# generate tengine header file
FILE (MAKE_DIRECTORY ${CMAKE_CURRENT_BINARY_DIR}/tengine)
FILE (COPY ${CMAKE_SOURCE_DIR}/source/api/c_api.h DESTINATION ${CMAKE_CURRENT_BINARY_DIR}/tengine)
FILE (COPY ${CMAKE_SOURCE_DIR}/source/api/c_api_ex.h DESTINATION ${CMAKE_CURRENT_BINARY_DIR}/tengine)
if (TENGINE_ENABLE_OPENDLA)
function (tengine_opendla_op_test name file)
file(GLOB TENGINE_UTIL_SOURCE_FILES ${PROJECT_SOURCE_DIR}/tests/common/util/*.c)
add_executable (${name} ${CMAKE_CURRENT_SOURCE_DIR}/${file} "${TENGINE_UTIL_SOURCE_FILES}" "${PROJECT_SOURCE_DIR}/tests/common/tengine_operations.c")
target_link_libraries (${name} PRIVATE ${CMAKE_PROJECT_NAME})
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/source")
target_include_directories (${name} PRIVATE "${CMAKE_CURRENT_BINARY_DIR}")
target_include_directories (${name} PRIVATE "${PROJECT_BINARY_DIR}")
target_include_directories (${name} PRIVATE "${PROJECT_BINARY_DIR}/source")
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/tests/common")
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/tests/common/util")
if (${TENGINE_TARGET_PROCESSOR} MATCHES "ARM" AND (NOT ANDROID AND NOT OHOS) AND TENGINE_TARGET_PROCESSOR_32Bit)
target_compile_options (${name} PRIVATE "-mfp16-format=ieee")
endif()
add_test (${name} ${name})
# add to a virtual project group
SET_PROPERTY(TARGET ${name} PROPERTY FOLDER "tests/test_opendla")
endfunction()
tengine_opendla_op_test(test_opendla_op_concat op/test_opendla_op_concat.cpp)
tengine_opendla_op_test(test_opendla_op_convolution op/test_opendla_op_convolution.cpp)
tengine_opendla_op_test(test_opendla_op_deconv op/test_opendla_op_deconv.cpp)
tengine_opendla_op_test(test_opendla_op_groupconvolution op/test_opendla_op_groupconvolution.cpp)
tengine_opendla_op_test(test_opendla_op_eltwise op/test_opendla_op_eltwise.cpp)
tengine_opendla_op_test(test_opendla_op_fc op/test_opendla_op_fc.cpp)
tengine_opendla_op_test(test_opendla_op_pooling op/test_opendla_op_pooling.cpp)
tengine_opendla_op_test(test_opendla_op_relu op/test_opendla_op_relu.cpp)
tengine_opendla_op_test(test_opendla_op_split op/test_opendla_op_split.cpp)
endif()
if (TENGINE_ENABLE_TIM_VX)
function (tengine_timvx_op_test name file)
file(GLOB TENGINE_UTIL_SOURCE_FILES ${PROJECT_SOURCE_DIR}/tests/common/util/*.c)
add_executable (${name} ${CMAKE_CURRENT_SOURCE_DIR}/${file} "${TENGINE_UTIL_SOURCE_FILES}" "${PROJECT_SOURCE_DIR}/tests/common/tengine_operations.c")
target_link_libraries (${name} PRIVATE ${CMAKE_PROJECT_NAME})
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/source")
target_include_directories (${name} PRIVATE "${CMAKE_CURRENT_BINARY_DIR}")
target_include_directories (${name} PRIVATE "${PROJECT_BINARY_DIR}")
target_include_directories (${name} PRIVATE "${PROJECT_BINARY_DIR}/source")
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/tests/common")
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/tests/common/util")
if (${TENGINE_TARGET_PROCESSOR} MATCHES "ARM" AND (NOT ANDROID AND NOT OHOS) AND TENGINE_TARGET_PROCESSOR_32Bit)
target_compile_options (${name} PRIVATE "-mfp16-format=ieee")
endif()
add_test (${name} ${name})
# add to a virtual project group
SET_PROPERTY(TARGET ${name} PROPERTY FOLDER "tests/test_timvx")
endfunction()
tengine_timvx_op_test(test_timvx_op_clip op/test_timvx_op_clip.cpp)
tengine_timvx_op_test(test_timvx_op_concat op/test_timvx_op_concat.cpp)
tengine_timvx_op_test(test_timvx_op_convolution op/test_timvx_op_convolution.cpp)
tengine_timvx_op_test(test_timvx_op_crop op/test_timvx_op_crop.cpp)
tengine_timvx_op_test(test_timvx_op_deconv op/test_timvx_op_deconv.cpp)
tengine_timvx_op_test(test_timvx_op_dropout op/test_timvx_op_dropout.cpp)
tengine_timvx_op_test(test_timvx_op_eltwise_mul op/test_timvx_op_eltwise_mul.cpp)
tengine_timvx_op_test(test_timvx_op_eltwise_sum op/test_timvx_op_eltwise_sum.cpp)
tengine_timvx_op_test(test_timvx_op_elu op/test_timvx_op_elu.cpp)
tengine_timvx_op_test(test_timvx_op_fc op/test_timvx_op_fc.cpp)
tengine_timvx_op_test(test_timvx_op_flatten op/test_timvx_op_flatten.cpp)
tengine_timvx_op_test(test_timvx_op_gather op/test_timvx_op_gather.cpp)
tengine_timvx_op_test(test_timvx_op_hardswish op/test_timvx_op_hardswish.cpp)
tengine_timvx_op_test(test_timvx_op_interp op/test_timvx_op_interp.cpp)
tengine_timvx_op_test(test_timvx_op_leakyrelu op/test_timvx_op_leakyrelu.cpp)
tengine_timvx_op_test(test_timvx_op_mish op/test_timvx_op_mish.cpp)
tengine_timvx_op_test(test_timvx_op_pad op/test_timvx_op_pad.cpp)
tengine_timvx_op_test(test_timvx_op_permute op/test_timvx_op_permute.cpp)
tengine_timvx_op_test(test_timvx_op_pooling op/test_timvx_op_pooling.cpp)
tengine_timvx_op_test(test_timvx_op_prelu op/test_timvx_op_prelu.cpp)
tengine_timvx_op_test(test_timvx_op_reduction op/test_timvx_op_reduction.cpp)
tengine_timvx_op_test(test_timvx_op_relu op/test_timvx_op_relu.cpp)
# tengine_timvx_op_test(test_timvx_op_relu1 op/test_timvx_op_relu1.cpp)
tengine_timvx_op_test(test_timvx_op_reshape op/test_timvx_op_reshape.cpp)
tengine_timvx_op_test(test_timvx_op_resize op/test_timvx_op_resize.cpp)
tengine_timvx_op_test(test_timvx_op_sigmoid op/test_timvx_op_sigmoid.cpp)
tengine_timvx_op_test(test_timvx_op_slice op/test_timvx_op_slice.cpp)
tengine_timvx_op_test(test_timvx_op_softmax op/test_timvx_op_softmax.cpp)
tengine_timvx_op_test(test_timvx_op_split op/test_timvx_op_split.cpp)
tengine_timvx_op_test(test_timvx_op_tanh op/test_timvx_op_tanh.cpp)
tengine_timvx_op_test(test_timvx_op_transpose op/test_timvx_op_transpose.cpp)
tengine_timvx_op_test(test_timvx_op_upsampling op/test_timvx_op_upsampling.cpp)
# timvx model test, need opencv
FIND_PACKAGE(OpenCV QUIET)
IF (OpenCV_FOUND)
# macro for adding examples
FUNCTION (TENGINE_TIMVX_MODEL_TEST name file)
ADD_EXECUTABLE (${name} "${CMAKE_CURRENT_SOURCE_DIR}/${file}" "${CMAKE_CURRENT_SOURCE_DIR}/common/tengine_operations.c")
TARGET_INCLUDE_DIRECTORIES (${name} PRIVATE "${CMAKE_CURRENT_SOURCE_DIR}")
TARGET_INCLUDE_DIRECTORIES (${name} PRIVATE "${CMAKE_CURRENT_BINARY_DIR}")
TARGET_INCLUDE_DIRECTORIES (${name} PRIVATE "${PROJECT_SOURCE_DIR}/tests/common")
TARGET_LINK_LIBRARIES (${name} ${CMAKE_PROJECT_NAME} ${OpenCV_LIBS})
INSTALL (TARGETS ${name} DESTINATION bin)
ENDFUNCTION()
TENGINE_TIMVX_MODEL_TEST (test_timvx_model_yolov5s models/test_timvx_model_yolov5s.cpp)
ELSE()
MESSAGE (WARNING "OpenCV not found, some examples won't be built")
ENDIF()
endif()
if (TENGINE_ENABLE_TENSORRT)
function (tengine_tensorrt_op_test name file)
file(GLOB TENGINE_UTIL_SOURCE_FILES ${PROJECT_SOURCE_DIR}/tests/common/util/*.c)
add_executable (${name} ${CMAKE_CURRENT_SOURCE_DIR}/${file} "${TENGINE_UTIL_SOURCE_FILES}" "${PROJECT_SOURCE_DIR}/tests/common/tengine_operations.c")
target_link_libraries (${name} PRIVATE ${CMAKE_PROJECT_NAME})
target_include_directories (${name} PRIVATE "${PROJECT_SOURCE_DIR}/source")
target_include_directories (${name} PRIVATE "${CMA
没有合适的资源?快使用搜索试试~ 我知道了~
Tengine-tensorflow安装
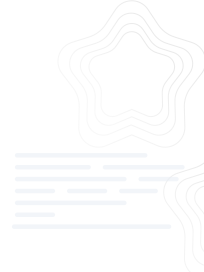
共1760个文件
c:460个
cpp:260个
h:244个

需积分: 1 0 下载量 122 浏览量
2024-11-08
22:08:02
上传
评论
收藏 16.74MB ZIP 举报
温馨提示
Tengine is a lite, high performance, modular inference engine for embedded device tensorflow安装 tensorflow安装 tensorflow安装 tensorflow安装 tensorflow安装
资源推荐
资源详情
资源评论
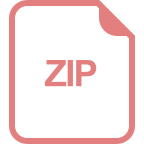
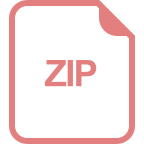
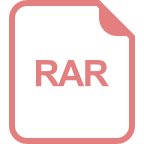
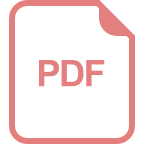
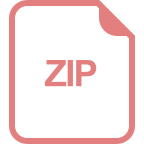
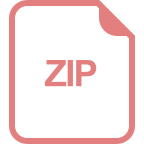
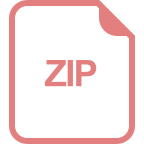
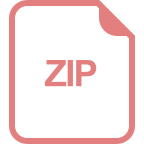
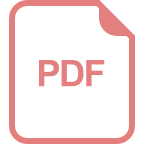
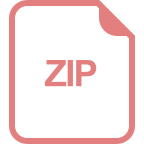
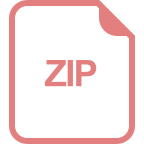
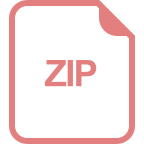
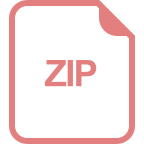
收起资源包目录

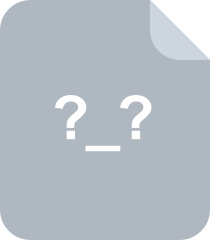
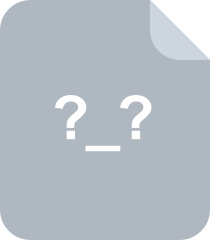
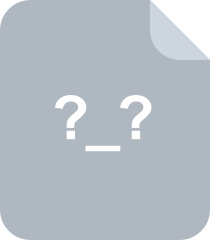
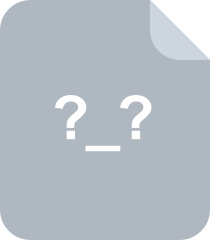
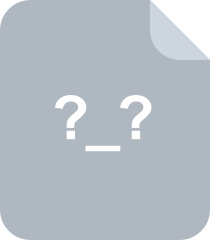
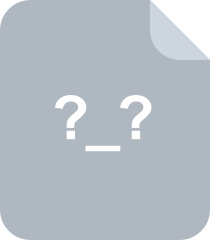
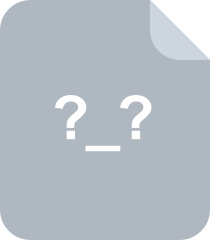
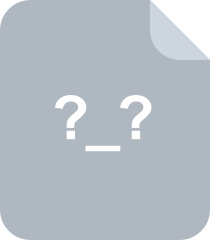
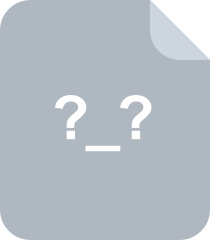
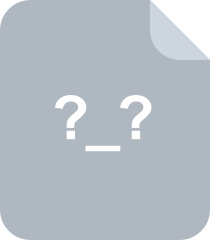
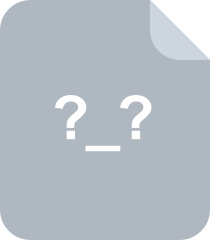
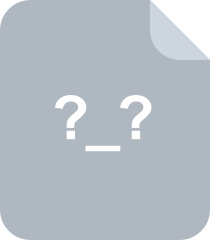
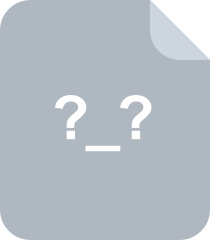
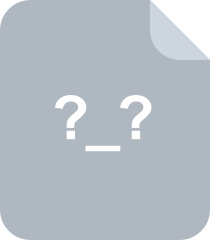
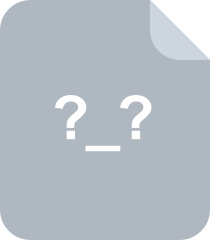
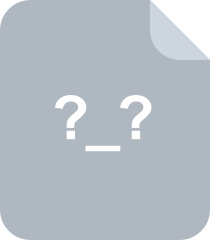
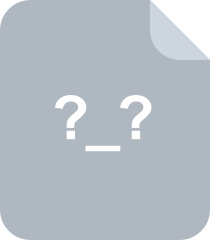
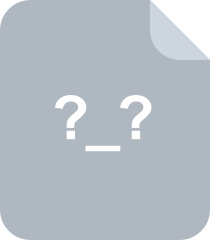
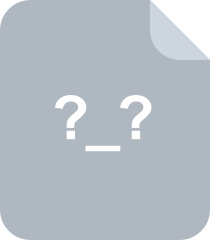
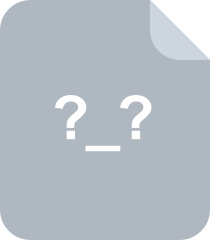
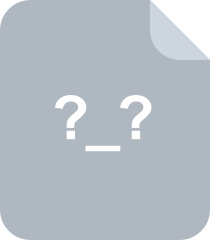
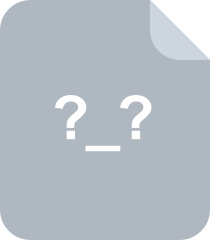
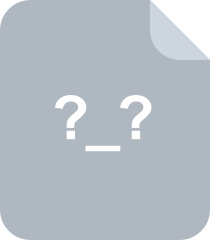
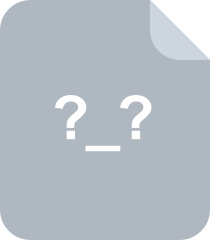
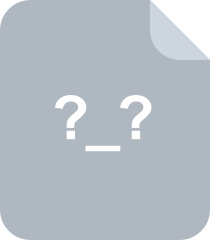
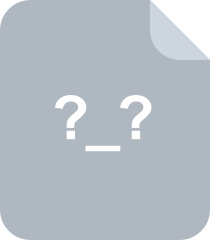
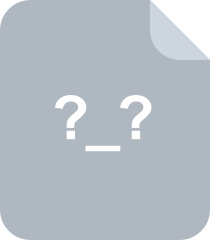
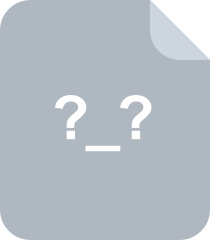
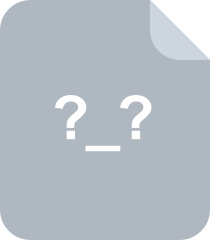
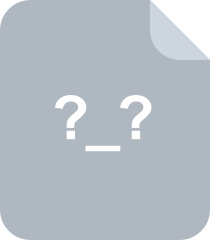
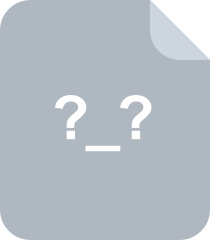
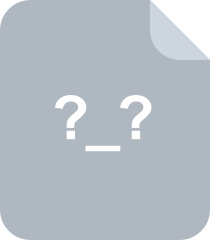
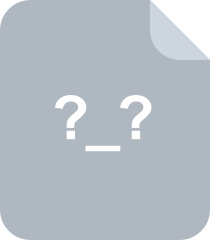
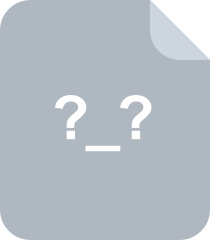
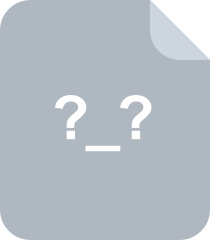
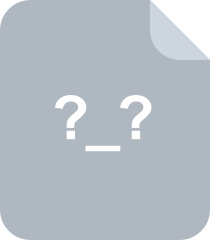
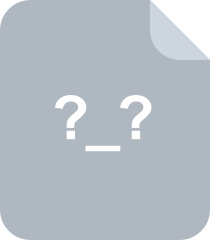
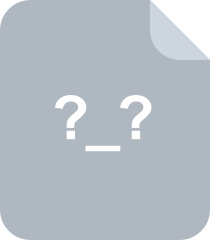
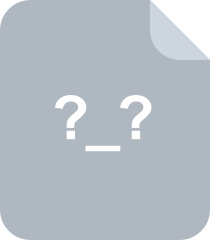
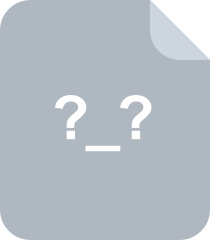
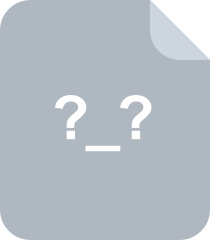
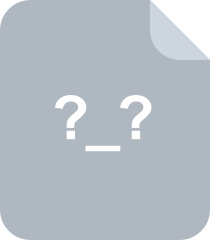
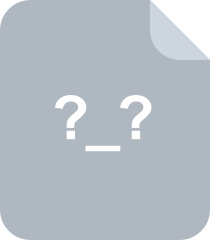
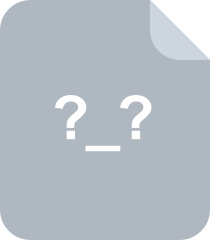
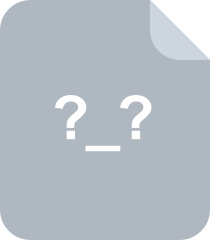
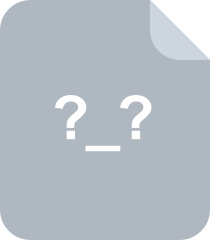
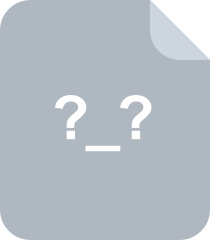
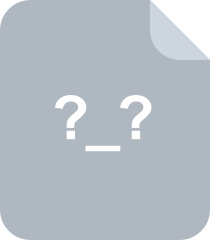
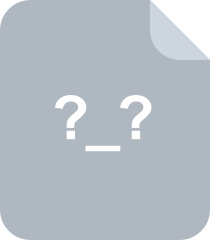
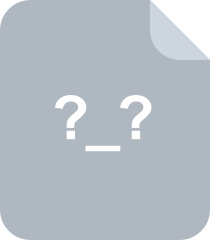
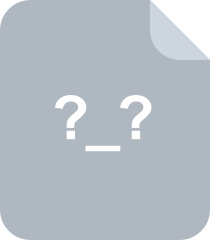
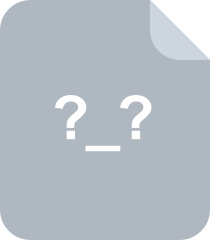
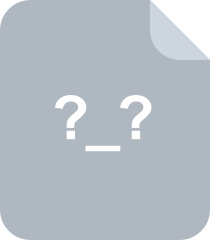
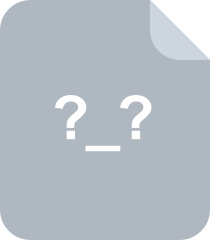
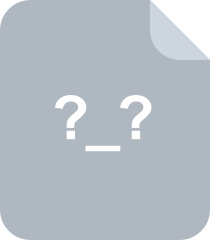
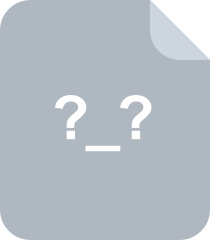
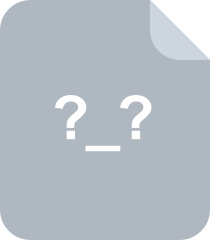
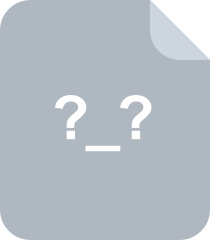
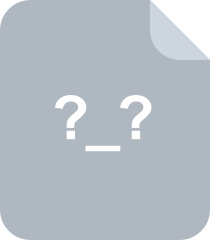
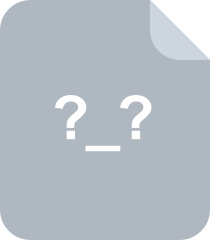
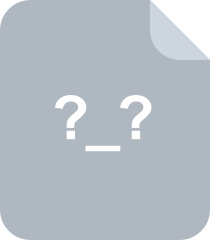
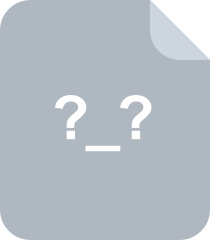
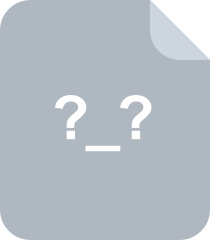
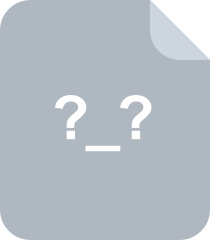
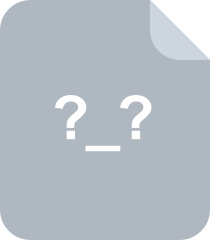
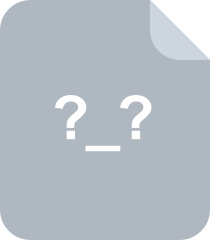
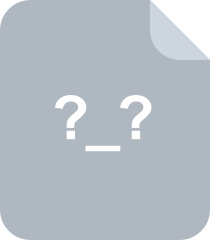
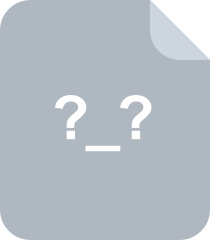
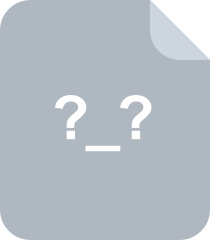
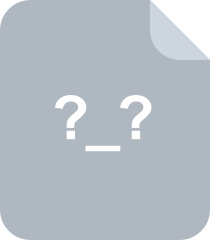
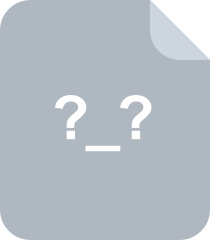
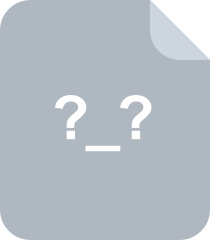
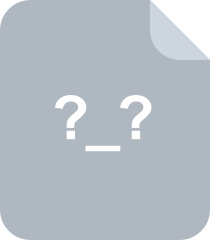
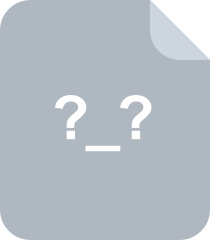
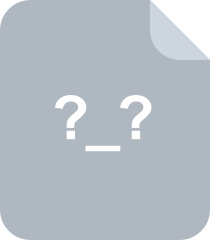
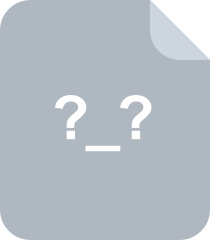
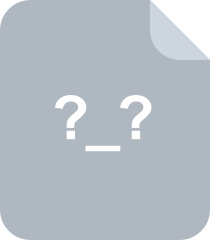
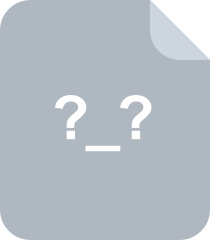
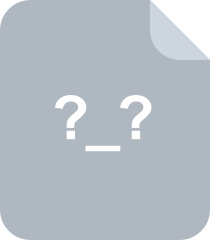
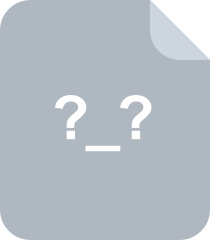
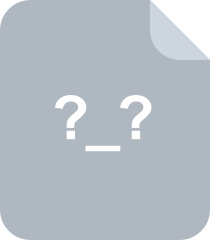
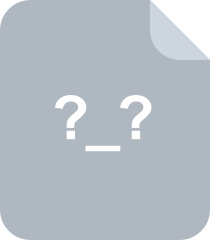
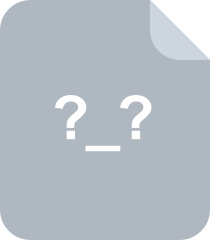
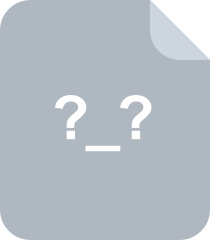
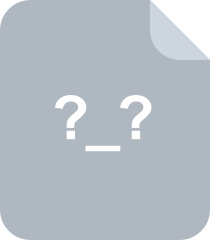
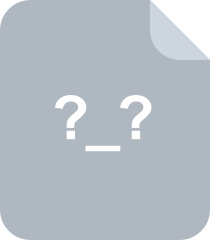
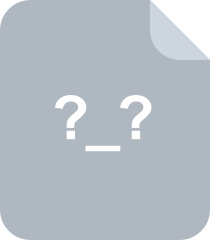
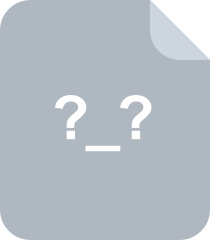
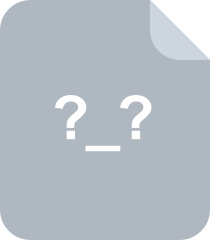
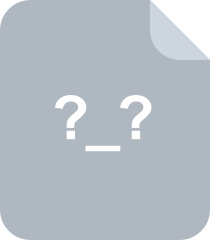
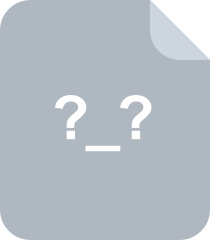
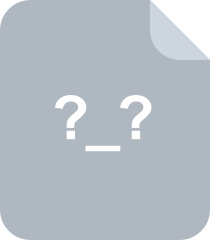
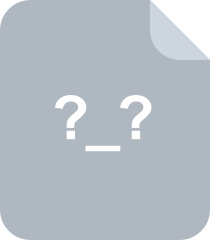
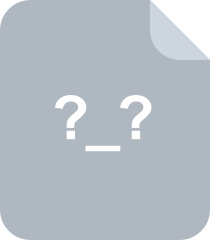
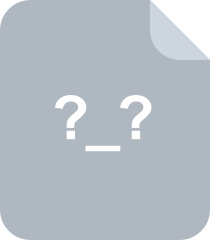
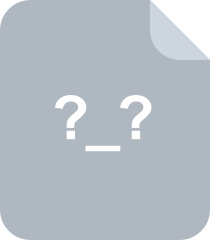
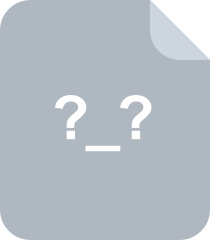
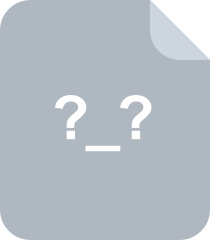
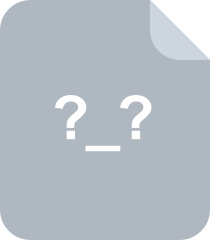
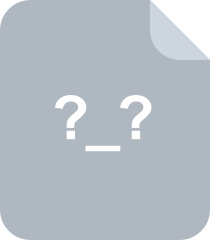
共 1760 条
- 1
- 2
- 3
- 4
- 5
- 6
- 18
资源评论
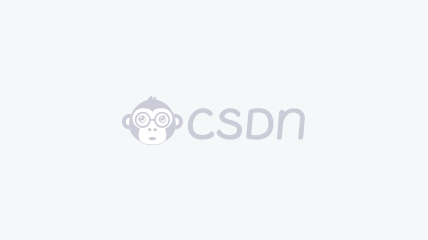
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

lly202406
- 粉丝: 3163
- 资源: 5551
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

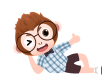
最新资源
- 四旋翼飞行器多场景轨迹跟踪仿真 包含多种地图 路径规划与轨迹 最小加速度轨迹 最小捕捉轨迹 四旋翼无人机
- use-effect patch
- android compose TCP通信
- ZenIdentityServer4 webapi + 认证+web
- 综合交通运输系统PDF(1).zip
- PyCharm+根据甄嬛传中人物的眼睛进行人物识别
- html+css+js网页设计 体育 腾讯体育7个页面
- 大模型Llama架构:从理论到实战
- xdu 电院题目,模拟电子技术,数字电路
- 洞见研报2024化妆品包装行业白皮书(化妆品、护肤品、美妆、包装)
- Pokemon 图像数据集(26K+ 样本,1K类别)PNG+CSV
- 使用D3.js绘制雷达图
- 河北省2024年县级地图-标准shape文件+mxd文件+Tif图片
- 基于Fano共振的SPP传感器
- 机械设计光纤镭雕机(sw22可编辑+工程图)全套技术资料100%好用.zip
- comsol静态相位法计算GH相移
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


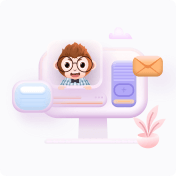
安全验证
文档复制为VIP权益,开通VIP直接复制
