package java.util;
/**
* AbstractSequentialList is an abstract implementation of the List interface.
* This implementation does not support adding. A subclass must implement the
* abstract method listIterator().
*
* @since 1.2
*/
public abstract class AbstractSequentialList<E> extends AbstractList<E> {
/**
* Constructs a new instance of this AbstractSequentialList.
*/
protected AbstractSequentialList() {
super();
}
@Override
public void add(int location, E object) {
listIterator(location).add(object);
}
@Override
public boolean addAll(int location, Collection<? extends E> collection) {
ListIterator<E> it = listIterator(location);
Iterator<? extends E> colIt = collection.iterator();
int next = it.nextIndex();
while (colIt.hasNext()) {
it.add(colIt.next());
}
return next != it.nextIndex();
}
@Override
public E get(int location) {
try {
return listIterator(location).next();
} catch (NoSuchElementException e) {
throw new IndexOutOfBoundsException();
}
}
@Override
public Iterator<E> iterator() {
return listIterator(0);
}
@Override
public abstract ListIterator<E> listIterator(int location);
@Override
public E remove(int location) {
try {
ListIterator<E> it = listIterator(location);
E result = it.next();
it.remove();
return result;
} catch (NoSuchElementException e) {
throw new IndexOutOfBoundsException();
}
}
@Override
public E set(int location, E object) {
ListIterator<E> it = listIterator(location);
if (!it.hasNext()) {
throw new IndexOutOfBoundsException();
}
E result = it.next();
it.set(object);
return result;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
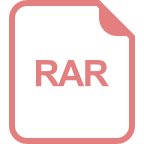
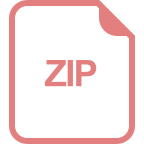
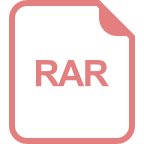
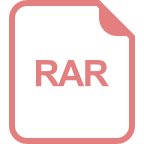
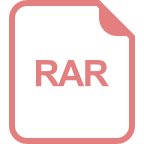
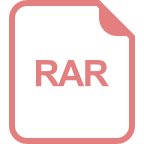
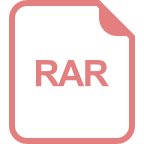
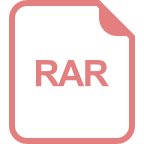
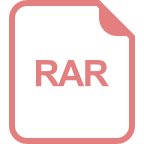
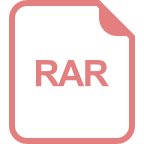
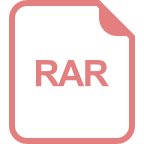
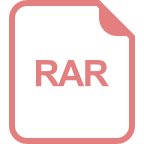
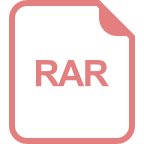
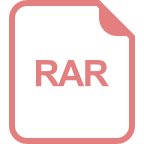
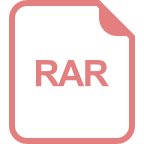
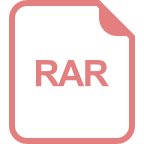
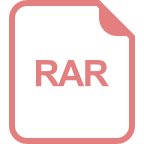
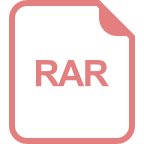
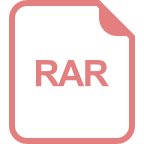
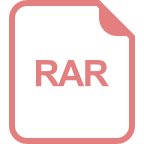
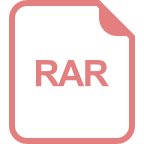
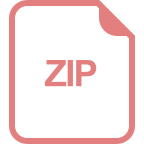
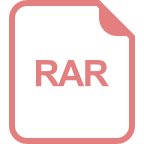
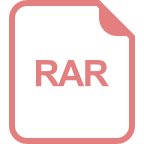
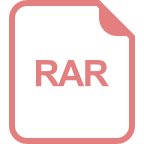
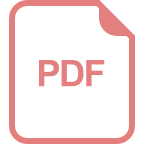
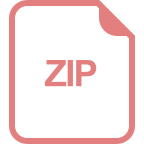
收起资源包目录

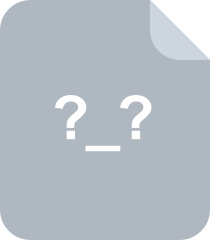
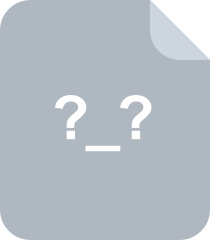
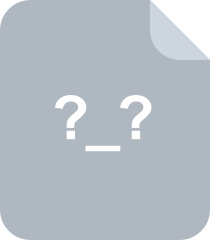
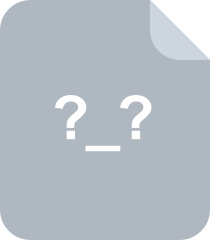
共 4 条
- 1
资源评论
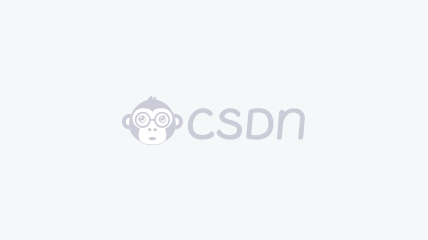

我虽横行却不霸道
- 粉丝: 90
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

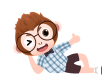
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


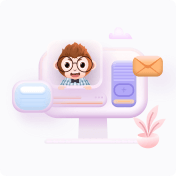
安全验证
文档复制为VIP权益,开通VIP直接复制
