// MyFireworkView.cpp : implementation of the CMyFireworkView class
//
#include "stdafx.h"
#include "MyFirework.h"
#include "MyFireworkDoc.h"
#include "MyFireworkView.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CMyFireworkView
IMPLEMENT_DYNCREATE(CMyFireworkView, CView)
BEGIN_MESSAGE_MAP(CMyFireworkView, CView)
//{{AFX_MSG_MAP(CMyFireworkView)
ON_WM_CREATE()
ON_WM_DESTROY()
ON_WM_SIZE()
ON_WM_TIMER()
//}}AFX_MSG_MAP
// Standard printing commands
ON_COMMAND(ID_FILE_PRINT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW, CView::OnFilePrintPreview)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CMyFireworkView construction/destruction
CMyFireworkView::CMyFireworkView()
{
// TODO: add construction code here
}
CMyFireworkView::~CMyFireworkView()
{
}
BOOL CMyFireworkView::PreCreateWindow(CREATESTRUCT& cs)
{
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
////////////////////////////////////////////////////////////////
//设置窗口类型
cs.style |=WS_CLIPCHILDREN | WS_CLIPSIBLINGS;
////////////////////////////////////////////////////////////////
return CView::PreCreateWindow(cs);
}
/////////////////////////////////////////////////////////////////////////////
// CMyFireworkView drawing
void CMyFireworkView::OnDraw(CDC* pDC)
{
CMyFireworkDoc* pDoc = GetDocument();
ASSERT_VALID(pDoc);
// TODO: add draw code for native data here
//////////////////////////////////////////////////////////////////
RenderScene(); //渲染场景
//////////////////////////////////////////////////////////////////
}
/////////////////////////////////////////////////////////////////////////////
// CMyFireworkView printing
BOOL CMyFireworkView::OnPreparePrinting(CPrintInfo* pInfo)
{
// default preparation
return DoPreparePrinting(pInfo);
}
void CMyFireworkView::OnBeginPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add extra initialization before printing
}
void CMyFireworkView::OnEndPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add cleanup after printing
}
/////////////////////////////////////////////////////////////////////////////
// CMyFireworkView diagnostics
#ifdef _DEBUG
void CMyFireworkView::AssertValid() const
{
CView::AssertValid();
}
void CMyFireworkView::Dump(CDumpContext& dc) const
{
CView::Dump(dc);
}
CMyFireworkDoc* CMyFireworkView::GetDocument() // non-debug version is inline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CMyFireworkDoc)));
return (CMyFireworkDoc*)m_pDocument;
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CMyFireworkView message handlers
int CMyFireworkView::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CView::OnCreate(lpCreateStruct) == -1)
return -1;
// TODO: Add your specialized creation code here
//////////////////////////////////////////////////////////////////
//初始化OpenGL和设置定时器
m_pDC = new CClientDC(this);
SetTimer(1, 20, NULL);
InitializeOpenGL(m_pDC);
//////////////////////////////////////////////////////////////////
Init();
InitializeTexture();
return 0;
}
void CMyFireworkView::OnDestroy()
{
CView::OnDestroy();
// TODO: Add your message handler code here
/////////////////////////////////////////////////////////////////
//删除调色板和渲染上下文、定时器
::wglMakeCurrent(0,0);
::wglDeleteContext( m_hRC);
if (m_hPalette)
DeleteObject(m_hPalette);
if ( m_pDC )
{
delete m_pDC;
}
KillTimer(1);
/////////////////////////////////////////////////////////////////
}
void CMyFireworkView::OnSize(UINT nType, int cx, int cy)
{
CView::OnSize(nType, cx, cy);
// TODO: Add your message handler code here
/////////////////////////////////////////////////////////////////
//添加窗口缩放时的图形变换函数
glViewport(0,0,cx,cy);
/////////////////////////////////////////////////////////////////
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(-1.33, 1.33, 0, 2);
glMatrixMode(GL_MODELVIEW);
WindW = cx;
WindH = cy;
}
void CMyFireworkView::OnTimer(UINT nIDEvent)
{
// TODO: Add your message handler code here and/or call default
/////////////////////////////////////////////////////////////////
//添加定时器响应函数和场景更新函数
Invalidate(FALSE);
/////////////////////////////////////////////////////////////////
CView::OnTimer(nIDEvent);
}
/////////////////////////////////////////////////////////////////////
// 设置逻辑调色板
//////////////////////////////////////////////////////////////////////
void CMyFireworkView::SetLogicalPalette(void)
{
struct
{
WORD Version;
WORD NumberOfEntries;
PALETTEENTRY aEntries[256];
} logicalPalette = { 0x300, 256 };
BYTE reds[] = {0, 36, 72, 109, 145, 182, 218, 255};
BYTE greens[] = {0, 36, 72, 109, 145, 182, 218, 255};
BYTE blues[] = {0, 85, 170, 255};
for (int colorNum=0; colorNum<256; ++colorNum)
{
logicalPalette.aEntries[colorNum].peRed =
reds[colorNum & 0x07];
logicalPalette.aEntries[colorNum].peGreen =
greens[(colorNum >> 0x03) & 0x07];
logicalPalette.aEntries[colorNum].peBlue =
blues[(colorNum >> 0x06) & 0x03];
logicalPalette.aEntries[colorNum].peFlags = 0;
}
m_hPalette = CreatePalette ((LOGPALETTE*)&logicalPalette);
}
//////////////////////////////////////////////////////////
// 初始化openGL场景
//////////////////////////////////////////////////////////
BOOL CMyFireworkView::InitializeOpenGL(CDC* pDC)
{
m_pDC = pDC;
SetupPixelFormat();
//生成绘制描述表
m_hRC = ::wglCreateContext(m_pDC->GetSafeHdc());
//置当前绘制描述表
::wglMakeCurrent(m_pDC->GetSafeHdc(), m_hRC);
return TRUE;
}
//////////////////////////////////////////////////////////
// 设置像素格式
//////////////////////////////////////////////////////////
BOOL CMyFireworkView::SetupPixelFormat()
{
PIXELFORMATDESCRIPTOR pfd = {
sizeof(PIXELFORMATDESCRIPTOR), // pfd结构的大小
1, // 版本号
PFD_DRAW_TO_WINDOW | // 支持在窗口中绘图
PFD_SUPPORT_OPENGL | // 支持 OpenGL
PFD_DOUBLEBUFFER, // 双缓存模式
PFD_TYPE_RGBA, // RGBA 颜色模式
24, // 24 位颜色深度
0, 0, 0, 0, 0, 0, // 忽略颜色位
0, // 没有非透明度缓存
0, // 忽略移位位
0, // 无累加缓存
0, 0, 0, 0, // 忽略累加位
32, // 32 位深度缓存
0, // 无模板缓存
0, // 无辅助缓存
PFD_MAIN_PLANE, // 主层
0, // 保留
0, 0, 0 // 忽略层,可见性和损毁掩模
};
int pixelformat;
pixelformat = ::ChoosePixelFormat(m_pDC->GetSafeHdc(), &pfd);//选择像素格式
::SetPixelFormat(m_pDC->GetSafeHdc(), pixelformat, &pfd); //设置像素格式
if(pfd.dwFlags & PFD_NEED_PALETTE)
SetLogicalPalette(); //设置逻辑调色板
return TRUE;
}
//////////////////////////////////////////////////////////
// 场景绘制与渲染
//////////////////////////////////////////////////////////
BOOL CMyFireworkView::RenderScene()
{
float s;
int i, flag_temp;
struct firework *fw_temp, *fw_temp2;
AddFirework(); // 添加烟火
glClear(GL_COLOR_BUFFER_BIT);
// 绘制烟火
g
没有合适的资源?快使用搜索试试~ 我知道了~
OpenGL的绘制烟花程序,非常漂亮,可以绘制不同形状的烟花.zip
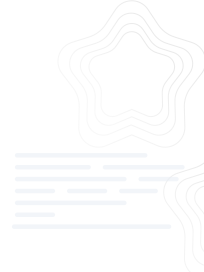
共26个文件
h:7个
cpp:6个
ico:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 180 浏览量
2023-01-30
11:49:09
上传
评论
收藏 56KB ZIP 举报
温馨提示
OpenGL的绘制烟花程序,非常漂亮,可以绘制不同形状的烟花.zip
资源推荐
资源详情
资源评论
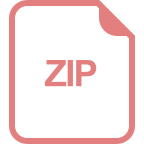
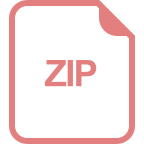
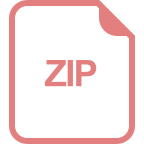
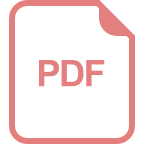
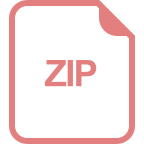
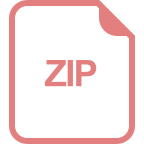
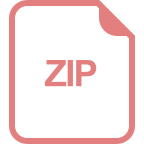
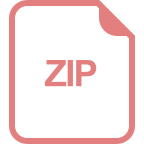
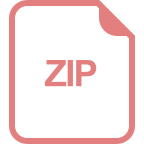
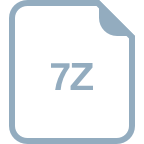
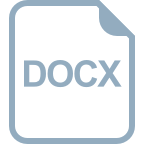
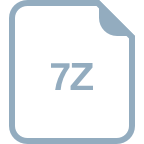
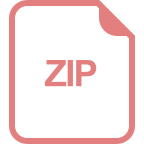
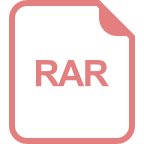
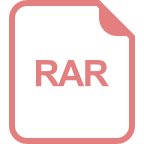
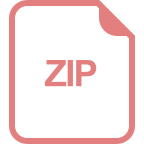
收起资源包目录



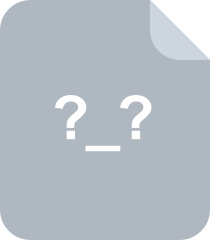
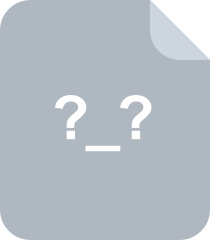
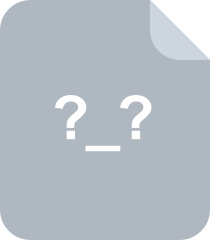
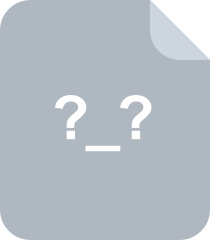
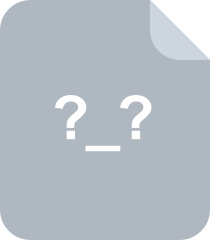
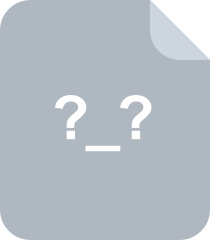
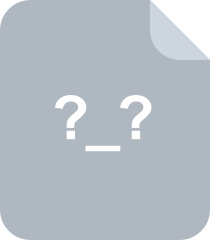
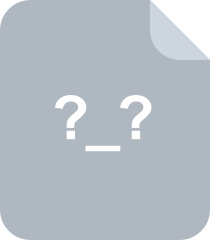
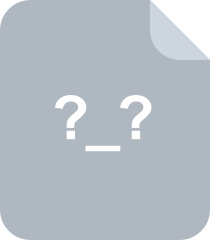
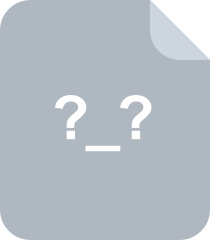
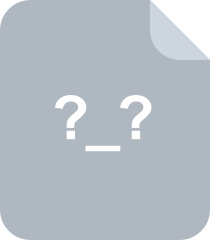
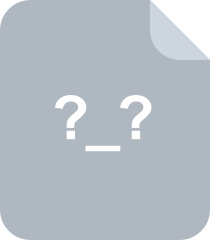
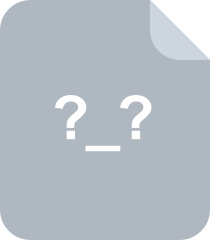
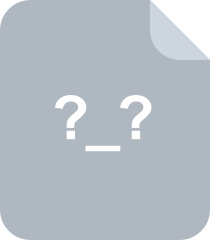

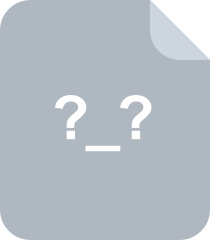
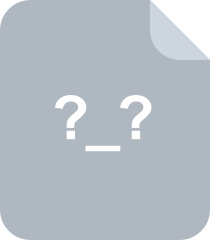
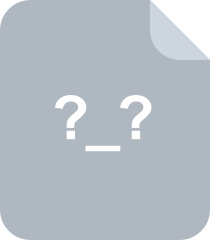
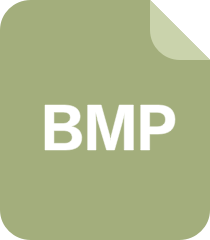
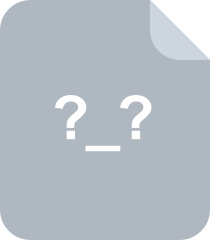
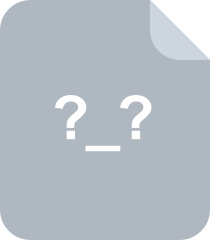
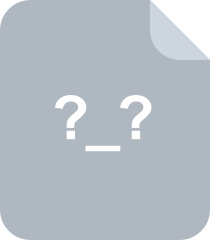
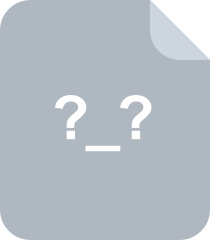
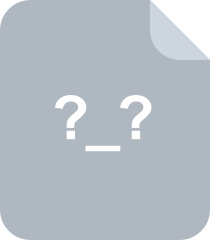
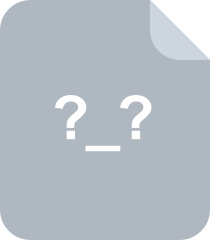
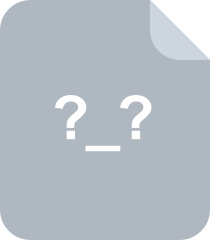
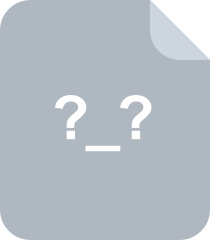

共 26 条
- 1
资源评论
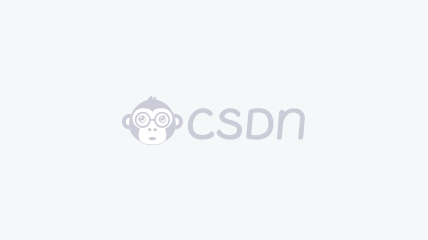

处处清欢
- 粉丝: 158
- 资源: 2511

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

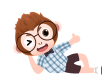
安全验证
文档复制为VIP权益,开通VIP直接复制
