# Eclipse Paho JavaScript client
[](https://travis-ci.org/eclipse/paho.mqtt.javascript)
The Paho JavaScript Client is an MQTT browser-based client library written in Javascript that uses WebSockets to connect to an MQTT Broker.
## Project description:
The Paho project has been created to provide reliable open-source implementations of open and standard messaging protocols aimed at new, existing, and emerging applications for Machine-to-Machine (M2M) and Internet of Things (IoT).
Paho reflects the inherent physical and cost constraints of device connectivity. Its objectives include effective levels of decoupling between devices and applications, designed to keep markets open and encourage the rapid growth of scalable Web and Enterprise middleware and applications.
## Links
- Project Website: [https://www.eclipse.org/paho](https://www.eclipse.org/paho)
- Eclipse Project Information: [https://projects.eclipse.org/projects/iot.paho](https://projects.eclipse.org/projects/iot.paho)
- Paho Javascript Client Page: [https://eclipse.org/paho/clients/js/](https://eclipse.org/paho/clients/js)
- GitHub: [https://github.com/eclipse/paho.mqtt.javascript](https://github.com/eclipse/paho.mqtt.javascript)
- Twitter: [@eclipsepaho](https://twitter.com/eclipsepaho)
- Issues: [github.com/eclipse/paho.mqtt.javascript/issues](https://github.com/eclipse/paho.mqtt.javascript/issues)
- Mailing-list: [https://dev.eclipse.org/mailman/listinfo/paho-dev](https://dev.eclipse.org/mailman/listinfo/paho-dev)
## Using the Paho Javascript Client
### Downloading
A zip file containing the full and a minified version the Javascript client can be downloaded from the [Paho downloads page](https://projects.eclipse.org/projects/iot.paho/downloads)
Alternatively the Javascript client can be downloaded directly from the projects git repository: [https://raw.githubusercontent.com/eclipse/paho.mqtt.javascript/master/src/paho-mqtt.js](https://raw.githubusercontent.com/eclipse/paho.mqtt.javascript/master/src/paho-mqtt.js).
Please **do not** link directly to this url from your application.
### Building from source
There are two active branches on the Paho Java git repository, ```master``` which is used to produce stable releases, and ```develop``` where active development is carried out. By default cloning the git repository will download the ```master``` branch, to build from develop make sure you switch to the remote branch: ```git checkout -b develop remotes/origin/develop```
The project contains a maven based build that produces a minified version of the client, runs unit tests and generates it's documentation.
To run the build:
```
$ mvn
```
The output of the build is copied to the ```target``` directory.
### Tests
The client uses the [Jasmine](http://jasmine.github.io/) test framework. The tests for the client are in:
```
src/tests
```
To run the tests with maven, use the following command:
```
$ mvn test
```
The parameters passed in should be modified to match the broker instance being tested against.
### Documentation
Reference documentation is online at: [http://www.eclipse.org/paho/files/jsdoc/index.html](http://www.eclipse.org/paho/files/jsdoc/index.html)
### Compatibility
The client should work in any browser fully supporting WebSockets, [http://caniuse.com/websockets](http://caniuse.com/websockets) lists browser compatibility.
## Getting Started
The included code below is a very basic sample that connects to a server using WebSockets and subscribes to the topic ```World```, once subscribed, it then publishes the message ```Hello``` to that topic. Any messages that come into the subscribed topic will be printed to the Javascript console.
This requires the use of a broker that supports WebSockets natively, or the use of a gateway that can forward between WebSockets and TCP.
```JS
// Create a client instance
var client = new Paho.MQTT.Client(location.hostname, Number(location.port), "clientId");
// set callback handlers
client.onConnectionLost = onConnectionLost;
client.onMessageArrived = onMessageArrived;
// connect the client
client.connect({onSuccess:onConnect});
// called when the client connects
function onConnect() {
// Once a connection has been made, make a subscription and send a message.
console.log("onConnect");
client.subscribe("World");
message = new Paho.MQTT.Message("Hello");
message.destinationName = "World";
client.send(message);
}
// called when the client loses its connection
function onConnectionLost(responseObject) {
if (responseObject.errorCode !== 0) {
console.log("onConnectionLost:"+responseObject.errorMessage);
}
}
// called when a message arrives
function onMessageArrived(message) {
console.log("onMessageArrived:"+message.payloadString);
}
```
## Breaking Changes
Previously the Client's Namepsace was `Paho.MQTT`, as of version 1.1.0 (develop branch) this has now been simplified to `Paho`.
You should be able to simply do a find and replace in your code to resolve this, for example all instances of `Paho.MQTT.Client` will now be `Paho.Client` and `Paho.MQTT.Message` will be `Paho.Message`.
没有合适的资源?快使用搜索试试~ 我知道了~
paho.mqtt.javascript.zip
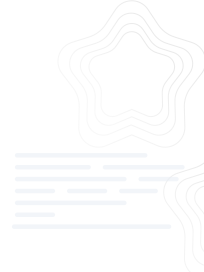
共36个文件
js:9个
md:5个
txt:3个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 182 浏览量
2024-11-25
01:10:59
上传
评论
收藏 67KB ZIP 举报
温馨提示
Eclipse Paho JavaScript 客户端Paho JavaScript 客户端是一个用 Javascript 编写的基于 MQTT 浏览器的客户端库,它使用 WebSockets 连接到 MQTT 代理。项目描述Paho 项目的创建旨在为面向机器对机器 (M2M) 和物联网 (IoT) 的新应用、现有应用和新兴应用的开放标准消息传递协议提供可靠的开源实现。Paho 反映了设备连接固有的物理和成本限制。其目标包括有效分离设备和应用,旨在保持市场开放并促进可扩展 Web 和企业中间件及应用的快速增长。链接项目网站https://www.eclipse.org/pahoEclipse 项目信息https://projects.eclipse.org/projects/iot.pahoPaho Javascript 客户端页面 https: //eclipse.org/paho/clients/js/GitHubhttps ://github.com/eclipse/paho.mqtt.javascriptTwitter: @eclipsepaho问题
资源推荐
资源详情
资源评论
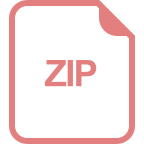
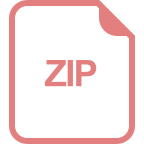
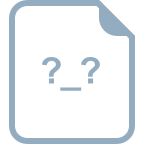
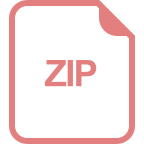
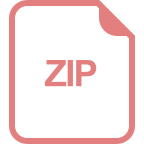
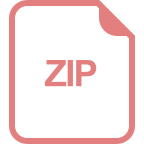
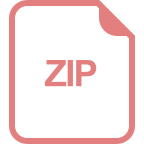
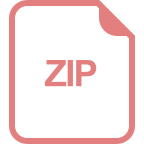
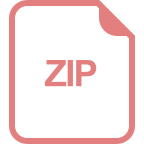
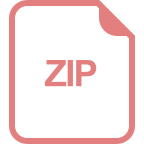
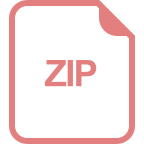
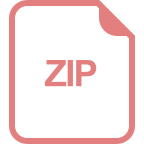
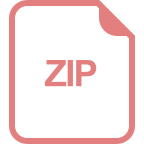
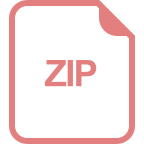
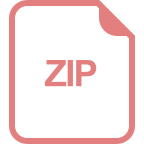
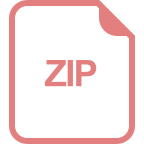
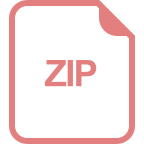
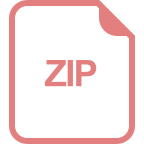
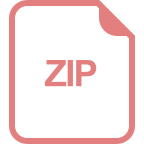
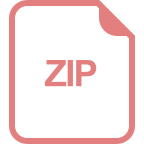
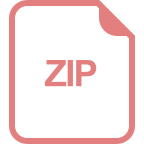
收起资源包目录

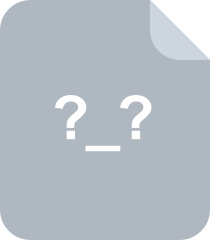
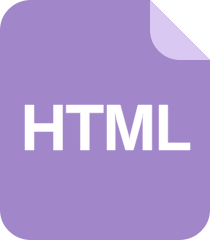

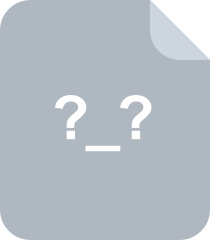
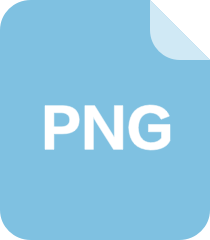
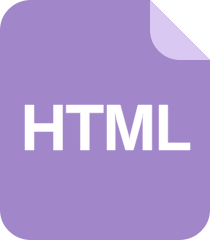
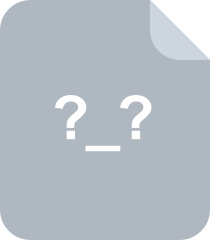
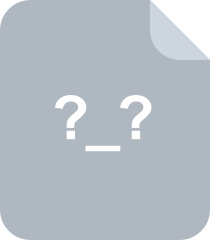
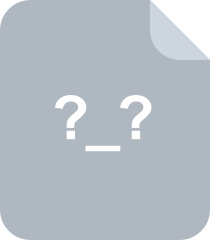
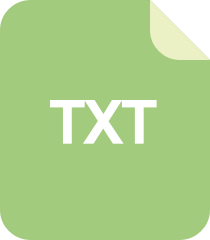


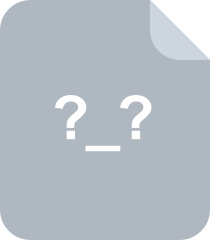
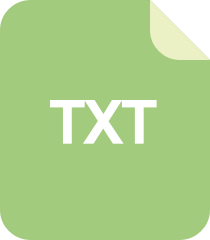
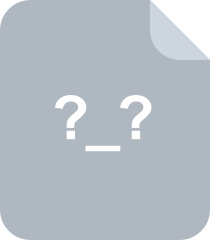

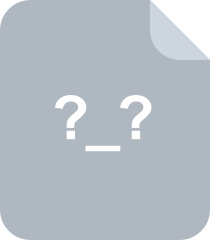
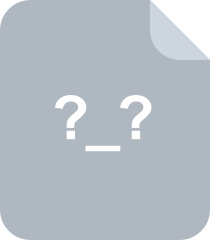
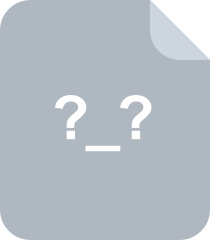
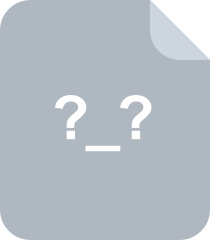
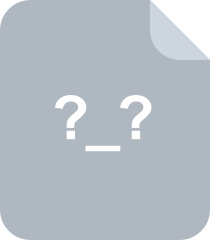
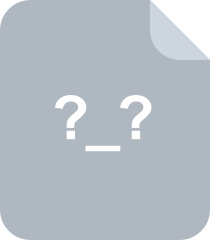
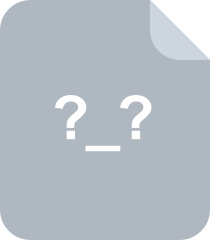
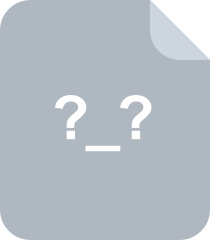
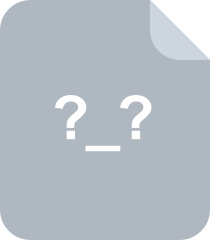
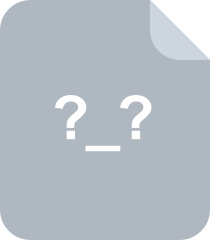
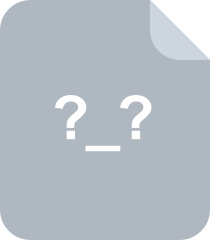
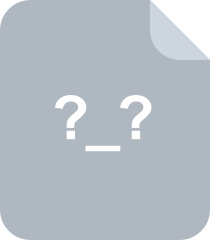
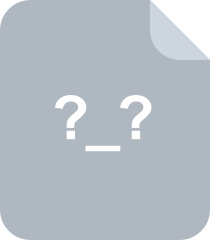
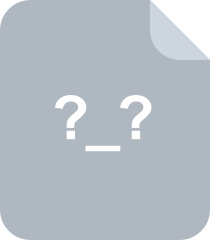
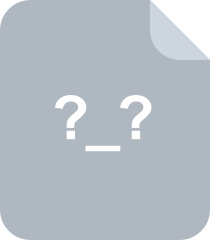
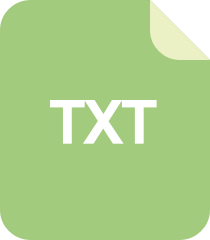
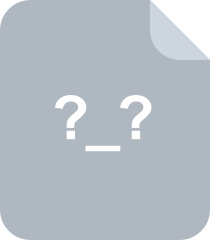
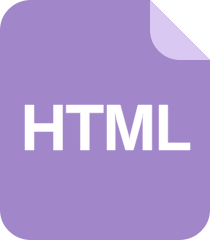
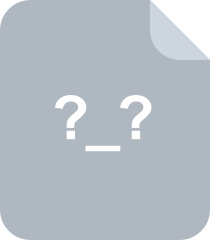
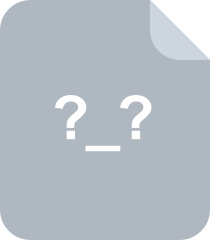
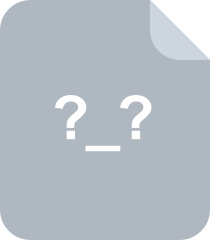
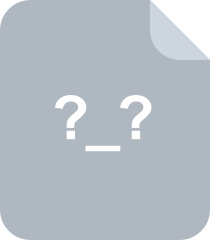
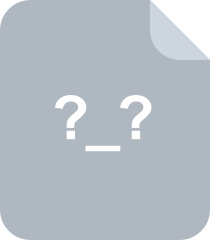
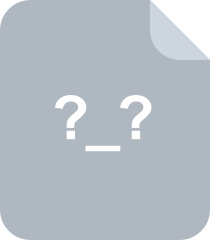
共 36 条
- 1
资源评论
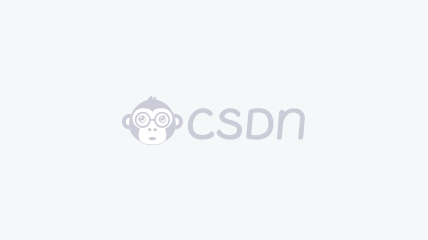

赵闪闪168
- 粉丝: 1525
- 资源: 2758
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

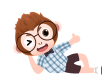
最新资源
- (源码)基于Spark的实时用户行为分析系统.zip
- (源码)基于Spring Boot和Vue的个人博客后台管理系统.zip
- 将流行的 ruby faker gem 引入 Java.zip
- (源码)基于C#和ArcGIS Engine的房屋管理系统.zip
- (源码)基于C语言的Haribote操作系统项目.zip
- (源码)基于Spring Boot框架的秒杀系统.zip
- (源码)基于Qt框架的待办事项管理系统.zip
- 将 Java 8 的 lambda 表达式反向移植到 Java 7、6 和 5.zip
- (源码)基于JavaWeb的学生管理系统.zip
- (源码)基于C++和Google Test框架的数独游戏生成与求解系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


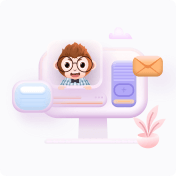
安全验证
文档复制为VIP权益,开通VIP直接复制
