python中requests小技巧
Requests 使用的是 urllib3,因此继承了它的所有特性。Requests 支持 HTTP 连接保持和连接池,支持使用 cookie 保持会话,支持文件上传,支持自动确定响应内容的编码,支持国际化的 URL 和 POST 数据自动编码。现代、国际化、人性化。 ### Python中requests模块的小技巧详解 #### 概述 `requests`是Python中非常流行的用于发送HTTP请求的库。它不仅简洁易用,而且功能强大,支持诸如HTTP连接保持、连接池、Cookie会话管理、文件上传等功能。下面将详细介绍在实际开发中使用`requests`时的一些实用技巧。 #### 技巧1:保持请求之间的Cookies 在进行多次请求的过程中,往往需要保持Cookies的一致性来维持用户的登录状态或其他会话信息。通过`requests.Session()`可以轻松实现这一点: ```python import requests s = requests.Session() s.get('http://httpbin.org/cookies/set/sessioncookie/123456789') r = s.get("http://httpbin.org/cookies") print(r.text) ``` #### 技巧2:简化Headers设置 为了使请求更加符合实际应用场景,通常需要设置Headers。直接在每次请求中添加Headers会导致代码重复且不易维护: ```python headers = {'User-Agent': 'Mozilla/5.0'} response = requests.get('http://example.com', headers=headers) ``` 可以使用`requests.Session()`进一步简化此操作: ```python s = requests.Session() s.headers.update({'User-Agent': 'Mozilla/5.0'}) response = s.get('http://example.com') ``` #### 技巧3:配置重试机制 在网络不稳定的情况下,简单的失败重试机制可以提高程序的健壮性。利用`requests`的`Session`和`HTTPAdapter`可以轻松实现: ```python from requests.adapters import HTTPAdapter from requests.packages.urllib3.util.retry import Retry s = requests.Session() retries = Retry(total=3, backoff_factor=0.1, status_forcelist=[500, 502, 503, 504]) s.mount('http://', HTTPAdapter(max_retries=retries)) s.mount('https://', HTTPAdapter(max_retries=retries)) response = s.get('http://example.com') ``` #### 技巧4:禁用重定向 在某些场景下,可能需要禁止自动重定向,以确保请求行为的准确性: ```python response = requests.get('http://github.com', allow_redirects=False) print(response.status_code) # 输出301表示重定向 ``` #### 技巧5:简化JSON数据传输 当发送POST请求时,可以通过`requests`内置的JSON处理功能来简化数据传输: ```python import json data = {'key1': 'value1', 'key2': 'value2'} response = requests.post('http://httpbin.org/post', data=json.dumps(data)) # 简化为 response = requests.post('http://httpbin.org/post', json=data) ``` #### 技巧6:获取Debug信息 对于复杂的API调试,可以通过设置日志级别来获取详细的请求和响应信息: ```python import logging import http.client logging.basicConfig() logging.getLogger().setLevel(logging.DEBUG) requests_log = logging.getLogger("requests.packages.urllib3") requests_log.setLevel(logging.DEBUG) requests_log.propagate = True http.client.HTTPConnection.debuglevel = 1 response = requests.get('http://httpbin.org/get') print(response.text) ``` #### 技巧7:使用grequests实现异步请求 对于高并发场景,使用`grequests`库可以帮助我们实现异步请求: ```python import grequests urls = ['http://httpbin.org/get?a={}'.format(i) for i in range(10)] rs = (grequests.get(u) for u in urls) responses = grequests.map(rs) for response in responses: print(response.json()) ``` #### 技巧8:发送自定义Cookies 在特定情况下,可能需要发送自定义的Cookies: ```python cookies = dict(cookies_are='working') response = requests.get('http://httpbin.org/cookies', cookies=cookies) print(response.text) ``` #### 技巧9:使用Mock或Httpretty模拟API 在前端和后端并行开发时,可以通过模拟API来提前进行测试: ```python import httpretty import requests httpretty.enable() httpretty.register_uri(httpretty.GET, "http://httpbin.org/get", body="Success") response = requests.get('http://httpbin.org/get') print(response.text) httpretty.disable() ``` #### 技巧10:统计API请求时间 在性能测试或监控中,统计API请求时间非常重要: ```python import time start_time = time.time() response = requests.get('http://httpbin.org/get') elapsed_time = time.time() - start_time print(f"Request took {elapsed_time:.2f} seconds.") ``` #### 技巧11:设置请求超时 为了避免因长时间未响应而阻塞程序,可以通过设置超时来控制请求的最大等待时间: ```python try: response = requests.get('http://httpbin.org/delay/3', timeout=2) except requests.exceptions.Timeout: print("Request timed out.") else: print("Request completed successfully.") ``` 以上技巧涵盖了使用`requests`库进行高效网络通信的多个方面,希望能帮助开发者更好地利用这一强大的工具。
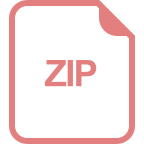
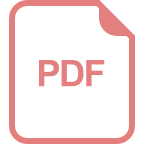
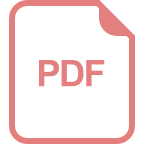
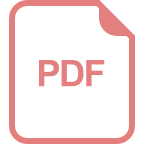
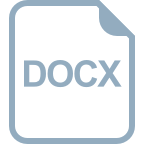
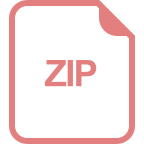
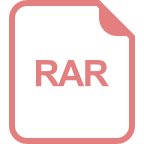
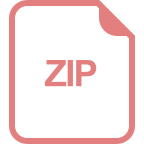
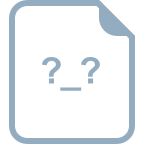
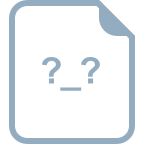
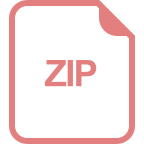
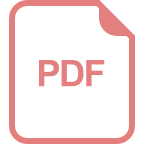
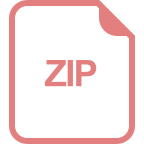
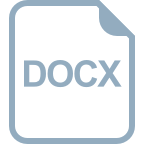
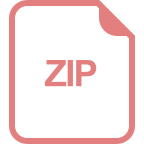
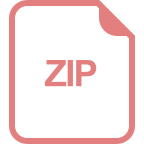
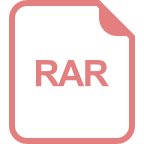
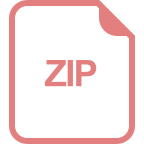
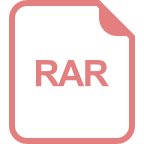
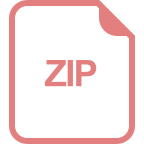
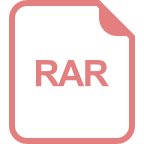
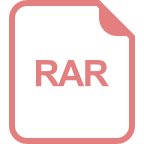
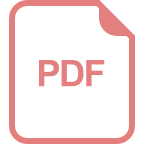
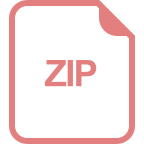
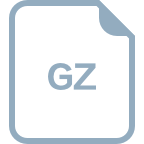
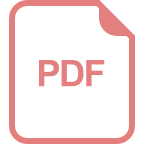
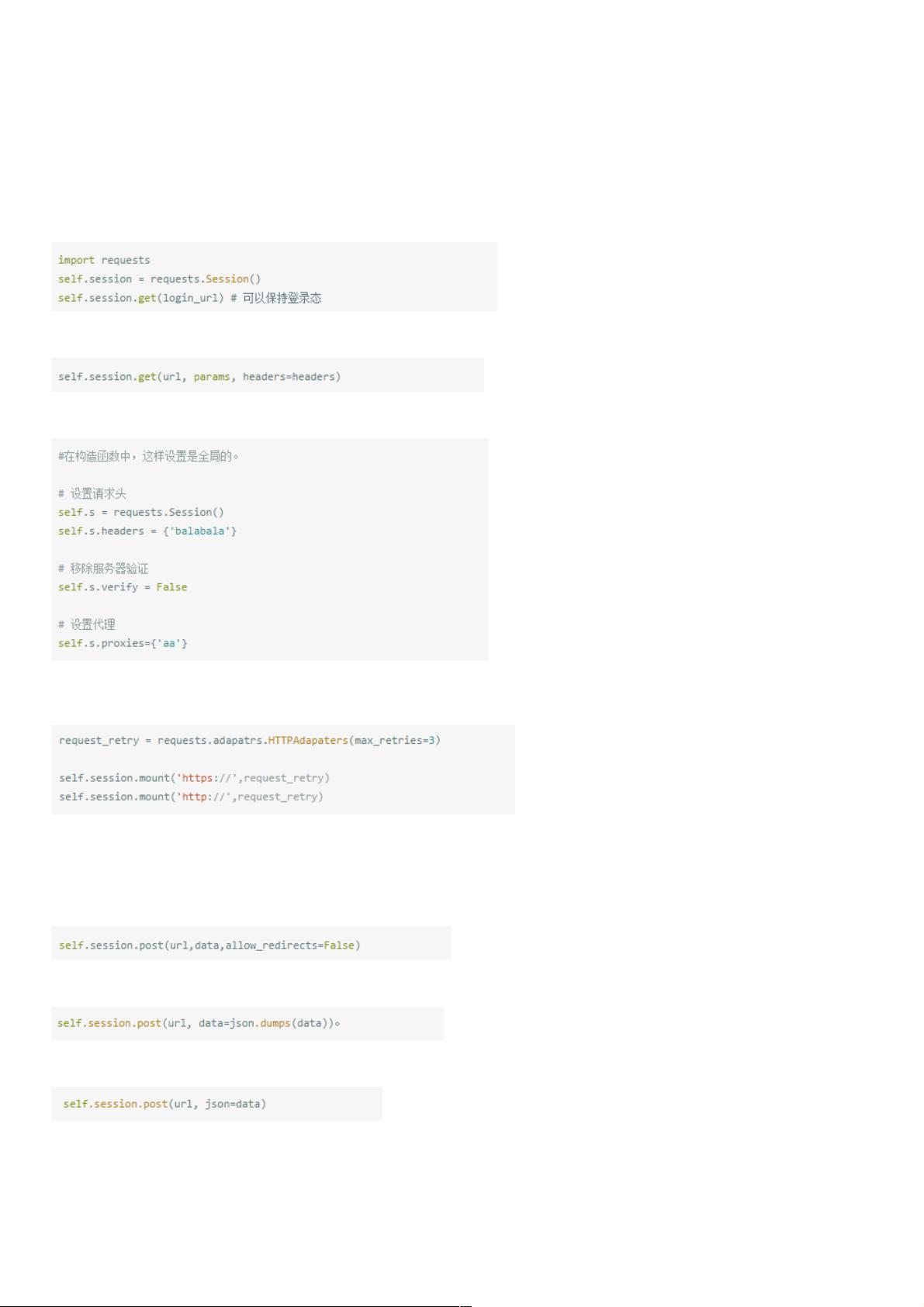
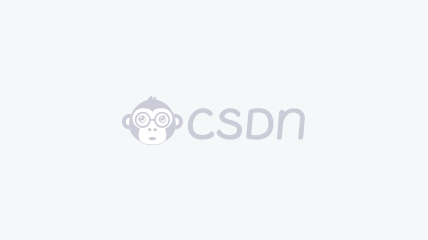

- 粉丝: 4
- 资源: 885
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

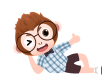
最新资源
- (源码)基于Spring Boot和Vue的后台管理系统.zip
- 用于将 Power BI 嵌入到您的应用中的 JavaScript 库 查看文档网站和 Wiki 了解更多信息 .zip
- (源码)基于Arduino、Python和Web技术的太阳能监控数据管理系统.zip
- (源码)基于Arduino的CAN总线传感器与执行器通信系统.zip
- (源码)基于C++的智能电力系统通信协议实现.zip
- 用于 Java 的 JSON-RPC.zip
- 用 JavaScript 重新实现计算机科学.zip
- (源码)基于PythonOpenCVYOLOv5DeepSort的猕猴桃自动计数系统.zip
- 用 JavaScript 编写的贪吃蛇游戏 .zip
- (源码)基于ASP.NET Core的美术课程管理系统.zip

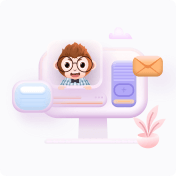
