NN_test.rar_MLP
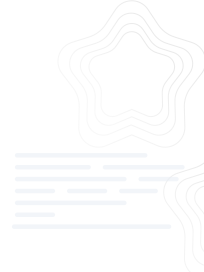

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
**标题解析:** "NN_test.rar_MLP" 这个标题暗示了我们处理的是一个关于多层感知器(MLP)神经网络的测试项目。".rar" 是一个压缩文件格式,通常用于打包多个相关文件,而"_MLP" 指明了文件内容与机器学习中的多层感知器网络有关。 **描述解析:** "Running/Simulating an MLP Neural Network" 描述指出我们将探讨如何运行或模拟一个MLP神经网络。这可能包括设置网络结构、训练过程、数据预处理、模型评估等步骤。 **标签解析:** "mlp" 这个标签进一步确认了主要内容是关于多层感知器,这是神经网络的一个基本类型,常用于分类和回归问题。 **文件名称列表:** "NN_test.cpp" 表示这是一个用C++编写的程序,很可能包含了实现MLP网络的代码,用于测试或演示其功能。 **详细知识点:** 1. **多层感知器(MLP)**:MLP是一种前馈神经网络,由至少一个隐藏层和输入/输出层组成,允许非线性数据建模。每个神经元执行加权求和和激活函数的操作。 2. **神经网络结构**:MLP包含输入层,一个或多个隐藏层,以及一个输出层。每个层由多个神经元组成,相邻层的神经元之间有连接权重。 3. **激活函数**:常见的激活函数有sigmoid、ReLU(Rectified Linear Unit)、tanh等,它们引入非线性,使网络能够学习更复杂的模式。 4. **反向传播(Backpropagation)**:这是训练MLP的主要算法,通过计算损失函数关于权重的梯度来更新权重,以最小化预测与真实值之间的差异。 5. **损失函数**:如均方误差(MSE)或交叉熵损失,衡量模型预测与实际结果的差距。 6. **训练过程**:通过迭代的方式,用训练数据集反复调整权重,直到模型性能达到预定标准。 7. **超参数**:如学习率、批次大小、网络层数和每层的神经元数量,这些在训练前需要设定。 8. **数据预处理**:包括标准化、归一化、填充缺失值等,使数据更适合神经网络模型。 9. **模型评估**:使用验证集进行模型性能评估,可能的指标有准确率、精确率、召回率、F1分数等。 10. **C++实现**:在"NN_test.cpp"中,会涉及C++的数据结构、函数、类和库(如OpenCV、Eigen等)来实现和运行MLP模型。 11. **编译与运行**:C++代码需要通过编译器转化为可执行文件,然后才能运行并观察神经网络的模拟结果。 12. **调试与优化**:代码可能需要经过多次迭代,调整参数,以获得最佳性能。 以上就是基于标题、描述和标签生成的多层感知器神经网络相关知识点,涵盖了理论基础、实现细节和评估方法。
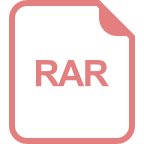
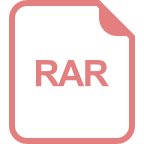
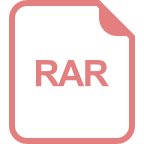
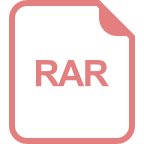
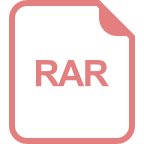
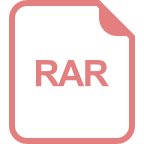
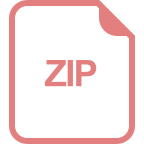
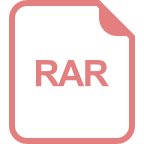
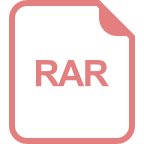
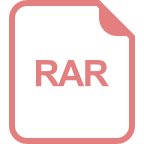
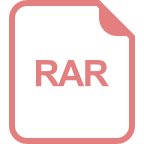
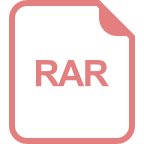
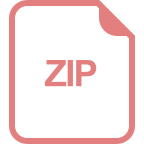
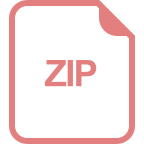
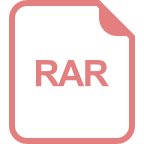
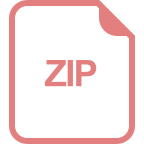
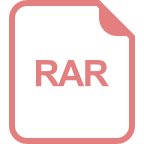
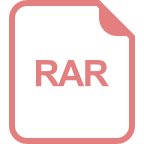
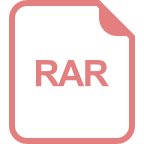
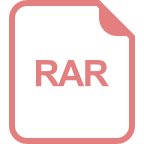
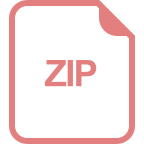
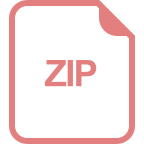
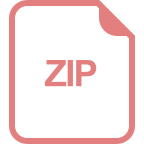
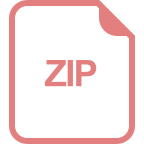

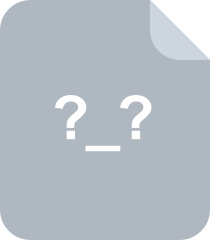
- 1
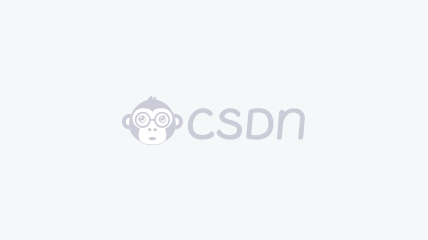

- 粉丝: 94
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

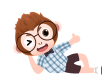
最新资源
- 基于区块链的乳制品溯源系统文档+源码+全部资料+高分项目.zip
- 基于区块链技术之可溯源珠宝电商平台文档+源码+全部资料+高分项目.zip
- 基于区块链的药品溯源系统(学习开发中)文档+源码+全部资料+高分项目.zip
- 基于事件驱动+事件溯源+Saga的微服务示例文档+源码+全部资料+高分项目.zip
- 基于使用Axon框架基于DDD领域驱动设计、CQRS读写分离和事件溯源来实现货物运输系统文档+源码+全部资料+高分项目.zip
- 基于若依后台管理系统的代码溯源系统文档+源码+全部资料+高分项目.zip
- 基于以太坊 Solidity 语言开发秒钛坊区块链智能合约致辞供应链金融信贷周期全流程溯源文档+源码+全部资料+高分项目.zip
- 基于事件溯源基于事件回溯的高性能架构,例如:秒杀、抢红包、12306卖票等,实现cqrs最复杂的模型, 通过事件是追加的特性,然后结合事件批量提交的手段,避免在
- Visual Studio Code中的IntelliSense功能详解.pdf
- 基于溯源图的入侵威胁检测相关论文及阅读笔记文档+源码+全部资料+高分项目.zip
- Keil C51 插件 检测所有if语句
- 基于优雅的Laravel框架开发咖啡壶是一个免费、开源、高效且漂亮的资产管理平台。资产管理、归属使用者追溯、盘点以及可靠的服务器状态管理面板文档+源码+全部资料+高分项目.zip
- 基于云链聚合的隐私保护数据共享与溯源平台文档+源码+全部资料+高分项目.zip
- 各种排序算法java实现的源代码.zip
- java考试题目总132
- 用c语言实现各种排序算法

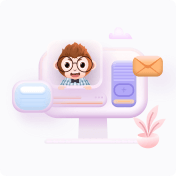
