#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define USERNAME "admin" // 预设用户名
#define PASSWORD "123456" // 预设密码
// 定义图书信息结构体
struct Book {
int id; // 图书编号
char title[100]; // 书名
char author[50]; // 作者
char publisher[50]; // 出版社
};
// 声明全局的结构体数组和书籍计数变量
struct Book library[50]; // 长度为50的结构体数组,用于存储图书信息
int book_count = 0; // 表示当前数据条数
// 将图书信息存储到CSV文件中
void save_to_csv(const char* filename) {
FILE* file = NULL;
if (fopen_s(&file, filename, "w") != 0) {
perror("无法打开文件以写入");
return;
}
// 写入表头
fprintf(file, "ID,Title,Author,Publisher\n");
// 写入每一本书的信息
for (int i = 0; i < book_count; i++) {
fprintf(file, "%d,%s,%s,%s\n",
library[i].id,
library[i].title,
library[i].author,
library[i].publisher);
}
fclose(file);
}
// 从CSV文件读取图书信息
void load_from_csv(const char* filename) {
FILE* file = NULL;
if (fopen_s(&file, filename, "r") != 0) {
perror("无法打开文件以读取");
return;
}
char line[256];
book_count = 0; // 清空原有数据
// 跳过表头
fgets(line, sizeof(line), file);
// 读取每一行数据
while (fgets(line, sizeof(line), file)) {
if (book_count >= 50) {
printf("数组已满,无法加载更多数据。\n");
break;
}
// 使用 sscanf_s 解析CSV行数据
sscanf_s(line, "%d,%99[^,],%49[^,],%49[^\n]",
&library[book_count].id,
library[book_count].title, (unsigned)_countof(library[book_count].title),
library[book_count].author, (unsigned)_countof(library[book_count].author),
library[book_count].publisher, (unsigned)_countof(library[book_count].publisher));
book_count++;
}
fclose(file);
}
// 检查图书编号是否重复的函数
int isIdDuplicate(int id) {
for (int i = 0; i < book_count; i++) {
if (library[i].id == id) {
return 1; // 如果编号重复,返回1
}
}
return 0; // 不重复,返回0
}
// 添加图书信息的函数,使用 scanf_s 接收用户输入
void addBook() {
if (book_count >= 50) {
printf("图书库已满,无法添加新书。\n");
return;
}
int id;
char title[100], author[50], publisher[50];
// 逐行接收用户输入
printf("请输入图书编号: ");
scanf_s("%d", &id);
// 检查图书编号是否重复
if (isIdDuplicate(id)) {
printf("图书编号重复,无法添加。\n");
return; // 直接返回函数
}
printf("请输入书名: ");
scanf_s("%99s", title, (unsigned int)sizeof(title));
printf("请输入作者: ");
scanf_s("%49s", author, (unsigned int)sizeof(author));
printf("请输入出版社: ");
scanf_s("%49s", publisher, (unsigned int)sizeof(publisher));
// 将数据保存到结构体数组中,使用 strcpy_s
library[book_count].id = id;
strcpy_s(library[book_count].title, sizeof(library[book_count].title), title);
strcpy_s(library[book_count].author, sizeof(library[book_count].author), author);
strcpy_s(library[book_count].publisher, sizeof(library[book_count].publisher), publisher);
book_count++; // 增加当前数据条数
printf("图书添加成功!\n");
}
// 检查图书编号是否存在的函数
int findBookById(int id) {
for (int i = 0; i < book_count; i++) {
if (library[i].id == id) {
return i; // 返回找到的图书索引
}
}
return -1; // 未找到,返回-1
}
// 修改图书信息的函数
void modifyBook() {
int id, choice;
printf("请输入要修改的图书编号: ");
scanf_s("%d", &id);
int index = findBookById(id);
if (index == -1) {
printf("未找到图书编号为 %d 的图书。\n", id);
return; // 图书未找到,直接返回
}
// 显示操作选项
printf("选择要修改的信息:\n");
printf("1. 书名\n");
printf("2. 作者\n");
printf("3. 出版社\n");
printf("请输入选择: ");
scanf_s("%d", &choice);
// 根据用户选择修改对应的字段
switch (choice) {
case 1: {
char newTitle[100];
printf("请输入新的书名: ");
scanf_s("%99s", newTitle, (unsigned int)sizeof(newTitle));
strcpy_s(library[index].title, sizeof(library[index].title), newTitle);
printf("书名修改成功!\n");
break;
}
case 2: {
char newAuthor[50];
printf("请输入新的作者: ");
scanf_s("%49s", newAuthor, (unsigned int)sizeof(newAuthor));
strcpy_s(library[index].author, sizeof(library[index].author), newAuthor);
printf("作者修改成功!\n");
break;
}
case 3: {
char newPublisher[50];
printf("请输入新的出版社: ");
scanf_s("%49s", newPublisher, (unsigned int)sizeof(newPublisher));
strcpy_s(library[index].publisher, sizeof(library[index].publisher), newPublisher);
printf("出版社修改成功!\n");
break;
}
default:
printf("无效选择。\n");
break;
}
}
// 查询图书信息的函数
void queryBook() {
int choice;
printf("选择查询条件:\n");
printf("1. 图书编号\n");
printf("2. 书名\n");
printf("3. 作者\n");
printf("4. 出版社\n");
printf("请输入选择: ");
scanf_s("%d", &choice);
// 根据选择进行查询
switch (choice) {
case 1: {
int id;
printf("请输入图书编号: ");
scanf_s("%d", &id);
// 输出表头
printf("\n");
printf("%-10s %-20s %-10s %-30s\n", "图书编号", "书名", "作者", "出版社");
printf("--------------------------------------------------------------\n");
for (int i = 0; i < book_count; i++) {
if (library[i].id == id) {
printf("%-10d %-20s %-10s %-30s\n", library[i].id, library[i].title, library[i].author, library[i].publisher);
return; // 找到后返回
}
}
printf("未找到图书编号为 %d 的图书。\n", id);
break;
}
case 2: {
char title[100];
printf("请输入书名: ");
scanf_s("%99s", title, (unsigned int)sizeof(title));
int found = 0;
// 输出表头
printf("\n");
printf("%-10s %-20s %-10s %-30s\n", "图书编号", "书名", "作者", "出版社");
printf("--------------------------------------------------------------\n");
for (int i = 0; i < book_count; i++) {
if (strcmp(library[i].title, title) == 0) {
printf("%-10d %-20s %-10s %-30s\n", library[i].id, library[i].title, library[i].author, library[i].publisher);
found = 1;
}
}
if (!found) {
printf("未找到书名为 %s 的图书。\n", title);
}
break;
}
case 3: {
char author[50];
printf("请输入作者: ");
scanf_s("%49s", author, (unsigned int)sizeof(author));
int found = 0;
// 输出表头
printf("\n");
printf("%-10s %-20s %-10s %-30s\n", "图书编号", "书名", "作者", "出版社");
printf("--------------------------------------------------------------\n");
for (int i = 0; i < book_count; i++)
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
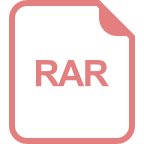
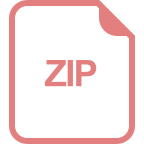
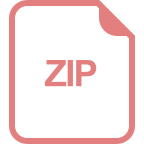
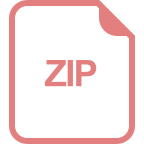
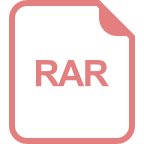
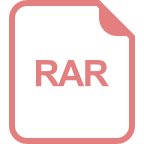
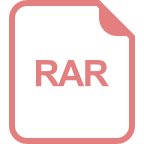
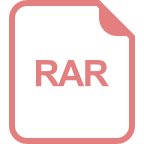
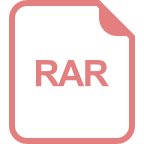
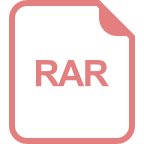
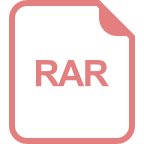
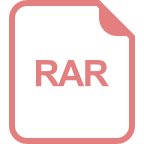
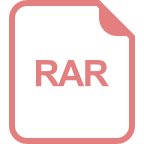
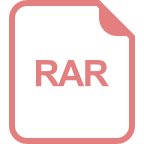
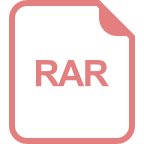
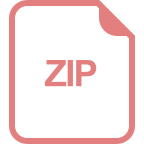
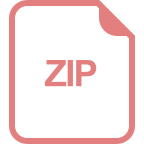
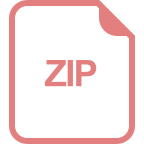
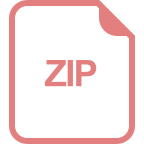
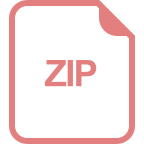
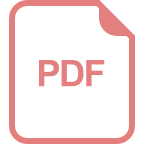
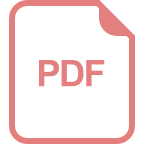
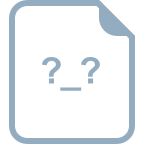
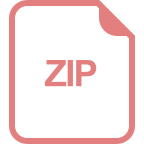
收起资源包目录





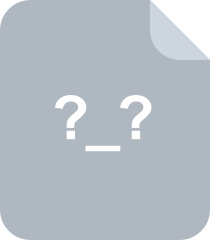
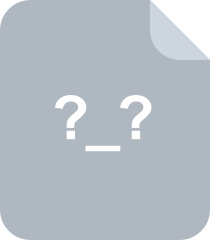
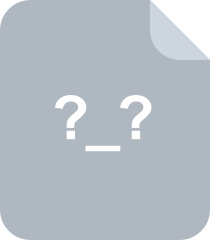
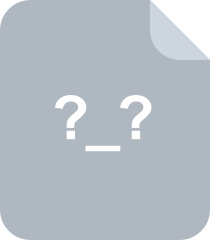



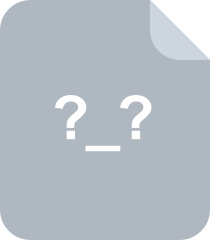
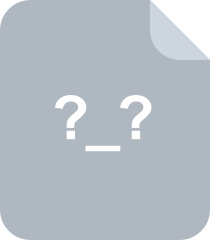

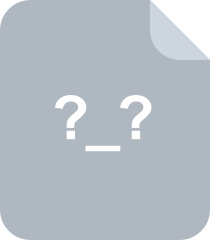
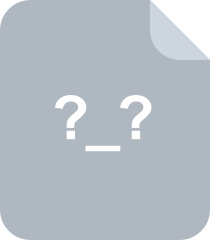


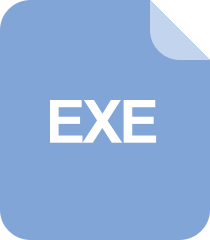
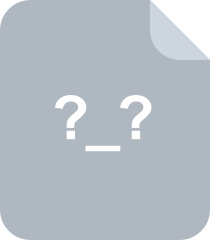

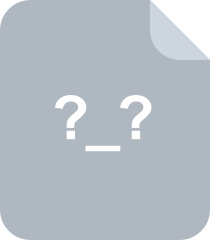


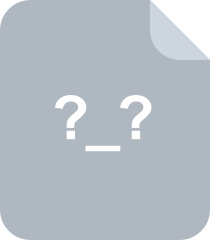
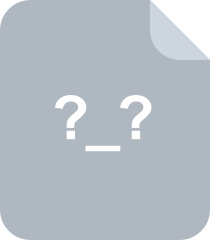
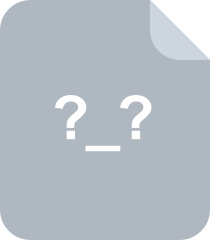
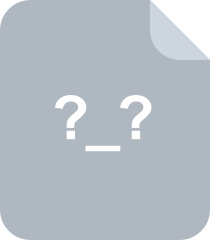

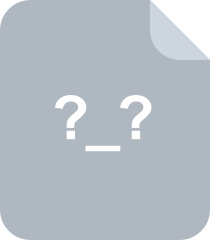
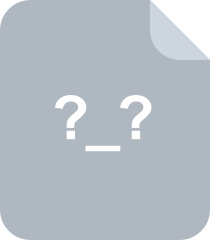
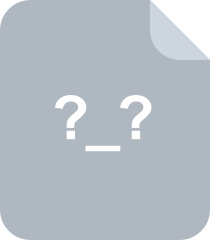
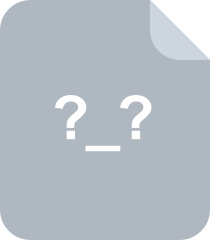
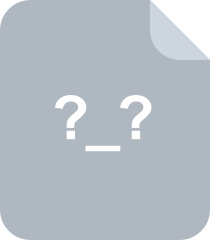
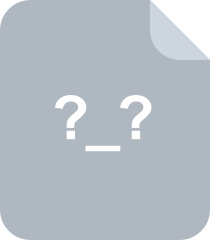
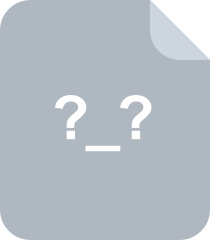
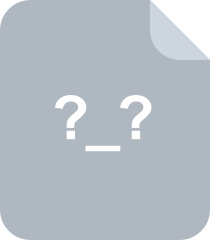
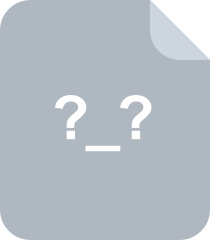
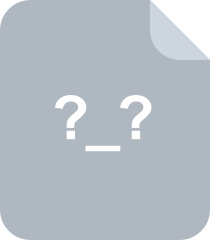
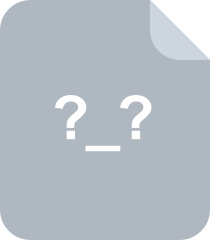
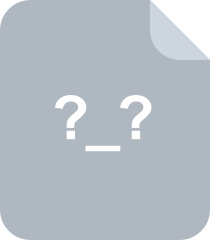
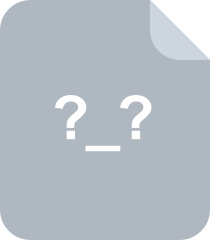
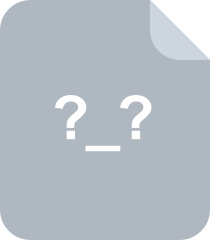
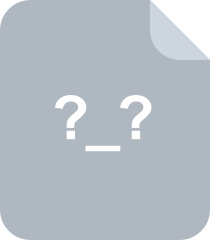
共 30 条
- 1
资源评论
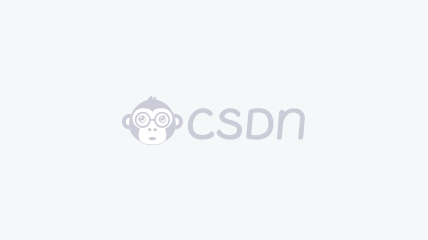

小辰代写
- 粉丝: 4187
- 资源: 100
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

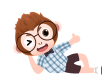
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


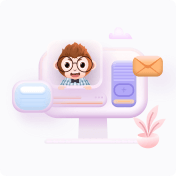
安全验证
文档复制为VIP权益,开通VIP直接复制
