package newscast;
import peersim.util.*;
import peersim.core.*;
import peersim.config.*;
import peersim.cdsim.*;
public class ReducedNewscast implements CDProtocol, Linkable {
// =============== static fields =======================================
// =====================================================================
// We are using static temporary arrays to avoid garbage collection
// of them. these are used by all SimpleNewscast protocols included
// in the protocol array so its size is the maximum of the cache sizes
/** Temp array for merging. Its size is the same as the cache size. */
protected static Node[] tn;
/** Temp array for merging. Its size is the same as the cache size. */
protected static int[] ts;
/** Identifier of the linkable protocol used as support */
private static int linkID;
/** config parameter name for the cache size */
public static final String PAR_CACHE = "cache";
/** config parameter name for the cache size */
public static final String PAR_LINK = "linkableID";
// =================== fields ==========================================
// =====================================================================
/** Neighbors currently in the cache */
protected Node[] cache;
/** Time stamps currently in the cache */
protected int[] tstamps;
/** True if active */
protected boolean active;
// ====================== initialization ===============================
// =====================================================================
public ReducedNewscast(String n) {
final int cachesize = Configuration.getInt(n+"."+PAR_CACHE);
linkID = Configuration.getPid(n+"."+PAR_LINK);
if(ReducedNewscast.tn == null || ReducedNewscast.tn.length < cachesize)
{
ReducedNewscast.tn = new Node[cachesize];
ReducedNewscast.ts = new int[cachesize];
}
cache = new Node[cachesize];
tstamps = new int[cachesize];
active=true;
}
// ---------------------------------------------------------------------
public Object clone() throws CloneNotSupportedException {
ReducedNewscast sn = (ReducedNewscast)super.clone();
sn.cache = new Node[cache.length];
sn.tstamps = new int[tstamps.length];
//System.arraycopy(cache, 0, sn.cache, 0, cache.length);
//System.arraycopy(tstamps, 0, sn.tstamps, 0, tstamps.length);
sn.active = true;
return sn;
}
// ====================== helper methods ==============================
// ====================================================================
/**
* Returns a peer node which is accessible (has ok fail state).
* This implementation starts with a random node.
* If that is not reachable, proceed first towards
* the older and then younger nodes until the first reachable is found.
* @return null if no accessible peers are found, the peer otherwise.
*/
protected Node getPeer() {
final int d = _degree();
//System.out.println(d);
if( d == 0 ) return null;
int index = CommonRandom.r.nextInt(d);
Node result = cache[index];
if( result.isUp() ) return result;
// proceed towards older entries
for(int i=index+1; i<d; ++i)
if( cache[i].isUp() ) return cache[i];
// proceed towards younger entries
for(int i=index-1; i>=0; --i)
if( cache[i].isUp() ) return cache[i];
// no accessible peer
return null;
}
// --------------------------------------------------------------------
/**
* Merge the content of two nodes and adds a new version of
* the identifier. The result is in the static temporary arrays.
* The first element is not defined, it is reserved for the freshest
* new updates so it will be different for peer and this.
* The elements of the static temporary arrays will not contain neither
* peerNode nor thisNode.
* @param thisNode the node that hosts this newscast protocol instance (process)
* @param peer The peer with which we perform cache exchange
* @param peerNode the node that hosts the peer newscast protocol instance
* @param timestamp the timestamp now
*/
protected void merge( Node thisNode, ReducedNewscast peer,
Node peerNode, int timestamp ) {
int i1 = 0; /* Index first cache */
int i2 = 0; /* Index second cache */
boolean first;
boolean lastTieWinner = CommonRandom.r.nextBoolean();
int i = 1; // Index new cache. first element set in the end
// SimpleNewscast.tn[0] is always null. it's never written anywhere
final int d1 = _degree();
final int d2 = peer._degree();
// cachesize is cache.length
// merging two arrays
while( i < cache.length && i1 < d1 && i2 < d2 )
{
if( tstamps[i1] == peer.tstamps[i2] )
{
lastTieWinner = first = !lastTieWinner;
}
else
{
first = tstamps[i1] > peer.tstamps[i2];
}
if( first )
{
if( cache[i1] != peerNode &&
!ReducedNewscast.contains( i, cache[i1] ) )
{
ReducedNewscast.tn[i] = cache[i1];
ReducedNewscast.ts[i] = tstamps[i1];
i++;
}
i1++;
}
else
{
if( peer.cache[i2] != thisNode &&
!ReducedNewscast.contains( i, peer.cache[i2] ) )
{
ReducedNewscast.tn[i]=peer.cache[i2];
ReducedNewscast.ts[i]=peer.tstamps[i2];
i++;
}
i2++;
}
}
// if one of the original arrays got fully copied into
// tn and there is still place, fill the rest with the other
// array
if( i < cache.length )
{
// only one of the for cycles will be entered
for(; i1<d1 && i<cache.length; ++i1)
{
if( cache[i1] != peerNode &&
!ReducedNewscast.contains( i, cache[i1] ) )
{
ReducedNewscast.tn[i] = cache[i1];
ReducedNewscast.ts[i] = tstamps[i1];
i++;
}
}
for(; i2<d2 && i<cache.length; ++i2)
{
if( peer.cache[i2] != thisNode &&
!ReducedNewscast.contains( i, peer.cache[i2] ) )
{
ReducedNewscast.tn[i]=peer.cache[i2];
ReducedNewscast.ts[i]=peer.tstamps[i2];
i++;
}
}
}
// if the two arrays were not enough to fill the buffer
// fill in the rest with nulls
if( i < cache.length )
{
for(; i<cache.length; ++i)
{
ReducedNewscast.tn[i] = null;
}
}
//if(testNode==null)testNode=peerNode;
//if(peerNode==testNode) System.out.println(CommonState.getTime());
}
//private static Node testNode=null;
// --------------------------------------------------------------------
protected static boolean contains(int size, Node peer)
{
for (int i=0; i < size; i++) {
if (ReducedNewscast.tn[i] == peer)
return true;
}
return false;
}
// --------------------------------------------------------------------
/** Normally it does the same as {@link #degree}. It is necessary to allow
* other implementations of {@link #degree} in extending classes which fool
* the system into
* thinking that the graph is different from what it is. Methods of this class
* should use this as degree.*/
protected int _degree() {
int len = cache.length-1;
while(len>=0 && cache[len]==null) len--;
return len+1;
}
// ====================== Linkable implementation =====================
// ====================================================================
/**
* Does not check if the index is out of bound
* (larger than {@link #degree()})
*/
public Node getNeighbor(int i) {
return cache[i];
}
//--------------------------------------------------------------------
public void removeNeighbor(int i) {
int len = cache.length-1;
while(len>=0 && cache[len]==null) len--;
cache[i] = cache[len];
tstamps[i] = tstamps[len];
cache[len] = null;
}
public void removeNeighbor(Node node) {
int len = cache.length-1;
while(len>=0 && cache[len]==null) len--;
if (len >= 0) {
int where = len;
while (where>=0 && cache[where]!=node) where--;
if (where >= 0) {
cache[where] = cache[len];
tstamps[where] = tstamps[len];
cache[len] = null;
return;
}
}
//System.out.print("X");
}
public void reset(No
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
提出了一个新奇的用来构建和维护覆盖网拓扑的一般机制。该机制基于gossip模式,节点和随机选择的对等体交换信息,并按照特定的P2P应用需求来重新安排拓扑,本协议非常的高效和鲁棒,能够处理节点持续的加入和离开系统的流,且即使现存的所有SP移除也能修复。
资源推荐
资源详情
资源评论
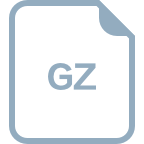
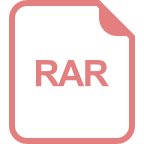
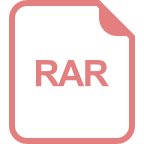
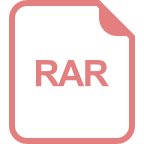
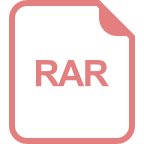
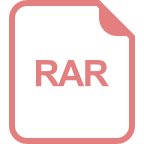
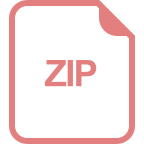
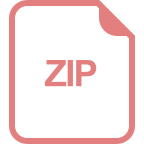
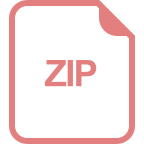
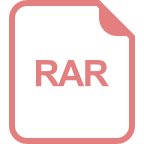
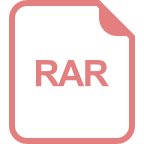
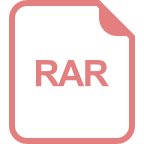
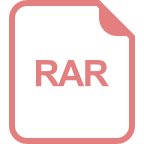
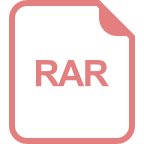
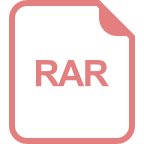
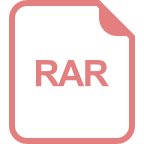
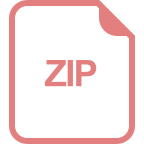
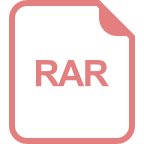
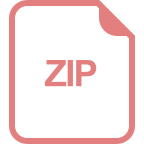
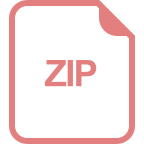
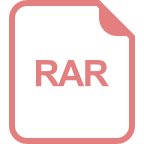
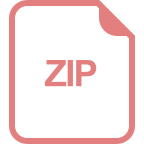
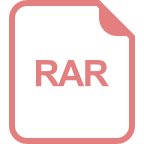
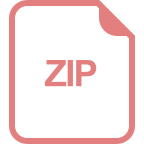
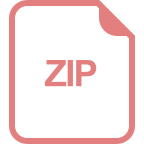
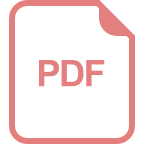
收起资源包目录



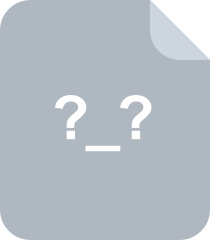
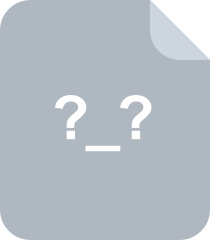
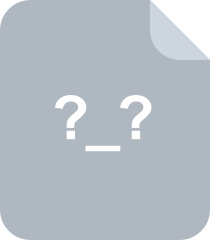
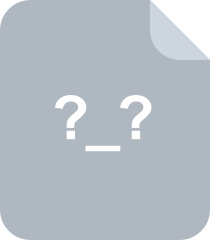
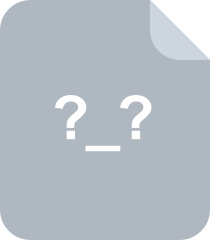
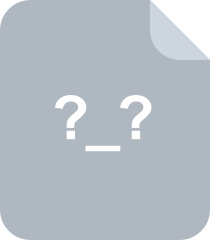
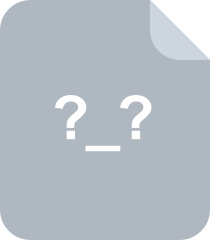
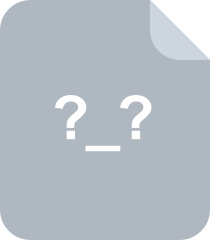
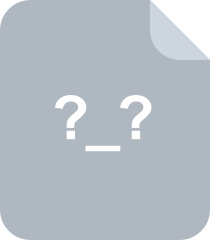
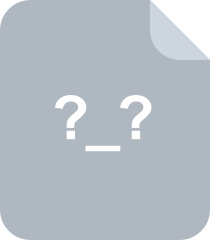
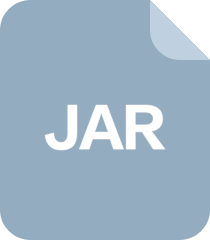
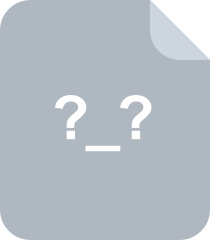
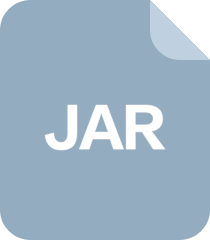


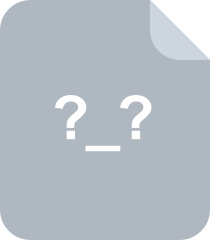
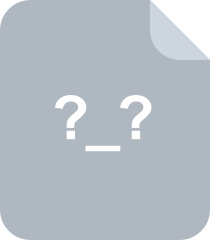

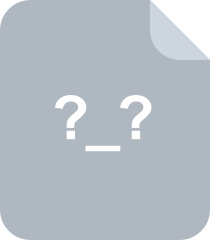
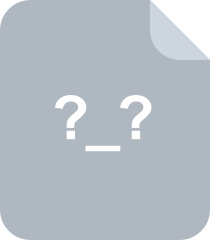
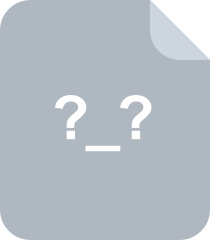
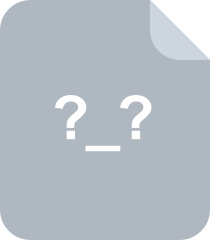
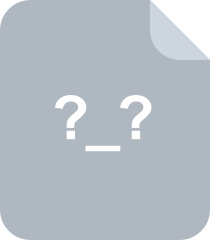
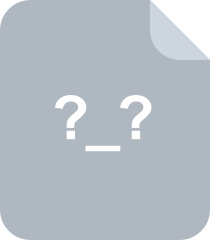
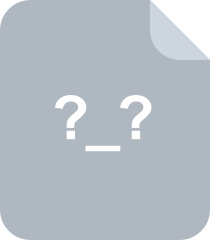
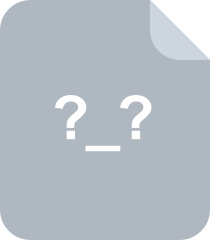
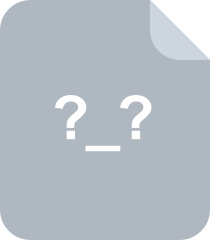
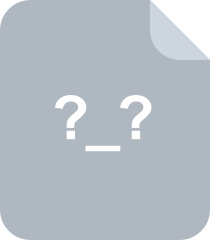


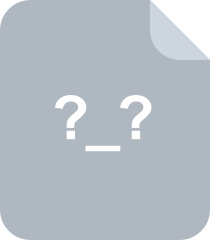
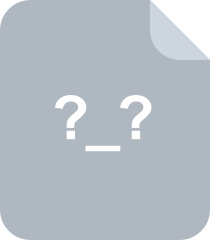

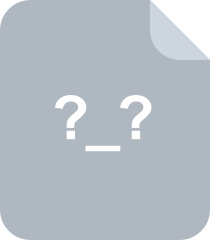
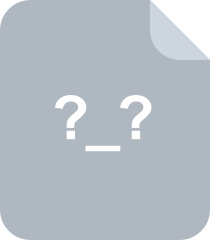
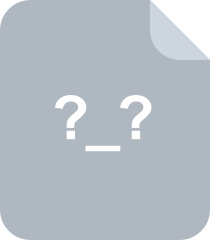
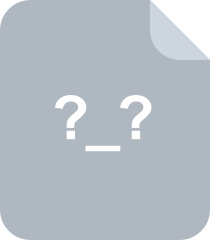
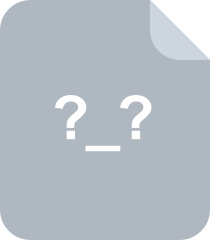
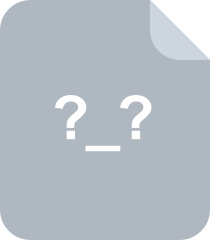
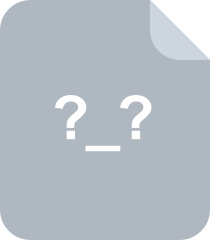
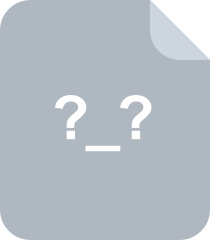
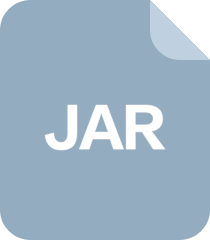
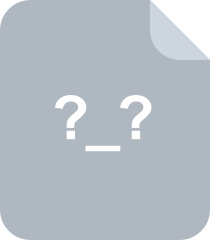
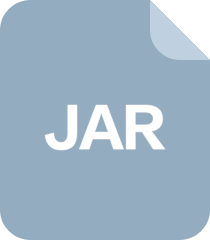
共 38 条
- 1
资源评论
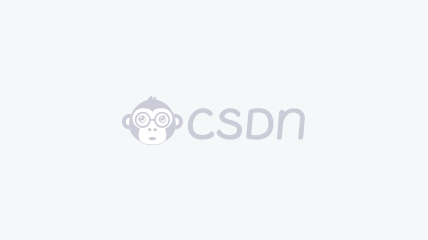

刘良运
- 粉丝: 77
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

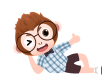
最新资源
- 机械手自动排列控制PLC与触摸屏程序设计
- uDDS源程序publisher
- 中国风格, 节日 主题, PPT模板
- 生菜生长记录数据集.zip
- 微环谐振腔的光学频率梳matlab仿真 微腔光频梳仿真 包括求解LLE方程(Lugiato-Lefever equation)实现微环中的光频梳,同时考虑了色散,克尔非线性,外部泵浦等因素,具有可延展
- 企业宣传PPT模板, 企业宣传PPT模板
- jetbra插件工具,方便开发者快速开发
- agv 1223.fbx
- 全国职业院校技能大赛网络建设与运维规程
- 混合动力汽车动态规划算法理论油耗计算与视频教学,使用matlab编写快速计算程序,整个工程结构模块化,可以快速改为串联,并联,混联等 控制量可以快速扩展为档位,转矩,转速等 状态量一般为SOC,目
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


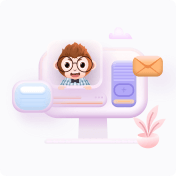
安全验证
文档复制为VIP权益,开通VIP直接复制
