JavaScript 是一种广泛应用于 web 开发的轻量级脚本语言,尤其在前端开发中占据了核心地位。继承是面向对象编程中的一个关键概念,它允许一个对象(子类)继承另一个对象(父类)的属性和方法。在 JavaScript 中,继承可以通过多种方式实现,包括原型链继承、构造函数继承、组合继承、寄生式继承、寄生组合式继承等。这篇博客 "java script 继承的实现" 会深入探讨这些继承模式。 我们来谈谈**原型链继承**。这是 JavaScript 最初实现继承的方式,利用了原型(prototype)对象的特性。每个 JavaScript 函数都有一个 prototype 属性,这个属性是一个对象,它的 prototype 属性又指向另一个对象,形成一个链状结构。当试图访问一个对象的属性时,如果该对象上没有找到,就会去其原型对象上查找,再找不到就继续向上查找,直到找到或者查到原型链顶端(null)。 ```javascript function Parent() {} Parent.prototype.name = 'parent'; function Child() {} Child.prototype = Object.create(Parent.prototype); Child.prototype.constructor = Child; Child.prototype.name = 'child'; let instance = new Child(); console.log(instance.name); // 输出 'child' ``` 接下来是**构造函数继承**,通过调用父类的构造函数来复制父类的属性和方法,但这种方式会创建重复的属性,且无法继承原型上的方法。 ```javascript function Parent(name) { this.name = name; } Parent.prototype.sayName = function() { console.log(this.name); } function Child(name) { Parent.call(this, name); // 调用父类构造函数 } let child = new Child('child'); console.log(child.name); // 输出 'child' child.sayName(); // 报错,因为 Child 没有继承 sayName ``` **组合继承**结合了原型链和构造函数继承的优点,避免了属性的重复创建,但仍然存在调用两次构造函数的问题。 ```javascript function Parent(name) { this.name = name; } Parent.prototype.sayName = function() { console.log(this.name); } function Child(name) { Parent.call(this, name); // 调用父类构造函数 } Child.prototype = Object.create(Parent.prototype); Child.prototype.constructor = Child; let child = new Child('child'); console.log(child.name); // 输出 'child' child.sayName(); // 输出 'child' ``` **寄生式继承**是为了优化组合继承,通过创建父类的副本,然后修改这个副本,最后再将副本赋值给子类的原型。 ```javascript function inheritPrototype(subType, superType) { let prototype = Object.create(superType.prototype); prototype.constructor = subType; subType.prototype = prototype; } function Parent() {} Parent.prototype.name = 'parent'; function Child() {} inheritPrototype(Child, Parent); Child.prototype.name = 'child'; ``` **寄生组合式继承**是最常用的继承模式,它解决了以上所有问题,既不调用两次构造函数,也不复制父类的属性。 ```javascript function object(o) { function F() {} // 创建一个空函数作为原型对象的构造器 F.prototype = o; // 将空函数的原型指向 o return new F(); // 返回 o 的副本 } function inheritPrototype(subType, superType) { subType.prototype = object(superType.prototype); subType.prototype.constructor = subType; } function Parent(name) { this.name = name; } Parent.prototype.sayName = function() { console.log(this.name); } function Child(name) { Parent.call(this, name); } inheritPrototype(Child, Parent); Child.prototype.name = 'child'; ``` 在提供的 `zinherit.js` 文件中,可能包含了上述某种或多种继承模式的实现示例,你可以打开文件查看具体的代码细节,学习并理解每种继承方式的工作原理。理解并熟练运用这些继承模式对于深入 JavaScript 面向对象编程至关重要,有助于你构建更高效、可维护的代码。
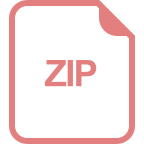
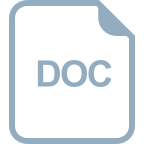
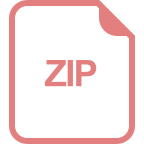
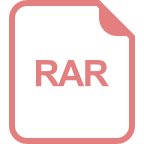
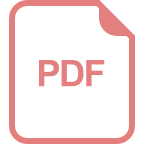
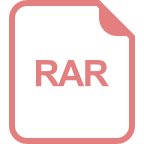
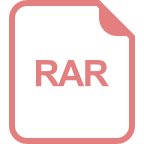
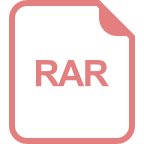
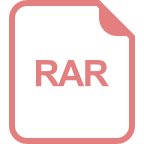
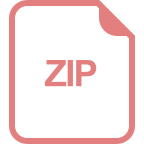
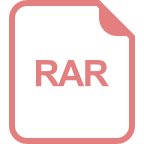
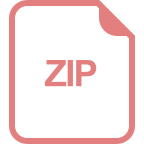
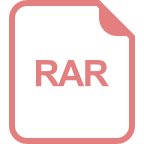
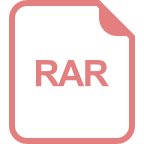
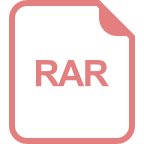
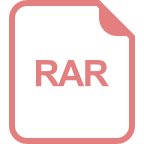
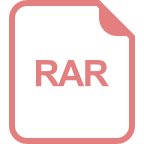
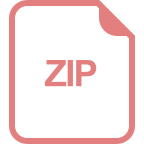
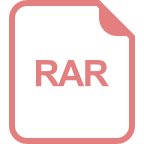
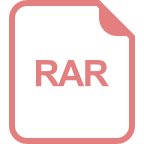
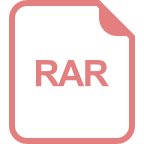
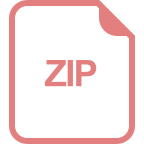
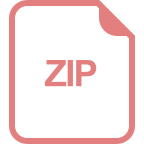
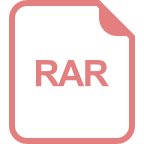
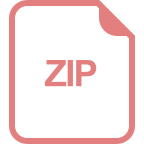

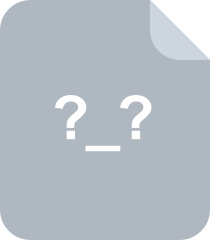
- 1
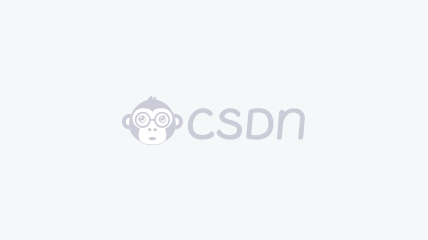

- 粉丝: 387
- 资源: 6万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

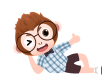
最新资源

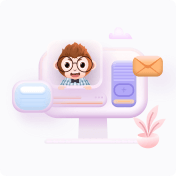
