import java.awt.AWTEvent;
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Rectangle;
import java.awt.SystemColor;
import java.awt.event.MouseEvent;
import java.awt.event.WindowEvent;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.Socket;
import java.net.SocketException;
import java.util.Vector;
import javax.swing.BorderFactory;
import javax.swing.DefaultListModel;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPopupMenu;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.ListCellRenderer;
import javax.swing.ListModel;
import javax.swing.border.Border;
class FindFriend2 extends JFrame { //查找好友类
JLabel jLabel1 = new JLabel();
JButton find2 = new JButton();
JButton jButton1 = new JButton();
JButton jButton2 = new JButton();
JButton jButton3 = new JButton();
JList list2;
///////////////////以下是好友的呢称,性别等信息
Vector nickname = new Vector();
Vector sex = new Vector();
Vector place = new Vector();
Vector jicq = new Vector();
Vector ip = new Vector();
Vector pic = new Vector();
Vector status = new Vector();
Vector emails = new Vector();
Vector infos = new Vector();
//以下临时保存好友的呢称,性别等信息
Vector tmpjicq = new Vector(); //jicqid
Vector tmpname = new Vector(); //jicqname
Vector tmpip = new Vector(); //ip
Vector tmppic = new Vector(); //pic info
Vector tmpstatus = new Vector(); //status
Vector tmpemail = new Vector();
Vector tmpinfo = new Vector();
//以下创建网络相关变量
Socket socket;
BufferedReader in;
PrintWriter out;
int myid;
String serverhost;
int servport;
DatagramPacket sendPacket;
DatagramSocket sendSocket;
int sendPort = 5000;
//////////////////
JPopupMenu findmenu = new JPopupMenu();
JMenuItem look = new JMenuItem();
JMenuItem add = new JMenuItem();
public FindFriend2(int whoami, String host, int port) { //查找好友类构造函数
enableEvents(AWTEvent.WINDOW_EVENT_MASK);
try {
serverhost = host;
servport = port;
myid = whoami;
jbInit();
} catch (Exception e) {
e.printStackTrace();
} //以下与服务器连接
try {
socket = new Socket(InetAddress.getByName(serverhost), servport);
in =
new BufferedReader(
new InputStreamReader(socket.getInputStream()));
out =
new PrintWriter(
new BufferedWriter(
new OutputStreamWriter(socket.getOutputStream())),
true);
sendSocket = new DatagramSocket();
} catch (IOException e1) {
}
}
private void jbInit() throws Exception { //以下是程序界面
jLabel1.setText("下面是在线的朋友");
jLabel1.setBounds(new Rectangle(11, 11, 211, 18));
this.getContentPane().setLayout(new FlowLayout());
find2.setText("查找");
find2.setBounds(new Rectangle(8, 289, 79, 29));
find2.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(MouseEvent e) {
find2_mouseClicked(e);
}
});
jButton1.setText("next");
jButton1.setBounds(new Rectangle(110, 288, 79, 29));
jButton2.setText("up");
jButton2.setBounds(new Rectangle(211, 285, 79, 29));
jButton3.setText("cancel");
jButton3.setBounds(new Rectangle(317, 289, 79, 29));
// nickname=new Vector();
// sex=new Vector();
// place=new Vector();
ListModel model = new FindListModel(nickname, sex, place); //列表模型
ListCellRenderer renderer = new FindListCellRenderer();
list2 = new JList(model);
list2.setSize(200, 200);
list2.setBackground(new Color(255, 255, 210));
list2.setAlignmentX((float) 1.0);
list2.setAlignmentY((float) 1.0);
list2.setCellRenderer(renderer);
list2.setVisibleRowCount(7);
list2.addMouseListener(new java.awt.event.MouseAdapter() {
public void mousePressed(MouseEvent e) {
list2_mousePressed(e);
}
});
look.setText("查看资料");
add.setText("加为好友");
add.addMouseListener(new java.awt.event.MouseAdapter() {
public void mousePressed(MouseEvent e) {
add_mousePressed(e);
}
});
this.getContentPane().add(jLabel1, null);
this.getContentPane().add(new JScrollPane(list2));
this.getContentPane().add(find2, null);
this.getContentPane().add(jButton1, null);
this.getContentPane().add(jButton2, null);
this.getContentPane().add(jButton3, null);
findmenu.add(look);
findmenu.add(add);
} //以下是关闭本窗口
protected void processWindowEvent(WindowEvent e) {
super.processWindowEvent(e);
if (e.getID() == WindowEvent.WINDOW_CLOSING) {
//this.dispose();
this.hide();
}
}
//以下向服务器发送查找好友请求
void find2_mouseClicked(MouseEvent e) {
out.println("find");
DefaultListModel mm = (DefaultListModel) list2.getModel();
/////////////////find friend info
try {
String s = " ";
//从服务器读取好友信息
do {
s = in.readLine();
if (s.equals("over"))
break;
nickname.add(s);
sex.add(in.readLine());
place.add(in.readLine());
ip.add(in.readLine());
emails.add(in.readLine());
infos.add(in.readLine());
} while (!s.equals("over"));
/////////////end find info
//read their jicqno
int theirjicq, picinfo, sta;
for (int x = 0; x < nickname.size(); x++) {
theirjicq = Integer.parseInt(in.readLine());
//System.out.println(theirjicq);
jicq.add(new Integer(theirjicq));
picinfo = Integer.parseInt(in.readLine());
pic.add(new Integer(picinfo));
sta = Integer.parseInt(in.readLine());
//System.out.println(sta);
status.add(new Integer(sta));
//System.out.println(jicq.get(x));
}
//在列表中显示
for (int i = 0; i < nickname.size(); i++) {
mm.addElement(
new Object[] { nickname.get(i), sex.get(i), place.get(i)});
} //for
} catch (IOException e4) {
System.out.println("false");
}
}
//显示查找好友菜单
void list2_mousePressed(MouseEvent e) {
findmenu.show(this, e.getX() + 20, e.getY() + 50);
}
/////////////add frined
//以下将添加的好友存储在临时矢量
void add_mousePressed(MouseEvent e) {
//add friend to database
int dd;
dd = list2.getSelectedIndex();
tmpjicq.add(jicq.get(dd));
tmpname.add(nickname.get(dd));
tmpip.add(ip.get(dd)
没有合适的资源?快使用搜索试试~ 我知道了~
wssf.rar_Java 编写 用户登录 系统_java c/s _socket java_socket好友_好友socket
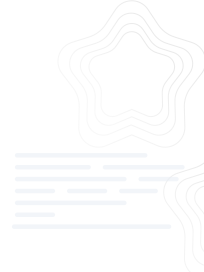
共41个文件
class:36个
java:4个
txt:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 78 浏览量
2022-09-14
14:30:49
上传
评论
收藏 53KB RAR 举报
温馨提示
JICQ是用JAVA语言编写的一个基于客户机/服务器(C/S)模式的局域短信实时通信工具系统,系统采用了Microsoft公司的SQL Server 2000作为后台数据库,系统通过JDBC访问数据库。系统分为服务器程序和客户程序两部分,服务器与客户间采用“传输控制协议”(TCP),通过套接字(Socket)连接,客户之间采用“用户数据报协议”(UDP),通过数据报套接字(DatagramSocket)建立连接。系统具有用户注册、用户登录、添加好友、删除好友、发送和接收消息等功能。
资源推荐
资源详情
资源评论
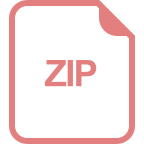
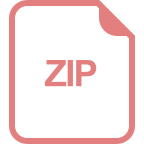
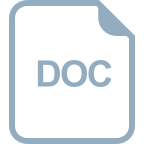
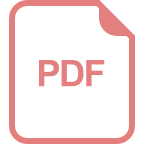
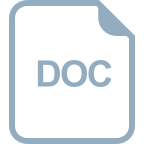
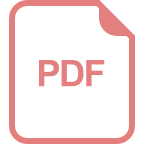
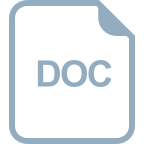
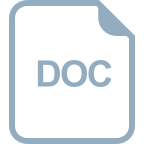
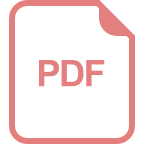
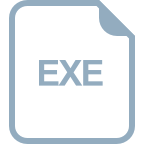
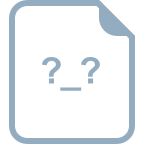
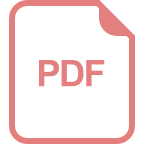
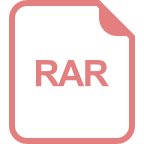
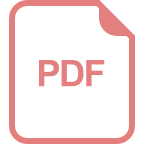
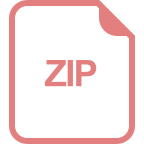
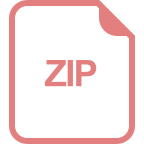
收起资源包目录


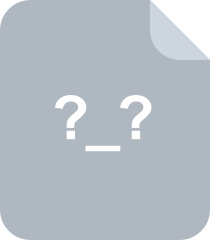
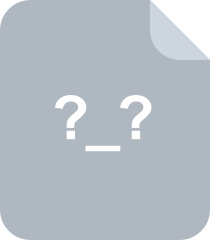
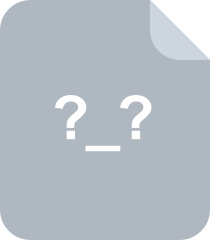
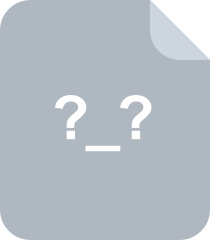
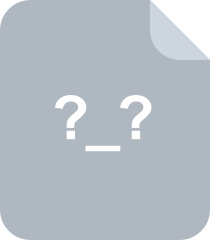
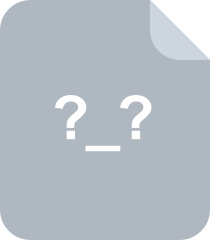
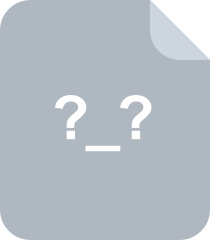
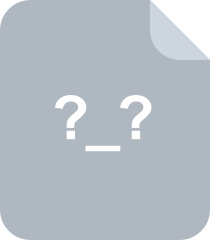
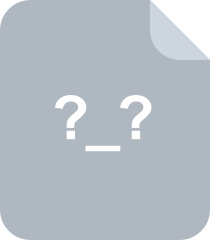
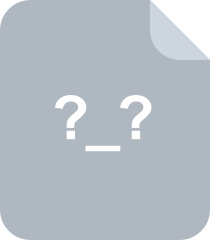
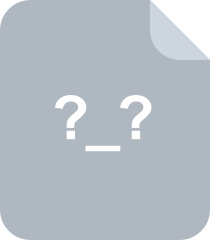
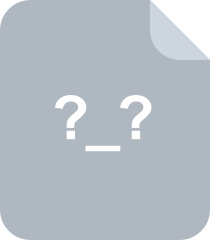
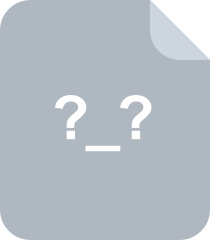
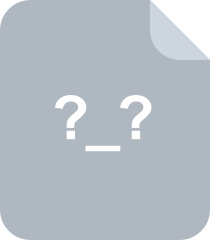
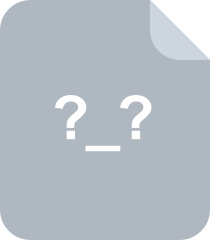
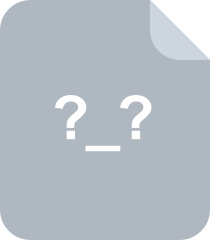
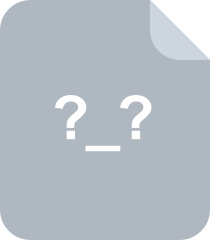
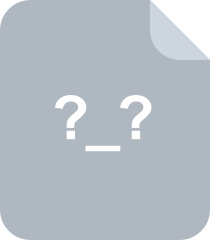
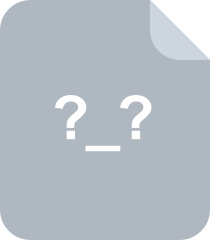
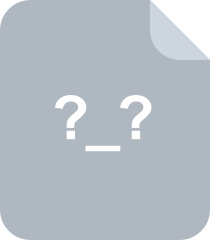
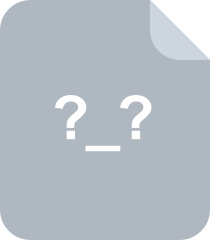
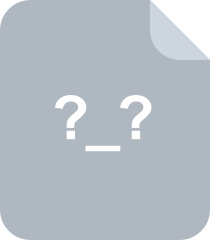
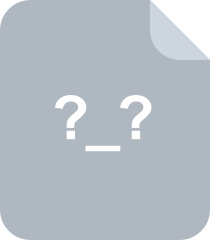
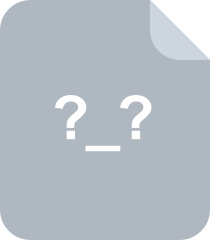
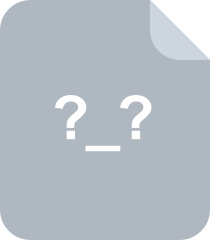
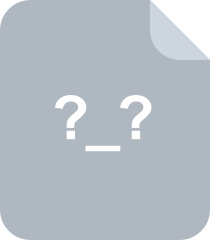
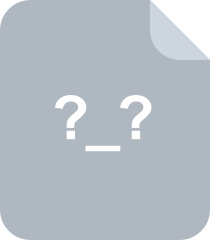
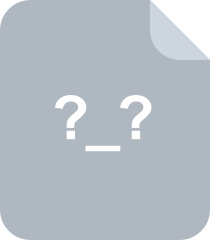
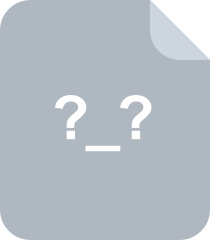
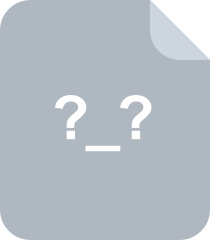
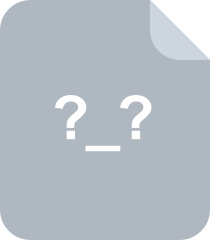
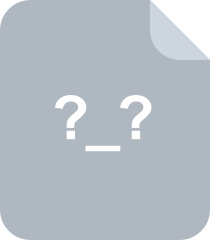
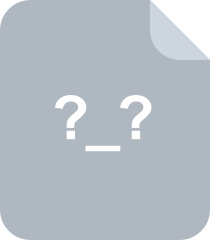
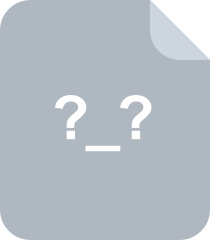
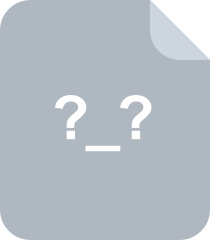
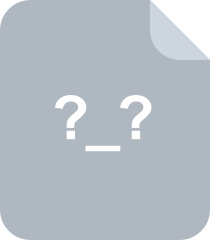
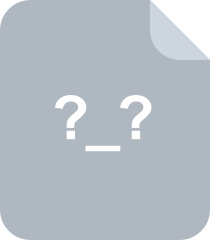
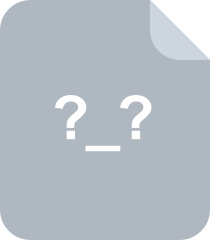
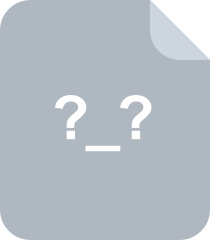
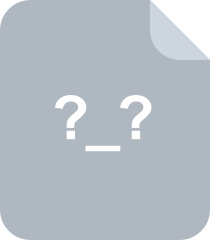
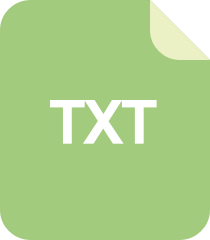
共 41 条
- 1
资源评论
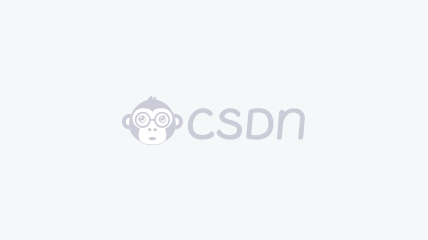

朱moyimi
- 粉丝: 75
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

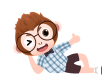
安全验证
文档复制为VIP权益,开通VIP直接复制
