zuiduanlujing.rar_zuiduanlujing
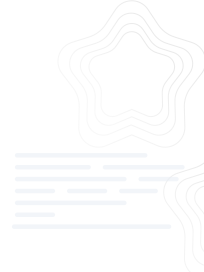

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
标题中的"zuiduanlujing.rar_zuiduanlujing"可能是指一个关于“最短路径”的压缩文件,这通常包含与图论和算法相关的资料。描述中提到的“以邻接矩阵为存储结构,实现弗洛伊德算法求解每一对顶点之间的最短路径及最短路径长度”是计算机科学中的一个重要概念,特别是对于网络分析、路由选择和优化问题。下面将详细介绍这两个关键知识点。 **邻接矩阵**: 在图论中,邻接矩阵是一种用于表示图中顶点之间连接关系的数据结构。它是一个二维数组,其中的元素代表两个顶点之间是否存在边以及边的权重。如果图是无向的,邻接矩阵是对称的;如果是有向的,则不对称。对于无权图,矩阵中的元素通常用1或0表示边的存在与否;对于有权图,元素则存储边的具体权重。 **弗洛伊德算法**(Floyd-Warshall Algorithm): 弗洛伊德算法是一种解决所有顶点对之间最短路径问题的动态规划方法,适用于有权图。该算法通过不断更新每个顶点对之间的最短路径,逐步考虑所有中间顶点作为路径的一部分。其基本步骤如下: 1. 初始化:根据邻接矩阵初始化所有顶点对的最短路径。对于无权图,最短路径就是直接相连的距离;对于有权图,最短路径即为边的权重。 2. 遍历所有可能的中间节点k:对于每一对顶点i和j,检查是否存在通过节点k的更短路径。如果有,更新最短路径为`d[i][j] = min(d[i][j], d[i][k] + d[k][j])`。 3. 迭代:重复步骤2,遍历所有节点k,直到所有节点都检查过。 弗洛伊德算法的时间复杂度为O(V^3),其中V是图中顶点的数量。尽管不是最高效的算法(例如,Johnson's算法在稀疏图上可能更快),但其简洁性和易于理解使其在许多场景下仍然实用。 在压缩包文件"zuiduanlujing"中,可能包含的资源可能有代码示例、解释文档或者练习题目,帮助学习者理解和应用上述概念。通过学习和实践这个算法,可以提升对图论和算法的理解,这对于计算机科学和相关领域的专业人员来说是非常有价值的。


















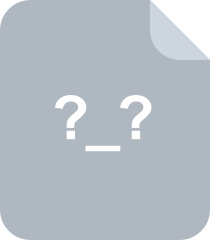
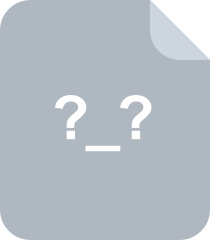
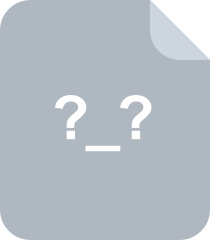
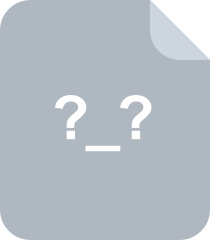
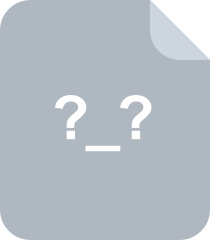

- 1
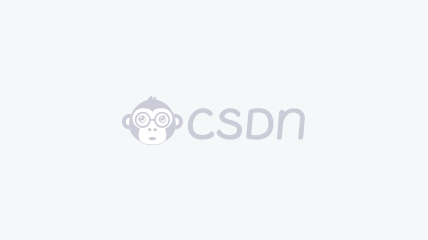

- 粉丝: 70
- 资源: 1万+





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

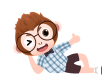
最新资源
- 化工行业:基于强化学习的DeepSeek实验方案生成微调策略.pdf
- 教育个性化学习:知识蒸馏构建学科能力评估模型.pdf
- 建筑工程:DeepSeek+BIM模型自动生成施工方案全流程.pdf
- 教育行业:零代码构建DeepSeek智能题库系统,日均成本仅5美元.pdf
- 金融合规检查:增量训练构建反洗钱模型快速迭代方案.pdf
- 教育行业落地:用提示词工程构建智能题库生成系统.pdf
- 教育行业突破:用DeepSeek-Coder实现编程教学智能批改.pdf
- 金融量化投资:DeepSeek微调实现多因子策略生成.pdf
- 金融领域适配技巧:量化训练实现信贷风控模型成本降低90%.pdf
- 金融行业:基于LoRA的DeepSeek信贷风险评估微调方案,成本直降80%.pdf
- 跨境电商:DeepSeek多语言客服模型训练数据增强技巧.pdf
- 跨境贸易:DeepSeek多语言合同风险扫描系统搭建指南.pdf
- 零代码适配术:用DeepSeek打造医疗问诊知识库,3天上线临床决策系统.pdf
- 零售库存管理:联邦学习实现多门店销量预测系统.pdf
- 零售门店数字化:DeepSeek摄像头数据实时分析+货架陈列优化模型训练指南.pdf
- 零售业实战:知识蒸馏技术赋能DeepSeek库存预测轻量化.pdf

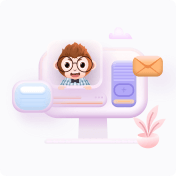
