# segyio #
[](https://travis-ci.org/equinor/segyio)
[](https://ci.appveyor.com/project/statoil-travis/segyio)
[](https://pyup.io/repos/github/equinor/segyio/)
[](https://pyup.io/repos/github/equinor/segyio/)
[readthedocs](https://segyio.readthedocs.io/)
## Index ##
* [Introduction](#introduction)
* [Feature summary](#feature-summary)
* [Getting started](#getting-started)
* [Quick start](#quick-start)
* [Get segyio](#get-segyio)
* [Build segyio](#build-segyio)
* [Tutorial](#tutorial)
* [Basics](#basics)
* [Modes](#modes)
* [Mode examples](#mode-examples)
* [Goals](#project-goals)
* [Contributing](#contributing)
* [Examples](#examples)
* [Common issues](#common-issues)
* [History](#history)
## Introduction ##
Segyio is a small LGPL licensed C library for easy interaction with SEG-Y and
Seismic Unix formatted seismic data, with language bindings for Python and
Matlab. Segyio is an attempt to create an easy-to-use, embeddable,
community-oriented library for seismic applications. Features are added as they
are needed; suggestions and contributions of all kinds are very welcome.
To catch up on the latest development and features, see the
[changelog](changelog.md). To write future proof code, consult the planned
[breaking changes](breaking-changes.md).
## Feature summary ##
* A low-level C interface with few assumptions; easy to bind to other
languages
* Read and write binary and textual headers
* Read and write traces and trace headers
* Simple, powerful, and native-feeling Python interface with numpy
integration
* Read and write seismic unix files
* xarray integration with netcdf_segy
* Some simple applications with unix philosophy
## Getting started ##
When segyio is built and installed, you're ready to start programming! Check
out the [tutorial](#tutorial), [examples](#examples), [example
programs](python/examples), and [example
notebooks](https://github.com/equinor/segyio-notebooks). For a technical
reference with examples and small recipes, [read the
docs](https://segyio.readthedocs.io/). API docs are also available with pydoc -
start your favourite Python interpreter and type `help(segyio)`, which should
integrate well with IDLE, pycharm and other Python tools.
### Quick start ###
```python
import segyio
import numpy as np
with segyio.open('file.sgy') as f:
for trace in f.trace:
filtered = trace[np.where(trace < 1e-2)]
```
See the [examples](#examples) for more.
### Get segyio ###
A copy of segyio is available both as pre-built binaries and source code:
* In Debian [unstable](https://packages.debian.org/source/sid/segyio)
* `apt install python3-segyio`
* Wheels for Python from [PyPI](https://pypi.python.org/pypi/segyio/)
* `pip install segyio`
* Source code from [github](https://github.com/equinor/segyio)
* `git clone https://github.com/statoil/segyio`
* Source code in [tarballs](https://github.com/equinor/segyio/releases)
### Build segyio ###
To build segyio you need:
* A C99 compatible C compiler (tested mostly on gcc and clang)
* A C++ compiler for the Python extension, and C++11 for the tests
* [CMake](https://cmake.org/) version 2.8.12 or greater
* [Python](https://www.python.org/) 2.7 or 3.x.
* [numpy](http://www.numpy.org/) version 1.10 or greater
* [setuptools](https://pypi.python.org/pypi/setuptools) version 28 or greater
* [setuptools-scm](https://pypi.python.org/pypi/setuptools_scm)
* [pytest](https://pypi.org/project/pytest)
To build the documentation, you also need
[sphinx](https://pypi.org/project/Sphinx)
To build and install segyio, perform the following actions in your console:
```bash
git clone https://github.com/equinor/segyio
mkdir segyio/build
cd segyio/build
cmake .. -DCMAKE_BUILD_TYPE=Release -DBUILD_SHARED_LIBS=ON
make
make install
```
`make install` must be done as root for a system install; if you want to
install in your home directory, add `-DCMAKE_INSTALL_PREFIX=~/` or some other
appropriate directory, or `make DESTDIR=~/ install`. Please ensure your
environment picks up on non-standard install locations (PYTHONPATH,
LD_LIBRARY_PATH and PATH).
If you have multiple Python installations, or want to use some alternative
interpreter, you can help cmake find the right one by passing
`-DPYTHON_EXECUTABLE=/opt/python/binary` along with install prefix and build
type.
To build the matlab bindings, invoke CMake with the option `-DBUILD_MEX=ON`. In
some environments the Matlab binaries are in a non-standard location, in which
case you need to help CMake find the matlab binaries by passing
`-DMATLAB_ROOT=/path/to/matlab`.
#### Developers ####
It's recommended to build in debug mode to get more warnings and to embed debug
symbols in the objects. Substituting `Debug` for `Release` in the
`CMAKE_BUILD_TYPE` is plenty.
Tests are located in the language/tests directories, and it's highly
recommended that new features added are demonstrated for correctness and
contract by adding a test. All tests can be run by invoking `ctest`. Feel free
to use the tests already written as a guide.
After building segyio you can run the tests with `ctest`, executed from the
build directory.
Please note that to run the Python examples you need to let your environment
know where to find the Python library. It can be installed as a user, or on
adding the segyio/build/python library to your pythonpath.
## Tutorial ##
All code in this tutorial assumes segyio is imported, and that numpy is
available as np.
```python
import segyio
import numpy as np
```
This tutorial assumes you're familiar with Python and numpy. For a refresh,
check out the [python tutorial](https://docs.python.org/3/tutorial/) and [numpy
quickstart](https://docs.scipy.org/doc/numpy-dev/user/quickstart.html)
### Basics ###
Opening a file for reading is done with the `segyio.open` function, and
idiomatically used with context managers. Using the `with` statement, files are
properly closed even in the case of exceptions. By default, files are opened
read-only.
```python
with segyio.open(filename) as f:
...
```
Open accepts several options (for more a more comprehensive reference, check
the open function's docstring with `help(segyio.open)`. The most important
option is the second (optional) positional argument. To open a file for
writing, do `segyio.open(filename, 'r+')`, from the C `fopen` function.
Files can be opened in *unstructured* mode, either by passing `segyio.open` the
optional arguments `strict=False`, in which case not establishing structure
(inline numbers, crossline numbers etc.) is not an error, and
`ignore_geometry=True`, in which case segyio won't even try to set these
internal attributes.
The segy file object has several public attributes describing this structure:
* `f.ilines`
Inferred inline numbers
* `f.xlines`
Inferred crossline numbers
* `f.offsets`
Inferred offsets numbers
* `f.samples`
Inferred sample offsets (frequency and recording time delay)
* `f.unstructured`
True if unstructured, False if structured
* `f.ext_headers`
The number of extended textual headers
If the file is opened *unstructured*, all the line properties will will be
`None`.
### Modes ###
In segyio, data is retrieved and written through so-called *modes*. Modes are
abstract arrays, or addressing schemes, and change what names and indices mean.
All modes are properties on the file handle object, support the `len` function,
and reads and writes are done through `f.mode[]`. Writes are done with
assignment. Modes support array slicing inspired by numpy. The following modes
are available:
* `trace`
The trace mode offers raw addressing of traces as they are
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
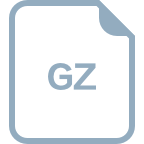
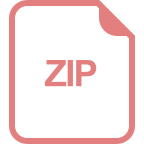
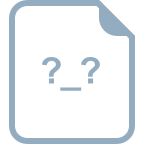
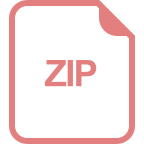
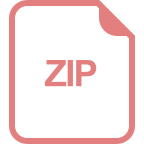
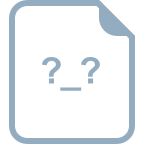
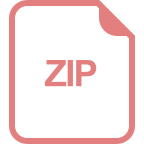
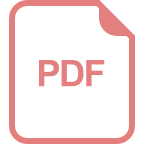
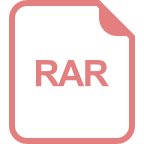
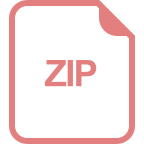
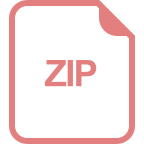
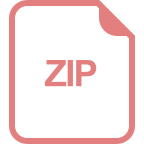
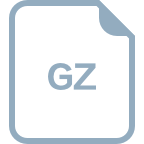
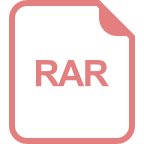
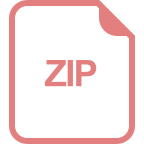
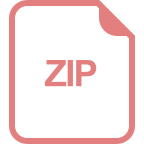
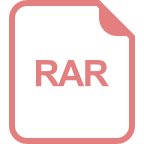
收起资源包目录

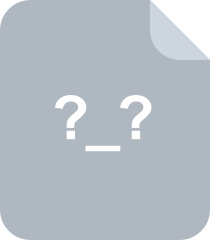
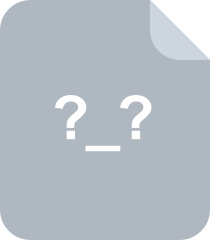
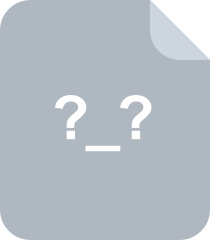
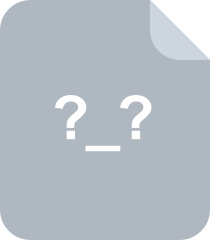
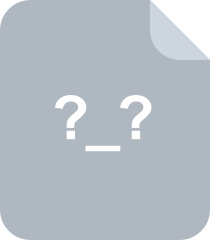
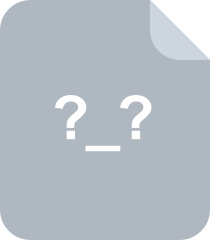
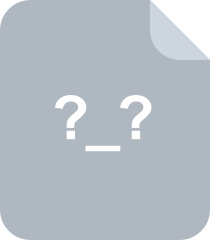
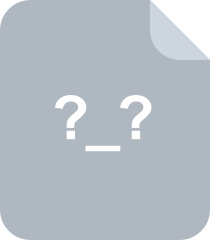
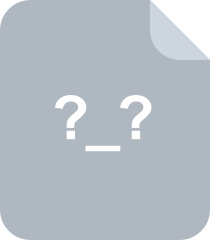
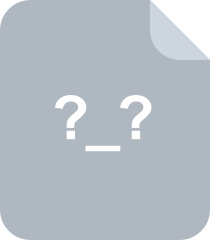
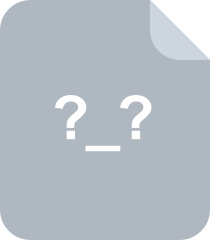
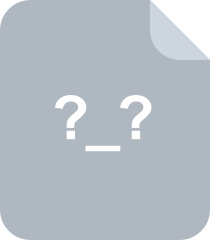
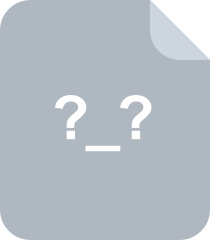
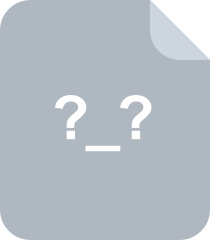
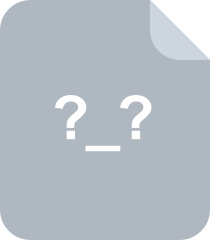
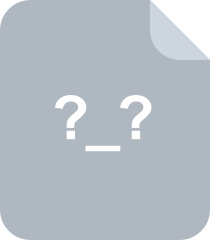
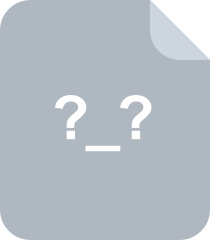
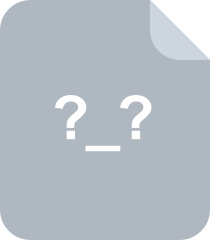
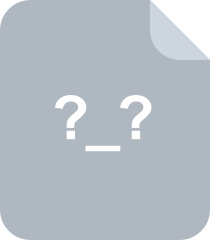
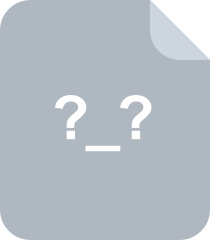
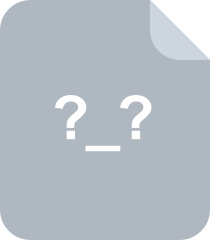
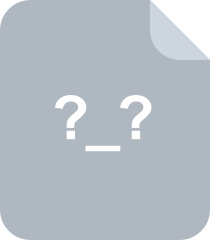
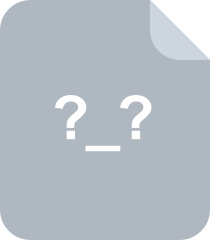
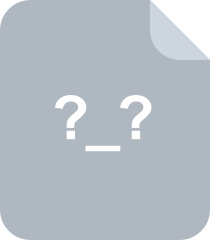
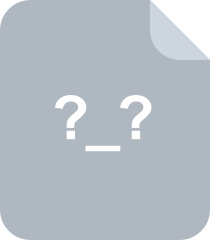
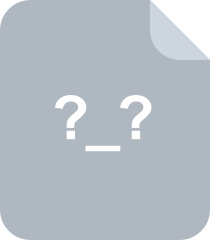
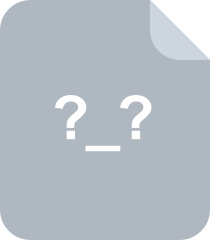
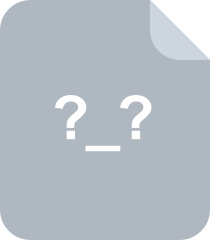
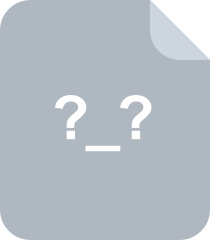
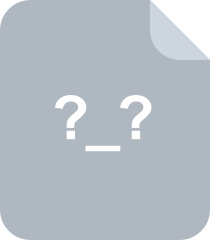
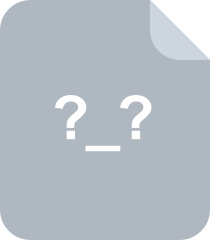
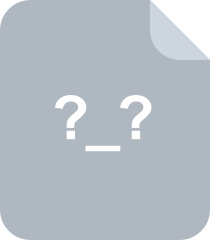
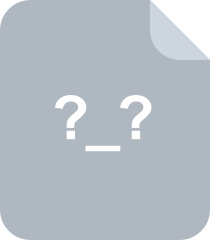
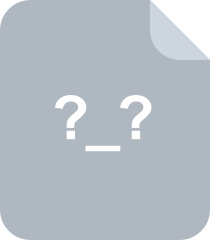
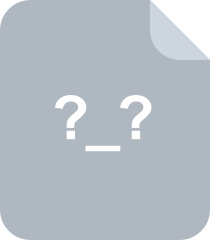
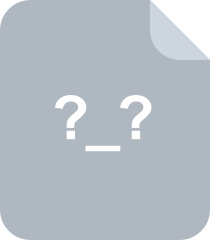
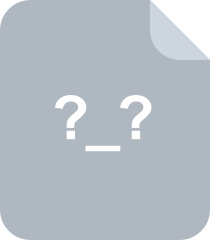
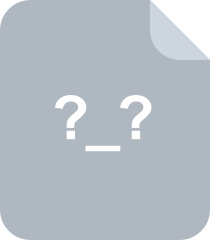
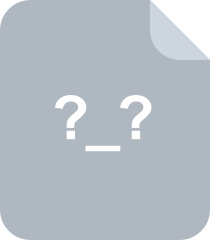
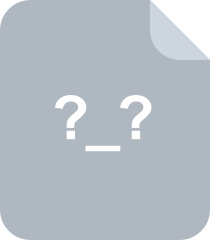
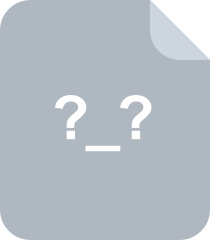
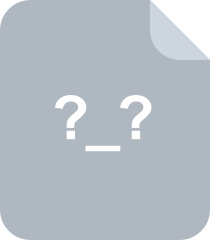
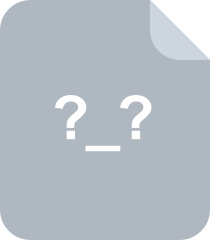
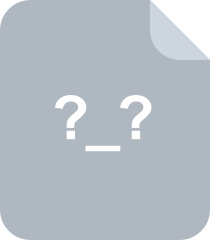
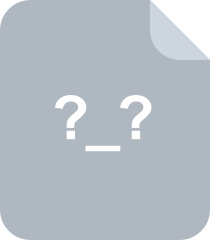
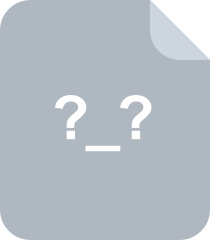
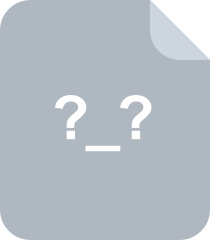
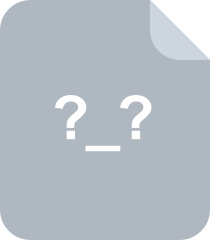
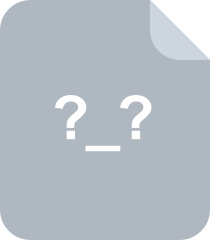
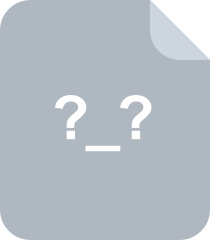
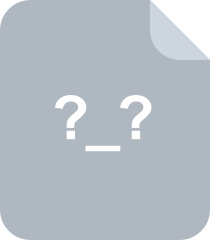
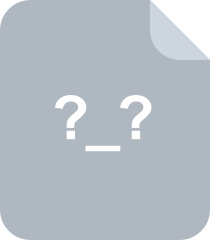
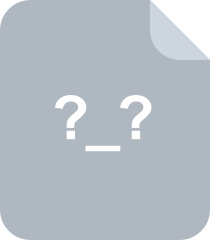
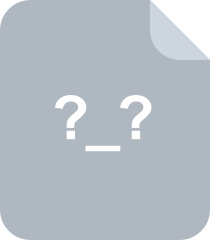
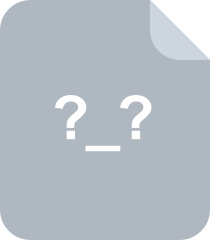
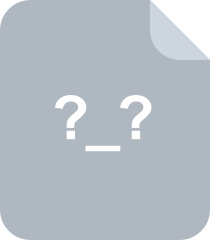
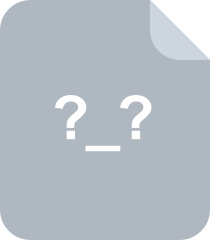
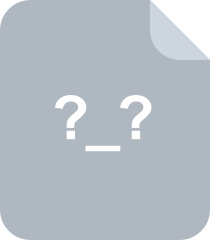
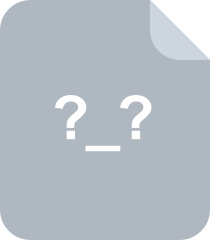
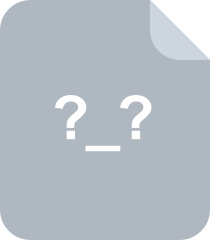
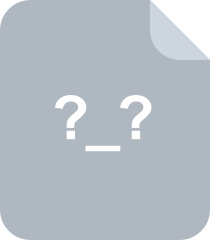
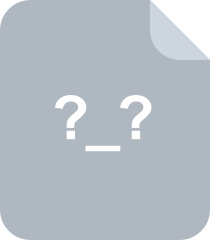
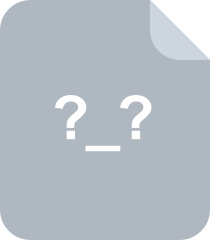
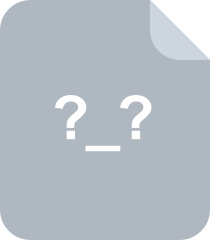
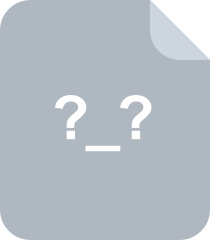
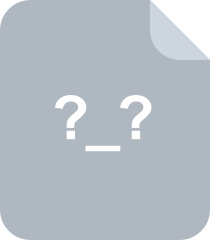
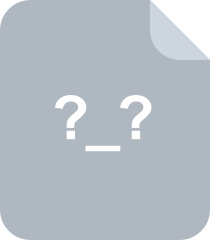
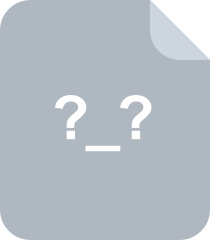
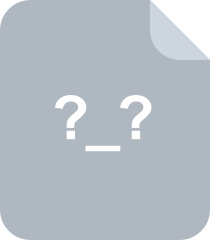
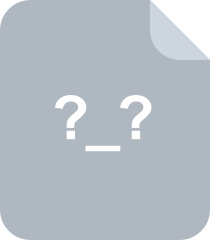
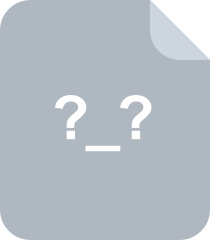
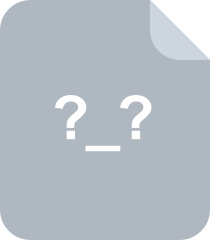
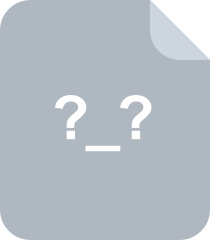
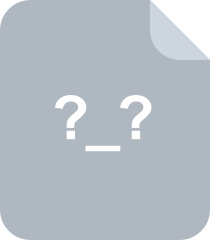
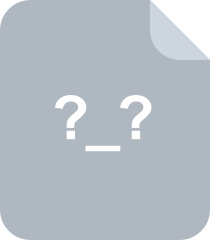
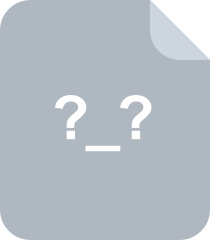
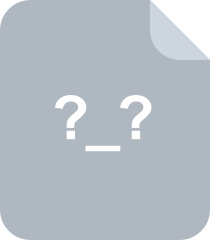
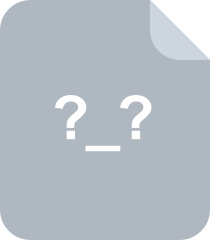
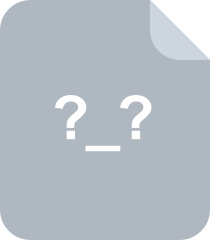
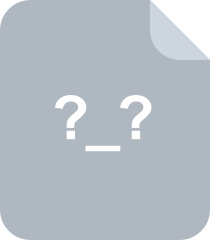
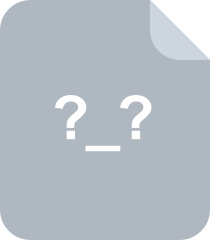
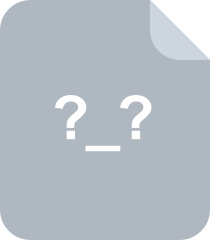
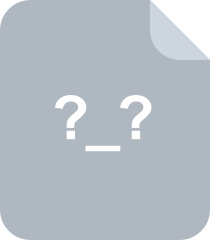
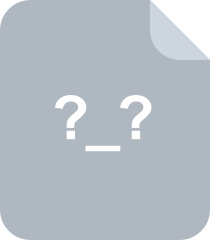
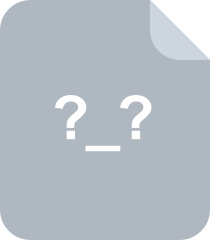
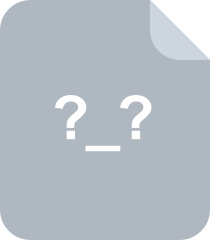
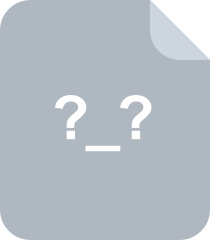
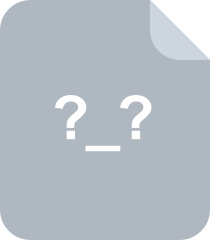
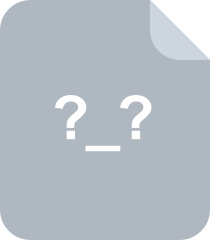
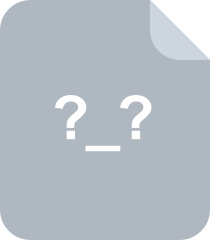
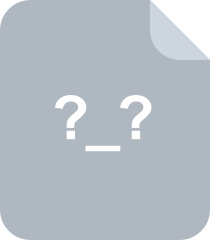
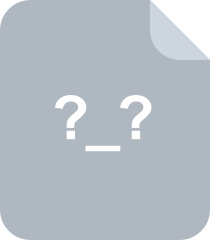
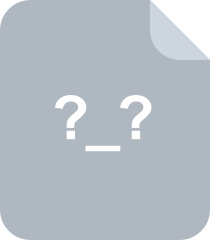
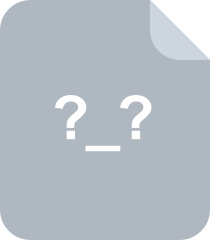
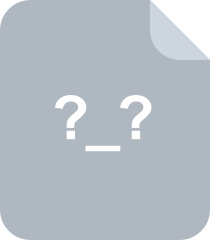
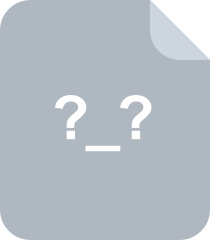
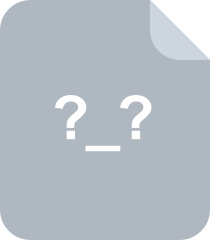
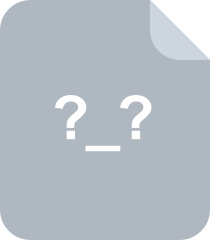
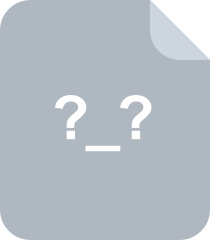
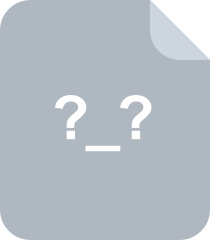
共 182 条
- 1
- 2
资源评论
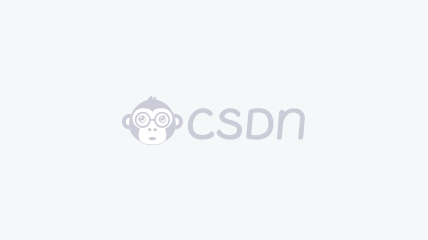

weixin_38744435
- 粉丝: 373
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

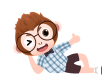
最新资源
- TestBank.java
- js-leetcode题解之146-lru-cache.js
- js-leetcode题解之145-binary-tree-postorder-traversal.js
- js-leetcode题解之144-binary-tree-preorder-traversal.js
- js-leetcode题解之143-reorder-list.js
- js-leetcode题解之142-linked-list-cycle-ii.js
- js-leetcode题解之141-linked-list-cycle.js
- js-leetcode题解之140-word-break-ii.js
- js-leetcode题解之139-word-break.js
- js-leetcode题解之138-copy-list-with-random-pointer.js
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


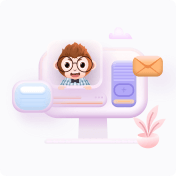
安全验证
文档复制为VIP权益,开通VIP直接复制
