在iOS开发中,有时我们需要创建各种图形来呈现数据或实现特定的UI效果,例如扇形图。扇形图通常用于表示部分与整体的关系,比如饼状图。在本实例中,我们将详细介绍如何在iOS应用中绘制扇形图。 我们要知道在iOS中绘制图形的基本方法是使用`UIView`的`drawRect:`方法。当`drawRect:`被调用时,我们可以在这个方法内进行图形绘制。`drawRect:`接收一个`CGRect`参数,即需要重绘的矩形区域。为了绘制扇形图,我们需要自定义一个`UIView`的子类,例如命名为`PieView`。 以下是一个简单的`PieView`类的实现: ```swift @interface PieView : UIView @end @implementation PieView - (void)drawRect:(CGRect)rect { CGContextRef ctx = UIGraphicsGetCurrentContext(); CGPoint center = CGPointMake((self.frame.size.width / 2) - radius / 2, (self.frame.size.height / 2) - radius / 2); CGContextAddEllipseInRect(ctx, CGRectMake(center.x, center.y, radius * 2, radius * 2)); [[UIColor greenColor] set]; CGContextFillPath(ctx); } @end ``` 这段代码创建了一个圆形,但还不是扇形。为了绘制扇形,我们需要计算角度并使用`CGContextAddArc`方法。`CGContextAddArc`函数允许我们在给定的起点角度(起点弧度)和终点角度(终点弧度)之间添加一段弧线到当前路径。我们可以使用`radians`辅助函数将角度转换为弧度。 ```swift // 计算度转弧度的辅助函数 static inline float radians(double degrees) { return degrees * M_PI / 180; } - (void)drawRect:(CGRect)rect { CGPoint center = CGPointMake((self.frame.size.width / 2) - radius / 2, (self.frame.size.height / 2) - radius / 2); CGContextRef ctx = UIGraphicsGetCurrentContext(); CGContextClearRect(ctx, rect); float angle_start = radians(0.0); float angle_end = radians(120.0); CGContextMoveToPoint(ctx, center.x, center.y); // 设置路径起点 CGContextAddArc(ctx, center.x, center.y, radius, angle_start, angle_end, NO); // 添加弧线 CGContextClosePath(ctx); // 关闭路径 CGContextSetFillColorWithColor(ctx, [UIColor blueColor].CGColor); // 设置填充颜色 CGContextFillPath(ctx); // 填充路径 } ``` 这样我们就绘制了一个120度的蓝色扇形。为了绘制多个扇形,你可以调整`angle_start`和`angle_end`,并重复这个过程。你还可以添加更多的颜色和数据逻辑来创建多段扇形,以显示不同部分的百分比。 在`UIViewController`中添加`PieView`实例,设置其大小和位置,并添加到视图层次结构,即可在运行时看到扇形图: ```swift @interface PieViewController : UIViewController @end @implementation PieViewController - (void)viewDidLoad { [super viewDidLoad]; PieView *pieView = [[PieView alloc] initWithFrame:CGRectMake(4, 150, 150, 300)]; [self.view addSubview:pieView]; } // ...其他方法 @end ``` 通过以上步骤,你可以在iOS应用中成功地创建并显示扇形图。如果你需要更复杂的功能,如动态更新数据、动画效果或交互性,可以进一步扩展`PieView`类,利用Core Graphics框架提供的更多功能。同时,还可以考虑使用第三方库,如`Charts`库,它提供了更丰富的图表类型和配置选项。
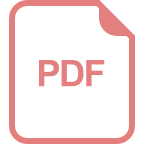
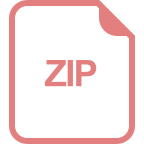
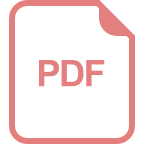
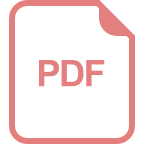
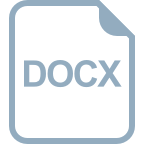
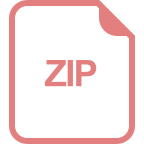
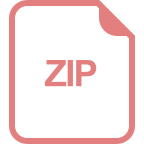
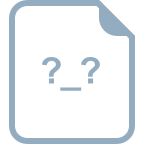
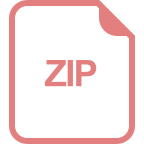
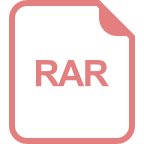
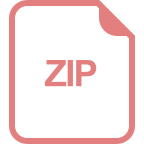
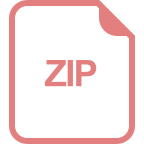
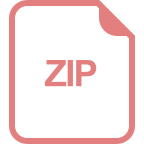
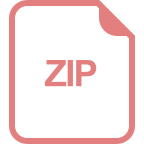
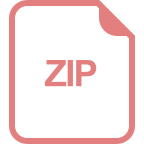
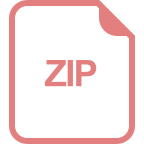
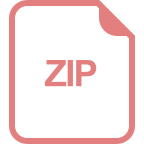
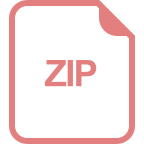
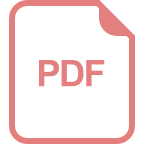
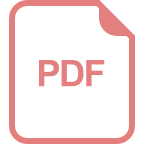
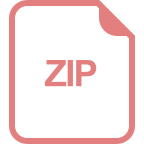
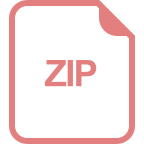
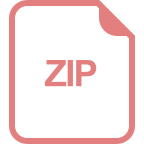
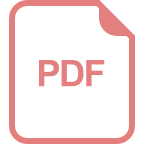
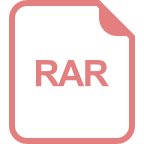
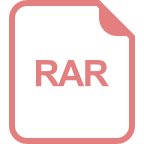
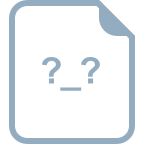
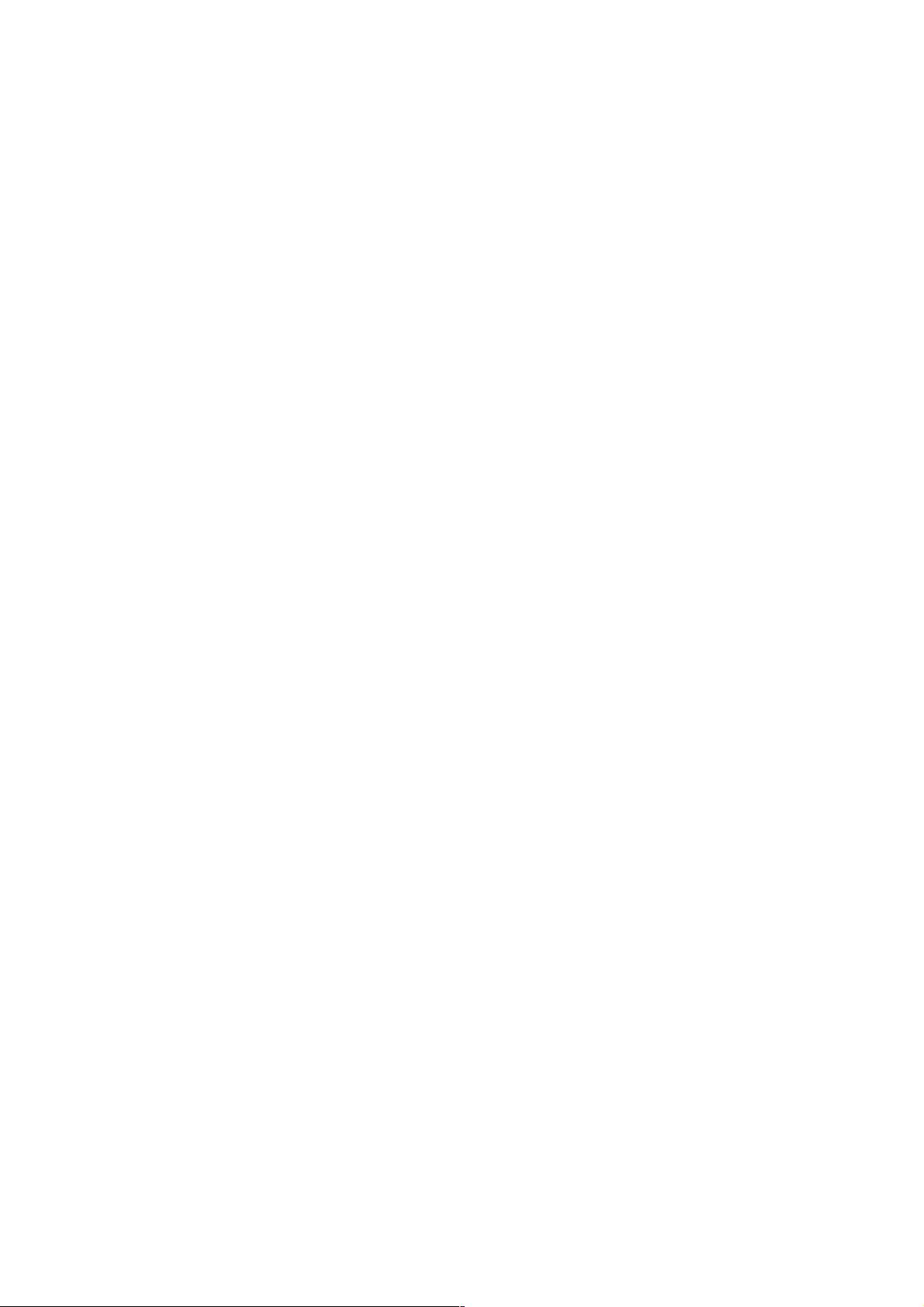
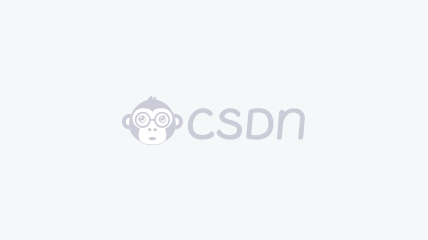

- 粉丝: 8
- 资源: 908
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

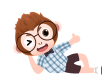
最新资源

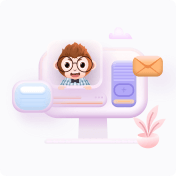
