没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
intel fpga opencl 编程指南 The Intel® FPGA SDK for OpenCL™ Programming Guide provides descriptions, recommendations and usage information on the Intel Software Development Kit (SDK) for OpenCL compiler and tools. The Intel FPGA SDK for OpenCL1 is an OpenCL2-based heterogeneous parallel programming environment for Intel FPGA products.
资源推荐
资源详情
资源评论
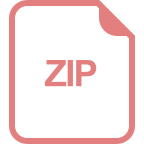
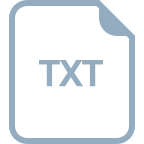
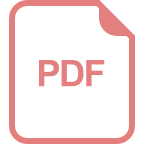
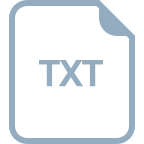
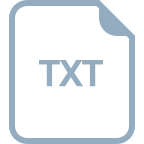
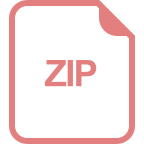
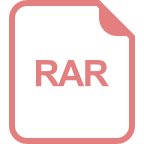
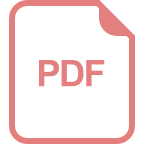
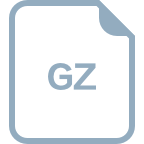
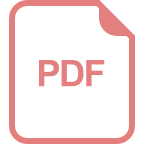
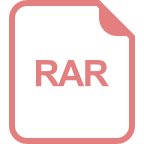
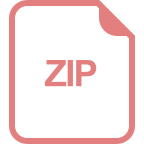
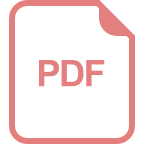
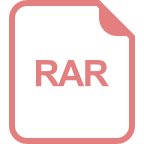
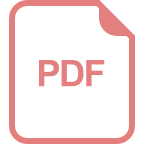
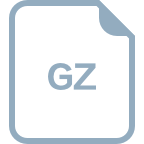
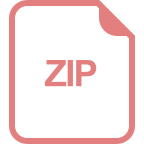
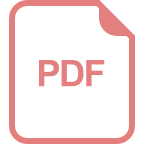
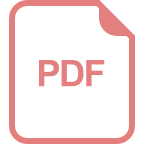
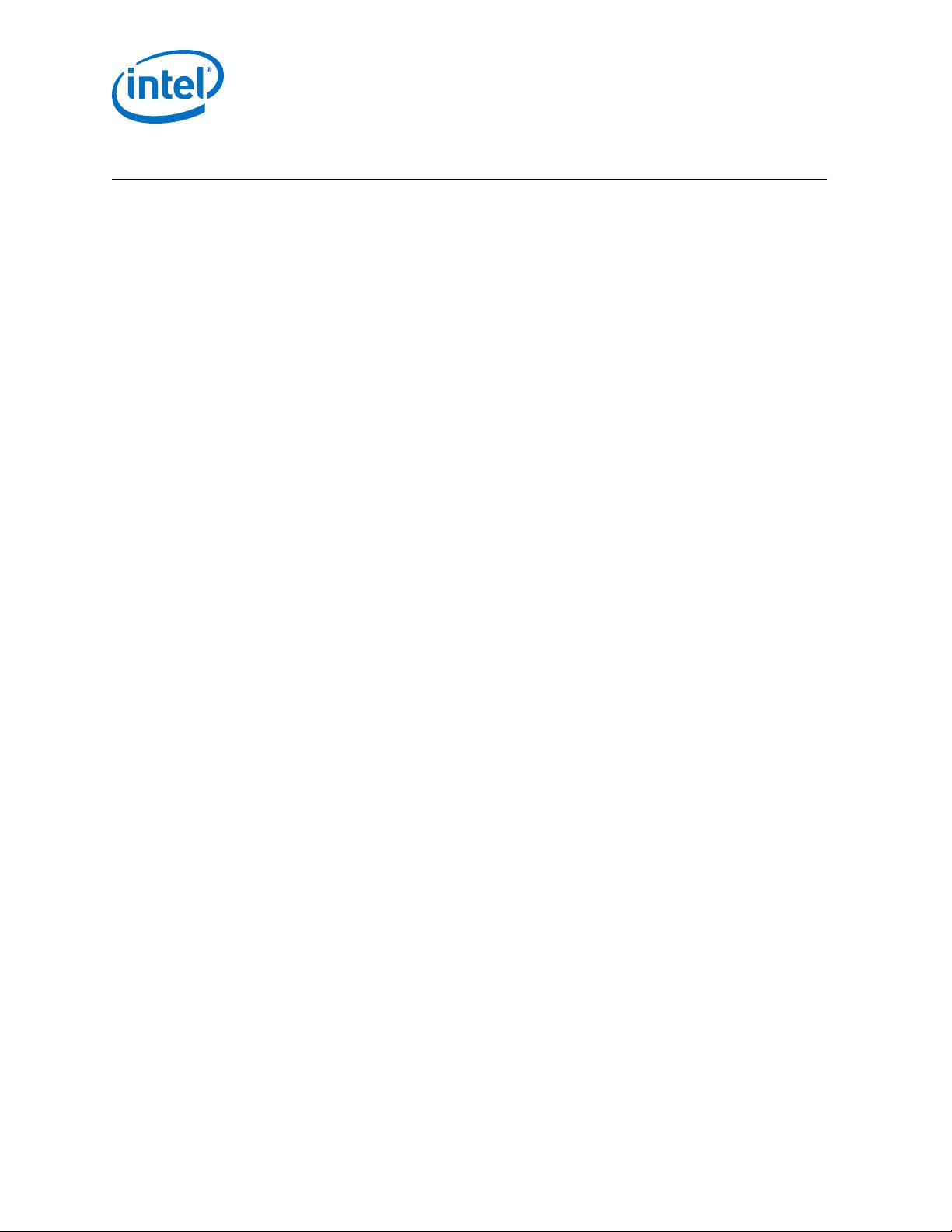
Contents
1 Intel
®
FPGA SDK for OpenCL
™
Programming Guide.......................................................... 5
1.1 Intel FPGA SDK for OpenCL Programming Guide Prerequisites...................................... 5
1.2 Intel FPGA SDK for OpenCL FPGA Programming Flow...................................................6
1.3 Intel FPGA SDK for OpenCL Offline Compiler Kernel Compilation Flows...........................7
1.3.1 One-Step Compilation for Simple Kernels....................................................... 8
1.3.2 Multistep Intel FPGA SDK for OpenCL Design Flow...........................................9
1.4 Obtaining General Information on Software, Compiler, and Custom Platform................. 11
1.4.1 Displaying the Software Version (version).................................................... 12
1.4.2 Displaying the Compiler Version (--version).................................................. 12
1.4.3 Listing the Intel FPGA SDK for OpenCL Utility Command Options (help)............12
1.4.4 Listing the Intel FPGA SDK for OpenCL Offline Compiler Command Options
(no argument, --help, or -h)......................................................................13
1.4.5 Listing the Available FPGA Boards in Your Custom Platform (--list-boards)........ 13
1.4.6 Displaying the Compilation Environment of an OpenCL Binary (env).................14
1.5 Managing an FPGA Board....................................................................................... 14
1.5.1 Installing an FPGA Board (install)................................................................ 15
1.5.2 Uninstalling the FPGA Board (uninstall)........................................................ 17
1.5.3 Querying the Device Name of Your FPGA Board (diagnose)............................. 17
1.5.4 Running a Board Diagnostic Test (diagnose <device_name>)..........................18
1.5.5 Programming the FPGA Offline or without a Host (program <device_name>).... 18
1.5.6 Programming the Flash Memory (flash <device_name>)................................ 18
1.6 Structuring Your OpenCL Kernel..............................................................................19
1.6.1 Guidelines for Naming the Kernel................................................................ 20
1.6.2 Programming Strategies for Optimizing Data Processing Efficiency...................21
1.6.3 Programming Strategies for Optimizing Local Memory Efficiency......................25
1.6.4 Implementing the Intel FPGA SDK for OpenCL Channels Extension.................. 26
1.6.5 Implementing OpenCL Pipes.......................................................................45
1.6.6 Implementing Arbitrary Precision Integers....................................................61
1.6.7 Using Predefined Preprocessor Macros in Conditional Compilation.................... 62
1.6.8 Declaring __constant Address Space Qualifiers............................................. 63
1.6.9 Including Structure Data Types as Arguments in OpenCL Kernels.....................64
1.6.10 Inferring a Register................................................................................. 68
1.6.11 Enabling Double Precision Floating-Point Operations.....................................69
1.6.12 Single-Cycle Floating-Point Accumulator for Single Work-Item Kernels............ 70
1.7 Designing Your Host Application..............................................................................72
1.7.1 Host Programming Requirements................................................................ 73
1.7.2 Allocating OpenCL Buffers for Manual Partitioning of Global Memory.................74
1.7.3 Collecting Profile Data During Kernel Execution............................................. 78
1.7.4 Accessing Custom Platform-Specific Functions...............................................80
1.7.5 Modifying Host Program for Structure Parameter Conversion...........................81
1.7.6 Managing Host Application......................................................................... 82
1.7.7 Allocating Shared Memory for OpenCL Kernels Targeting SoCs........................ 93
1.8 Compiling Your OpenCL Kernel................................................................................95
1.8.1 Compiling Your Kernel to Create Hardware Configuration File.......................... 95
1.8.2 Compiling Your Kernel without Building Hardware (-c)....................................96
1.8.3 Specifying the Location of Header Files (-I <directory>).................................96
Contents
Intel
®
FPGA SDK for OpenCL Programming Guide
2
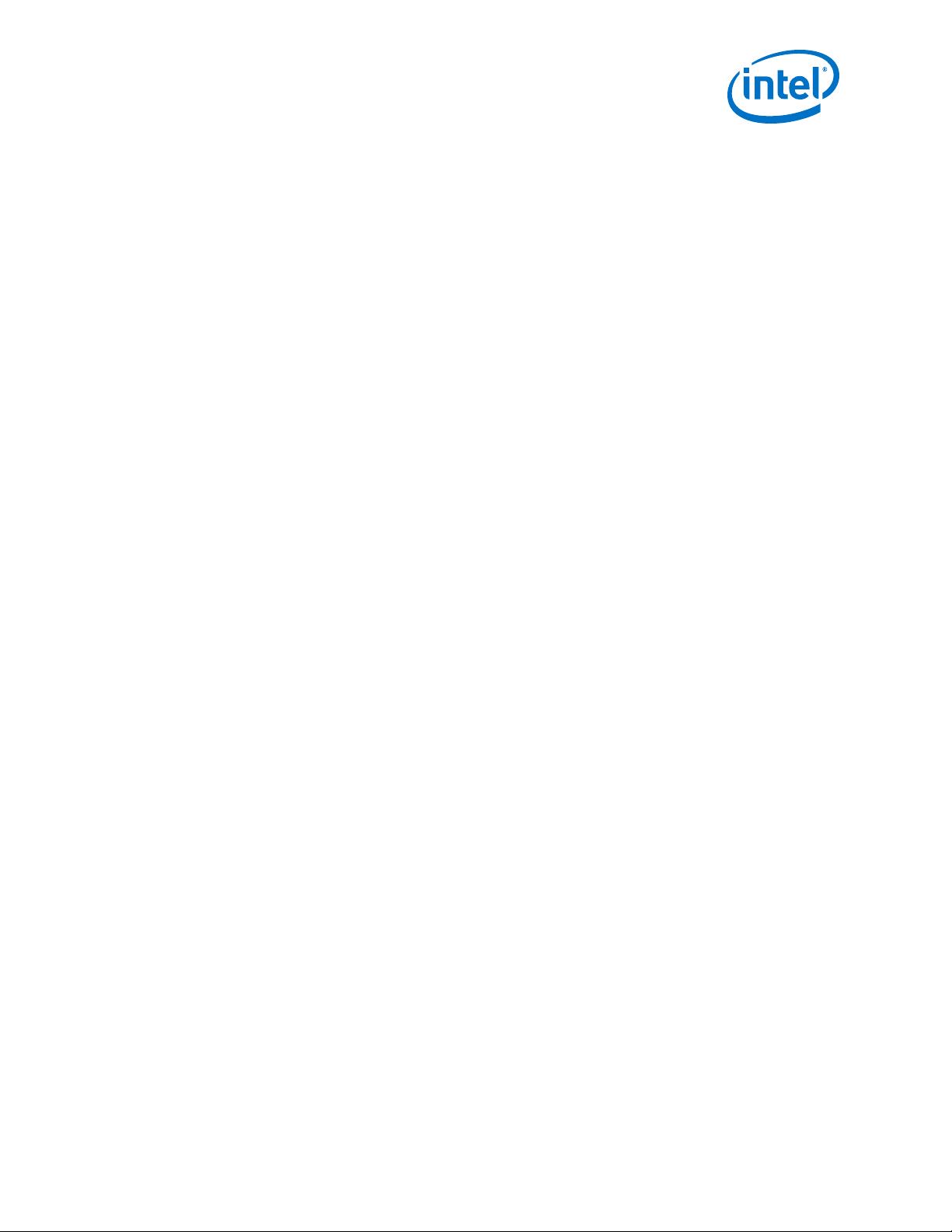
1.8.4 Specifying the Name of an Intel FPGA SDK for OpenCL Offline Compiler
Output File (-o <filename>)...................................................................... 97
1.8.5 Compiling a Kernel for a Specific FPGA Board (--board <board_name>)........... 97
1.8.6 Resolving Hardware Generation Fitting Errors during Kernel Compilation (--
high-effort)............................................................................................. 99
1.8.7 Defining Preprocessor Macros to Specify Kernel Parameters (-D
<macro_name>)..................................................................................... 99
1.8.8 Generating Compilation Progress Report (-v).............................................. 101
1.8.9 Displaying the Estimated Resource Usage Summary On-Screen (--report).......102
1.8.10 Suppressing Warning Messages from the Intel FPGA SDK for OpenCL
Offline Compiler (-W)..............................................................................102
1.8.11 Converting Warning Messages from the Intel FPGA SDK for OpenCL
Offline Compiler into Error Messages (-Werror)...........................................102
1.8.12 Removing Debug Data from Compiler Reports and Source Code from
the .aocx File (-g0).................................................................................103
1.8.13 Disabling Burst-Interleaving of Global Memory (--no-interleaving
<global_memory_type>)........................................................................ 103
1.8.14 Configuring Constant Memory Cache Size (--const-cache-bytes <N>)...........104
1.8.15 Relaxing the Order of Floating-Point Operations (--fp-relaxed)..................... 104
1.8.16 Reducing Floating-Point Rounding Operations (--fpc).................................. 105
1.9 Emulating and Debugging Your OpenCL Kernel........................................................ 105
1.9.1 Modifying Channels Kernel Code for Emulation............................................ 106
1.9.2 Compiling a Kernel for Emulation (-march=emulator)...................................107
1.9.3 Emulating Your OpenCL Kernel.................................................................. 109
1.9.4 Debugging Your OpenCL Kernel on Linux.................................................... 110
1.9.5 Limitations of the Intel FPGA SDK for OpenCL Emulator................................111
1.10 Reviewing Your Kernel's report.html File............................................................... 112
1.11 Profiling Your OpenCL Kernel...............................................................................112
1.11.1 Instrumenting the Kernel Pipeline with Performance Counters (--profile)....... 113
1.11.2 Launching the Intel FPGA SDK for OpenCL Profiler GUI (report)................... 113
1.12 Conclusion........................................................................................................113
1.13 Document Revision History................................................................................. 114
2 Intel FPGA SDK for OpenCL Advanced Features........................................................... 120
2.1 OpenCL Library...................................................................................................120
2.1.1 Understanding RTL Modules and the OpenCL Pipeline................................... 122
2.1.2 Packaging an OpenCL Helper Function File for an OpenCL Library...................131
2.1.3 Packaging an RTL Component for an OpenCL Library ...................................132
2.1.4 Verifying the RTL Modules........................................................................ 135
2.1.5 Packaging Multiple Object Files into a Library File.........................................135
2.1.6 Specifying an OpenCL Library when Compiling an OpenCL Kernel...................135
2.1.7 Using an OpenCL Library that Works with Simple Functions (Example 1).........136
2.1.8 Using an OpenCL Library that Works with External Memory (Example 2).........137
2.1.9 OpenCL Library Command-Line Options......................................................138
2.2 Kernel Attributes for Configuring Local Memory System............................................139
2.2.1 Restrictions on the Usage of Local Variable-Specific Kernel Attributes............. 141
2.3 Kernel Attributes for Reducing the Overhead on Hardware Usage...............................142
2.3.1 Hardware for Kernel Interface................................................................... 142
2.4 Kernel Replication Using the num_compute_units(X,Y,Z) Attribute............................. 145
2.4.1 Customization of Replicated Kernels Using the get_compute_id() Function...... 146
2.4.2 Using Channels with Kernel Copies............................................................ 147
2.5 Document Revision History...................................................................................148
Contents
Intel
®
FPGA SDK for OpenCL Programming Guide
3
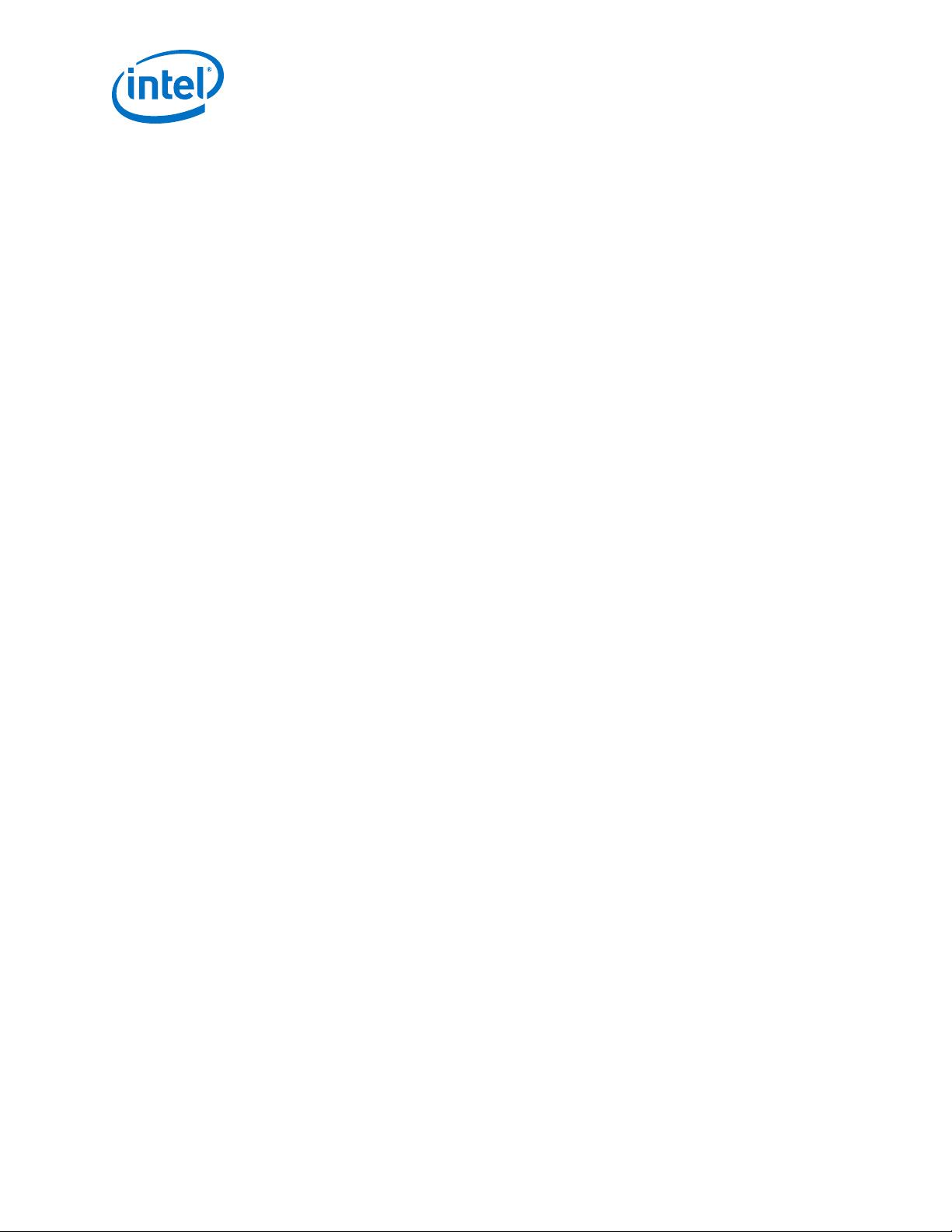
A Support Statuses of OpenCL Features ......................................................................... 149
A.1 Support Statuses of OpenCL 1.0 Features...............................................................149
A.1.1 OpenCL1.0 C Programming Language Implementation................................. 149
A.1.2 OpenCL C Programming Language Restrictions............................................151
A.1.3 Argument Types for Built-in Geometric Functions.........................................152
A.1.4 Numerical Compliance Implementation...................................................... 153
A.1.5 Image Addressing and Filtering Implementation.......................................... 153
A.1.6 Atomic Functions.................................................................................... 153
A.1.7 Embedded Profile Implementation............................................................. 154
A.2 Support Statuses of OpenCL 1.2 Features...............................................................154
A.2.1 OpenCL 1.2 Runtime Implementation.........................................................154
A.2.2 OpenCL 1.2 C Programming Language Implementation................................ 155
A.3 Support Statuses of OpenCL 2.0 Features...............................................................156
A.3.1 OpenCL 2.0 Headers................................................................................156
A.3.2 OpenCL 2.0 Runtime Implementation.........................................................156
A.3.3 OpenCL 2.0 C Programming Language Restrictions for Pipes......................... 156
A.4 Intel FPGA SDK for OpenCL Allocation Limits...........................................................157
A.5 Document Revision History...................................................................................158
Contents
Intel
®
FPGA SDK for OpenCL Programming Guide
4
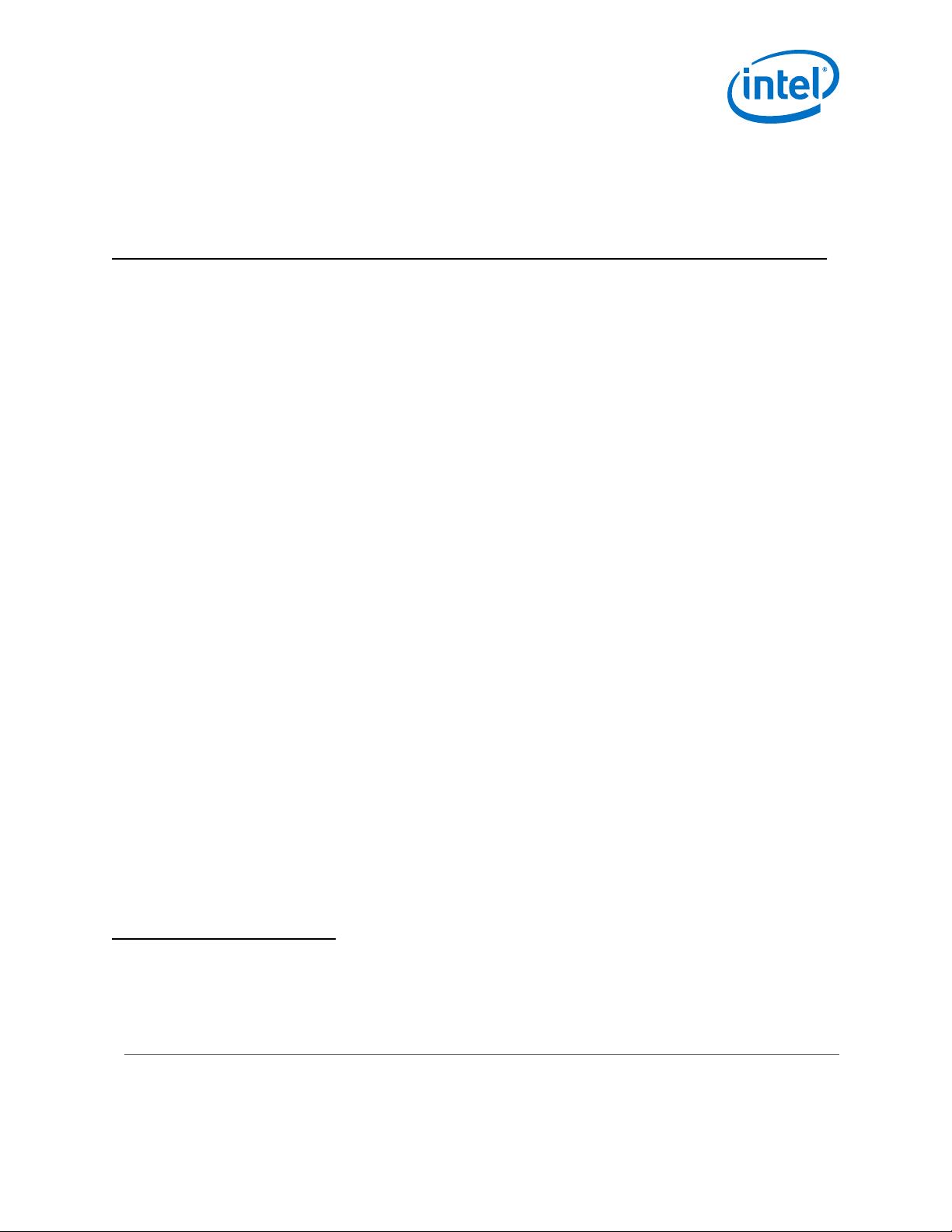
1 Intel
®
FPGA SDK for OpenCL
™
Programming Guide
The Intel
®
FPGA SDK for OpenCL
™
Programming Guide provides descriptions,
recommendations and usage information on the Intel Software Development Kit (SDK)
for OpenCL compiler and tools. The Intel FPGA SDK for OpenCL
1
is an OpenCL
2
-based
heterogeneous parallel programming environment for Intel FPGA products.
1.1 Intel FPGA SDK for OpenCL Programming Guide Prerequisites
The Intel FPGA SDK for OpenCL Programming Guide assumes that you are
knowledgeable in OpenCL concepts and application programming interfaces (APIs). It
also assumes that you have experience creating OpenCL applications and are familiar
with the OpenCL Specification version 1.0.
Before using the Intel FPGA SDK for OpenCL or the Intel FPGA Runtime Environment
(RTE) for OpenCL to program your device, familiarize yourself with the respective
getting started guides. This document assumes that you have performed the following
tasks:
• For developing and deploying OpenCL kernels, download the tar file and run the
installers to install the SDK, the Quartus
®
Prime software, and device support.
• For deployment of OpenCL kernels, download and install the RTE.
• If you want to use the SDK or the RTE to program a Cyclone
®
V SoC Development
Kit, you also have to download and install the SoC Embedded Design Suite (EDS).
• Install and set up your FPGA board.
• Program your device with the device-compatible version of the hello_world
example OpenCL application
If you have not performed the tasks described above, refer to the SDK's getting
starting guides for more information.
Prior to creating an OpenCL design and programming your FPGA board, review the
Intel FPGA SDK for OpenCL allocation limits.
Related Links
• Intel FPGA SDK for OpenCL Allocation Limits on page 157
• OpenCL References Pages
1 The Intel FPGA SDK for OpenCL is based on a published Khronos Specification, and has passed
the Khronos Conformance Testing Process. Current conformance status can be found at
www.khronos.org/conformance.
2 OpenCL and the OpenCL logo are trademarks of Apple Inc. used by permission of the Khronos
Group
™
.
1 Intel
®
FPGA SDK for OpenCL
™
Programming Guide
Intel Corporation. All rights reserved. Intel, the Intel logo, Altera, Arria, Cyclone, Enpirion, MAX, Nios, Quartus
and Stratix words and logos are trademarks of Intel Corporation or its subsidiaries in the U.S. and/or other
countries. Intel warrants performance of its FPGA and semiconductor products to current specifications in
accordance with Intel's standard warranty, but reserves the right to make changes to any products and services
at any time without notice. Intel assumes no responsibility or liability arising out of the application or use of any
information, product, or service described herein except as expressly agreed to in writing by Intel. Intel
customers are advised to obtain the latest version of device specifications before relying on any published
information and before placing orders for products or services.
*Other names and brands may be claimed as the property of others.
ISO
9001:2008
Registered
剩余158页未读,继续阅读
资源评论
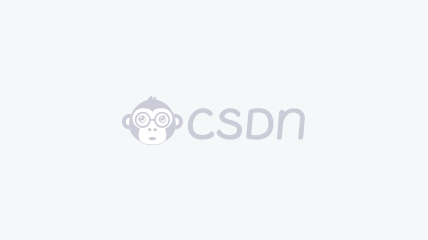

weixin_37593498
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

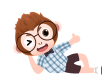
最新资源
- STM32F401,使用ST-link时候,不能识别,显示ST-LINK USB communication error
- 快速排序算法Python实现:详解分治法原理与高效排序步骤
- 陀螺仪选型陀螺仪陀螺仪选型型陀螺仪选型
- Intouch2020R2SP1与西门子1500PLC通讯配置手册
- 英特尔2021-2024年网络连接性和IPU路线图
- RuoYi-Cloud-Plus 微服务通用权限管理系统
- 家庭用具检测15-YOLO(v8至v11)数据集合集.rar
- deploy.yaml
- PHP快速排序算法实现与优化
- 2023-04-06-项目笔记 - 第三百五十五阶段 - 4.4.2.353全局变量的作用域-353 -2025.12.22
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


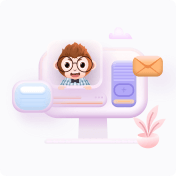
安全验证
文档复制为VIP权益,开通VIP直接复制
