<?php if(!defined('DIR')){header('HTTP/1.1 404 Not Found');header("status: 404 Not Found");exit;}
//允许跨域访问
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: Access-Control-Allow-Private-Network,Content-Type, AccessToken, X-CSRF-Token, Authorization, Token,X-Token,X-Cid");
AccessControl();
//鉴权验证 Cookie验证通过,验证二级密码,Cookie验证失败时尝试验证token
if(!empty(trim($_REQUEST['token']))){ $_COOKIE = []; } //兼容浏览器插件,避免干扰
//获取请求方法
$method = htmlspecialchars(trim($_GET['method']),ENT_QUOTES);
$LoginConfig = unserialize($USER_DB['LoginConfig']);
$api_model = $LoginConfig['api_model']; //API模式
if(!is_login()){
//没登录,尝试验证token
if( empty($USER_DB['Token']) ){
msg(-1,'鉴权失败:未登录且未设置token');
}
//获取请求token
$token = trim($_REQUEST['token']);
if(empty($token)){
msg(-1,'鉴权失败:未登录且请求未携带token');
}else{
if($token === $USER_DB['Token']){
//验证通过
}else{
msg(-1,'鉴权失败:未登录且token错误');
}
}
//Cookie登录验证OK,验证二级密码
}elseif(Check_Password2($LoginConfig)){
// Cookie 二级密码验证成功(未设置时也认为成功)
}else{
msg(-1,'请先验证二级密码!');
}
//扩展API
if($global_config['api_extend'] == 1 && is_file('./system/api_extend.php')){
require './system/api_extend.php';
}
//兼容API
$compatible_list = ['add_link','edit_link','del_link','link_list','get_a_link','q_category_link','category_list','get_a_category','add_category','edit_category','app_info','check_login','global_search'];
if(in_array($api_model,['compatible','compatible+open']) && in_array($method,$compatible_list)){
require 'api_compatible.php';
exit;
}
//站长相关方法名
$root = ['write_subscribe','write_sys_settings','write_default_settings','read_user_list','write_user_info','read_purview_list','read_users_list','write_users','read_regcode_list','write_regcode','other_upsys','read_log','other_root'];
if(in_array($method,$root)){
require('api_root.php');
//非站长接口则判断是否加载防火墙
}elseif($global_config['XSS_WAF'] == 1 || $global_config['SQL_WAF'] == 1){
require DIR.'/system/firewall.php';
}
//函数名过滤和检测是否存在,存在则执行,否则报错
if ( preg_match("/^read_|^write_|^other_/",$method) && function_exists($method) ) {
$method();
}else{
Amsg(-1,'方法未找到 >> '.$method);
}
//读分类列表
function read_category_list(){
if($_GET['type'] == 'onlyf'){
$where = ['uid'=>UID,'fid'=>0,'ORDER' => ['weight'=>'ASC']];
}else{
$where = ['uid'=>UID,'ORDER' => ['weight'=>'ASC']];
}
//
if($_GET['type'] == 'share'){
$categorys = [];
//获取父分类
$category_parent = select_db('user_categorys',['cid(id)','fid','name','font_icon'],['uid'=>UID,'fid'=>0]);
//遍历父分类下的二级分类
foreach ($category_parent as $category) {
array_push($categorys,$category);
$category_subs = select_db('user_categorys',['cid(id)','fid','name','font_icon'],['uid'=>UID,'fid'=>$category['id']]);
$categorys = array_merge ($categorys,$category_subs);
}
msgA(['code'=>1,'msg'=>'获取成功','count'=>count($categorys),'data'=>$categorys ]);
}
//精简数据(链接列表调用)
if($_GET['type'] === 'Simplify'){
$categorys = [];
//获取父分类
$category_parent = select_db('user_categorys',['cid','fid','name','font_icon'],['uid'=>UID,'fid'=>0]);
//遍历父分类下的二级分类
foreach ($category_parent as $category) {
array_push($categorys,$category);
$category_subs = select_db('user_categorys',['cid','fid','name','font_icon'],['uid'=>UID,'fid'=>$category['cid']]);
$categorys = array_merge ($categorys,$category_subs);
}
//数据处理
$new_categorys=[];
foreach ($categorys as $data){
$new_categorys[$data['cid']]['name']=$data['name'];
$new_categorys[$data['cid']]['font_icon']=$data['font_icon'];
$new_categorys[$data['cid']]['fid'] = $data['fid'];
}
msgA(['code'=>1,'msg'=>'获取成功','count'=>count($new_categorys),'data'=>$new_categorys ]);
}
$datas = select_db('user_categorys',['cid','fid','pid(pwd_id)','status','property','name','add_time','up_time','weight','description','font_icon','icon'],$where);
foreach ($datas as $key => $data){
$datas[$key]['count'] = count_db('user_links',['uid'=>UID,'fid'=>$data['cid']]);
if(!empty($datas[$key]['pwd_id'])){
$datas[$key]['pwd'] = get_db('user_pwd_group','password',['uid'=>UID,'pid'=>$datas[$key]['pwd_id']]);
}
}
msgA(['code'=>1,'msg'=>'获取成功','count'=>count($datas),'data'=>$datas ]);
}
//读一个分类信息
function read_one_category(){
$cid = intval(trim($_REQUEST['cid']));
//虚拟分类是指通过书签分享时产生的分类,主要配合前端编辑
if(empty($cid)){msg(-1,$_REQUEST['cid'] == 'share'?'虚拟分类不能编辑':'id不能为空!');}
$where['cid'] = $cid;
$where['uid'] = UID;
$category_info = get_db('user_categorys',['cid','fid','property','name','font_icon','description','icon'],$where);
if(empty($category_info)){
msgA(['code'=>-1,'msg'=>'没有找到分类信息','data'=>[]]);
}else{
msgA(['code'=>1,'data'=>$category_info]);
}
}
//写分类列表(新增/修改/删除/排序)
function write_category(){
check_purview('category',2);
//新增/修改时名称和图标不能为空
if(in_array($_GET['type'],['add','edit'])){
if(empty($_POST['name'])){
msg(-1,'分类名称不能为空');
}elseif(!preg_match('/^(fa fa-|layui-icon layui-icon-)([A-Za-z0-9]|-)+$/',$_POST['font_icon'])){
$_POST['font_icon'] = 'fa fa-star-o';
//msg(-1,'无效的分类图标');
}
}
//新增
if($_GET['type'] === 'add'){
//分类名查重
if(get_db('user_categorys','cid',['uid'=>UID ,"name" => $_POST['name']])){
msg(-1,'分类名称已存在');
}
//父分类不能是二级分类
if(intval($_POST['fid']) !=0 && get_db('user_categorys','fid',['uid'=>UID ,"cid" => intval($_POST['fid']) ]) !=0 ){
msg(-1,'父分类不能是二级分类');
}
//加密组pid是否存在
if(intval($_POST['pwd_id']) !=0 && empty(get_db('user_pwd_group','pid',['uid'=>UID ,"pid" => intval($_POST['pwd_id'])]))){
msg(-1,'加密组不存在');
}
//长度检测
$length_limit = unserialize(get_db("global_config","v",["k"=>"length_limit"]));
if($length_limit['c_name'] > 0 && strlen($_POST['name']) > $length_limit['c_name'] ){
msg(-1,'名称长度不能大于'.$length_limit['c_name'].'个字节');
}
if($length_limit['c_desc'] > 0 && strlen($_POST['description']) > $length_limit['c_desc'] ){
msg(-1,'名称长度不能大于'.$length_limit['c_desc'].'个字节');
}
//取最大CID
$cid = get_maxid('category_id');
//插入数据库
insert_db('user_categorys',[
'uid'=>UID,
'cid'=>$cid,
'fid'=>intval($_POST['fid']??'0'),
'pid'=>intval($_POST['pwd_id']??'0'),
'status'=>1,
'property'=>intval($_POST['property']??'0'),
'name'=>htmlspecialchars($_POST['name'],ENT_QUOTES),
'add_time'=>time(),
'up_time'=>time(),
'weight'=>$cid,
'description'=>htmlspecialchars($_POST['description'],ENT_QUOTES),
'font_icon'=>$_POST['font_icon'],
'icon'=>$_POST['icon']??''
没有合适的资源?快使用搜索试试~ 我知道了~
TwoNav导航免授权版本:多模板导航网源码
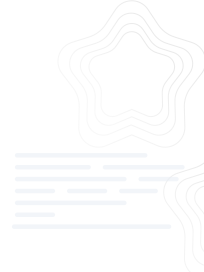
共613个文件
php:143个
js:110个
png:68个

6 下载量 44 浏览量
2023-09-17
23:18:17
上传
评论 1
收藏 13.43MB ZIP 举报
温馨提示
程序目前已是最新过了授权 环境要求 PHP版本: 7.4 数据库: MySQL5.6 安装教程: 把目录上传 导入数据库文件:dhw.sql 修改数据库配置 data/config.php 后台路径:域名/?c=admin&u=admin 账号:admin 密码:bygoukai.com
资源推荐
资源详情
资源评论
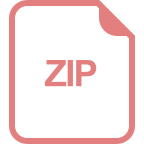
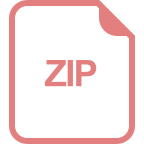
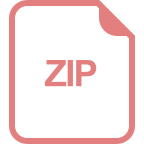
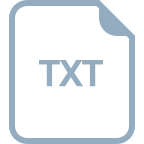
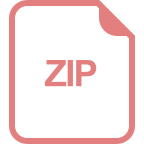
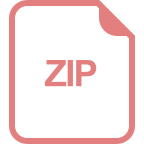
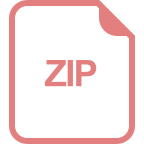
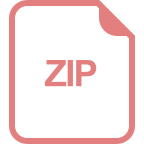
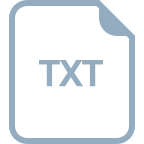
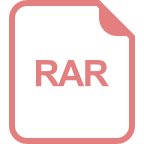
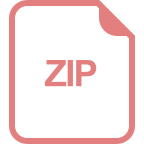
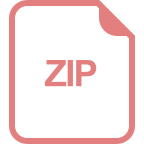
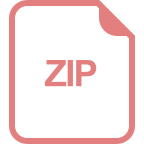
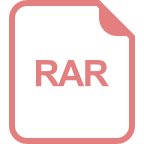
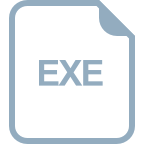
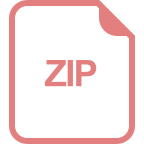
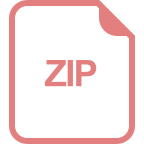
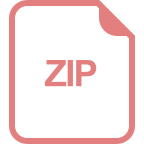
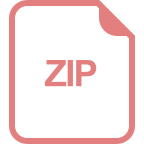
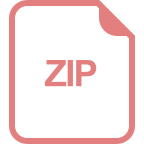
收起资源包目录

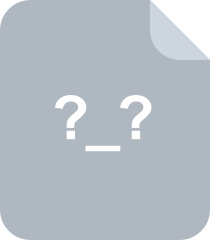
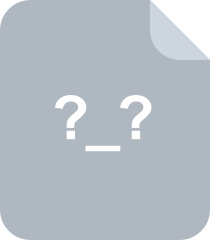
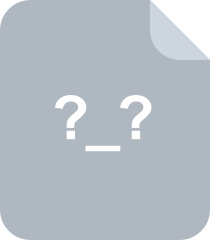
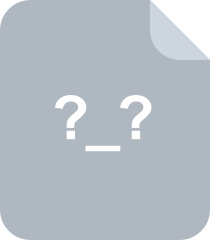
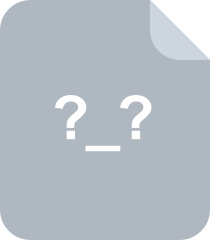
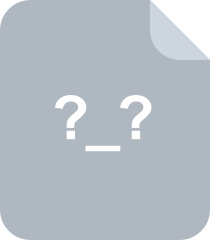
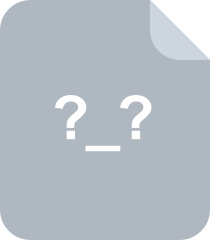
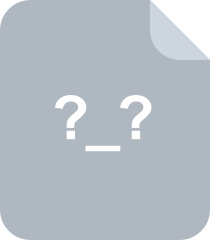
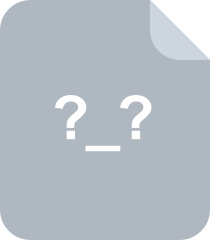
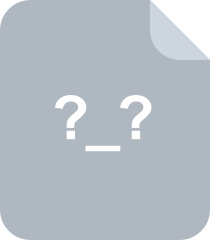
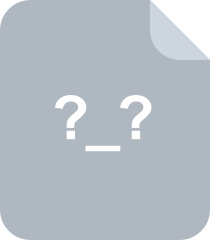
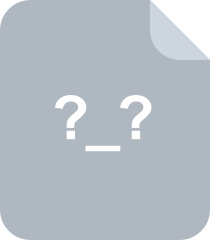
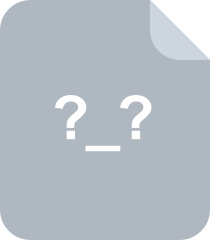
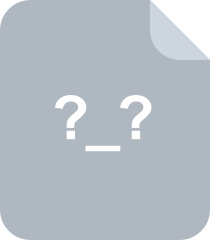
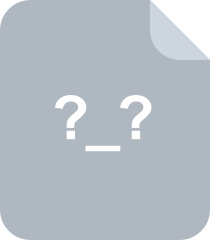
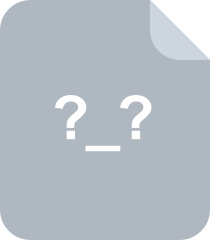
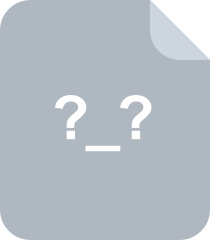
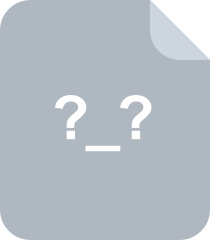
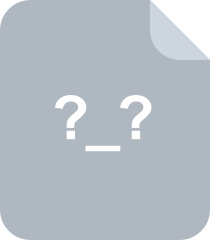
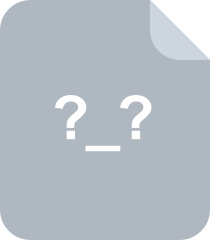
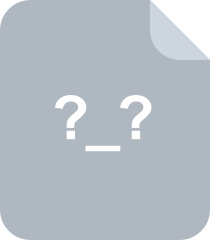
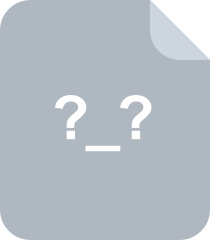
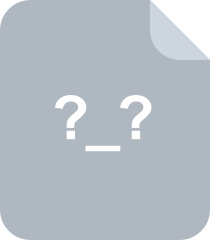
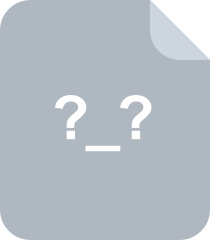
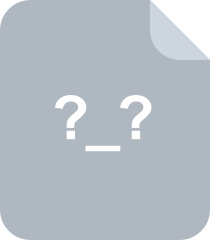
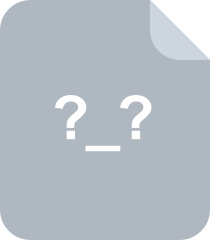
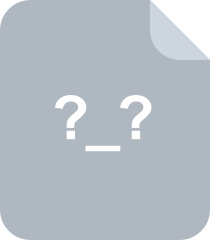
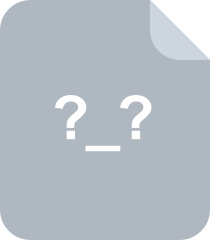
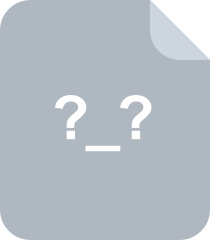
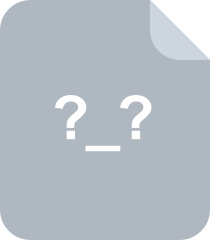
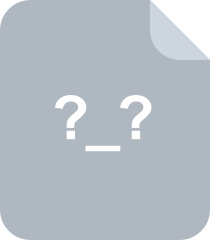
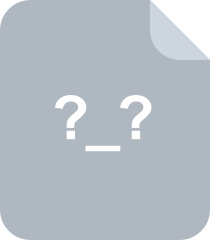
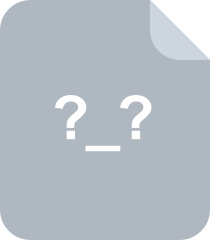
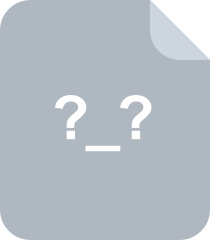
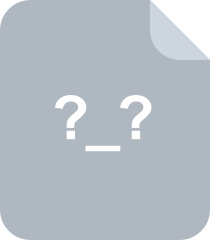
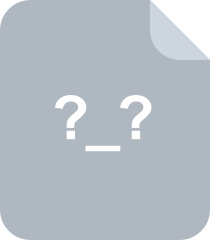
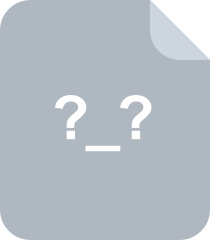
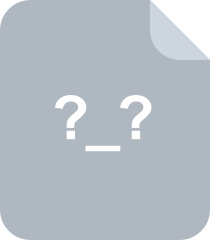
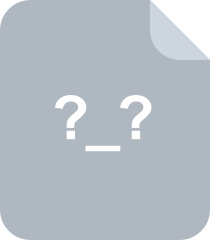
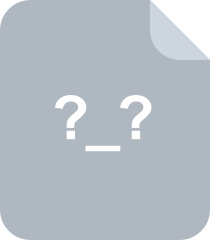
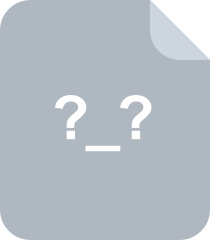
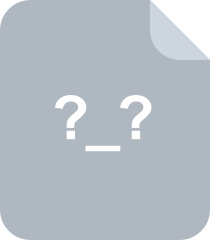
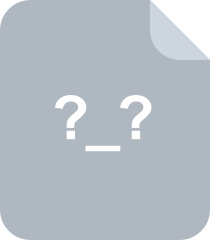
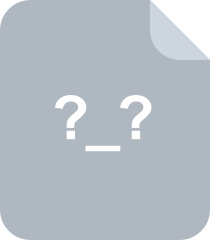
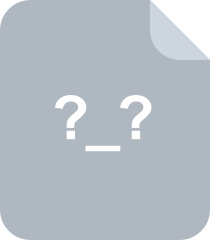
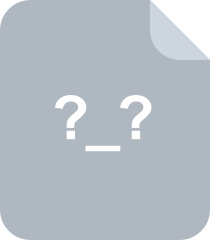
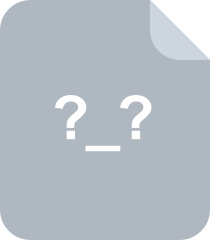
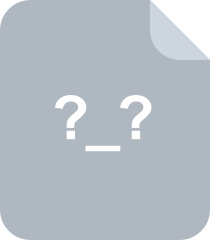
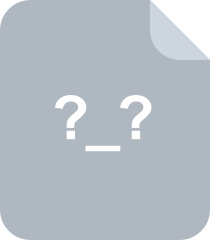
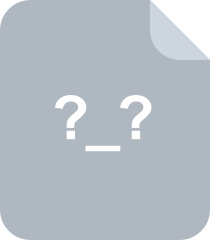
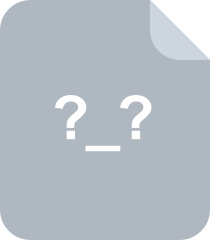
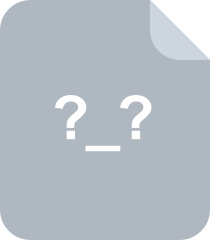
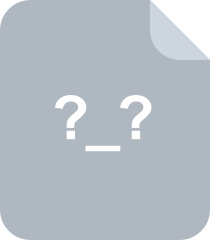
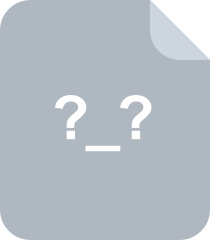
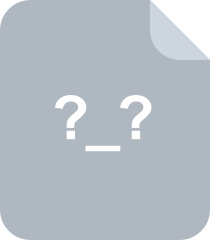
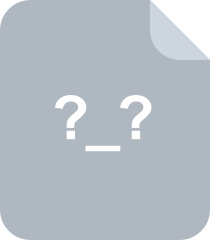
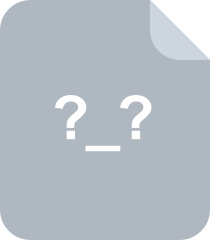
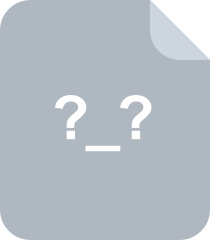
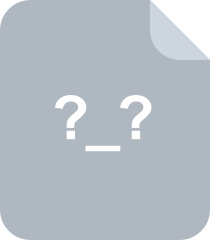
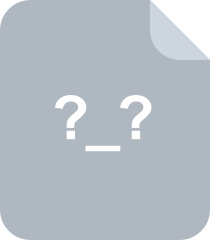
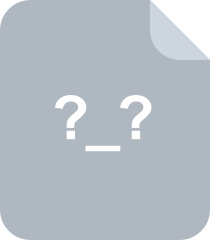
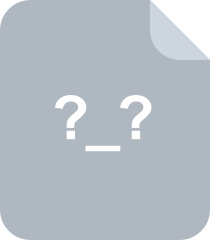
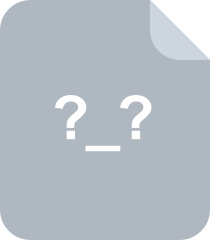
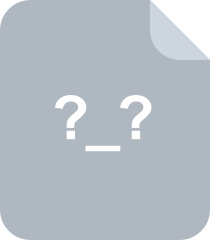
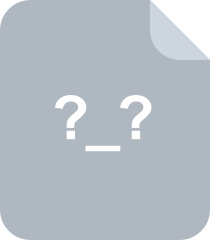
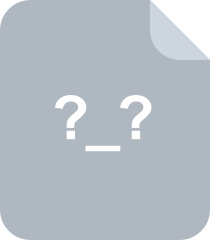
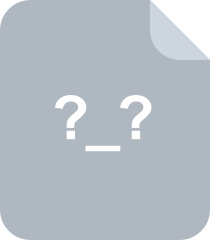
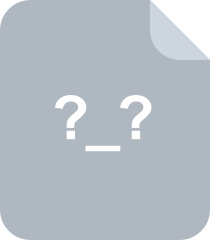
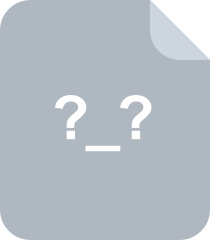
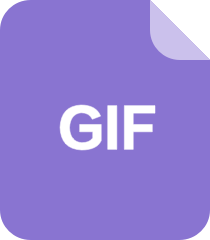
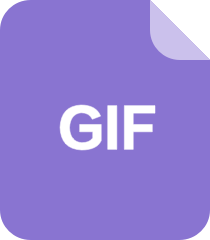
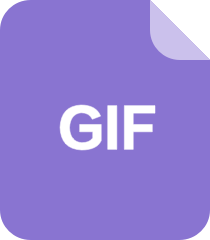
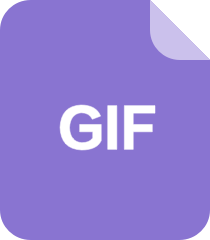
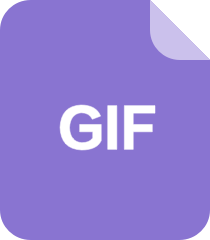
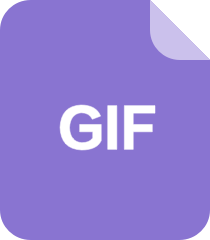
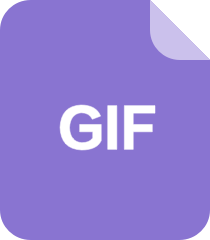
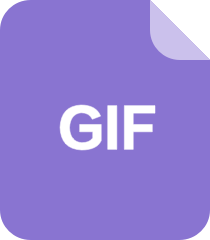
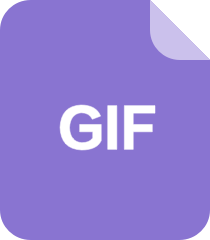
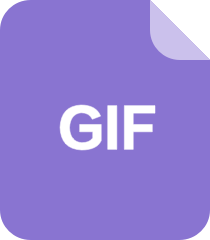
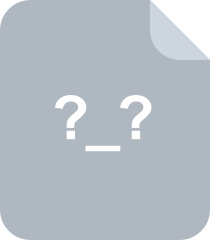
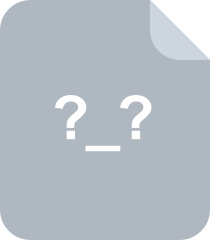
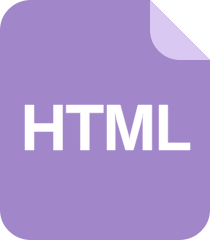
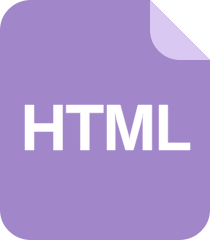
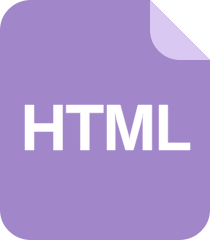
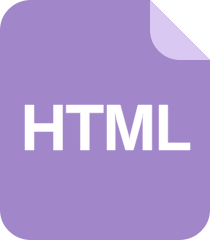
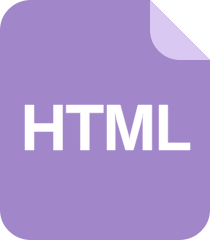
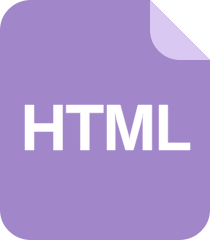
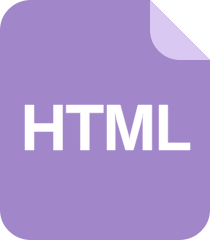
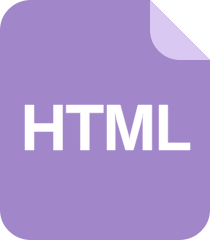
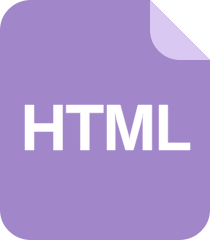
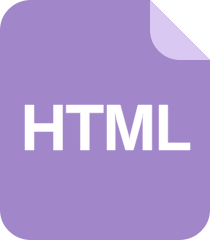
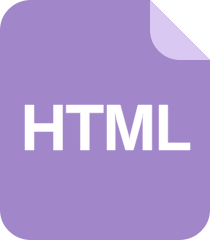
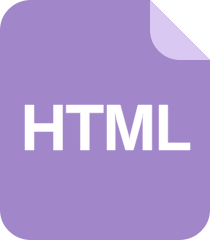
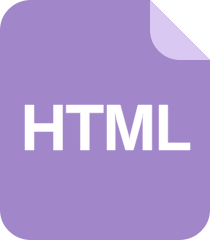
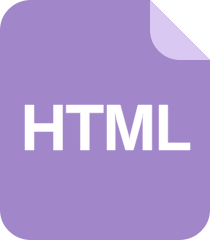
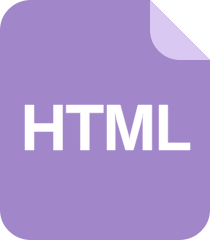
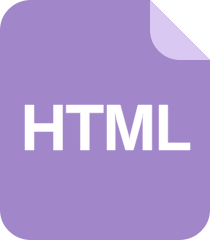
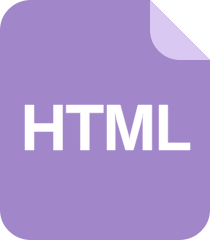
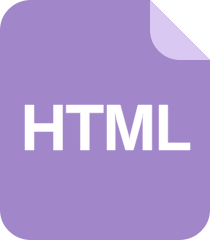
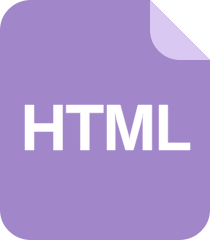
共 613 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
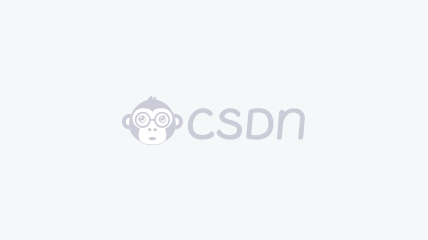


行动之上
- 粉丝: 2155
- 资源: 928
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

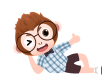
安全验证
文档复制为VIP权益,开通VIP直接复制
