/**
******************************************************************************
* @file NorFlash.c
* @author YqZhao
* @version V1.0.0
* @date 26-April-2013
* @brief This file provides firmware functions to manage the following
* functionalities of the NorFlash FSMC peripheral:
* - Initialization FSMC bus of NorFlash
* - Write datas of the NorFlash
* - Read datas of the NorFlash
* - Read ID
* - Erase the section of the NorFlash
*
* @verbatim
*
* ===================================================================
* How to use this driver
* ===================================================================
* 1. FSMC pins configuration about NorFlash in User.h
*
* 2. Initialise and configure the NorFlash using S29glInit
* PLS refer to the stm32f2xx_fsmc.c file
*
* 3. Erase the desired section using s29glSectorErase if writing data needed
*
*
* 4. Write the desired data using s29glWrite.
*
* 5. Read the desired data using s29glRead.
*
* 6. If needed read the ID using S29gReadId.
* @endverbatim
*
******************************************************************************
* @attention
* SectionNum Address ________ __________
0 0x000000 ________| | ________ 16bits
1 0x020000 ________| | Data
2 0x040000 | |
| |
| |
| |
255 0x1FE0000 ________| |
|_________________ |
*
******************************************************************************
*/
#include "include.h"
#ifndef WP_NORFLASH
#define WP_NORFLASH PE_O.BitSet.v01
#endif
#ifndef RESET_NORFLASH
#define RESET_NORFLASH PE_O.BitSet.v01
#endif
/*******************************************************************************
Function: Nor Flash Erase Operation
Param : sectorNum stands for the beigin of section
sectorCount stans for how many sections need erase
Return : NOR_Status
NOR_SUCCESS = 0, // Stands for success
NOR_ONGOING, // Stands for ongoing something
NOR_ERROR, // Stands for error
NOR_TIMEOUT // Stands for time out
Comment : When need write data must erase the section respectively first
*******************************************************************************/
NOR_Status s29glSectorErase( int sectorNum, int sectorCount)
{
int ii,jj;
uint16_t wTemp1,wTemp2;
volatile uint16_t* pFlash;
unsigned int timeout;
for ( ii = 0; ii < sectorCount; ii++ )
{
pFlash = ( uint16_t * ) ( NOR_FLASH_START_ADDR+( sectorNum + ii ) * ( 128*1024 ) );
NOR_WRITE(ADDR_SHIFT(0x00), 0xf0);/* RESET ????*/
NOR_WRITE(ADDR_SHIFT(0x555),0xaa);
NOR_WRITE(ADDR_SHIFT(0x2aa),0x55);
NOR_WRITE(ADDR_SHIFT(0x555),0x80);
NOR_WRITE(ADDR_SHIFT(0x555),0xaa);
NOR_WRITE(ADDR_SHIFT(0x2aa),0x55);
*pFlash = 0x30;
wTemp1 = *pFlash & 0x40;
timeout = 0xf000000;
while ( 1 )
{
FEED_DOG();
wTemp2 = *pFlash & 0x40;
if ( wTemp1 == wTemp2 )
{
break;
}
else
{
wTemp1 = wTemp2;
}
if ( timeout-- <= 0 )
{
return ( NOR_TIMEOUT );
}
}
NOR_WRITE(ADDR_SHIFT(0x00), 0xf0f0);/* RESET ????*/
for ( jj = 0; jj < 128*1024/2; jj++ )/* Maybe too long time */
{
if ( *pFlash != 0xffff )
{
return ( NOR_ERROR );
}
pFlash ++;
}
}
return(NOR_SUCCESS);
}
/*******************************************************************************
Function: Nor Flash Write Operation
Param : address stands for the beigin of address
buffer stans for the datas be written into the NorFlash
length stans for how many bytes need to write NorFlash
Return : NOR_Status
NOR_SUCCESS = 0, // Stands for success
NOR_ONGOING, // Stands for ongoing something
NOR_ERROR, // Stands for error
NOR_TIMEOUT // Stands for time out
Comment :
*******************************************************************************/
NOR_Status s29glWrite( uint32_t address, uint8_t * buffer, int length )
{
uint16_t ii;
uint32_t timeout;
uint16_t *pBuffer=(uint16_t *)buffer;
volatile uint16_t *pFlash;
uint16_t wTemp1, wTemp2;
pFlash =(uint16_t *)(NOR_FLASH_START_ADDR + address);
for ( ii = 0; ii <(length>>1); ii++ )
{
NOR_WRITE(ADDR_SHIFT(0x555),0xaa);
NOR_WRITE(ADDR_SHIFT(0x2aa),0x55);
NOR_WRITE(ADDR_SHIFT(0x555),0xa0);
*pFlash = *pBuffer;
wTemp1 = *pFlash & 0x40;
timeout = 0x5000000;
while ( 1 )
{
FEED_DOG();
wTemp2 = *pFlash & 0x40;
if ( wTemp1 == wTemp2 )
{
break;
}
else
{
wTemp1 = wTemp2;
}
if ( timeout-- <= 0 )
{
return ( NOR_TIMEOUT );
}
}
NOR_WRITE(ADDR_SHIFT(0x00), 0xf0);
if ( *pFlash != *pBuffer )
{
return ( NOR_ERROR );
}
pFlash ++;
pBuffer ++;
}
return (NOR_SUCCESS);
}
/*******************************************************************************
Function: Nor Flash Read Operation
Param : address stands for the beigin of address
buffer stans for the datas to be read our of the NorFlash
length stans for how many bytes need to read NorFlash
Return : No
Comment :
*******************************************************************************/
void s29glRead(uint32_t address, uint8_t * buffer, int length )
{
uint16_t *pBuffer=(uint16_t *)buffer;
volatile uint16_t *pFlash;
uint32_t ii;
NOR_WRITE(ADDR_SHIFT(0x0555), 0x00AA);
NOR_WRITE(ADDR_SHIFT(0x02AA), 0x0055);
NOR_WRITE((NOR_FLASH_START_ADDR + address), 0x00F0);
pFlash =(uint16_t *)(NOR_FLASH_START_ADDR + address);
for( ii=0; ii<(length>>1); ii++)
{
*pBuffer++ = *pFlash++;
}
}
/*******************************************************************************
Function: Nor Flash initiation Operation
Param : NOR_ID save the ID read form chip
Return : No
Comment :
*******************************************************************************/
void S29gReadId(NOR_IDTypeDef* NOR_ID)
{
NOR_WRITE(ADDR_SHIFT(0x0555), 0x00AA);
NOR_WRITE(ADDR_SHIFT(0x02AA), 0x0055);
NOR_WRITE(ADDR_SHIFT(0x0555), 0x0090);
NOR_ID->Manufacturer_Code = *(vu16 *) ADDR_SHIFT(0x0000);
NOR_ID->Device_Code1 = *(vu16 *) ADDR_SHI

小波思基
- 粉丝: 86
- 资源: 1万+
最新资源
- AI视觉云台_案例程序的加载方法.zip
- Python实现HTML压缩功能
- 云原生-k8s知识学习-CKA考前培训
- 对象检测23-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 快速排序在Go中的高效实现与应用
- 根据SQL代码查询数据后,自动打印
- 用HTML5和JavaScript实现动态过年鞭炮场景
- Windows检查电池健康度的批处理脚本实现
- 贝尔金F9L1101V2 无线网卡驱动 V1027.2.1001.2014-11-13-2014-6.1-x64,WIN7 X64亲测可用 下载并解压后只有4个小文件,需手动更新,浏览指到下载文件夹
- 中科岩创桥梁自动化监测解决方案
- An End-to-End Learning Framework for Video Compression
- jieba分词哈工大停用词表
- C#自定义事件 2024年12月23日
- (2147634)经典C程序100例 很经典的例子
- (22151828)图书管理系统!
- 快速排序算法详解及Python实现
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


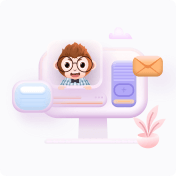
评论0