# Gossip
Gossip protocol is a method for a group of nodes to discover and check the liveliness of a cluster. More information can be found at http://en.wikipedia.org/wiki/Gossip_protocol.
# Usage
## Maven
```xml
<dependency>
<groupId>net.lvsq</groupId>
<artifactId>jgossip</artifactId>
<version>1.5.0</version>
</dependency>
```
#### First you need one or more seed members
```java
List<SeedMember> seedNodes = new ArrayLis<>();
SeedMember seed = new SeedMember();
seed.setCluster(cluster);
seed.setIpAddress(ipAddress);
seed.setPort(port);
seedNodes.add(seed);
```
#### Then, instantiation a `GossipService` object
```java
GossipService gossipService = new GossipService(cluster,ipAddress, port, id, seedNodes, new GossipSettings(), (member, state) -> {
//Do anything what you want
});
```
#### Run `GossipService`
```java
gossipService.start();
```
#### Stop
```java
gossipService.shutdown();
```
#### Get offline nodes
```java
gossipService.getGossipManager().getDeadMembers();
```
#### Get online nodes
```java
gossipService.getGossipManager().getLiveMembers();
```
# Settings
* gossipInterval - How often (in milliseconds) to gossip list of members to other node(s). Default is 1000ms
* networkDelay - Network delay in ms. Default is 200ms
* msgService - Which message sync implementation. Default is **UDPMsgService.class** use UDP protocol to send message, certainly you can extand it.
* deleteThreshold - Delete the deadth node when the sync message is not received more than [deleteThreshold] times. Default is 3
# Event Listener
Currently, **jgossip** has four events
```java
GossipState.UP;
GossipState.DOWN;
GossipState.JOIN;
GossipState.RCV;
```
# Example
```java
int gossip_port = 60001;
String cluster = "gossip_cluster";
GossipSettings settings = new GossipSettings();
settings.setGossipInterval(1000);
try {
String myIpAddress = InetAddress.getLocalHost().getHostAddress();
List<SeedMember> seedNodes = new ArrayList<>();
SeedMember seed = new SeedMember();
seed.setCluster(cluster);
seed.setIpAddress(myIpAddress);
seed.setPort(60001);
seedNodes.add(seed);
gossipService = new GossipService(cluster, myIpAddress, gossip_port, null, seedNodes, settings, (member, state, payload) -> {
if (state == GossipState.RCV) {
System.out.println("member:" + member + " state: " + state + " payload: " + payload);
}
if (state == GossipState.DOWN) {
System.out.println("[[[[[[[[[member:" + member + " was down!!! ]]]]]]]]]");
}});
} catch (Exception e) {
e.printStackTrace();
}
gossipService.start();
```
Run the above code in each application to create a cluster based on the Gossip protocol. You can provide a meaningful `GossipListener` as the last parameter of `GossipService`. When state of a node changes, you can capture this change and make some responses.
# Publish Messages
If you want to send messages to gossip cluster:
```java
gossipService.getGossipManager().publish("Hello World");
```
If a node in cluster received messages, it will trigger the `GossipState.RCV` event, and handler predefined by `GossipListener` can consume these messages, such as <html><span style="color: green">"Hello World"</span><html> above.
The type of message is arbitrary, but only if it can be serialized. **jgossip** will try its best to deliver to every node. By default, messages will stay in memory for a while, and then **jgossip** will automatically delete them. So the best scenario for this feature is to send some simple messages regularly.
Please pay attention, if you need to send mass messages in a short time, which will consume a lot of resources, this requires you to weigh the actual situation.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一个 Java 版的 Gossip 协议实现。 Gossip 算法又被称为反熵(Anti-Entropy),熵是物理学上的一个概念,代表杂乱无章,而反熵就是在杂乱无章中寻求一致,这充分说明了 Gossip 的特点:在一个有界网络中,每个节点都随机地与其他节点通信,经过一番杂乱无章的通信,最终所有节点的状态都会达成一致。每个节点可能知道所有其他节点,也可能仅知道几个邻居节点,只要这些节可以通过网络连通,最终他们的状态都是一致的,Gossip 天然具有分布式容错的优点。 jgossip 使用很简单,配置方便,支持 JOIN、UP、DOWN 三种事件, 使用 UDP 协议进行数据同步。
资源推荐
资源详情
资源评论
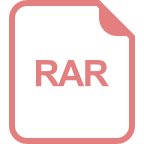
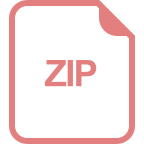
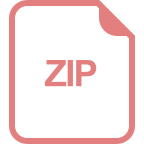
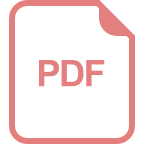
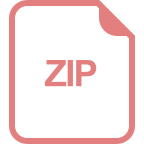
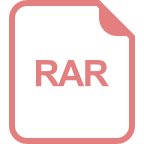
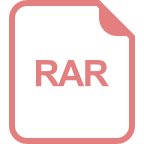
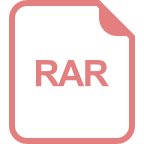
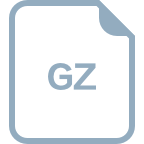
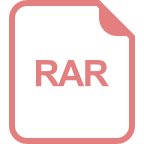
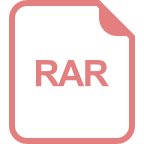
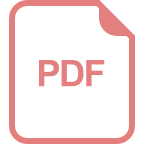
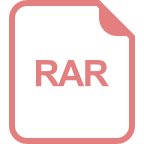
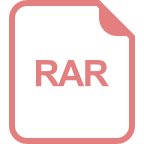
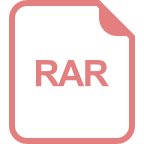
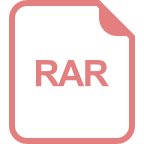
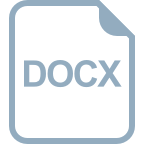
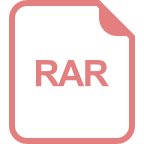
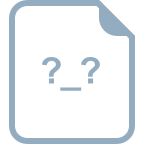
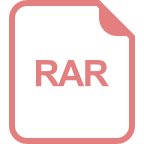
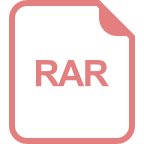
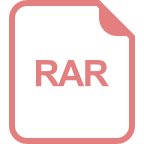
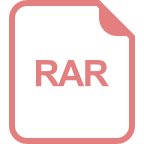
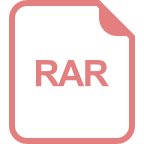
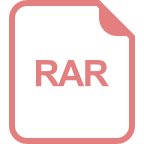
收起资源包目录


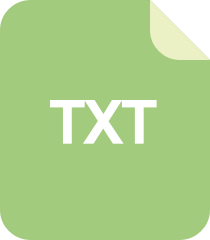

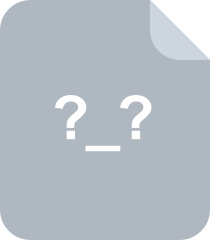






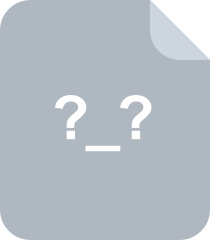
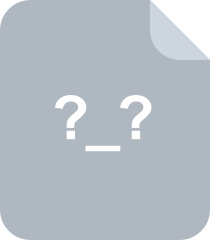
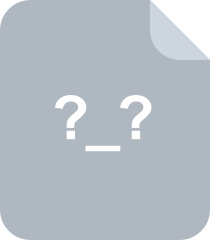
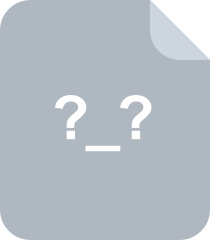
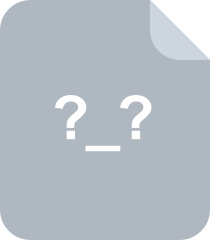






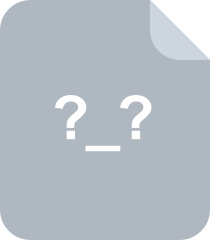
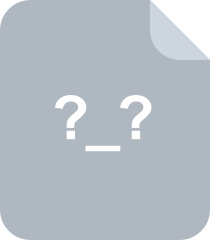
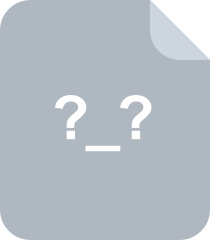
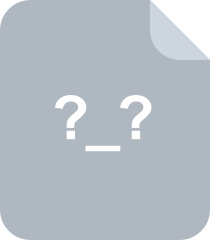
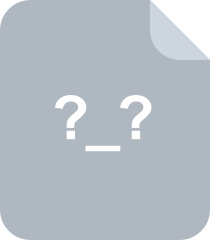
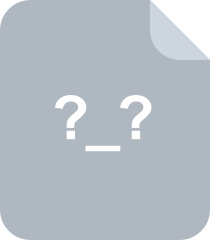


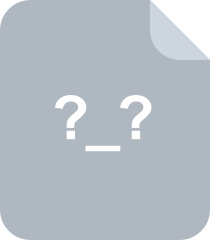
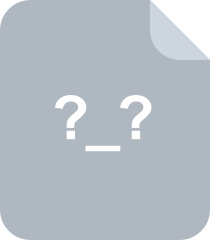

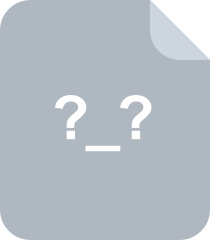

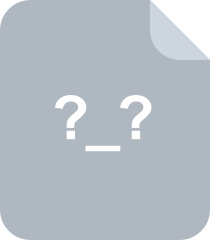
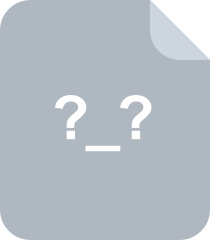
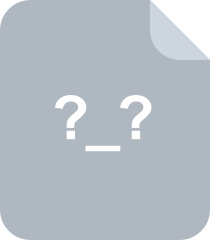
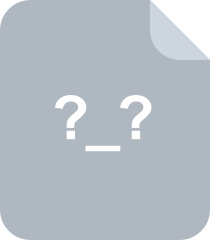
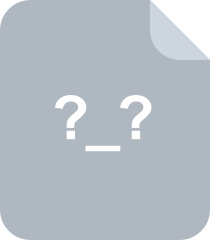
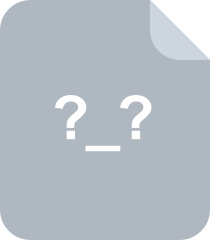
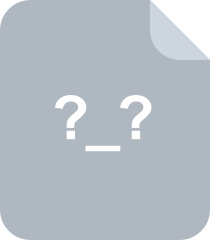
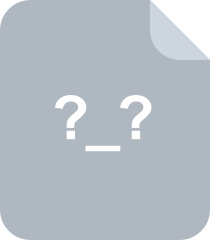
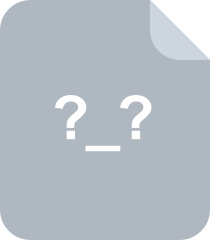
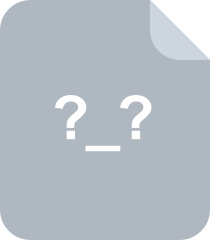

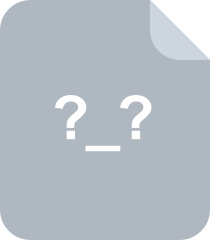
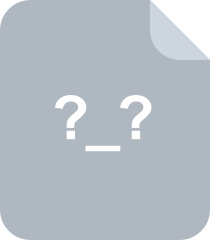
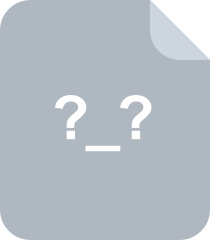
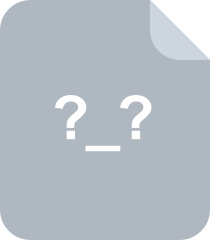
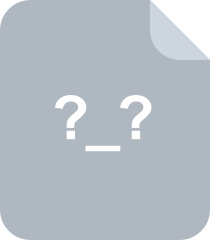
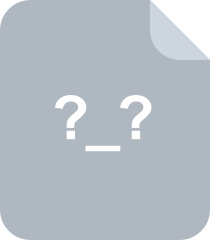
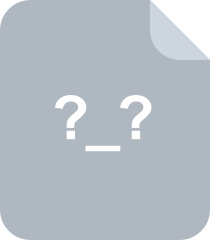
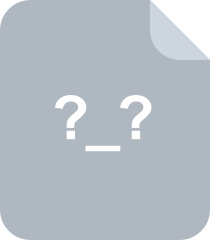
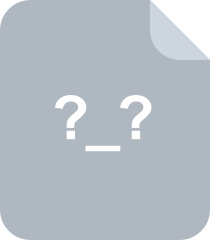
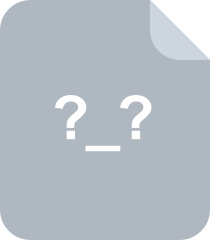
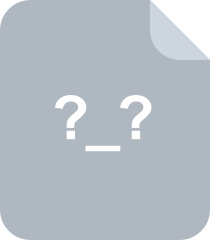
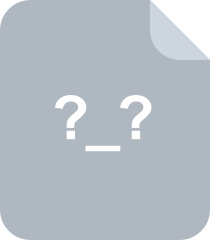
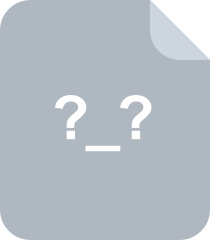
共 39 条
- 1
资源评论
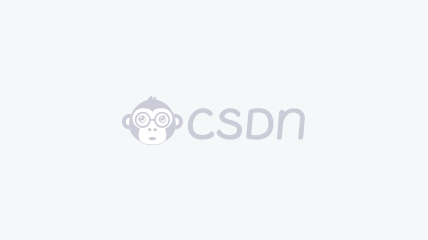
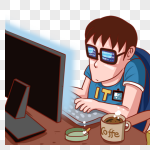
野生的狒狒
- 粉丝: 3396
- 资源: 2436
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

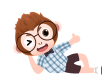
最新资源
- 快速定制中国传统节日头像(源码)
- hcia 复习内容的实验
- 准Z源光伏并网系统MATLAB仿真模型,采用了三次谐波注入法SPWM调制,具有更高的电压利用效率 并网部分采用了电压外环电流内环 电池部分采用了扰动观察法,PO Z源并网和逆变器研究方向的同学可
- 海面目标检测跟踪数据集.zip
- 欧美风格, 节日主题模板
- 西门子1200和三菱FXU通讯程序
- 11种概率分布的拟合与ks检验,可用于概率分析,可靠度计算等领域 案例中提供11种概率分布,具体包括:gev、logistic、gaussian、tLocationScale、Rayleigh、Log
- 机械手自动排列控制PLC与触摸屏程序设计
- uDDS源程序publisher
- 中国风格, 节日 主题, PPT模板
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


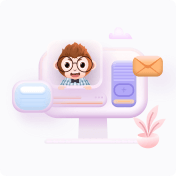
安全验证
文档复制为VIP权益,开通VIP直接复制
