/*
* =====================================================================================
*
* Filename: BuddyView.cpp
*
* Description: 显示好友列表的控件
*
* Version: 1.0
* Created: 2007年06月28日 19时26分39秒 CST
* Revision: none
* Compiler: gcc
*
* Author: wind (xihe), xihels@gmail.com
* Company: cyclone
*
* 纪念5.12汶川地震中消逝的那些生命
* =====================================================================================
*/
#include <glib/gi18n.h>
#include <fstream>
#include <unistd.h>
#include "BuddyView.h"
#include "BuddyList.h"
#include "Bodies.h"
#include "Unit.h"
#include "pixmaps.h"
#include "sounds.h"
#include "Unit.h"
#include "TreeViewTooltips.h"
#include "RoomItem.h"
#include "Buddy.h"
BuddyView::BuddyView(MainWindow & f_parent):
m_parent(f_parent)
, SHOWALL(false)
, EXPAND(false)
, m_filterText("")
{
set_headers_visible(false);
set_border_width(5);
set_name("icalk_blist_treeview");
add_events(Gdk::POINTER_MOTION_MASK | Gdk::BUTTON_MOTION_MASK |
Gdk::BUTTON_PRESS_MASK | Gdk::
BUTTON_RELEASE_MASK | Gdk::ENTER_NOTIFY_MASK | Gdk::
LEAVE_NOTIFY_MASK);
m_tooltips = new TreeViewTooltips(this);
m_treestore = TreeModelDnd::create(buddyColumns);
//set_model(m_treestore);
m_treemodelfilter = Gtk::TreeModelFilter::create(m_treestore);
m_treemodelfilter->set_visible_func(sigc::mem_fun(*this, &BuddyView::
list_visible_func));
set_model(m_treemodelfilter);
append_column("ICON", buddyColumns.icon);
//set_show_expanders(false); //gtkmm 2.12
/*
Gtk::TreeView::Column * col =
Gtk::manage(new Gtk::TreeView::Column("iCalk"));
col->pack_start(buddyColumns.nickname, false); //false = don't expand
vector < Gtk::CellRenderer * >rends = col->get_cell_renderers();
col->clear_attributes(*rends[0]);
col->add_attribute(*rends[0], "markup", 1);
*/
Gtk::TreeView::Column * col =
Gtk::manage(new Gtk::TreeView::Column("iCalk"));
col->pack_start(m_rendtext);
//Tell the view column how to render the model values:
//将ViewColumn控件和Model values关联起来
col->set_cell_data_func(m_rendtext,
sigc::mem_fun(*this,
&BuddyView::
tvc_connect_cell_data));
//make the cellrenderer ellipsize
#ifdef GLIBMM_PROPERTIES_ENABLED
//m_rendtext.property_editable()=true;
m_rendtext.property_ellipsize() = Pango::ELLIPSIZE_END;
#else
m_rendtext.set_property("editable", ture);
#endif
m_rendtext.signal_editing_started().
connect(sigc::
mem_fun(*this,
&BuddyView::cellrender_on_editing_start));
m_rendtext.signal_edited().
connect(sigc::
mem_fun(*this, &BuddyView::cellrender_on_edited));
col->add_attribute(m_rendtext.property_markup(),
buddyColumns.nickname);
col->set_resizable(true);
col->set_expand();
this->append_column(*col);
this->append_column("Voip", buddyColumns.audioicon);
m_treestore->
set_default_sort_func(sigc::
mem_fun(*this,
&BuddyView::on_sort_compare));
m_treestore->
set_sort_column_id(Gtk::TreeSortable::DEFAULT_SORT_COLUMN_ID,
Gtk::SORT_ASCENDING);
//设置可托拽
this->enable_model_drag_source();
this->enable_model_drag_dest();
this->set_tooltip_window( *m_tooltips);
this->set_has_tooltip();
this->signal_query_tooltip().connect(sigc::mem_fun(*this,
&BuddyView::on_tooltip_show));
/*
this->signal_motion_notify_event().
connect(sigc::mem_fun(*this, &BuddyView::on_motion_event),
false);
this->signal_leave_notify_event().
connect(sigc::mem_fun(*this, &BuddyView::on_leave_event),
false);
*/
/*
this->signal_enter_notify_event().connect(sigc::mem_fun(
*this,&BuddyView::on_enter_event));
*/
show_all_children();
}
BuddyView::~BuddyView()
{
delete m_tooltips;
}
int BuddyView::on_sort_compare(const Gtk::TreeModel::iterator & a,
const Gtk::TreeModel::iterator & b)
{
int result;
if ((result =
(*a)[buddyColumns.status] - (*b)[buddyColumns.status]) == 0) {
Glib::ustring an = (*a)[buddyColumns.nickname];
Glib::ustring bn = (*b)[buddyColumns.nickname];
result = an.lowercase().compare(bn.lowercase());
}
return result;
}
bool BuddyView::on_motion_event(GdkEventMotion * ev)
{
Gtk::TreeModel::Path path;
Gtk::TreeViewColumn * column;
int cell_x, cell_y;
if (m_tipTimeout.connected()) {
m_tipTimeout.disconnect();
m_tooltips->hideTooltip();
}
if (this->
get_path_at_pos((int) ev->x, (int) ev->y, path, column, cell_x,
cell_y)) {
Gtk::TreeModel::iterator iter =
this->get_model()->get_iter(path);
int type = (*iter)[buddyColumns.status];
int delay = 600;
if (STATUS_GROUP != type)
m_tipTimeout =
Glib::signal_timeout().connect(sigc::bind <
GdkEventMotion *
> (sigc::
mem_fun(*this,
&BuddyView::
tooltip_timeout),
ev), delay);
else
m_tooltips->hideTooltip();
} else
m_tooltips->hideTooltip();
return true;
}
bool BuddyView::on_tooltip_show(int x,int y, bool key_mode,const Glib::RefPtr<Gtk::Tooltip>& tooltip)
{
Gtk::TreeModel::Path path;
Gtk::TreeViewColumn * column;
int cell_x, cell_y;
if (this->get_path_at_pos(x, y, path, column, cell_x,cell_y)){
Gtk::TreeModel::iterator iter =
this->get_model()->get_iter(path);
if (!iter)
return false;
int type = (*iter)[buddyColumns.status];
Glib::ustring jid = (*iter)[buddyColumns.id];
Glib::ustring text_, status_, status_sub, msg_;
if (STATUS_ROOM != type) {
Buddy *buddy =
Bodies::Get_Bodies().get_buddy_list().
find_buddy(jid);
if (NULL == buddy)
return false;
SubscriptionType substrEnum =
buddy->getSubscription();
status_sub = "<span weight='bold'>";
if (S10nBoth == substrEnum)
status_sub =
status_sub +
_("subscribed: </span>Both \n");
else
status_sub =
status_sub
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
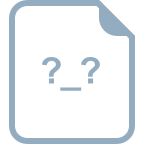
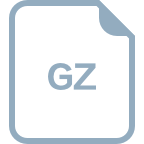
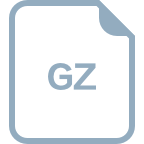
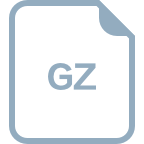
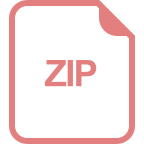
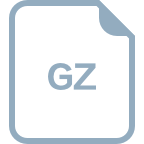
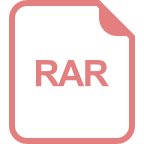
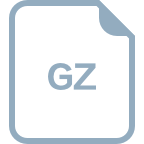
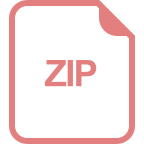
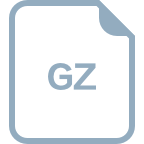
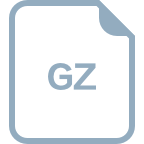
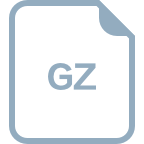
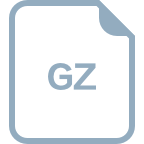
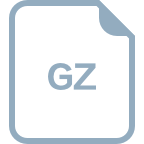
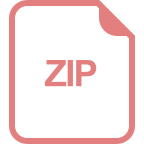
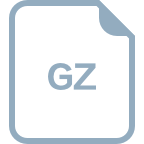
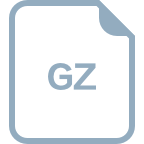
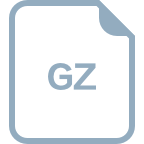
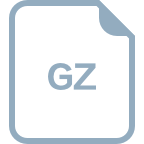
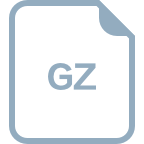
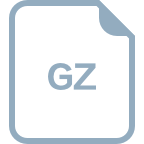
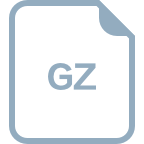
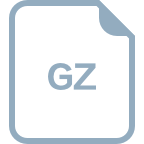
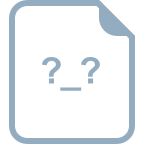
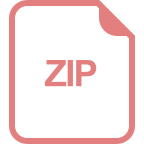
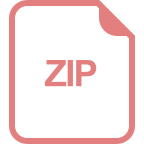
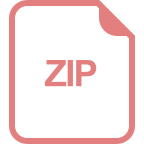
收起资源包目录

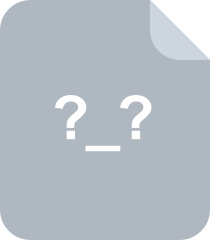
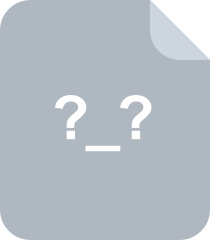
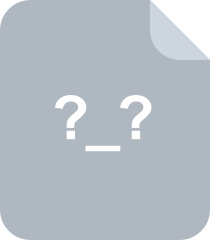
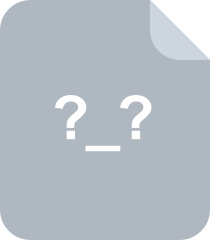
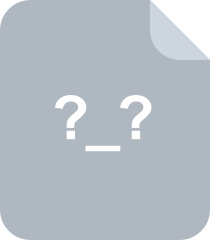
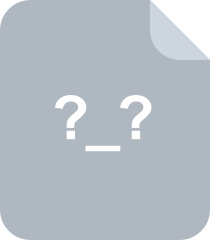
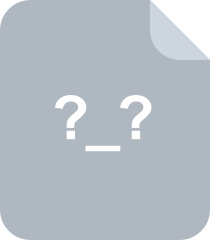
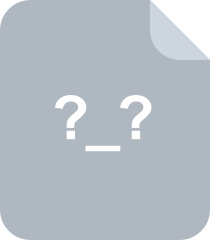
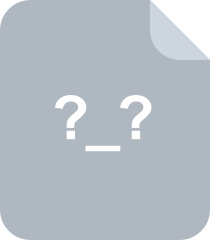
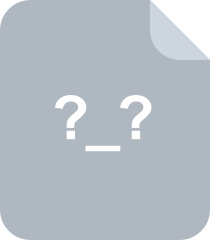
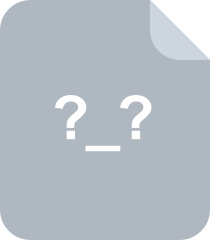
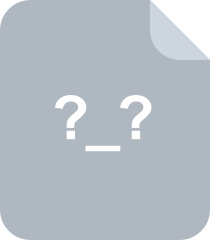
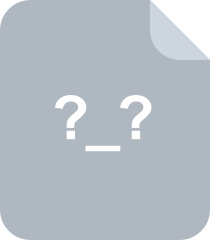
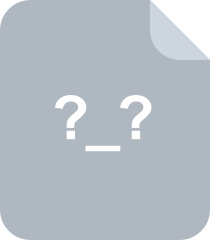
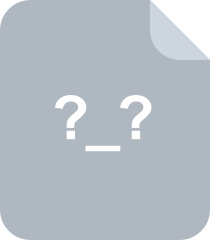
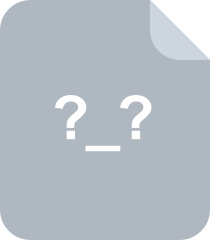
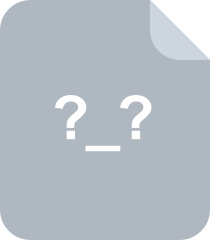
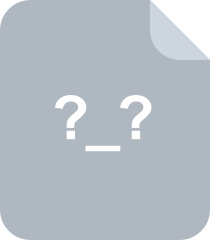
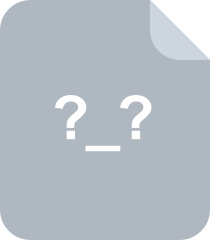
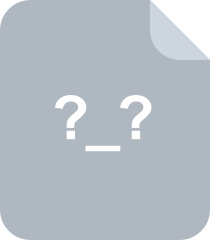
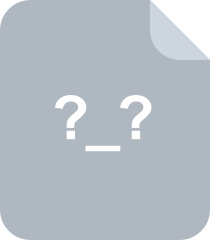
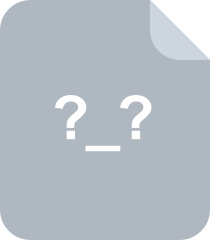
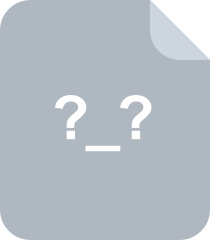
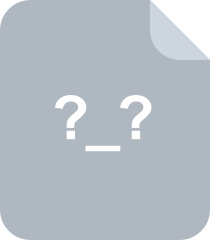
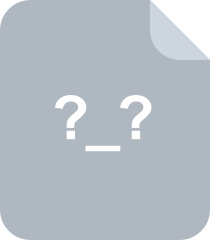
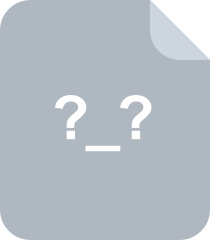
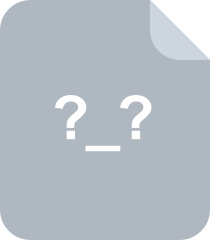
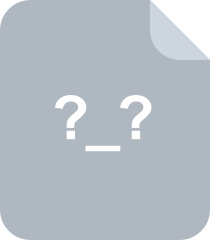
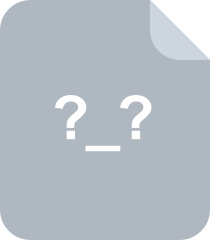
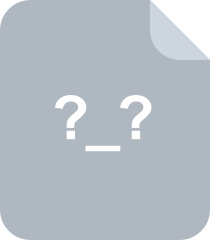
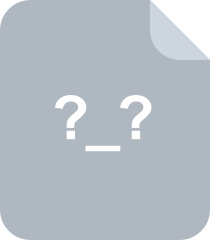
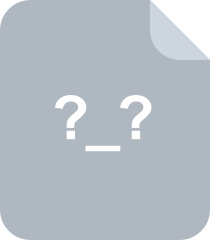
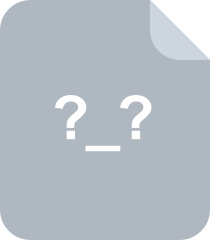
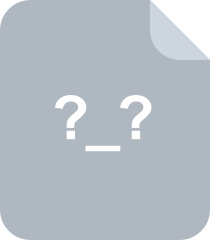
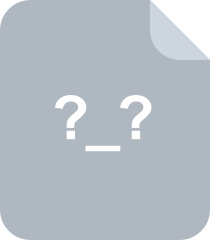
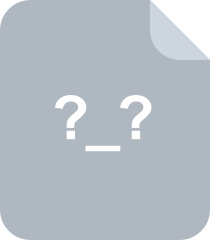
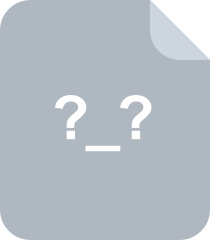
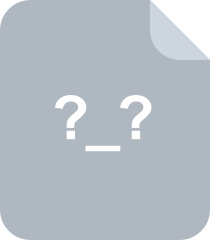
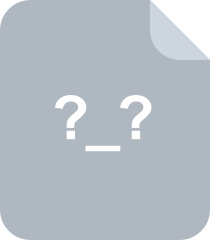
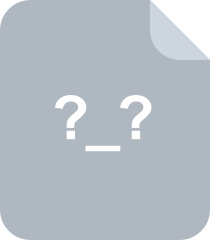
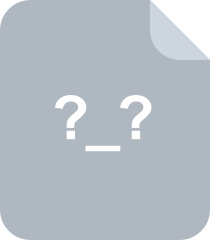
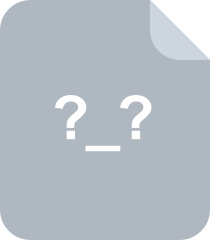
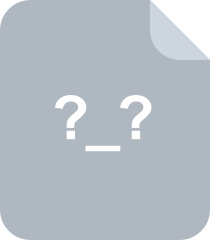
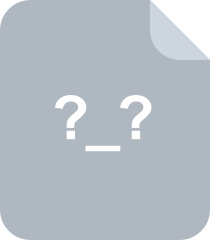
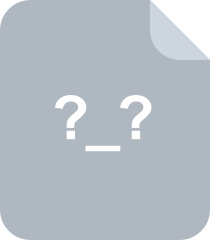
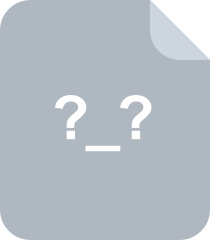
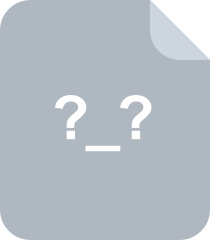
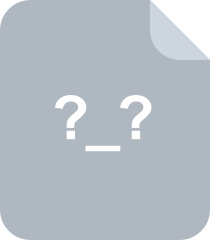
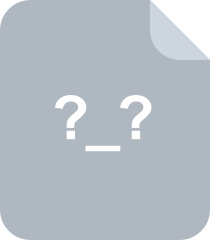
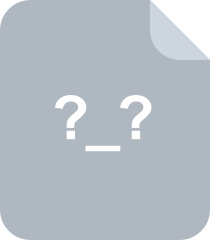
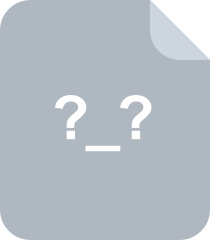
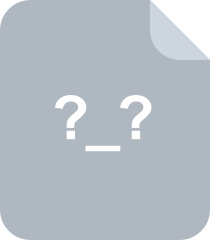
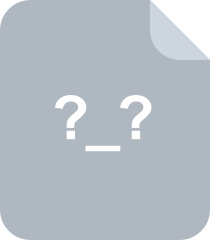
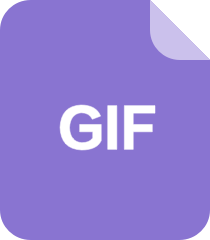
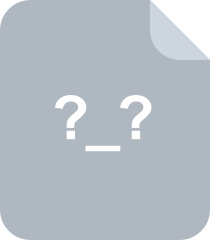
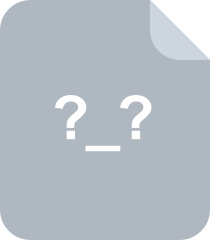
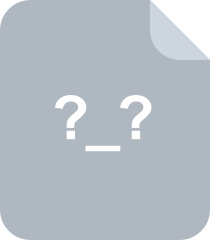
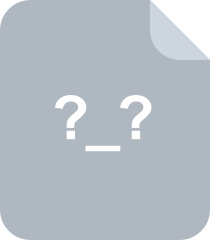
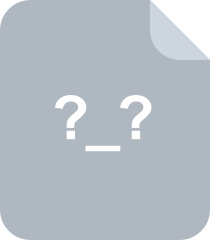
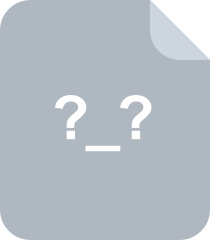
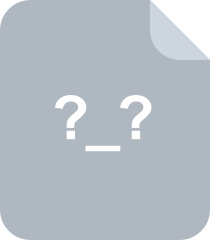
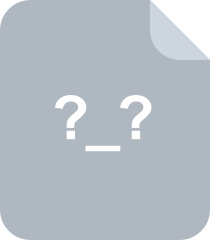
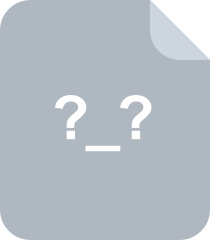
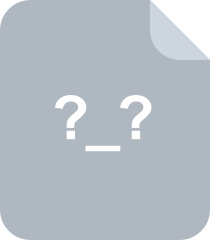
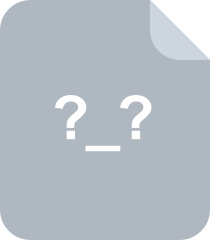
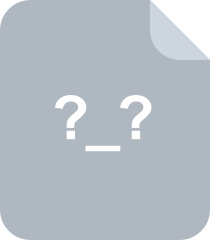
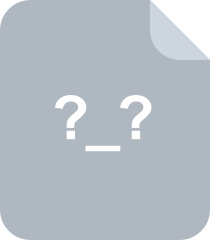
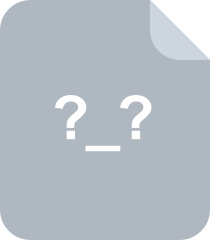
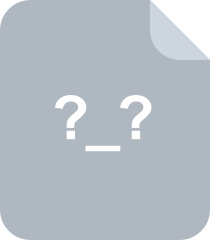
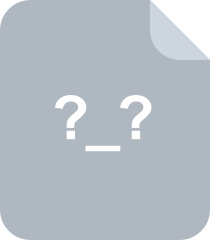
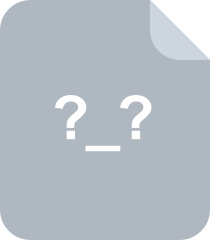
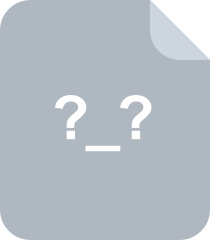
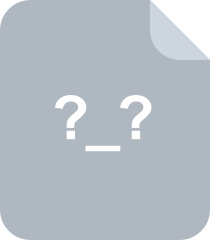
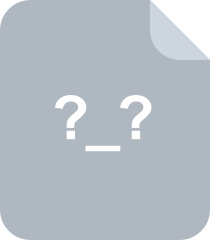
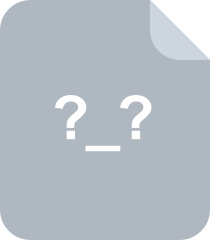
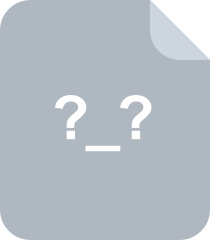
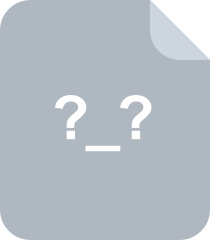
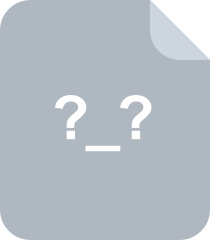
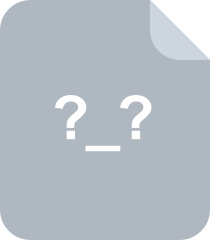
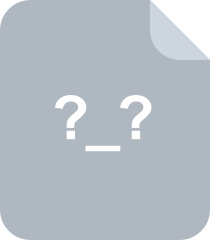
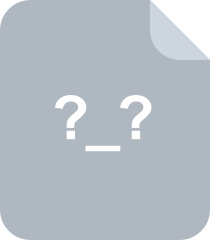
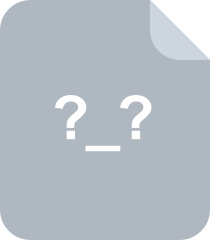
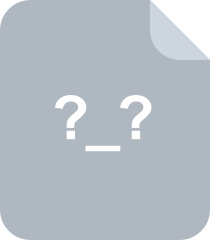
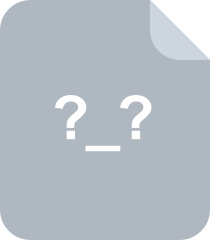
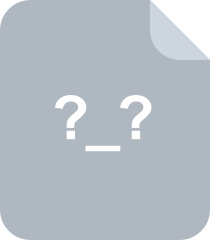
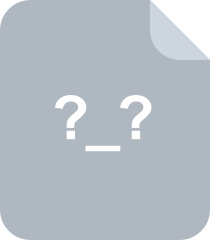
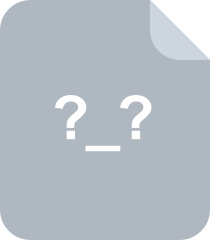
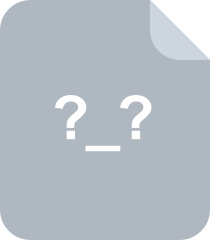
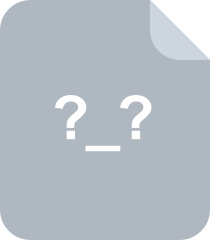
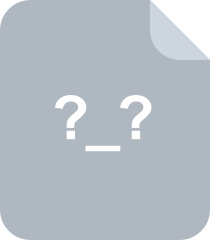
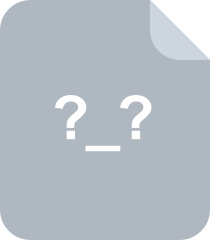
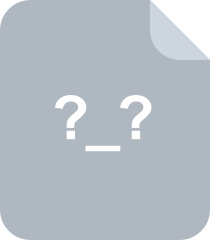
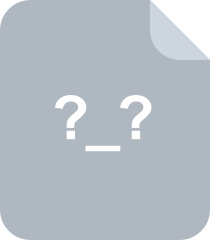
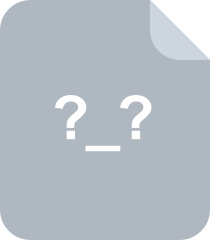
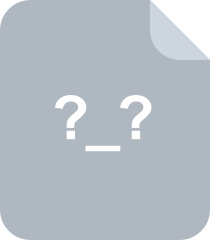
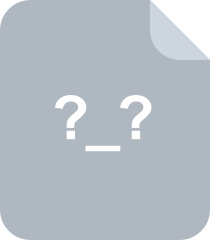
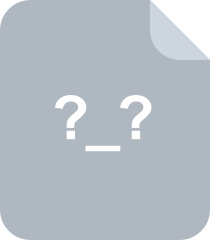
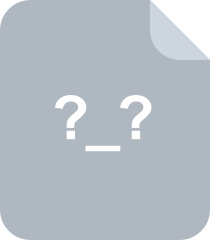
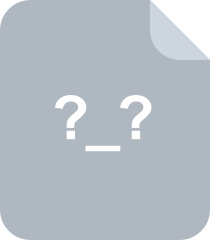
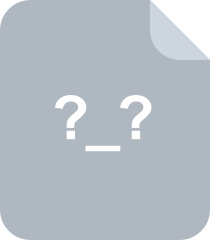
共 319 条
- 1
- 2
- 3
- 4
资源评论
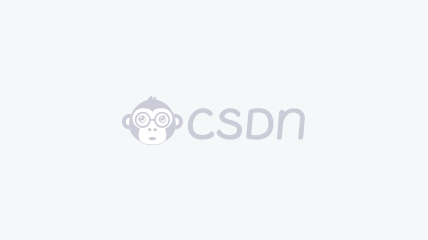

处处清欢
- 粉丝: 2082
- 资源: 2863
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

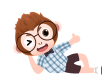
最新资源
- (174808034)webgis课程设计文件
- (177121232)windows电脑下载OpenHarmony鸿蒙命令行工具hdc-std
- (177269606)使用Taro开发鸿蒙原生应用.zip
- (170644008)Eclipse+MySql+JavaSwing选课成绩管理系统
- (14173842)条形码例子
- (176419244)订餐系统-小程序.zip
- Java Web实现电子购物系统
- (30485858)SSM(Spring+springmvc+mybatis)项目实例.zip
- (172760630)数据结构课程设计文档1
- 基于simulink的悬架仿真模型,有主动悬架被动悬架天棚控制半主动悬架 1基于pid控制的四自由度主被动悬架仿真模型 2基于模糊控制的二自由度仿真模型,对比pid控制对比被动控制,的比较说明
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


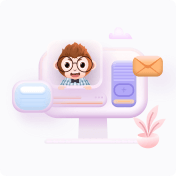
安全验证
文档复制为VIP权益,开通VIP直接复制
