# Complex YOLOv4
[![python-image]][python-url]
[![pytorch-image]][pytorch-url]
The PyTorch Implementation based on YOLOv4 of the paper: [Complex-YOLO: Real-time 3D Object Detection on Point Clouds](https://arxiv.org/pdf/1803.06199.pdf)
---
## Features
- [x] Realtime 3D object detection based on YOLOv4
- [x] Support [distributed data parallel training](https://github.com/pytorch/examples/tree/master/distributed/ddp)
- [x] Tensorboard
- [x] Mosaic/Cutout augmentation for training
- [x] Use [GIoU](https://arxiv.org/pdf/1902.09630v2.pdf) loss of rotated boxes for optimization.
- **Update 2020.08.26**: [Super Fast and Accurate 3D Object Detection based on 3D LiDAR Point Clouds](https://github.com/maudzung/Super-Fast-Accurate-3D-Object-Detection)
- Faster training, faster inference
- An Anchor-free approach
- No need for Non-Max-Suppression
- Demonstration (on a GTX 1080Ti)
[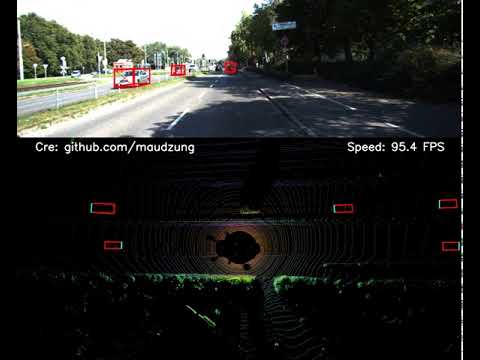](http://www.youtube.com/watch?v=FI8mJIXkgX4)
**[Youtube link](https://youtu.be/FI8mJIXkgX4)**
## 2. Getting Started
### 2.1. Requirement
```shell script
pip install -U -r requirements.txt
```
For [`mayavi`](https://docs.enthought.com/mayavi/mayavi/installation.html) and [`shapely`](https://shapely.readthedocs.io/en/latest/project.html#installing-shapely)
libraries, please refer to the installation instructions from their official websites.
### 2.2. Data Preparation
Download the 3D KITTI detection dataset from [here](http://www.cvlibs.net/datasets/kitti/eval_object.php?obj_benchmark=3d).
The downloaded data includes:
- Velodyne point clouds _**(29 GB)**_: input data to the Complex-YOLO model
- Training labels of object data set _**(5 MB)**_: input label to the Complex-YOLO model
- Camera calibration matrices of object data set _**(16 MB)**_: for visualization of predictions
- Left color images of object data set _**(12 GB)**_: for visualization of predictions
Please make sure that you construct the source code & dataset directories structure as below.
For 3D point cloud preprocessing, please refer to the previous works:
- [VoxelNet-Pytorch](https://github.com/skyhehe123/VoxelNet-pytorch)
- [Complex-YOLOv2](https://github.com/AI-liu/Complex-YOLO)
- [Complex-YOLOv3](https://github.com/ghimiredhikura/Complex-YOLOv3)
### 2.3. Complex-YOLO architecture

This work has been based on the paper [YOLOv4: Optimal Speed and Accuracy of Object Detection](https://arxiv.org/abs/2004.10934).
Please refer to several implementations of YOLOv4 using PyTorch DL framework:
- [Tianxiaomo/pytorch-YOLOv4](https://github.com/Tianxiaomo/pytorch-YOLOv4)
- [Ultralytics/yolov3_and_v4](https://github.com/ultralytics/yolov3)
- [WongKinYiu/PyTorch_YOLOv4](https://github.com/WongKinYiu/PyTorch_YOLOv4)
- [VCasecnikovs/Yet-Another-YOLOv4-Pytorch](https://github.com/VCasecnikovs/Yet-Another-YOLOv4-Pytorch)
### 2.4. How to run
#### 2.4.1. Visualize the dataset (both BEV images from LiDAR and camera images)
```shell script
cd src/data_process
```
- To visualize BEV maps and camera images (with 3D boxes), let's execute _**(the `output-width` param can be changed to
show the images in a bigger/smaller window)**_:
```shell script
python kitti_dataloader.py --output-width 608
```
- To visualize mosaics that are composed from 4 BEV maps (Using during training only), let's execute:
```shell script
python kitti_dataloader.py --show-train-data --mosaic --output-width 608
```
By default, there is _**no padding**_ for the output mosaics, the feature could be activated by executing:
```shell script
python kitti_dataloader.py --show-train-data --mosaic --random-padding --output-width 608
```
- To visualize cutout augmentation, let's execute:
```shell script
python kitti_dataloader.py --show-train-data --cutout_prob 1. --cutout_nholes 1 --cutout_fill_value 1. --cutout_ratio 0.3 --output-width 608
```
#### 2.4.2. Inference
Download the trained model from [**_here_**](https://drive.google.com/drive/folders/1RHD9PBvk-9SjbKwoi_Q1kl9-UGFo2Pth?usp=sharing),
then put it to `${ROOT}/checkpoints/` and execute:
```shell script
python test.py --gpu_idx 0 --pretrained_path ../checkpoints/complex_yolov4/complex_yolov4_mse_loss.pth --cfgfile ./config/cfg/complex_yolov4.cfg --show_image
```
#### 2.4.3. Evaluation
```shell script
python evaluate.py --gpu_idx 0 --pretrained_path <PATH> --cfgfile <CFG> --img_size <SIZE> --conf-thresh <THRESH> --nms-thresh <THRESH> --iou-thresh <THRESH>
```
(The `conf-thresh`, `nms-thresh`, and `iou-thresh` params can be adjusted. By default, these params have been set to _**0.5**_)
#### 2.4.4. Training
##### 2.4.4.1. Single machine, single gpu
```shell script
python train.py --gpu_idx 0 --batch_size <N> --num_workers <N>...
```
##### 2.4.4.2. Multi-processing Distributed Data Parallel Training
We should always use the `nccl` backend for multi-processing distributed training since it currently provides the best
distributed training performance.
- **Single machine (node), multiple GPUs**
```shell script
python train.py --dist-url 'tcp://127.0.0.1:29500' --dist-backend 'nccl' --multiprocessing-distributed --world-size 1 --rank 0
```
- **Two machines (two nodes), multiple GPUs**
_**First machine**_
```shell script
python train.py --dist-url 'tcp://IP_OF_NODE1:FREEPORT' --dist-backend 'nccl' --multiprocessing-distributed --world-size 2 --rank 0
```
_**Second machine**_
```shell script
python train.py --dist-url 'tcp://IP_OF_NODE2:FREEPORT' --dist-backend 'nccl' --multiprocessing-distributed --world-size 2 --rank 1
```
To reproduce the results, you can run the bash shell script
```bash
./train.sh
```
#### Tensorboard
- To track the training progress, go to the `logs/` folder and
```shell script
cd logs/<saved_fn>/tensorboard/
tensorboard --logdir=./
```
- Then go to [http://localhost:6006/](http://localhost:6006/):
### 2.5. List of usage for Bag of Freebies (BoF) & Bag of Specials (BoS) in this implementation
| |Backbone | Detector |
|---|---|---|
|**BoF** |[x] Dropblock <br> [x] Random rescale, rotation (global) <br> [x] Mosaic/Cutout augmentation|[x] Cross mini-Batch Normalization <br>[x] Dropblock <br> [x] Random training shapes <br> |
|**BoS** |[x] Mish activation <br> [x] Cross-stage partial connections (CSP) <br> [x] Multi-input weighted residual connections (MiWRC) |[x] Mish activation <br> [x] SPP-block <br> [x] SAM-block <br> [x] PAN path-aggregation block <br> [x] GIoU loss <br> [ ] CIoU loss |
## Contact
If you think this work is useful, please give me a star! <br>
If you find any errors or have any suggestions, please contact me (**Email:** `nguyenmaudung93.kstn@gmail.com`). <br>
Thank you!
## Citation
```bash
@article{Complex-YOLO,
author = {Martin Simon, Stefan Milz, Karl Amende, Horst-Michael Gross},
title = {Complex-YOLO: Real-time 3D Object Detection on Point Clouds},
year = {2018},
journal = {arXiv},
}
@article{YOLOv4,
author = {Alexey Bochkovskiy, Chien-Yao Wang, Hong-Yuan Mark Liao},
title = {YOLOv4: Optimal Speed and Accuracy of Object Detection},
year = {2020},
journal = {arXiv},
}
```
## Folder structure
```
${ROOT}
└── checkpoints/
├── complex_yolov3/
└── complex_yolov4/
└── dataset/
└── kitti/
├──ImageSets/
│ ├── train.txt
│ └── val.txt
├── training/
│ ├── image_2/ <-- for visualization
│ ├── calib/
│ ├── label_2/
│ └── velodyne/
└── testing/
│ ├── image_2/ <-- for visualization
│ ├── calib/
│ └── velodyne/
└── classes_names.txt
└── src/
├── config/
├── cfg/
│ ├── complex_yolov3.cfg
│ ├── complex_yolov3_tiny.cfg
│ ├�
没有合适的资源?快使用搜索试试~ 我知道了~
Complex-YOLOv4-Pytorch:本文基于YOLOv4的PyTorch实现
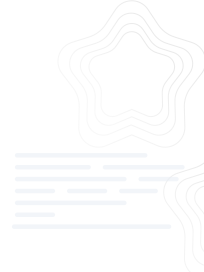
共44个文件
py:28个
txt:5个
cfg:4个


温馨提示
复杂的YOLOv4 本文基于YOLOv4的PyTorch实现: 特征 基于YOLOv4的实时3D对象检测 支持 张量板 镶嵌/切口增强训练 使用旋转框的损失进行优化。 更新2020.08.26 : 更快的训练,更快的推理 无锚的方法 无需非最大抑制 示范(在GTX 1080Ti上) 2.入门 2.1。要求 pip install -U -r requirements.txt 有关和库的信息,请参阅其官方网站上的安装说明。 2.2。资料准备 从下载3D KITTI检测数据集。 下载的数据包括: Velodyne点云(29 GB) :将数据输入到Complex-YOLO模型 对象数据集的训练标签(5 MB) :Complex-YOLO模型的输入标签 对象数据集的摄像机校准矩阵(16 MB) :用于可视化预测 对象数据集的左侧彩色图像(12 GB) :用于可视化预测 请确保您按照以下方式
资源详情
资源评论
资源推荐
收起资源包目录


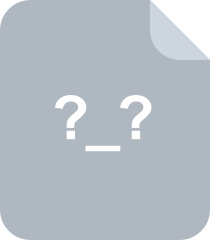
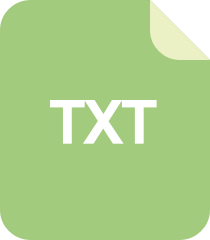


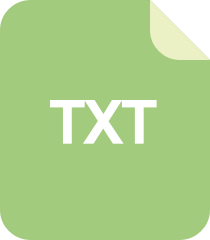

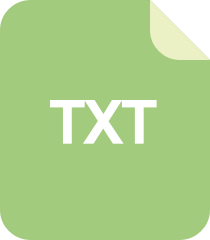
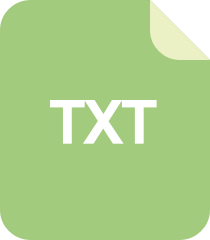
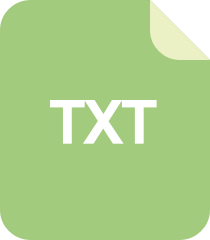

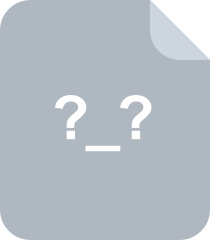
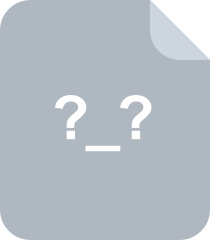

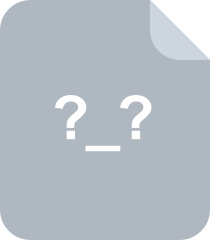
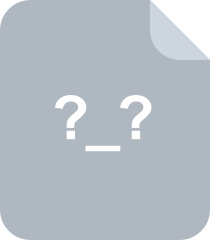
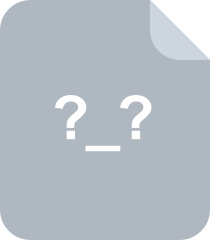
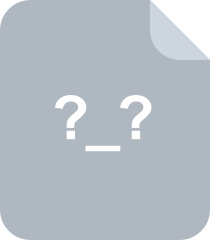
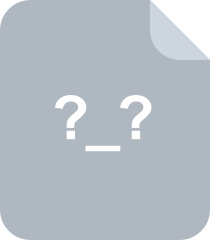

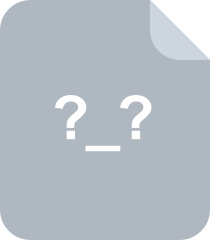
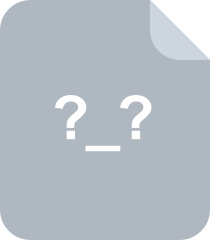
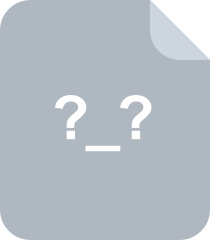
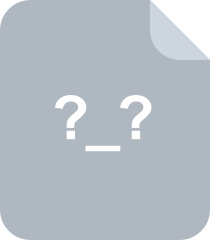
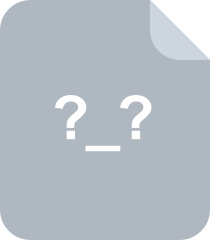
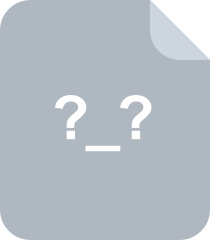
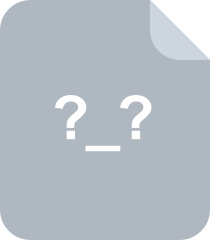
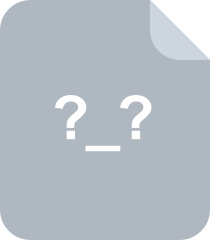
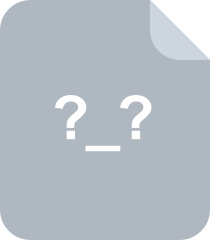
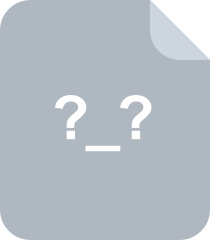

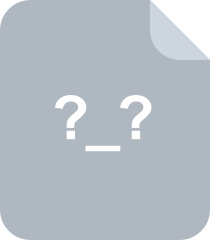

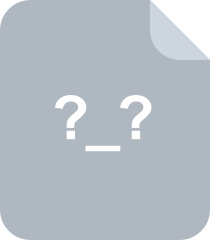
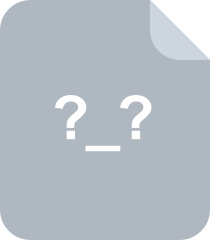
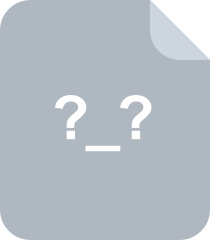
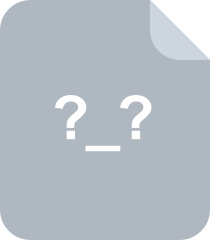
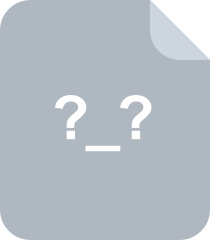
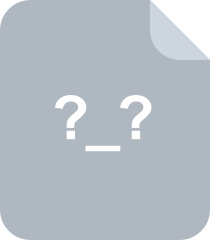
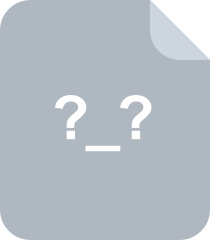

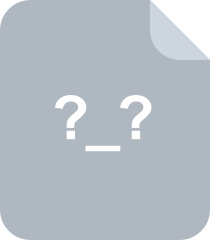
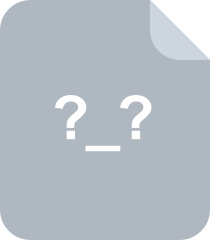
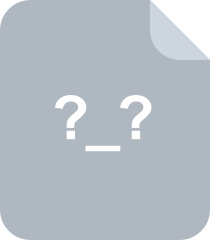
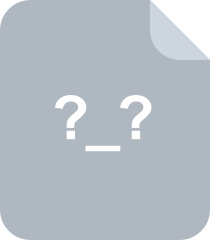
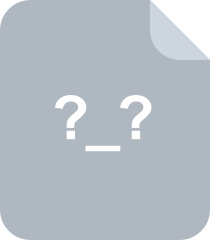
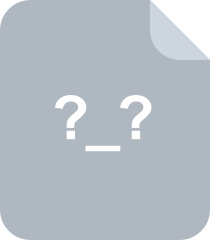
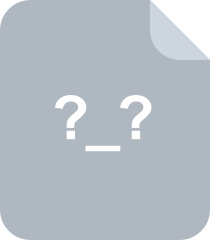
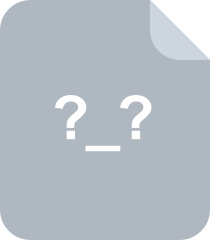
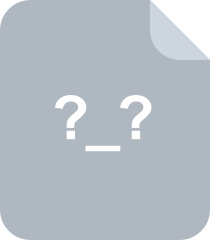
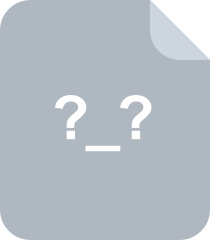
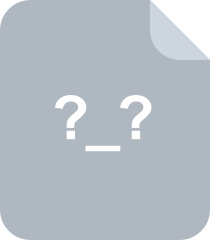

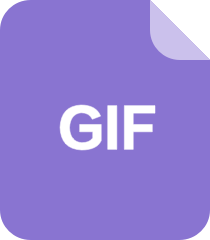
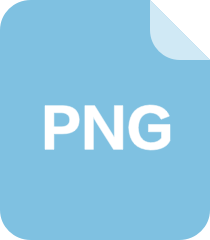
共 44 条
- 1
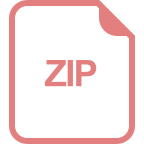
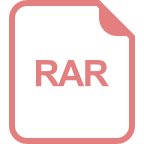
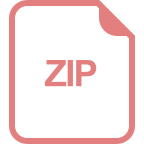
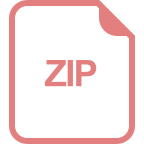
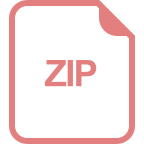
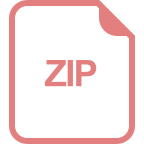
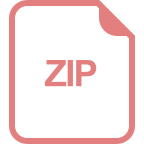
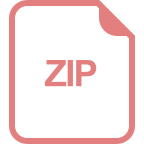
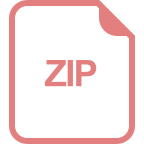
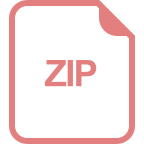
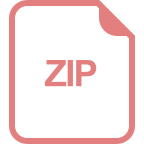
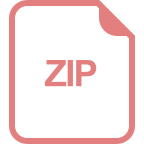
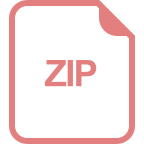
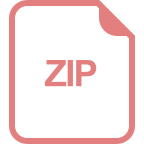
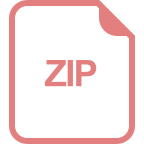
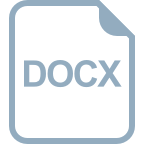
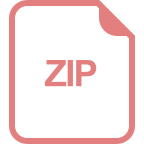
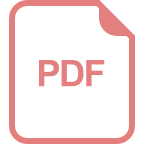
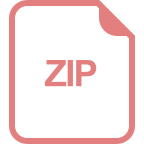
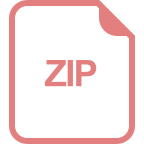
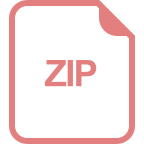
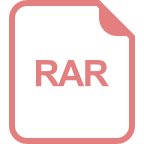
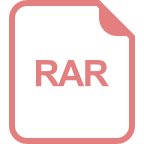
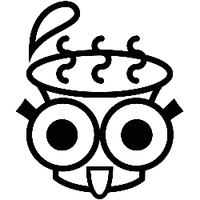
ywnwx
- 粉丝: 33
- 资源: 4624
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

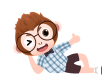
最新资源
- TH2024003基于ssm143校园一卡通系统软件的设计与实现+jsp.zip
- nuget 库官方下载包,可使用解压文件打开解压使用
- 谷歌股票数据集,google股票数据集,Alphabet股份数据集(2004-2024)
- 富芮坤FR8003作为主机连接FR8003抓包文件20241223-135206.pcapng
- 台球检测11-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- uniapp-小程序-vue
- 计算机接口实验报告.zip
- 特斯拉股票数据集,特斯拉历史股票价格数据
- 极验w参数加密JS算法
- 这是一个好玩的整人代码:)
- QT实现QGraphicsView绘图实现边框动画,实现点在QPainterPath路径上移动动画效果的示例项目源码
- VueWarn解决办法.md
- 台球检测38-YOLO(v5至v11)、COCO、CreateML、TFRecord、VOC数据集合集.rar
- NSFileHandleOperationException如何解决.md
- 按键显示系统考试3.3试题
- GeneratorExit.md
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


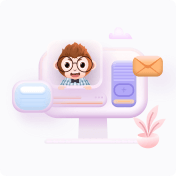
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1