# genanki: A Library for Generating Anki Decks
`genanki` allows you to programatically generate decks in Python 3 for Anki, a popular spaced-repetition flashcard
program. Please see below for concepts and usage.
*This library and its author(s) are not affiliated/associated with the main Anki project in any way.*
[](https://travis-ci.com/github/kerrickstaley/genanki)
## Notes
The basic unit in Anki is the `Note`, which contains a fact to memorize. `Note`s correspond to one or more `Card`s.
Here's how you create a `Note`:
```python
my_note = genanki.Note(
model=my_model,
fields=['Capital of Argentina', 'Buenos Aires'])
```
You pass in a `Model`, discussed below, and a set of `fields` (encoded as HTML).
## Models
A `Model` defines the fields and cards for a type of `Note`. For example:
```python
my_model = genanki.Model(
1607392319,
'Simple Model',
fields=[
{'name': 'Question'},
{'name': 'Answer'},
],
templates=[
{
'name': 'Card 1',
'qfmt': '{{Question}}',
'afmt': '{{FrontSide}}<hr id="answer">{{Answer}}',
},
])
```
This note-type has two fields and one card. The card displays the `Question` field on the front and the `Question` and
`Answer` fields on the back, separated by a `<hr>`. You can also pass a `css` argument to `Model()` to supply custom
CSS.
You need to pass a `model_id` so that Anki can keep track of your model. It's important that you use a unique `model_id`
for each `Model` you define. Use `import random; random.randrange(1 << 30, 1 << 31)` to generate a suitable model_id, and hardcode it
into your `Model` definition.
## Generating a Deck/Package
To import your notes into Anki, you need to add them to a `Deck`:
```python
my_deck = genanki.Deck(
2059400110,
'Country Capitals')
my_deck.add_note(my_note)
```
Once again, you need a unique `deck_id` that you should generate once and then hardcode into your `.py` file.
Then, create a `Package` for your `Deck` and write it to a file:
```python
genanki.Package(my_deck).write_to_file('output.apkg')
```
You can then load `output.apkg` into Anki using File -> Import...
## Media Files
To add sounds or images, set the `media_files` attribute on your `Package`:
```python
my_package = genanki.Package(my_deck)
my_package.media_files = ['sound.mp3', 'images/image.jpg']
```
`media_files` should have the path (relative or absolute) to each file. To use them in notes, first add a field to your model, and reference that field in your template:
```python
my_model = genanki.Model(
1091735104,
'Simple Model with Media',
fields=[
{'name': 'Question'},
{'name': 'Answer'},
{'name': 'MyMedia'}, # ADD THIS
],
templates=[
{
'name': 'Card 1',
'qfmt': '{{Question}}<br>{{MyMedia}}', # AND THIS
'afmt': '{{FrontSide}}<hr id="answer">{{Answer}}',
},
])
```
Then, set the `MyMedia` field on your card to `[sound:sound.mp3]` for audio and `<img src="image.jpg">` for images.
You *cannot* put `<img src="{MyMedia}">` in the template and `image.jpg` in the field. See these sections in the Anki manual for more information: [Importing Media](https://docs.ankiweb.net/#/importing?id=importing-media) and [Media & LaTeX](https://docs.ankiweb.net/#/templates/fields?id=media-amp-latex).
You should only put the filename (aka basename) and not the full path in the field; `<img src="images/image.jpg">` will *not* work. Media files should have unique filenames.
## Note GUIDs
`Note`s have a `guid` property that uniquely identifies the note. If you import a new note that has the same GUID as an
existing note, the new note will overwrite the old one (as long as their models have the same fields).
This is an important feature if you want to be able to tweak the design/content of your notes, regenerate your deck, and
import the updated version into Anki. Your notes need to have stable GUIDs in order for the new note to replace the
existing one.
By default, the GUID is a hash of all the field values. This may not be desirable if, for example, you add a new field
with additional info that doesn't change the identity of the note. You can create a custom GUID implementation to hash
only the fields that identify the note:
```python
class MyNote(genanki.Note):
@property
def guid(self):
return genanki.guid_for(self.fields[0], self.fields[1])
```
## sort_field
Anki has a value for each `Note` called the `sort_field`. Anki uses this value to sort the cards in the Browse
interface. Anki also is happier if you avoid having two notes with the same `sort_field`, although this isn't strictly
necessary. By default, the `sort_field` is the first field, but you can change it by passing `sort_field=` to `Note()`
or implementing `sort_field` as a property in a subclass (similar to `guid`).
You can also pass `sort_field_index=` to `Model()` to change the sort field. `0` means the first field in the Note, `1` means the second, etc.
## YAML for Templates (and Fields)
You can create your template definitions in the YAML format and pass them as a `str` to `Model()`. You can also do this
for fields.
## Using genanki inside an Anki addon
`genanki` supports adding generated notes to the local collection when running inside an Anki 2.1 addon (Anki 2.0
may work but has not been tested). See the [`.write_to_collection_from_addon() method`](
https://github.com/kerrickstaley/genanki/blob/0c2cf8fea9c5e382e2fae9cd6d5eb440e267c637/genanki/__init__.py#L275).
## FAQ
### My field data is getting garbled
If fields in your notes contain literal `<`, `>`, or `&` characters, you need to HTML-encode them: field data is HTML, not plain text. You can use the [`html.escape`](https://docs.python.org/3/library/html.html#html.escape) function.
For example, you should write
```
fields=['AT&T was originally called', 'Bell Telephone Company']
```
or
```
fields=[html.escape(f) for f in ['AT&T was originally called', 'Bell Telephone Company']]
```
This applies even if the content is LaTeX; for example, you should write
```
fields=['Piketty calls this the "central contradiction of capitalism".', '[latex]r > g[/latex]']
```
## Publishing to PyPI
If your name is Kerrick, you can publish the `genanki` package to PyPI by running these commands from the root of the `genanki` repo:
```
rm -rf dist/*
python3 setup.py sdist bdist_wheel
python3 -m twine upload dist/*
```
Note that this directly uploads to prod PyPI and skips uploading to test PyPI.

彭仕安
- 粉丝: 29
- 资源: 4678
最新资源
- 储能变流器三相并网电压矢量控制控制(双向充放电) 0.0~0.7s:储能向电网供电50kW 0.7 ~1.2s:电网向电池充电50kW 0.7秒电池充电切放电,电网380AC,母线电压800V,电池
- 高频注入仿真pmsm 无感控制 解决0速转矩输出问题 插入式永磁同步电机,凸极,高频注入 MATLAB simulink仿真,供研究学习
- 机械设计饺子机sw18可编辑非常好的设计图纸100%好用.zip
- 氢电混合储能系统仿真(光伏,锂电池,燃料电池) 储能共直流母线 光伏储能共交流母线 储能由氢燃料电池锂电池组成 直流母线电压稳定在800v 考虑光伏故障下系统的运行特性
- 机械设计立式超声波焊接设备sw17非常好的设计图纸100%好用.zip
- 磁链观测器(仿真+闭环代码+参考文档) 1.仿真采用simulink搭建,2018b版本 2.代码采用Keil软件编译,思路参考vesc中使用的方法,自己编写的代码能够实现0速闭环启动,并且标注有大量
- 纯Java常用方法工具类
- Ceph一些介绍和使用说明.zip
- 电气仿真 分布式电源接入对配电网的影响 分布式电源是双馈感应发电机(DFIG)实现了变速变桨控制风力涡轮机模型 (lunwen5)参考lunwen可提供
- 机械设计精密电子自动除尘生产线sw17可编辑非常好的设计图纸100%好用.zip
- 机械设计快走丝电火花线切割机床(毕设ug8+cad+说明书)非常好的设计图纸100%好用.zip
- 激光熔覆仿真comsol通过激光进行熔覆工艺进行仿真,对温度与应力进行研究 采用COMSOL中的固体传热等物理场进行耦合仿真 对激光熔覆工艺完成后的温度分布与应力分布以云图形式输出,并研究某一点温度与
- CSS3.0博客学习配套完整参考手册 v4.2.4
- 全新版本码支付个人免签支付系统源码
- 西门子变频器 SINAMICS STARTER V5.6 HF2 软件 STARTER V56 STARTERV56HF2-cd-2.zip.003
- 纯java安全线程池工具类
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


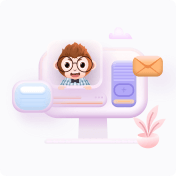
评论0