);
return 0;
} //if
//读取像素数据
if( fread(pixels, total_bytes, 1, pFile) <= 0 ){
free(pixels);
fclose(pFile);
return 0;
} //if
/* 在旧版本的OpenGL中
* 如果图像的宽度和高度不是整数次方,则需要进行缩放
* 这里并没有检查OpenGL版本,出于对版本兼容性的考虑,按旧版本处理
* 另外,无论旧版本还是新版本
* 当图像的宽度和高度超过当前OpenGL实现所支持的最大值时,也要进行缩放
*/
{
GLint max;
glGetIntegerv(GL_MAX_TEXTURE_SIZE, &max);
if( !power_of_two(width)|| !power_of_two(height)|| width > max|| height > max ){
//规定缩放后新的大小为边长的正方形
const GLint new_width = 256;
const GLint new_height = 256;
GLint new_line_bytes, new_total_bytes;
GLubyte* new_pixels = 0;
//计算每行需要的字节数和总字节数
new_line_bytes = new_width * 3;
while( new_line_bytes % 4 != 0 )
++new_line_bytes;
new_total_bytes = new_line_bytes * new_height;
//分配内存
new_pixels = (GLubyte*)malloc(new_total_bytes);
if( new_pixels == 0 ){
free(pixels);
fclose(pFile);
return 0;
}//if
//进行像素缩放
gluScaleImage(GL_RGB,width, height, GL_UNSIGNED_BYTE, pixels,new_width, new_height, GL_UNSIGNED_BYTE, new_pixels);
//释放原来的像素数据,把pixels指向新的像素数据,并重新设置width和height
free(pixels);
pixels = new_pixels;
width = new_width;
height = new_height;
}//if
}//{
//分配一个新的纹理编号
glGenTextures(1, &texture_ID);
if( texture_ID == 0 ) {
free(pixels);
fclose(pFile);
return 0;
} //if
//绑定新的纹理,载入纹理并设置纹理参数
//在绑定前,先获得原来绑定的纹理编号,以便在最后进程恢复
glGetIntegerv(GL_TEXTURE_BINDING_2D, &last_texture_ID);
//在分配了纹理对象编号后,使用glBingTexture函数来指定“当前所使用的纹理对象”
glBindTexture(GL_TEXTURE_2D, texture_ID);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT);
glTexEnvf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_REPLACE);
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, width, height, 0, GL_BGR_EXT, GL_UNSIGNED_BYTE, pixels);
glBindTexture(GL_TEXTURE_2D, last_texture_ID);
//之前为pixels分配的内存可在使用glTexImage2D以后释放
//因为此时像素数据已经被OpenGL另行保存了一份(可能被保存到专门的图形硬件中)
free(pixels);
return texture_ID;
}
//set the names of the texture objects 定义纹理对象的名称
GLuint texblackboard,texwindow,texwindow1,texceiling,
texdoor,texbackwall,texgaodi,textdesk,textwall,textfloor,textdesk1;
//draw the scene of the classroom
void drawscence()
{
//draw the ceiling
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, texceiling);
glColor3f(0.3,0.3,0.3);
glBegin(GL_QUADS);
glNormal3f(0.0f, -1.0f, 0.0f);
glTexCoord2f(0.0f, 0.0f);
glVertex3f(-40.0f,30.0f, 30.0f);
glTexCoord2f(0.0f, 3.0f);
glVertex3f(-40.0f, 30.0f, -30.0f);
glTexCoord2f(6.0f, 3.0f);
glVertex3f(40.0f, 30.0f, -30.0f);
glTexCoord2f(6.0f, 0.0f);
glVertex3f(40.0f, 30.0f, 30.0f);
glEnd();
glDisable(GL_TEXTURE_2D);
//draw the floor
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, textfloor);
glColor3f(1.0f, 1.0f, 1.0f);
glBegin(GL_QUADS);
glNormal3f(0.0f, 1.0f, 0.0f);
glVertex3f(-40.0f,0.0f, 30.0f);
glVertex3f(-40.0f, 0.0f, -30.0f);
glVertex3f(40.0f, 0.0f, -30.0f);
glVertex3f(40.0f, 0.0f, 30.0f);
glEnd();
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, textwall);
//the wall and the windows in left
glColor3f(0.8f,0.8f, 0.8f);
glBegin(GL_QUADS);
glNormal3f(1.0f, 0.0f, 0.0f);
glVertex3f(-40.0f,0.0f, 30.0f);
glVertex3f(-40.0f, 30.0f, 30.0f);
glVertex3f(-40.0f, 30.0f, -30.0f);
glVertex3f(-40.0f, 0.0f, -30.0f);
glEnd();
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, texwindow);
for(int n=0;n<=1;n++)
{
glBegin(GL_QUADS);
glNormal3f(1.0, 0.0f, 0.0f);
glTexCoord2f(1.0f, 0.0f);glVertex3f(-39.9, 10, -8+n*18);
glTexCoord2f(1.0f, 1.0f);glVertex3f(-39.9, 20, -8+n*18);
glTexCoord2f(0.0f, 1.0f);glVertex3f(-39.9, 20, -18+n*18);
glTexCoord2f(0.0f, 0.0f);glVertex3f(-39.9, 10, -18+n*18);
glEnd();
}
glDisable(GL_TEXTURE_2D);
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, textwall);
//the wall and the window in right
glColor3f(0.8f,0.8f, 0.8f);
glBegin(GL_QUADS);
glNormal3f(-1.0f, 0.0f, 0.0f);
glVertex3f(40.0f,0.0f, 30.0f);
glVertex3f(40.0f, 30.0f, 30.0f);
glVertex3f(40.0f, 30.0f, -30.0f);
glVertex3f(40.0f, 0.0f, -30.0f);
glEnd();
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, texwindow1);
glBegin(GL_QUADS);
glNormal3f(-1.0, 0.0f, 0.0f);
glTexCoord2f(1.0f, 0.0f);glVertex3f(39.5, 10, 10);
glTexCoord2f(1.0f, 1.0f);glVertex3f(39.5, 20, 10);
glTexCoord2f(0.0f, 1.0f);glVertex3f(39.5, 20, 0);
glTexCoord2f(0.0f, 0.0f);glVertex3f(39.5, 10, 0);
glEnd();
glDisable(GL_TEXTURE_2D);
//backwall
gl
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
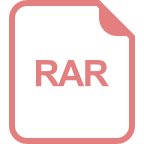
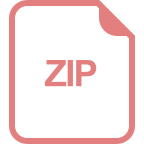
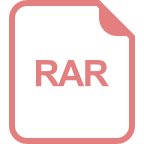
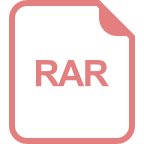
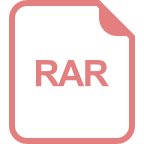
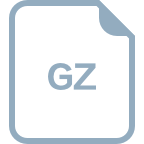
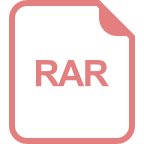
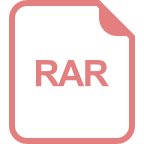
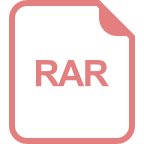
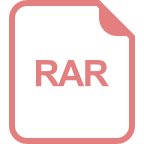
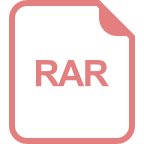
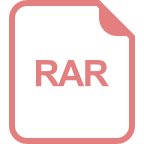
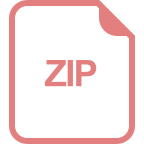
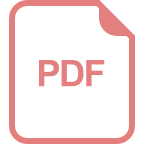
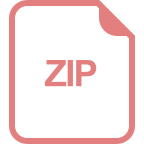
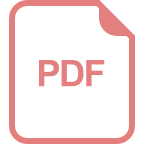
收起资源包目录


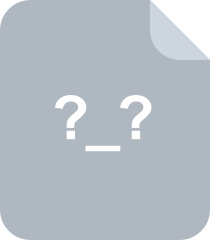
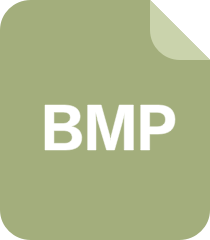
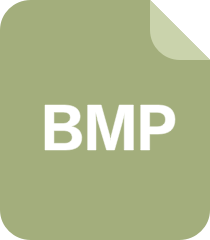
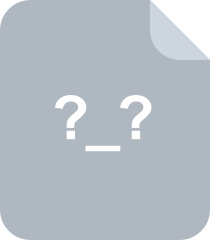

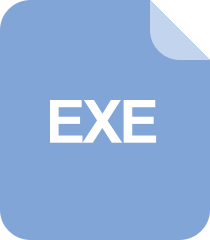
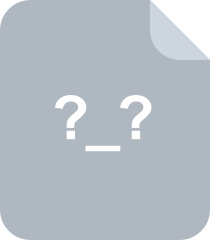
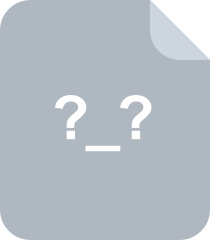
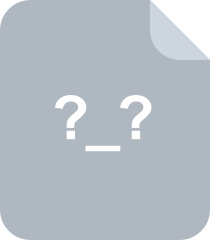
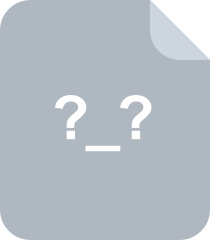
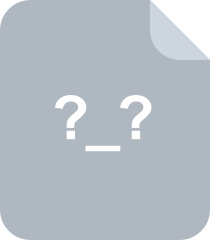
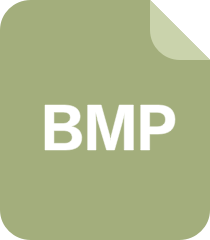
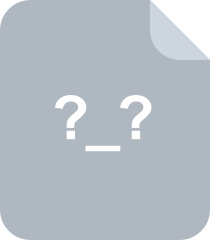
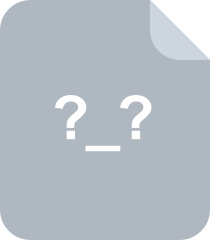
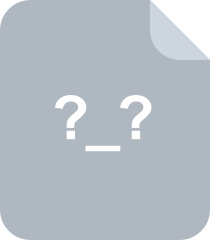
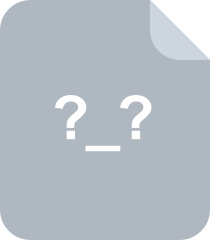
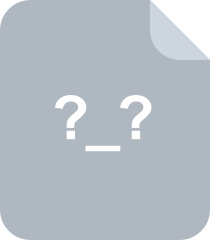
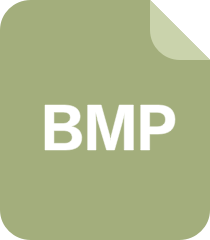
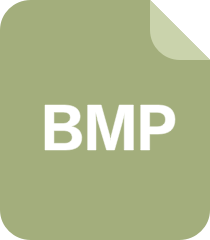
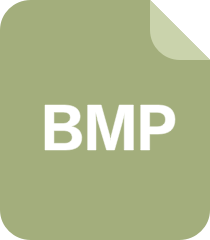
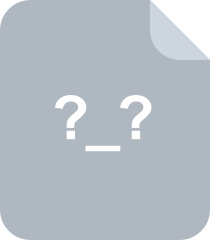
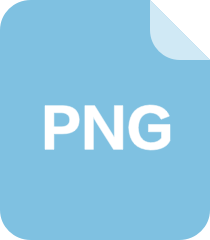
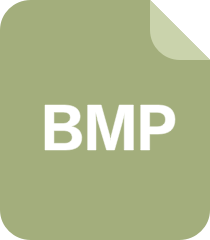
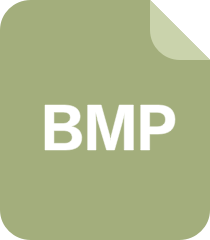
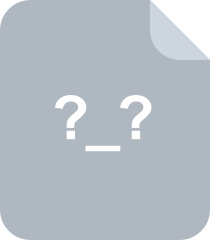
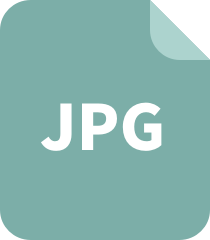

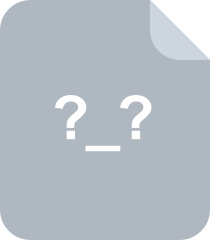
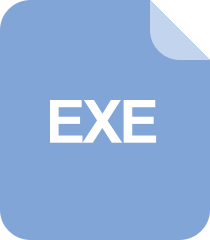
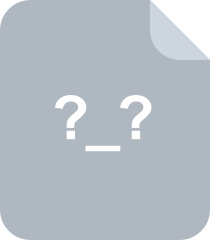
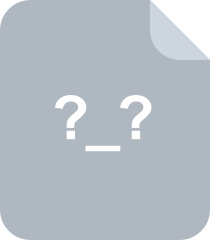
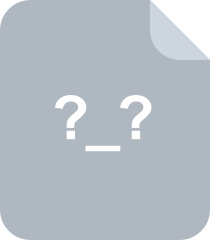
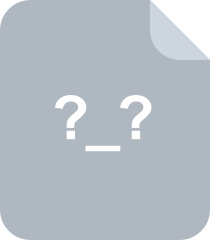
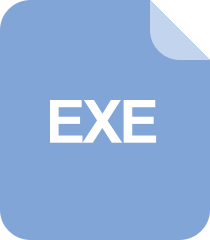
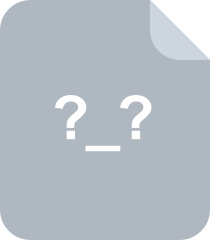
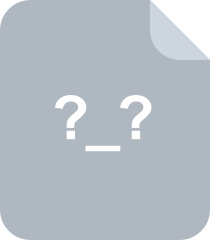
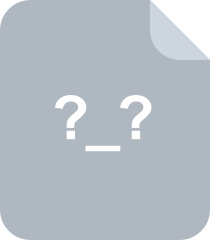
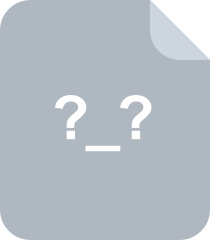
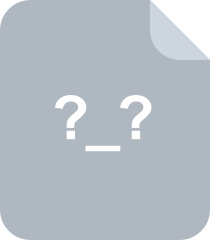
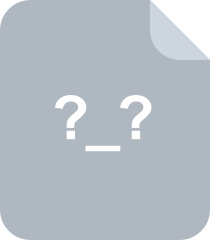
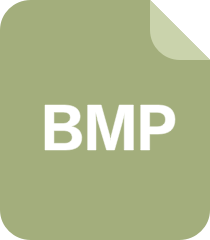
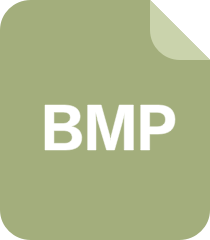
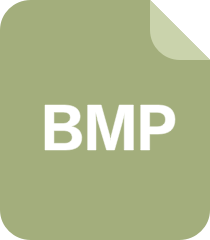
共 41 条
- 1
资源评论
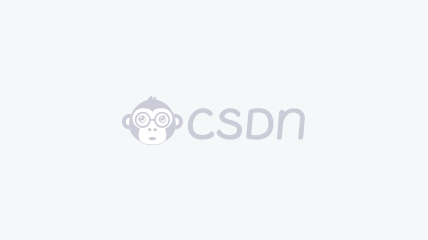
- qq_521024552023-05-30资源很不错,内容和描述一致,值得借鉴,赶紧学起来!

JaniceLu
- 粉丝: 78
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

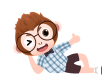
安全验证
文档复制为VIP权益,开通VIP直接复制
