#ifndef _IPATH_KERNEL_H
#define _IPATH_KERNEL_H
/*
* Copyright (c) 2006, 2007, 2008 QLogic Corporation. All rights reserved.
* Copyright (c) 2003, 2004, 2005, 2006 PathScale, Inc. All rights reserved.
*
* This software is available to you under a choice of one of two
* licenses. You may choose to be licensed under the terms of the GNU
* General Public License (GPL) Version 2, available from the file
* COPYING in the main directory of this source tree, or the
* OpenIB.org BSD license below:
*
* Redistribution and use in source and binary forms, with or
* without modification, are permitted provided that the following
* conditions are met:
*
* - Redistributions of source code must retain the above
* copyright notice, this list of conditions and the following
* disclaimer.
*
* - Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials
* provided with the distribution.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/*
* This header file is the base header file for infinipath kernel code
* ipath_user.h serves a similar purpose for user code.
*/
#include <linux/interrupt.h>
#include <linux/pci.h>
#include <linux/dma-mapping.h>
#include <linux/mutex.h>
#include <linux/list.h>
#include <linux/scatterlist.h>
#include <asm/io.h>
#include <rdma/ib_verbs.h>
#include "ipath_common.h"
#include "ipath_debug.h"
#include "ipath_registers.h"
/* only s/w major version of InfiniPath we can handle */
#define IPATH_CHIP_VERS_MAJ 2U
/* don't care about this except printing */
#define IPATH_CHIP_VERS_MIN 0U
/* temporary, maybe always */
extern struct infinipath_stats ipath_stats;
#define IPATH_CHIP_SWVERSION IPATH_CHIP_VERS_MAJ
/*
* First-cut critierion for "device is active" is
* two thousand dwords combined Tx, Rx traffic per
* 5-second interval. SMA packets are 64 dwords,
* and occur "a few per second", presumably each way.
*/
#define IPATH_TRAFFIC_ACTIVE_THRESHOLD (2000)
/*
* Struct used to indicate which errors are logged in each of the
* error-counters that are logged to EEPROM. A counter is incremented
* _once_ (saturating at 255) for each event with any bits set in
* the error or hwerror register masks below.
*/
#define IPATH_EEP_LOG_CNT (4)
struct ipath_eep_log_mask {
u64 errs_to_log;
u64 hwerrs_to_log;
};
struct ipath_portdata {
void **port_rcvegrbuf;
dma_addr_t *port_rcvegrbuf_phys;
/* rcvhdrq base, needs mmap before useful */
void *port_rcvhdrq;
/* kernel virtual address where hdrqtail is updated */
void *port_rcvhdrtail_kvaddr;
/*
* temp buffer for expected send setup, allocated at open, instead
* of each setup call
*/
void *port_tid_pg_list;
/* when waiting for rcv or pioavail */
wait_queue_head_t port_wait;
/*
* rcvegr bufs base, physical, must fit
* in 44 bits so 32 bit programs mmap64 44 bit works)
*/
dma_addr_t port_rcvegr_phys;
/* mmap of hdrq, must fit in 44 bits */
dma_addr_t port_rcvhdrq_phys;
dma_addr_t port_rcvhdrqtailaddr_phys;
/*
* number of opens (including slave subports) on this instance
* (ignoring forks, dup, etc. for now)
*/
int port_cnt;
/*
* how much space to leave at start of eager TID entries for
* protocol use, on each TID
*/
/* instead of calculating it */
unsigned port_port;
/* non-zero if port is being shared. */
u16 port_subport_cnt;
/* non-zero if port is being shared. */
u16 port_subport_id;
/* number of pio bufs for this port (all procs, if shared) */
u32 port_piocnt;
/* first pio buffer for this port */
u32 port_pio_base;
/* chip offset of PIO buffers for this port */
u32 port_piobufs;
/* how many alloc_pages() chunks in port_rcvegrbuf_pages */
u32 port_rcvegrbuf_chunks;
/* how many egrbufs per chunk */
u32 port_rcvegrbufs_perchunk;
/* order for port_rcvegrbuf_pages */
size_t port_rcvegrbuf_size;
/* rcvhdrq size (for freeing) */
size_t port_rcvhdrq_size;
/* next expected TID to check when looking for free */
u32 port_tidcursor;
/* next expected TID to check */
unsigned long port_flag;
/* what happened */
unsigned long int_flag;
/* WAIT_RCV that timed out, no interrupt */
u32 port_rcvwait_to;
/* WAIT_PIO that timed out, no interrupt */
u32 port_piowait_to;
/* WAIT_RCV already happened, no wait */
u32 port_rcvnowait;
/* WAIT_PIO already happened, no wait */
u32 port_pionowait;
/* total number of rcvhdrqfull errors */
u32 port_hdrqfull;
/*
* Used to suppress multiple instances of same
* port staying stuck at same point.
*/
u32 port_lastrcvhdrqtail;
/* saved total number of rcvhdrqfull errors for poll edge trigger */
u32 port_hdrqfull_poll;
/* total number of polled urgent packets */
u32 port_urgent;
/* saved total number of polled urgent packets for poll edge trigger */
u32 port_urgent_poll;
/* pid of process using this port */
struct pid *port_pid;
struct pid *port_subpid[INFINIPATH_MAX_SUBPORT];
/* same size as task_struct .comm[] */
char port_comm[16];
/* pkeys set by this use of this port */
u16 port_pkeys[4];
/* so file ops can get at unit */
struct ipath_devdata *port_dd;
/* A page of memory for rcvhdrhead, rcvegrhead, rcvegrtail * N */
void *subport_uregbase;
/* An array of pages for the eager receive buffers * N */
void *subport_rcvegrbuf;
/* An array of pages for the eager header queue entries * N */
void *subport_rcvhdr_base;
/* The version of the library which opened this port */
u32 userversion;
/* Bitmask of active slaves */
u32 active_slaves;
/* Type of packets or conditions we want to poll for */
u16 poll_type;
/* port rcvhdrq head offset */
u32 port_head;
/* receive packet sequence counter */
u32 port_seq_cnt;
};
struct sk_buff;
struct ipath_sge_state;
struct ipath_verbs_txreq;
/*
* control information for layered drivers
*/
struct _ipath_layer {
void *l_arg;
};
struct ipath_skbinfo {
struct sk_buff *skb;
dma_addr_t phys;
};
struct ipath_sdma_txreq {
int flags;
int sg_count;
union {
struct scatterlist *sg;
void *map_addr;
};
void (*callback)(void *, int);
void *callback_cookie;
int callback_status;
u16 start_idx; /* sdma private */
u16 next_descq_idx; /* sdma private */
struct list_head list; /* sdma private */
};
struct ipath_sdma_desc {
__le64 qw[2];
};
#define IPATH_SDMA_TXREQ_F_USELARGEBUF 0x1
#define IPATH_SDMA_TXREQ_F_HEADTOHOST 0x2
#define IPATH_SDMA_TXREQ_F_INTREQ 0x4
#define IPATH_SDMA_TXREQ_F_FREEBUF 0x8
#define IPATH_SDMA_TXREQ_F_FREEDESC 0x10
#define IPATH_SDMA_TXREQ_F_VL15 0x20
#define IPATH_SDMA_TXREQ_S_OK 0
#define IPATH_SDMA_TXREQ_S_SENDERROR 1
#define IPATH_SDMA_TXREQ_S_ABORTED 2
#define IPATH_SDMA_TXREQ_S_SHUTDOWN 3
#define IPATH_SDMA_STATUS_SCORE_BOARD_DRAIN_IN_PROG (1ull << 63)
#define IPATH_SDMA_STATUS_ABORT_IN_PROG (1ull << 62)
#define IPATH_SDMA_STATUS_INTERNAL_SDMA_ENABLE (1ull << 61)
#define IPATH_SDMA_STATUS_SCB_EMPTY (1ull << 30)
/* max dwords in small buffer packet */
#define IPATH_SMALLBUF_DWORDS (dd->ipath_piosize2k >> 2)
/*
* Possible IB config parameters for ipath_f_get/set_ib_cfg()
*/
#define IPATH_IB_CFG_LIDLMC 0 /* Get/set LID (LS16b) and Mask (MS16b) */
#define IPATH_IB_CFG_HRTBT 1 /* Get/set Heartbeat off/enable/auto */
#define IPATH_IB_HRTBT_ON 3 /* Heartbeat enabled, sent every 100msec */
#define IPATH_IB_HRTBT_OFF 0 /* Hea
没有合适的资源?快使用搜索试试~ 我知道了~
scsi_transport_api.rar_Transport.h_purpose
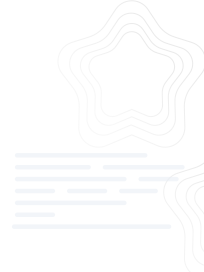
共2个文件
c:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 78 浏览量
2022-09-19
22:01:13
上传
评论
收藏 14KB RAR 举报
温馨提示
This header file is the base header file for infinipath kernel code ipath_user.h serves a similar purpose for user code.
资源推荐
资源详情
资源评论
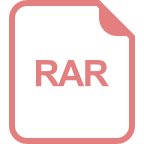
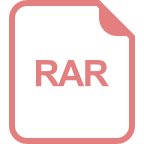
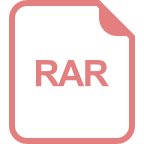
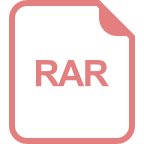
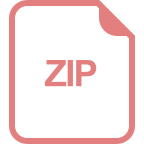
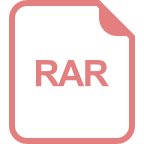
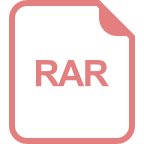
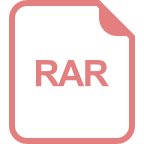
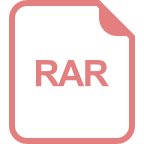
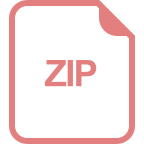
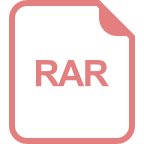
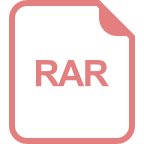
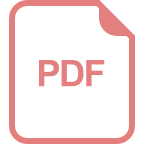
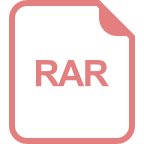
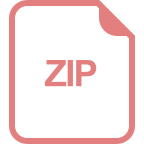
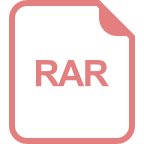
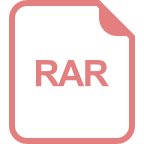
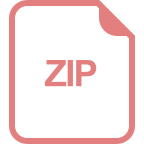
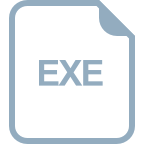
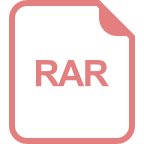
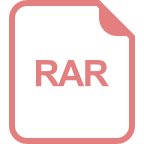
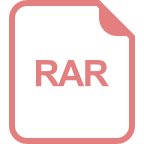
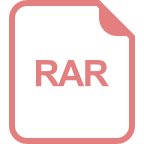
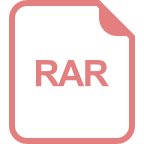
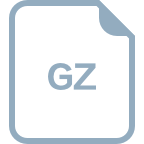
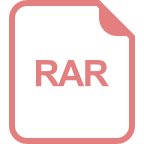
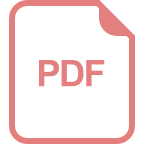
收起资源包目录

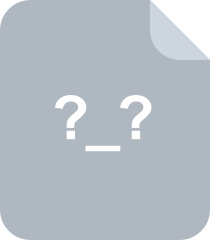
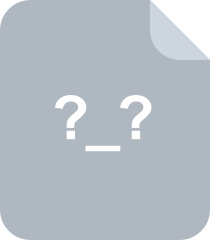
共 2 条
- 1
资源评论
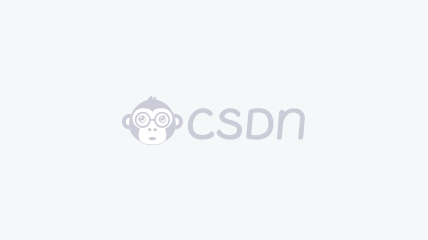
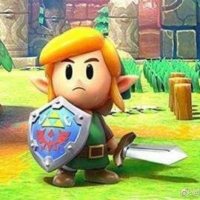
御道御小黑
- 粉丝: 74
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

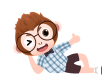
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


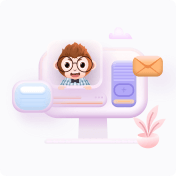
安全验证
文档复制为VIP权益,开通VIP直接复制
