COP1000_graph:如何在JavaScript中构建有效图的示例
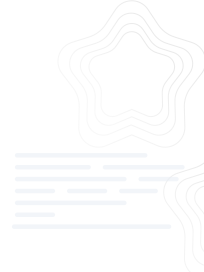

在JavaScript中构建有效的图结构是数据结构和算法学习中的一个重要环节。图是由节点(或顶点)和边组成的集合,可以用来表示各种复杂的关系。在这个示例中,我们将深入探讨如何在JavaScript中创建和操作图。 让我们定义图的基本结构。在JavaScript中,我们可以使用对象来表示节点,并通过数组或对象字面量来存储边。一个简单的图类可能如下所示: ```javascript class Graph { constructor() { this.vertices = []; // 存储所有节点 this.adjList = {}; // 邻接表,用于存储每个节点的相邻节点 } addVertex(vertex) { if (!this.vertices.includes(vertex)) { this.vertices.push(vertex); this.adjList[vertex] = []; // 初始化邻接表,表示该节点没有相邻节点 } } addEdge(vertex1, vertex2) { if (this.vertices.includes(vertex1) && this.vertices.includes(vertex2)) { this.adjList[vertex1].push(vertex2); this.adjList[vertex2].push(vertex1); // 图可能是无向的,所以两边都需要添加 } else { console.log("One or both vertices not found in the graph."); } } removeVertex(vertex) { const index = this.vertices.indexOf(vertex); if (index !== -1) { this.vertices.splice(index, 1); delete this.adjList[vertex]; for (let v of this.vertices) { this.adjList[v] = this.adjList[v].filter(vn => vn !== vertex); } } else { console.log("Vertex not found in the graph."); } } removeEdge(vertex1, vertex2) { if (this.vertices.includes(vertex1) && this.vertices.includes(vertex2)) { this.adjList[vertex1] = this.adjList[vertex1].filter(vn => vn !== vertex2); this.adjList[vertex2] = this.adjList[vertex2].filter(vn => vn !== vertex1); } else { console.log("One or both vertices not found in the graph."); } } traverseDFS(vertex, visited = {}) { visited[vertex] = true; console.log(vertex); for (let neighbor of this.adjList[vertex]) { if (!visited[neighbor]) { this.traverseDFS(neighbor, visited); } } } traverseBFS(vertex, visited = {}) { const queue = []; queue.push(vertex); visited[vertex] = true; while (queue.length > 0) { const current = queue.shift(); console.log(current); for (let neighbor of this.adjList[current]) { if (!visited[neighbor]) { visited[neighbor] = true; queue.push(neighbor); } } } } } ``` 以上代码定义了一个`Graph`类,包含了添加、移除节点和边的方法,以及深度优先遍历(DFS)和广度优先遍历(BFS)的功能。在实际应用中,根据具体需求,你可能还需要添加其他方法,如查找最短路径(Dijkstra算法)、判断图是否为有环图(Tarjan或Kosaraju算法)等。 在使用这个`Graph`类时,你可以创建一个新的图实例,然后添加节点和边: ```javascript const graph = new Graph(); graph.addVertex('A'); graph.addVertex('B'); graph.addVertex('C'); graph.addEdge('A', 'B'); graph.addEdge('B', 'C'); graph.addEdge('C', 'A'); // 打印出图的遍历结果 console.log("Depth First Search:"); graph.traverseDFS('A'); console.log("\n Breadth First Search:"); graph.traverseBFS('A'); ``` 在这个例子中,我们创建了一个包含三个节点'A'、'B'和'C'的无向图,节点'A'和'B'、'B'和'C'、'C'和'A'之间存在边。然后,我们对这个图进行了DFS和BFS遍历,打印出了遍历顺序。 在实际项目中,这样的图结构可以应用于许多场景,例如网络路由、社交网络分析、推荐系统等。理解如何在JavaScript中有效地构建和操作图,对于提升编程技能和解决复杂问题有着重要的意义。
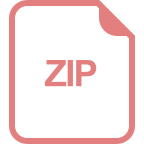
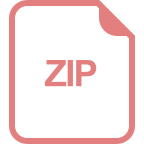
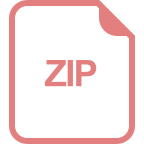
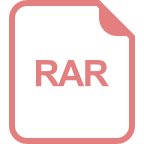
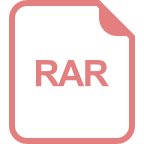
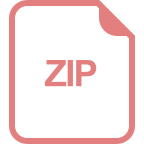
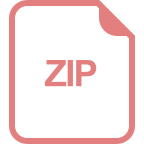
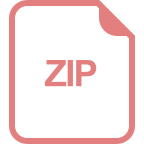
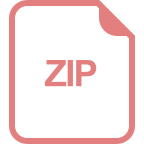
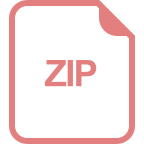
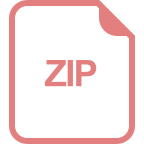
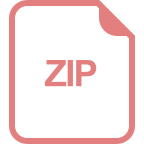
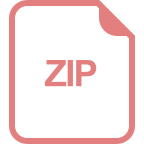
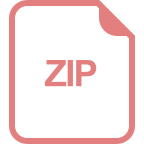
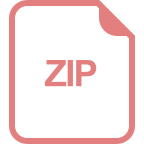
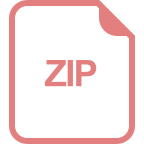
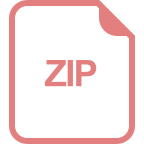
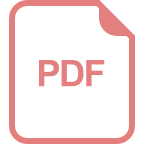
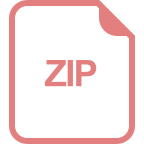
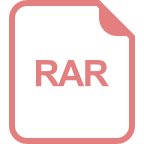
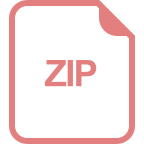
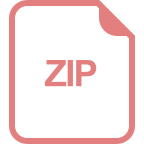
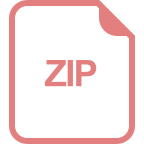
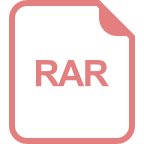
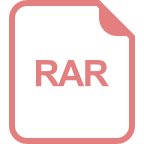
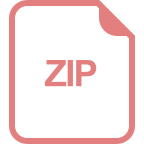


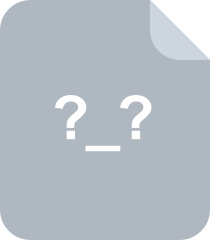
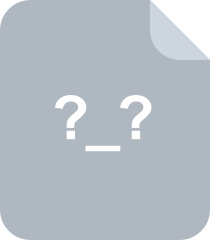
- 1
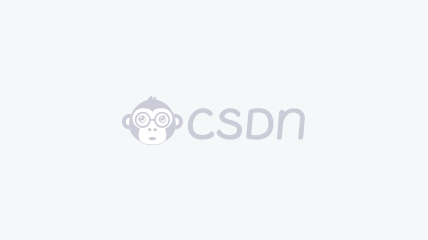

- 粉丝: 26
- 资源: 4602
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

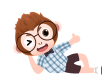
最新资源

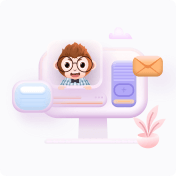
