在前端开发中,Element UI 是一款非常流行的 Vue.js 组件库,它提供了丰富的 UI 元素和组件,包括上传组件。在某些场景下,我们需要处理多个上传组件,而每个组件都需要绑定对应的回调函数,这可能导致代码重复和维护困难。本文将介绍如何解决这个问题,以及如何实现一个简单的倒计时功能。 我们来看如何解决 Element 上传组件的循环引用问题。在传统的做法中,每个上传按钮都需要绑定一个独立的 `on-success` 回调函数,这显然不适用于需要大量上传组件的情况。通过使用 Vue 的数据对象和动态绑定,我们可以实现一个更简洁的解决方案。以下是一个例子: ```html <div v-for="(item, index) in items" :key="index"> <el-upload :action="uploadAction" :on-success="handleSuccess" :before-upload="beforeUpload" :file-list="item.fileList"> <!-- ... --> </el-upload> </div> ``` 在 Vue 的 `data` 对象中,我们可以定义一个通用的 `handleSuccess` 函数,然后通过参数来区分不同的上传操作: ```javascript data() { return { items: [ // ... ], handleSuccess: function (res, file, index) { this.items[index].fileList = [res.file]; }, beforeUpload: function (file, index) { // ... }, }; }, created() { this.items.forEach((item, index) => { item.onSuccess = this.handleSuccess.bind(this, index); item.beforeUpload = this.beforeUpload.bind(this, index); }); } ``` 这样,我们只需要一个 `handleSuccess` 函数,通过 `v-for` 中的 `index` 参数来确定当前处理的是哪个上传组件。 接下来,我们探讨如何实现一个简单的倒计时功能。在前端应用中,倒计时常用于显示某个事件即将发生的时间,例如验证码的有效期。我们可以创建一个名为 `countdown` 的方法,利用 `setTimeout` 或 `setInterval` 来定时更新状态: ```javascript data() { return { countdownTime: 60, // 倒计时总秒数 countdownRunning: false, }; }, methods: { startCountdown() { if (!this.countdownRunning) { this.countdownRunning = true; const countdownInterval = setInterval(() => { if (this.countdownTime > 0) { this.countdownTime--; } else { clearInterval(countdownInterval); this.countdownTime = 60; this.countdownRunning = false; } }, 1000); } }, stopCountdown() { this.countdownRunning = false; }, } ``` 在模板中,我们可以根据 `countdownTime` 属性显示剩余时间,并通过 `startCountdown` 和 `stopCountdown` 方法来控制倒计时的开始和结束。 总结起来,通过合理利用 Vue 的数据绑定和方法,我们可以有效地解决 Element 上传组件的循环引用问题,同时实现一个简单的倒计时功能。这种编程模式提高了代码的复用性和可维护性,使得前端开发更加高效。在实际项目中,根据具体需求,还可以对这些基础示例进行扩展和优化,以适应复杂的应用场景。
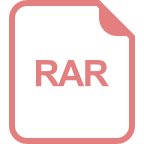
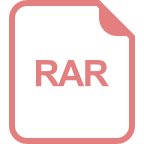
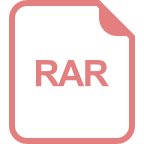
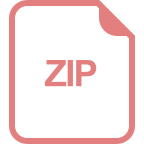
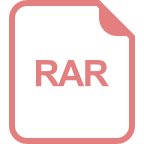
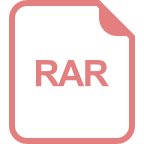
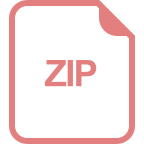
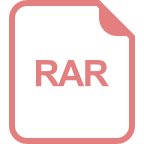
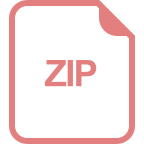
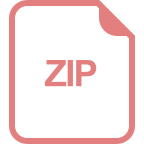
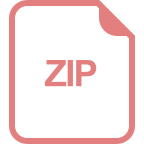
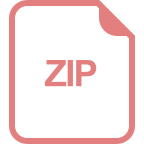
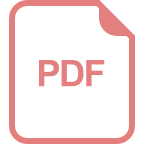
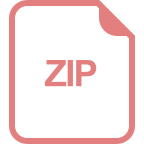
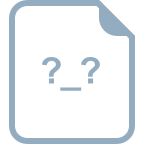
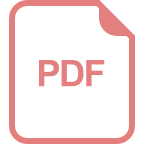
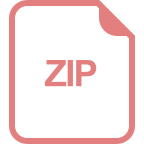
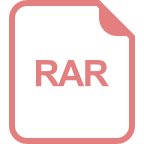
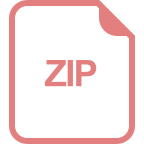
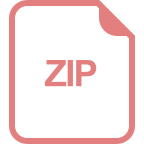
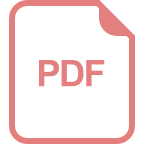
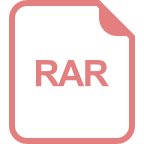
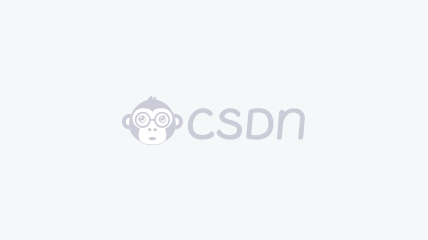

- 粉丝: 3
- 资源: 967
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

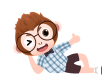
