package cn.controller;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.List;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.apache.log4j.Logger;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.alibaba.fastjson.JSONArray;
import cn.pojo.Category;
import cn.pojo.Entry;
import cn.service.EbookService;
import cn.tools.PageSupport;
@Controller
public class EbookController extends BaseController{
@SuppressWarnings("unused")
private Logger logger = Logger.getLogger(EbookController.class);
@Resource
private EbookService ebookService;
//查询数据,支持模糊查询,分页功能
@RequestMapping(value = "/list")
public String getBillList(
Model model,HttpSession session,
@RequestParam(value = "category", required = false) String category,
@RequestParam(value = "pageIndex", required = false) String pageIndex) {
List<Entry> entryList = null;
int categoryId =0;
if (category != null) {
categoryId = Integer.valueOf(category);
}
// 设置页面容量
int pageSize = 3;
// 当前页码
int currentPageNo = 1;
// 设置页码数
if (pageIndex != null) {
currentPageNo = Integer.valueOf(pageIndex);
}
// 总数量(表)
int totalCount = 0;
try {
totalCount = ebookService.getEntryCount();
} catch (Exception e) {
e.printStackTrace();
}
// 总页数
PageSupport pages = new PageSupport();
pages.setCurrentPageNo(currentPageNo);
pages.setPageSize(pageSize);
pages.setTotalCount(totalCount);
int totalPageCount = pages.getTotalPageCount();
// 控制首页和尾页
if (currentPageNo < 1) {
currentPageNo = 1;
} else if (currentPageNo > totalPageCount) {
currentPageNo = totalPageCount;
}
try {
if (categoryId !=1 && categoryId !=2 && categoryId !=3&& categoryId !=4) {
categoryId =0;
}
entryList = ebookService.getEntryList(categoryId, currentPageNo, pageSize);
} catch (Exception e) {
e.printStackTrace();
}
model.addAttribute("entryList",entryList);
model.addAttribute("totalPageCount", totalPageCount);
model.addAttribute("totalCount", totalCount);
model.addAttribute("currentPageNo", currentPageNo);
model.addAttribute("pages", pages);
return "projectinfosList";
}
@RequestMapping(value = "/del", method = RequestMethod.GET)
@ResponseBody
public Object deluser(@RequestParam String id) throws NumberFormatException, Exception {
int count = ebookService.delete(Integer.parseInt(id));
return JSONArray.toJSONString(count);
}
@RequestMapping(value = "/add")
public String addBill() {
return "add";
}
@RequestMapping(value = "/addsave", method = RequestMethod.POST)
public String addPro(HttpSession session,
@RequestParam(value = "title", required = false) String title,
@RequestParam(value = "summary", required = false) String summary,
@RequestParam(value = "uploaduser", required = false) String uploaduser,
@RequestParam(value = "categoryId", required = false) String categoryId,
@RequestParam(value = "createdate", required = false) String createdate) throws Exception{
DateFormat format1 = new SimpleDateFormat("yyyy-MM-dd");
try {
Entry projectinfo=new Entry();
projectinfo.setTitle(title);
projectinfo.setSummary(summary);
projectinfo.setUploaduser(uploaduser);
projectinfo.setCreatedate(format1.parse(createdate));
projectinfo.setCategoryId(Integer.parseInt(categoryId));
int num=ebookService.add(projectinfo);
if(num>0){
return "redirect:/list";
}else{
return "add";
}
} catch (ParseException e) {
e.printStackTrace();
}
return "redirect:/list";
}
/**
* 按照id查询信息并跳转到修改页面
* @param id
* @param model
* @param request
* @return
* @throws Exception
* @throws NumberFormatException
*/
@RequestMapping(value = "/upd/{id}", method = RequestMethod.GET)
public String getProById(@PathVariable String id, Model model,
HttpServletRequest request) throws NumberFormatException, Exception {
Entry entry=new Entry();
entry=ebookService.getEntryById(Integer.valueOf(id));
model.addAttribute("entry",entry);
return "updBook";
}
/**
* 修改
* @param id
* @param statu
* @param session
* @return
*/
@RequestMapping(value ="/updsave", method = RequestMethod.POST)
public String modifySave(
@RequestParam(value = "id", required = false) String id,
@RequestParam(value = "title", required = false) String title,
@RequestParam(value = "summary", required = false) String summary,
@RequestParam(value = "uploaduser", required = false) String uploaduser,
@RequestParam(value = "userRole", required = false) String userRole,
@RequestParam(value = "createdate", required = false) String createdate,
HttpSession session) {
DateFormat format1 = new SimpleDateFormat("yyyy-MM-dd");
try {
Entry projectinfo=new Entry();
projectinfo.setId(Integer.valueOf(id));
projectinfo.setTitle(title);
projectinfo.setSummary(summary);
projectinfo.setUploaduser(uploaduser);
projectinfo.setCreatedate(format1.parse(createdate));
projectinfo.setCategoryId(Integer.parseInt(userRole));
if (ebookService.modify(projectinfo)>0) {
session.setAttribute("tishi", "修改成功!");
return "redirect:/list";
}
} catch (Exception e) {
e.printStackTrace();
}
session.setAttribute("tishi", "修改失败!");
return "redirect:/list";
}
/**
* 异步获取图书分类
* @return
*/
@RequestMapping(value="/rolelist.json",method=RequestMethod.GET)
@ResponseBody
public List<Category> getCategoryList(){
List<Category>categoryList = null;
try {
categoryList = ebookService.getCategory();
} catch (Exception e) {
e.printStackTrace();
}
logger.info("categoryList 数量:"+categoryList.size());
return categoryList;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
电子图书管理系统
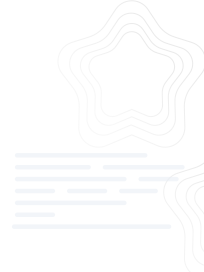
共78个文件
jar:25个
xml:10个
class:9个


温馨提示
使用 SSM(Spring MVC + Spring + MyBatis)框架来实现电子图书管理系统,MySQL 5.5作为后台数据库,该系统包括查看所有的电子图书信息、根据图书分类查询、增加、更新、删除电子图书功能
资源推荐
资源详情
资源评论
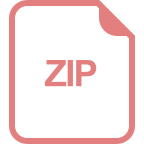
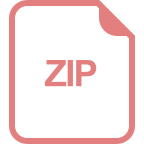
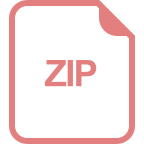
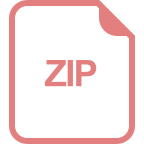
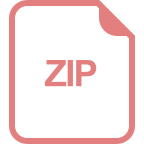
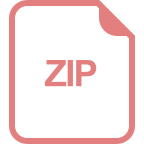
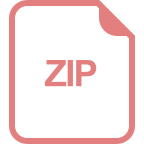
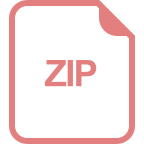
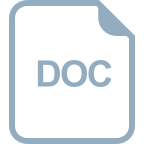
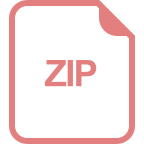
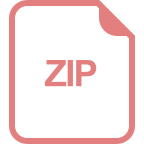
收起资源包目录


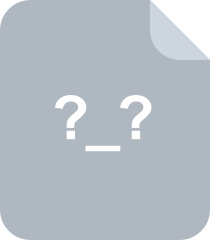

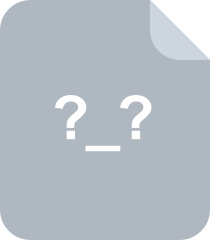
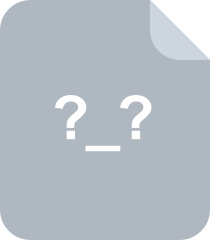
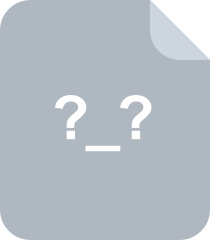
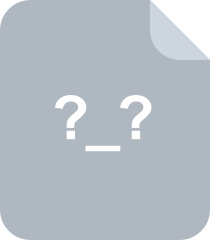
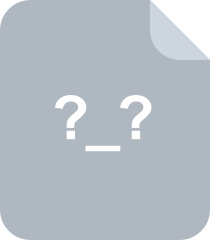

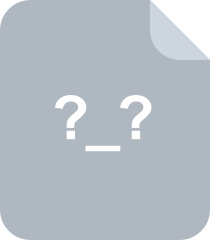
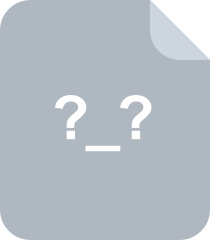
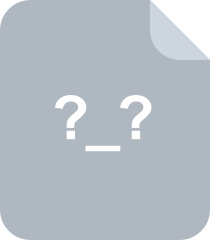
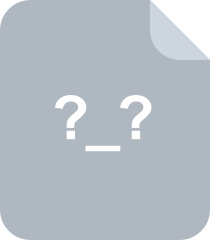
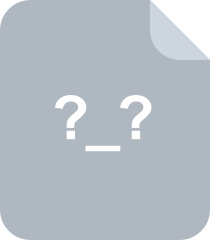
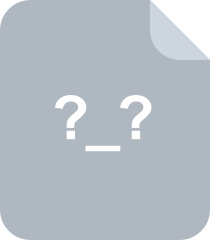
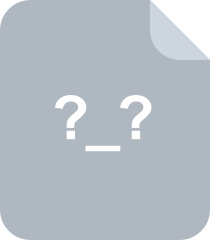
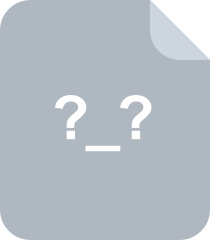



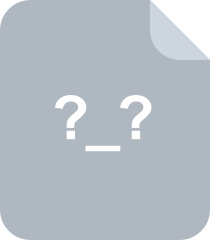

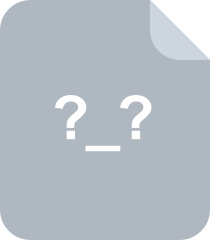
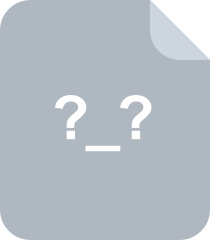
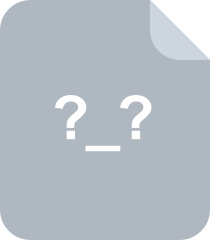
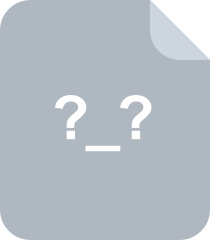
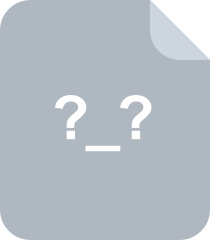

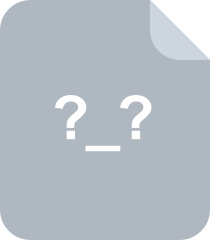

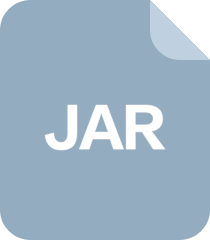
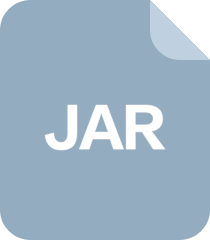
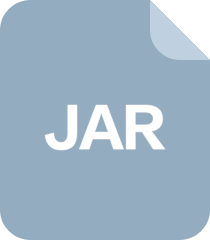
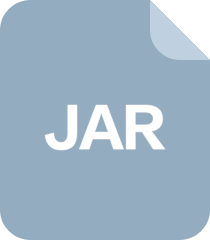
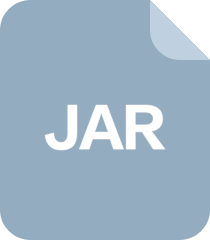
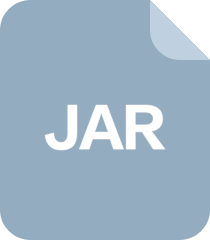
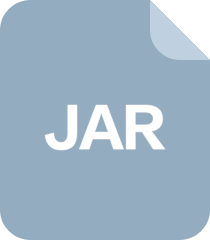
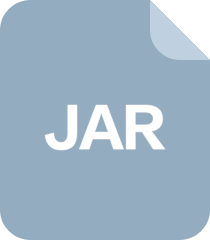
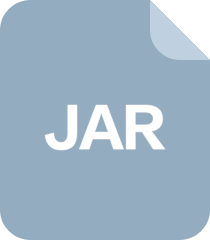
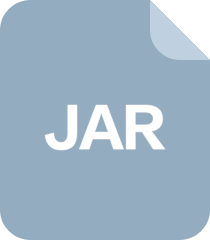
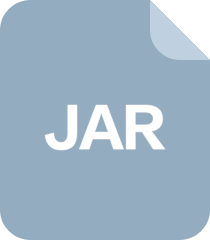
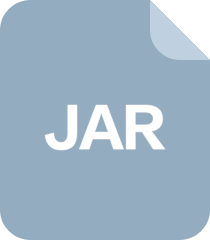
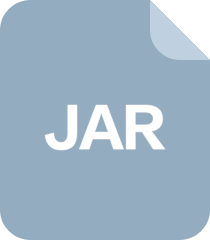
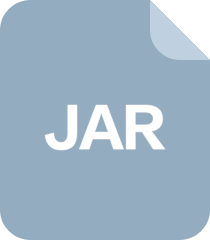
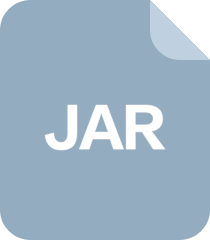
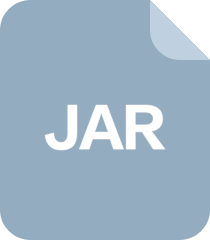
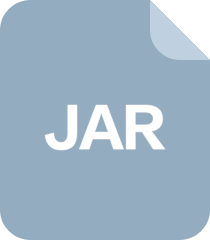
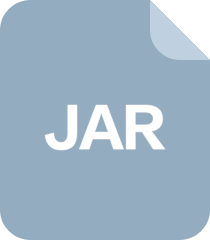
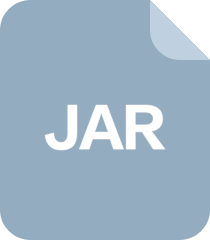
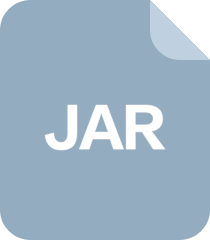
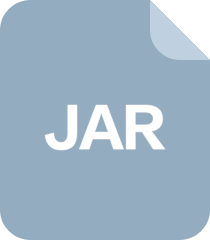
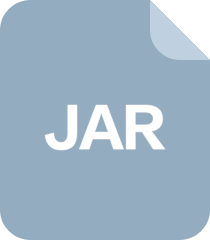
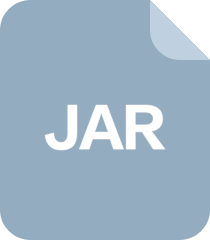
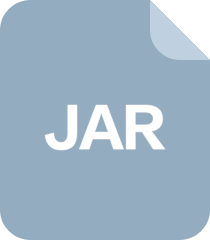
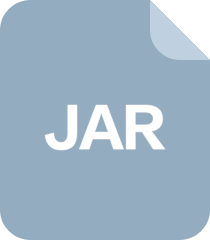

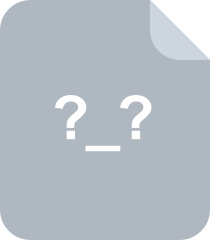
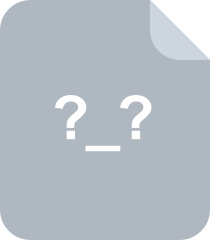


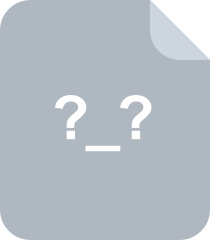
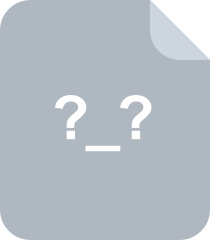

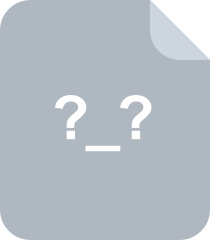
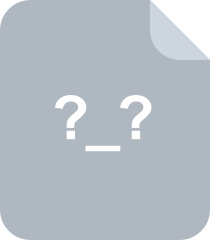

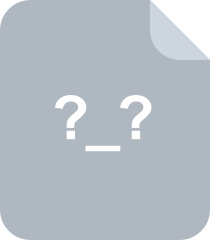
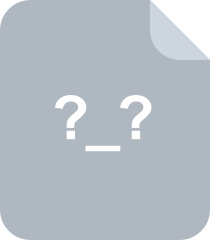

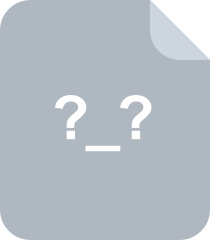
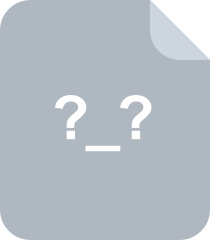

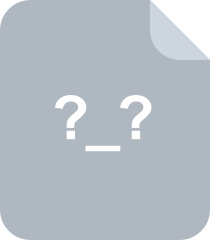
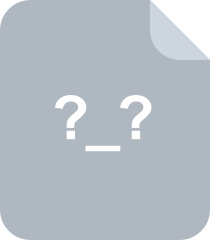
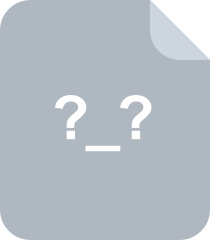
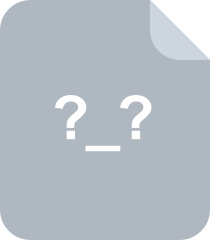
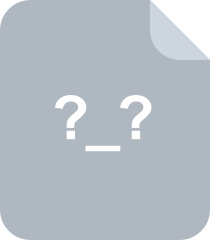

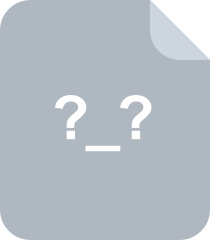
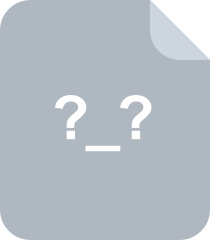
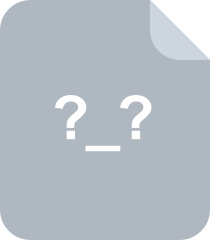
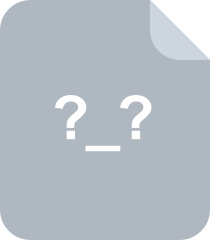
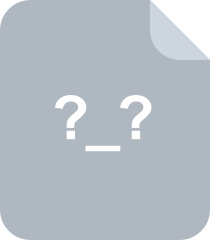



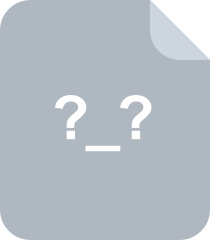
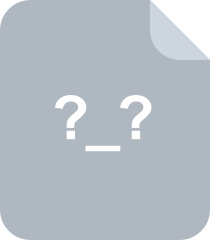

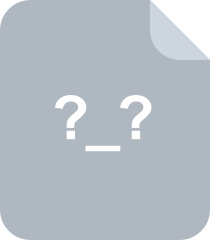
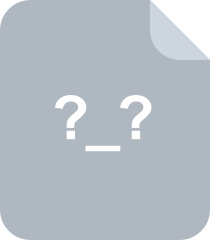

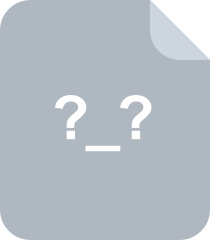
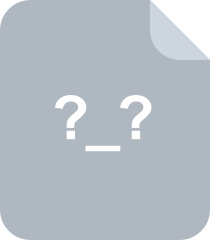

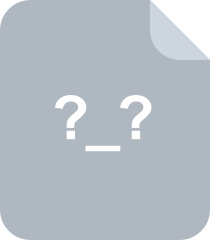
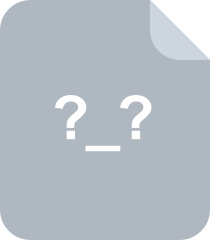

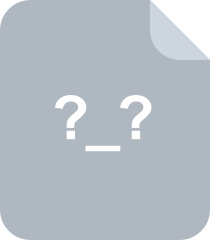
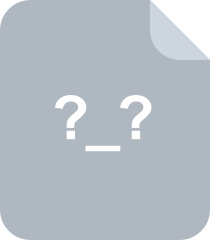
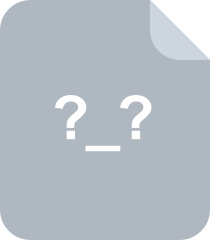
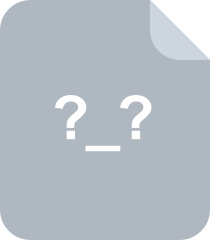
共 78 条
- 1
资源评论
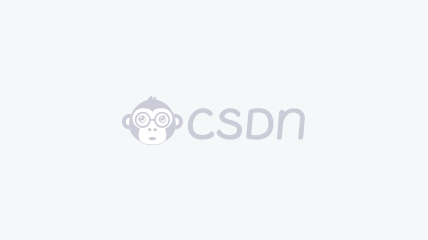
- weixin_420935042019-04-15资源不错,可以使用
- gudou2020-06-04没成功……没教程让门外汉怎么办

Rokie-770880
- 粉丝: 9
- 资源: 15
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

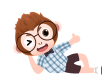
最新资源
- (源码)基于C++和Qt框架的游戏工作室服务器管理系统.zip
- (源码)基于Spring Boot的赛事管理系统.zip
- (源码)基于C#和ASP.NET Core的智能家居管理系统.zip
- (源码)基于rosserial的STM32嵌入式ROS通信系统库(Yoneken版改进版).zip
- 9.4 使用生成的识别器模型faceModel.xml预测新图像,并输出匹配结果标签和置信度
- (源码)基于Spring Boot和Shiro的电商管理系统.zip
- (源码)基于Arduino和Blinker的智能时钟控制系统.zip
- (源码)基于C++编程语言的WyoOS操作系统.zip
- 9.3 使用EigenFaceRecognizer训练人脸分类器,并将模型保存为faceModel.xml文件
- (源码)基于Spring Boot 2的管理后台系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


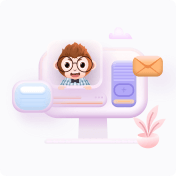
安全验证
文档复制为VIP权益,开通VIP直接复制
