/*
* gmac.h
* Copyright (C) 2000 by the University of Southern California
* $Id: gmac.h,v 1.11 2005/08/25 18:58:07 johnh Exp $
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License,
* version 2, as published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along
* with this program; if not, write to the Free Software Foundation, Inc.,
* 59 Temple Place, Suite 330, Boston, MA 02111-1307, USA.
*
*
* The copyright of this module includes the following
* linking-with-specific-other-licenses addition:
*
* In addition, as a special exception, the copyright holders of
* this module give you permission to combine (via static or
* dynamic linking) this module with free software programs or
* libraries that are released under the GNU LGPL and with code
* included in the standard release of ns-2 under the Apache 2.0
* license or under otherwise-compatible licenses with advertising
* requirements (or modified versions of such code, with unchanged
* license). You may copy and distribute such a system following the
* terms of the GNU GPL for this module and the licenses of the
* other code concerned, provided that you include the source code of
* that other code when and as the GNU GPL requires distribution of
* source code.
*
* Note that people who make modified versions of this module
* are not obligated to grant this special exception for their
* modified versions; it is their choice whether to do so. The GNU
* General Public License gives permission to release a modified
* version without this exception; this exception also makes it
* possible to release a modified version which carries forward this
* exception.
*
*/
// gmac is designed and developed by Wei Ye (SCADDS/ISI)
// and is ported into ns by Padma Haldar, June'02.
// Contributors: Yuan Li
// This module implements Sensor-MAC
// See http://www.isi.edu/scadds/papers/gmac_infocom.pdf for details
//
// It has the following functions.
// 1) Both virtual and physical carrier sense
// 2) RTS/CTS for hidden terminal problem
// 3) Backoff and retry
// 4) Broadcast packets are sent directly without using RTS/CTS/ACK.
// 5) A long unicast message is divided into multiple TOS_MSG (by upper
// layer). The RTS/CTS reserves the medium for the entire message.
// ACK is used for each TOS_MSG for immediate error recovery.
// 6) Node goes to sleep when its neighbor is communicating with another
// node.
// 7) Each node follows a periodic listen/sleep schedule
// 8.1) At bootup time each node listens for a fixed SYNCPERIOD and then
// tries to send out a sync packet. It suppresses sending out of sync pkt
// if it happens to receive a sync pkt from a neighbor and follows the
// neighbor's schedule.
// 8.2) Or a node can choose its own schecule instead of following others, the
// schedule start time is user configurable
// 9) Neighbor Discovery: in order to prevent that two neighbors can not
// find each other due to following complete different schedules, each
// node periodically listen for a whole period of the SYNCPERIOD
// 10) Duty cycle is user configurable
// New features including adaptive listen
// See http://www.isi.edu/~weiye/pub/gmac_ton.pdf
#ifndef NS_GMAC
#define NS_GMAC
//test features described in Journal paper, adaptive listen, etc
//#ifndef JOURNAL_PAPER
//#define JOURNAL_PAPER
//#endif
#ifndef dc_aware
#define dc_aware
#endif
#include "mac.h"
#include "mac-802_11.h"
#include "cmu-trace.h"
#include "random.h"
#include "timer-handler.h"
#ifdef dc_aware
#endif
/* User-adjustable MAC parameters
*--------------------------------
* The default values can be overriden in Each application's Makefile
* GMAC_MAX_NUM_NEIGHB: maximum number of neighbors.
* GMAC_MAX_NUM_SCHED: maximum number of different schedules.
* GMAC_DUTY_CYCLE: duty cycle in percentage. It controls the length of sleep
* interval.
* GMAC_RETRY_LIMIT: maximum number of RTS retries for sending a single message.
* GMAC_EXTEND_LIMIT: maximum number of times to extend Tx time when ACK timeout
happens.
*/
#ifndef GMAC_MAX_NUM_NEIGHBORS
#define GMAC_MAX_NUM_NEIGHBORS 20
#endif
#ifndef GMAC_MAX_NUM_SCHEDULES
#define GMAC_MAX_NUM_SCHEDULES 4
#endif
#ifndef GMAC_DUTY_CYCLE
#define GMAC_DUTY_CYCLE 10
#endif
#ifndef GMAC_RETRY_LIMIT
#define GMAC_RETRY_LIMIT 5
#endif
#ifndef GMAC_EXTEND_LIMIT
#define GMAC_EXTEND_LIMIT 5
#endif
#ifdef JOURNAL_PAPER
#ifndef GMAC_UPDATE_NEIGHB_PERIOD
#define GMAC_UPDATE_NEIGHB_PERIOD 50
#endif
#ifndef GUARDTIME
#define GUARDTIME 0.001
#endif
#endif
/* Internal MAC parameters
*--------------------------
* Do NOT change them unless for tuning S-MAC
* SYNC_CW: number of slots in the sync contention window, must be 2^n - 1
* DATA_CW: number of slots in the data contention window, must be 2^n - 1
* SYNC_PERIOD: period to send a sync pkt, in cycles.
* SRCH_CYCLES_LONG: # of SYNC periods during which a node performs a neighbor discovery
* SRCH_CYCLES_SHORT: if there is no known neighbor, a node need to seach neighbor more aggressively
*/
#define SYNC_CW 31
#define DATA_CW 63
#define SYNCPERIOD 10
#define SYNCPKTTIME 3 // an adhoc value used for now later shld converge with durSyncPkt_
#define SRCH_CYCLES_SHORT 3
#define SRCH_CYCLES_LONG 22
/* Physical layer parameters
*---------------------------
* Based on the parameters from PHY_RADIO and RADIO_CONTROL
* CLOCK_RES: clock resolution in ms.
* BANDWIDTH: bandwidth (bit rate) in kbps. Not directly used.
* PRE_PKT_BYTES: number of extra bytes transmitted before each pkt. It equals
* preamble + start symbol + sync bytes.
* ENCODE_RATIO: output/input ratio of the number of bytes of the encoding
* scheme. In Manchester encoding, 1-byte input generates 2-byte output.
* PROC_DELAY: processing delay of each packet in physical and MAC layer, in ms
*/
#define CLOCKRES 1 // clock resolution is 1ms
#define BANDWIDTH 20 // kbps =>CHANGE BYTE_TX_TIME WHENEVER BANDWIDTH CHANGES
//#define BYTE_TX_TIME 4/10 // 0.4 ms to tx one byte => changes when bandwidth does
#define PRE_PKT_BYTES 5
#define ENCODE_RATIO 2 /* Manchester encoding has 2x overhead */
#define PROC_DELAY 1
// Note everything is in clockticks (CLOCKRES in ms) for tinyOS
// so we need to convert that to sec for ns
#define CLKTICK2SEC(x) ((x) * (CLOCKRES / 1.0e3))
#define SEC2CLKTICK(x) ((x) / (CLOCKRES / 1.0e3))
// MAC states
#define SLEEP 0 // radio is turned off, can't Tx or Rx
#define IDLE 1 // radio in Rx mode, and can start Tx
//#define CHOOSE_SCHED 2 // node in boot-up phase, needs to choose a schedule
#define CR_SENSE 2 // medium is free, do it before initiate a Tx
//#define BACKOFF 3 // medium is busy, and cannot Tx
#define WAIT_CTS 3 // sent RTS, waiting for CTS
#define WAIT_DATA 4 // sent CTS, waiting for DATA
#define WAIT_ACK 5 // sent DATA, waiting for ACK
#ifdef JOURNAL_PAPER
#define TX_NEXT_FRAG 6 // send one fragment, waiting for next from upper layer
#else
#define WAIT_NEXTFRAG 6 // send one fragment, waiting for next from upper lay

四散
- 粉丝: 65
- 资源: 1万+
最新资源
- Spring Cloud商城项目专栏 049 支付
- sensors-18-03721.pdf
- Facebook.apk
- 推荐一款JTools的call-this-method插件
- json的合法基色来自红包东i请各位
- 项目采用YOLO V4算法模型进行目标检测,使用Deep SORT目标跟踪算法 .zip
- 针对实时视频流和静态图像实现的对象检测和跟踪算法 .zip
- 部署 yolox 算法使用 deepstream.zip
- 基于webmagic、springboot和mybatis的MagicToe Java爬虫设计源码
- 通过实时流协议 (RTSP) 使用 Yolo、OpenCV 和 Python 进行深度学习的对象检测.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


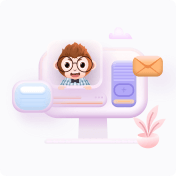