TSP的Java实现.rar.rar_ tsp java_TSP in Java_TSP ja_TSPLIB_java T
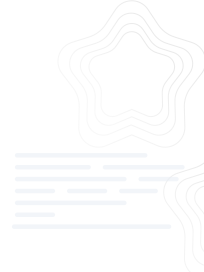

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)

**旅行商问题(Traveling Salesman Problem, TSP)**是运筹学和计算机科学中的一个著名问题,属于组合优化的范畴。这个问题的基本设定是:一个旅行商需要访问多个城市,每个城市只访问一次,并在最后返回出发地,目标是最小化旅行的总距离。在实际应用中,TSP广泛应用于物流、电路设计、生产计划等领域。 **Java实现TSP**,主要是通过编程技术来解决这个问题。Java是一种多平台的、面向对象的编程语言,因其丰富的库支持和良好的性能而常被用于处理复杂计算问题。在这个案例中,开发者可能使用了Java的算法库和数据结构来构建TSP的解决方案。 **源程序**通常包括了算法的设计和实现,可能包含了以下部分: 1. **图数据结构**:用邻接矩阵或邻接表来表示城市的连接关系。 2. **搜索算法**:如深度优先搜索(DFS)、广度优先搜索(BFS)或者更高级的算法,如动态规划、遗传算法、模拟退火等。 3. **路径优化**:可能包含回溯和剪枝策略,以避免无效的搜索。 4. **性能优化**:利用Java的并发库进行多线程计算,以加速大规模问题的求解。 **调试过程生成的文件**可能是日志文件、中间结果输出、性能报告等,这些文件可以帮助开发者追踪代码执行情况,找出潜在的错误或性能瓶颈。 **www.pudn.com.txt** 可能是下载来源的说明或者问题的背景介绍,通常这类文件不包含核心的程序代码,但可能提供了解决问题的上下文信息。 **TSP的Java实现** 文件可能包含整个TSP问题的具体Java代码实现,包括类定义、方法实现、主程序等,它是理解整个项目的核心。 在Java中实现TSP,需要对算法有深入的理解,因为TSP是一个NP完全问题,对于大规模问题没有多项式时间的精确解法。因此,开发者通常会采用启发式算法或近似算法来寻找接近最优的解决方案。例如,遗传算法、模拟退火、贪心算法等都是常见的选择。在实际开发中,还需要考虑算法的效率、内存使用和代码可读性等因素。
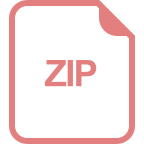
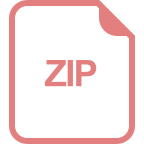
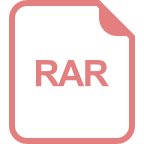
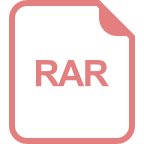
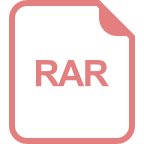
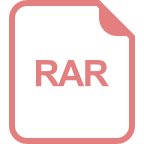
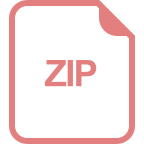
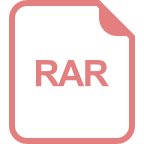
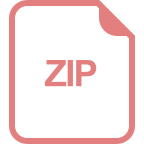
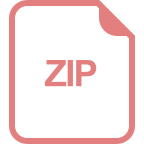
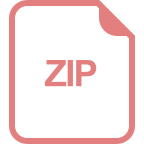
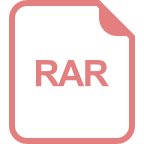
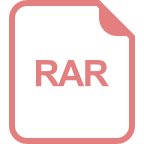
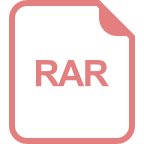
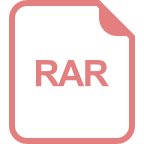
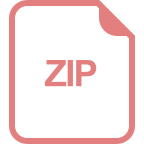


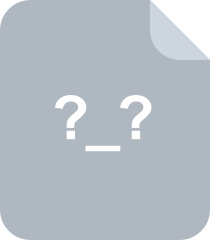
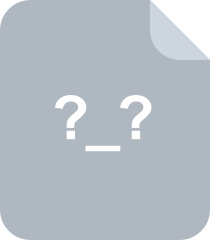
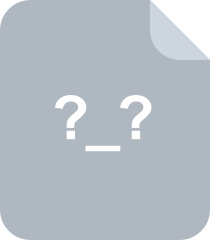
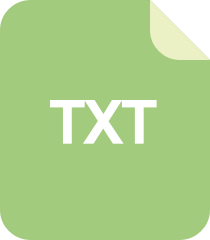
- 1
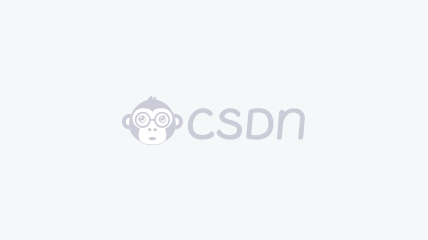
- kefu1252023-02-08果断支持这个资源,资源解决了当前遇到的问题,给了新的灵感,感谢分享~

- 粉丝: 77
- 资源: 1万+





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

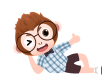
最新资源
- 小区监控视频监控方案.doc
- 某小区视频监控系统设计方案.doc
- 数电期末练习题.doc
- 数电期末试题.doc
- 数电习题试卷.doc
- 进程调度模拟算法.doc
- 操作系统模拟进程调度算法.doc
- C语言程序设计期末考试试题含答案.doc
- 数电期末试卷及答案.doc
- 汇编实验课程综合设计.doc
- 汇编实验子程序程序设计.doc
- 汇编实验算数运算程序设计.docx
- 多元统计分析重点.docx
- 基于卷积-长短期记忆网络加注意力机制(CNN-LSTM-Attention)的时间序列预测程序,预测精度很高 可用于做风电功率预测,电力负荷预测等等 标记注释清楚,可直接数据运行 代码实现训练与测
- C++语言程序设计期末考试试题及答案.doc
- Linux期末考试复习试题含答案.doc

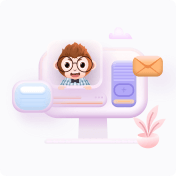
