#include <string.h>
#include <stdlib.h>
#include "..\inc\44b.h"
#include "..\inc\44blib.h"
#include "..\inc\def.h"
#include "..\inc\rtc.h"
#define KEY_BUFLEN 100
#define AFC_BUFLEN 0x100
#define IrDA_BUFLEN 0x100
char Uart_IntGetkey(void);
void Uart_Port(void);
void Return_Port(void);
void IrDA_Port(void);
void __irq Uart0_TxFifoInt(void);
void __irq Uart0_RxFifoInt(void);
void __irq Uart0_RxFifoErrorInt(void);
void __irq Uart0_RxInt(void);
void __irq Uart0_TxInt(void);
void __irq Uart1_TxFifoInt(void);
void __irq Uart1_RxFifoInt(void);
void __irq Uart1_RxFifoErrorInt(void);
void __irq Uart1_RxInt(void);
void __irq Uart1_TxInt(void);
void __irq U1AFC_TxInt(void);
void __irq U0AFC_RxInt(void);
void __irq U0AFC_RxErrorInt(void);
void __irq Exint2(void);
void __irq Test_Done(void);
void __irq Error(void);
void __irq U1IrDA_TxInt(void);
void __irq U1IrDA_RxInt(void);
void __irq U1IrDA_RxErrorInt(void);
#define UCON_RXMODE_DISABLE 0x00
#define UCON_RXMODE_INT_POLL 0x01
#define UCON_RXMODE_BDMA0 0x02
#define UCON_RXMODE_BDMA1 0x03
#define UCON_TXMODE_DISABLE (0x00<<2)
#define UCON_TXMODE_INT_POLL (0x01<<2)
#define UCON_TXMODE_BDMA0 (0x02<<2)
#define UCON_TXMODE_BDMA1 (0x03<<2)
#define UCON_SENDBREAK (0x01<<4)
#define UCON_LOOPBACK (0x01<<5)
#define UCON_RXERR_INTEN (0x01<<6)
#define UCON_RXTIMEOUT_INTEN (0x01<<7)
#define UCON_RXINTTYPE_PULSE (0x00<<8)
#define UCON_RXINTTYPE_LEV (0x01<<8)
#define UCON_TXINTTYPE_PULSE (0x00<<9)
#define UCON_TXINTTYPE_LEV (0x01<<9)
#define UFCON_TX_TRILEV_0 (0x00<<6)
#define UFCON_TX_TRILEV_4 (0x01<<6)
#define UFCON_TX_TRILEV_8 (0x02<<6)
#define UFCON_TX_TRILEV_12 (0x03<<6)
#define UFCON_RX_TRILEV_0 (0x00<<4)
#define UFCON_RX_TRILEV_4 (0x01<<4)
#define UFCON_RX_TRILEV_8 (0x02<<4)
#define UFCON_RX_TRILEV_12 (0x03<<4)
#define UFCON_TX_RESET (0x01<<2)
#define UFCONF_RX_RESET (0x01<<1)
#define UFCONF_EN 0x01
static unsigned char keyBuf[KEY_BUFLEN];
volatile static int IrDA_BAUD,keyBufRdPt=0;
volatile static int keyBufWrPt=0;
volatile char out=1;
static char *uart0TxStr;
static char *uart1TxStr;
volatile U32 save_UC,save_UE,save_UF,save_UPC,save_UPE,save_UPF;
char Uart_IntGetkey(void)
{
if (KEY_BUFLEN == keyBufRdPt)
{
keyBufRdPt=0;
}
while(keyBufWrPt==keyBufRdPt); //until FIFO is triggered
return keyBuf[keyBufRdPt++];
}
////////UART 0 TEST////////////////
void Test_Uart0Fifo(void)
{
int key;
int i, j;
keyBufRdPt=keyBufWrPt=0;
pISR_UTXD0=(unsigned)Uart0_TxFifoInt;
pISR_URXD0=(unsigned)Uart0_RxFifoInt;
pISR_UERR01=(unsigned)Uart0_RxFifoErrorInt;
/*********** UART0 Tx FIFO test with interrupt ***********/
Uart_Printf("[UART 0 :FIFO Mode Output Test, \"123456789abcdef\" will be output.]\n");
Uart_TxEmpty(0); //wait until tx shifter is empty.
uart0TxStr="123456789abcdef, test OK";//UART0 Tx FIFO interrupt test is good!!!!\r\n";
//rUFCON0=(2<<6)|(1<<4)|(6)|1;
rUFCON0 = UFCON_TX_TRILEV_8 |UFCON_RX_TRILEV_4| UFCONF_EN;
//FIFO trigger:tx/rx:8byte,tx/rx_fifo reset(will be cleared),FIFO enable.
//rUCON0 = 0x244; //tx:levl,rx:edge,error int,normal*2,interrupt(Start)
rUCON0 = UCON_TXINTTYPE_LEV | UCON_RXINTTYPE_PULSE | UCON_RXERR_INTEN;
rINTMSK=~(BIT_GLOBAL|BIT_UTXD0);
for (i = 0; i < 100000; ++ i) //延迟等待上一过程结束
/*********** UART0 Tx FIFO test with BDMA0 ***********/
Uart_Init(0,115200);
Uart_Printf("\n\n[UART 0: FIFO+BDMA0 Mode Output Test:]\n");
uart0TxStr="UART 0: FIFO+BDMA0 Mode Output Test OK!!!!。\r\n";
Uart_TxEmpty(0);
//rUCON0=0x48; //tx:BDMA0 rx:disable
rUCON0 = UCON_RXERR_INTEN | UCON_TXMODE_BDMA0;
rBDICNT0=0x00;
rBDCON0 =0x00;
rBDISRC0=(unsigned int)uart0TxStr|(0<<30)|(1<<28); // byte,inc
rBDIDES0=UTXH0 |(1<<30)|(3<<28); //L/B endian,M2IO,fix
rBDICNT0=strlen(uart0TxStr)|(2<<30)|(1<<26)|(1<<20); //UART0,start,polling
while(!((rBDCON0&0x30)==0x20)); //等待DMA结束
Uart_TxEmpty(0);
/*********** UART0 Rx FIFO test with interrupt ***********/
rUCON0=0x245|0x80; //tx:level,rx:edge,tx/rx:int,rcv_time_out enabled,error int enable
Uart_Printf("\n[Uart channel 0 FIFO Rx Interrupt Test]:Type any key!!!\n");
Uart_Printf("You have to see the typed character. To quit, press Enter key.\n");
rINTMSK=~(BIT_GLOBAL|BIT_URXD0|BIT_UERR01);
while( (rUFSTAT0&0xf) >0 )
key=RdURXH0(); //To clear the Rx FIFO
// rUERSTAT0; //To clear the error state
while((key=Uart_IntGetkey())!='\r')
Uart_SendByte(key);
rUFCON0=(2<<6)|(1<<4)|(6)|0;
//FIFO trigger:tx/rx:8byte, txrx_fifo reset(will be cleared), FIFO disable.
rINTMSK=~BIT_GLOBAL;
rUCON0=0x45; //rcv_time_out disabled
Uart_Printf("\n");
}
void __irq Uart0_TxFifoInt(void)
{
int i;
//while( !(rUFSTAT0 & 0x200) && (*uart0TxStr != '\0') ) //until tx fifo full or end of string
while( !(rUFSTAT0 == 16) && (*uart0TxStr != '\0') ) //until tx fifo full or end of string
{
rUTXH0=*uart0TxStr++;
for(i=0;i<700;i++); //to avoid overwriting FIFO
}
if(*uart0TxStr == '\0')
{
rUCON0 &= 0x3f3;
rINTMSK |= (BIT_GLOBAL | BIT_UTXD0);
}
rI_ISPC=BIT_UTXD0;
}
void __irq Uart0_RxFifoInt(void)
{
rI_ISPC=BIT_URXD0;
// if(rUFSTAT0==0)
// Uart_Printf("time out\n");
while( (rUFSTAT0&0xf) >0 ) //until FIFO is empty
{
keyBuf[keyBufWrPt++]=rURXH0;//rx buffer->keyBuf[]
if(keyBufWrPt==KEY_BUFLEN)
keyBufWrPt=0;
}
}
void __irq Uart0_RxFifoErrorInt(void)
{
rI_ISPC=BIT_UERR01;
switch(rUERSTAT0)//to clear and check the status of register bits
{
case '1':
Uart_Printf("Overrun error\n");
break;
case '2':
Uart_Printf("Parity error\n");
break;
case '4':
Uart_Printf("Frame error\n");
break;
case '8':
Uart_Printf("Breake detect\n");
break;
default :
break;
}
while( (rUFSTAT0&0xf) >0 )
{
keyBuf[keyBufWrPt++]=rURXH0;
if(keyBufWrPt==KEY_BUFLEN)
keyBufWrPt=0;
}
}
void Test_Uart0(void)
{
int key;
char szTemp[20];
unsigned nTemp;
keyBufRdPt=keyBufWrPt=0;
pISR_UTXD0=(unsigned)Uart0_TxInt;
pISR_URXD0=(unsigned)Uart0_RxInt;
/*********** UART0 Tx test with interrupt ***********/
Uart_Printf("[Uart 0 TX Interrupt Mode Test...]\n");
Uart_TxEmpty(0); //wait until tx shifter is empty.
uart0TxStr="Uart 0 TX Interrupt Mode Test OK!!!!";
rINTMSK = ~(BIT_GLOBAL|BIT_UTXD0); //允许UART0 的TX 中断
// rUCON0 &= 0x3f3;
//rUCON0 = 0x244; //tx:level,rx:edge,error int,normal*2,interrupt(Start)
rUCON0 = UCON_TXINTTYPE_LEV | UCON_RXINTTYPE_PULSE | UCON_RXERR_INTEN |UCON_TXMODE_INT_POLL;
Delay (3000);
/*********** UART0 Tx test with BDMA0 ***********/
//nTemp = UCON_TXINTTYPE_LEV | UCON_RXINTTYPE_PULSE | UCON_RXERR_INTEN;
rUCON0 = UCON_TXINTTYPE_LEV | UCON_RXINTTYPE_PULSE | UCON_RXERR_INTEN |UCON_TXMODE_INT_POLL;
Uart_Printf ("\r\n\n[Uart0 TX BDMA0 Mode Test...]\n");
Uart_TxEmpty (0);
uart0TxStr = "Uart0 TX BDMA0 Mode Test OK!!!!";
//切换成 DMA 模式
//rUCON0=0x48; //tx:BDMA0 rx:disable
rUCON0 = UCON_RXERR_INTEN | UCON_TXMODE_BDMA0;
rBDICNT0=0x0;
rBDCON0 =0x0;
rBDISRC0=(unsigned int)uart0TxStr|(0<<30)|(1<<28); // byte,inc
rBDIDES0=UTXH0 |(1<<30)|(3<<28); //L/B endian,M2IO,fix
rBDICNT0=strlen(uart0TxStr)|(2<<30)|(1<<26)|(1<<20); //UART0,
while(!((rBDCON0&0x30)==0x20)); //等待DMA结束
Uart_TxEmpty(0);
/*********** UART0 Rx test with interrupt ***********/
keyBufRdPt =0;
keyBufWrPt =0;
Uart_Printf ("\r\n\n[Uart0 Int
没有合适的资源?快使用搜索试试~ 我知道了~
44B0-test-program.rar_44_44b0
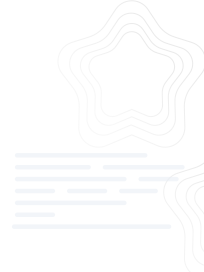
共68个文件
h:24个
o:19个
c:15个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 70 浏览量
2022-09-23
07:19:04
上传
评论
收藏 296KB RAR 举报
温馨提示
三星44B0学习用,主板测试程序,带有源码
资源推荐
资源详情
资源评论
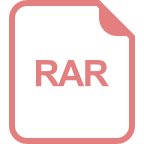
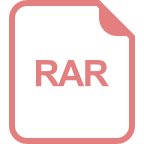
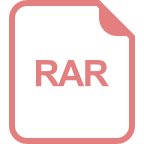
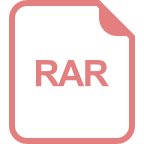
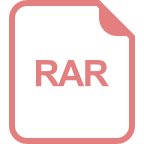
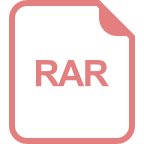
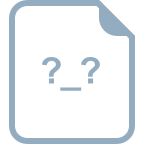
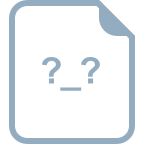
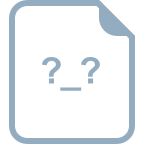
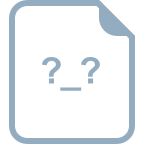
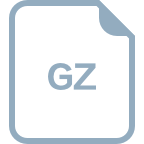
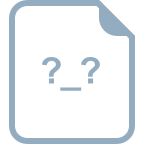
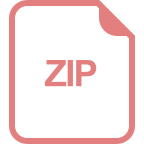
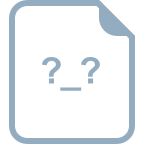
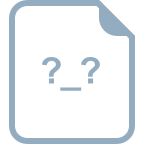
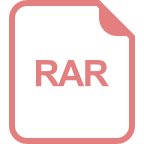
收起资源包目录



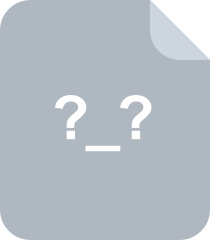

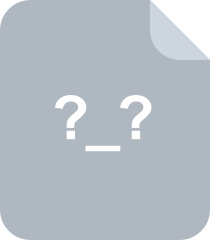
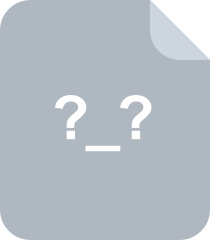
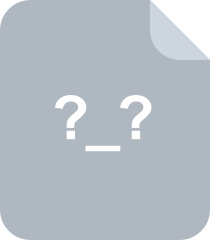
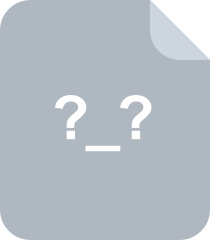
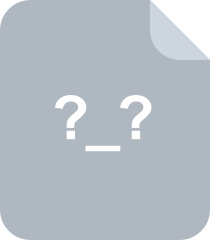
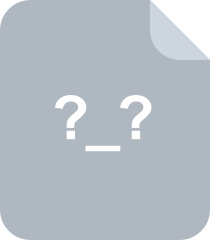
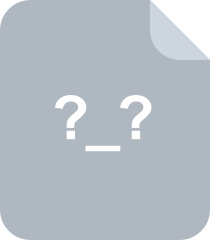
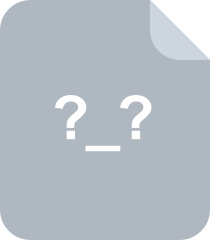
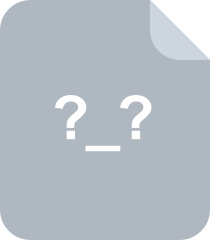
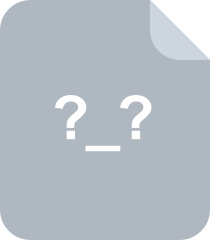
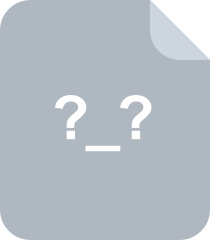
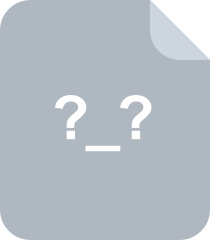
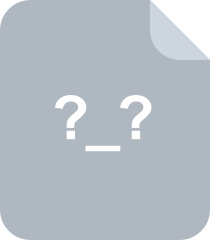
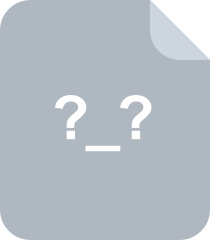
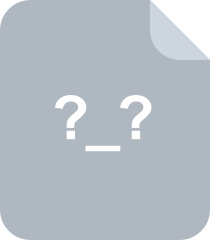
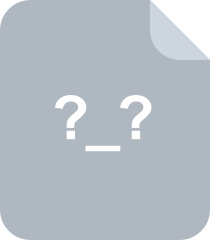
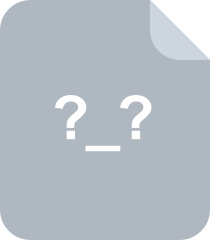

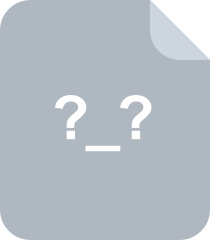
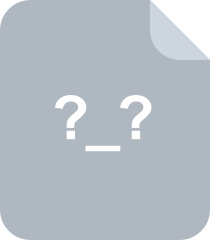
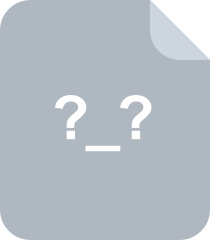
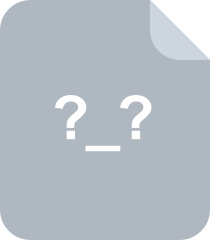
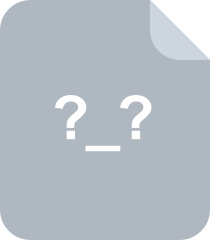
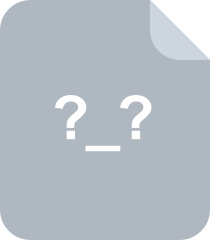
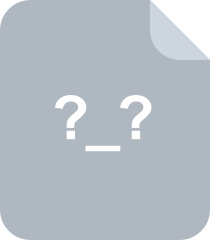
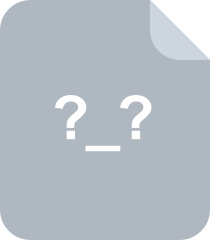
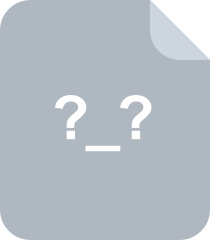
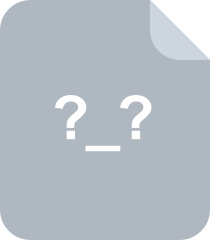
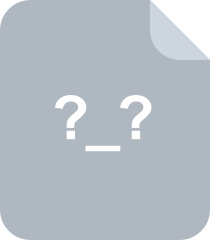
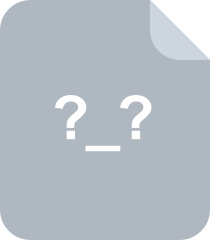
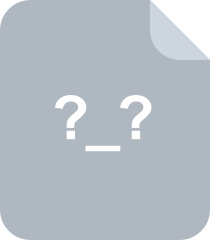
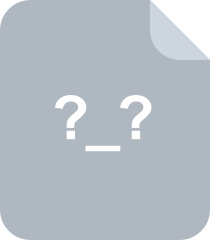
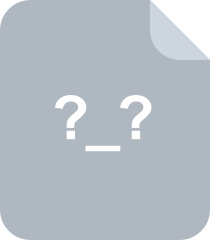
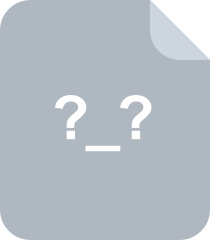
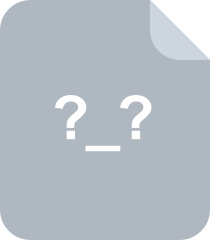
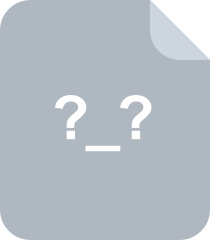
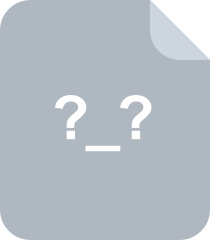
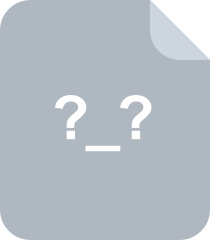
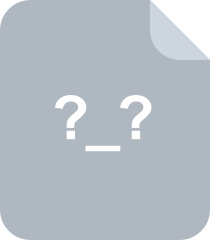
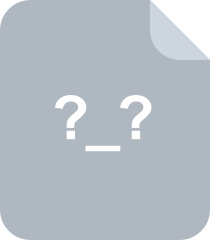
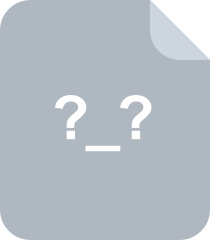
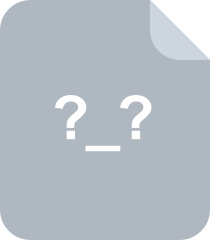
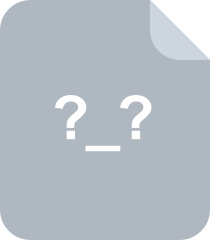
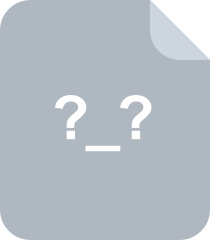


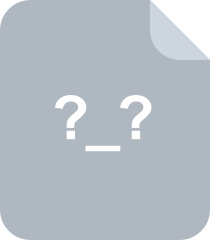



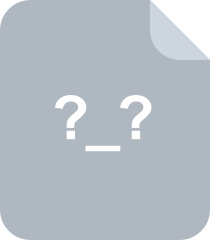
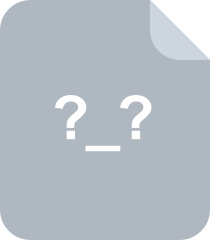
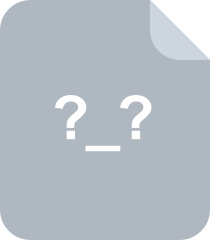
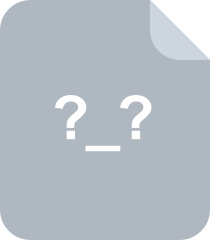
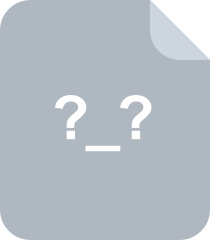
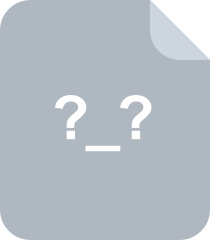
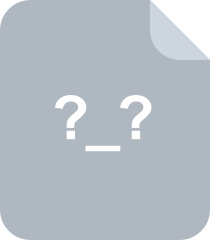
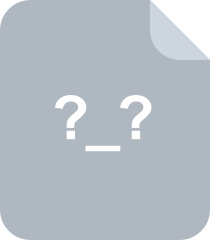
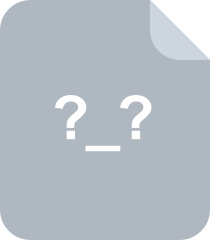
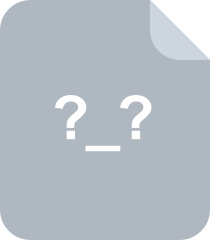
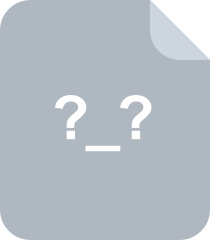
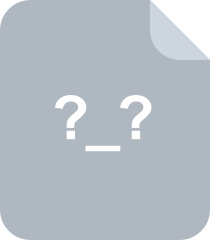
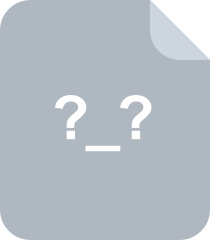
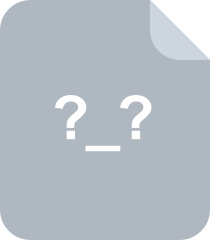
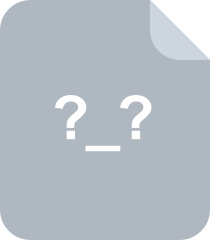
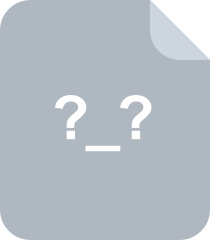
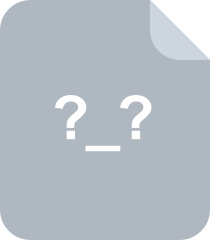
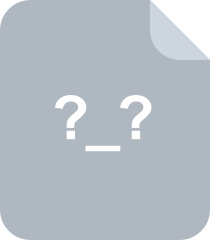
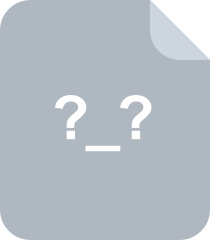
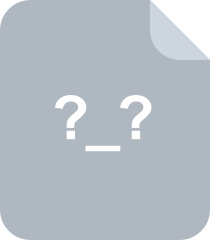
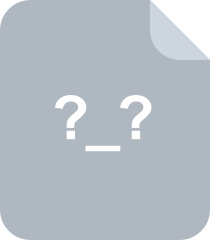
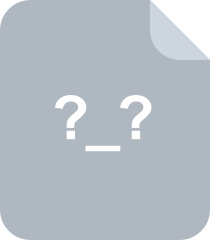
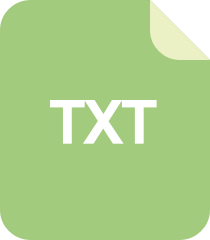
共 68 条
- 1
资源评论
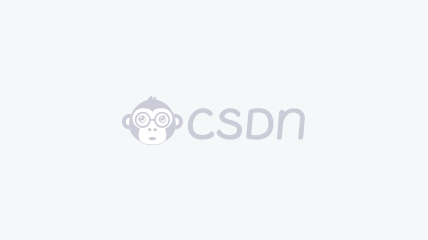

邓凌佳
- 粉丝: 79
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

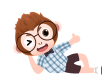
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


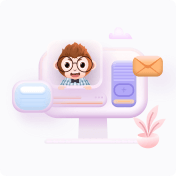
安全验证
文档复制为VIP权益,开通VIP直接复制
