# Highlight.js
[](https://www.npmjs.com/package/highlight.js)
[](https://github.com/highlightjs/highlight.js/blob/main/LICENSE)
[](https://packagephobia.now.sh/result?p=highlight.js)

[](https://www.npmjs.com/package/highlight.js)
[](https://www.jsdelivr.com/package/gh/highlightjs/cdn-release)
<!-- [](https://github.com/highlightjs/highlight.js/actions?query=workflow%3A%22Node.js+CI%22) -->

[](https://lgtm.com/projects/g/highlightjs/highlight.js/?mode=list)
[](https://snyk.io/test/github/highlightjs/highlight.js?targetFile=package.json)

[](https://discord.gg/M24EbU7ja9)
[](https://github.com/highlightjs/highlight.js/issues)
[](https://github.com/highlightjs/highlight.js/issues?q=is%3Aopen+is%3Aissue+label%3A%22help+welcome%22)
[](https://github.com/highlightjs/highlight.js/issues?q=is%3Aopen+is%3Aissue+label%3A%22good+first+issue%22)
<!-- [](https://github.com/highlightjs/highlight.js/actions?query=workflow%3A%22Node.js+CI%22) -->
<!-- [](https://github.com/highlightjs/highlight.js/commits/main) -->
<!-- [](https://www.jsdelivr.com/package/gh/highlightjs/cdn-release) -->
<!-- []() -->
<!-- [](https://bundlephobia.com/result?p=highlight.js) -->
Highlight.js is a syntax highlighter written in JavaScript. It works in
the browser as well as on the server. It can work with pretty much any
markup, doesn’t depend on any other frameworks, and has automatic language
detection.
**Contents**
- [Basic Usage](#basic-usage)
- [In the Browser](#in-the-browser)
- [Plaintext Code Blocks](#plaintext-code-blocks)
- [Ignoring a Code Block](#ignoring-a-code-block)
- [Node.js on the Server](#nodejs-on-the-server)
- [Supported Languages](#supported-languages)
- [Custom Usage](#custom-usage)
- [Using custom HTML](#using-custom-html)
- [Using with Vue.js](#using-with-vuejs)
- [Using Web Workers](#using-web-workers)
- [Importing the Library](#importing-the-library)
- [Node.js / `require`](#nodejs--require)
- [ES6 Modules / `import`](#es6-modules--import)
- [Getting the Library](#getting-the-library)
- [Fetch via CDN](#fetch-via-cdn)
- [cdnjs (link)](#cdnjs-link)
- [jsdelivr (link)](#jsdelivr-link)
- [unpkg (link)](#unpkg-link)
- [Download prebuilt CDN assets](#download-prebuilt-cdn-assets)
- [Download from our website](#download-from-our-website)
- [Install via NPM package](#install-via-npm-package)
- [Build from Source](#build-from-source)
- [Requirements](#requirements)
- [License](#license)
- [Links](#links)
---
#### Upgrading to Version 11
As always, major releases do contain breaking changes which may require action from users. Please read [VERSION_11_UPGRADE.md](https://github.com/highlightjs/highlight.js/blob/main/VERSION_11_UPGRADE.md) for a detailed summary of breaking changes and any actions you may need to take.
#### Support for older versions <!-- omit in toc -->
Please see [SECURITY.md](https://github.com/highlightjs/highlight.js/blob/main/SECURITY.md) for long-term support information.
---
## Basic Usage
### In the Browser
The bare minimum for using highlight.js on a web page is linking to the
library along with one of the themes and calling [`highlightAll`][1]:
```html
<link rel="stylesheet" href="/path/to/styles/default.min.css">
<script src="/path/to/highlight.min.js"></script>
<script>hljs.highlightAll();</script>
```
This will find and highlight code inside of `<pre><code>` tags; it tries
to detect the language automatically. If automatic detection doesn’t
work for you, or you simply prefer to be explicit, you can specify the language manually in the using the `class` attribute:
```html
<pre><code class="language-html">...</code></pre>
```
#### Plaintext Code Blocks
To apply the Highlight.js styling to plaintext without actually highlighting it, use the `plaintext` language:
```html
<pre><code class="language-plaintext">...</code></pre>
```
#### Ignoring a Code Block
To skip highlighting of a code block completely, use the `nohighlight` class:
```html
<pre><code class="nohighlight">...</code></pre>
```
### Node.js on the Server
The bare minimum to auto-detect the language and highlight some code.
```js
// load the library and ALL languages
hljs = require('highlight.js');
html = hljs.highlightAuto('<h1>Hello World!</h1>').value
```
To load only a "common" subset of popular languages:
```js
hljs = require('highlight.js/lib/common');
```
To highlight code with a specific language, use `highlight`:
```js
html = hljs.highlight('<h1>Hello World!</h1>', {language: 'xml'}).value
```
See [Importing the Library](#importing-the-library) for more examples of `require` vs `import` usage, etc. For more information about the result object returned by `highlight` or `highlightAuto` refer to the [api docs](https://highlightjs.readthedocs.io/en/latest/api.html).
## Supported Languages
Highlight.js supports over 180 languages in the core library. There are also 3rd party
language definitions available to support even more languages. You can find the full list of supported languages in [SUPPORTED_LANGUAGES.md][9].
## Custom Usage
If you need a bit more control over the initialization of
Highlight.js, you can use the [`highlightElement`][3] and [`configure`][4]
functions. This allows you to better control *what* to highlight and *when*.
For example, here’s the rough equivalent of calling [`highlightAll`][1] but doing the work manually instead:
```js
document.addEventListener('DOMContentLoaded', (event) => {
document.querySelectorAll('pre code').forEach((el) => {
hljs.highlightElement(el);
});
});
```
Please refer to the documentation for [`configure`][4] options.
### Using custom HTML
We strongly recommend `<pre><code>` wrapping for code blocks. It's quite
semantic and "just works" out of the box with zero fiddling. It is possible to
use other HTML elements (or combos), but you may need to pay special attention to
preserving linebreaks.
Let's say your markup for code bl
没有合适的资源?快使用搜索试试~ 我知道了~
java 开发的 简单的 储物柜
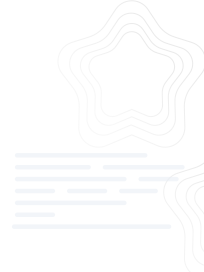
共1667个文件
js:801个
css:273个
scss:243个

0 下载量 165 浏览量
2024-09-02
08:23:03
上传
评论
收藏 8.52MB RAR 举报
温馨提示
java 开发的 简单的 储物柜 可以生成二维码 放在物品柜子或者纸盒子上 扫描二维码直接进入系统 可以查看该盒子内部放置了哪些东西 方便收纳物件
资源推荐
资源详情
资源评论
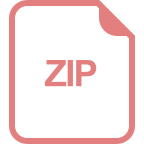
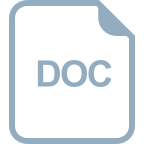
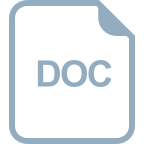
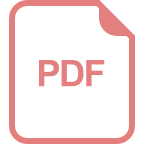
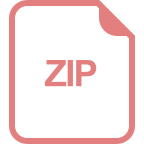
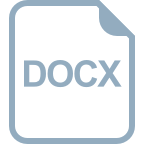
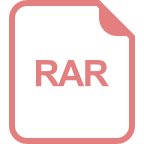
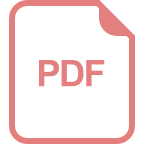
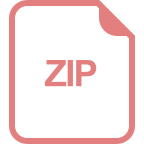
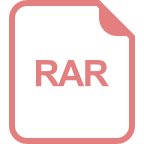
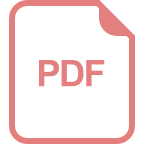
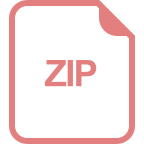
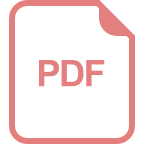
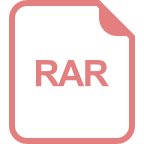
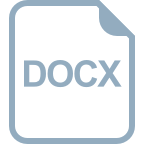
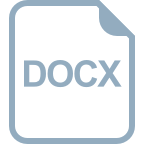
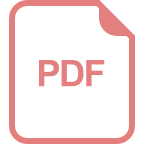
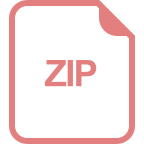
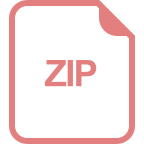
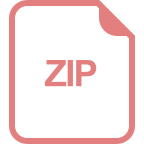
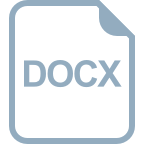
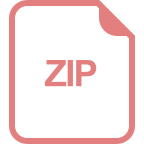
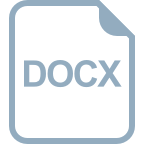
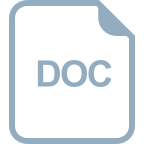
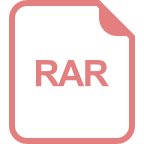
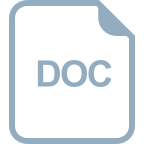
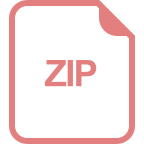
收起资源包目录

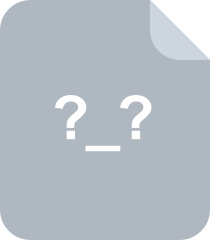
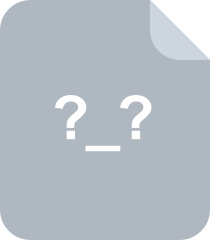
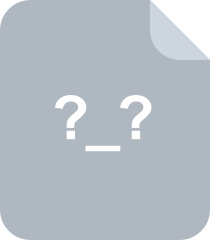
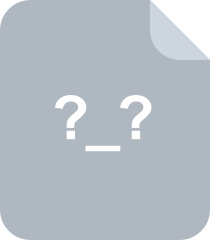
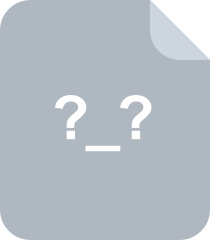
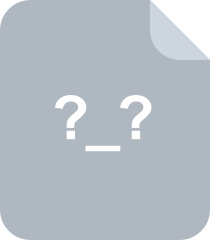
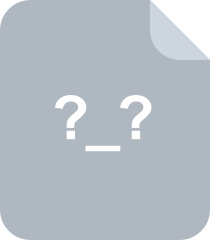
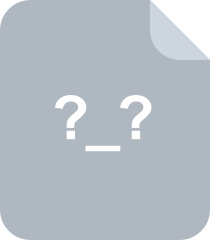
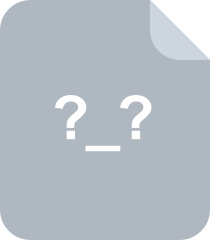
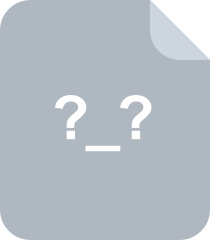
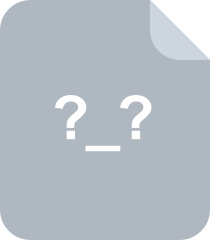
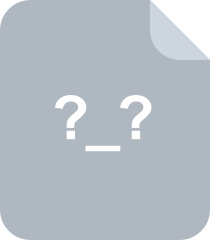
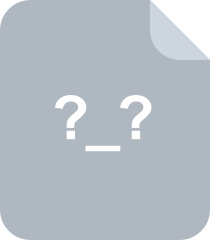
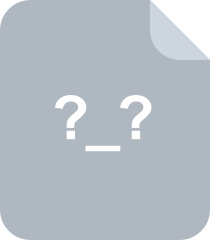
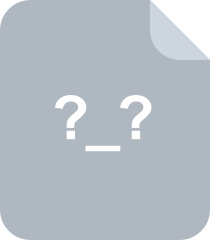
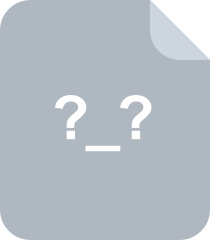
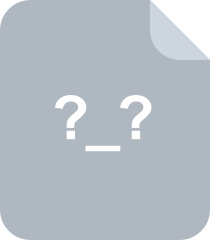
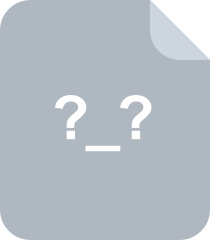
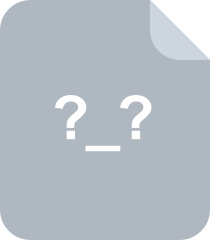
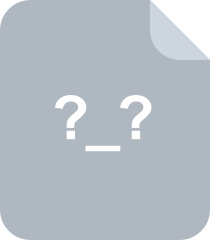
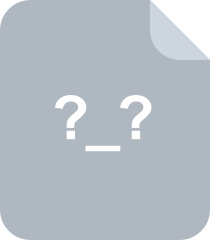
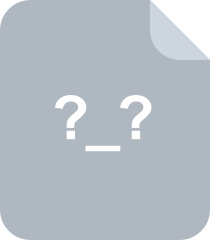
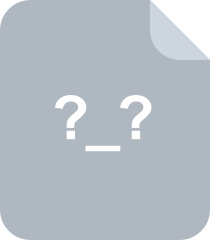
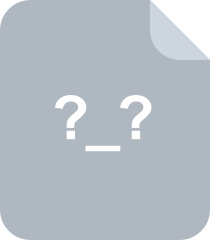
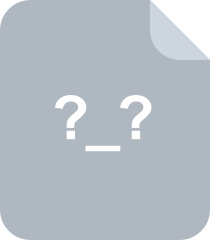
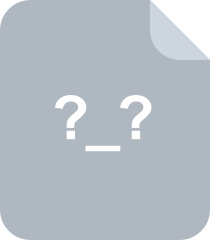
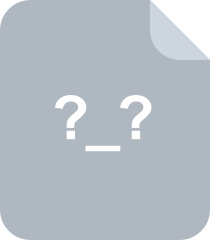
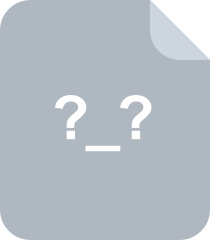
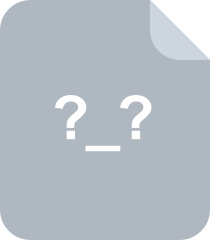
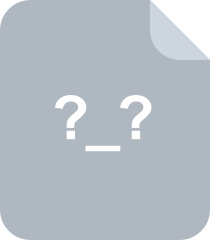
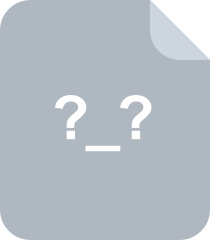
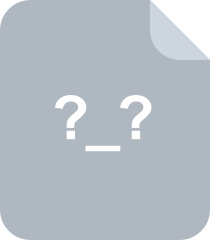
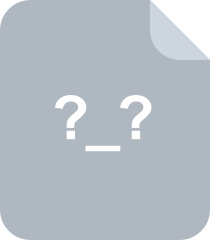
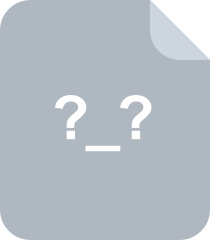
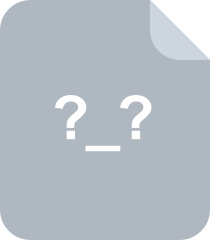
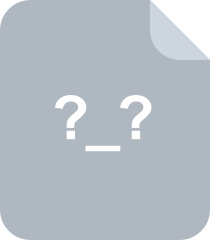
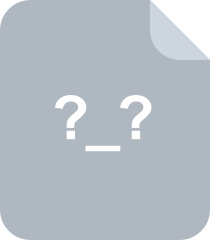
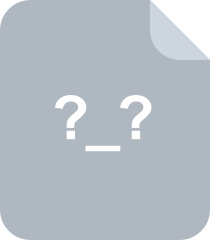
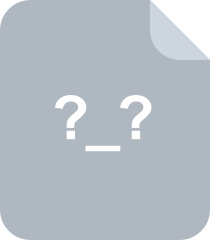
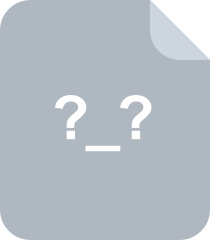
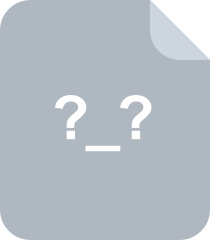
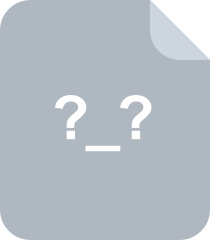
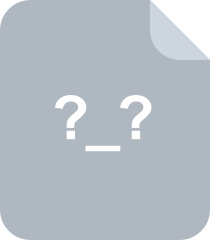
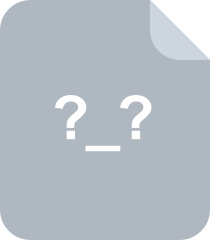
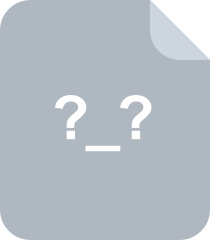
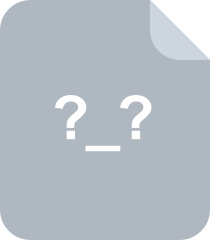
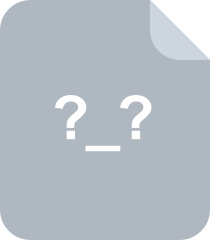
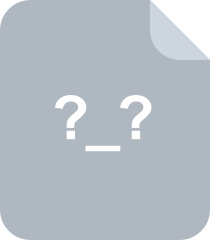
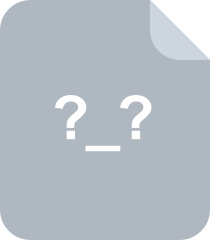
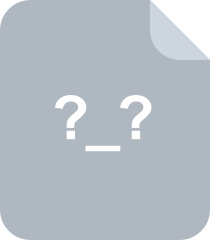
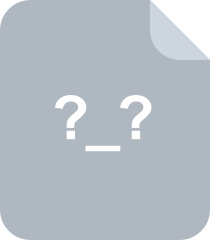
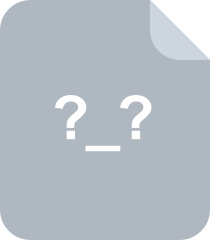
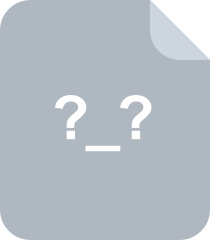
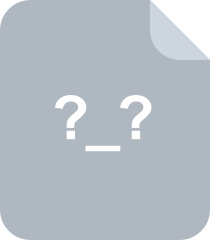
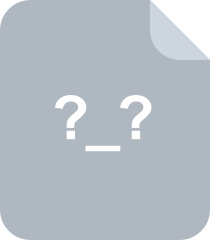
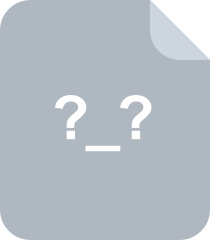
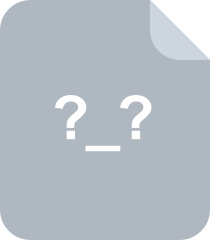
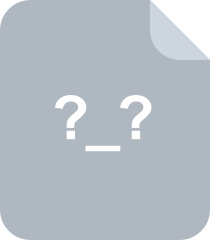
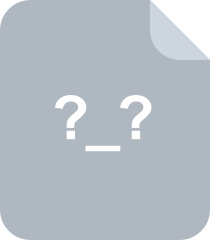
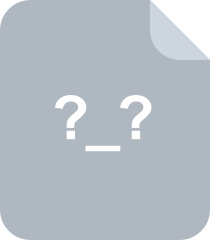
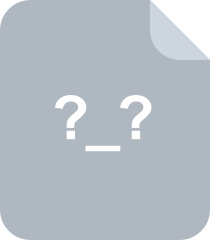
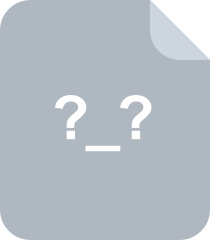
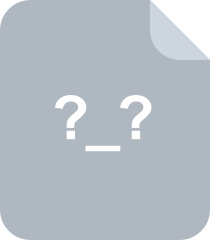
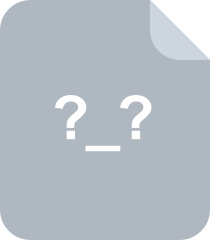
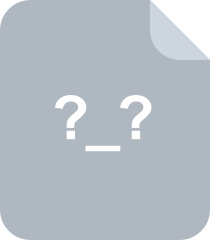
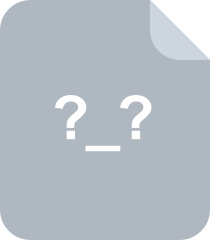
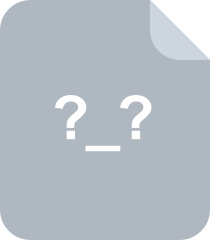
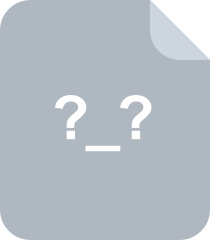
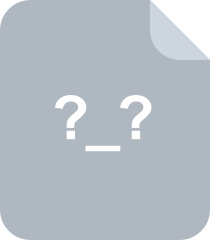
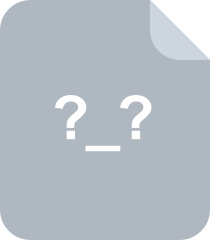
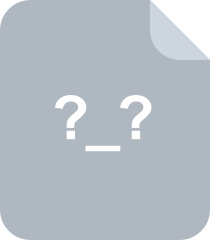
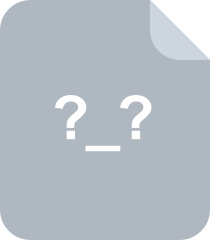
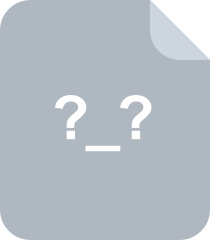
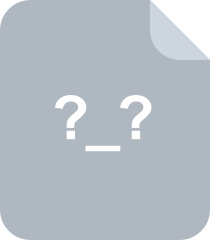
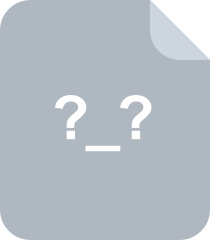
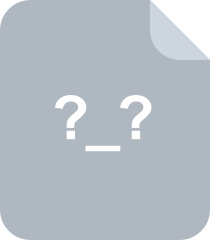
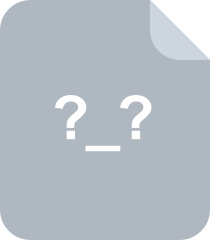
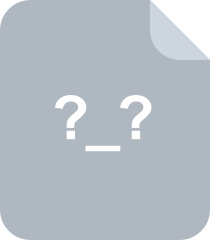
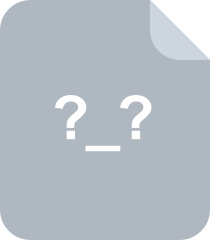
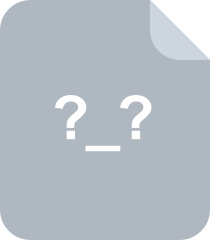
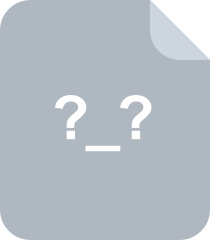
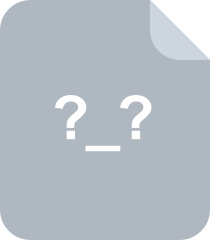
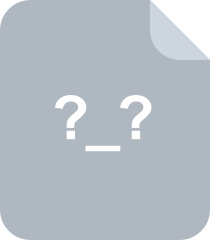
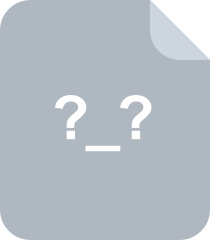
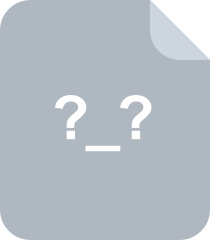
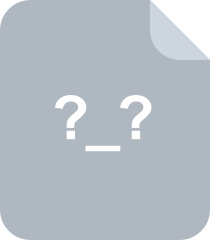
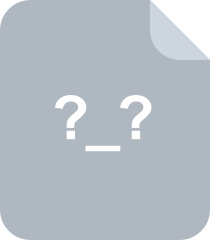
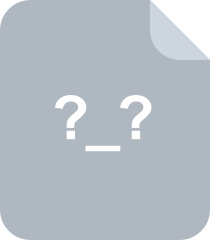
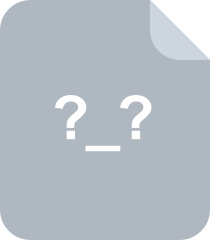
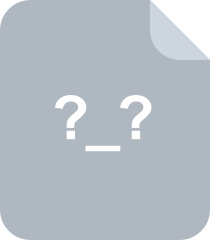
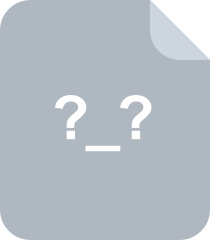
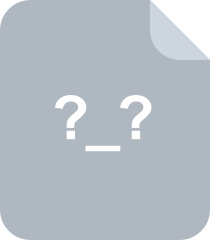
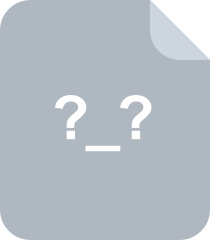
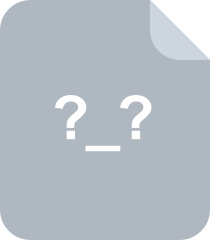
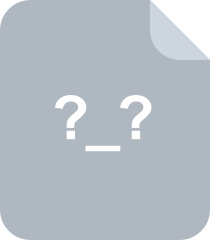
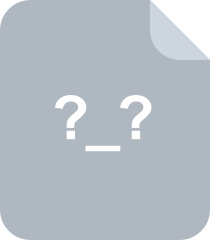
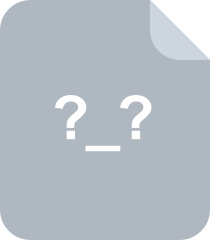
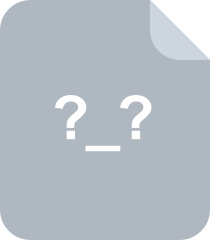
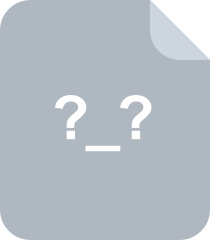
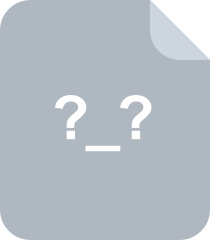
共 1667 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
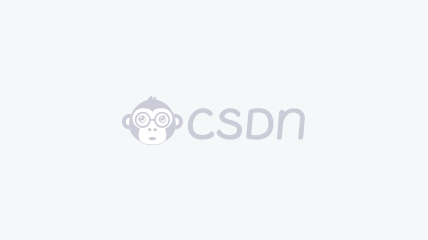

从入门到放弃-咖啡豆
- 粉丝: 29
- 资源: 16
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

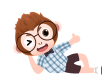
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


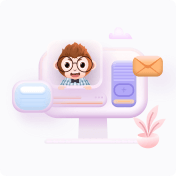
安全验证
文档复制为VIP权益,开通VIP直接复制
